Unit 4
PPS
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
- Why Use Structures
• Ordinary variables can hold one piece of information
• arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure
Calledemployee with three elements id, name and salary. The syntax of this structure is as
Follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
Struct keyword is used to declare structure.
Members of structure are enclosed within opening and closing braces.
Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
Declaration of Structure reserves no space.
It is nothing but the “ Template / Map / Shape ” of the structure .
Memory is created , very first time when the variable is created / Instance is created.
- Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1 ,e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
- Memory allocation for structure
Memory is allocated to the structure only when we create the variable of structure.
Consider following example
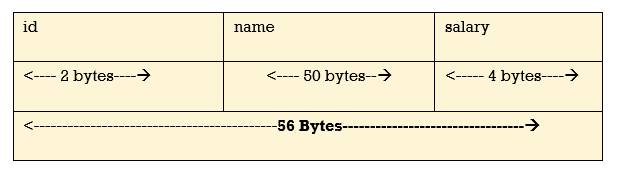
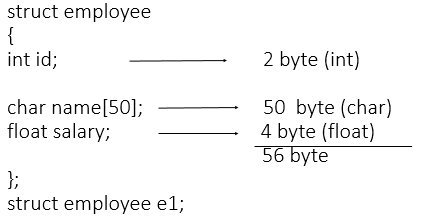
- Structure Initialization
1. When we declare a structure, memory is not allocated for un-initialized variable.
2. Let us discuss very familiar example of structure student , we can initialize structure variable
In different ways –
Way 1 : Declare and Initialize
Structstudent
{
Charname[20];
Introll;
Floatmarks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2 : Declaring and Initializing Multiple Variables
Structstudent
{
Charname[20];
Introll;
Floatmarks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3 : Initializing Single member
Structstudent
{
Intmark1;
Intmark2;
Intmark3;
} sub1={67};
Though there are three members of structure,only one is initialized , Then remaining two members
Are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4 : Initializing inside main
Structstudent
{
Intmark1;
Intmark2;
Intmark3;
};
Voidmain()
{
Structstudent s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below
Declaration statement will never allocate memory
Structstudent
{
Intmark1;
Intmark2;
Intmark3;
};
We need to initialize structure variable to allocate some memory to the structure.
Structstudent s1 = {89,54,65};
We can declare a variable for the structure so that we can access the member of the structure easily. There are two ways to declare structure variable:
- By struct keyword within main() function
- By declaring a variable at the time of defining the structure.
1st way:
Let's see the example to declare the structure variable by struct keyword. It should be declared within the main function.
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- };
Now write given code inside the main() function.
- Struct employee e1, e2;
The variables e1 and e2 can be used to access the values stored in the structure. Here, e1 and e2 can be treated in the same way as the objects in C++ and Java.
2nd way:
Let's see another way to declare variable at the time of defining the structure.
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- }e1,e2;
Which approach is good
If number of variables are not fixed, use the 1st approach. It provides you the flexibility to declare the structure variable many times.
If no. Of variables are fixed, use 2nd approach. It saves your code to declare a variable in main() function.
Accessing members of the structure
There are two ways to access structure members:
- By . (member or dot operator)
- By -> (structure pointer operator)
Let's see the code to access the id member of p1 variable by. (member) operator.
- p1.id
C Structure example
Let's see a simple example of structure in C language.
- #include<stdio.h>
- #include <string.h>
- Struct employee
- { int id;
- Char name[50];
- }e1; //declaring e1 variable for structure
- Int main( )
- {
- //store first employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- //printing first employee information
- Printf( "employee 1 id : %d\n", e1.id);
- Printf( "employee 1 name : %s\n", e1.name);
- Return 0;
- }
Output:
Employee 1 id : 101
Employee 1 name : Sonoo Jaiswal
Let's see another example of the structure in C language to store many employees information.
- #include<stdio.h>
- #include <string.h>
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- }e1,e2; //declaring e1 and e2 variables for structure
- Int main( )
- {
- //store first employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- e1.salary=56000;
- //store second employee information
- e2.id=102;
- Strcpy(e2.name, "James Bond");
- e2.salary=126000;
- //printing first employee information
- Printf( "employee 1 id : %d\n", e1.id);
- Printf( "employee 1 name : %s\n", e1.name);
- Printf( "employee 1 salary : %f\n", e1.salary);
- //printing second employee information
- Printf( "employee 2 id : %d\n", e2.id);
- Printf( "employee 2 name : %s\n", e2.name);
- Printf( "employee 2 salary : %f\n", e2.salary);
- Return 0;
- }
Output:
Employee 1 id : 101
Employee 1 name : Sonoo Jaiswal
Employee 1 salary : 56000.000000
Employee 2 id : 102
Employee 2 name : James Bond
Employee 2 salary : 126000.000000
Structure members cannot be initialized with declaration. For example the following C program fails in compilation.
StructPoint { Intx = 0; // COMPILER ERROR: cannot initialize members here Inty = 0; // COMPILER ERROR: cannot initialize members here }; |
The reason for above error is simple, when a datatype is declared, no memory is allocated for it. Memory is allocated only when variables are created.
Structure members can be initialized using curly braces ‘{}’. For example, following is a valid initialization.
StructPoint { Intx, y; };
Intmain() { // A valid initialization. Member x gets value 0 and y // gets value 1. The order of declaration is followed. StructPoint p1 = {0, 1}; } |
How to access structure elements?
Structure members are accessed using dot (.) operator.
#include<stdio.h>
StructPoint { Intx, y; };
Intmain() { StructPoint p1 = {0, 1};
// Accessing members of point p1 p1.x = 20; Printf("x = %d, y = %d", p1.x, p1.y);
Return0; } |
Output:
x = 20, y = 1
What is designated Initialization?
Designated Initialization allows structure members to be initialized in any order. This feature has been added in C99 standards.
#include<stdio.h>
StructPoint { Intx, y, z; };
Intmain() { // Examples of initialization using designated initialization StructPoint p1 = {.y = 0, .z = 1, .x = 2}; StructPoint p2 = {.x = 20};
Printf("x = %d, y = %d, z = %d\n", p1.x, p1.y, p1.z); Printf("x = %d", p2.x); Return0; } |
Output:
x = 2, y = 0, z = 1
x = 20
This feature is not available in C++ and works only in C.
Two variables of the same structure type can be copied the same way as ordinary variables. If persona1 and person2 belong to the same structure, then the following statements Are valid.
Person1 = person2;
Person2 = person1;
C does not permit any logical operators on structure variables In case, we need to compare them, we may do so by comparing members individually.
Person1 = = person2
Person1 ! = person2
Statements are not permitted.
Ex: Program for comparison of structure variables
Struct class
{
Int no;
Char name [20];
Float marks;
}
Main ( )
{
Int x;
Struct class stu1 = { 111, “Rao”, 72.50};
Struct class stu2 = {222, “Reddy”, 67.80};
Struct class stu3;
Stu3 = stu2;
x = ( ( stu3.no= = stu2.no) && ( stu3.marks = = stu2.marks))?1:0;
If ( x==1)
{
Printf (“ \n student 2 & student 3 are same \n”);
Printf (“%d\t%s\t%f “ stu3.no, stu3.name, stu3.marks);
}
Else
Printf ( “\n student 2 and student 3 are different “);
}
Out Put:
Student 2 and student 3 are same
222 reddy 67.0000
Structure is collection of different data type. An object of structure represents a singlerecord in memory, if we want more than one record of structure type, we have tocreate an array of structure or object. As we know, an array is a collection of similartype, therefore an array can be of structure type.
Syntax for declaring structure array
Structstruct-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
- - - - - - - - - -
DatatypevarN;
};
Structstruct-name obj [ size ];
Example for declaring structure array
#include<stdio.h>
Struct Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
};
Void main()
{
Inti;
Struct Employee Emp[ 3 ]; //Statement 1
For(i=0;i<3;i++)
{
Printf("\nEnter details of %d Employee",i+1);
Printf("\n\tEnter Employee Id : ");
Scanf("%d",&Emp[i].Id);
Printf("\n\tEnter Employee Name : ");
Scanf("%s",&Emp[i].Name);
Printf("\n\tEnter Employee Age : ");
Scanf("%d",&Emp[i].Age);
Printf("\n\tEnter Employee Salary : ");
Scanf("%ld",&Emp[i].Salary);
}
Printf("\nDetails of Employees");
For(i=0;i<3;i++)
Printf("\n%d\t%s\t%d\t%ld",Emp[i].Id,Emp[i].Name,Emp[i].Age,Emp[i].Salary);
}
Output :
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name :Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using
Array of object. Statement 1 is creating an array of Employee Emp to store the records
Of 3 employees.
Array within Structure
As we know, structure is collection of different data type. Like normal data type, It can
Also store an array as well.
Syntax for array within structure
Structstruct-name
{
Datatype var1; // normal variable
Datatype array [size]; // array variable
- - - - - - - - - -
- - - - - - - - - -
DatatypevarN;
};
Structstruct-name obj;
Example for array within structure
Struct Student
{
Int Roll;
Char Name[25];
Int Marks[3]; //Statement 1 : array of marks
Int Total;
FloatAvg;
};
Void main()
{
Inti;
Struct Student S;
Printf("\n\nEnter Student Roll : ");
Scanf("%d",&S.Roll);
Printf("\n\nEnter Student Name : ");
Scanf("%s",&S.Name);
S.Total = 0;
For(i=0;i<3;i++)
{
Printf("\n\nEnter Marks %d : ",i+1);
Scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
S.Avg = S.Total / 3;
Printf("\nRoll : %d",S.Roll);
Printf("\nName : %s",S.Name);
Printf("\nTotal : %d",S.Total);
Printf("\nAverage : %f",S.Avg);
}
Output :
Enter Student Roll : 10
Enter Student Name : Kumar
Enter Marks 1 : 78
Enter Marks 2 : 89
Enter Marks 3 : 56
Roll : 10
Name : Kumar
Total : 223
Average : 74.00000
In the above example, we have created an array Marks[ ] inside structure representing
3 marks of a single student.Marks[ ] is now a member of structure student and to
Access Marks[ ] we have used dot operator(.) along with object S.
Why use structure?
In C, there are cases where we need to store multiple attributes of an entity. It is not necessary that an entity has all the information of one type only. It can have different attributes of different data types. For example, an entity Student may have its name (string), roll number (int), marks (float). To store such type of information regarding an entity student, we have the following approaches:
- Construct individual arrays for storing names, roll numbers, and marks.
- Use a special data structure to store the collection of different data types.
Let's look at the first approach in detail.
- #include<stdio.h>
- Void main ()
- {
- Char names[2][10],dummy; // 2-dimensioanal character array names is used to store the names of the students
- Int roll_numbers[2],i;
- Float marks[2];
- For (i=0;i<3;i++)
- {
- Printf("Enter the name, roll number, and marks of the student %d",i+1);
- Scanf("%s %d %f",&names[i],&roll_numbers[i],&marks[i]);
- Scanf("%c",&dummy); // enter will be stored into dummy character at each iteration
- }
- Printf("Printing the Student details ...\n");
- For (i=0;i<3;i++)
- {
- Printf("%s %d %f\n",names[i],roll_numbers[i],marks[i]);
- }
- }
Output
Enter the name, roll number, and marks of the student 1Arun 90 91
Enter the name, roll number, and marks of the student 2Varun 91 56
Enter the name, roll number, and marks of the student 3Sham 89 69
Printing the Student details...
Arun 90 91.000000
Varun 91 56.000000
Sham 89 69.000000
The above program may fulfill our requirement of storing the information of an entity student. However, the program is very complex, and the complexity increase with the amount of the input. The elements of each of the array are stored contiguously, but all the arrays may not be stored contiguously in the memory. C provides you with an additional and simpler approach where you can use a special data structure, i.e., structure, in which, you can group all the information of different data type regarding an entity.
What is Structure
Structure in c is a user-defined data type that enables us to store the collection of different data types. Each element of a structure is called a member. Structures ca; simulate the use of classes and templates as it can store various information
The ,struct keyword is used to define the structure. Let's see the syntax to define the structure in c.
- Struct structure_name
- {
- Data_type member1;
- Data_type member2;
- .
- .
- Data_type memeberN;
- };
Let's see the example to define a structure for an entity employee in c.
- Struct employee
- { int id;
- Char name[20];
- Float salary;
- };
The following image shows the memory allocation of the structure employee that is defined in the above example.
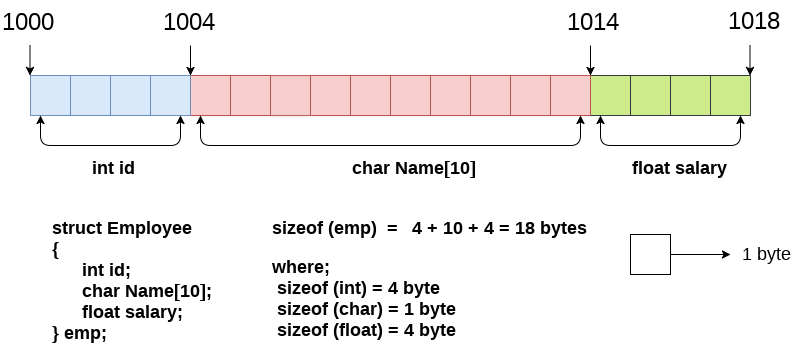
Here, struct is the keyword; employee is the name of the structure; id, name, and salary are the members or fields of the structure. Let's understand it by the diagram given below:
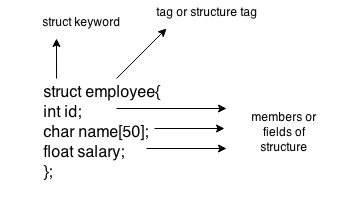
Declaring structure variable
We can declare a variable for the structure so that we can access the member of the structure easily. There are two ways to declare structure variable:
- By struct keyword within main() function
- By declaring a variable at the time of defining the structure.
1st way:
Let's see the example to declare the structure variable by struct keyword. It should be declared within the main function.
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- };
Now write given code inside the main() function.
- Struct employee e1, e2;
The variables e1 and e2 can be used to access the values stored in the structure. Here, e1 and e2 can be treated in the same way as the objects in C++ and Java.
2nd way:
Let's see another way to declare variable at the time of defining the structure.
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- }e1,e2;
Which approach is good
If number of variables are not fixed, use the 1st approach. It provides you the flexibility to declare the structure variable many times.
If no. Of variables are fixed, use 2nd approach. It saves your code to declare a variable in main() function.
Accessing members of the structure
There are two ways to access structure members:
- By . (member or dot operator)
- By -> (structure pointer operator)
Let's see the code to access the id member of p1 variable by. (member) operator.
- p1.id
C Structure example
Let's see a simple example of structure in C language.
- #include<stdio.h>
- #include <string.h>
- Struct employee
- { int id;
- Char name[50];
- }e1; //declaring e1 variable for structure
- Int main( )
- {
- //store first employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- //printing first employee information
- Printf( "employee 1 id : %d\n", e1.id);
- Printf( "employee 1 name : %s\n", e1.name);
- Return 0;
- }
Output:
Employee 1 id : 101
Employee 1 name : Sonoo Jaiswal
Let's see another example of the structure in C language to store many employees information.
- #include<stdio.h>
- #include <string.h>
- Struct employee
- { int id;
- Char name[50];
- Float salary;
- }e1,e2; //declaring e1 and e2 variables for structure
- Int main( )
- {
- //store first employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- e1.salary=56000;
- //store second employee information
- e2.id=102;
- Strcpy(e2.name, "James Bond");
- e2.salary=126000;
- //printing first employee information
- Printf( "employee 1 id : %d\n", e1.id);
- Printf( "employee 1 name : %s\n", e1.name);
- Printf( "employee 1 salary : %f\n", e1.salary);
- //printing second employee information
- Printf( "employee 2 id : %d\n", e2.id);
- Printf( "employee 2 name : %s\n", e2.name);
- Printf( "employee 2 salary : %f\n", e2.salary);
- Return 0;
- }
Output:
Employee 1 id : 101
Employee 1 name : Sonoo Jaiswal
Employee 1 salary : 56000.000000
Employee 2 id : 102
Employee 2 name : James Bond
Employee 2 salary : 126000.000000
C provides us the feature of nesting one structure within another structure by using which, complex data types are created. For example, we may need to store the address of an entity employee in a structure. The attribute address may also have the subparts as street number, city, state, and pin code. Hence, to store the address of the employee, we need to store the address of the employee into a separate structure and nest the structure address into the structure employee. Consider the following program.
- #include<stdio.h>
- Struct address
- {
- Char city[20];
- Int pin;
- Char phone[14];
- };
- Struct employee
- {
- Char name[20];
- Struct address add;
- };
- Void main ()
- {
- Struct employee emp;
- Printf("Enter employee information?\n");
- Scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
- Printf("Printing the employee information....\n");
- Printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
- }
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
The structure can be nested in the following ways.
- By separate structure
- By Embedded structure
1) Separate structure
Here, we create two structures, but the dependent structure should be used inside the main structure as a member. Consider the following example.
- Struct Date
- {
- Int dd;
- Int mm;
- Int yyyy;
- };
- Struct Employee
- {
- Int id;
- Char name[20];
- Struct Date doj;
- }emp1;
As you can see, doj (date of joining) is the variable of type Date. Here doj is used as a member in Employee structure. In this way, we can use Date structure in many structures.
2) Embedded structure
The embedded structure enables us to declare the structure inside the structure. Hence, it requires less line of codes but it can not be used in multiple data structures. Consider the following example.
- Struct Employee
- {
- Int id;
- Char name[20];
- Struct Date
- {
- Int dd;
- Int mm;
- Int yyyy;
- }doj;
- }emp1;
Accessing Nested Structure
We can access the member of the nested structure by Outer_Structure.Nested_Structure.member as given below:
- e1.doj.dd
- e1.doj.mm
- e1.doj.yyyy
C Nested Structure example
Let's see a simple example of the nested structure in C language.
- #include <stdio.h>
- #include <string.h>
- Struct Employee
- {
- Int id;
- Char name[20];
- Struct Date
- {
- Int dd;
- Int mm;
- Int yyyy;
- }doj;
- }e1;
- Int main( )
- {
- //storing employee information
- e1.id=101;
- Strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
- e1.doj.dd=10;
- e1.doj.mm=11;
- e1.doj.yyyy=2014;
- //printing first employee information
- Printf( "employee id : %d\n", e1.id);
- Printf( "employee name : %s\n", e1.name);
- Printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
- Return 0;
- }
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Passing structure to function
Just like other variables, a structure can also be passed to a function. We may pass the structure members into the function or pass the structure variable at once. Consider the following example to pass the structure variable employee to a function display() which is used to display the details of an employee.
- #include<stdio.h>
- Struct address
- {
- Char city[20];
- Int pin;
- Char phone[14];
- };
- Struct employee
- {
- Char name[20];
- Struct address add;
- };
- Void display(struct employee);
- Void main ()
- {
- Struct employee emp;
- Printf("Enter employee information?\n");
- Scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
- Display(emp);
- }
- Void display(struct employee emp)
- {
- Printf("Printing the details....\n");
- Printf("%s %s %d %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
- }
Structures and Functions in C: The C Programming allows us to pass the structures as the function parameters. Please refer to Functions in C Post before reading this post. It will help you to understand the concept of the function.
We can pass the C structures to functions in 3 ways:
- Passing each item of the structure as a function argument. It is similar to passing normal values as arguments. Although it is easy to implement, we don’t use this approach because if the size of a structure is a bit larger, then our life becomes miserable.
- Pass the whole structure as a value.
- We can also Pass the address of the structure (pass by reference).
Structures and Functions in C Programming examples
The following examples will explain to you, How to Pass Structures to functions in C by value and By reference
Pass Structure to a Function By Value in C
If the structure is passed to the function by the value, then Changes made to the structure variable members within the function will not reflect the original structure members.
This program for Structures and Functions in C, User is asked to enter, Student Name, First Year Marks, and Second Year Marks. By using these values, this program will check whether the student is eligible for a scholarship or not.
/* Passing Structure to a Function By Value */
#include <stdio.h>
Struct Student
{
CharStudent_Name[50];
FloatFirst_Year_Marks;
FloatSecond_Year_Marks;
};
VoidPassBy_Value(struct Student Students);
Int main()
{
Struct Student Student1;
Printf("\nPlease Enter the Student Name \n");
Scanf("%s",&Student1.Student_Name);
Printf("\nPlease Enter Student Inter First Year Marks \n");
Scanf("%f",&Student1.First_Year_Marks);
Printf("\nPlease Enter Student Inter Second Year Marks \n");
Scanf("%f",&Student1.Second_Year_Marks);
PassBy_Value(Student1);
Return 0;
}
VoidPassBy_Value(struct Student Students)
{
Float Sum, Average;
Sum = Students.First_Year_Marks + Students.Second_Year_Marks;
Average = Sum/2;
If(Average > 900)
{
Printf("\n %s is Eligible for Scholorship",Students.Student_Name);
}
Else
{
Printf("\n %s is Not Eligible for Scholorship",Students.Student_Name);
}
}
OUTPUT:
ANALYSIS
In these Structures and Functions in C example, Declared the Student structure with Student Name, First Year Marks, Second Year Marks members with appropriate Data Types.
Within the C programming main() function,
We created the Student structure variable Student1 using the below statement:
Struct Student Student1;
Printf and scanf statements in the next lines used to ask the users to enter the Student Name, First Year Marks, Second Year Marks.
As per our example, the values for Student1 are as follows
Student Name = Tutorialgateway.org;
First Year Marks = 800;
Second Year Marks = 750;
In the next line, we called the user-defined function
PassBy_Value(Student1);
When the compiler reaches this function, then it will traverse to the top to check for the function definition or else function declaration of the PassBy_Value (struct) function. If the function fails to identify the function with PassBy_Value name, then it will throw an error.
Function Definition
We declared 2 float local variables Sum and Average to calculate the Sum and average of every student’s first-year marks and second-year marks. Depending upon the result, this program will find whether the student is eligible for Scholarship or Not.
Sum = Students.First_Year_Marks + Students.Second_Year_Marks;
Sum = 800 + 750 = 1550
Average = Sum/2;
Average = 1550/2 = 775
In the next line, We used If statement to check whether the calculated average is greater than 900 or not
- If the calculated Average is Greater than 900 then, He is Eligible for Scholarship
- If the calculated Average is Less than or Equal to 900 then, He is Not Eligible for Scholarship
NOTE: Dot operator (.) is used to Assign or Access the values of Structure Variable
Passing Structure to a Function By Reference
In this Structure and Functions in C program, User asked to enter Lecturer Name, Total years of experience, and Total years of experience in this particular college.
/* Passing Structure to function by reference */
#include <stdio.h>
#include <string.h>
Struct Lecturer
{
CharLecturer_Name[50];
IntTotal_Experience;
IntExperience_In_This_College;
};
VoidPassBy_Reference(struct Lecturer *Lecturers);
Int main()
{
Struct Lecturer Lecturer1;
Printf("\nPlease Enter the Lecturer Name \n");
Scanf("%s",&Lecturer1.Lecturer_Name);
Printf("Please Enter Lecturers Total Years of Experience\n");
Scanf("%d",&Lecturer1.Total_Experience);
Printf("Enter Lecturers Total Years of Experience in this College\n");
Scanf("%d",&Lecturer1.Experience_In_This_College);
PassBy_Reference(&Lecturer1);
Printf("\n Lecturer Name = %s", Lecturer1.Lecturer_Name);
Printf("\n Lecturers Total Years of Experience = %d", Lecturer1.Total_Experience);
Printf("\n Years of Experience in this College = %d", Lecturer1.Experience_In_This_College);
Return 0;
}
VoidPassBy_Reference(struct Lecturer *Lecturers)
{
Strcpy(Lecturers->Lecturer_Name, "Tutorial Gateway");
Lecturers->Total_Experience = 5;
Lecturers->Experience_In_This_College = 3;
}
OUTPUT:
ANALYSIS:
Within this Structure and Functions in C example, Declared the Lecturer structure with Lecturer Name, Lecturers Total Years of Experience, and Lecturers Total Years of Experience in this College member with appropriate Data Types.
Within the main() function, we created Lecturer structure variable Lecturer1 using the below statement:
Struct Lecturer Lecturer1;
Printf and scanf statements in the next lines used to ask the users to enter the Lecturer Name, Lecturers Total Years of Experience, and Lecturers Total Years of Experience in this College.
As per our example, the values for Lecturer1 are as follows
Lecturer Name = Suresh
Lecturers Total Years of Experience = 10
Lecturers Total Years of Experience = 6
In the next line, we called the user-defined function
PassBy_Reference(&Lecturer1);
Here, We passed the address of the Lecturer1 structure to the function instead of its Clone. It means any changes made to the structural members within the function will reflect the original values.
Function Definition
Within the function, We haven’t done anything special, Just assigned new values to the Lecturer structure.
Strcpy(Lecturers->Lecturer_Name, "Tutorial Gateway");
Lecturers->Total_Experience = 5;
Lecturers->Experience_In_This_College = 3;
It means
Lecturer Name = Tutorial Gateway
Lecturers Total Years of Experience = 5
Lecturers Total Years of Experience = 3
Since we passed the address of the Lecturer1 to the PassBy_Reference() function, these new values will replace the user entered values. Please refer Call by Value and Call by Reference post for better understanding
NOTE: -> operator is used to Assign or Access the values of Pointer Structure Variable
Structure and Unions
What is Structure?
Structure is a user-defined data type in C programming language that combines logically related data items of different data types together.
All the structure elements are stored at contiguous memory locations. Structure type variable can store more than one data item of varying data types under one name.
What is Union
Union is a user-defined data type, just like a structure. Union combines objects of different types and sizes together. The union variable allocates the memory space equal to the space to hold the largest variable of union. It allows varying types of objects to share the same location.
Syntax of Declaring Structure
Struct [name of the structure]
{
Type member1;
Type member2;
Type member3;
};
Structure is declared using the "struct" keyword and name of structure. Number 1, number 2, number 3 are individual members of structure. The body part is terminated with a semicolon (;).
Example of Structure in C Programming
#include <stdio.h>
Struct student {
Char name[60];
Introll_no;
Float marks;
} sdt;
Int main() {
Printf("Enter the following information:\n");
Printf("Enter student name: ");
Fgets(sdt.name, sizeof(sdt.name), stdin);
Printf("Enter student roll number: ");
Scanf("%d", &sdt. Roll_no);
Printf("Enter students marks: ");
Scanf("%f", &sdt.marks);
Printf("The information you have entered is: \n");
Printf("Student name: ");
Printf("%s", sdt.name);
Printf("Student roll number: %d\n", sdt. Roll_no);
Printf("Student marks: %.1f\n", sdt.marks);
Return 0;
}
In the above program, a structure called student is created. This structure has three data members: 1) name (string), 2) roll_no (integer), and 3) marks (float).
After this, a structure variable sdt is created to store student information and display it on the computer screen.
Output:
Enter the following information:
Enter student name: James
Enter student roll number: 21
Enter student marks: 67
The information you have entered is:
Student name: John
Student roll number: 21
Student marks: 67.0
Syntax of Declaring Union
Union [name of union]
{
Type member1;
Type member2;
Type member3;
};
Union is declared using the "union" keyword and name of union. Number 1, number 2, number 3 are individual members of union. The body part is terminated with a semicolon (;).
Example of Union in C Programming
#include <stdio.h>
Union item
{
Int x;
Float y;
Charch;
};
Int main( )
{
Union item it;
It.x = 12;
It.y = 20.2;
It.ch = 'a';
Printf("%d\n", it.x);
Printf("%f\n", it.y);
Printf("%c\n", it.ch);
Return 0;
}
Output:
1101109601
20.199892
a
In the above program, you can see that the values of x and y gets corrupted. Only variable ch prints the expected result. It is because, in union, the memory location is shared among all member data types.
Therefore, the only data member whose value is currently stored, will occupy memory space. The value of the variable ch was stored at last, so the value of the rest of the variables is lost.
Structure Vs. Union
Here is the important difference between structure and union:
Structure | Union |
You can use a struct keyword to define a structure. | You can use a union keyword to define a union. |
Every member within structure is assigned a unique memory location. | In union, a memory location is shared by all the data members. |
Changing the value of one data member will not affect other data members in structure. | Changing the value of one data member will change the value of other data members in union. |
It enables you to initialize several members at once. | It enables you to initialize only the first member of union. |
The total size of the structure is the sum of the size of every data member. | The total size of the union is the size of the largest data member. |
It is mainly used for storing various data types. | It is mainly used for storing one of the many data types that are available. |
It occupies space for each and every member written in inner parameters. | It occupies space for a member having the highest size written in inner parameters. |
You can retrieve any member at a time. | You can access one member at a time in the union. |
It supports flexible array. | It does not support a flexible array. |
Advantages of structure
Here are pros/benefits for using structure:
- Structures gather more than one piece of data about the same subject together in the same place.
- It is helpful when you want to gather the data of similar data types and parameters like first name, last name, etc.
- It is very easy to maintain as we can represent the whole record by using a single name.
- In structure, we can pass complete set of records to any function using a single parameter.
- You can use an array of structure to store more records with similar types.
Advantages of union
Here, are pros/benefits for using union:
- It occupies less memory compared to structure.
- When you use union, only the last variable can be directly accessed.
- Union is used when you have to use the same memory location for two or more data members.
- It enables you to hold data of only one data member.
- Its allocated space is equal to maximum size of the data member.
Disadvantages of structure
Here are cons/drawbacks for using structure:
- If the complexity of IT project goes beyond the limit, it becomes hard to manage.
- Change of one data structure in a code necessitates changes at many other places. Therefore, the changes become hard to track.
- Structure is slower because it requires storage space for all the data.
- You can retrieve any member at a time in structure whereas you can access one member at a time in the union.
- Structure occupies space for each and every member written in inner parameters while union occupies space for a member having the highest size written in inner parameters.
- Structure supports flexible array. Union does not support a flexible array.
Disadvantages of union
Here, are cons/drawbacks for using union:
- You can use only one union member at a time.
- All the union variables cannot be initialized or used with varying values at a time.
- Union assigns one common storage space for all its members.
KEY DIFFERENCES:
- Every member within structure is assigned a unique memory location while in union a memory location is shared by all the data members.
- Changing the value of one data member will not affect other data members in structure whereas changing the value of one data member will change the value of other data members in union.
- Structure is mainly used for storing various data types while union is mainly used for storing one of the many data types.
- In structure, you can retrieve any member at a time on the other hand in union, you can access one member at a time.
- Structure supports flexible array while union does not support a flexible array.
The sizeof for a struct is not always equal to the sum of sizeof of each individual member. This is because of the padding added by the compiler to avoid alignment issues. Padding is only added when a structure member is followed by a member with a larger size or at the end of the structure.
Different compilers might have different alignment constraints as C standards state that alignment of structure totally depends on the implementation.
Let’s take a look at the following examples for better understanding:
- Case 1:
// C program to illustrate // size of struct #include <stdio.h>
Intmain() {
StructA {
// sizeof(int) = 4 Intx; // Padding of 4 bytes
// sizeof(double) = 8 Doublez;
// sizeof(short int) = 2 Shortinty; // Padding of 6 bytes };
Printf("Size of struct: %ld", sizeof(structA));
Return0; } |
Output:
Size of struct: 24
The red portion represents the padding added for data alignment and the green portion represents the struct members. In this case, x (int) is followed by z (double), which is larger in size as compared to x. Hence padding is added after x. Also, padding is needed at the end for data alignment.
- Case 2:
// C program to illustrate // size of struct #include <stdio.h>
Intmain() {
StructB { // sizeof(double) = 8 Doublez;
// sizeof(int) = 4 Intx;
// sizeof(short int) = 2 Shortinty; // Padding of 2 bytes };
Printf("Size of struct: %ld", sizeof(structB));
Return0; } |
Output:
Size of struct: 16
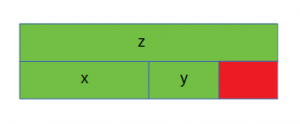
In this case, the members of the structure are sorted in decreasing order of their sizes. Hence padding is required only at the end.
- Case 3:
// C program to illustrate // size of struct #include <stdio.h>
Intmain() {
StructC { // sizeof(double) = 8 Doublez;
// sizeof(short int) = 2 Shortinty; // Padding of 2 bytes
// sizeof(int) = 4 Intx; };
Printf("Size of struct: %ld", sizeof(structC));
Return0; } |
Output:
Size of struct: 16
In this case, y (short int) is followed by x (int) and hence padding is required after y. No padding is needed at the end in this case for data alignment.
C language doesn’t allow the compilers to reorder the struct members to reduce the amount of padding. In order to minimize the amount of padding, the struct members must be sorted in a descending order (similar to the case 2).
Bit Fields
Suppose your C program contains a number of TRUE/FALSE variables grouped in a structure called status, as follows −
Struct{
UnsignedintwidthValidated;
UnsignedintheightValidated;
} status;
This structure requires 8 bytes of memory space but in actual, we are going to store either 0 or 1 in each of the variables. The C programming language offers a better way to utilize the memory space in such situations.
If you are using such variables inside a structure then you can define the width of a variable which tells the C compiler that you are going to use only those number of bytes. For example, the above structure can be re-written as follows −
Struct{
UnsignedintwidthValidated:1;
UnsignedintheightValidated:1;
} status;
The above structure requires 4 bytes of memory space for status variable, but only 2 bits will be used to store the values.
If you will use up to 32 variables each one with a width of 1 bit, then also the status structure will use 4 bytes. However as soon as you have 33 variables, it will allocate the next slot of the memory and it will start using 8 bytes. Let us check the following example to understand the concept −
#include<stdio.h>
#include<string.h>
/* define simple structure */
Struct{
UnsignedintwidthValidated;
UnsignedintheightValidated;
} status1;
/* define a structure with bit fields */
Struct{
UnsignedintwidthValidated:1;
UnsignedintheightValidated:1;
} status2;
Int main(){
Printf("Memory size occupied by status1 : %d\n",sizeof(status1));
Printf("Memory size occupied by status2 : %d\n",sizeof(status2));
Return0;
}
When the above code is compiled and executed, it produces the following result −
Memory size occupied by status1 : 8
Memory size occupied by status2 : 4
Bit Field Declaration
The declaration of a bit-field has the following form inside a structure −
Struct{
Type[member_name]: width ;
};
The following table describes the variable elements of a bit field −
Sr.No. | Element & Description |
1 | Type An integer type that determines how a bit-field's value is interpreted. The type may be int, signed int, or unsigned int. |
2 | Member_name The name of the bit-field. |
3 | Width The number of bits in the bit-field. The width must be less than or equal to the bit width of the specified type. |
The variables defined with a predefined width are called bit fields. A bit field can hold more than a single bit; for example, if you need a variable to store a value from 0 to 7, then you can define a bit field with a width of 3 bits as follows −
Struct{
Unsignedint age :3;
}Age;
The above structure definition instructs the C compiler that the age variable is going to use only 3 bits to store the value. If you try to use more than 3 bits, then it will not allow you to do so. Let us try the following example −
#include<stdio.h>
#include<string.h>
Struct{
Unsignedint age :3;
}Age;
Int main(){
Age.age=4;
Printf("Sizeof( Age ) : %d\n",sizeof(Age));
Printf("Age.age : %d\n",Age.age);
Age.age=7;
Printf("Age.age : %d\n",Age.age);
Age.age=8;
Printf("Age.age : %d\n",Age.age);
Return0;
}
When the above code is compiled it will compile with a warning and when executed, it produces the following result −
Sizeof( Age ) : 4
Age.age : 4
Age.age : 7
Age.age : 0
In programming, we may require some specific input data to be generated several numbers of times. Sometimes, it is not enough to only display the data on the console. The data to be displayed may be very large, and only a limited amount of data can be displayed on the console, and since the memory is volatile, it is impossible to recover the programmatically generated data again and again. However, if we need to do so, we may store it onto the local file system which is volatile and can be accessed every time. Here, comes the need of file handling in C.
File handling in C enables us to create, update, read, and delete the files stored on the local file system through our C program. The following operations can be performed on a file.
- Creation of the new file
- Opening an existing file
- Reading from the file
- Writing to the file
- Deleting the file
Functions for file handling
There are many functions in the C library to open, read, write, search and close the file. A list of file functions are given below:
No. | Function | Description |
1 | Fopen() | Opens new or existing file |
2 | Fprintf() | Write data into the file |
3 | Fscanf() | Reads data from the file |
4 | Fputc() | Writes a character into the file |
5 | Fgetc() | Reads a character from file |
6 | Fclose() | Closes the file |
7 | Fseek() | Sets the file pointer to given position |
8 | Fputw() | Writes an integer to file |
9 | Fgetw() | Reads an integer from file |
10 | Ftell() | Returns current position |
11 | Rewind() | Sets the file pointer to the beginning of the file |
Opening File: fopen()
We must open a file before it can be read, write, or update. The fopen() function is used to open a file. The syntax of the fopen() is given below.
- FILE *fopen( const char * filename, const char * mode );
The fopen() function accepts two parameters:
- The file name (string). If the file is stored at some specific location, then we must mention the path at which the file is stored. For example, a file name can be like "c://some_folder/some_file.ext".
- The mode in which the file is to be opened. It is a string.
We can use one of the following modes in the fopen() function.
Mode | Description |
r | Opens a text file in read mode |
w | Opens a text file in write mode |
a | Opens a text file in append mode |
r+ | Opens a text file in read and write mode |
w+ | Opens a text file in read and write mode |
a+ | Opens a text file in read and write mode |
Rb | Opens a binary file in read mode |
Wb | Opens a binary file in write mode |
Ab | Opens a binary file in append mode |
Rb+ | Opens a binary file in read and write mode |
Wb+ | Opens a binary file in read and write mode |
Ab+ | Opens a binary file in read and write mode |
The fopen function works in the following way.
- Firstly, It searches the file to be opened.
- Then, it loads the file from the disk and place it into the buffer. The buffer is used to provide efficiency for the read operations.
- It sets up a character pointer which points to the first character of the file.
Consider the following example which opens a file in write mode.
- #include<stdio.h>
- Void main( )
- {
- FILE *fp ;
- Char ch ;
- Fp = fopen("file_handle.c","r") ;
- While ( 1 )
- {
- Ch = fgetc ( fp ) ;
- If ( ch == EOF )
- Break ;
- Printf("%c",ch) ;
- }
- Fclose (fp ) ;
- }
Output
The content of the file will be printed.
#include;
Void main( )
{
FILE *fp; // file pointer
Charch;
Fp = fopen("file_handle.c","r");
While ( 1 )
{
Ch = fgetc ( fp ); //Each character of the file is read and stored in the character file.
If ( ch == EOF )
Break;
Printf("%c",ch);
}
Fclose (fp );
}
Most of the programs are written to store the information fetched from the program. One such way is to store the fetched information in a file. Different operations that can be performed on a file are:
- Creation of a new file (fopen with attributes as “a” or “a+” or “w” or “w++”)
- Opening an existing file (fopen)
- Reading from file (fscanf or fgets)
- Writing to a file (fprintf or fputs)
- Moving to a specific location in a file (fseek, rewind)
- Closing a file (fclose)
The text in the brackets denotes the functions used for performing those operations.
Functions in File Operations:
File opening modes in C:
“r” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file cannot be opened fopen( ) returns NULL.
“w” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“a” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer that points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“r+” – Searches file. If is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. Returns NULL, if unable to open the file.
“w+” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist a new file is created. Returns NULL, if unable to open file.
“a+” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
As given above, if you want to perform operations on a binary file, then you have to append ‘b’ at the last. For example, instead of “w”, you have to use “wb”, instead of “a+” you have to use “a+b”. For performing the operations on the file, a special pointer called File pointer is used which is declared as
FILE *filePointer;
So, the file can be opened as
FilePointer = fopen(“fileName.txt”, “w”)
The second parameter can be changed to contain all the attributes listed in the above table.
Reading from a file –
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Writing a file –:
The file write operations can be perfomed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as :
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Closing a file –:
After every successful fie operations, you must always close a file. For closing a file, you have to use fclose function. The snippet for closing a file is given as :
FILE *filePointer ;
FilePointer= fopen(“fileName.txt”, “w”);
---------- Some file Operations -------
Fclose(filePointer)
Example 1: Program to Open a File, Write in it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Write in it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Get the data to be written in file
ChardataToBeWritten[50]
= "GeeksforGeeks-A Computer Science Portal for Geeks";
// Open the existing file GfgTest.c using fopen()
// in write mode using "w" attribute
FilePointer = fopen("GfgTest.c", "w") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Write the dataToBeWritten into the file
If ( strlen ( dataToBeWritten ) > 0 )
{
// writing in the file using fputs()
Fputs(dataToBeWritten, filePointer) ;
Fputs("\n", filePointer) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully written in file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
Example 2: Program to Open a File, Read from it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Read from it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Declare the variable for the data to be read from file
ChardataToBeRead[50];
// Open the existing file GfgTest.c using fopen()
// in read mode using "r" attribute
FilePointer = fopen("GfgTest.c", "r") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Read the dataToBeRead from the file
// using fgets() method
While(fgets ( dataToBeRead, 50, filePointer ) != NULL )
{
// Print the dataToBeRead
Printf( "%s" , dataToBeRead ) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully read from file GfgTest.c\n");
Printf("The file is now closed.") ;4.13 Input/Output opening on files
Functions used in File Handling are as follows :
1. open
- Before opening a file it should be linked to appropriate stream i.e. input, output or input/output.
- If we want to create input stream we will write
Ifstreaminfile;
For output stream we will write
Ofstreamoutfile;
For Input/Output stream we will write
Fstreamiofile;
- Assume we want to write data to file named “Example” so we will use output stream. Then file can be opened as follows,
Ofstreamoutfile;
Outfile.open(“Example”);
- Now if we want to read data from file, so we will use input stream. Then file can be opened as follows,
Ifstreaminfile;
Infile.open(“Example”);
2. Close()
We use member function close() to close a file. The close function neither takes parameters nor returns any value.
e.g. Outfile.close();
Program to illustrate working with files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Char str1[50];
Ofstreamfout; // create out stream
Fout.open(“Sample”); //opening file named “Sample” with open function
Cout<<”Enter string”;
Cin>>str; //accepting string from user
Fout<<str<<\n”; //writing string to “Sample”file
Fout.close(); //closing “Sample” file
Ifstream fin; //create in stream
Fin.open(“Sample”); // open “Sample” file for reading”
Fin>>str; reading string from “Sample” file
Cout<<”String is”<<str; // display string to user
Fin.close();
Return 0 ;
}
Output
Enter String
C++
String is C++
3. read()
- It is used to read binary file.
- It is written as follows.
In.read((char *)&S, sizeof(S));
Where “in” is input stream.
‘S’ is variable.
- It takes two arguments. First argument is the address of variable S and second is length of variable S in bytes.
4. write()
- It is used to write binary file.
- It is written as follows
Out.write((char *)&S, sizeof(S));
Where “out” is output streams and ‘S’ is variable.
Program to illustrate I/O operations (read and write) on binary files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int marks[5],i;
Cout<<”Enter marks”;
For(i=0;i<=5;i++)
Cin>>marks[i];
Ofstreamfout;
Fout.open(“Example”);// open file for writing
Fout.write((char*)& marks, sizeof(marks));// write data to file
Fout.close();
Ifstream fin;
Fin.open(“Example”); // open file for reading
Fin.read((char*)& marks, sizeof (marks));// reading data from file
Cout<<”Marks are”;
For(i=0;i<=5;i++)
Cout<<marks[i]; //displaying data to screen
Fin.close();
Return 0 ;
}
5. Detecting end of file
- If there is no more data to read in a file, this condition is called as end of file.
- End of file in C++ can be detected by using function eof().
- It is a member function of ios class.
- It returns a non zero value (true) if end of file is encountered, otherwise returns zero.
Program to illustrate eof() function.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int x;
Ifstream fin; // declares stream
Fin.open(“myfile”, ios::in); // open file in read mode
Fin>>x; //get first number
While(!fin.eof()) //if not at end of file, continue
{ reading numbers
Cout<<x<<endl; //print numbers
Fin>>x; //get another number
}
Fin.close(); //close file
Return 0 ;
}
Error handling helps you during the processing of input-output statements by catching severe errors that might not otherwise be noticed. For input-output operations, there are several important error-handling phrases and clauses. These are as follows:
- AT END phrase
- INVALID KEY phrase
- NO DATA phrase
- USE AFTER EXCEPTION/ERROR declarative procedure
- FILE STATUS clause.
During input-output operations, errors are detected by the system, which sends messages; the messages are then monitored by ILE COBOL. As well, ILE COBOL will detect some errors during an input-output operation without system support. Regardless of how an error is detected during an input-output operation, the result will always be an internal file status of other than zero, a runtime message, or both.
An important characteristic of error handling is the issuing of a runtime message when an error occurs during the processing of an input-output statement if there is no AT END/INVALID KEY phrase in the input-output statement, USE AFTER EXCEPTION/ERROR procedure, or FILE STATUS clause in the SELECT statement for the file.
One thing to remember about input-output errors is that you choose whether or not your program will continue running after a less-than-severe input-output error occurs. ILE COBOL does not perform corrective action. If you choose to have your program continue (by incorporating error-handling code into your design), you must also code the appropriate error-recovery procedure.
Besides the error-handling phrases and clauses that specifically relate to input-output statements, user defined ILE condition handlers and ILE COBOL error handling APIs can also be used to handle I/O errors.
For each I/O statement, ILE COBOL registers a condition handler to catch the various I/O related conditions. These condition handlers are registered at priority level 185 which allows user defined condition handlers to receive control first.
As mentioned above, an ILE COBOL runtime message is issued when an error occurs and no appropriate AT END, INVALID KEY, USE procedure, or FILE STATUS clause exists for a file. The message, LNR7057, is an escape message. This message can be handled by a user-defined condition handler. If no condition handler can handle this message, message LNR7057 will be resent as a function check.
ILE COBOL has a function check condition handler that will eventually issue inquiry message LNR7207 unless an ILE COBOL error handling API has been defined.
The most important function of C/C++ is main() function. It is mostly defined with a return type of int and without parameters :
Int main() { /* ... */ }
We can also give command-line arguments in C and C++. Command-line arguments are given after the name of the program in command-line shell of Operating Systems.
To pass command line arguments, we typically define main() with two arguments : first argument is the number of command line arguments and second is list of command-line arguments.
Int main(intargc, char *argv[]) { /* ... */ }
Or
Int main(intargc, char **argv) { /* ... */ }
Argc (ARGument Count) is int and stores number of command-line arguments passed by the user including the name of the program. So if we pass a value to a program, value of argc would be 2 (one for argument and one for program name)
The value of argc should be non negative.
Argv(ARGument Vector) is array of character pointers listing all the arguments.
If argc is greater than zero,the array elements from argv[0] to argv[argc-1] will contain pointers to strings.
Argv[0] is the name of the program , After that till argv[argc-1] every element is command -line arguments.
TEXT BOOKS:
C & Data Structures (A practical approach) – by G.S. Baluja and G.K. Baluja, Dhanapatrai& Co publishers.