UNIT 3
Arrays
One dimensional Array
The variable allows us to store a single value at a time, what if we want to store roll no. Of 100 students? For this task, we have to declare 100 variables, then assign values to each of them. What if there are 10000 students or more? As you can see declaring that many variables for a single entity (i.e student) is not a good idea. In a situation like these arrays provide a better way to store data.
What is an Array?
An array is a collection of one or more values of the same type. Each value is called an element of the array. The elements of the array share the same variable name but each element has its own unique index number (also known as a subscript). An array can be of any type, For example: int, float, char etc. If an array is of type int then it's elements must be of type int only.
To store roll no. Of 100 students, we have to declare an array of size 100 i.e roll_no[100]. Here size of the array is 100 , so it is capable of storing 100 values. In C, index or subscript starts from 0, so roll_no[0] is the first element, roll_no[1] is the second element and so on. Note that the last element of the array will be at roll_no[99] not at roll_no[100] because the index starts at 0.
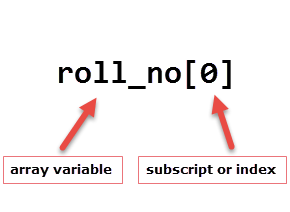
Arrays can be single or multidimensional. The number of subscript or index determines the dimensions of the array. An array of one dimension is known as a one-dimensional array or 1-D array, while an array of two dimensions is known as a two-dimensional array or 2-D array.
Let's start with a one-dimensional array.
One-dimensional array
Conceptually you can think of a one-dimensional array as a row, where elements are stored one after another.
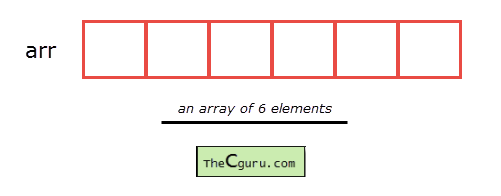
Syntax: datatype array_name[size];
Datatype: It denotes the type of the elements in the array.
Array_name: Name of the array. It must be a valid identifier.
Size: Number of elements an array can hold. Here are some example of array declarations:
1 2 3 | Int num[100]; Float temp[20]; Char ch[50]; |
Num is an array of type int, which can only store 100 elements of type int.
temp is an array of type float, which can only store 20 elements of type float.
ch is an array of type char, which can only store 50 elements of type char.
Note: When an array is declared it contains garbage values.
The individual elements in the array:
1 2 3 | Num[0], num[1], num[2], ....., num[99] Temp[0], temp[1], temp[2], ....., temp[19] Ch[0], ch[1], ch[2], ....., ch[49] |
We can also use variables and symbolic constants to specify the size of the array.
1 2 3 4 5 6 7 8 9 10 | #define SIZE 10
Int main() { Int size = 10;
Int my_arr1[SIZE]; // ok Int my_arr2[size]; // not allowed until C99 // ... } |
Note: Until C99 standard, we were not allowed to use variables to specify the size of the array. If you are using a compiler which supports C99 standard, the above code would compile successfully. However, If you're using an older version of C compiler like Turbo C++, then you will get an error.
The use of symbolic constants makes the program maintainable, because later if you want to change the size of the array you need to modify it at once place only i.e in the #define directive.
Accessing elements of an array
The elements of an array can be accessed by specifying array name followed by subscript or index inside square brackets (i.e []). Array subscript or index starts at 0. If the size of an array is 10 then the first element is at index 0, while the last element is at index 9. The first valid subscript (i.e 0) is known as the lower bound, while last valid subscript is known as the upper bound.
Int my_arr[5];
Then elements of this array are;
First element – my_arr[0]
Second element – my_arr[1]
Third element – my_arr[2]
Fourth element – my_arr[3]
Fifth element – my_arr[4]
Array subscript or index can be any expression that yields an integer value. For example:
1 2 3 4 | Int i = 0, j = 2; My_arr[i]; // 1st element My_arr[i+1]; // 2nd element My_arr[i+j]; // 3rd element |
In the array my_arr, the last element is at my_arr[4], What if you try to access elements beyond the last valid index of the array?
1 2 3 | Printf("%d", my_arr[5]); // 6th element Printf("%d", my_arr[10]); // 11th element Printf("%d", my_arr[-1]); // element just before 0 |
Sure indexes 5, 10 and -1 are not valid but C compiler will not show any error message instead some garbage value will be printed. The C language doesn't check bounds of the array. It is the responsibility of the programmer to check array bounds whenever required.
Processing 1-D arrays
The following program uses for loop to take input and print elements of a 1-D array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include<stdio.h>
Int main() { Int arr[5], i;
For(i = 0; i < 5; i++) { Printf("Enter a[%d]: ", i); Scanf("%d", &arr[i]); }
Printf("\nPrinting elements of the array: \n\n");
For(i = 0; i < 5; i++) { Printf("%d ", arr[i]); }
// signal to operating system program ran fine Return 0; } |
Expected Output:
1 2 3 4 5 6 7 8 9 | Enter a[0]: 11 Enter a[1]: 22 Enter a[2]: 34 Enter a[3]: 4 Enter a[4]: 34
Printing elements of the array:
11 22 34 4 34 |
How it works:
In Line 5, we have declared an array of 5 integers and variable i of type int. Then a for loop is used to enter five elements into an array. In scanf() we have used & operator (also known as the address of operator) on element arr[i] of an array, just like we had done with variables of type int, float, char etc. Line 13 prints "Printing elements of the array" to the console. The second for loop prints all the elements of an array one by one.
The following program prints the sum of elements of an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include<stdio.h>
Int main() { Int arr[5], i, s = 0;
For(i = 0; i < 5; i++) { Printf("Enter a[%d]: ", i); Scanf("%d", &arr[i]); }
For(i = 0; i < 5; i++) { s += arr[i]; }
Printf("\nSum of elements = %d ", s);
// signal to operating system program ran fine Return 0; } |
Expected Output:
1 2 3 4 5 6 7 | Enter a[0]: 22 Enter a[1]: 33 Enter a[2]: 56 Enter a[3]: 73 Enter a[4]: 23
Sum of elements = 207 |
How it works: The first for loop asks the user to enter five elements into the array. The second for loop reads all the elements of an array one by one and accumulate the sum of all the elements in the variable s. Note that it is necessary to initialize the variable s to 0, otherwise, we will get the wrong answer because of the garbage value of s.
Initializing Array
When an array is declared inside a function the elements of the array have garbage value. If an array is global or static, then its elements are automatically initialized to 0. We can explicitly initialize elements of an array at the time of declaration using the following syntax:
Syntax: datatype array_name[size] = { val1, val2, val3, ..... ValN };
Datatype is the type of elements of an array.
Array_name is the variable name, which must be any valid identifier.
Size is the size of the array.
Val1, val2 ... Are the constants known as initializers. Each value is separated by a comma(,) and then there is a semi-colon (;) after the closing curly brace (}).
Here is are some examples:
1 2 3 | Float temp[5] = {12.3, 4.1, 3.8, 9.5, 4.5}; // an array of 5 floats
Int arr[9] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; // an array of 9 ints |
While initializing 1-D array it is optional to specify the size of the array, so you can also write the above statements as:
1 2 3 | Float temp[] = {12.3, 4.1, 3.8, 9.5, 4.5}; // an array of 5 floats
Int arr[] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; // an array of 9 ints |
If the number of initializers is less than the specified size then the remaining elements of the array are assigned a value of 0.
Float temp[5] = {12.3, 4.1};
Here the size of temp array is 5 but there are only two initializers. After this initialization the elements of the array are as follows:
Temp[0] is 12.3
temp[1] is 4.1
temp[2] is 0
temp[3] is 0
temp[4] is 0
If the number of initializers is greater than the size of the array then, the compiler will report an error. For example:
Int num[5] = {1, 2, 3, 4, 5, 6, 7, 8} // error
The following program finds the highest and lowest elements in an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | #include<stdio.h> #define SIZE 10
Int main() { Int my_arr[SIZE] = {34,56,78,15,43,71,89,34,70,91}; Int i, max, min;
Max = min = my_arr[0];
For(i = 0; i < SIZE; i++) { // if value of current element is greater than previous value // then assign new value to max If(my_arr[i] > max) { Max = my_arr[i]; }
// if the value of current element is less than previous element // then assign new value to min If(my_arr[i] < min) { Min = my_arr[i]; } }
Printf("Lowest value = %d\n", min); Printf("Highest value = %d", max);
// signal to operating system everything works fine Return 0; } |
Expected Output:
1 2 | Lowest value = 15 Highest value = 91 |
How it works: In line 6, first, we have declared and initialized an array of 10 integers. In the next line, we have declared three more variables of type int namely: i, max and min. In line 9, we have assigned the value of the first element of my_arr to max and min. A for loop is used to iterate through all the elements of an array. Inside the for loop, the first if condition (my_arr[i] > max) checks whether the current element is greater than max, if it is, we assign the value of the current element to max.
The second if statement checks whether the value of the current element is smaller than the value of min. If it is, we assign the value of the current element to min. This process continues until there are elements in the array left to iterate.
When the process is finished, max and min variables will have maximum and minimum values respectively.
Two dimensional Array
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns. However, 2D arrays are created to implement a relational database lookalike data structure. It provides ease of holding the bulk of data at once which can be passed to any number of functions wherever required.
Declaration of two dimensional Array in C
The syntax to declare the 2D array is given below.
- Data_type array_name[rows][columns];
Consider the following example.
- Int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Initialization of 2D Array in C
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array. The two-dimensional array can be declared and defined in the following way.
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Two-dimensional array example in C
- #include<stdio.h>
- Int main(){
- Int i=0,j=0;
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
- //traversing 2D array
- For(i=0;i<4;i++){
- For(j=0;j<3;j++){
- Printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
- }//end of j
- }//end of i
- Return 0;
- }
Output
Arr[0][0] = 1
Arr[0][1] = 2
Arr[0][2] = 3
Arr[1][0] = 2
Arr[1][1] = 3
Arr[1][2] = 4
Arr[2][0] = 3
Arr[2][1] = 4
Arr[2][2] = 5
Arr[3][0] = 4
Arr[3][1] = 5
Arr[3][2] = 6
C 2D array example: Storing elements in a matrix and printing it.
- #include <stdio.h>
- Void main ()
- {
- Int arr[3][3],i,j;
- For (i=0;i<3;i++)
- {
- For (j=0;j<3;j++)
- {
- Printf("Enter a[%d][%d]: ",i,j);
- Scanf("%d",&arr[i][j]);
- }
- }
- Printf("\n printing the elements ....\n");
- For(i=0;i<3;i++)
- {
- Printf("\n");
- For (j=0;j<3;j++)
- {
- Printf("%d\t",arr[i][j]);
- }
- }
- }
Output
Enter a[0][0]: 56
Enter a[0][1]: 10
Enter a[0][2]: 30
Enter a[1][0]: 34
Enter a[1][1]: 21
Enter a[1][2]: 34
Enter a[2][0]: 45
Enter a[2][1]: 56
Enter a[2][2]: 78
Printing the elements ....
56 10 30
34 21 34
45 56 78
A string is a collection of characters, stored in an array followed by null ('\0') character. Null character represents the end of string.
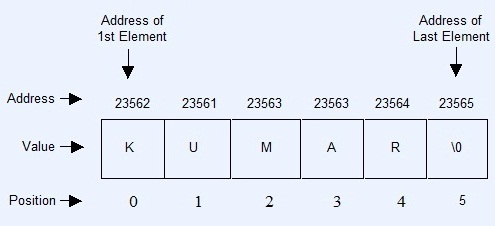
Address of first element is random, address of next element depend upon the type of array. Here, the type is character and character takes one byte in memory, therefore every next address will increment by one.
Index of array will always starts with zero.
Declaration of String or Character array
Declaration of array means creating sequential blocks of memory to hold fixed number of values.
Syntax for string declaration:
Char String-name[size of String ];
Example for string declaration:
Char String [25]; //Statement 1
In the above example we have declared a character array which can hold twenty-five characters at a time.
Initialization of String
Initialization of string means assigning value to declared character array or string.
Examples 1: Using sequence of characters.
Char String [ ] = {'H','e','l','l','o',' ','W','o','r','l','d','\0'};
In the above example, we are declaring and initializing a string at same time. When we declare and initialize a string at same time, giving the size of array is optional and it is programmer's job to specify the null character at the end to terminate the string.
Example 2: Using assignment operator
Char String [ ] = "Hello World";
In this approach, programmer doesn't need to provide null character, compiler will automatically add null character at the end of string.
Input string using getche() function
The getche() read characters from console (output window) one by one and put these characters into string using loop.
Examples for input string with getche() function
#include<stdio.h>
Void main()
{
Char String [50];
Char ch;
Int i;
Printf("\n\n\tEnter your name : ");
For(i=0;i<50;i++)
{
Ch = getche(); //Statement 1
If(ch==13) //Statement 2
Break;
String [i] = ch;
}
String [i] = '\0'; //Statement 3
Printf("\n\n\tHello, ");
For(i=0; String [i]!='\0';i++)
Printf("%c", String [i]);
}
Output :
Enter your name : Kumar
Hello Kumar
In the above example, A for loop will execute upto 50 time and getche() which inside for loop will read single character from console and put the character into ch. If user press the enter key, condition given in statement 2 will satisfy and terminate the loop otherwise every character will assign to String. Statement 4 is assigning null character to String.
Input string using scanf() function
The scanf() can read the entire word at a time. The format specifier for a string is "%s". The scanf() ignores the any occurrence of leading whitespace characters and terminate the string at the first occurrence of whitespace after any input.
Examples for input string with scanf() function
#include<stdio.h>
Void main()
{
Char String [50];
Printf("\n\n\tEnter your name : ");
Scanf("%s", String );
Printf("\n\n\tHello %s", String );
}
Output :
Enter your name : Kumar Wadhwa
Hello Kumar
In the output of above example there is five leading whitespaces in the input which is ignored by the compiler.
Input string using gets() function
The gets() can read the entire line at a time and the string will not terminate until user press the enter key. The gets() will put all the leading and trailing whitespaces into str.
Examples for input string with gets() function
#include<stdio.h>
Void main()
{
Char String [50];
Printf("\n\n\tEnter your name : ");
Gets( String );
Printf("\n\n\tHello %s", String );
}
Output :
Enter your name : Kumar Wadhwa
Hello Kumar Wadhwa
In the output of above example there is five leading whitespaces in the input which is not ignored by the compiler.
Searching
Searching is the process of finding some particular element in the list. If the element is present in the list, then the process is called successful and the process returns the location of that element, otherwise the search is called unsuccessful.
There are two popular search methods that are widely used in order to search some item into the list. However, choice of the algorithm depends upon the arrangement of the list.
- Linear Search
- Binary Search
Linear Search
Linear search is the simplest search algorithm and often called sequential search. In this type of searching, we simply traverse the list completely and match each element of the list with the item whose location is to be found. If the match found then location of the item is returned otherwise the algorithm return NULL.
Linear search is mostly used to search an unordered list in which the items are not sorted. The algorithm of linear search is given as follows.
Algorithm
- LINEAR_SEARCH(A, N, VAL)
- Step 1: [INITIALIZE] SET POS = -1
- Step 2: [INITIALIZE] SET I = 1
- Step 3: Repeat Step 4 while I<=N
- Step 4: IF A[I] = VAL
SET POS = I
PRINT POS
Go to Step 6
[END OF IF]
SET I = I + 1
[END OF LOOP] - Step 5: IF POS = -1
PRINT " VALUE IS NOT PRESENTIN THE ARRAY "
[END OF IF] - Step 6: EXIT
Complexity of algorithm
Complexity | Best Case | Average Case | Worst Case |
Time | O(1) | O(n) | O(n) |
Space |
|
| O(1) |
C Program
- #include<stdio.h>
- Void main ()
- {
- Int a[10] = {10, 23, 40, 1, 2, 0, 14, 13, 50, 9};
- Int item, i,flag;
- Printf("\nEnter Item which is to be searched\n");
- Scanf("%d",&item);
- For (i = 0; i< 10; i++)
- {
- If(a[i] == item)
- {
- Flag = i+1;
- Break;
- }
- Else
- Flag = 0;
- }
- If(flag != 0)
- {
- Printf("\nItem found at location %d\n",flag);
- }
- Else
- {
- Printf("\nItem not found\n");
- }
- }
Output:
Enter Item which is to be searched
20
Item not found
Enter Item which is to be searched
23
Item found at location 2
Java Program
- Import java.util.Scanner;
- Public class Leniear_Search {
- Public static void main(String[] args) {
- Int[] arr = {10, 23, 15, 8, 4, 3, 25, 30, 34, 2, 19};
- Int item,flag=0;
- Scanner sc = new Scanner(System.in);
- System.out.println("Enter Item ?");
- Item = sc.nextInt();
- For(int i = 0; i<10; i++)
- {
- If(arr[i]==item)
- {
- Flag = i+1;
- Break;
- }
- Else
- Flag = 0;
- }
- If(flag != 0)
- {
- System.out.println("Item found at location" + flag);
- }
- Else
- System.out.println("Item not found");
- }
- }
Output:
Enter Item ?
23
Item found at location 2
Enter Item ?
22
Item not found
C# Program
- Using System;
- Public class LinearSearch
- {
- Public static void Main()
- {
- Int item, flag = 0;
- Int[] a= {10, 23, 5, 90, 89, 34, 12, 34, 1, 78};
- Console.WriteLine("Enter the item value");
- Item = Convert.ToInt32(Console.ReadLine());
- For(int i=0;i<10;i++)
- {
- If(item == a[i])
- {
- Flag = i+1;
- Break;
- }
- Else
- Flag = 0;
- }
- If(flag != 0 )
- {
- Console.WriteLine("Item Found at Location " + flag);
- }
- Else
- Console.WriteLine("Item Not Found");
- }
- }
Output:
Enter the item value
78
Item Found at Location 10
Enter the item value
22
Item not found
Python Program
- Arr = [10,2,3,4,23,5,21,45,90,100];
- Item = int(input("Enter the item which you want to search "));
- For i in range (0,len(arr)):
- If arr[i] == item:
- Flag = i+1;
- Break;
- Else:
- Flag = 0;
- If flag != 0:
- Print("Item found at location %d" % (flag));
- Else :
- Print("Item not found");
Output:
Enter the item which you want to search 2
Item found at location 2
Enter the item which you want to search 101
Item not found
Binary Search
Binary search is the search technique which works efficiently on the sorted lists. Hence, in order to search an element into some list by using binary search technique, we must ensure that the list is sorted.
Binary search follows divide and conquer approach in which, the list is divided into two halves and the item is compared with the middle element of the list. If the match is found then, the location of middle element is returned otherwise, we search into either of the halves depending upon the result produced through the match.
Binary search algorithm is given below.
BINARY_SEARCH(A, lower_bound, upper_bound, VAL)
- Step 1: [INITIALIZE] SET BEG = lower_bound
END = upper_bound, POS = - 1 - Step 2: Repeat Steps 3 and 4 while BEG <=END
- Step 3: SET MID = (BEG + END)/2
- Step 4: IF A[MID] = VAL
SET POS = MID
PRINT POS
Go to Step 6
ELSE IF A[MID] > VAL
SET END = MID - 1
ELSE
SET BEG = MID + 1
[END OF IF]
[END OF LOOP] - Step 5: IF POS = -1
PRINT "VALUE IS NOT PRESENT IN THE ARRAY"
[END OF IF] - Step 6: EXIT
Complexity
SN | Performance | Complexity |
1 | Worst case | O(log n) |
2 | Best case | O(1) |
3 | Average Case | O(log n) |
4 | Worst case space complexity | O(1) |
Example
Let us consider an array arr = {1, 5, 7, 8, 13, 19, 20, 23, 29}. Find the location of the item 23 in the array.
In 1st step :
- BEG = 0
- END = 8ron
- MID = 4
- a[mid] = a[4] = 13 < 23, therefore
In Second step:
- Beg = mid +1 = 5
- End = 8
- Mid = 13/2 = 6
- a[mid] = a[6] = 20 < 23, therefore;
In third step:
- Beg = mid + 1 = 7
- End = 8
- Mid = 15/2 = 7
- a[mid] = a[7]
- a[7] = 23 = item;
- Therefore, set location = mid;
- The location of the item will be 7.
Binary Search Program using Recursion
C program
- #include<stdio.h>
- Int binarySearch(int[], int, int, int);
- Void main ()
- {
- Int arr[10] = {16, 19, 20, 23, 45, 56, 78, 90, 96, 100};
- Int item, location=-1;
- Printf("Enter the item which you want to search ");
- Scanf("%d",&item);
- Location = binarySearch(arr, 0, 9, item);
- If(location != -1)
- {
- Printf("Item found at location %d",location);
- }
- Else
- {
- Printf("Item not found");
- }
- }
- Int binarySearch(int a[], int beg, int end, int item)
- {
- Int mid;
- If(end >= beg)
- {
- Mid = (beg + end)/2;
- If(a[mid] == item)
- {
- Return mid+1;
- }
- Else if(a[mid] < item)
- {
- Return binarySearch(a,mid+1,end,item);
- }
- Else
- {
- Return binarySearch(a,beg,mid-1,item);
- }
- }
- Return -1;
- }
Output:
Enter the item which you want to search
19
Item found at location 2
Java
- Import java.util.*;
- Public class BinarySearch {
- Public static void main(String[] args) {
- Int[] arr = {16, 19, 20, 23, 45, 56, 78, 90, 96, 100};
- Int item, location = -1;
- System.out.println("Enter the item which you want to search");
- Scanner sc = new Scanner(System.in);
- Item = sc.nextInt();
- Location = binarySearch(arr,0,9,item);
- If(location != -1)
- System.out.println("the location of the item is "+location);
- Else
- System.out.println("Item not found");
- }
- Public static int binarySearch(int[] a, int beg, int end, int item)
- {
- Int mid;
- If(end >= beg)
- {
- Mid = (beg + end)/2;
- If(a[mid] == item)
- {
- Return mid+1;
- }
- Else if(a[mid] < item)
- {
- Return binarySearch(a,mid+1,end,item);
- }
- Else
- {
- Return binarySearch(a,beg,mid-1,item);
- }
- }
- Return -1;
- }
- }
Output:
Enter the item which you want to search
45
The location of the item is 5
C#
- Using System;
- Public class LinearSearch
- {
- Public static void Main()
- {
- Int[] arr = {16, 19, 20, 23, 45, 56, 78, 90, 96, 100};
- Int location=-1;
- Console.WriteLine("Enter the item which you want to search ");
- Int item = Convert.ToInt32(Console.ReadLine());
- Location = binarySearch(arr, 0, 9, item);
- If(location != -1)
- {
- Console.WriteLine("Item found at location "+ location);
- }
- Else
- {
- Console.WriteLine("Item not found");
- }
- }
- Public static int binarySearch(int[] a, int beg, int end, int item)
- {
- Int mid;
- If(end >= beg)
- {
- Mid = (beg + end)/2;
- If(a[mid] == item)
- {
- Return mid+1;
- }
- Else if(a[mid] < item)
- {
- Return binarySearch(a,mid+1,end,item);
- }
- Else
- {
- Return binarySearch(a,beg,mid-1,item);
- }
- }
- Return -1;
- }
- }
Output:
Enter the item which you want to search
20
Item found at location 3
Python
- Def binarySearch(arr,beg,end,item):
- If end >= beg:
- Mid = int((beg+end)/2)
- If arr[mid] == item :
- Return mid+1
- Elif arr[mid] < item :
- Return binarySearch(arr,mid+1,end,item)
- Else:
- Return binarySearch(arr,beg,mid-1,item)
- Return -1
- Arr=[16, 19, 20, 23, 45, 56, 78, 90, 96, 100];
- Item = int(input("Enter the item which you want to search ?"))
- Location = -1;
- Location = binarySearch(arr,0,9,item);
- If location != -1:
- Print("Item found at location %d" %(location))
- Else:
- Print("Item not found")
Output:
Enter the item which you want to search ?
96
Item found at location 9
Enter the item which you want to search ?
101
Item not found
Binary Search function using Iteration
- Int binarySearch(int a[], int beg, int end, int item)
- {
- Int mid;
- While(end >= beg)
- {
- Mid = (beg + end)/2;
- If(a[mid] == item)
- {
- Return mid+1;
- }
- Else if(a[mid] < item)
- {
- Beg = mid + 1;
- }
- Else
- {
- End = mid - 1;
- }
- }
- Return -1;
- }
Bubble Sort
In Bubble sort, Each element of the array is compared with its adjacent element. The algorithm processes the list in passes. A list with n elements requires n-1 passes for sorting. Consider an array A of n elements whose elements are to be sorted by using Bubble sort. The algorithm processes like following.
- In Pass 1, A[0] is compared with A[1], A[1] is compared with A[2], A[2] is compared with A[3] and so on. At the end of pass 1, the largest element of the list is placed at the highest index of the list.
- In Pass 2, A[0] is compared with A[1], A[1] is compared with A[2] and so on. At the end of Pass 2 the second largest element of the list is placed at the second highest index of the list.
- In pass n-1, A[0] is compared with A[1], A[1] is compared with A[2] and so on. At the end of this pass. The smallest element of the list is placed at the first index of the list.
Algorithm :
- Step 1: Repeat Step 2 For i = 0 to N-1
- Step 2: Repeat For J = i + 1 to N - I
- Step 3: IF A[J] > A[i]
SWAP A[J] and A[i]
[END OF INNER LOOP]
[END OF OUTER LOOP - Step 4: EXIT
Complexity
Scenario | Complexity |
Space | O(1) |
Worst case running time | O(n2) |
Average case running time | O(n) |
Best case running time | O(n2) |
C Program
- #include<stdio.h>
- Void main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
C++ Program
- #include<iostream>
- Using namespace std;
- Int main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] < a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Cout <<"Printing Sorted Element List ...\n";
- For(i = 0; i<10; i++)
- {
- Cout <<a[i]<<"\n";
- }
- Return 0;
- }
Output:
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Java Program
- Public class BubbleSort {
- Public static void main(String[] args) {
- Int[] a = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(int i=0;i<10;i++)
- {
- For (int j=0;j<10;j++)
- {
- If(a[i]<a[j])
- {
- Int temp = a[i];
- a[i]=a[j];
- a[j] = temp;
- }
- }
- }
- System.out.println("Printing Sorted List ...");
- For(int i=0;i<10;i++)
- {
- System.out.println(a[i]);
- }
- }
- }
Output:
Printing Sorted List . . .
7
9
10
12
23
34
34
44
78
101
C# Program
- Using System;
- Public class Program
- {
- Public static void Main()
- {
- Int i, j,temp;
- Int[] a = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Console.WriteLine("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Console.WriteLine(a[i]);
- }
- }
- }
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
Python Program
- a=[10, 9, 7, 101, 23, 44, 12, 78, 34, 23]
- For i in range(0,len(a)):
- For j in range(i+1,len(a)):
- If a[j]<a[i]:
- Temp = a[j]
- a[j]=a[i]
- a[i]=temp
- Print("Printing Sorted Element List...")
- For i in a:
- Print(i)
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
Rust Program
- Fn main()
- {
- Let mut temp;
- Let mut a: [i32; 10] = [10, 9, 7, 101, 23, 44, 12, 78, 34, 23];
- For i in 0..10
- {
- For j in (i+1)..10
- {
- If a[j] < a[i]
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Println!("Printing Sorted Element List ...\n");
- For i in &a
- {
- Println!("{} ",i);
- }
- }
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
4
JavaScript Program
- <html>
- <head>
- <title>
- Bubble Sort
- </title>
- </head>
- <body>
- <script>
- Var a = [10, 9, 7, 101, 23, 44, 12, 78, 34, 23];
- For(i=0;i<10;i++)
- {
- For (j=0;j<10;j++)
- {
- If(a[i]<a[j])
- {
- Temp = a[i];
- a[i]=a[j];
- a[j] = temp;
- }
- }
- }
- Txt = "<br>";
- Document.writeln("Printing Sorted Element List ..."+txt);
- For(i=0;i<10;i++)
- {
- Document.writeln(a[i]);
- Document.writeln(txt);
- }
- </script>
- </body>
- </html>
Output:
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
PHP Program
- <html>
- <head>
- <title>Bubble Sort</title>
- </head>
- <body>
- <?php
- $a = array(10, 9, 7, 101, 23, 44, 12, 78, 34, 23);
- For($i=0;$i<10;$i++)
- {
- For ($j=0;$j<10;$j++)
- {
- If($a[$i]<$a[$j])
- {
- $temp = $a[$i];
- $a[$i]=$a[$j];
- $a[$j] = $temp;
- }
- }
- }
- Echo "Printing Sorted Element List ...\n";
- For($i=0;$i<10;$i++)
- {
- Echo $a[$i];
- Echo "\n";
- }
- ?>
- </body>
- </html>
Output:
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Selection Sort
Selection sort is a simple sorting algorithm. This sorting algorithm is an in-place comparison-based algorithm in which the list is divided into two parts, the sorted part at the left end and the unsorted part at the right end. Initially, the sorted part is empty and the unsorted part is the entire list.
The smallest element is selected from the unsorted array and swapped with the leftmost element, and that element becomes a part of the sorted array. This process continues moving unsorted array boundary by one element to the right.
This algorithm is not suitable for large data sets as its average and worst case complexities are of Ο(n2), where n is the number of items.
How Selection Sort Works?
Consider the following depicted array as an example.

For the first position in the sorted list, the whole list is scanned sequentially. The first position where 14 is stored presently, we search the whole list and find that 10 is the lowest value.

So we replace 14 with 10. After one iteration 10, which happens to be the minimum value in the list, appears in the first position of the sorted list.

For the second position, where 33 is residing, we start scanning the rest of the list in a linear manner.

We find that 14 is the second lowest value in the list and it should appear at the second place. We swap these values.

After two iterations, two least values are positioned at the beginning in a sorted manner.

The same process is applied to the rest of the items in the array.
Following is a pictorial depiction of the entire sorting process −
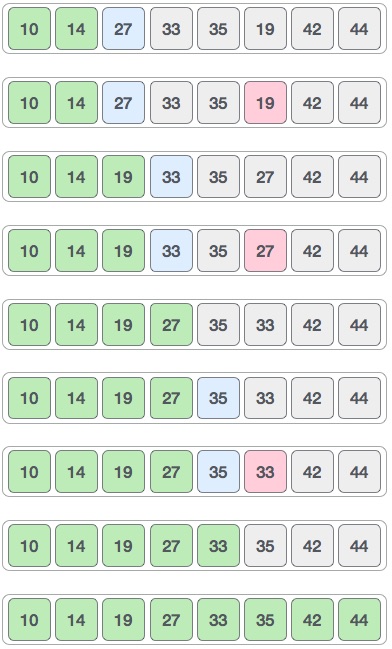
Now, let us learn some programming aspects of selection sort.
Algorithm
Step 1 − Set MIN to location 0
Step 2 − Search the minimum element in the list
Step 3 − Swap with value at location MIN
Step 4 − Increment MIN to point to next element
Step 5 − Repeat until list is sorted
Pseudocode
Procedure selection sort
List : array of items
n : size of list
For i = 1 to n - 1
/* set current element as minimum*/
Min = i
/* check the element to be minimum */
For j = i+1 to n
If list[j] < list[min] then
Min = j;
End if
End for
/* swap the minimum element with the current element*/
If indexMin != i then
Swap list[min] and list[i]
End if
End for
End procedure
Selection Sort Program
Selection sort is a simple sorting algorithm. This sorting algorithm is an in-place comparison-based algorithm in which the list is divided into two parts, the sorted part at the left end and the unsorted part at the right end. Initially, the sorted part is empty and the unsorted part is the entire list.
Implementation in C
#include <stdio.h>
#include <stdbool.h>
#define MAX 7
Int intArray[MAX] = {4,6,3,2,1,9,7};
Void printline(int count) {
Int i;
For(i = 0;i < count-1;i++) {
Printf("=");
}
Printf("=\n");
}
Void display() {
Int i;
Printf("[");
// navigate through all items
For(i = 0;i < MAX;i++) {
Printf("%d ", intArray[i]);
}
Printf("]\n");
}
Void selectionSort() {
Int indexMin,i,j;
// loop through all numbers
For(i = 0; i < MAX-1; i++) {
// set current element as minimum
IndexMin = i;
// check the element to be minimum
For(j = i+1;j < MAX;j++) {
If(intArray[j] < intArray[indexMin]) {
IndexMin = j;
}
}
If(indexMin != i) {
Printf("Items swapped: [ %d, %d ]\n" , intArray[i], intArray[indexMin]);
// swap the numbers
Int temp = intArray[indexMin];
IntArray[indexMin] = intArray[i];
IntArray[i] = temp;
}
Printf("Iteration %d#:",(i+1));
Display();
}
}
Void main() {
Printf("Input Array: ");
Display();
Printline(50);
SelectionSort();
Printf("Output Array: ");
Display();
Printline(50);
}
If we compile and run the above program, it will produce the following result −
Output
Input Array: [4 6 3 2 1 9 7 ]
==================================================
Items swapped: [ 4, 1 ]
Iteration 1#:[1 6 3 2 4 9 7 ]
Items swapped: [ 6, 2 ]
Iteration 2#:[1 2 3 6 4 9 7 ]
Iteration 3#:[1 2 3 6 4 9 7 ]
Items swapped: [ 6, 4 ]
Iteration 4#:[1 2 3 4 6 9 7 ]
Iteration 5#:[1 2 3 4 6 9 7 ]
Items swapped: [ 9, 7 ]
Iteration 6#:[1 2 3 4 6 7 9 ]
Output Array: [1 2 3 4 6 7 9 ]
==================================================
Insertion Sort
This is an in-place comparison-based sorting algorithm. Here, a sub-list is maintained which is always sorted. For example, the lower part of an array is maintained to be sorted. An element which is to be 'insert'ed in this sorted sub-list, has to find its appropriate place and then it is to be inserted there. Hence the name insertion sort.
Implementation in C
#include <stdio.h>
#include <stdbool.h>
#define MAX 7
Int intArray[MAX] = {4,6,3,2,1,9,7};
Void printline(int count) {
Int i;
For(i = 0;i < count-1;i++) {
Printf("=");
}
Printf("=\n");
}
Void display() {
Int i;
Printf("[");
// navigate through all items
For(i = 0;i < MAX;i++) {
Printf("%d ",intArray[i]);
}
Printf("]\n");
}
Void insertionSort() {
Int valueToInsert;
Int holePosition;
Int i;
// loop through all numbers
For(i = 1; i < MAX; i++) {
// select a value to be inserted.
ValueToInsert = intArray[i];
// select the hole position where number is to be inserted
HolePosition = i;
// check if previous no. Is larger than value to be inserted
While (holePosition > 0 && intArray[holePosition-1] > valueToInsert) {
IntArray[holePosition] = intArray[holePosition-1];
HolePosition--;
Printf(" item moved : %d\n" , intArray[holePosition]);
}
If(holePosition != i) {
Printf(" item inserted : %d, at position : %d\n" , valueToInsert,holePosition);
// insert the number at hole position
IntArray[holePosition] = valueToInsert;
}
Printf("Iteration %d#:",i);
Display();
}
}
Void main() {
Printf("Input Array: ");
Display();
Printline(50);
InsertionSort();
Printf("Output Array: ");
Display();
Printline(50);
}
If we compile and run the above program, it will produce the following result −
Output
Input Array: [4 6 3 2 1 9 7 ]
==================================================
Iteration 1#:[4 6 3 2 1 9 7 ]
Item moved : 6
Item moved : 4
Item inserted : 3, at position : 0
Iteration 2#:[3 4 6 2 1 9 7 ]
Item moved : 6
Item moved : 4
Item moved : 3
Item inserted : 2, at position : 0
Iteration 3#:[2 3 4 6 1 9 7 ]
Item moved : 6
Item moved : 4
Item moved : 3
Item moved : 2
Item inserted : 1, at position : 0
Iteration 4#:[1 2 3 4 6 9 7 ]
Iteration 5#:[1 2 3 4 6 9 7 ]
Item moved : 9
Item inserted : 7, at position : 5
Iteration 6#:[1 2 3 4 6 7 9 ]
Output Array: [1 2 3 4 6 7 9 ]
==================================================
The standard form of a quadratic equation is:
Ax2 + bx + c = 0, where
a, b and c are real numbers and
a != 0
The term b2-4ac is known as the discriminant of a quadratic equation. It tells the nature of the roots.
- If the discriminant is greater than 0, the roots are real and different.
- If the discriminant is equal to 0, the roots are real and equal.
- If the discriminant is less than 0, the roots are complex and different.
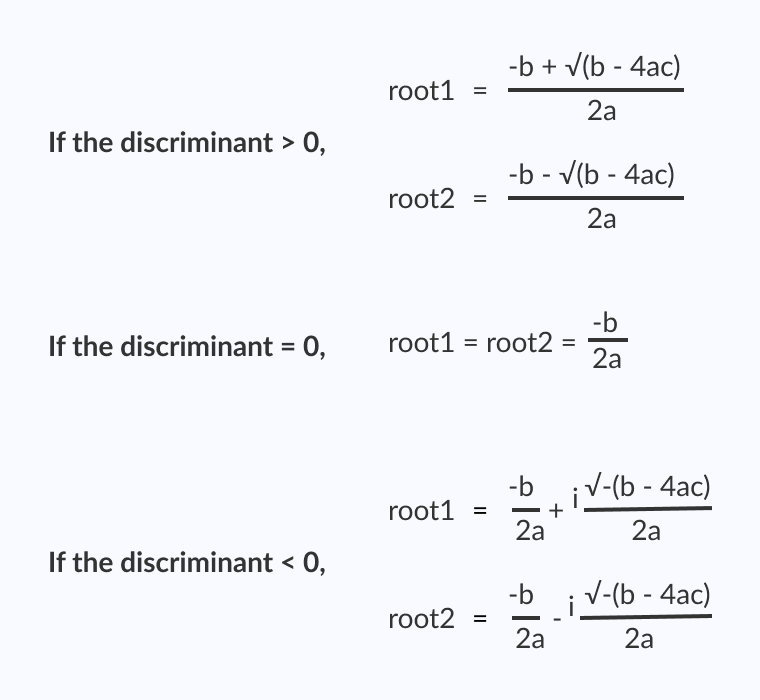
Program to Find Roots of a Quadratic Equation
#include <math.h>
#include <stdio.h>
Int main() {
Double a, b, c, discriminant, root1, root2, realPart, imagPart;
Printf("Enter coefficients a, b and c: ");
Scanf("%lf %lf %lf", &a, &b, &c);
Discriminant = b * b - 4 * a * c;
// condition for real and different roots
If (discriminant > 0) {
Root1 = (-b + sqrt(discriminant)) / (2 * a);
Root2 = (-b - sqrt(discriminant)) / (2 * a);
Printf("root1 = %.2lf and root2 = %.2lf", root1, root2);
}
// condition for real and equal roots
Else if (discriminant == 0) {
Root1 = root2 = -b / (2 * a);
Printf("root1 = root2 = %.2lf;", root1);
}
// if roots are not real
Else {
RealPart = -b / (2 * a);
ImagPart = sqrt(-discriminant) / (2 * a);
Printf("root1 = %.2lf+%.2lfi and root2 = %.2f-%.2fi", realPart, imagPart, realPart, imagPart);
}
Return 0;
}
Output
Enter coefficients a, b and c: 2.3
4
5.6
Root1 = -0.87+1.30i and root2 = -0.87-1.30i
In this program, the sqrt() library function is used to find the square root of a number.
Imagine a classroom of 100 students in which you gave your pen to one person. Now, you want that pen. Here are some ways to find the pen and what the O order is.
O(n2): You go and ask the first person of the class, if he has the pen. Also, you ask this person about other 99 people in the classroom if they have that pen and so on,
This is what we call O(n2).
O(n): Going and asking each student individually is O(N).
O(log n): Now I divide the class into two groups, then ask: “Is it on the left side, or the right side of the classroom?” Then I take that group and divide it into two and ask again, and so on. Repeat the process till you are left with one student who has your pen. This is what you mean by O(log n).
I might need to do the O(n2) search if only one student knows on which student the pen is hidden. I’d use the O(n) if one student had the pen and only they knew it. I’d use the O(log n) search if all the students knew, but would only tell me if I guessed the right side.
NOTE :
We are interested in rate of growth of time with respect to the inputs taken during the program execution .
Another Example
Time Complexity of algorithm/code is not equal to the actual time required to execute a particular code but the number of times a statement executes. We can prove this by using time command. For example, Write code in C/C++ or any other language to find maximum between N numbers, where N varies from 10, 100, 1000, 10000. And compile that code on Linux based operating system (Fedora or Ubuntu) with below command:
Gcc program.c – o program
Run it with time ./program
You will get surprising results i.e. for N = 10 you may get 0.5ms time and for N = 10, 000 you may get 0.2 ms time. Also, you will get different timings on the different machine. So, we can say that actual time requires to execute code is machine dependent (whether you are using pentium1 or pentiun5) and also it considers network load if your machine is in LAN/WAN. Even you will not get the same timings on the same machine for the same code, the reason behind that the current network load.
Now, the question arises if time complexity is not the actual time require executing the code then what is it?
The answer is : Instead of measuring actual time required in executing each statement in the code, we consider how many times each statement execute.
For example:
#include <stdio.h> Int main() { Printf("Hello World"); } |
Output:
Hello World
In above code “Hello World!!!” print only once on a screen. So, time complexity is constant: O(1) i.e. every time constant amount of time require to execute code, no matter which operating system or which machine configurations you are using.
Now consider another code:
#include <stdio.h> Void main() { Int i, n = 8; For (i = 1; i <= n; i++) { Printf("Hello Word !!!"); } } |
Output:
Hello Word !!!Hello Word !!!Hello Word !!!Hello Word !!!
Hello Word !!!Hello Word !!!Hello Word !!!Hello Word !!!
In above code “Hello World!!!” will print N times. So, time complexity of above code is O(N).
ADDITIONAL INFORMATION :
For example:
Let us consider a model machine which has the following specifications:
–Single processor
–32 bit
–Sequential execution
–1 unit time for arithmetic and logical operations
–1 unit time for assignment and return statements
1.Sum of 2 numbers :
Pseudocode: Sum(a,b){ Return a+b //Takes 2 unit of time(constant) one for arithmetic operation and one for return.(as per above conventions) cost=2 no of times=1 } |
Tsum= 2 = C =O(1)
2.Sum of all elements of a list :
Pseudocode: List_Sum(A,n){//A->array and n->number of elements in the array Total =0 // cost=1 no of times=1 For i=0 to n-1 // cost=2 no of times=n+1 (+1 for the end false condition) Sum = sum + A[i] // cost=2 no of times=n Return sum // cost=1 no of times=1 } |
Tsum=1 + 2 * (n+1) + 2 * n + 1 = 4n + 1 =C1 * n + C2 = O(n)
3.Sum of all elements of a matrix :
For this one the complexity is a polynomial equation (quadratic equation for a square matrix)
Matrix nxn => Tsum= an2 +bn + c
For this Tsum if in order of n2 = O(n2)
The above codes do not run in the IDE as they are pseudo codes and do not resemble any programming language .
So from the above, we can conclude that the time of execution increases with the type of operations we make using the inputs.
The above O -> is called Big – Oh which is an asymptotic notation. There are other asymptotic notations like theta and Ohm.
Reference
1. Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill.
2. E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
3. Brian W. Kernighan and Dennis M. Ritchie, The C Programming Language, Prentice Hall of India