UNIT- 3
Function
In c, we can divide a large program into the basic building blocks known as function. The function contains the set of programming statements enclosed by {}. A function can be called multiple times to provide reusability and modularity to the C program. In other words, we can say that the collection of functions creates a program. The function is also known as procedureor subroutinein other programming languages.
Advantage of functions in C
There are the following advantages of C functions.
- By using functions, we can avoid rewriting same logic/code again and again in a program.
- We can call C functions any number of times in a program and from any place in a program.
- We can track a large C program easily when it is divided into multiple functions.
- Reusability is the main achievement of C functions.
- However, Function calling is always a overhead in a C program.
Function Aspects
There are three aspects of a C function.
- Function declaration A function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type.
- Function call Function can be called from anywhere in the program. The parameter list must not differ in function calling and function declaration. We must pass the same number of functions as it is declared in the function declaration.
- Function definition It contains the actual statements which are to be executed. It is the most important aspect to which the control comes when the function is called. Here, we must notice that only one value can be returned from the function.
SN | C function aspects | Syntax |
1 | Function declaration | Return_type function_name (argument list); |
2 | Function call | Function_name (argument_list) |
3 | Function definition | Return_type function_name (argument list) {function body;} |
The syntax of creating function in c language is given below:
- Return_type function_name(data_type parameter...){
- //code to be executed
- }
Types of Functions
There are two types of functions in C programming:
- Library Functions: are the functions which are declared in the C header files such as scanf(), printf(), gets(), puts(), ceil(), floor() etc.
- User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times. It reduces the complexity of a big program and optimizes the code.
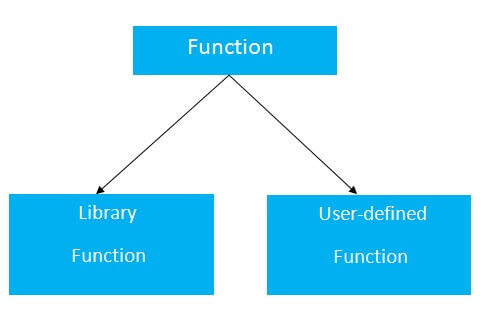
Return Value
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
Let's see a simple example of C function that doesn't return any value from the function.
Example without return value:
- Void hello(){
- Printf("hello c");
- }
If you want to return any value from the function, you need to use any data type such as int, long, char, etc. The return type depends on the value to be returned from the function.
Let's see a simple example of C function that returns int value from the function.
Example with return value:
- Int get(){
- Return 10;
- }
In the above example, we have to return 10 as a value, so the return type is int. If you want to return floating-point value (e.g., 10.2, 3.1, 54.5, etc), you need to use float as the return type of the method.
- Float get(){
- Return 10.2;
- }
Now, you need to call the function, to get the value of the function.
Different aspects of function calling
A function may or may not accept any argument. It may or may not return any value. Based on these facts, There are four different aspects of function calls.
- Function without arguments and without return value
- Function without arguments and with return value
- Function with arguments and without return value
- Function with arguments and with return value
Example for Function without argument and return value
Example 1
- #include<stdio.h>
- Void printName();
- Void main ()
- {
- Printf("Hello ");
- PrintName();
- }
- Void printName()
- {
- Printf("Javatpoint");
- }
Output
Hello Javatpoint
Example 2
- #include<stdio.h>
- Void sum();
- Void main()
- {
- Printf("\nGoing to calculate the sum of two numbers:");
- Sum();
- }
- Void sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Printf("The sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example for Function without argument and with return value
Example 1
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Int result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Result = sum();
- Printf("%d",result);
- }
- Int sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the area of the square
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Printf("Going to calculate the area of the square\n");
- Float area = square();
- Printf("The area of the square: %f\n",area);
- }
- Int square()
- {
- Float side;
- Printf("Enter the length of the side in meters: ");
- Scanf("%f",&side);
- Return side * side;
- }
Output
Going to calculate the area of the square
Enter the length of the side in meters: 10
The area of the square: 100.000000
Example for Function with argument and without return value
Example 1
- #include<stdio.h>
- Void sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Sum(a,b);
- }
- Void sum(int a, int b)
- {
- Printf("\nThe sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the average of five numbers.
- #include<stdio.h>
- Void average(int, int, int, int, int);
- Void main()
- {
- Int a,b,c,d,e;
- Printf("\nGoing to calculate the average of five numbers:");
- Printf("\nEnter five numbers:");
- Scanf("%d %d %d %d %d",&a,&b,&c,&d,&e);
- Average(a,b,c,d,e);
- }
- Void average(int a, int b, int c, int d, int e)
- {
- Float avg;
- Avg = (a+b+c+d+e)/5;
- Printf("The average of given five numbers : %f",avg);
- }
Output
Going to calculate the average of five numbers:
Enter five numbers:10
20
30
40
50
The average of given five numbers : 30.000000
Example for Function with argument and with return value
Example 1
- #include<stdio.h>
- Int sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Result = sum(a,b);
- Printf("\nThe sum is : %d",result);
- }
- Int sum(int a, int b)
- {
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers:10
20
The sum is : 30
Example 2: Program to check whether a number is even or odd
- #include<stdio.h>
- Int even_odd(int);
- Void main()
- {
- Int n,flag=0;
- Printf("\nGoing to check whether a number is even or odd");
- Printf("\nEnter the number: ");
- Scanf("%d",&n);
- Flag = even_odd(n);
- If(flag == 0)
- {
- Printf("\nThe number is odd");
- }
- Else
- {
- Printf("\nThe number is even");
- }
- }
- Int even_odd(int n)
- {
- If(n%2 == 0)
- {
- Return 1;
- }
- Else
- {
- Return 0;
- }
- }
Output
Going to check whether a number is even or odd
Enter the number: 100
The number is even
C Library Functions
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers. All C standard library functions are defined inside the different header files saved with the extension .h. We need to include these header files in our program to make use of the library functions defined in such header files. For example, To use the library functions such as printf/scanf we need to include stdio.h in our program which is a header file that contains all the library functions regarding standard input/output.
The list of mostly used header files is given in the following table.
SN | Header file | Description |
1 | Stdio.h | This is a standard input/output header file. It contains all the library functions regarding standard input/output. |
2 | Conio.h | This is a console input/output header file. |
3 | String.h | It contains all string related library functions like gets(), puts(),etc. |
4 | Stdlib.h | This header file contains all the general library functions like malloc(), calloc(), exit(), etc. |
5 | Math.h | This header file contains all the math operations related functions like sqrt(), pow(), etc. |
6 | Time.h | This header file contains all the time-related functions. |
7 | Ctype.h | This header file contains all character handling functions. |
8 | Stdarg.h | Variable argument functions are defined in this header file. |
9 | Signal.h | All the signal handling functions are defined in this header file. |
10 | Setjmp.h | This file contains all the jump functions. |
11 | Locale.h | This file contains locale functions. |
12 | Errno.h | This file contains error handling functions. |
13 | Assert.h | This file contains diagnostics functions. |
There are two methods to pass the data into the function in C language, i.e., call by value and call by reference.
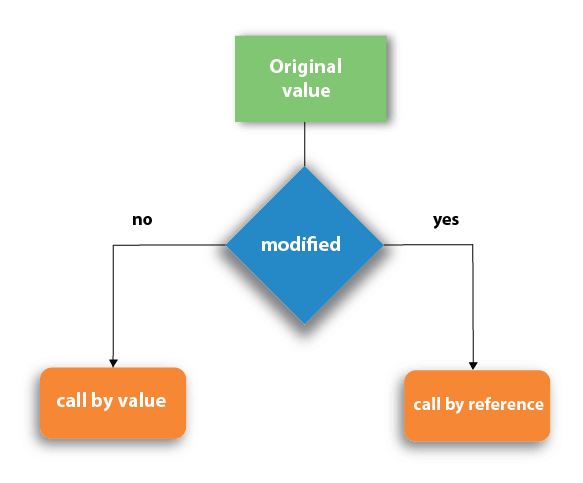
Let's understand call by value and call by reference in c language one by one.
Call by value in C
- In call by value method, the value of the actual parameters is copied into the formal parameters. In other words, we can say that the value of the variable is used in the function call in the call by value method.
- In call by value method, we cannot modify the value of the actual parameter by the formal parameter.
- In call by value, different memory is allocated for actual and formal parameters since the value of the actual parameter is copied into the formal parameter.
- The actual parameter is the argument which is used in the function call whereas formal parameter is the argument which is used in the function definition.
Let's try to understand the concept of call by value in c language by the example given below:
- #include<stdio.h>
- Void change(int num) {
- Printf("Before adding value inside function num=%d \n",num);
- Num=num+100;
- Printf("After adding value inside function num=%d \n", num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(x);//passing value in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
Call by Value Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int , int); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(a,b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The value of actual parameters do not change by changing the formal parameters in call by value, a = 10, b = 20
- }
- Void swap (int a, int b)
- {
- Int temp;
- Temp = a;
- a=b;
- b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",a,b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
Call by reference in C
- In call by reference, the address of the variable is passed into the function call as the actual parameter.
- The value of the actual parameters can be modified by changing the formal parameters since the address of the actual parameters is passed.
- In call by reference, the memory allocation is similar for both formal parameters and actual parameters. All the operations in the function are performed on the value stored at the address of the actual parameters, and the modified value gets stored at the same address.
Consider the following example for the call by reference.
- #include<stdio.h>
- Void change(int *num) {
- Printf("Before adding value inside function num=%d \n",*num);
- (*num) += 100;
- Printf("After adding value inside function num=%d \n", *num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(&x);//passing reference in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
Call by reference Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int *, int *); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(&a,&b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The values of actual parameters do change in call by reference, a = 10, b = 20
- }
- Void swap (int *a, int *b)
- {
- Int temp;
- Temp = *a;
- *a=*b;
- *b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",*a,*b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
Difference between call by value and call by reference in c
No. | Call by value | Call by reference |
1 | A copy of the value is passed into the function | An address of value is passed into the function |
2 | Changes made inside the function is limited to the function only. The values of the actual parameters do not change by changing the formal parameters. | Changes made inside the function validate outside of the function also. The values of the actual parameters do change by changing the formal parameters. |
3 | Actual and formal arguments are created at the different memory location | Actual and formal arguments are created at the same memory location |
In C, there are various general problems which requires passing more than one variable of the same type to a function. For example, consider a function which sorts the 10 elements in ascending order. Such a function requires 10 numbers to be passed as the actual parameters from the main function. Here, instead of declaring 10 different numbers and then passing into the function, we can declare and initialize an array and pass that into the function. This will resolve all the complexity since the function will now work for any number of values.
As we know that the array_name contains the address of the first element. Here, we must notice that we need to pass only the name of the array in the function which is intended to accept an array. The array defined as the formal parameter will automatically refer to the array specified by the array name defined as an actual parameter.
Consider the following syntax to pass an array to the function.
- Functionname(arrayname);//passing array
Methods to declare a function that receives an array as an argument
There are 3 ways to declare the function which is intended to receive an array as an argument.
First way:
- Return_type function(type arrayname[])
Declaring blank subscript notation [] is the widely used technique.
Second way:
- Return_type function(type arrayname[SIZE])
Optionally, we can define size in subscript notation [].
Third way:
- Return_type function(type *arrayname)
You can also use the concept of a pointer. In pointer chapter, we will learn about it.
C language passing an array to function example
- #include<stdio.h>
- Int minarray(int arr[],int size){
- Int min=arr[0];
- Int i=0;
- For(i=1;i<size;i++){
- If(min>arr[i]){
- Min=arr[i];
- }
- }//end of for
- Return min;
- }//end of function
- Int main(){
- Int i=0,min=0;
- Int numbers[]={4,5,7,3,8,9};//declaration of array
- Min=minarray(numbers,6);//passing array with size
- Printf("minimum number is %d \n",min);
- Return 0;
- }
Output
Minimum number is 3
C function to sort the array
- #include<stdio.h>
- Void Bubble_Sort(int[]);
- Void main ()
- {
- Int arr[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Bubble_Sort(arr);
- }
- Void Bubble_Sort(int a[]) //array a[] points to arr.
- {
- Int i, j,temp;
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] < a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Output
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Returning array from the function
As we know that, a function cannot return more than one value. However, if we try to write the return statement as return a, b, c; to return three values (a,b,c), the function will return the last mentioned value which is c in our case. In some problems, we may need to return multiple values from a function. In such cases, an array is returned from the function.
Returning an array is similar to passing the array into the function. The name of the array is returned from the function. To make a function returning an array, the following syntax is used.
- Int * Function_name() {
- //some statements;
- Return array_type;
- }
To store the array returned from the function, we can define a pointer which points to that array. We can traverse the array by increasing that pointer since pointer initially points to the base address of the array. Consider the following example that contains a function returning the sorted array.
- #include<stdio.h>
- Int* Bubble_Sort(int[]);
- Void main ()
- {
- Int arr[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Int *p = Bubble_Sort(arr), i;
- Printf("printing sorted elements ...\n");
- For(i=0;i<10;i++)
- {
- Printf("%d\n",*(p+i));
- }
- }
- Int* Bubble_Sort(int a[]) //array a[] points to arr.
- {
- Int i, j,temp;
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] < a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Return a;
- }
Output
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Recursion is the process which comes into existence when a function calls a copy of itself to work on a smaller problem. Any function which calls itself is called recursive function, and such function calls are called recursive calls. Recursion involves several numbers of recursive calls. However, it is important to impose a termination condition of recursion. Recursion code is shorter than iterative code however it is difficult to understand.
Recursion cannot be applied to all the problem, but it is more useful for the tasks that can be defined in terms of similar subtasks. For Example, recursion may be applied to sorting, searching, and traversal problems.
Generally, iterative solutions are more efficient than recursion since function call is always overhead. Any problem that can be solved recursively, can also be solved iteratively. However, some problems are best suited to be solved by the recursion, for example, tower of Hanoi, Fibonacci series, factorial finding, etc.
In the following example, recursion is used to calculate the factorial of a number.
- #include <stdio.h>
- Int fact (int);
- Int main()
- {
- Int n,f;
- Printf("Enter the number whose factorial you want to calculate?");
- Scanf("%d",&n);
- f = fact(n);
- Printf("factorial = %d",f);
- }
- Int fact(int n)
- {
- If (n==0)
- {
- Return 0;
- }
- Else if ( n == 1)
- {
- Return 1;
- }
- Else
- {
- Return n*fact(n-1);
- }
- }
Output
Enter the number whose factorial you want to calculate?5
Factorial = 120
We can understand the above program of the recursive method call by the figure given below:
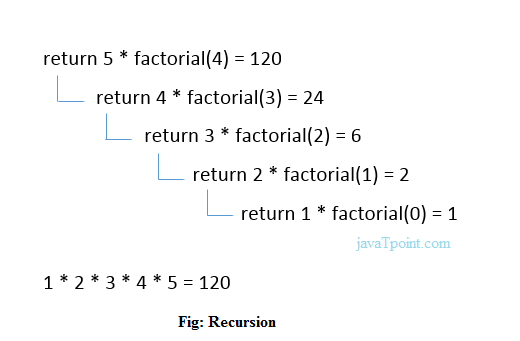
Recursive Function
A recursive function performs the tasks by dividing it into the subtasks. There is a termination condition defined in the function which is satisfied by some specific subtask. After this, the recursion stops and the final result is returned from the function.
The case at which the function doesn't recur is called the base case whereas the instances where the function keeps calling itself to perform a subtask, is called the recursive case. All the recursive functions can be written using this format.
Pseudocode for writing any recursive function is given below.
- If (test_for_base)
- {
- Return some_value;
- }
- Else if (test_for_another_base)
- {
- Return some_another_value;
- }
- Else
- {
- // Statements;
- Recursive call;
- }
Example of recursion in C
Let's see an example to find the nth term of the Fibonacci series.
- #include<stdio.h>
- Int fibonacci(int);
- Void main ()
- {
- Int n,f;
- Printf("Enter the value of n?");
- Scanf("%d",&n);
- f = fibonacci(n);
- Printf("%d",f);
- }
- Int fibonacci (int n)
- {
- If (n==0)
- {
- Return 0;
- }
- Else if (n == 1)
- {
- Return 1;
- }
- Else
- {
- Return fibonacci(n-1)+fibonacci(n-2);
- }
- }
Output
Enter the value of n?12
144
Memory allocation of Recursive method
Each recursive call creates a new copy of that method in the memory. Once some data is returned by the method, the copy is removed from the memory. Since all the variables and other stuff declared inside function get stored in the stack, therefore a separate stack is maintained at each recursive call. Once the value is returned from the corresponding function, the stack gets destroyed. Recursion involves so much complexity in resolving and tracking the values at each recursive call. Therefore, we need to maintain the stack and track the values of the variables defined in the stack.
Let us consider the following example to understand the memory allocation of the recursive functions.
- Int display (int n)
- {
- If(n == 0)
- Return 0; // terminating condition
- Else
- {
- Printf("%d",n);
- Return display(n-1); // recursive call
- }
- }
Explanation
Let us examine this recursive function for n = 4. First, all the stacks are maintained which prints the corresponding value of n until n becomes 0, Once the termination condition is reached, the stacks get destroyed one by one by returning 0 to its calling stack. Consider the following image for more information regarding the stack trace for the recursive functions.
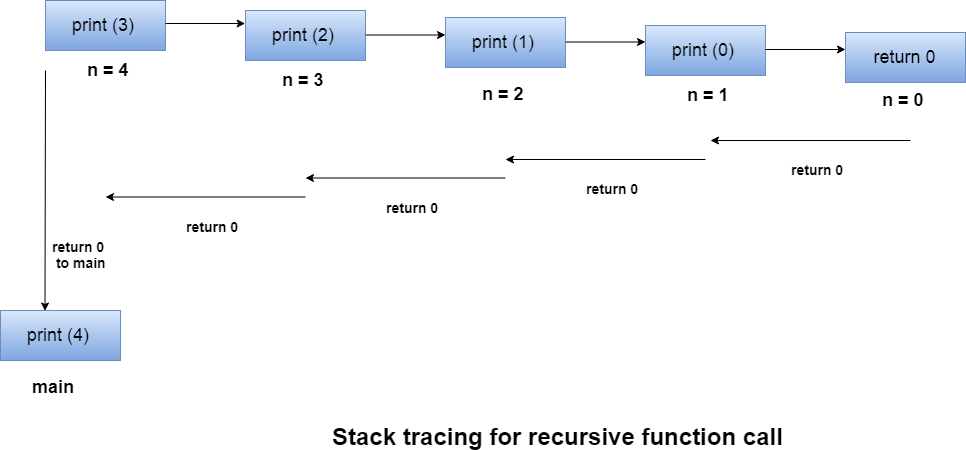
A function that calls itself is known as a recursive function. And, this technique is known as recursion.
How recursion works?
Void recurse()
{
... .. ...
Recurse();
... .. ...
}
Int main()
{
... .. ...
Recurse();
... .. ...
}
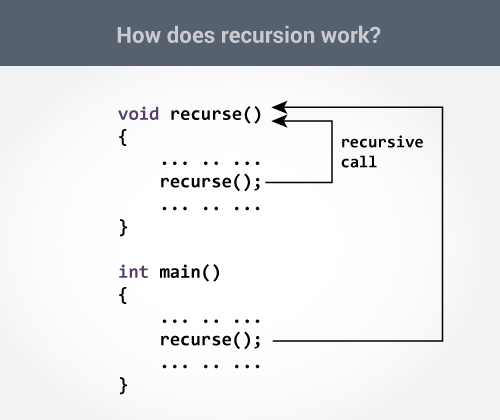
The recursion continues until some condition is met to prevent it.
To prevent infinite recursion, if...else statement (or similar approach) can be used where one branch makes the recursive call, and other doesn't.
Example: Sum of Natural Numbers Using Recursion
#include <stdio.h>
Int sum(int n);
Int main() {
Int number, result;
Printf("Enter a positive integer: ");
Scanf("%d", &number);
Result = sum(number);
Printf("sum = %d", result);
Return 0;
}
Int sum(int n) {
If (n != 0)
// sum() function calls itself
Return n + sum(n-1);
Else
Return n;
}
Output
Enter a positive integer:3
Sum = 6
Initially, the sum() is called from the main() function with number passed as an argument.
Suppose, the value of n inside sum() is 3 initially. During the next function call, 2 is passed to the sum() function. This process continues until n is equal to 0.
When n is equal to 0, the if condition fails and the else part is executed returning the sum of integers ultimately to the main() function.
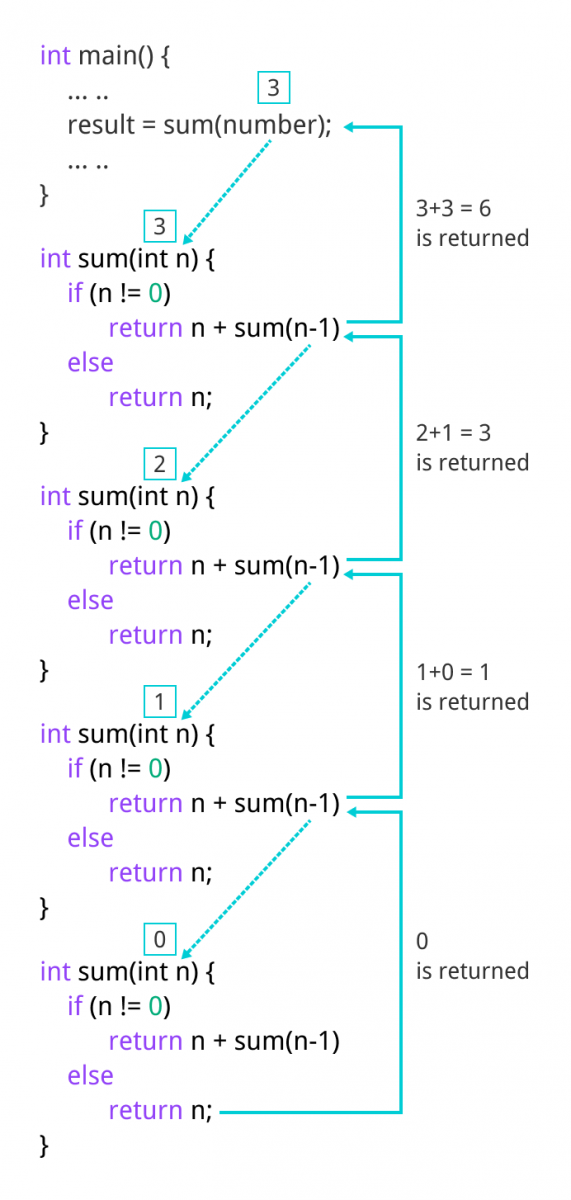
Advantages and Disadvantages of Recursion
Recursion makes program elegant. However, if performance is vital, use loops instead as recursion is usually much slower.
That being said, recursion is an important concept. It is frequently used in data structure and algorithms. For example, it is common to use recursion in problems such as tree traversal.
Program to find factorial of number using Recursion
This Program prompts user for entering any integer number, finds the factorial of input number and displays the output on screen. We will use a recursive user defined function to perform the task. Here we have a function find_factorial that calls itself in a recursive manner to find out the factorial of input number. We have involved the user interaction in the below program, however if you do not want that part then you can simply assign an integer value to variable num and ignore the scanf statement. In short you can tweak it in any way you want, the logic would be the same for each case.
Program to find factorial
/* Program Name: Find Factorial
Written by: Chaitanya Singh
Published on: beginnersbook.com
*/
#include<stdio.h>
Int find_factorial(int);
Int main()
{
Int num, fact;
//Ask user for the input and store it in num
Printf("\nEnter any integer number:");
Scanf("%d",&num);
//Calling our user defined function
Fact =find_factorial(num);
//Displaying factorial of input number
Printf("\nfactorial of %d is: %d",num, fact);
Return 0;
}
Int find_factorial(int n)
{
//Factorial of 0 is 1
If(n==0)
Return(1);
//Function calling itself: recursion
Return(n*find_factorial(n-1));
}
Output:
Enter any integer number: 4
Factorial of 4 is: 24
Fibonacci Series
Fibonacci Series in C: In case of fibonacci series, next number is the sum of previous two numbers for example 0, 1, 1, 2, 3, 5, 8, 13, 21 etc. The first two numbers of fibonacci series are 0 and 1.
There are two ways to write the fibonacci series program:
- Fibonacci Series without recursion
- Fibonacci Series using recursion
Fibonacci Series in C without recursion
Let's see the fibonacci series program in c without recursion.
- #include<stdio.h>
- Int main()
- {
- Int n1=0,n2=1,n3,i,number;
- Printf("Enter the number of elements:");
- Scanf("%d",&number);
- Printf("\n%d %d",n1,n2);//printing 0 and 1
- For(i=2;i<number;++i)//loop starts from 2 because 0 and 1 are already printed
- {
- n3=n1+n2;
- Printf(" %d",n3);
- n1=n2;
- n2=n3;
- }
- Return 0;
- }
Output:
Enter the number of elements:15
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377
Fibonacci Series using recursion in C
Let's see the fibonacci series program in c using recursion.
- #include<stdio.h>
- Void printFibonacci(int n){
- Static int n1=0,n2=1,n3;
- If(n>0){
- n3 = n1 + n2;
- n1 = n2;
- n2 = n3;
- Printf("%d ",n3);
- PrintFibonacci(n-1);
- }
- }
- Int main(){
- Int n;
- Printf("Enter the number of elements: ");
- Scanf("%d",&n);
- Printf("Fibonacci Series: ");
- Printf("%d %d ",0,1);
- PrintFibonacci(n-2);//n-2 because 2 numbers are already printed
- Return 0;
- }
Output:
Enter the number of elements:15
0 1 1 2 3 5 8 13 21 34 55 89 144 233 377
Ackermann Function
In computability theory, the Ackermann function, named after Wilhelm Ackermann, is one of the simplest and earliest-discovered examples of a total computable function that is not primitive recursive. All primitive recursive functions are total and computable, but the Ackermann function illustrates that not all total computable functions are primitive recursive. It’s a function with two arguments each of which can be assigned any non-negative integer.
Ackermann function is defined as:
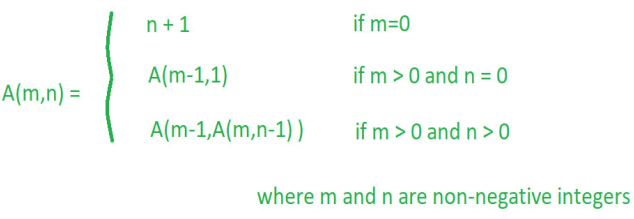
Ackermann algorithm:
Ackermann(m, n)
{next and goal are arrays indexed from 0 to m, initialized so that next[O]
Through next[m] are 0, goal[O] through goal[m - l] are 1, and goal[m] is -1}
Repeat
Value <-- next[O] + 1
Transferring <-- true
Current <-- O
While transferring do begin
If next[current] = goal[current] then goal[current] <-- value
Else transferring <-- false
Next[current] <-- next[current]+l
Current <-- current + 1
End while
Until next[m] = n + 1
Return value {the value of A(m, n)}
End Ackermann
Here’s the explanation of the given Algorithm:
Let me explain the algorithm by taking the example A(1, 2) where m = 1 and n = 2
So according to the algorithm initially the value of next, goal, value and current are:

Though next[current] != goal[current], so else statement will execute and transferring become false.
So now, the value of next, goal, value and current are:

Similarly by tracing the algorithm until next[m] = 3 the value of next, goal, value and current are changing accordingly. Here’s the explanation how the values are changing,


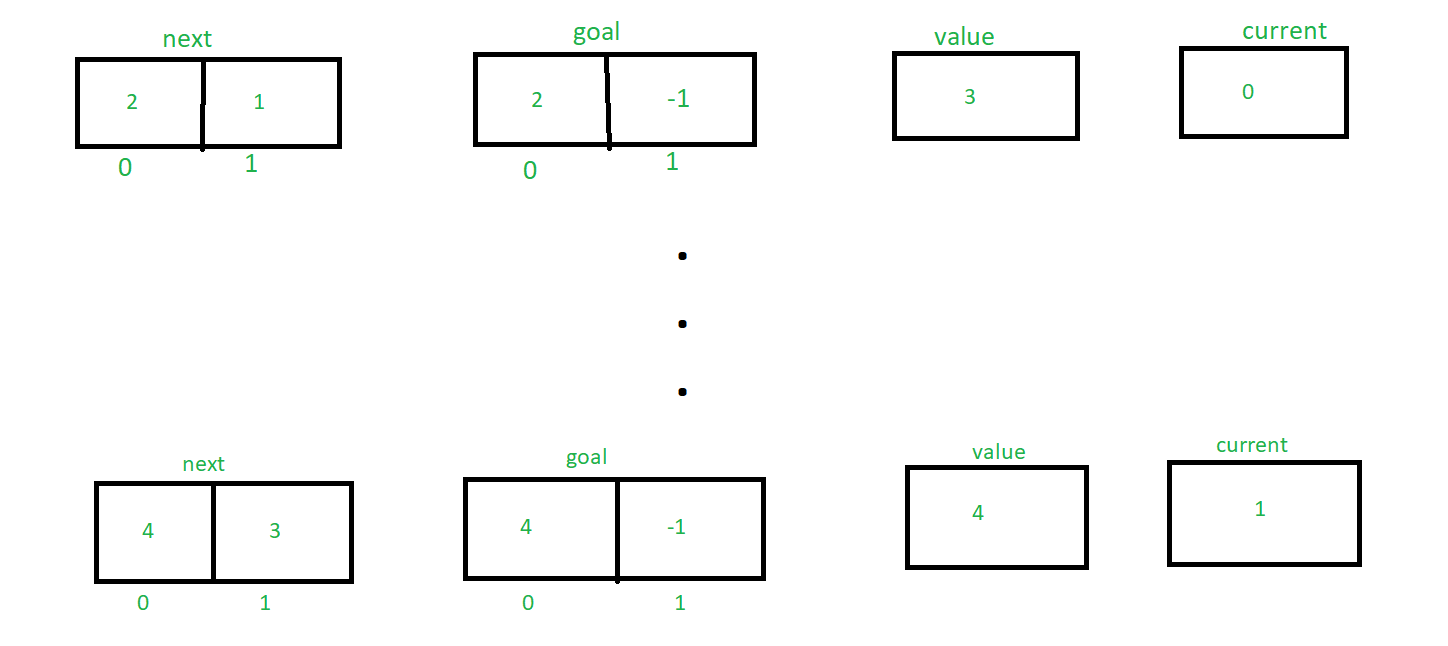
Finally returning the value e.g 4
Analysis of this algorithm:
- The time complexity of this algorithm is: O(mA(m, n)) to compute A(m, n)
- The space complexity of this algorithm is: O(m) to compute A(m, n)
Let’s understand the definition by solving a problem!
Solve A(1, 2)?
Answer:
Given problem is A(1, 2)
Here m = 1, n = 2 e.g m > 0 and n > 0
Hence applying third condition of Ackermann function
A(1, 2) = A(0, A(1, 1)) ———- (1)
Now, Let’s find A(1, 1) by applying third condition of Ackermann function
A(1, 1) = A(0, A(1, 0)) ———- (2)
Now, Let’s find A(1, 0) by applying second condition of Ackermann function
A(1, 0) = A(0, 1) ———- (3)
Now, Let’s find A(0, 1) by applying first condition of Ackermann function
A(0, 1) = 1 + 1 = 2
Now put this value in equation 3
Hence A(1, 0) = 2
Now put this value in equation 2
A(1, 1) = A(0, 2) ———- (4)
Now, Let’s find A(0, 2) by applying first condition of Ackermann function
A(0, 2) = 2 + 1 = 3
Now put this value in equation 4
Hence A(1, 1) = 3
Now put this value in equation 1
A(1, 2) = A(0, 3) ———- (5)
Now, Let’s find A(0, 3) by applying first condition of Ackermann function
A(0, 3) = 3 + 1 = 4
Now put this value in equation 5
Hence A(1, 2) = 4
So, A (1, 2) = 4
Let’s solve another two questions on this by yourself!
Question: Solve A(2, 1)?
Answer: 5
Question: Solve A(2, 2)?
Answer: 7
Here is the simplest c and python recursion function code for generating Ackermann function
// C++ program to illustrate Ackermann function #include <iostream> Using namespace std;
Int ack(int m, int n) { If (m == 0){ Return n + 1; } Else if((m > 0) && (n == 0)){ Return ack(m - 1, 1); } Else if((m > 0) && (n > 0)){ Return ack(m - 1, ack(m, n - 1)); } }
// Driver code Int main() { Int A; A = ack(1, 2); Cout << A << endl; Return 0; }
3.5 Recursion as a different way of solving problems Recursion is a common form of the general-purpose problem-solving technique called divide and conquer''. The principle of divide-and-conquer, is that you solve a given problem P in 3 steps:
This is often recursive, because in order to solve one or more of the subproblems, you might use this very same strategy. For instance, you might solve P1 be subdividing it into P1a,P1b..., solving each one of them, and putting together their solutions to get the solution S1 to problem P1. An Everyday Example To give a simple example, suppose you want to solve this problem: P = carry a pile of 50 books from your office to your car. Well, you can't even lift such a big pile, never mind carry it. So you split the original pile into 3 piles: P1 contains 10 books, P2 contains 15 books, P3 contains 25 books. Your plan, of course, is to carry each pile to the car separately, and then put them back together into one big pile once they are all there. This is a recursive divide-and-conquer strategy; you have divided the original problem P into 3 problems of the same kind. Now suppose you can directly solve P1 and P2 - they are small enough that you can carry them to the car. So, P1 and P2 are now sitting out there by the car. Once you get P3 there, you will pile them up again. But P3 is too big to be carried. You apply the same strategy recursively: divide P3 into smaller problems, solve them, and combine the results to make the solution for P3. You divide P3 into two piles, P3a has 11 books, P3b has 14, and carry these separately to the car. Then you put P3a back onto P3b to form the pile P3 there at the car. Now you have solved all the subproblems: P1, P2, P3 are sitting at the car. You stack them into one big pile, and presto, you have solved the original problem. This may seem like a rather hockey example, but it illustrates very well the principles of divide-and-conquer. Let us see how we can use the very same ideas to solve mathematical and computer science problems. A Mathematical Example Find an algorithm for raising a number X to the power N, where N is a positive integer. You may use only two operations: multiplication, subtraction. We would like to multiply N copies of X all at once, but, like the piles of books, we do not have an operation that directly allows us to do that: we can only multiply two numbers at a time. In other words, the problems we can solve directly are these:
Any other problem we have to solve indirectly, by breaking it down into smaller and smaller problems until we have reduced it to one of these base cases. So, if N > 2, we split the original problem into two subproblems:
How do we solve P1 and P2? They are problems of exactly the same type as original problem, so we can apply to them exactly the same strategy that we applied to the original problem. We check to see if we can solve them directly... If I=1 or I=2 we can solve P1 directly; if N-I=1 or N-I=2 we can solve P2 directly. The problems we cannot solve directly, we solve recursively, as just described. An interesting feature of this strategy is that it works no matter how we split up N. We can't directly solve this problem, so we divide it into:
We can directly solve P1, the answer is S1 = 9. But P2 we have to further decompose:
Again, P2.1 can be directly solved, S2.1 = 3, but P2.2 must be further decomposed:
These both can be directly solved; S2.2.1 = S2.2.2 = 9. These are combined to give S2.2 = 9*9 = 81. Now that we have the solutions to P2.1 and P2.2 we combine them to get the solution to P2, S2 = 3*81 = 243. Now that we have solutions for P1 and P2, we combine them to get the final solution, S = S1*S2 = 9*243 = 2187. I said that the strategy would work no matter how we chose to subdivide the problem: any choice of I' will give the correct answer. However, some choices of I' compute the final answer faster than others. Which do you think is faster: choosing I=1 (every time) or I=2? Answer: The computation will be faster if you choose I=2 because there will be half the number of recursive calls, and therefore half the overhead. This is true of most recursive strategies: they usually work correctly for many different ways of decomposing the problem, but some ways are much more efficient than others. In our first example, we also were free to divide the pile of books into as many piles and whatever sizes we liked. But it obviously would be very inefficient to carry one book at a time. Data Structures Examples Many problems involving data structures are naturally solved recursively. This is because most data structures are collections of components and they can easily be divided into subcollections that can be independently processed, just as, in our first example, we divided the pile of books into smaller piles that could be carried to the car separately. For example, suppose we want to add up the numbers stored in array X between positions FIRST and LAST. We can solve this directly in two very simple cases: FIRST=LAST: There is one number to be added, the answer is X[FIRST]. FIRST+1=LAST: There are 2 numbers to be added, the answer is X[FIRST] + X[LAST]. Otherwise, there are too many numbers to be added all at once, so we divide the problem into two subproblems by dividing the array into two parts:
The final answer is obtained by combining S1 and S2 in an appropriate way. In order to get the sum of all the numbers in the array, we add together the two partial sums: S = S1 + S2. The exact way we divide up the array does not affect the correctness of this strategy: M can be any value at all, FIRST<M<LAST. The structure' of the collection dictates which are the easiest ways to divide up the collection. As we've just seen arrays are very flexible, it is easy to divide them into a front and a back at any division point we care to choose. Other data structures are easily divided only in certain ways. For example, suppose we want to add up all the numbers in a stack. We'll base our solution on the recursive definition of stack we saw earlier: a stack is Empty, or has 2 parts, Top and Remainder. We can directly add up all the numbers in an Empty stack! There are none, and by convention, the sum of no numbers is 0. To add up the numbers in a non-empty stack, we will divide the stack into two parts, add up the parts separately, and combine the results. This is exactly what we did for arrays, but now we have to think, what is an easy way to divide a stack into two parts? The definition of stack gives us an immediate answer: a stack naturally decomposes into a Top element (which is a number, not a stack), and a Remainder, which is a stack. So we can decompose the problem of adding up the numbers in a nonempty stack into these two subproblems:
Now, combine S1 and S2 in the appropriate way to construct the final solution: S=S1+S2, or, S=Top value + S2. Of course, there is nothing special about adding'. The very same strategy would work if we wanted to multiply together all the values in a collection, to find the largest value, to print out the elements, to count the number of elements, and so on. Suppose we wished to sort the values in array X between positions FIRST and LAST. We can solve this directly in two very simple cases: FIRST=LAST: There is one number to be sorted, nothing needs to be done. FIRST+1=LAST: There are 2 numbers to be sorted; compare them and, if they are out of order, swap them. Otherwise, there are too many numbers to be sorted all at once, so we divide the problem into two subproblems by dividing the array into two parts:
The final answer is obtained by combining S1 and S2 in an appropriate way. The method for combining them is called merging', and it is a simple, efficient process. You can read about it in the textbook (section 5.4) or wait until we study sorting in detail later in the course. As before, the exact way we divide up the array does not affect the correctness of this strategy: M can be any value at all, FIRST<M<LAST. Certain values of M correspond to well-known sorting algorithms: M = 1 Gives rise to the sorting algorithm called insertion sort. M = the midpoint between FIRST and LAST Gives rise to the MergeSort algorithm. Both are correct, but their efficiency is very different: MergeSort is extremely fast (optimal in fact), whereas insertion sort is very slow.
|
Quick Sort
Quick sort is a highly efficient sorting algorithm and is based on partitioning of array of data into smaller arrays. A large array is partitioned into two arrays one of which holds values smaller than the specified value, say pivot, based on which the partition is made and another array holds values greater than the pivot value.
Implementation in C
#include <stdio.h>
#include <stdbool.h>
#define MAX 7
Int intArray[MAX] = {4,6,3,2,1,9,7};
Void printline(int count) {
Int i;
For(i = 0;i < count-1;i++) {
Printf("=");
}
Printf("=\n");
}
Void display() {
Int i;
Printf("[");
// navigate through all items
For(i = 0;i < MAX;i++) {
Printf("%d ",intArray[i]);
}
Printf("]\n");
}
Void swap(int num1, int num2) {
Int temp = intArray[num1];
IntArray[num1] = intArray[num2];
IntArray[num2] = temp;
}
Int partition(int left, int right, int pivot) {
Int leftPointer = left -1;
Int rightPointer = right;
While(true) {
While(intArray[++leftPointer] < pivot) {
//do nothing
}
While(rightPointer > 0 && intArray[--rightPointer] > pivot) {
//do nothing
}
If(leftPointer >= rightPointer) {
Break;
} else {
Printf(" item swapped :%d,%d\n", intArray[leftPointer],intArray[rightPointer]);
Swap(leftPointer,rightPointer);
}
}
Printf(" pivot swapped :%d,%d\n", intArray[leftPointer],intArray[right]);
Swap(leftPointer,right);
Printf("Updated Array: ");
Display();
Return leftPointer;
}
Void quickSort(int left, int right) {
If(right-left <= 0) {
Return;
} else {
Int pivot = intArray[right];
Int partitionPoint = partition(left, right, pivot);
QuickSort(left,partitionPoint-1);
QuickSort(partitionPoint+1,right);
}
}
Int main() {
Printf("Input Array: ");
Display();
Printline(50);
QuickSort(0,MAX-1);
Printf("Output Array: ");
Display();
Printline(50);
}
If we compile and run the above program, it will produce the following result −
Output
Input Array: [4 6 3 2 1 9 7 ]
==================================================
Pivot swapped :9,7
Updated Array: [4 6 3 2 1 7 9 ]
Pivot swapped :4,1
Updated Array: [1 6 3 2 4 7 9 ]
Item swapped :6,2
Pivot swapped :6,4
Updated Array: [1 2 3 4 6 7 9 ]
Pivot swapped :3,3
Updated Array: [1 2 3 4 6 7 9 ]
Output Array: [1 2 3 4 6 7 9 ]
==================================================
Merge Sort
Merge sort is a sorting technique based on divide and conquer technique. With the worst-case time complexity being Ο(n log n), it is one of the most respected algorithms.
Implementation in C
We shall see the implementation of merge sort in C programming language here −
#include <stdio.h>
#define max 10
Int a[11] = { 10, 14, 19, 26, 27, 31, 33, 35, 42, 44, 0 };
Int b[10];
Void merging(int low, int mid, int high) {
Int l1, l2, i;
For(l1 = low, l2 = mid + 1, i = low; l1 <= mid && l2 <= high; i++) {
If(a[l1] <= a[l2])
b[i] = a[l1++];
Else
b[i] = a[l2++];
}
While(l1 <= mid)
b[i++] = a[l1++];
While(l2 <= high)
b[i++] = a[l2++];
For(i = low; i <= high; i++)
a[i] = b[i];
}
Void sort(int low, int high) {
Int mid;
If(low < high) {
Mid = (low + high) / 2;
Sort(low, mid);
Sort(mid+1, high);
Merging(low, mid, high);
} else {
Return;
}
}
Int main() {
Int i;
Printf("List before sorting\n");
For(i = 0; i <= max; i++)
Printf("%d ", a[i]);
Sort(0, max);
Printf("\nList after sorting\n");
For(i = 0; i <= max; i++)
Printf("%d ", a[i]);
}
If we compile and run the above program, it will produce the following result −
Output
List before sorting
10 14 19 26 27 31 33 35 42 44 0
List after sorting
0 10 14 19 26 27 31 33 35 42 44
Text Books
(i) Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill
(ii) E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
Reference Books
(i) Brian W. Kernighan and Dennis M. Ritchie, The C Programming Language, Prentice Hall of India.