Unit - 4
IO Streams and Applet
Stream:
A stream is a method to sequentially access a file.
I/O Stream means an input source or output destination representing different types of sources
e.g., disk files. The java.io package provides classes that allow you to convert between Unicode character streams and byte streams of non-Unicode text.
Stream – A sequence of data.
Input Stream: reads data from source.
Output Stream: writes data to destination.
Byte Stream Classes:
Byte Stream classes are used to read bytes from the input stream and write bytes to the output stream.
In other word, Byte Stream classes read/write the data of 8-bits. We can store video, audio, characters, etc., by using Byte Stream classes.
These classes are part of the java.io package.
The Byte Stream classes are divided into two types of classes, i.e., Input Stream and Output Stream. These classes are abstract and the super classes of all the Input/Output stream classes.
Input Stream Class:
The Input Stream class provides methods to read bytes from a file, console or memory. It is an abstract class
SN | Class | Description | ||
1 | Buffered Input Stream | This class provides methods to read bytes from the buffer. | ||
2 | Byte Array Input Stream | This class provides methods to read bytes from the byte array. | ||
3 | Data Input Stream | This class provides methods to read Java primitive data types. | ||
4 | File Input Stream | This class provides methods to read bytes from a file. | ||
5 | Filter Input Stream | This class contains methods to read bytes from the other input streams, which are used as the primary source of data. | ||
6 | Object Input Stream | This class provides methods to read objects. | ||
7 | Piped Input Stream | This class provides methods to read from a piped output stream to which the piped input stream must be connected. | ||
8 | Sequence Input Stream | This class provides methods to connect multiple Input Stream and read data from them. | ||
The Input Stream class contains various methods to read the data from an input stream. These methods are overridden by the classes that inherit the Input Stream class.
However, the methods are given in the following table.
SN | Method | Description |
1 | Int read() | This method returns an integer, an integral representation of the next available byte of the input. The integer -1 is returned once the end of the input is encountered. |
2 | Int read (byte buffer []) | This method is used to read the specified buffer length bytes from the input and returns the total number of bytes successfully read. It returns -1 once the end of the input is encountered. |
3 | Int read (byte buffer [], int loc, int nBytes) | This method is used to read the 'nBytes' bytes from the buffer starting at a specified location, 'loc'. It returns the total number of bytes successfully read from the input. It returns -1 once the end of the input is encountered. |
4 | Int available () | This method returns the number of bytes that are available to read. |
5 | Void mark(int nBytes) | This method is used to mark the current position in the input stream until the specified nBytes are read. |
6 | Void reset () | This method is used to reset the input pointer to the previously set mark. |
7 | Long skip (long nBytes) | This method is used to skip the nBytes of the input stream and returns the total number of bytes that are skipped. |
8 | Void close () | This method is used to close the input source. If an attempt is made to read even after the closing, IO Exception is thrown by the method. |
Output Stream Class
The Output Stream is an abstract class that is used to write 8-bit bytes to the stream. It is the superclass of all the output stream classes.
it is inherited by various subclasses that are given in the following table.
SN | Class | Description |
1 | Buffered Output Stream | This class provides methods to write the bytes to the buffer. |
2 | Byte Array Output Stream | This class provides methods to write bytes to the byte array. |
3 | Data Output Stream | This class provides methods to write the java primitive data types. |
4 | File Output Stream | This class provides methods to write bytes to a file. |
5 | Filter Output Stream | This class provides methods to write to other output streams. |
6 | Object Output Stream | This class provides methods to write objects. |
7 | Piped Output Stream | It provides methods to write bytes to a piped output stream. |
8 | Print Stream | It provides methods to print Java primitive data types. |
The Output Stream class provides various methods to write bytes to the output streams. The methods are given in the following table.
SN | Method | Description |
1 | Void write (int i) | This method is used to write the specified single byte to the output stream. |
2 | Void write (byte buffer []) | It is used to write a byte array to the output stream. |
3 | Void write (bytes buffer [], int loc, int nBytes) | It is used to write nByte bytes to the output stream from the buffer starting at the specified location. |
4 | Void flush () | It is used to flush the output stream and writes the pending buffered bytes. |
5 | Void close () | It is used to close the output stream. However, if we try to close the already closed output stream, the IO Exception will be thrown by this method. |
Character Stream
Character Stream classes to overcome the limitations of Byte Stream classes, which can only handle the 8-bit bytes and is not compatible to work directly with the Unicode characters.
Character Stream classes are used to work with 16-bit Unicode characters. They can perform operations on characters, char arrays and Strings.
Character Stream classes are mainly used to read characters from the source and write them to the destination.
These classes are divided into two types of classes, I.e., Reader class and Writer class.
Reader Class
Reader class is used to read the 16-bit characters from the input stream.
All methods of the Reader class throw an IO Exception. The subclasses of the Reader class are given in the following table
SN | Class | Description |
1. | Buffered Reader | It provides methods to read characters from the buffer. |
2. | Char Array Reader | It provides methods to read characters from the char array. |
3. | File Reader | It provides methods to read characters from the file. |
4. | Filter Reader | It provides methods to read characters from the underlying character input stream. |
5 | Input Stream Reader | It provides methods to convert bytes to characters. |
6 | Piped Reader | It provides methods to read characters from the connected piped output stream. |
7 | String Reader | It provides methods to read characters from a string. |
The Reader class methods are given in the following table.
SN | Method | Description |
1 | Int read () | It returns the integral representation of the next character present in the input. It returns -1 if the end of the input is encountered. |
2 | Int read (char buffer []) | It is used to read from the specified buffer. It returns the total number of characters successfully read. It returns -1 if the end of the input is encountered. |
3 | Int read (char buffer [], int loc, int nChars) | It is used to read the specified nChars from the buffer at the specified location. It returns the total number of characters successfully read. |
4 | Void mark (int nchars) | It is used to mark the current position in the input stream until nChars characters are read. |
5 | Void reset () | It is used to reset the input pointer to the previous set mark. |
6 | Long skip (long nChars) | It is used to skip the specified nChars characters from the input stream and returns the number of characters skipped. |
7 | Boolean ready () | It returns a boolean value true if the next request of input is ready. Otherwise, it returns false. |
8 | Void close () | It is used to close the input stream. However, if the program attempts to access the input, it generates IO Exception. |
Writer Class
Writer class is used to write 16-bit Unicode characters to the output stream.
SN | Class | Description |
1 | Buffered Writer | It provides methods to write characters to the buffer. |
2 | File Writer | It provides methods to write characters to the file. |
3 | Char Array Writer | It provides methods to write the characters to the character array. |
4 | Output Stream Writer | It provides methods to convert from bytes to characters. |
5 | Piped Writer | It provides methods to write the characters to the piped output stream. |
6 | String Writer | It provides methods to write the characters to the string. |
The subclasses of the Writer class are used to write the characters onto the
Output stream. The subclasses of the Writer class are given in the below table.
To write the characters to the output stream, the Write class provides various methods given in the following table.
SN | Method | Description |
1 | Void write () | It is used to write the data to the output stream. |
2 | Void write (int i) | It is used to write a single character to the output stream. |
3 | Void write (char buffer []) | It is used to write the array of characters to the output stream. |
4 | Void write (char buffer [], int loc, int nChars) | It is used to write the nChars characters to the character array from the specified location. |
5 | Void close () | It is used to close the output stream. However, this generates the IO Exception if an attempt is made to write to the output stream after closing the stream. |
6 | Void flush () | It is used to flush the output stream and writes the waiting buffered characters. |
The File class is an abstract representation of file and directory pathname. A pathname can be either absolute or relative.
The File class have several methods for working with directories and files such as creating new directories or files, deleting and renaming directories or files, listing the contents of a directory etc.
Fields
Modifier | Type | Field | Description |
Static | String | PathSeparator | It is system-dependent path-separator character, represented as a stringfor convenience. |
Static | Char | PathSeparatorChar | It is system-dependent path-separator character. |
Static | String | Separator | It is system-dependent default name-separator character, represented as a string for convenience. |
Static | Char | SeparatorChar | It is system-dependent default name-separator character. |
Constructors
Constructor | Description |
File(File parent, String child) | It creates a new File instance from a parent abstract pathname and a child pathname string. |
File(String pathname) | It creates a new File instance by converting the given pathname string into an abstract pathname. |
File(String parent, String child) | It creates a new File instance from a parent pathname string and a child pathname string. |
File(URI uri) | It creates a new File instance by converting the given file: URI into an abstract pathname. |
Useful Methods
Modifier and Type | Method | Description |
Static File | CreateTempFile(String prefix, String suffix) | It creates an empty file in the default temporary-file directory, using the given prefix and suffix to generate its name. |
Boolean | CreateNewFile() | It atomically creates a new, empty file named by this abstract pathname if and only if a file with this name does not yet exist. |
Boolean | CanWrite() | It tests whether the application can modify the file denoted by this abstract pathname.String[] |
Boolean | CanExecute() | It tests whether the application can execute the file denoted by this abstract pathname. |
Boolean | CanRead() | It tests whether the application can read the file denoted by this abstract pathname. |
Boolean | IsAbsolute() | It tests whether this abstract pathname is absolute. |
Boolean | IsDirectory() | It tests whether the file denoted by this abstract pathname is a directory. |
Boolean | IsFile() | It tests whether the file denoted by this abstract pathname is a normal file. |
String | GetName() | It returns the name of the file or directory denoted by this abstract pathname. |
String | GetParent() | It returns the pathname string of this abstract pathname's parent, or null if this pathname does not name a parent directory. |
Path | ToPath() | It returns a java.nio.file.Path object constructed from the this abstract path. |
URI | ToURI() | It constructs a file: URI that represents this abstract pathname. |
File[] | ListFiles() | It returns an arrayof abstract pathnames denoting the files in the directory denoted by this abstract pathname |
Long | GetFreeSpace() | It returns the number of unallocated bytes in the partition named by this abstract path name. |
String[] | List(FilenameFilter filter) | It returns an array of strings naming the files and directories in the directory denoted by this abstract pathname that satisfy the specified filter. |
Boolean | Mkdir() | It creates the directory named by this abstract pathname. |
File Example 1
Import java.io.*;
Public class FileDemo {
Public static void main(String[] args) {
Try {
File file = new File("javaFile123.txt");
If (file.createNewFile()) {
System.out.println("New File is created!");
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
New File is created!
File Example 2
Import java.io.*;
Public class FileDemo2 {
Public static void main(String[] args) {
String path = "";
Boolean bool = false;
Try {
// createing new files
File file = new File("testFile1.txt");
File.createNewFile();
System.out.println(file);
// createing new canonical from file object
File file2 = file.getCanonicalFile();
// returns true if the file exists
System.out.println(file2);
Bool = file2.exists();
// returns absolute pathname
Path = file2.getAbsolutePath();
System.out.println(bool);
// if file exists
If (bool) {
// prints
System.out.print(path + " Exists? " + bool);
}
} catch (Exception e) {
// if any error occurs
e.printStackTrace();
}
}
}
Output:
TestFile1.txt
/home/Work/Project/File/testFile1.txt
True
/home/Work/Project/File/testFile1.txt Exists? true
Random Access File
This class is used for reading and writing to random access file. A random access file behaves like a large array of bytes. There is a cursor implied to the array called file pointer, by moving the cursor we do the read write operations. If end-of-file is reached before the desired number of byte has been read than EOF Exception is thrown. It is a type of IO Exception.
Constructor
Constructor | Description |
RandomAccessFile(File file, Stringmode) | Creates a random access file stream to read from, and optionally to write to, the file specified by the File argument. |
RandomAccessFile(String name, String mode) | Creates a random access file stream to read from, and optionally to write to, a file with the specified name. |
Method
Modifier and Type | Method | Method |
Void | Close() | It closes this random access file stream and releases any system resources associated with the stream. |
FileChannel | GetChannel() | It returns the unique FileChannelobject associated with this file. |
Int | ReadInt() | It reads a signed 32-bit integer from this file. |
String | ReadUTF() | It reads in a string from this file. |
Void | Seek(long pos) | It sets the file-pointer offset, measured from the beginning of this file, at which the next read or write occurs. |
Void | WriteDouble(double v) | It converts the double argument to a long using the doubleToLongBits method in class Double, and then writes that long value to the file as an eight-byte quantity, high byte first. |
Void | WriteFloat(float v) | It converts the float argument to an int using the floatToIntBits method in class Float, and then writes that int value to the file as a four-byte quantity, high byte first. |
Void | Write(int b) | It writes the specified byte to this file. |
Int | Read() | It reads a byte of data from this file. |
Long | Length() | It returns the length of this file. |
Void | Seek(long pos) | It sets the file-pointer offset, measured from the beginning of this file, at which the next read or write occurs. |
Example:
Import java.io.IOException;
Import java.io.RandomAccessFile;
Public class RandomAccessFileExample {
Static final String FILEPATH ="myFile.TXT";
Public static void main(String[] args) {
Try {
System.out.println(new String(readFromFile(FILEPATH, 0, 18)));
WriteToFile(FILEPATH, "I love my country and my people", 31);
} catch (IOException e) {
e.printStackTrace();
}
}
Private static byte[] readFromFile(String filePath, int position, int size)
Throws IOException {
RandomAccessFile file = new RandomAccessFile(filePath, "r");
File.seek(position);
Byte[] bytes = new byte[size];
File.read(bytes);
File.close();
Return bytes;
}
Private static void writeToFile(String filePath, String data, int position)
Throws IOException {
RandomAccessFile file = new RandomAccessFile(filePath, "rw");
File.seek(position);
File.write(data.getBytes());
File.close();
}
}
The myFile.TXT contains text "This class is used for reading and writing to random access file."
After running the program it will contains
This class is used for reading I love my country and my people.
Serialization in Java is a mechanism of writing the state of an object into a byte-stream. It is mainly used in Hibernate, RMI, JPA, EJB and JMS technologies.
The reverse operation of serialization is called deserialization where byte-stream is converted into an object.
The serialization and deserialization process is platform-independent, it means you can serialize an object on one platform and deserialize it on a different platform.
For serializing the object, we call the writeObject() method of Object Output Stream class, and for deserialization we call the readObject() method of Object Input Stream class.
Java.io.Serializable interface
Serializable is a marker interface (has no data member and method). It is used to "mark" Java classes so that the objects of these classes may get a certain capability. The Cloneable and Remote are also marker interfaces.
The Serializable interface must be implemented by the class whose object needs to be persisted.
The String class and all the wrapper classes implement the java.io.Serializable interface by default.
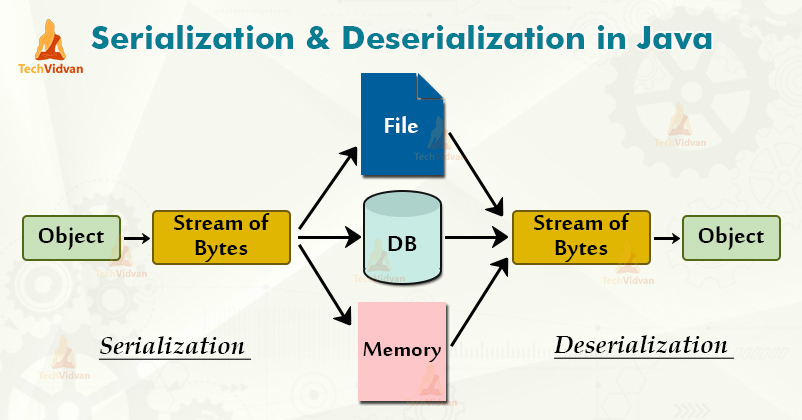
Object Output Stream class:
The Object Output Stream class is used to write primitive data types, and Java objects to an Output Stream.
Only objects that support the java.io.Serializable interface can be written to streams.
Constructor
1) public ObjectOutputStream(OutputStream out) throws IOException {} | It creates an ObjectOutputStream that writes to the specified OutputStream. |
Important Methods
Method | Description |
1) public final void writeObject(Object obj) throws IOException {} | It writes the specified object to the Object Output Stream. |
2) public void flush() throws IOException {} | It flushes the current output stream. |
3) public void close() throws IOException {} | It closes the current output stream. |
Object Input Stream class
An Object Input Stream deserializes objects and primitive data written using an Object Output Stream.
Constructor
1) public ObjectInputStream(InputStream in) throws IOException {} | It creates an Object Input Stream that reads from the specified Input Stream. |
Methods are
Method | Description |
1) public final Object readObject() throws IOException, ClassNotFoundException{} | It reads an object from the input stream. |
2) public void close() throws IOException {} | It closes Object Input Stream. |
The Collection in Java is a framework that provides an architecture to store and manipulate the group of objects.
Java Collections can achieve all the operations that you perform on a data such as searching, sorting, insertion, manipulation, and deletion.
Java Collection means a single unit of objects. Java Collection framework provides many interfaces (Set, List, Queue, Deque) and classes (Array List, Vector, LinkedList, Priority Queue, HashSet, Linked Hash Set, Tree Set).
Introduction
A Collection represents a single unit of objects, i.e., a group.
Framework in Java
- It provides readymade architecture.
- It represents a set of classes and interfaces.
- It is optional.
What is Collection framework
The Collection framework represents a unified architecture for storing and manipulating a group of objects. It has:
- Interfaces and its implementations, i.e., classes
- Algorithm
Hierarchy of Collection Framework
Let us see the hierarchy of Collection framework. The java.util package contains all the classes and interfaces for the Collection framework.
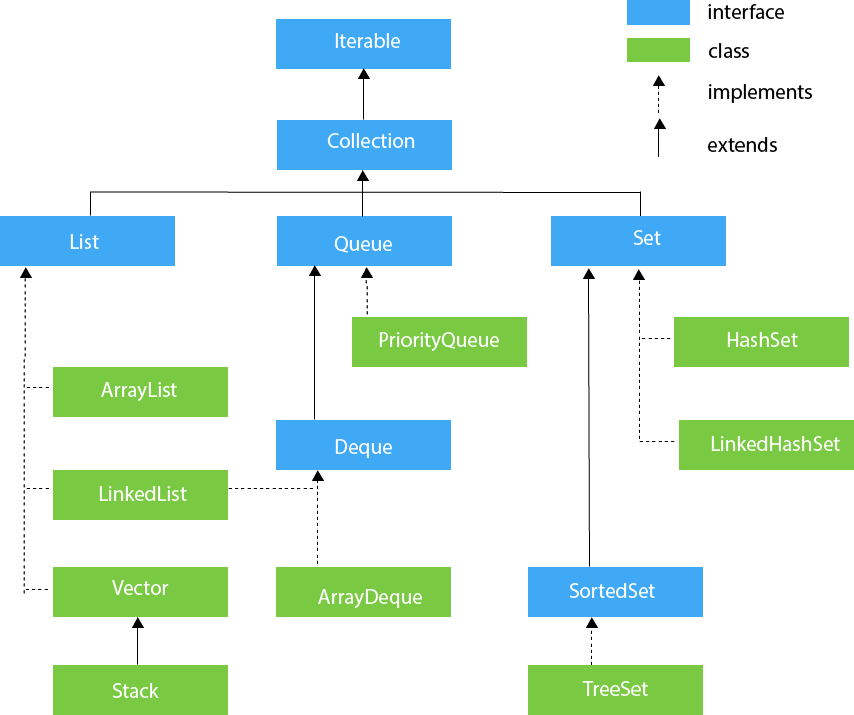
Methods of Collection interface
There are many methods declared in the Collection interface. They are as follows:
Method | Description |
Public boolean add(E e) | It is used to insert an element in this collection. |
Public boolean addAll(Collection<? extends E> c) | It is used to insert the specified collection elements in the invoking collection. |
Public boolean remove(Object element) | It is used to delete an element from the collection. |
Public boolean removeAll(Collection<?> c) | It is used to delete all the elements of the specified collection from the invoking collection. |
Default boolean removeIf(Predicate<? super E> filter) | It is used to delete all the elements of the collection that satisfy the specified predicate. |
Public boolean retainAll(Collection<?> c) | It is used to delete all the elements of invoking collection except the specified collection. |
Public int size() | It returns the total number of elements in the collection. |
Public void clear() | It removes the total number of elements from the collection. |
Public boolean contains(Object element) | It is used to search an element. |
Public boolean containsAll(Collection<?> c) | It is used to search the specified collection in the collection. |
Public Iterator iterator() | It returns an iterator. |
Public Object[] toArray() | It converts collection into array. |
Public <T> T[] toArray(T[] a) | It converts collection into array. Here, the runtime type of the returned array is that of the specified array. |
Public boolean isEmpty() | It checks if collection is empty. |
Default Stream<E> parallelStream() | It returns a possibly parallel Stream with the collection as its source. |
Default Stream<E> stream() | It returns a sequential Stream with the collection as its source. |
Default Spliterator<E> spliterator() | It generates a Spliterator over the specified elements in the collection. |
Public boolean equals(Object element) | It matches two collections. |
Public int hashCode() | It returns the hash code number of the collection. |
The java.util.Interfaces contains the collections framework, legacy collection classes, event model, date and time facilities, internationalization, and miscellaneous utility classes (a string tokenizer, a random-number generator, and a bit array)
- Collection<E>: This is the root interface in the collection hierarchy.
- Comparator<T>: It is a comparison function, which imposes a total ordering on some collection of objects
- Set<E>: This is a collection that contains no duplicate elements.
- Sorted Map<K, V>: This is a Map that further provides a total ordering on its keys
- Sorted Set<E>: This is a Set that further provides a total ordering on its elements.
- RandomAccess(): This is the Marker interface used by List implementations to indicate that they support fast (generally constant time) random access.
- Map. Entry<K, V>: This is a map entry (key-value pair).
- Queue<E>: This is a collection designed for holding elements prior to processing.
- Observer: This is a class can implement the Observer interface when it wants to be informed of changes in observable objects.
- Event Listener: This is a tagging interface that all event listener interfaces must extend.
- Map<K, V>: This is an object that maps keys to values.
- Deque<E>: This is a linear collection that supports element insertion and removal at both ends.
- List<E>: This is an ordered collection (also known as a sequence).
- List Iterator<E>: This is an iterator for lists that allows the programmer to traverse the list in either direction, modify the list during iteration, and obtain the iterator's current position in the list.
Set Interface in Java is present in java.util package.
It extends the Collection interface.
It represents the unordered set of elements which doesn't allow us to store the duplicate items. We can store at most one null value in Set. Set is implemented by HashSet, Linked Hash Set, and TreeSet.
Set can be instantiated as:
Set<data-type> s1 = new HashSet<data-type>();
Set<data-type> s2 = new LinkedHashSet<data-type>();
Set<data-type> s3 = new TreeSet<data-type>();
HashSet
HashSet class implements Set Interface. It represents the collection that uses a hash table for storage. Hashing is used to store the elements in the HashSet. It contains unique items.
Consider the following example.
Import java.util.*;
Public class TestJavaCollection7{
Public static void main(String args[]){
//Creating HashSet and adding elements
HashSet<String> set=new HashSet<String>();
Set.add("Ravi");
Set.add("Vijay");
Set.add("Ravi");
Set.add("Ajay");
//Traversing elements
Iterator<String> itr=set.iterator();
While(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output:
Vijay
Ravi
Ajay
Linked Hash Set
Linked Hash Set class represents the LinkedList implementation of Set Interface. It extends the HashSet class and implements Set interface. Like HashSet, It also contains unique elements. It maintains the insertion order and permits null elements.
Consider the following example
Import java.util.*;
Public class TestJavaCollection8{
Public static void main(String args[]){
LinkedHashSet<String> set=new LinkedHashSet<String>();
Set.add("Ravi");
Set.add("Vijay");
Set.add("Ravi");
Set.add("Ajay");
Iterator<String> itr=set.iterator();
While(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output:
Ravi
Vijay
Ajay
TreeSet
Java TreeSet class implements the Set interface that uses a tree for storage. Like HashSet, TreeSet also contains unique elements. However, the access and retrieval time of TreeSet is quite fast. The elements in TreeSet stored in ascending order.
Consider the following example:
Import java.util.*;
Public class TestJavaCollection9{
Public static void main(String args[]){
//Creating and adding elements
TreeSet<String> set=new TreeSet<String>();
Set.add("Ravi");
Set.add("Vijay");
Set.add("Ravi");
Set.add("Ajay");
//traversing elements
Iterator<String> itr=set.iterator();
While(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output:
Ajay
Ravi
Vijay
Map Interface
A map contains values on the basis of key, i.e. key and value pair. Each key and value pair is known as an entry. A Map contains unique keys.
A Map is useful if you have to search, update or delete elements on the basis of a key.
Java Map Hierarchy
There are two interfaces for implementing Map in java: Map and Sorted Map, and three classes: HashMap, Linked Hash Map, and Tree Map. The hierarchy of Java Map is given below:

A Map doesn't allow duplicate keys, but you can have duplicate values. HashMap and Linked Hash Map allow null keys and values, but Tree Map doesn't allow any null key or value.
A Map can't be traversed, so you need to convert it into Set using keySet() or entrySet() method.
Class | Description |
HashMap | HashMap is the implementation of Map, but it doesn't maintain any order. |
LinkedHashMap | LinkedHashMap is the implementation of Map. It inherits HashMap class. It maintains insertion order. |
TreeMap | TreeMap is the implementation of Map and SortedMap. It maintains ascending order. |
Useful methods of Map interface
Method | Description |
V put(Object key, Object value) | It is used to insert an entry in the map. |
Void putAll(Map map) | It is used to insert the specified map in the map. |
V putIfAbsent(K key, V value) | It inserts the specified value with the specified key in the map only if it is not already specified. |
V remove(Object key) | It is used to delete an entry for the specified key. |
Boolean remove(Object key, Object value) | It removes the specified values with the associated specified keys from the map. |
Set keySet() | It returns the Set view containing all the keys. |
Set<Map.Entry<K,V>> entrySet() | It returns the Set view containing all the keys and values. |
Void clear() | It is used to reset the map. |
V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a mapping for the specified key and its current mapped value (or null if there is no current mapping). |
V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) | It is used to compute its value using the given mapping function, if the specified key is not already associated with a value (or is mapped to null), and enters it into this map unless null. |
V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) | It is used to compute a new mapping given the key and its current mapped value if the value for the specified key is present and non-null. |
Boolean containsValue(Object value) | This method returns true if some value equal to the value exists within the map, else return false. |
Boolean containsKey(Object key) | This method returns true if some key equal to the key exists within the map, else return false. |
Boolean equals(Object o) | It is used to compare the specified Object with the Map. |
Void forEach(BiConsumer<? super K,? super V> action) | It performs the given action for each entry in the map until all entries have been processed or the action throws an exception. |
V get(Object key) | This method returns the object that contains the value associated with the key. |
V getOrDefault(Object key, V defaultValue) | It returns the value to which the specified key is mapped, or defaultValue if the map contains no mapping for the key. |
Int hashCode() | It returns the hash code value for the Map |
Boolean isEmpty() | This method returns true if the map is empty; returns false if it contains at least one key. |
V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) | If the specified key is not already associated with a value or is associated with null, associates it with the given non-null value. |
Map Example
Import java.util.*;
Class MapExample2{
Public static void main(String args[]){
Map<Integer,String> map=new HashMap<Integer,String>();
Map.put(100,"Amit");
Map.put(101,"Vijay");
Map.put(102,"Rahul");
//Elements can traverse in any order
For(Map.Entry m:map.entrySet()){
System.out.println(m.getKey()+" "+m.getValue());
}
}
}
Output:
102 Rahul
100 Amit
101 Vijay
Map Example: comparingByKey()
Import java.util.*;
Class MapExample3{
Public static void main(String args[]){
Map<Integer,String> map=new HashMap<Integer,String>();
Map.put(100,"Amit");
Map.put(101,"Vijay");
Map.put(102,"Rahul");
//Returns a Set view of the mappings contained in this map
Map.entrySet()
//Returns a sequential Stream with this collection as its source
.stream()
//Sorted according to the provided Comparator
.sorted(Map.Entry.comparingByKey())
//Performs an action for each element of this stream
.forEach(System.out::println);
}
}
Output:
100=Amit
101=Vijay
102=Rahul
Map Example: comparingByKey() in Descending Order
Import java.util.*;
Class MapExample4{
Public static void main(String args[]){
Map<Integer,String> map=new HashMap<Integer,String>(); map.put(100,"Amit");
Map.put(101,"Vijay");
Map.put(102,"Rahul");
//Returns a Set view of the mappings contained in this map
Map.entrySet()
//Returns a sequential Stream with this collection as its source
.stream()
//Sorted according to the provided Comparator
.sorted(Map.Entry.comparingByKey(Comparator.reverseOrder()))
//Performs an action for each element of this stream
.forEach(System.out::println);
}
}
Output:
102=Rahul
101=Vijay
100=Amit
List in Java provides the facility to maintain the ordered collection.
It contains the index-based methods to insert, update, delete and search the elements.
It can have the duplicate elements also. We can also store the null elements in the list.
The implementation classes of List interface are Array List, Linked List, Stack and Vector.
Declaration:
Public interface List<E> extends Collection<E>
Java List Methods:
Method | Description | |
Void add(int index, E element) | It is used to insert the specified element at the specified position in a list. | |
Boolean add(E e) | It is used to append the specified element at the end of a list. | |
Boolean addAll(Collection<? extends E> c) | It is used to append all of the elements in the specified collection to the end of a list. | |
Boolean addAll(int index, Collection<? extends E> c) | It is used to append all the elements in the specified collection, starting at the specified position of the list. | |
Void clear() | It is used to remove all of the elements from this list. | |
Boolean equals(Object o) | It is used to compare the specified object with the elements of a list. | |
Int hashcode() | It is used to return the hash code value for a list. | |
E get(int index) | It is used to fetch the element from the particular position of the list. | |
Boolean isEmpty() | It returns true if the list is empty, otherwise false. | |
Int lastIndexOf(Object o) | It is used to return the index in this list of the last occurrence of the specified element, or -1 if the list does not contain this element. | |
Object[] toArray() | It is used to return an array containing all of the elements in this list in the correct order. | |
<T> T[] toArray(T[] a) | It is used to return an array containing all of the elements in this list in the correct order. | |
Boolean contains(Object o) | It returns true if the list contains the specified element | |
Boolean containsAll(Collection<?> c) | It returns true if the list contains all the specified element | |
Int indexOf(Object o) | It is used to return the index in this list of the first occurrence of the specified element, or -1 if the List does not contain this element. | |
E remove(int index) | It is used to remove the element present at the specified position in the list. |
|
Boolean remove(Object o) | It is used to remove the first occurrence of the specified element. |
|
Boolean removeAll(Collection<?> c) | It is used to remove all the elements from the list. |
|
Void replaceAll(UnaryOperator<E> operator) | It is used to replace all the elements from the list with the specified element. |
|
Void retainAll(Collection<?> c) | It is used to retain all the elements in the list that are present in the specified collection. |
|
E set(int index, E element) | It is used to replace the specified element in the list, present at the specified position. |
|
Void sort(Comparator<? super E> c) | It is used to sort the elements of the list on the basis of specified comparator. |
|
Spliterator<E> spliterator() | It is used to create spliterator over the elements in a list. |
|
List<E> subList(int fromIndex, int toIndex) | It is used to fetch all the elements lies within the given range. |
|
Int size() | It is used to return the number of elements present in the list. |
|
Example:
- Creating a List of type String using ArrayList
List<String> list=new ArrayList<String>();
2. Creating a List of type Integer using ArrayList
List<Integer> list=new ArrayList<Integer>();
3. Creating a List of type Book using ArrayList
List<Book> list=new ArrayList<Book>();
4. Creating a List of type String using LinkedList
List<String> list=new LinkedList<String>();
List Example
Let's see a simple example of List where we are using the Array List class as the implementation.
Import java.util.*;
Public class ListExample1{
Public static void main(String args[]){
//Creating a List
List<String> list=new ArrayList<String>();
//Adding elements in the List
List.add("Mango");
List.add("Apple");
List.add("Banana");
List.add("Grapes");
//Iterating the List element using for-each loop
For(String fruit:list)
System.out.println(fruit);
}
}
Output:
Mango
Apple
Banana
Grapes
How to convert Array to List
We can convert the Array to List by traversing the array and adding the element in list one by one using list.add() method. Let's see a simple example to convert array elements into List.
Import java.util.*;
Public class ArrayToListExample{
Public static void main(String args[]){
//Creating Array
String[] array={"Java","Python","PHP","C++"};
System.out.println("Printing Array: "+Arrays.toString(array));
//Converting Array to List
List<String> list=new ArrayList<String>();
For(String lang:array){
List.add(lang);
}
System.out.println("Printing List: "+list);
}
}
Output:
Printing Array: [Java, Python, PHP, C++]
Printing List: [Java, Python, PHP, C++]
How to convert List to Array
We can convert the List to Array by calling the list.toArray() method. Let's see a simple example to convert list elements into array.
Import java.util.*;
Public class ListToArrayExample{
Public static void main(String args[]){
List<String> fruitList = new ArrayList<>();
FruitList.add("Mango");
FruitList.add("Banana");
FruitList.add("Apple");
FruitList.add("Strawberry");
//Converting ArrayList to Array
String[] array = fruitList.toArray(new String[fruitList.size()]);
System.out.println("Printing Array: "+Arrays.toString(array));
System.out.println("Printing List: "+fruitList);
}
}
Output:
Printing Array: [Mango, Banana, Apple, Strawberry]
Printing List: [Mango, Banana, Apple, Strawberry]
Difference between List, Set, and Map interface:
The List, Set and Map interfaces are significant members of the Java
- Ordering: -
- List: - represents an ordered sequence in Java whose elements are accessible by index.
- Set: - represents a distinct collection of elements in Java which can be either ordered or unordered, depending on the implementation.
Example: a) HashSet implementation is unordered
b) TreeSet implementation is ordered by natural order or provided comparator.
3. Map: - represents the mapping of the key to values in Java. The ordering in Map is also implementation-specific
Example: Tree Map class is ordered while the HashMap class is not.
B. Duplicates:
- List: List can have duplicate elements
- Set: A set contain only distinct elements
- Map: Map doesn’t permit duplicate keys, i.e., each key can map to at most one value.
C. Null Values:
- List: It allows any number of null values
- Set: a set contains at most one null element.
- Map: Map typically allows null as a key and value, but some implementations prohibit null keys and values.
D. Implementing Classes:
- List: The most popular implementing classes of the List interface are Array List and LinkedList
- Set: set interfaces included HashSet, TreeSet, Linked Hash Set
- Map: Map interfaces offers Tree Map, Linked Hash Map, Tree Map
E. When to use List, Map and Set:
- List: List can be used when the insertion order of an elements need to be maintained.
- Set: We can use set if we need to maintain a collection that contain no duplicates.
- Map: when data is key-value pairs and need fast retrieval of value based on some key.
Getter And Setter classes in java
Getter method:
A method which is used to retrieve/get the value of a variable or return the value of the private member variable is called getter method in Java.
This method is also known as accessor method. For every private variable, we should create a getter method.
Depending on the access level giving to the variable, we can set the access modifier of its getter method.
If we declare instance variables as private, we will have to add public getter methods for each one.
The signature of a getter method in Java is as follows:
Public returnType getPropertyName()
If the returnType is boolean, the getter method should defined
Public boolean isPropertyName()
A method which is used for updating or setting the value of a variable is called setter method in Java. This method is also known as mutator method.
we can modify the value of a variable.
The signature of setter method in Java is as follows:
Public void setPropertyName(dataType propertyValue)
Examples:
- If the variable is the type of int, you will declare like this:
Private int number;
Then appropriate getter and setter will be
Public getNumber(); { }
Public void setNumber(int number);
Similarly,
2. If the variable is a type of boolean then
Private boolean rich;
Public getRich() { }
Public setRich() { }
An applet is a Java program that can be embedded into a web page. It runs inside the web browser and works at client side. An applet is embedded in an HTML page using the APPLET or OBJECT tag and hosted on a web server.
Important points:
- All applets are sub-classes (either directly or indirectly) of java.applet.Applet class.
- Applets are not stand-alone programs. Instead, they run within either a web browser or an applet viewer. JDK provides a standard applet viewer tool called applet viewer.
- In general, execution of an applet does not begin at main() method.
- Output of an applet window is not performed by System.out.println(). Rather it is handled with various AWT methods, such as drawString().
The life cycle of a Java Applet has five main steps.
- Initialize the Applet
- Start the Applet
- Paint Applet
- Stop the Applet
- Destroy the Applet
1. Initialize the Applet
In this step, the Applet is initialized public void init() is the method used to initialize an Applet. It is only invoked once
2. Start the Applet:
In this step the Applet is start with the help of public void start()
Method.The start()method is invoked after calling the init() method.
3. Paint Applet:
Public void paint(Graphics g) method is used to paint the Applet.
The method provides graphics class object that can be used to draw shapes as oval, rectangle, arc etc.
4. Stop Applet:
The Applet is stooped with the help of public void stop()method.
The method is invoked when the applet is stopped of the browser is minimized.
5. Destroy Applet:
This is the Final step in the Applet life cycle. The Applet initalized is destroyed in this step. It is only invoked once and the method used to invoke, is public void destory()
AWT (Abstract Window Toolkit) is an API to develop Graphical User Interface (GUI) or windows-based applications in Java.
Java AWT components are platform-dependent i.e. components are displayed according to the view of operating system. AWT is heavy weight i.e. its components are using the resources of underlying operating system (OS).
The java.awt package provides classes for AWT API such as Text Field, Label, Text Area, Radio Button, Check Box, Choice, List etc.
The AWT tutorial will help the user to understand Java GUI programming in simple and easy steps.
an AWT application will look like a windows application in Windows OS whereas it will look like a Mac application in the MAC OS.
AWT Hierarchy
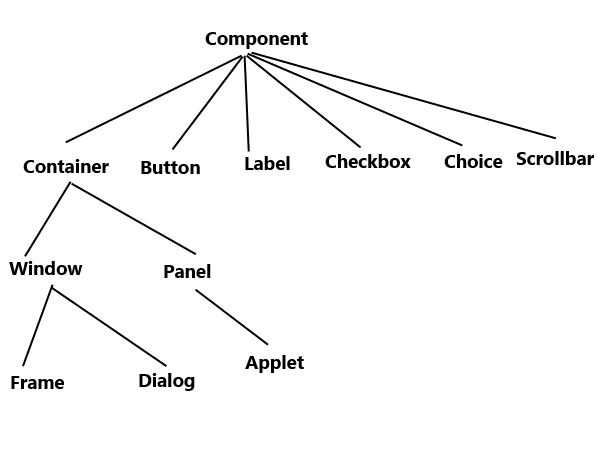
Introduction to GUI
GUI stands for Graphical User Interface. We interact with the screen in two ways one is console-based and the second one is a graphical user interface.
In console based we interact with help of text command eg. Command prompt whereas in GUI we interact with the help of graphical components which is also known as visual indicators eg. Calculator.
For graphical user interface there are two types of application programming interface (API’S) and they are AWT (abstract windowing toolkit) and swing.
Creating Hello World applet:
Let’s begin with the HelloWorld applet:
/ A Hello World Applet
// Save file as HelloWorld.java
Import java.applet.Applet;
Import java.awt.Graphics;
// HelloWorld class extends Applet
Public class HelloWorld extends Applet
{
// Overriding paint() method
@Override
Public void paint(Graphics g)
{
g.drawString("Hello World", 20, 20);
}
}
How to run
1. The java program begins with two import statements. The first import statement imports the Applet class from applet package. Every AWT-based applet that you create must be a subclass (either directly or indirectly) of Applet class. The second statement import the Graphics class from AWT package.
2. The next line in the program declares the class HelloWorld. This class must be declared as public because it will be accessed by code that is outside the program. Inside HelloWorld, paint() is declared. This method is defined by the AWT and must be overridden by the applet.
3. Inside paint() is a call to drawString( ), which is a member of the Graphics class. This method outputs a string beginning at the specified X, Y location. It has the following general form:
Void drawString(String message, int x, int y)
Here, message is the string to be output beginning at x, y. In a Java window, the upper-left corner is location 0,0. The call to drawString( ) in the applet causes the message “Hello World” to be displayed beginning at location 20,20.
The applet does not have a main() method. Unlike Java programs, applets do not begin execution at main(). In fact, most applets don’t even have a main( ) method. Instead, an applet begins execution when the name of its class is passed to an applet viewer or to a network browser.
Running the HelloWorld Applet:
After you enter the source code for HelloWorld.java, compile in the same way that you have been compiling java programs (using javac command). However, running HelloWorld with the java command will generate an error because it is not an application.
There are two standard ways in which you can run an applet:
1. Executing the applet within a Java-compatible web browser.
To execute an applet in a web browser we have to write a short HTML text file that contains a tag that loads the applet. We can use APPLET or OBJECT tag for this purpose. Using APPLET, here is the HTML file that executes HelloWorld:
<applet code="HelloWorld" width=200 height=60>
</applet>
The APPLET tag contains several other options. After you create this html file, you can use it to execute the applet.
Using an applet viewer, such as the standard tool, applet-viewer. An applet viewer executes your applet in a window. This is generally the fastest and easiest way to test your applet.
To execute HelloWorld with an applet viewer, you may also execute the HTML file. For example, HTML file is RunHelloWorld.html, then the following command line will run HelloWorld:
Applet viewer RunHelloWorld.html
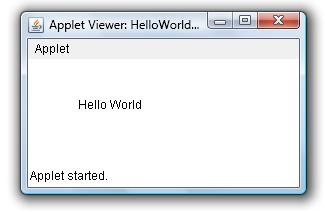
Components
All the elements like the button, text fields, scroll bars, etc. are called components. In Java AWT, there are classes for each component as shown in above diagram. In order to place every component in a particular position on a screen, we need to add them to a container.
Container
The Container is a component in AWT that can contain another components like buttons, text fields, labels etc. The classes that extends Container class are known as container such as Frame, Dialog and Panel.
i.e Container itself is a components, therefore we can add a container inside container
There are four types of containers in Java AWT:
- Window
- Panel
- Frame
- Dialog
Window
The window is the container that have no borders and menu bars. You must use frame, dialog or another window for creating a window. We need to create an instance of Window class to create this container.
Panel
The Panel is the container that doesn't contain title bar, border or menu bar. It is generic container for holding the components. It can have other components like button, text field etc. An instance of Panel class creates a container, in which we can add components.
Frame
The Frame is the container that contain title bar and border and can have menu bars. It can have other components like button, text field, scrollbar etc. Frame is most widely used container while developing an AWT application.
Methods of Component Class
Method | Description |
Public void add(Component c) | Inserts a component on this component. |
Public void setSize(int width,int height) | Sets the size (width and height) of the component. |
Public void setLayout(LayoutManager m) | Defines the layout manager for the component. |
Public void setVisible(boolean status) | Changes the visibility of the component, by default false. |
Hierarchy of components
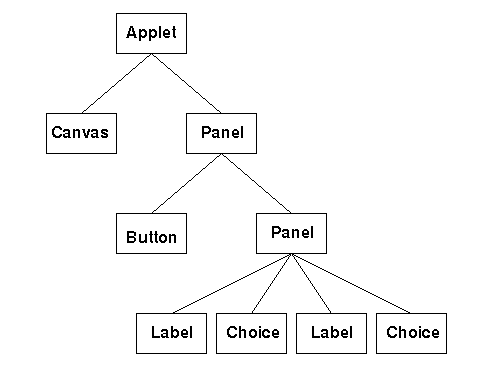
Method | Description |
Public void add(Component c) | Inserts a component on this component. |
Public void setSize(int width, int height) | Sets the size (width and height) of the component. |
Public void setLayout(LayoutManager m) | Defines the layout manager for the component. |
Public void setVisible(boolean status) | Changes the visibility of the component, by default false. |
Event handling is elemental to Java programming because it is used to make event driven programs eg Applets, GUI based windows application, Web Application.
Two Event Handling Mechanisms in Java
- Original Version of Java (1.0)
- Modern Versions of Java, beginning with Java version 1.1
Event handling mechanisms have been changed quite between the original version of Java (1.0) and all following versions of Java, beginning with version 1.1.
The modern technique to handling events is based on the delegation event model
What is an Event?
Change in the state of an object is known as event i.e. event describes the change in state of source.
What is Event Handling?
Event Handling is the mechanism that controls the event and decides what should happen if an event occurs. This mechanism has the code which is known as event handler that is executed when an event occurs. Java Uses the Delegation Event Model to handle the events. This model defines the standard mechanism to generate and handle the events.
The Delegation Event Model has the following key participants namely:
- Source - The source is an object on which event occurs.
- Source is responsible for delivering information of the occurred event to its handler. Java provides classes for source object.
- Listener - It is also known as event handler. Listener is responsible for generating the response to an event. From java execution point of view, the listener is also an object. The listener waits until it receives an event. Once the event is received , the listener process the event and then returns.
Delegation Event Model
Writing event driven program is a two step method
- Implement the suitable interface in the listener so that it can receive the type of event desired.
- Implement code to register and unregister (if necessary) the listener as a recipient for the event notifications
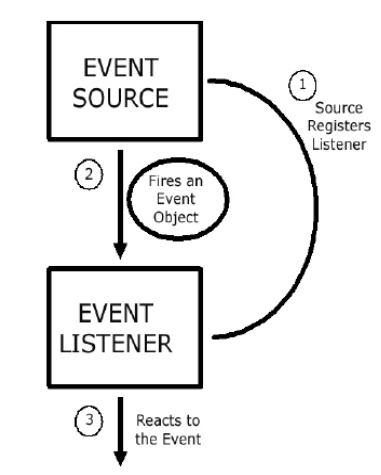
Event and Listener (Java Event Handling)
Changing the state of an object is known as an event. For example, click on button, dragging mouse etc. The java.awt.event package provides many event classes and Listener interfaces for event handling.
Java Event classes and Listener interfaces
Event Classes | Listener Interfaces |
ActionEvent | ActionListener |
MouseEvent | MouseListener and MouseMotionListener |
MouseWheelEvent | MouseWheelListener |
KeyEvent | KeyListener |
ItemEvent | ItemListener |
TextEvent | TextListener |
AdjustmentEvent | AdjustmentListener |
WindowEvent | WindowListener |
ComponentEvent | ComponentListener |
ContainerEvent | ContainerListener |
FocusEvent | FocusListener |
We can put the event handling code into one of the following places:
- Within class
- Other class
- Anonymous class
Following steps are required to perform event handling:
- Register the component with the Listener
- Button: public void addActionListener(ActionListener a){}
- Menu Item: public void addActionListener(ActionListener a){}
- Text Field: public void addActionListener(ActionListener a){}
Public void addTextListener(TextListener a){}
- Text Area: public void addTextListener(TextListener a){}
- Checkbox: public void addItemListener(ItemListener a){}
- Choice: public void addItemListener(ItemListener a){}
- List: public void addActionListener(ActionListener a){}
Public void addItemListener(ItemListener a){}
Java event handling by implementing ActionListener
Import java.awt.*;
Import java.awt.event.*;
Class AEvent extends Frame implements ActionListener{
TextField tf;
AEvent(){
//create components
Tf=new TextField();
Tf.setBounds(60,50,170,20);
Button b=new Button("click me");
b.setBounds(100,120,80,30);
//register listener
b.addActionListener(this);//passing current instance
//add components and set size, layout and visibility
Add(b);add(tf);
SetSize(300,300);
SetLayout(null);
SetVisible(true);
}
Public void actionPerformed(ActionEvent e){
Tf.setText("Welcome");
}
Public static void main(String args[]){
New AEvent();
}
}
8.2M
119
SQL CREATE TABLE
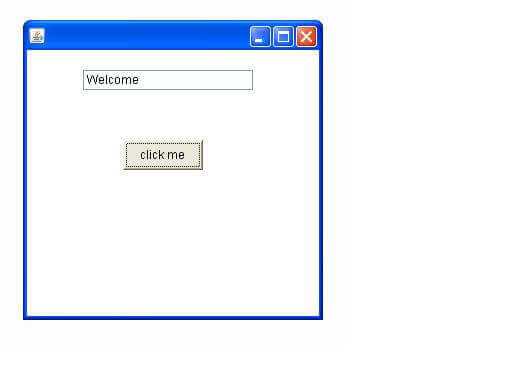
Public void setBounds(int xaxis, int yaxis, int width, int height); have been used in the above example that sets the position of the component it may be button, text field etc.
- Java adapter classes provide the default implementation of listener interfaces.
- The adapter classes are found in java.awt.event, java.awt.dnd and javax.swing.event packages.
Java.awt.event Adapter classes
Adapter class | Listener interface |
WindowAdapter | WindowListener |
KeyAdapter | KeyListener |
MouseAdapter | MouseListener |
MouseMotionAdapter | MouseMotionListener |
FocusAdapter | FocusListener |
ComponentAdapter | ComponentListener |
ContainerAdapter | ContainerListener |
HierarchyBoundsAdapter | HierarchyBoundsListener |
Java.awt.dnd Adapter classes
Adapter class | Listener interface |
DragSourceAdapter | DragSourceListener |
DragTargetAdapter | DragTargetListener |
Javax.swing.event Adapter classes
Adapter class | Listener interface |
MouseInputAdapter | MouseInputListener |
InternalFrameAdapter | InternalFrameListener |
References:
1. Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
2. CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
3. JAVA Complete Reference (9th Edition) Herbait Schelidt.