Unit 1
PPS
C program basically consists of the following parts −
- Preprocessor Commands
- Functions
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" –
#include <stdio.h>
Int main() {
/* my first program in C */
Printf("Hello, World! \n");
Return 0;
}
The various parts of the above program −
- The first line of the program #include<stdio.h> is a preprocessor command, which tells a C compiler to include stdio.h file before going to actual compilation.
- The next line intmain() is the main function where the program execution begins.
- The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. Such lines are called comments in the program.
- The next line printf(...) is another function available in C which causes the message "Hello, World!" to be displayed on the screen.
- The next line return 0; terminates the main() function and returns the value 0.
A constant is a value or variable that can't be changed in the program, for example: 10, 20, 'a', 3.4, "c programming" etc.
There are different types of constants in C programming.
List of Constants in C
Constant | Example |
Decimal Constant | 10, 20, 450 etc. |
Real or Floating-point Constant | 10.3, 20.2, 450.6 etc. |
Octal Constant | 021, 033, 046 etc. |
Hexadecimal Constant | 0x2a, 0x7b, 0xaa etc. |
Character Constant | 'a', 'b', 'x' etc. |
String Constant | "c", "c program", "c in javatpoint" etc. |
2 ways to define constant in C
There are two ways to define constant
- Const keyword
- #define preprocessor
1) C const keyword
The const keyword is used to define constant in C programming.
- Const float PI=3.14;
Now, the value of PI variable can't be changed.
- #include<stdio.h>
- Int main(){
- Const float PI=3.14;
- Printf("The value of PI is: %f",PI);
- Return 0;
- }
Output:
The value of PI is: 3.140000
If you try to change the the value of PI, it will render compile time error.
- #include<stdio.h>
- Int main(){
- Const float PI=3.14;
- PI=4.5;
- Printf("The value of PI is: %f",PI);
- Return 0;
- }
Output:
Compile Time Error: Cannot modify a const object
Variables are the names you give to computer memory locations which are used to store values in a computer program.
Here are the following three simple steps −
- Create variables with appropriate names.
- Store your values in those two variables.
- Retrieve and use the stored values from the variables.
When creating a variable, we need to declare the data type it contains.
Programming languages define data types differently.
For example, almost all languages differentiate between ‘integers’ (or whole numbers, eg 12), ‘non-integers’ (numbers with decimals, eg 0.24), and ‘characters’ (letters of the alphabet or words).
- Char – a single 16-bit Unicode character, such as a letter, decimal or punctuation symbol.
- Boolean – can have only two possible values: true (1) or false (0). This data type is useful in conditional statements.
- Byte - has a minimum value of -128 and a maximum value of 127 (inclusive).
- Short– has a minimum value of -32,768 and a maximum value of 32,767
- Int: – has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive).
- Long – has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive).
- Float – a floating point number with 32-bits of precision
- Double – this is a double precision floating point number.
Data types:
Data Declarations
Declarations serve two purposes:
They tell the compiler to set aside an appropriate amount of space in memory for the program’s data (variables).
They enable the compiler to correctly operate on the variables. The compiler needs to know the data type to correctly operate on a variable. The compiler needs to know how many bytes are used by variables and the format of the bits. The meaning of the binary bits of a variable are different for different data types.
- Char is 8 bit (1 byte) ASCII, but can also store numeric data.
- Int is 4 byte 2’s complement.
- Short is 2 byte 2’s complement.
- Long is 8 byte 2’s complement.
- Unsigned (int, short, and long) are straight binary
- Float is 4 byte IEEE Floating Point Single Precision Standard.
- Double is 8 byte IEEE Floating Point Double Precision Standard.
Character - integer relation
The ASCII code is a set of integer numbers used to represent characters.
Char c = 'a'; /* 'a' has ASCII value 97 */
Inti = 65; /* 65 is ASCII for 'A' */
Printf( "%c", c + 1 ); /* b */
Printf( "%d", c + 2 ); /* 99 */
Printf( "%c", i + 3 ); /* D */
Integers
A variations of int (unsigned, long, ...) are stored binary data which is directly translated to it’s base-10 value.
Floating point data
Variables of type float and double are stored in three parts: the sign, the mantissa (normalized value), and an exponent.
Sizeof
Because some variables take different amount of memory of different systems, C provides an operator which returns the number of bytes needed to store a given type of data.
i = sizeof(char);
j = sizeof(long);
k = sizeof(double);
The sizeof operator is very useful when manually allocating memory and dealing with complex data structures.
Conversions and Casts
Two mechanisms exist to convert data from one data type to another, implicit conversion and explicit conversion, which is also called casting.
Implicit conversion
If an arithmetic operation on two variables of differing types is performed, one of the variables is converted or promoted to the same data as the other before the operation is performed. In general, smaller data types are always promoted to the larger data type.
Explicit conversion or casts
The programmer can tell the compiler what types of conversions should be performed by using a cast. A cast is formed by putting a data type keyword in parenthesis in front of a variable name or expression.
x = (float)(m * j);
i = (int)x + k;
Every expression in C language contains set of operators and operands. Operators are the special symbols which are used to indicate the type of operation whereas operands are the data member or variables operating as per operation specified by operator. One expression contains one or more set of operators and operands. So to avoid the ambiguity in execution of expression, C compiler fixed the precedence of operator. Depending on the operation, operators are classified into following category.
- Arithmetic Operator: These are the operators which are useful in performing mathematical calculations. Following are the list of arithmetic operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
+ | Used to add two operands | Result of A+B is 15 | |
2. | - | Used to subtract two operands | Result of A-B is 5 |
3. | * | Used to multiply two operands | Result of A*B is 50 |
4. | / | Used to divide two operands | Result of A/B is 2 |
5. | % | Find reminder of the division of two operand | Result of A%B is 0 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Inta,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of addition of %d and %d is %d",a,b,a+b);
Printf("\n Result of subtraction of %d and %d is %d",a,b,a-b);
Printf("\n Result of multiplication %d and %d is %d",a,b,a*b);
Printf("\n Result of division of %d and %d is %d",a,b,a/b);
Printf("\n Result of modulus of %d and %d is %d",a,b,a%b);
Getch();
}
Output:
Enter two numbers
5
3
Result of addition of 5 and 3 is 8
Result of subtraction of 5 and 3 is 2
Result of multiplication of 5 and 3 is 15
Result of division of 5 and 3 is 1
Result of modulus of 5 and 3 is 2
2. Relational Operators: Relational operator used to compare two operands. Relational operator produce result in terms of binary values. It returns 1 when the result is true and 0 when result is false. Following are the list of relational operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | < | This is less than operator which is used to check whether the value of left operand is less than the value of right operand or not | Result of A<B is false
|
2. | > | This is greater than operator which is used to check whether the value of left operand is greater than the value of right operand or not | Result of A>B is true |
3. | <= | This is less than or equal to operator | Result of A<=B is false |
4. | >= | This is greater than or equal to operator | Result of A>=B is true |
5. | == | This is equal to operator which is used to check value of both operands are equal or not | Result of A==B is false |
6. | != | This is not equal to operator which is used to check value of both operands are equal or not | Result of A!=B is true |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Inta,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of less than operator of %d and %d is %d",a,b,a<b);
Printf("\n Result of greater than operator of %d and %d is %d",a,b,a>b);
Printf("\n Result of leass than or equal to operator %d and %d is %d",a,b,a<=b);
Printf("\n Result of greater than or equal to operator of %d and %d is %d", a,b,a>=b);
Printf("\n Result of double equal to operator of %d and %d is %d",a,b,a==b);
Printf("\n Result of not equal to operator of %d and %d is %d",a,b,a!=b);
Getch();
}
Output:
Enter two numbers
5
3
Result of less than operator of 5 and 3 is 0
Result of greater than operator of 5 and 3 is 1
Result of less than or equal to operator of 5 and 3 is 0
Result of greater than or equal to operator of 5 and 3 is 1
Result of double equal to operator of 5 and 3 is 0
Result of not equal to operator of 5 and 3 is 1
Logical Operators
Following table shows all the logical operators supported by C language. Assume variable A holds 1 and variable B holds 0, then −
Operator | Description | Example |
&& | Called Logical AND operator. If both the operands are non-zero, then the condition becomes true. | (A && B) is false. |
|| | Called Logical OR Operator. If any of the two operands is non-zero, then the condition becomes true. | (A || B) is true. |
! | Called Logical NOT Operator. It is used to reverse the logical state of its operand. If a condition is true, then Logical NOT operator will make it false. | !(A && B) is true. |
Bitwise Operators
Bitwise operator works on bits and perform bit-by-bit operation. The truth tables for &, |, and ^ is as follows −
p | q | p & q | p | q | p ^ q |
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume A = 60 and B = 13 in binary format, they will be as follows −
A = 0011 1100
B = 0000 1101
-----------------
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~A = 1100 0011
The following table lists the bitwise operators supported by C. Assume variable 'A' holds 60 and variable 'B' holds 13, then −
Operator | Description | Example |
& | Binary AND Operator copies a bit to the result if it exists in both operands. | (A & B) = 12, i.e., 0000 1100 |
| | Binary OR Operator copies a bit if it exists in either operand. | (A | B) = 61, i.e., 0011 1101 |
^ | Binary XOR Operator copies the bit if it is set in one operand but not both. | (A ^ B) = 49, i.e., 0011 0001 |
~ | Binary One's Complement Operator is unary and has the effect of 'flipping' bits. | (~A ) = ~(60), i.e,. -0111101 |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | A << 2 = 240 i.e., 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | A >> 2 = 15 i.e., 0000 1111 |
Assignment Operators
The following table lists the assignment operators supported by the C language −
Operator | Description | Example |
= | Simple assignment operator. Assigns values from right side operands to left side operand | C = A + B will assign the value of A + B to C |
+= | Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator. It subtracts the right operand from the left operand and assigns the result to the left operand. | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator. It multiplies the right operand with the left operand and assigns the result to the left operand. | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator. It divides the left operand with the right operand and assigns the result to the left operand. | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator. It takes modulus using two operands and assigns the result to the left operand. | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator. | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator. | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator. | C &= 2 is same as C = C & 2 |
^= | Bitwise exclusive OR and assignment operator. | C ^= 2 is same as C = C ^ 2 |
|= | Bitwise inclusive OR and assignment operator. | C |= 2 is same as C = C | 2 |
Misc Operators ↦ sizeof& ternary
Besides the operators discussed above, there are a few other important operators including sizeofand ? : supported by the C Language.
Operator | Description | Example |
Sizeof() | Returns the size of a variable. | Sizeof(a), where a is integer, will return 4. |
& | Returns the address of a variable. | &a; returns the actual address of the variable. |
* | Pointer to a variable. | *a; |
? : | Conditional Expression. | If Condition is true ? then value X : otherwise value Y |
Operators Precedence in C
Operator precedence determines the grouping of terms in an expression and decides how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has a higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has a higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* &sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | <<>> | Left to right |
Relational | <<= >>= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
Expressions
An expression is a formula in which operands are linked to each other by the use of operators to compute a value. An operand can be a function reference, a variable, an array element or a constant.
Let's see an example:
- a-b;
In the above expression, minus character (-) is an operator, and a, and b are the two operands.
There are four types of expressions exist in C:
- Arithmetic expressions
- Relational expressions
- Logical expressions
- Conditional expressions
Each type of expression takes certain types of operands and uses a specific set of operators. Evaluation of a particular expression produces a specific value.
For example:
- x = 9/2 + a-b;
The entire above line is a statement, not an expression. The portion after the equal is an expression.
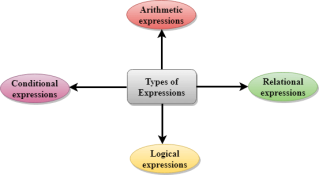
Arithmetic Expressions
An arithmetic expression is an expression that consists of operands and arithmetic operators. An arithmetic expression computes a value of type int, float or double.
When an expression contains only integral operands, then it is known as pure integer expression when it contains only real operands, it is known as pure real expression, and when it contains both integral and real operands, it is known as mixed mode expression.
Evaluation of Arithmetic Expressions
The expressions are evaluated by performing one operation at a time. The precedence and associativity of operators decide the order of the evaluation of individual operations.
When individual operations are performed, the following cases can be happened:
- When both the operands are of type integer, then arithmetic will be performed, and the result of the operation would be an integer value. For example, 3/2 will yield 1 not 1.5 as the fractional part is ignored.
- When both the operands are of type float, then arithmetic will be performed, and the result of the operation would be a real value. For example, 2.0/2.0 will yield 1.0, not 1.
- If one operand is of type integer and another operand is of type real, then the mixed arithmetic will be performed. In this case, the first operand is converted into a real operand, and then arithmetic is performed to produce the real value. For example, 6/2.0 will yield 3.0 as the first value of 6 is converted into 6.0 and then arithmetic is performed to produce 3.0.
Let's understand through an example.
6*2/ (2+1 * 2/3 + 6) + 8 * (8/4)
Evaluation of expression | Description of each operation |
6*2/( 2+1 * 2/3 +6) +8 * (8/4) | An expression is given. |
6*2/(2+2/3 + 6) + 8 * (8/4) | 2 is multiplied by 1, giving value 2. |
6*2/(2+0+6) + 8 * (8/4) | 2 is divided by 3, giving value 0. |
6*2/ 8+ 8 * (8/4) | 2 is added to 6, giving value 8. |
6*2/8 + 8 * 2 | 8 is divided by 4, giving value 2. |
12/8 +8 * 2 | 6 is multiplied by 2, giving value 12. |
1 + 8 * 2 | 12 is divided by 8, giving value 1. |
1 + 16 | 8 is multiplied by 2, giving value 16. |
17 | 1 is added to 16, giving value 17. |
Relational Expressions
- A relational expression is an expression used to compare two operands.
- It is a condition which is used to decide whether the action should be taken or not.
- In relational expressions, a numeric value cannot be compared with the string value.
- The result of the relational expression can be either zero or non-zero value. Here, the zero value is equivalent to a false and non-zero value is equivalent to true.
Relational Expression | Description |
x%2 = = 0 | This condition is used to check whether the x is an even number or not. The relational expression results in value 1 if x is an even number otherwise results in value 0. |
a!=b | It is used to check whether a is not equal to b. This relational expression results in 1 if a is not equal to b otherwise 0. |
a+b = = x+y | It is used to check whether the expression "a+b" is equal to the expression "x+y". |
a>=9 | It is used to check whether the value of a is greater than or equal to 9. |
Let's see a simple example:
- #include <stdio.h>
- Int main()
- {
- Int x=4;
- If(x%2==0)
- {
- Printf("The number x is even");
- }
- Else
- Printf("The number x is not even");
- Return 0;
- }
Output
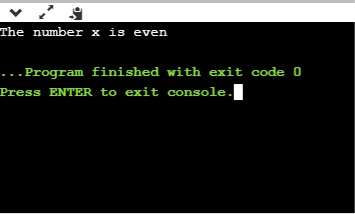
Logical Expressions
- A logical expression is an expression that computes either a zero or non-zero value.
- It is a complex test condition to take a decision.
Let's see some example of the logical expressions.
Logical Expressions | Description |
( x > 4 ) && ( x < 6 ) | It is a test condition to check whether the x is greater than 4 and x is less than 6. The result of the condition is true only when both the conditions are true. |
x > 10 || y <11 | It is a test condition used to check whether x is greater than 10 or y is less than 11. The result of the test condition is true if either of the conditions holds true value. |
! ( x > 10 ) && ( y = = 2 ) | It is a test condition used to check whether x is not greater than 10 and y is equal to 2. The result of the condition is true if both the conditions are true. |
Let's see a simple program of "&&" operator.
- #include <stdio.h>
- Int main()
- {
- Int x = 4;
- Int y = 10;
- If ( (x <10) && (y>5))
- {
- Printf("Condition is true");
- }
- Else
- Printf("Condition is false");
- Return 0;
- }
Output
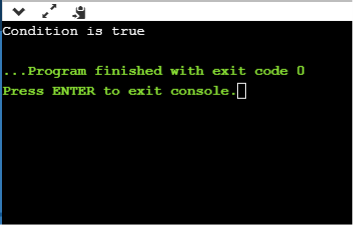
Let's see a simple example of "| |" operator
- #include <stdio.h>
- Int main()
- {
- Int x = 4;
- Int y = 9;
- If ( (x <6) || (y>10))
- {
- Printf("Condition is true");
- }
- Else
- Printf("Condition is false");
- Return 0;
- }
Output
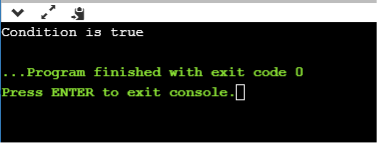
Conditional Expressions
- A conditional expression is an expression that returns 1 if the condition is true otherwise 0.
- A conditional operator is also known as a ternary operator.
The Syntax of Conditional operator
Suppose exp1, exp2 and exp3 are three expressions.
Exp1 ?exp2 : exp3
The above expression is a conditional expression which is evaluated on the basis of the value of the exp1 expression. If the condition of the expression exp1 holds true, then the final conditional expression is represented by exp2 otherwise represented by exp3.
Let's understand through a simple example.
- #include<stdio.h>
- #include<string.h>
- Int main()
- {
- Int age = 25;
- Char status;
- Status = (age>22) ? 'M': 'U';
- If(status == 'M')
- Printf("Married");
- Else
- Printf("Unmarried");
- Return 0;
- }
Output
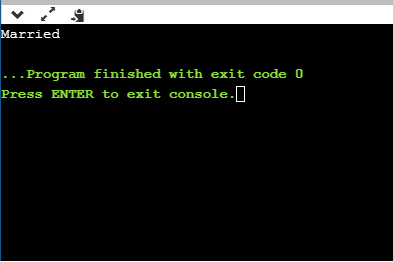
Arithmetic Expressions consist of numeric literals, arithmetic operators, and numeric variables. They simplify to a single value, when evaluated.
Here is an example of an arithmetic expression with no variables:
3.14*10*10
This expression evaluates to 314, the approximate area of a circle with radius 10. Similarly, the expression
3.14*radius*radius
Would also evaluate to 314, if the variable radius stored the value 10.
Note that the parentheses in the last expression helps dictate which order to evaluate the expression.
Multiplication and division have a higher order of precedence than addition and subtraction. In an arithmetic expression, it is evaluated left to right, only performing the multiplications and divisions.
After doing this, process the expression again from left to right, doing all the additions and subtractions.
So,
3+4*5
First evaluates to
3+20 which then evaluates to 23.
Consider this expression:
3 + 4*5 - 6/3*4/8 + 2*6 - 4*3*2
First go through and do all the multiplications and divisions:
3 + 20 - 1 + 12 - 24
Integer Division: In particular, if the division has a leftover remainder or fraction, this is simply discarded.
For example:
13/4 evaluates to 3
19/3 evaluates to 6 but
Similarly, if you have an expression with integer variables part of a division, this evaluates to an integer as well.
For example, in this segment of code, y gets set to 2.
Int x = 8;
Int y = x/3;
However, if we did the following,
Double x = 8;
Double y = x/3;
y would equal 2.66666666 (approximately).
The way C decides whether it will do an integer division (as in the first example), or a real number division (as in the second example), is based on the TYPE of the operands.
If both operands are ints, an integer division is done.
If either operand is a float or a double, then a real division is done. The compiler will treat constants without the decimal point as integers, and constants with the decimal point as a float. Thus, the expressions 13/4 and 13/4.0 will evaluate to 3 and 3.25 respectively.
The mod operator (%)
The one new operator is the mod operator, which is denoted by the percent sign(%). This operator is ONLY defined for integer operands. It is defined as follows:
a%b evaluates to the remainder of a divided by b.
For example,
12%5 = 2
19%6 = 1
14%7 = 0
19%200 = 19
The precedence of the mod operator is the same as the precedence of multiplication and division.
Implicit type conversion:
Also known as ‘automatic type conversion’.
- Done by the compiler on its own, without any external trigger from the user.
- Generally, takes place when in an expression more than one data type is present. In such condition type conversion (type promotion) takes place to avoidtoloose data.
- All the data types of the variables are upgraded to the data type of the variable with largest data type.
Bool -> char -> short int ->int ->
Unsignedint -> long -> unsigned ->
Longlong -> float -> double -> long double
It is possible for implicit conversions to lose information, signs can be lost (when signed is implicitly converted to unsigned), and overflow can occur (when long is implicitly converted to float).
Example of Type Implicit Conversion:
// An example of implicit conversion #include<stdio.h> Intmain() { Intx = 10; // integer x Chary = 'a'; // character c
// y implicitly converted to int. ASCII // value of 'a' is 97 x = x + y;
// x is implicitly converted to float Floatz = x + 1.0;
Printf("x = %d, z = %f", x, z); Return0; } |
|
Output:
x=107, z = 108.000000
Explicit Type Conversion–
This process is also called type casting and it is user defined. Here the user can type cast the result to make it of a particular data type.
The syntax in C:
(type) expression
Type indicated the data type to which the result is converted.
// C program to demonstrate explicit type casting #include<stdio.h>
Intmain() { Doublex = 1.2;
// Explicit conversion from double to int Intsum = (int)x + 1;
Printf("sum = %d", sum);
Return0; }
Output:
Sum =2
Advantages of type conversion:
This is done to take advantage of certain features of type hierarchies or type representations.
It helps us to compute the expression containing variables of different data types.
|
#include<stdio.h>
Void main()
{
Inta,b,c;
Printf("Please enter any two numbers: \n");
Scanf("%d %d", &a, &b);
c = a + b;
Printf("The addition of two number is: %d", c);
}
Please enter any two numbers:
12
3
The addition of two number is:15
Managing Input/Output
I/O operations are useful for program to interact with users .stdlib is the standard library for input-output operations. In C two important streams play their role:
These are:
- Standard Input
- Standard Output
Standard input or stdin is used for taking input from devices such as keyboard as a data stream. Standard output or stdout is used for giving output to a device such as a monitor. For using I/O functionality programmers must include stdio header-file within the program.
Reading Character in C
The easiest and simplest I/O operations are taking character as input by reading that character from standard input .getchar() function can be used to read a single character. This function is alternate to scanf() function.
Syntax:
Var_name = getchar();
Example:
#include<stdio.h>
Void main()
{
Char title;
Title= getchar();
}
Writing Character in C
Syntax:
Putchar(var_name);
Example:
#include<stdio.h>
Void main()
{
Char result = ‘P’
Putchar(result);
Putchar(‘\n’);
}
C language provides us console input/output functions. As the name says, the console input/output functions allow us to -
- Read the input from the keyboard by the user accessing the console.
- Display the output to the user at the console.
Note: These input and output values could be of any primitive data type.
There are two kinds of console input/output functions -
- Formatted input/output functions.
- Unformatted input/output functions.
Formatted input/output functions
Formatted console input/output functions are used to take one or more inputs from the user at console and it also allows us to display one or multiple values in the output to the user at the console.
Some of the most important formatted console input/output functions are -
Functions | Description |
Scanf() |
|
Printf() |
|
Sscanf() |
|
Sprintf() |
|
While calling any of the formatted console input/output functions, we must use a specificformat specifiers in them, which allow us to read or display any value of a specific primitive data type. Let's see what are these format specifiers.
Format specifiers in console formatted I/O functions
Some of the most commonly used format specifiers used in console formatted input/output functions are displayed in the table below -
Format Specifiers | Description |
%hi |
|
%hu |
|
%d |
|
%u |
|
%ld |
|
%lu |
|
%c |
|
%c |
|
%f |
|
%lf |
|
%Lf |
|
%s |
|
Optional specifiers within a format specifier
We could specify two more optional specifiers within each format specifier, such as integer value and a sign.
An integer value specifies the number of columns used on the screen for printing a value i.e. width. This integer value may or may not have a minus sign before it.
- A (-)minussign before the integer value means left justification of the value to be printed on the screen and integer value following the minus sign is the number of blanks on its right.
- No minus sign before the integer value means right justification of the value to be printed on the screen and integer value specifies the number of blanks on its left.
Introduction to DCS :
A Control Statement is a statement that determine the control flow of a set of instructions.
A Control statement can either comprise of one or more instructions.
The three fundamentals methods of control flow a programming language are sequential, selection and iterative control.
Decision Control Statements
Sequential control Selection control Iterative control
Statements StatementsStatements
stat 1 stat 1 stat 1
Stat 2 stat 2 stat 2
………. ……….. ……….
………. test_conditiontest_condition
Stat n ……….
True False Loop ………. True
……. …….. ………
……. …….. False
This statement permits the programmer to allocate condition on the execution of a statement. If the evaluated condition found to be true, the single statement following the "if" is execute. If the condition is found to be false, the following statement is skipped. Syntax of the if statement is as follows
if (condition)
statement1;
statement2;
In the above syntax, “if” is the keyword and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and then statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
Syntax of if Statement
If test_expression :
Stat 1 Test Exp False
stat n
stat x True
Eg. x = 10 stat Block 1
If ( x> 0) :
x = x + stat x
Print ( x)
O / P : x = 11
Eg: age =int (input ( “Enter the age : “ ))
If (age > = 18 ) :
Print (“You are eligible to vote )
O/ P : Enter the age : 35
You are eligible to vote
Eg :char = input (“Press any key : “)
If (char .isalpha());
Print(“The user has entered a character”)
If(char.isdigit());
Print(“The user has entered a digit “)
If(char.isspace());
Print(“The user entered a write space characters”)
O/P : Press any key : 7
The user has entered a digit.
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
- If(expression){
- //code to be executed
- }
Flowchart of if statement in C
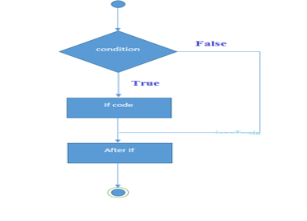
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 s largest
If-----else statement
This statement permits the programmer to execute a statement out of the two statements. If the evaluated condition is found to be true, the single statement following the "if" is executed and statement following else is skipped. If the condition is found to be false, statement following the "if" is skipped and statement following else is executed.
In this statement “if” part is compulsory whereas “else” is the optional part. For every “if” statement there may be or may not be “else” statement but for every “else” statement there must be “if” part otherwise compiler will gives “Misplaced else” error.
Syntax of the “if----else” statement is as follows
If (condition)
Statement1;
Else
Statement2;
In the above syntax, “if” and “else” are the keywords and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and skip statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
if –else statement
Syntax of if-else stat
If(test exp): True Test False
Stat block 1 Exp
else :
Stat block 2
stat x

Eg: age =int(input(“Enter the age :”))
If(age >=18)
Print(“You are eligible to vote”)
Else
Yrs =18 – age
Print(“You have to wait for another “ + str/yrs “years to cast your vote”)
O/P : Enter the age = 10
You have to wait for another 8 years to cast your vote
Eg. a =int (input(“Enter the value of a:”))
b=int (input(“Enter the value of b:”))
If(a<b):
Large =a
Else:
Nested if-----else statements :
Nested “if-----else” statements are used when programmer wants to check multiple conditions. Nested “if---else” contains several “if---else” a statement out of which only one statement is executed. Number of “if----else” statements is equal to the number of conditions to be checked. Following is the syntax for nested “if---else” statements for three conditions
If (condition1)
Statement1;
Else if (condition2)
Statement2;
Else if (condition3)
Statement3;
Else
Statement4;
In the above syntax, compiler first check condition1, if it trues then it will execute statement1 and skip all the remaining statements. If condition1 is false then compiler directly checks condition2, if it is true then compiler execute statement2 and skip all the remaining statements. If condition2 is also false then compiler directly checks condition3, if it is true then compiler execute statement3 otherwise it will execute statement 4.
Note:
If the test expression is evaluated to true,
• Statements inside the body of if are executed.
• Statements inside the body of else are skipped from execution.
If the test expression is evaluated to false,
• Statements inside the body of else are executed
• Statements inside the body of if are skipped from execution.
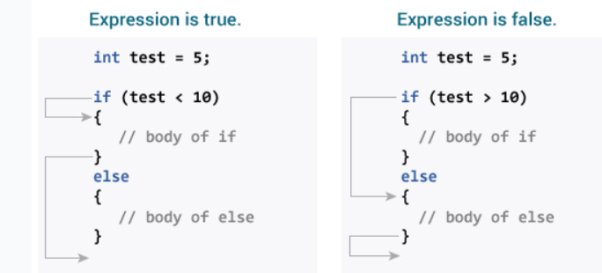
// Check whether an integer is odd or even
#include <stdio.h>
Int main() {
Int number;
Printf("Enter an integer: ");
Scanf("%d", &number);
// True if the remainder is 0
If (number%2 == 0) {
Printf("%d is an even integer.",number);
}
Else {
Printf("%d is an odd integer.",number);
}
Return 0;
}
Program to relate two integers using =, > or < symbol
#include <stdio.h>
Int main() {
Int number1, number2;
Printf("Enter two integers: ");
Scanf("%d %d", &number1, &number2);
//checks if the two integers are equal.
If(number1 == number2) {
Printf("Result: %d = %d",number1,number2);
}
//checks if number1 is greater than number2.
Else if (number1 > number2) {
Printf("Result: %d > %d", number1, number2);
}
//checks if both test expressions are false
Else {
Printf("Result: %d < %d",number1, number2);
}
Return 0;
}
If else-if ladder Statement
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
- If(condition1){
- //code to be executed if condition1 is true
- }else if(condition2){
- //code to be executed if condition2 is true
- }
- Else if(condition3){
- //code to be executed if condition3 is true
- }
- ...
- Else{
- //code to be executed if all the conditions are false
- }
Flowchart of else-if ladder statement in C
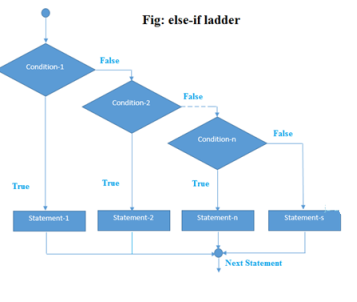
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats !you scored grade A ...
This statement permits the programmer to choose one option out of several options depending on one condition. When the “switch” statement is executed, the expression in the switch statement is evaluated and the control is transferred directly to the group of statements whose “case” label value matches with the value of the expression. Syntax for switch statement is as follows:
Switch(expression)
{
Case constant1:
Statements 1;
Break;
Case constant2:
Statements 2;
Break;
…………………..
Default:
Statements n;
Break;
}
In the above, “switch”, “case”, “break” and “default” are keywords. Out of which “switch” and “case” are the compulsory keywords whereas “break” and “default” is optional keywords.
- “switch” keyword is used to start switch statement with conditional expression.
- “case” is the compulsory keyword which labeled with a constant value. If the value of expression matches with the case value then statement followed by “case” statement is executed.
- “break” is the optional keyword in switch statement. The execution of “break” statement causes the transfer of flow of execution outside the “switch” statements scope. Absence of “break” statement causes the execution of all the following “case” statements without concerning value of the expression.
- “default” is the optional keyword in “switch” statement. When the value of expression is not match with the any of the “case” statement then the statement following “default” keyword is executed.
Example: Program to spell user entered single digit number in English is as follows
#include<stdio.h>
#include<conio.h>
Void main()
{
Int n;
Clrscr();
Printf("Enter a number");
Scanf("%d",&n);
Switch(n)
{
Case 0: printf("\n Zero");
Break;
Case 1: printf("\n One");
Break;
Case 2: printf("\n Two");
Break;
Case 3: printf("\n Three");
Break;
Case 4: printf("\n Four");
Break;
Case 5: printf("\n Five");
Break;
Case 6: printf("\n Six");
Break;
Case 7: printf("\n Seven");
Break;
Case 8: printf("\n Eight");
Break;
Case 9: printf("\n Nine");
Break;
Default: printf("Given number is not single digit number");
}
Getch();
}
Output
Enter a number
5
Five
Conditional Operator in C
The conditional operator is also known as a ternary operator. The conditional statements are the decision-making statements which depends upon the output of the expression. It is represented by two symbols, i.e., '?' and ':'.
As conditional operator works on three operands, so it is also known as the ternary operator.
The behavior of the conditional operator is similar to the 'if-else' statement as 'if-else' statement is also a decision-making statement.
Syntax of a conditional operator
- Expression1? expression2: expression3;
The pictorial representation of the above syntax is shown below:
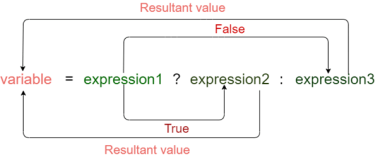
Meaning of the above syntax.
- In the above syntax, the expression1 is a Boolean condition that can be either true or false value.
- If the expression1 results into a true value, then the expression2 will execute.
- The expression2 is said to be true only when it returns a non-zero value.
- If the expression1 returns false value then the expression3 will execute.
- The expression3 is said to be false only when it returns zero value.
Let's understand the ternary or conditional operator through an example.
- #include <stdio.h>
- Int main()
- {
- Int age; // variable declaration
- Printf("Enter your age");
- Scanf("%d",&age); // taking user input for age variable
- (age>=18)? (printf("eligible for voting")) : (printf("not eligible for voting")); // conditional operator
- Return 0;
- }
In the above code, we are taking input as the 'age' of the user. After taking input, we have applied the condition by using a conditional operator. In this condition, we are checking the age of the user. If the age of the user is greater than or equal to 18, then the statement1 will execute, i.e., (printf("eligible for voting")) otherwise, statement2 will execute, i.e., (printf("not eligible for voting")).
Let's observe the output of the above program.
If we provide the age of user below 18, then the output would be:
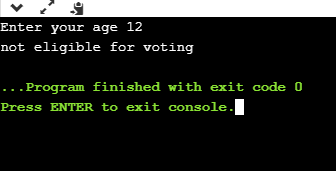
If we provide the age of user above 18, then the output would be:
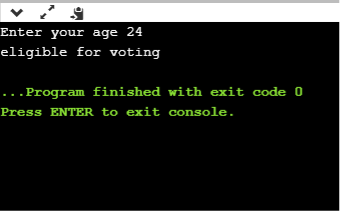
As we can observe from the above two outputs that if the condition is true, then the statement1 is executed; otherwise, statement2 will be executed.
Till now, we have observed that how conditional operator checks the condition and based on condition, it executes the statements. Now, we will see how a conditional operator is used to assign the value to a variable.
Let's understand this scenario through an example.
- #include <stdio.h>
- Int main()
- {
- Int a=5,b; // variable declaration
- b=((a==5)?(3):(2)); // conditional operator
- Printf("The value of 'b' variable is : %d",b);
- Return 0;
- }
In the above code, we have declared two variables, i.e., 'a' and 'b', and assign 5 value to the 'a' variable. After the declaration, we are assigning value to the 'b' variable by using the conditional operator. If the value of 'a' is equal to 5 then 'b' is assigned with a 3 value otherwise 2.
Output
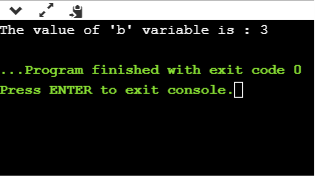
The above output shows that the value of 'b' variable is 3 because the value of 'a' variable is equal to 5.
As we know that the behavior of conditional operator and 'if-else' is similar but they have some differences. Let's look at their differences.
- A conditional operator is a single programming statement, while the 'if-else' statement is a programming block in which statements come under the parenthesis.
- A conditional operator can also be used for assigning a value to the variable, whereas the 'if-else' statement cannot be used for the assignment purpose.
- It is not useful for executing the statements when the statements are multiple, whereas the 'if-else' statement proves more suitable when executing multiple statements.
- The nested ternary operator is more complex and cannot be easily debugged, while the nested 'if-else' statement is easy to read and maintain.
Goto statement: “goto” statement is used to transfer the flow of program to any part of the program. The syntax of “go to” statement is as follows
goto label name;
In the above syntax “goto” is keyword which is used to transfer the flow of execution to the label specified after it. Label is an identifier that is used to mark the target statement to which the control is transferred. The target statement must be labeled and the syntax is as follows
Label name statement;
In the above syntax label name can be anything but it should be same as that of name of the label specified in “goto” statement, a colon must follow the label. Each labeled statement within the function must have a unique label, i.e., no two statements can have the same label.
The goto statement is known as jump statement in C. As the name suggests, goto is used to transfer the program control to a predefined label. The gotostatment can be used to repeat some part of the code for a particular condition. It can also be used to break the multiple loops which can't be done by using a single break statement. However, using goto is avoided these days since it makes the program less readable and complecated.
Syntax:
- Label:
- //some part of the code;
- Goto label;
Goto example
Let's see a simple example to use goto statement in C language.
- #include <stdio.h>
- Int main()
- {
- Int num,i=1;
- Printf("Enter the number whose table you want to print?");
- Scanf("%d",&num);
- Table:
- Printf("%d x %d = %d\n",num,i,num*i);
- i++;
- If(i<=10)
- Goto table;
- }
Output:
Enter the number whose table you want to print?10
10 x 1 = 10
10 x 2 = 20
10 x 3 = 30
10 x 4 = 40
10 x 5 = 50
10 x 6 = 60
10 x 7 = 70
10 x 8 = 80
10 x 9 = 90
10 x 10 = 100
When should we use goto?
The only condition in which using goto is preferable is when we need to break the multiple loops using a single statement at the same time. Consider the following example.
- #include <stdio.h>
- Int main()
- {
- Int i, j, k;
- For(i=0;i<10;i++)
- {
- For(j=0;j<5;j++)
- {
- For(k=0;k<3;k++)
- {
- Printf("%d %d %d\n",i,j,k);
- If(j == 3)
- {
- Goto out;
- }
- }
- }
- }
- Out:
- Printf("came out of the loop");
- }
0 0 0
0 0 1
0 0 2
0 1 0
0 1 1
0 1 2
0 2 0
0 2 1
0 2 2
0 3 0
Came out of the loop
Whilestatement
The while loop in C is the most fundamental loop statement. It repeats statement or block while its controlling expression is true.
The general form is:
While(condition) {
// body of loop
}
The condition can be any boolean expression. The body of the loop will be executed as long as the conditional expression is true.
Here is more practical example.
//example program to illustrate while looping
#include <stdio.h>
Int main ()
{
Int n = 10;
While (n > 0)
{
Printf("tick %d", n);
Printf("\n");
n--;
}
}
The output of the program is:
Tick 10
Tick 9
Tick 8
Tick 7
Tick 6
Tick 5
Tick 4
Tick 3
Tick 2
Tick 1
While Loop
The while loop provides a mechanism to repeat one or more statements while a particularcondition is True .
Syntax :
statement x stat x
while(condition):
stat block update the
statement y condition exp condition
True
stat block False
Stat y

Print(c,end = )
i = i + 1
O/P : 0 1 2 3 4 5 6 7 8 9 10
Eg. m=int(input(“Enter the value of m : “))
n =int(input(“Enter the value of n : “))
s = 0
While(m< = n)
s = s + m
m = n + 1
Print(“Sum =”,s)
O/P : Enter the value of m : 3
Enter the value of n : 9
Sum = 42
The do – while loop
The do – while loop consist of consist of condition mentioned at the bottom with while. The do – while executes till condition is true. As the condition is mentioned at bottom do – while loop is executed at least once.
Structure of the do – while:
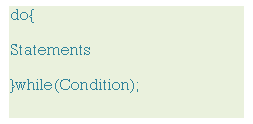
e.g.
Output is: 1 2 3 4 5 6 7 8 9 10.
The range () function
Therange() function is a built in function in Python that is used to iterate over a sequence of numbers. The Syntax of range() is
Range( beg, end,[step])
- The range() produces a sequence of numbers statement with beg(inclusive) and ending with one less than the number end.The step argument is optional.
- By default every number in the range is increment by 1.but we can specify a different using step.
- It can be both –ve& +ve , but not zero.
Eg. for i in range(1,5): for i in range(1,10,2):
Print(i,,end =” “) print(i,,end =” “)
O/P : 1 2 3 4 O/P 1 2 5 7 9
Key Points to remembers
- If range function is given a single argument , it produce an object with values from 0 to argument -1
For example:range (10) is equal to writing range (o, 10)
- If range () is called with 2 arguments, it produces values from the fist to second.
For example: range(0,10)
- If range() has interval of the sequence produced .In this case the 3rd argument must be an integer
For example:range (1, 20, 3)
For i in range(10): for i in range(1,15)
Print (i, end = ‘ ‘) print (i, end= ‘ ‘)
O/P 0 1 2 3 4 5 6 7 8 9 O/P : 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
For i in range(1,20,3):
Print(I,code=’ ‘)
O/P : 1 4 7 10 13 16 19
The for loop executes repeatedly until the condition specified in for loop is satisfied. When the condition becomes false it resumes with statement written in for loop.
Structure of the for loop:
Initialization is the assignment to the variable which controls the loop. Condition is an expression, specifies the execution of loop. Increment/ decrement operator changes the variable as the loop executes.
e.g.
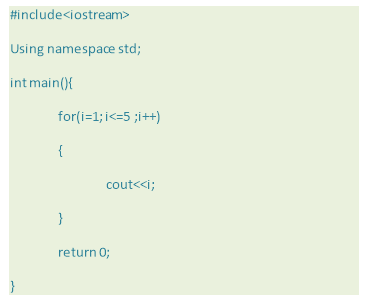
Output is 1 2 3 4 5.
Jump statements are used to interrupt the normal flow of program.
Types of Jump Statements
- Break
- Continue
- GoTo
Break Statement
The break statement is used inside loop or switch statement. When compiler finds the break statement inside a loop, compiler will abort the loop and continue to execute statements followed by loop.
Example of break statement
#include<stdio.h>
Void main()
{
Int a=1;
While(a<=10)
{
If(a==5)
Break;
Printf("\nStatement %d.",a);
a++;
}
Printf("\nEnd of Program.");
}
Output :
Statement 1.
Statement 2.
Statement 3.
Statement 4.
End of Program.
Continue Statement
The continue statement is also used inside loop. When compiler finds the break statement inside a loop, compiler will skip all the followling statements in the loop and resume the loop.
Example of continue statement
#include<stdio.h>
Void main()
{
Int a=0;
While(a<10)
{
a++;
If(a==5)
Continue;
Printf("\nStatement %d.",a);
}
Printf("\nEnd of Program.");
}
Output :
Statement 1.
Statement 2.
Statemnet 3.
Statement 4.
Statement 6.
Statement 7.
Statement 8.
Statement 9.
Statement 10.
End of Program.
Goto Statement
The goto statement is a jump statement which jumps from one point to another point within a function.
Syntax of goto statement
Goto label;
- - - - - - - - - -
- - - - - - - - - -
Label:
- - - - - - - - - -
- - - - - - - - - -
In the above syntax, label is an identifier. When, the control of program reaches to goto statement, the control of the program will jump to the label: and executes the code after it.
Example of goto statement
#include<stdio.h>
Void main()
{
Printf("\nStatement 1.");
Printf("\nStatement 2.");
Printf("\nStatement 3.");
Goto last;
Printf("\nStatement 4.");
Printf("\nStatement 5.");
Last:
Printf("\nEnd of Program.");
}
Output :
Statement 1.
Statement 2.
Statement 3.
End of Program.
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 is largest
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Else{
- Printf("%d is odd number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
- #include <stdio.h>
- Int main()
- {
- Int age;
- Printf("Enter your age?");
- Scanf("%d",&age);
- If(age>=18)
- {
- Printf("You are eligible to vote...");
- }
- Else
- {
- Printf("Sorry ... you can't vote");
- }
- }
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... You can't vote
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats !you scored grade A ...
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
- #include <stdio.h>
- Int main () {
- Int i = 10;
- Int j = 20;
- Switch(i) {
- Case 10:
- Printf("the value of i evaluated in outer switch: %d\n",i);
- Case 20:
- Switch(j) {
- Case 20:
- Printf("The value of j evaluated in nested switch: %d\n",j);
- }
- }
- Printf("Exact value of i is : %d\n", i );
- Printf("Exact value of j is : %d\n", j );
- Return 0;
- }
Output
The value of i evaluated in outer switch: 10
The value of j evaluated in nested switch: 20
Exact value of iis : 10
Exact value of j is : 20
do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- While(i<=10){
- Printf("%d \n",i);
- i++;
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
- #include<stdio.h>
- Int main(){
- Int i=1,number=0,b=9;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- While(i<=10){
- Printf("%d \n",(number*i));
- i++;
- }
- Return 0;
- }
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
C break statement with the nested loop
In such case, it breaks only the inner loop, but not outer loop.
- #include<stdio.h>
- Int main(){
- Int i=1,j=1;//initializing a local variable
- For(i=1;i<=3;i++){
- For(j=1;j<=3;j++){
- Printf("%d &d\n",i,j);
- If(i==2 && j==2){
- Break;//will break loop of j only
- }
- }//end of for loop
- Return 0;
- }
Output
1 1
1 2
1 3
2 1
2 2
3 1
3 2
3 3
As you can see the output on the console, 2 3 is not printed because there is a break statement after printing i==2 and j==2. But 3 1, 3 2 and 3 3 are printed because the break statement is used to break the inner loop only.
Break statement with while loop
Consider the following example to use break statement inside while loop.
- #include<stdio.h>
- Void main ()
- {
- Int i = 0;
- While(1)
- {
- Printf("%d ",i);
- i++;
- If(i == 10)
- Break;
- }
- Printf("came out of while loop");
- }
Output
0 1 2 3 4 5 6 7 8 9 came out of while loop
Break statement with do-while loop
Consider the following example to use the break statement with a do-while loop.
- #include<stdio.h>
- Void main ()
- {
- Int n=2,i,choice;
- Do
- {
- i=1;
- While(i<=10)
- {
- Printf("%d X %d = %d\n",n,i,n*i);
- i++;
- }
- Printf("do you want to continue with the table of %d , enter any non-zero value to continue.",n+1);
- Scanf("%d",&choice);
- If(choice == 0)
- {
- Break;
- }
- n++;
- }while(1);
- }
Output
2 X 1 = 2
2 X 2 = 4
2 X 3 = 6
2 X 4 = 8
2 X 5 = 10
2 X 6 = 12
2 X 7 = 14
2 X 8 = 16
2 X 9 = 18
2 X 10 = 20
Do you want to continue with the table of 3 , enter any non-zero value to continue.1
3 X 1 = 3
3 X 2 = 6
3 X 3 = 9
3 X 4 = 12
3 X 5 = 15
3 X 6 = 18
3 X 7 = 21
3 X 8 = 24
3 X 9 = 27
3 X 10 = 30
Do you want to continue with the table of 4 , enter any non-zero value to continue.0
C continue statement with inner loop
In such case, C continue statement continues only inner loop, but not outer loop.
- #include<stdio.h>
- Int main(){
- Int i=1,j=1;//initializing a local variable
- For(i=1;i<=3;i++){
- For(j=1;j<=3;j++){
- If(i==2 && j==2){
- Continue;//will continue loop of j only
- }
- Printf("%d %d\n",i,j);
- }
- }//end of for loop
- Return 0;
- }
Output
1 1
1 2
1 3
2 1
2 3
3 1
3 2
3 3
As you can see, 2 2 is not printed on the console because inner loop is continued at i==2 and j==2.
Let's see a simple example to use goto statement in C language.
- #include <stdio.h>
- Int main()
- {
- Int num,i=1;
- Printf("Enter the number whose table you want to print?");
- Scanf("%d",&num);
- Table:
- Printf("%d x %d = %d\n",num,i,num*i);
- i++;
- If(i<=10)
- Goto table;
- }
Output:
Enter the number whose table you want to print?10
10 x 1 = 10
10 x 2 = 20
10 x 3 = 30
10 x 4 = 40
10 x 5 = 50
10 x 6 = 60
10 x 7 = 70
10 x 8 = 80
10 x 9 = 90
10 x 10 = 100
TEXT BOOKS:
C & Data Structures (A practical approach) – by G.S. Baluja and G.K. Baluja, Dhanapatrai & Co publishers.