Unit – 2
Introduction to Classes and Objects, Inheritance and String Manipulations
Class can be defined as the core part of Java. It is the basic construct in Java upon which the entire language is created since it defines the shape and nature of an object.
A class consist of group of objects having similar properties. It is a logical entity which can be more complex in nature.
Class Declaration
To declare a class, use the class keyword. A class can be defined as:
Class class_name{
Type obj_varaible1;
Type obj_variable2;
.....
Type obj_variableN;
Type method_name1(parameters){
//method body
}
....
Type method_nameN(parameters){
//method body
}
}
All the data or variables declare inside a class are known as instance variables. The actual logical resides within the methods. And the collection of methods and variables in class are known as members.
Example of Class
Consider an example of class student that has two instances name and grade. There is no method declaration.
Class Student{
String name;
Int grade;
}
Whenever a class is declare that means a template is created, it is not an actual object. Therefore, object creation and declaration we will see in further topics.
Similarly till now there is no method created in above example.
Java provides methods so much power and flexibility. Methods are collection of statements that are combined together to perform an specific operation.
Methods can be declare as
Type method_name(parameters){
//method body
}
In the above syntax type represents the type of data returned by the method. If a method doesn’t return a value, in that case the data type of method will be void.
Parameters are those variables which are used to receive the value of arguments passed to the method. In case of no parameter, parenthesis will be blank.
Those methods which have a return types, a value is return by the method using the following syntax:
Return value;
Adding a Method to class
Class Student{
String name;
Int grade;
Void info() // display infor of students
System.out.print("Name is " + name + “ “ + “Grade is” + grade);
}
Class Example{
Public static void main(String args[]}
Student mystud1 = new Student();
Student mystud2 = new Student();
//assigning values to Student’s object
Mystud1.name = “Rahul”;
Mystud1.grade = 5;
Mystud2.name = “Sita”;
Mystud2.grade = 6;
Mystud1.info();
Mystud2.info();
}
}
Output is
Name is Rahul Grade is 5
Name is Sita Grade is 6
Returning a Value
When a method is called it either returns a value or returns nothing. When a program calls a method, the control of program gets transferred to the method.
After executing the method the control goes back to the program in two cases either returns statement is encountered or it reaches the method closing braces.
In case method doesn’t return a value, then it must be declared as void.
There are two points which need to take care while retiring values:
- Data type of the value return by the method must be same as that of return type specified by the method.
- The variable where the return value will be stored must of same data type as the data type of returning variable.
Passing Parameters by Value
Parameters make a method more generalized. Such methods can operate on different types of data or can be used in various situations.
An example of parameterized method is
Int cube(int i)
{
Return i*i*i;
}
An object is an instance of class. In Java object can be defined as the physical as well as logical entity.
Objects can be described using three parameters:
- State is used to define the data or value of an object.
- Behavior represents the functionality of an object.
- Identity is used to assign a unique id to every object,
Creating an object
To create an object in Java a class is used.
Let’s take above example of class student. To create an object of student class, specify the class name followed by the object name and use the new keyboard.
Syntax to create an object is
Classname object_name = new classname();
See the objects mystud1 and mystud2 in the above example of Student class.
Accessing Instance variables and methods
User created objects can access the instance variables and methods. Following are the syntax to access instance variables and methods by objects:
/* object creation */
Classname object_name = new classname();
/* call a variable */
Object_name.variableName;
/* call a class method */
Object_name.MethodName();
See the complete program above.
Data Hiding
Data hiding is a way of restricting the access to object details. It is one the feature of object oriented programming language.
This technique is used to ensure that on other class or an object try to penetrate into the code to access the data members of the class.
The same concept is in data encapsulation but there is a slight difference. Data hiding is used to limit the access of class data components while data encapsulation limits class data components as well as private methods.
To implement the concept of data hiding we use private access specifier with the class name.
Data Encapsulation
Encapsulation in Java represents a way of hiding the data (variables) and the methods as a single unit.
In encapsulation, the variables of a class will not be visible to other classes; they can only be accessed by the methods of their own class.
Data encapsulation sometimes is also known as data hiding.
To implement the concept of data encapsulation, declare all the variables of the class as private and declare methods as public to set and get the values of variable.
Example
Public class Demoencap {
Private String name;
Private int age;
Public int getAge() {
Return age;
}
Public String getName() {
Return name;
}
Public void setAge( int newAge) {
Age = newAge;
}
Public void setName(String newName) {
Name = newName;
}
}
Public class Usedemo{
Public static void main(String args[]) {
Demoencap cap = new Demoencap();
Cap.setName("Riya");
Cap.setAge(15);
System.out.print("Student Name : " + cap.getName() + “/n” + " Student Age : " + cap.getAge());
}
}
In the above example get() and set() methods are used to access the variables of the class. These methods are referred to as getter and setters.
Hence, if any class want to access their variable they have to use getter and setter for the same purpose.
The output of above program is:
Output
Student Name: Riya
Student Age: 15
Advantages of Encapsulation
- The members of a class can be declared as read-only or write-only.
- A class have complete control on the data stored inside its variables.
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Properties of a constructor:
1. Name of the constructor is the same as its class name.
2. A default constructor is always present in a class implicitly.
3. There are three main types of constructors namely default, parameterized and copy constructors.
4. A constructor in Java can not be abstract, final, static and Synchronized.
Public class NewClass{
//This is the constructor
NewClass(){
}}
The new keyword here creates the object of class NewClass and invokes the constructor to initialize this newly created object.
Default constructor:
A default constructor is a constructor that is created implicitly by the JVM.
Program to demonstrate default constructors:
Class student
{
Int id;
String name;
Student()
{
System.out.println("Student id is 20 and name is Amruta");
}
Public static void main(String args[])
{
Student s = new student();
}
}
Output:

Parameterized constructor:
It is a constructor that has to be created explicitly by the programmer. This constructor has parameters that can be passed when a constructor is called at the time of object creation.
Program:
Class Student4
{
Int id;
String name;
Student4(int i,String n)
{
Id = i;
Name = n;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student4 s1 = new Student4(20,"Rema");
Student4 s2 = new Student4(23,"Sadhna");
s1.display();
s2.display();
}
}
Output:

Copy constructor:
A content of one constructor can be copied into another constructor using the objects created. Such a constructor is called as a copy constructor.
Program:
Class Student6
{
Int id;
String name;
Student6(int i,String n)
{
Id = i;
Name = n;
}
Student6(Student6 s)
{
Id = s.id;
Name =s.name;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student6 s1 = new Student6(20,"AMRUTA");
Student6 s2 = new Student6(s1);
s1.display();
s2.display();
}
}
Output:

Static keyword in Java is mainly used for memory management. We can use static keyword with variables, methods, blocks and nested classes.
The main purpose of using static keyword is in the case when we want to share the same variable or method of a given class without creating their instances.
To declare such variable or methods prefix the static keyword with the variable name or method name.
If a member is declared as static, that means it can be accessed before the creation of any object of that class and without need of any object reference.
The best example of static keyword is the main(). We declare it as static so that it must be called before the existence of any object.
One more advantage of using static is that if any variable is declared using static then no copy of static variable is made separately for the created objects. Instead all the objects of the class use the same static variable.
Besides this their few restrictions in case of static method:
- They can call only other static methods and not any other type of methods.
- Only static data can access them.
- They cannot use this or super keyword.
Example
Class DemoStatic {
Static int x = 4; //static variable
Static int y;
Static void abc(int i) { //static method
System.out.println("i = " + i);
System.out.println("x = " + x);
System.out.println("y = " + y);
}
Static { //static block
System.out.println(“Example of static block initialized”);
y = x * 4;
}
Public static void main(String args[]) {
Abc(8);
}
}
Output
Example of static block initialized.
i = 8
x = 4
y = 16
To use a static method outside a class, following syntax will be used:
Classname.methodname()
This keyword is a reference variable that refers to current object. It can be used in number of scenarios.
- Used to refer instance variable of the current class
- can be used in a constructor
- can be passed as an argument in the method call and constructor call
- can be used to invoke current class constructor
Program:
Class thisDemo
{
Int i;
Int j;
// Parameterized constructor
ThisDemo(int i, int j)
{
This.i = i;
This.j = j;
}
Void display()
{
System.out.println("i=" +i);
System.out.println("j=" +j);
}
Public static void main(String[] args)
{
ThisDemo object = new thisDemo(10, 20);
Object.display();
}
}
Output:
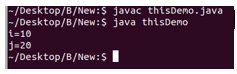
As the name suggests array of objects, Java provides us the flexibility to create an array of objects. This type of array store objects.
In an array, the element stores the reference variable of the object.
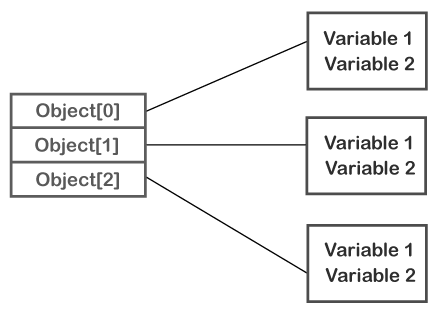
Array of Objects
Creating an Array of Objects
To create an array of Objects we use the Object class, which is the root class of all the classes.
Two syntax are there
Class_name[] objectArrayReference;
Or
Class_name objectArrayReference[];
Where objectArrayReference represents an array of objects.
Instantiating the Array of Objects
Syntax
Class_name obj[] = new Class_name[Array_length]
Consider a class name Person, and we want to instantiate that class with 5 objects or object reference then example will be
Person[] personObjects = new Person[2];
After instantiating an array of objects, we need to create the array of objects using the new keyboard. See the example below:
Class ExampleArray{
Public static void main(String args[])
{
//create an array of person object
Person[] obj = new Person[2] ;
//create & initialize actual person objects using constructor
Obj[0] = new Person("Rahul",24);
Obj[1] = new Person("Sita", 21);
//display the person object data
System.out.println("Person 1:");
Obj[0].display();
System.out.println("Person 2:");
Obj[1].display();
}
}
//Person class with person name and person age as attributes
Class Person
{
String per_name;
Int per_age;
//Person class constructor
Person(String n, int a)
{
Per_name = n;
Per_age = a;
}
Public void display()
{
System.out.print("Person Name = "+ per_name + " " + " Person age = "+per_age);
System.out.println();
}
}
Output
Person 1:
Person Name = Rahul Person age = 24
Person 2:
Person Name = Sita Person age = 21
Basically, there are two types of modifiers in Java, access modifiers and non-access modifiers.
In Java there are four types of access modifiers according to the level of access required for classes, variable, methods and constructors. Access modifiers like public, private, protected and default.
Default Access Modifier
In this access modifier we do not specify any modifier for a class, variable, method and constructor.
Such types of variables or method can be used by any other class. There are no restrictions in this access modifier.
There is no syntax for default modifier as declare the variables and methods without any modifier keyword.
Example-
String name = “Disha”;
Boolean processName() {
Return true;
}
Private Access Modifier
This access modifier uses the private keyword. By using this access modifier the methods, variable and constructors become private and can only be accessed by the member of that same class.
It is the most restrict access specifier. Class and interface cannot be declared using the private keyword.
By using private keyword we can implement the concept of encapsulation, which hides data from the outside world.
Example of private access modifier
Public class ExampleAcces {
Private String ID;
Public String getID() {
Return this.ID;
}
Public void setID(String ID) {
This.ID = ID;
}
}
In the above example the ID variable of the ExampleAccess class is private, so no other class can retrieve or set its value easily.
So, to access this variable for use in the outside world, we defined two public methods: getID(), which returns the value of ID, and setID(String), which sets its value.
Public Access Modifier
As the name suggest a public access modifier can be easily accessed by any other class or methods.
Whenever we specify public keyword to a class, method, constructor etc, then they can be accessed from any other class in the same package. Outside package we have to import that class for access because of the concept of inheritance.
In the below example, function uses public access modifier:
Public static void main(String[] arguments) {
// ...
}
It is necessary to declare the main method as public, else Java interpreter will not be able to called it to run the class.
Protected Access Modifier
In this access modifier, in a superclass if variables, methods and constructors are declared as protected then only the subclass in other package or any class within the same package can access it.
It cannot be assigned to class and interfaces. In general method’s fields can be declared as protected: however methods and fields of interface cannot be defined as protected.
It cannot be defined on the class.
An example of protected access modifier is shown below:
Class Person {
Protected int ID(ID pid) {
// implementation details
}
}
Class Employee extends Person {
Int ID(ID pid) {
// implementation details
}
}
Tips for Access Modifier
- A public method declared in a superlcass, must also be public in all subclasses.
- In a superclass if a method declared as protected, then in subclass it should be either protected or public, they cannot be private.
- Private methods cannot be inherit.
Inheritance is one of the main principles of OOP language. By using this principle one can inherit all the properties of other class. Inheritance allows us to manage information in a hierarchal manner.
Inheritance use two types of class: Superclass and subclass. Superclass also known as parent or base class is the class whose properties are inherited while subclass also known as derived or child class is the class who inherits the properties.
To inherit a class we simply use the extend keyword which allows to inherit the definition of class to another as shown below:
Class subclass-name extends superclass-name {
// body of class
}
Lets look at a simple example of inheritance:
Class First { // Superclass.
Int x, y;
Void showxy() {
System.out.println("x and y: " + x + " " + y);
}
}
Class Second extends First { // Subclass Second by extending class First.
Int z;
Void showz() {
System.out.println("z: " + z);
}
Void sum() {
System.out.println("x+y+z: " + (x+y+z));
}
}
Class Example {
Public static void main(String args[]) {
First superOb = new First();
Second subOb = new Second();
SuperOb.x = 100;
SuperOb.y = 200;
System.out.println("Data of superclass: ");
SuperOb.showxy();
System.out.println();
/* The subclass can access all public members of its superclass. */
SubOb.x = 45;
SubOb.y = 10;
SubOb.z = 5;
System.out.println("Contents of subOb: ");
SubOb.showxy();
SubOb.showz();
System.out.println();
System.out.println("Sum of i, j and k in subOb:");
SubOb.sum();
}
}
Output
Data of superclass:
x and y: 100 200
Contents of subOb:
x and y: 45 10
z: 5
Sum of x, y and z in subOb:
x+y+z: 60
Types of Inheritance
1. Single Inheritance
Also called as single level inheritance it is the mechanism of deriving a single sub class from a super class. The subclass will be defined by the keyword extends which signifies that the properties of the super class are extended to the sub class. The sub class will contain its own variables and methods as well as it can access methods and variables of the super class.
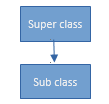
Syntax:
Class Subclass-name extends Superclass-name
{
//methods and fields
}
Program:
Class x
{
Void one()
{
System.out.println("Printing one");
}
}
Class y extends x
{
Void two()
{
System.out.println("Printing two");
}
}
Public class test
{
Public static void main(String args[])
{
y t= new y();
t.two();
t.one();
}
}
Output:
Printing two
Printing one
2. Multi- level Inheritance
It is the mechanism of deriving a sub class from a super class and again a sub class is derived from this derived subclass which acts as a base class for the newly derived class. It includes a multi level base class which allows building a chain of classes where a derived class acts as super class.
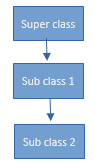
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}class three extends two
{
Void print_three()
{
System.out.println("Printing three");}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
t.print_three();
t.print_two(); t.print_one();
}
}
Output:
Printing three
Printing two
Printing one
3. Hierarchical Inheritance
In Hierarchical Inheritance, one class serves as a super class (base class) for more than one sub class.
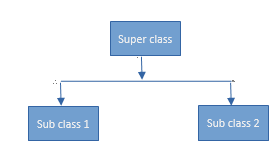
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}
Class three extends two
{
Void print_three()
{
System.out.println("Printing three");
}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
Two n= new two();t.print_three();
n.print_two();
t.print_one();
}}
Output:
Printing three
Printing two
Printing one
4. Hybrid Inheritance
It is the combination of Single inheritance and multi level inheritance.
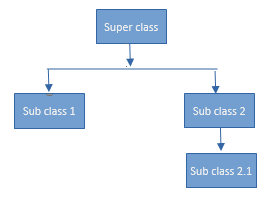
Inheritance is one of the most significant concept of object oriented programming which allow a class to inherit its properties to other class. This makes it easier to deploy and maintain an application.
Another benefit of using inheritance is code reusability. Code reusability means avoiding unnecessary code. In inheritance code can be used by other class and the subclass have to write its own unique properties only and rest of the properties will be inherited from the parent class or superclass.
Method overriding is also one benefit of using inheritance. Here a child class creates a method of same type as in parent class. In method overriding when we call the method, the child class method will be called. To call the parent class method we have to use super keyword.
In Java, when inheritance is implemented in that case constructor of base class gets automatically called in derived class.
In heritance constructors are called in the order they are derived i.e. from superclass to subclass.
The order of execution of constructor in inheritance will be from superclass to subclass inspite of the fact that super keyword is used or not.
If super() is used it will be the first statement to be executed by the subclass constructor. And if super() is not used in that case default or non parameterized constructor of each superclass will be executed first.
Example
Class P{ //Super class
P(){
System.out.println(“Superclass constructor”);
}}
Class C1 extends P{ //Sub class1
C1(){
System.out.println(“First subclass constructor”);
}}
Class C2 extends C1{ //Sub class2
C2(){
System.out.println(“Second subclass constructor”);
}}
Class Example{
Public static void main(String[] args)
C2 c = new C2();
}
}
Output of above program is
Superclass constructor
First subclass constructor
Second subclass constructor
It refers to immediate parent class object. There are 3 ways to use the ‘super’ keyword:
- To refer immediate parent class instance variable.
2. To invoke immediate parent class method.
3. To invoke immediate parent class constructor.
Program to refer immediate parent class instance variable using super keyword
Class transport
{
Int maxspeed = 200;
}
Class vehicle extends transport
{
Int maxspeed =100;
}
Class car extends vehicle
{
Int maxspeed = 120;
Void disp()
{System.out.println("Max speed= "+super.maxspeed);
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.disp();
}
}
Output:
Max speed= 100
Program to invoke immediate parent class method
Class transport
{
Void message()
{
System.out.println("transport");
}
}
Class vehicle extends transport
{
Void message()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Int maxspeed = 120;
Void message()
{
System.out.println("car");
}
Void display()
{
Message();
Super.message();
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.display();}
}
Output:
Car
Vehicle
Program to invoke immediate parent class constructor
Class vehicle
{
Vehicle()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Car()
{
Super();
System.out.println("car");
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
}
}
Output:
Vehicle
Car
Polymorphism can be defined as the ability to take different forms. It occurs when inheritance is used to have many classes that are related to each other.
Polymorphism most commonly used when a parent class reference is made to refer to a child class object.
Being one of the most important features of OOP, it must be supported by all languages based on OOP. Polymorphism in Java gives us the flexibility to use all the inherited properties of superclass to perform a task.
In real life, the simplest example to explain the concept of polymorphism is a person class. A single person can have different relationship with other person, like a mother, a daughter, a sister, a friend, an employee etc.
To better understand the concept of polymorphism we have to take a look at an example.
Consider a superclass named “Shapes” having a method name area(). The subclasses of this superclass can be Square, Rectangle, Triangle or any other shape. Using inheritance the subclass inherits the area() method also, but each shape has its own way of calculating area. Hence we can see that method name is same but it is used in different forms.
Class Shapes {
Public void area() {
System.out.println("Area of shape can be calculated as: \n");
}
}
Class Square extends Shapes {
Public void area() {
System.out.println("Area of Square is side * side ");
}
}
Class Rectangle extends Shapes {
Public void area() {
System.out.println("Area of Rectangle is base * height ");
}
}
Class Main {
Public static void main(String[] args) {
Shapes myShape = new Shapes(); // Create a Shapes object
Shapes mySquare = new Square(); // Create a Triangle object
Shapes myRectangle = new Rectangle(); // Create a Circle object
MyShape.area();
MySquare.area();
MyRectangle.area();
}
}
Output
Area of shape can be calculated as:
Area of Square is side * side
Area of Rectangle is base * height
Java supports two types of polymorphism:
- Compile time polymorphism
- Runtime polymorphism
Compile Time Polymorphism
It is also known as static polymorphism in Java. In this type, method call is made at compile time.
Compile type polymorphism is achieved through the concept of method overloading and operator overloading. However operator overloading is not supported in Java.
Method overloading can be defined as overloading of methods having same name i.e. when a class consist of multiple methods having same name but differs in the number, types, parameters and the return type of methods.
Java gives the flexibility to the user to create as many methods of same as long as it can be differentiate between them by the type and number of parameters.
Method overloading is explained in detail below.
Runtime Polymorphism
In Java, runtime polymorphism is also referred as Dynamic Binding or Dynamic Method Dispatch. In this process, at runtime we can call to an overridden method to process dynamically rather at compile time.
To implement the runtime polymorphism we us method overridden.
Method overridden happens when the child or subclass has a method with same definition as the parent or superclass, then that function overrides the function in the superclass.
In this process, a reference variable of a superclass is used to called an overridden method.
Detailed description of method overridden is discussed below.
The most significant use of polymorphism in Java is to write a method in different ways having multiple functionalities, with same method name.
Polymorphism helps to achieve consistency in a code.
Advantages of Polymorphism
- Code can be reusable in polymorphism, which means the classes that are already written can be used many times.
- We can use a single variable to store data values in multiple definition of methods. It will not change the value of a variable in the superclass, in fact allows storing subclass variable data also.
- Polymorphism allows lesser line of code, which helps the programmer to debug in case of error.
Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
Method overloading is an implementation of polymorphism in a code which is “One interface, multiple methods” paradigm.
To invoke an overloaded method, it is important to pass the right number or arguments or parameters; since this will determine which version of method to actually call.
All the overloaded methods have different return type but we cant distinguish method based on the type of data they return.
Consider a simple example of method overloading:
Class Addition {
// Overloaded method takes two int parameters
Public int add(int a, int b)
{
Return (a + b);
}
// Overloaded method takes three int parameters
Public int add(int a, int b, int c)
{
Return (a + b + c);
}
// Overloaded method takes two double parameters
Public double add(double a, double b)
{
Return (a + b);
}
Public static void main(String args[])
{
Addition a = new Addition();
System.out.println(a.add(100, 20));
System.out.println(a.add(11, 2, 3));
System.out.println(a.add(0.5, 2.5));
}
}
Output :
120
16
3.0
While creating a overloaded method it s not necessary that all the methods must relate to one another. For example one add() method can do addition, while other add() method can concatenate string; since each version of a method perform a separate activity you desire.
However, it is preferable to see a relationship between overloaded method as shown in the above example, where all add() method doing addition.
Benefits of Method Overloading
Technique over-burdening builds the coherence of the program.
This gives adaptability to developers with the goal that they can call similar strategy for various sorts of information.
This makes the code look spotless.
This lessens the execution time in light of the fact that the limiting is done in accumulation time itself.
Technique over-burdening limits the intricacy of the code.
With this, we can utilize the code once more, which saves memory.
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
Compiler differentiates between each constructor on the basis of their parameter list.
Example of constructor loading is given below:
Class Add {
Int x;
Int y;
// Overloaded constructor takes two int parameters
Add(int a, int b)
{
x = a;
y = b;
}
// Overloaded constructor single int parameters
Add(int a)
{
x = y = a;
}
// Overloaded constructor takes no parameters
Add()
{
x = 6;
y = 5;
}
Int sum() {
Return x + y;
}
}
Class OverloadAdd{
Public static void main(String args[])
{
// create objects using the various constructors
Add cons1 = new Add(23, 1);
Add cons2 = new Add(8);
Add cons3 = new Add();
Int s;
s = cons1.sum()
System.out.println(“Sum of constructor1 =” + s);
s = cons2.sum()
System.out.println(“Sum of constructor2 =” + s);
s = cons3.sum()
System.out.println(“Sum of constructor3 =” + s);
}
}
Output
Sum of constructor1 = 24
Sum of constructor2 = 16
Sum of constructor3 = 11
In Java, method overriding is a technique through which a subclass or derived class can provide its own version of method whose implementation is given by one its super class.
By doing this a subclass is defining a method having same name, same parameter, and same return type similar to method in the superclass. Then the method in the sublass is said to override the method in the superclass.
The concept of runtime polymorphism in Java can be implemented using Method overriding.
The next important question is which class method will be executed. So the answer is
- When an object of a parent class makes a call to method, then the parent class method will be executed.
- When an object of a child class makes a call to method, then the child class method will be executed.
Hence, the type of object used for referring determines which version of the method will be executed in the case of overridden method.
A simple example showing how overridden method are created and called.
//Parent class declaration
Class Parent{
Void display() //method defined in parent class
{
System.out.println(Method of parent class”);
}
}
//Child class declaration
Class Child extends Parent{
Void display() //child class method overrides parent class method
{
System.out.println(Method of child class”);
}
}
Class Demo{
Public static void main(String[] args)
{
Parent p = new Parent(); // object of parent class
Parent o = new Child(); // object of child class
p.display(); //reference made to parent class method by object p
o.display(); //reference made to child class method by object o
}
}
Output
Method of parent class
Method of child class
Rules for Method Overriding
- Overridden method must have same name and parameters.
- Method which is declared as final cannot be overridden.
- Static methods cannot be used to override.
- Overridden is not possible in private method.
- The return type of overriding method must be same.
- Constructors cannot be override.
- IS-A relationship must be fulfill in method overridden technique.
When a call to overridden method is made at run time instead of compile time, then this mechanism is known as Dynamic method dispatch. This is an example of run time polymorphism.
Like method overridden, here also the type of object decides which version of the method to be executed.
Advantages of using dynamic method dispatch are:
- It allows java to support runtime polymorphism.
- By using this, class methods can be shared by derived class methods with their own specific implementation.
- It allows subclass to create their own functionality in the derived method.
The most common thing for any programming language is string manipulations.
Java is a programming language where we can see an immense number of inherent functions to satisfy our aim of string manipulations.
You can do different things in Java programming like finding the string length, searching for a character in a string, String concatenation, finding substring, string modification, and so forth
We can likewise change the case of a given string; this is additionally a part of the string manipulations. We have some underlying functions to deal with this.
We can manipulate the string in following ways:
- String concatenations
- Change of case in String
- Sub string inside a string
- Removing unwanted character from string
- Reverse String
These are discussed below in String class.
The String class is a class which creates strings of fixed length. It is a built-in class from java.lang package. It represents a sequence of characters. The object of String class can be created implicitly or explicitly depending upon how the String is used. String objects are immutable, it means that String objects once made cannot be changed.
Syntax:
1.To create a String object implicitly, use a String literal and java creates a String object automatically to store the string.
String stringName;
StringName=”String”;
e.g.
String str;
Str=”Hello world”;
2. To create a String Object explicitly, instantiate a String class using the ‘new’ operator
String StringName= new StringName(“String”);
String str=new String(“Hello there!”);
Methods of String Class:
Method call | Task performed |
CharAt(int index) | Returns the char value at the specified index |
CompareTo(String anotherString) | Compares two strings |
CompareToIgnoreCase(String str) | Compares two strings alphabetically, ignoring case differences. |
Concat(String str) | Concatenates the specified string to the end of this string |
Contains(CharSequence s) | Returns true if and only if this string contains the specified sequence of char values |
Equals(Object anObject) | Compares this string to the specified object. |
EqualsIgnoreCase(String anotherString) | Compares this String to another String, ignoring case considerations. |
IndexOf(int ch) | Returns the index within this string of the first occurrence of the specified character. |
IsEmpty() | Returns true if, and only if, length() is 0. |
Length() | Returns the length of this string. |
Replace(char oldChar, char newChar) | Returns a new string resulting from replacing all occurrences of oldChar in this string with newChar. |
Substring(int beginIndex, int endIndex) | Returns a new string that is a substring of this string. |
ToLowerCase() | Converts the string to lowercase |
ToUpperCase() | Converts the string to uppercase |
Trim() | Removes leading and trailing whitespace omitted. |
Program to demonstrate the use of String class Methods
Import java.util.Scanner;
Public class string
{
Public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
System.out.println("PRESS \n 1.TO GET A CHARACTER AT 'N'(ANY POSITION) \n 2.TO GET THE LENGTH OF THE STRING \n 3. TO GET PART OF THE STRING FROM THE nth INDEX\n 4. TO GET PART OF THE STRING FROM nth INDEX TO n-1 INDEX \n 5. TO CHECK IF THE STRING CONTAINS ANOTHER STRING \n 6. TO CHECK IF STRING IS EQUAL TO ANOTHER STRING \n 7.TO CHECK IF THE STRING IS EQUAL TO ANOTHER STRING BY OMITTING THE CASE \n 8. TO CHECK IF STRING IS EMPTY \n 9. TO REPLACE PART OF THE STRING \n 10.TO ADD ANOTHER STRING TO EXISTING STRING \n 11.TO CONVERT A STRING TO UPPERCASE \n 12.TO CONVERT A STRING TO LOWERCASE \n 13.TO COMPARE THE STRING WITH ANOTHER STRING \n 14.REMOVE WHITE SPACES AT START AND END OF THE STRING");
Int choice = 0;
Do
{
System.out.println("\n ENTER YOUR CHOICE: ");
Choice = sc.nextInt();
Switch(choice)
{
Case 1: System.out.println("Enter the string: ");
String str = sc.next();
System.out.println("Enter the position: ");
Int pos = sc.nextInt();
System.out.println("The character at "+pos+" is "+str.charAt(pos-1));
Break;
Case 2: System.out.println("Enter the string: ");
String str2 = sc.next();
System.out.println("The length of the string is :"+str2.length());
Break;
Case 3: System.out.println("Enter the string: ");
String str3 = sc.next();System.out.println("Enter the position: ");
Int pos3 = sc.nextInt();
System.out.println("The part of the string is: "+str3.substring(pos3-1));
Break;
Case 4: System.out.println("Enter the string: ");
String str4 = sc.next();
System.out.println("Enter position 1: ");
Int pos1 = sc.nextInt();
System.out.println("Enter position 2: ");
Int pos2 = sc.nextInt();
System.out.println("The part of the string is: "+str4.substring(pos1-1,pos2));
Break;
Case 5: System.out.println("Enter string 1: ");
String str51 = sc.next();
System.out.println("Enter string 2: ");
String str52 = sc.next();
System.out.println(str51.contains(str52));
Break;
Case 6: System.out.println("Enter string 1: ");
String str61 = sc.next();
System.out.println("Enter string 2: ");
String str62 = sc.next();
System.out.println(str61.equals(str62));
Break;
Case 7: System.out.println("Enter string 1: ");
String str71 = sc.next();
System.out.println("Enter string 2: ");
String str72 = sc.next();
System.out.println(str71.equalsIgnoreCase(str72));
Break;
Case 8: System.out.println("Enter the string : ");
String str8 = sc.next();
System.out.println(str8.isEmpty());
Break;
Case 9: System.out.println("Enter the string : ");
String str9 = sc.next();
System.out.println("Enter character 1 : ");
Char c1 = sc.next().charAt(0);
System.out.println("Enter character 2 : ");
Char c2 = sc.next().charAt(0);System.out.println("new string: "+str9.replace(c1,c2));
Break;
Case 10:System.out.println("Enter string 1: ");
String str101 = sc.next();
System.out.println("Enter string 2: ");
String str102 = sc.next();
System.out.println("new string: "+str101.concat(str102));
Break;
Case 11:System.out.println("Enter the string:");
String str11 = sc.next();
System.out.println("new string: "+str11.toUpperCase());
Break;
Case 12:System.out.println("Enter the string:");
String str12 = sc.next();
System.out.println("new string: "+str12.toLowerCase());
Break;
Case 13:System.out.println("Enter string 1: ");
String str131 = sc.next();
System.out.println("Enter string 2: ");
String str132 = sc.next();
System.out.println(str131.compareTo(str132));
Break;
Case 14:System.out.println("Enter the string : ");
String str14 = sc.next();
System.out.println("new string:"+str14.trim());
Break;
}
}while(choice!=15);
}
}
Output:
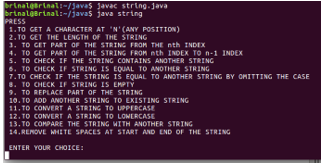
String Buffer class creates strings that can be modified in terms of length and content. This class can be used to insert characters and substrings in middle of string or append the string to the end. The objects of String Buffer class can be created by instantiating the String Buffer class.
Syntax:
StringBuffer stringName=new StringBuffer(“String”);
StringBuffer str=new StringBuffer(“Welcome students”);
Methods of StringBuffer class
Method call | Task Performed |
StringBuffer.delete(int start, int end) | Removes the characters in a substring of this sequence |
StringBuffer.deleteCharAt(int index) | Removes the char at the specified position in this sequence |
StringBuffer.append(String str) | Appends the specified string to this character sequence |
IndexOf(String str) | Returns the index within this string of the first occurrence of the specified substring |
StringBuffer.reverse() | Reverses the string |
StringBuffer.replace(int start, int end, String str) | Replaces the string with the given string at the given start and end position |
Program to demonstrate use of String Buffer class
Class stringBuffer
{
Public static void main(String args[])
{
StringBuffer str = new StringBuffer("Welcome");
System.out.println("Original String is: "+str);
System.out.println(str.append(" JAVA"));
System.out.println(str.insert(8,"HI "));
System.out.println(str.delete(8,11));
System.out.println(str.replace(8,12,"USER"));
System.out.println(str.charAt(8));
System.out.println("The length of the string is: "+ str.length());
System.out.println(str.substring(8));
System.out.println(str.indexOf("S"));
System.out.println(str.reverse());
}
}
In Java, String class are immutable i.e. no modification will be done in the succession of character. Therefore, StringBuilder came in use which creates a mutable or modifiable succession of characters.
The functionality of String Builder and String Buffer is almost same. Both of them helps in making mutable sequence of characters.
The StringBuilder class does not ensure synchronization while the String Buffer class does. Still StringBuilder class is the best option for working on a single thread, as it is much faster than String Buffer.
Class Declaration of StringBuilder
The class declaration is done using the java.lang.StringBuilder class which is a part of java.lang package.
Syntax is:
Public final class StringBuilder
Extends Object
Implements Serializable, CharSequence
Constructors in JavaStringBuilder
- StringBuilder(): Create a default string builder with no character in it having capacity of 16 characters.
- StringBuilder(int capacity): By using this we can create a string builder without characters in it and the capacity will be decided by the capacity argument.
- StringBuilder(CharSequence seq): It create a string builder having same characters as specified in CharSequence.
- StringBuilder(String str): It creates a string builder which initialized to the contents of the specified string.
Example of StringBuilder
Import java.util.*;
Import java.util.concurrent.LinkedBlockingQueue;
Public class Example {
Public static void main(String[] args)
Throws Exception
{
// StringBuilder object using StringBuilder constructor()
StringBuilder obj1 = new StringBuilder();
Obj1.append("My First Program");
System.out.println("String 1 = " + obj1.toString());
// StringBuilder object using StringBuilder(CharSequence) constructor
StringBuilder obj2 = new StringBuilder("Hello");
System.out.println("String 2 = " + obj2.toString());
// StringBuilder object using StringBuilder(capacity) constructor
StringBuilder obj3 = new StringBuilder(12);
System.out.println("String 3 = " + obj3.capacity());
// StringBuilder object using StringBuilder(String) constructor
StringBuilder obj4 = new StringBuilder(obj2.toString());
System.out.println("String 4 = " + obj4.toString());
}
}
Output
String 1 = My First Program
String 2 = Hello
String 3 = 12
String 4 = Hello
Different StringBuilder Methods
- StringBuilder append(String a): Use to append the given string with the already present string.
- StringBuilder insert(int i, String a): Use to insert the string into existing string on a particular character position.
- StringBuilder replace(int start, int end, String a): Use to replace the existing string with the new string only from the start to end index provided.
- StringBuilder delete(int start, int end): Use to delete a particular length string from the existing string based on the start and end index.
- StringBuilder reverse(): Use to reverse the string.
- Int capacity(): Reflect the capacity of current StringBuilder.
- Void ensureCapacity(int min): Use to set a minimum value for the StringBuilder capacity.
- Char charAt(int index): Use to return the specified index character.
- Int length(): Return the total length of the string.
- String substring(int start): Return the substring staring from the given index till end.
- String substring(int start, int end): Return the substring between the start and the end index.
- Int indexOf(String a): Return the index of first instance of string.
- Int lastIndexOf(String a): Return the index where the string ends.
- Void trimToSize(): Reduce the size of the StringBuilder.
Example based on some of the methods given above
Public class Example{
Public static void main(String[] args){
StringBuilder s = new StringBuilder(“Hello ”);
s.append(“World”);
System.out.println(s);
s.insert(2,”World”);
System.out.println(s);
s.replace(2,4,”World”);
System.out.println(s);
s.delete(2,4);
System.out.println(s);
s.reverse();
System.out.println(s);
}
}
Output
Hello World
HeWorldllo
HeWorldo
Heo
OlleH
In Java, there is a class in which a String is break down into tokens, this class is known as String Tokenizer and is available under java.util package.
In other words, we can say that a sentence is break down into words and then various operations can be applied on them like number of tokens counting.
String Tokenizer does break down of string by using the constructors and methods available in the class. While tokenizing string, it uses the delimiters given to the String Tokenizer class.
The most common types of delimiters are newline, tab, whitespace, carriage return and form fed. These are the default delimiters, besides this user can also create their own delimiter by defining them in the parameter as an argument.
String Tokenizer Constructors
1. StringTokenizer(String str)
By using all the default delimiters this constructor performs the tokenization of string. In this constructor the string is passed as the parameter.
2. StringTokenizer(String str, String delimiter)
In this string tokenization is done on the basis of the delimiter provided by the user in thr argument. Example shown below:
StringTokenizer s = new StringTokenizer(“My, first, program, on, StringTokenizer”, “,”);
While (s.hasMoreTokens())
System.out.println(s.nextToken());
Output
My
First
Program
StringTokenizer
3. StringTokenizer(String str, String delimiter, Boolean flag)
In this constructor tokenization is performed on the basis of delimiter and one extra Boolean expression to display delimiter also in the output. Example is shown below:
StringTokenizer s = new StringTokenizer(“My, First, Program”, “, ”, false);
While (s.hasMoreTokens())
System.out.println(s.nextToken());
Output
My
First
Program
If the Boolean expression value if false then in the output delimiter will not be shown. While if the Boolean expression value is TRUE, then the output must contain delimiter along with tokens as shown below:
StringTokenizer s = new StringTokenizer(“My, First, Program”, “, ”, true);
While (s.hasMoreTokens())
System.out.println(s.nextToken());
Output
My
,
First
,
Program
String Tokenizer Methods
- Int countTokens(): It is used to return the number of tokens which are separated by blank space in the existing string. This count can further be used in a loop parameter to process the string.
- String nextToken(): This method is used to get the next token in the string. Whenever we used it, it returns the next token as first token.
- String nextToken(String delimiter): This method is similar to the above method, the only difference is in the delimiter. The delimiter value decides the next token to be return.
- Boolean hasMoreTokens(): It is used to check the presence of more tokens in the string. A true value is return in case tokens are available and false in case no token is there.
- Object nextElement(): It is similar to nextToken(). The only difference is in the return value as nextElement return the value in form of object while nextToken() returns in form of string.
Example showing the use of all StringTokenizer method() and constructor is shown below:
Import java.util. * ;
Import java.io. * ;
Public class DemoString {
Public static void main(String[] args) throws IOException {
String s = "Hello Everyone doing code, on StringTokenizer class";
StringTokenizer str = new StringTokenizer(s);
Int n; // number of tokens
n = str.countTokens();
System.out.println("The string consist of tokens = " + n);
System.out.println(“Use of Default Constructor ”);
While (str.hasMoreTokens()) {
System.out.println(“The next token is” + str.nextToken());
}
StringTokenizer str1 = new StringTokenizer(s, “, ”);
System.out.println(“Token list with delimiter“, ”: ”);
While (str1.hasMoreTokens()) {
System.out.println(str1.nextToken());
}
StringTokenizer str2 = new StringTokenizer(s, “, ”, true);
System.out.println(“All tokens with delimiter“, ”and printing delimiter also: ”);
While (str2.hasMoreTokens()) {
System.out.println(str2.nextToken());
}
}
}
Output:
The string consist of tokens = 7
Use of Default constructor
The next token is Hello
The next token is Everone
The next token is doing
The next token is code,
The next token is on
The next token is StringTokenizer
The next token is class
Token list with delimiter , :
Hello Everyone doing code
On StringTokenizer class
All tokens with delimiter , and printing delimiter also :
Hello Everyone doing code
,
On StringTokenizer class
In Java we use primitive data type which is nothing but simple data types like int or double. They are used to store the basic data types supported by the language.
To increase the performance primitive data types are the preferred choice rather than objects. If we use objects for these values it will always create an extra burden on the simplest calculations, that is why, primitive types are used.
Besides performance parameter, there are chances when object reference will be needed. For example, primitive types cannot make reference to methods we need objects.
To handle these, Java provides type wrappers classes. Wrapper classes are those classes which are used to encapsulate a primitive data type inside an object.
The use of wrapper class in Java is to convert primitive types into object and object into primitive type. They are needed because Java is designed on the concept of object.
Need of Wrapper classes
- To convert the primitive data type into objects.
- Java provides the package name java.util.package which consists of all classes used to handle wrapper class.
- There are such data structures like ArrayList and Vector which doesn’t store primitive type, they only store Objects. Here we need wrapper class.
- It helps in synchronization of multithreading.
In Java, the java.lang package consist of total eight wrapper classes. These classes are:
Primitive Type | Wrapper class |
Boolean | Boolean |
Char | Character |
Byte | Byte |
Short | Short |
Int | Integer |
Long | Long |
Float | Float |
Double | Double |
Example of wrapper class
Public class Wrapper Demo {
Public static void main(String[] args) {
Integer myInt = 7;
Double myDouble = 8.1;
Character myChar = 'D';
System.out.println(myInt.intValue());
System.out.println(myDouble.doubleValue());
System.out.println(myChar.charValue());
}
}
Output
7
8.1
D
In Java, there are two types of constructor available for wrapper class:
- In first constructor, the primitive data type is passed as an argument.
- In second constructor, string is passed as an argument.
Example of both the constructor is:
Public class Demo
{
Public static void main(String[] args)
{
Byte b1 = new Byte((byte) 20); //Constructor which takes byte value as an argument
Byte b2 = new Byte("20"); //Constructor which takes String as an argument
Short s1 = new Short((short) 10); //Constructor which takes short value as an argument
Short s2 = new Short("5"); //Constructor which takes String as an argument
Integer i1 = new Integer(10); //Constructor which takes int value as an argument
Integer i2 = new Integer("10"); //Constructor which takes String as an argument
Long l1 = new Long(20); //Constructor which takes long value as an argument
Long l2 = new Long("20"); //Constructor which takes String as an argument
Float f1 = new Float(14.2f); //Constructor which takes float value as an argument
Float f2 = new Float("8.6"); //Constructor which takes String as an argument
Float f3 = new Float(8.6d); //Constructor which takes double value as an argument
Double d1 = new Double(7.8d); //Constructor which takes double value as an argument
Double d2 = new Double("7.8"); //Constructor which takes String as an argument
Boolean bl1 = new Boolean(true); //Constructor which takes boolean value as an argument
Boolean bl2 = new Boolean("false"); //Constructor which takes String as an argument
Character c1 = new Character('E'); //Constructor which takes char value as an argument
Character c2 = new Character("xyz"); //Compile time error : String abc can not be converted to character
}
}
Wrapper class are those class which encapsulates the primitive data type inside the object.
This encapsulation is done by converting the primitive data type into object and object into primitive data.
There are two concepts for the same:
- Autoboxing: converts primitive into object
- Unboxing: converts object into primitive
Autoboxing
Autoboxing defines the automatic conversion of the primitive data types to the object according to their desired wrapper classes. For example, conversion among int to Integer, long to Long, float to Float etc.
Example of autoboxing is
Class Demo
{
Public static void main(String[] args)
{
Int i = 10;
Integer a = Integer.valueOf(i); //int to Integer conversion
Integer y = i; //autoboxing, compiler will write Integer.valueOf(a) internally
System.out.println(i + “” + a + “” + y);
}
}
Output
10 10 10
Unboxing
The reverse of autoboxing is unboxing. It automatically converts an object into a corresponding primitive type. For example conversion Integer ti int, Long to long etc.
Public class Demo
Public static void main(String args[]){
Integer i = new Integer(10); //Converting Integer to int
Int j = i.intValue(); //converting Integer to int explicitly
Int k = i; //unboxing, now compiler will write a.intValue() internally
System.out.println(i+" "+j+" "+k);
}}
Output
10 10 10
References:
1. Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
2. CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
3. JAVA Complete Reference (9th Edition) Herbait Schelidt.