UNIT 3
Array
Arrays a kind of data structure that can store a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Instead of declaring individual variables, such as number0, number1, ..., and number99, you declare one array variable such as numbers and use numbers[0], numbers[1], and ..., numbers[99] to represent individual variables. A specific element in an array is accessed by an index.
All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.
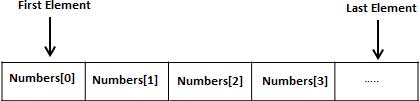
Declaring Arrays
To declare an array in C, a programmer specifies the type of the elements and the number of elements required by an array as follows −
Type arrayName [ arraySize ];
This is called a single-dimensional array. The arraySize must be an integer constant greater than zero and type can be any valid C data type. For example, to declare a 10-element array called balance of type double, use this statement −
Double balance[10];
Here balance is a variable array which is sufficient to hold up to 10 double numbers.
Initializing Arrays
You can initialize an array in C either one by one or using a single statement as follows −
Double balance[5] = {1000.0, 2.0, 3.4, 7.0, 50.0};
The number of values between braces { } cannot be larger than the number of elements that we declare for the array between square brackets [ ].
If you omit the size of the array, an array just big enough to hold the initialization is created. Therefore, if you write −
Double balance[] = {1000.0, 2.0, 3.4, 7.0, 50.0};
You will create exactly the same array as you did in the previous example. Following is an example to assign a single element of the array −
Balance[4] = 50.0;
The above statement assigns the 5th element in the array with a value of 50.0. All arrays have 0 as the index of their first element which is also called the base index and the last index of an array will be total size of the array minus 1. Shown below is the pictorial representation of the array we discussed above −

Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index of the element within square brackets after the name of the array. For example −
Double salary = balance[9];
The above statement will take the 10th element from the array and assign the value to salary variable. The following example Shows how to use all the three above mentioned concepts viz. Declaration, assignment, and accessing arrays −
#include <stdio.h>
Int main () {
Int n[ 10 ]; /* n is an array of 10 integers */
Int i,j;
/* initialize elements of array n to 0 */
For ( i = 0; i < 10; i++ ) {
n[ i ] = i + 100; /* set element at location i to i + 100 */
}
/* output each array element's value */
For (j = 0; j < 10; j++ ) {
Printf("Element[%d] = %d\n", j, n[j] );
}
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Element[0] = 100
Element[1] = 101
Element[2] = 102
Element[3] = 103
Element[4] = 104
Element[5] = 105
Element[6] = 106
Element[7] = 107
Element[8] = 108
Element[9] = 109
Arrays in Detail
Arrays are important to C and should need a lot more attention. The following important concepts related to array should be clear to a C programmer −
Sr.No. | Concept & Description |
1 | Multi-dimensional array C supports multidimensional arrays. The simplest form of the multidimensional array is the two-dimensional array. |
2 | Passing array to function You can pass to the function a pointer to an array by specifying the array's name without an index. |
3 | Return array from a function C allows a function to return an array. |
4 | Pointer to an array You can generate a pointer to the first element of an array by simply specifying the array name, without any index. |
C Array
An array is defined as the collection of similar type of data items stored at contiguous memory locations. Arrays are the derived data type in C programming language which can store the primitive type of data such as int, char, double, float, etc. It also has the capability to store the collection of derived data types, such as pointers, structure, etc. The array is the simplest data structure where each data element can be randomly accessed by using its index number.
C array is beneficial if you have to store similar elements. For example, if we want to store the marks of a student in 6 subjects, then we don't need to define different variables for the marks in the different subject. Instead of that, we can define an array which can store the marks in each subject at the contiguous memory locations.
By using the array, we can access the elements easily. Only a few lines of code are required to access the elements of the array.
Properties of Array
The array contains the following properties.
- Each element of an array is of same data type and carries the same size, i.e., int = 4 bytes.
- Elements of the array are stored at contiguous memory locations where the first element is stored at the smallest memory location.
- Elements of the array can be randomly accessed since we can calculate the address of each element of the array with the given base address and the size of the data element.
Advantage of C Array
1) Code Optimization: Less code to the access the data.
2) Ease of traversing: By using the for loop, we can retrieve the elements of an array easily.
3) Ease of sorting: To sort the elements of the array, we need a few lines of code only.
4) Random Access: We can access any element randomly using the array.
Disadvantage of C Array
1) Fixed Size: Whatever size, we define at the time of declaration of the array, we can't exceed the limit. So, it doesn't grow the size dynamically like LinkedList which we will learn later.
Declaration of C Array
We can declare an array in the c language in the following way.
- Data_type array_name[array_size];
Now, let us see the example to declare the array.
- Int marks[5];
Here, int is the data_type, marks are the array_name, and 5 is the array_size.
Initialization of C Array
The simplest way to initialize an array is by using the index of each element. We can initialize each element of the array by using the index. Consider the following example.
- Marks[0]=80;//initialization of array
- Marks[1]=60;
- Marks[2]=70;
- Marks[3]=85;
- Marks[4]=75;
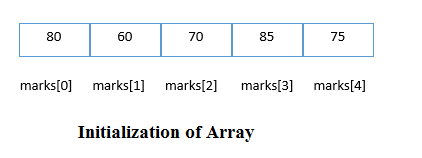
C array example
- #include<stdio.h>
- Int main(){
- Int i=0;
- Int marks[5];//declaration of array
- Marks[0]=80;//initialization of array
- Marks[1]=60;
- Marks[2]=70;
- Marks[3]=85;
- Marks[4]=75;
- //traversal of array
- For(i=0;i<5;i++){
- Printf("%d \n",marks[i]);
- }//end of for loop
- Return 0;
- }
Output
80
60
70
85
75
C Array: Declaration with Initialization
We can initialize the c array at the time of declaration. Let's see the code.
- Int marks[5]={20,30,40,50,60};
In such case, there is no requirement to define the size. So it may also be written as the following code.
- Int marks[]={20,30,40,50,60};
Let's see the C program to declare and initialize the array in C.
- #include<stdio.h>
- Int main(){
- Int i=0;
- Int marks[5]={20,30,40,50,60};//declaration and initialization of array
- //traversal of array
- For(i=0;i<5;i++){
- Printf("%d \n",marks[i]);
- }
- Return 0;
- }
Output
20
30
40
50
60
C Array Example: Sorting an array
In the following program, we are using bubble sort method to sort the array in ascending order.
- #include<stdio.h>
- Void main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Program to print the largest and second largest element of the array.
- #include<stdio.h>
- Void main ()
- {
- Int arr[100],i,n,largest,sec_largest;
- Printf("Enter the size of the array?");
- Scanf("%d",&n);
- Printf("Enter the elements of the array?");
- For(i = 0; i<n; i++)
- {
- Scanf("%d",&arr[i]);
- }
- Largest = arr[0];
- Sec_largest = arr[1];
- For(i=0;i<n;i++)
- {
- If(arr[i]>largest)
- {
- Sec_largest = largest;
- Largest = arr[i];
- }
- Else if (arr[i]>sec_largest && arr[i]!=largest)
- {
- Sec_largest=arr[i];
- }
- }
- Printf("largest = %d, second largest = %d",largest,sec_largest);
- }
One-dimensional array
Conceptually you can think of a one-dimensional array as a row, where elements are stored one after another.
Syntax: datatype array_name[size];
Datatype: It denotes the type of the elements in the array.
Array_name: Name of the array. It must be a valid identifier.
Size: Number of elements an array can hold. Here are some example of array declarations:
1 2 3 | Int num[100]; Float temp[20]; Char ch[50]; |
Num is an array of type int, which can only store 100 elements of type int.
temp is an array of type float, which can only store 20 elements of type float.
ch is an array of type char, which can only store 50 elements of type char.
Note: When an array is declared it contains garbage values.
The individual elements in the array:
1 2 3 | Num[0], num[1], num[2], ....., num[99] Temp[0], temp[1], temp[2], ....., temp[19] Ch[0], ch[1], ch[2], ....., ch[49] |
We can also use variables and symbolic constants to specify the size of the array.
1 2 3 4 5 6 7 8 9 10 | #define SIZE 10
Int main() { Int size = 10;
Int my_arr1[SIZE]; // ok Int my_arr2[size]; // not allowed until C99 // ... } |
Note: Until C99 standard, we were not allowed to use variables to specify the size of the array. If you are using a compiler which supports C99 standard, the above code would compile successfully. However, If you're using an older version of C compiler like Turbo C++, then you will get an error.
The use of symbolic constants makes the program maintainable, because later if you want to change the size of the array you need to modify it at once place only i.e in the #define directive.
Accessing elements of an array
The elements of an array can be accessed by specifying array name followed by subscript or index inside square brackets (i.e []). Array subscript or index starts at 0. If the size of an array is 10 then the first element is at index 0, while the last element is at index 9. The first valid subscript (i.e 0) is known as the lower bound, while last valid subscript is known as the upper bound.
Int my_arr[5];
Then elements of this array are;
First element – my_arr[0]
Second element – my_arr[1]
Third element – my_arr[2]
Fourth element – my_arr[3]
Fifth element – my_arr[4]
Array subscript or index can be any expression that yields an integer value. For example:
1 2 3 4 | Int i = 0, j = 2; My_arr[i]; // 1st element My_arr[i+1]; // 2nd element My_arr[i+j]; // 3rd element |
In the array my_arr, the last element is at my_arr[4], What if you try to access elements beyond the last valid index of the array?
1 2 3 | Printf("%d", my_arr[5]); // 6th element Printf("%d", my_arr[10]); // 11th element Printf("%d", my_arr[-1]); // element just before 0 |
Sure indexes 5, 10 and -1 are not valid but C compiler will not show any error message instead some garbage value will be printed. The C language doesn't check bounds of the array. It is the responsibility of the programmer to check array bounds whenever required.
Processing 1-D arrays
The following program uses for loop to take input and print elements of a 1-D array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include<stdio.h>
Int main() { Int arr[5], i;
For(i = 0; i < 5; i++) { Printf("Enter a[%d]: ", i); Scanf("%d", &arr[i]); }
Printf("\nPrinting elements of the array: \n\n");
For(i = 0; i < 5; i++) { Printf("%d ", arr[i]); }
// signal to operating system program ran fine Return 0; } |
Expected Output:
1 2 3 4 5 6 7 8 9 | Enter a[0]: 11 Enter a[1]: 22 Enter a[2]: 34 Enter a[3]: 4 Enter a[4]: 34
Printing elements of the array:
11 22 34 4 34 |
How it works:
In Line 5, we have declared an array of 5 integers and variable i of type int. Then a for loop is used to enter five elements into an array. In scanf() we have used & operator (also known as the address of operator) on element arr[i] of an array, just like we had done with variables of type int, float, char etc. Line 13 prints "Printing elements of the array" to the console. The second for loop prints all the elements of an array one by one.
The following program prints the sum of elements of an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include<stdio.h>
Int main() { Int arr[5], i, s = 0;
For(i = 0; i < 5; i++) { Printf("Enter a[%d]: ", i); Scanf("%d", &arr[i]); }
For(i = 0; i < 5; i++) { s += arr[i]; }
Printf("\nSum of elements = %d ", s);
// signal to operating system program ran fine Return 0; } |
Expected Output:
1 2 3 4 5 6 7 | Enter a[0]: 22 Enter a[1]: 33 Enter a[2]: 56 Enter a[3]: 73 Enter a[4]: 23
Sum of elements = 207 |
How it works: The first for loop asks the user to enter five elements into the array. The second for loop reads all the elements of an array one by one and accumulate the sum of all the elements in the variable s. Note that it is necessary to initialize the variable s to 0, otherwise, we will get the wrong answer because of the garbage value of s.
Initializing Array
When an array is declared inside a function the elements of the array have garbage value. If an array is global or static, then its elements are automatically initialized to 0. We can explicitly initialize elements of an array at the time of declaration using the following syntax:
Syntax: datatype array_name[size] = { val1, val2, val3, ..... ValN };
Datatype is the type of elements of an array.
Array_name is the variable name, which must be any valid identifier.
Size is the size of the array.
Val1, val2 ... Are the constants known as initializers. Each value is separated by a comma(,) and then there is a semi-colon (;) after the closing curly brace (}).
Here is are some examples:
1 2 3 | Float temp[5] = {12.3, 4.1, 3.8, 9.5, 4.5}; // an array of 5 floats
Int arr[9] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; // an array of 9 ints |
While initializing 1-D array it is optional to specify the size of the array, so you can also write the above statements as:
1 2 3 | Float temp[] = {12.3, 4.1, 3.8, 9.5, 4.5}; // an array of 5 floats
Int arr[] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; // an array of 9 ints |
If the number of initializers is less than the specified size then the remaining elements of the array are assigned a value of 0.
Float temp[5] = {12.3, 4.1};
Here the size of temp array is 5 but there are only two initializers. After this initialization the elements of the array are as follows:
Temp[0] is 12.3
temp[1] is 4.1
temp[2] is 0
temp[3] is 0
temp[4] is 0
If the number of initializers is greater than the size of the array then, the compiler will report an error. For example:
Int num[5] = {1, 2, 3, 4, 5, 6, 7, 8} // error
The following program finds the highest and lowest elements in an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | #include<stdio.h> #define SIZE 10
Int main() { Int my_arr[SIZE] = {34,56,78,15,43,71,89,34,70,91}; Int i, max, min;
Max = min = my_arr[0];
For(i = 0; i < SIZE; i++) { // if value of current element is greater than previous value // then assign new value to max If(my_arr[i] > max) { Max = my_arr[i]; }
// if the value of current element is less than previous element // then assign new value to min If(my_arr[i] < min) { Min = my_arr[i]; } }
Printf("Lowest value = %d\n", min); Printf("Highest value = %d", max);
// signal to operating system everything works fine Return 0; } |
Expected Output:
1 2 | Lowest value = 15 Highest value = 91 |
How it works: In line 6, first, we have declared and initialized an array of 10 integers. In the next line, we have declared three more variables of type int namely: i, max and min. In line 9, we have assigned the value of the first element of my_arr to max and min. A for loop is used to iterate through all the elements of an array. Inside the for loop, the first if condition (my_arr[i] > max) checks whether the current element is greater than max, if it is, we assign the value of the current element to max.
The second if statement checks whether the value of the current element is smaller than the value of min. If it is, we assign the value of the current element to min. This process continues until there are elements in the array left to iterate.
When the process is finished, max and min variables will have maximum and minimum values respectively.
Two Dimensional Array in C
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns. However, 2D arrays are created to implement a relational database lookalike data structure. It provides ease of holding the bulk of data at once which can be passed to any number of functions wherever required.
Declaration of two dimensional Array in C
The syntax to declare the 2D array is given below.
- Data_type array_name[rows][columns];
Consider the following example.
- Int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Initialization of 2D Array in C
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array. The two-dimensional array can be declared and defined in the following way.
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Two-dimensional array example in C
- #include<stdio.h>
- Int main(){
- Int i=0,j=0;
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
- //traversing 2D array
- For(i=0;i<4;i++){
- For(j=0;j<3;j++){
- Printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
- }//end of j
- }//end of i
- Return 0;
- }
Output
Arr[0][0] = 1
Arr[0][1] = 2
Arr[0][2] = 3
Arr[1][0] = 2
Arr[1][1] = 3
Arr[1][2] = 4
Arr[2][0] = 3
Arr[2][1] = 4
Arr[2][2] = 5
Arr[3][0] = 4
Arr[3][1] = 5
Arr[3][2] = 6
C 2D array example: Storing elements in a matrix and printing it.
- #include <stdio.h>
- Void main ()
- {
- Int arr[3][3],i,j;
- For (i=0;i<3;i++)
- {
- For (j=0;j<3;j++)
- {
- Printf("Enter a[%d][%d]: ",i,j);
- Scanf("%d",&arr[i][j]);
- }
- }
- Printf("\n printing the elements ....\n");
- For(i=0;i<3;i++)
- {
- Printf("\n");
- For (j=0;j<3;j++)
- {
- Printf("%d\t",arr[i][j]);
- }
- }
- }
Output
Enter a[0][0]: 56
Enter a[0][1]: 10
Enter a[0][2]: 30
Enter a[1][0]: 34
Enter a[1][1]: 21
Enter a[1][2]: 34
Enter a[2][0]: 45
Enter a[2][1]: 56
Enter a[2][2]: 78
Printing the elements ....
56 10 30
34 21 34
45 56 78
In Bubble sort, Each element of the array is compared with its adjacent element. The algorithm processes the list in passes. A list with n elements requires n-1 passes for sorting. Consider an array A of n elements whose elements are to be sorted by using Bubble sort. The algorithm processes like following.
- In Pass 1, A[0] is compared with A[1], A[1] is compared with A[2], A[2] is compared with A[3] and so on. At the end of pass 1, the largest element of the list is placed at the highest index of the list.
- In Pass 2, A[0] is compared with A[1], A[1] is compared with A[2] and so on. At the end of Pass 2 the second largest element of the list is placed at the second highest index of the list.
- In pass n-1, A[0] is compared with A[1], A[1] is compared with A[2] and so on. At the end of this pass. The smallest element of the list is placed at the first index of the list.
Algorithm :
- Step 1: Repeat Step 2 For i = 0 to N-1
- Step 2: Repeat For J = i + 1 to N - I
- Step 3: IF A[J] > A[i]
SWAP A[J] and A[i]
[END OF INNER LOOP]
[END OF OUTER LOOP - Step 4: EXIT
Complexity
Scenario | Complexity |
Space | O(1) |
Worst case running time | O(n2) |
Average case running time | O(n) |
Best case running time | O(n2) |
C Program
- #include<stdio.h>
- Void main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
C++ Program
- #include<iostream>
- Using namespace std;
- Int main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] < a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Cout <<"Printing Sorted Element List ...\n";
- For(i = 0; i<10; i++)
- {
- Cout <<a[i]<<"\n";
- }
- Return 0;
- }
Output:
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Java Program
- Public class BubbleSort {
- Public static void main(String[] args) {
- Int[] a = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(int i=0;i<10;i++)
- {
- For (int j=0;j<10;j++)
- {
- If(a[i]<a[j])
- {
- Int temp = a[i];
- a[i]=a[j];
- a[j] = temp;
- }
- }
- }
- System.out.println("Printing Sorted List ...");
- For(int i=0;i<10;i++)
- {
- System.out.println(a[i]);
- }
- }
- }
Output:
Printing Sorted List . . .
7
9
10
12
23
34
34
44
78
101
C# Program
- Using System;
- Public class Program
- {
- Public static void Main()
- {
- Int i, j,temp;
- Int[] a = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Console.WriteLine("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Console.WriteLine(a[i]);
- }
- }
- }
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
Python Program
- a=[10, 9, 7, 101, 23, 44, 12, 78, 34, 23]
- For i in range(0,len(a)):
- For j in range(i+1,len(a)):
- If a[j]<a[i]:
- Temp = a[j]
- a[j]=a[i]
- a[i]=temp
- Print("Printing Sorted Element List...")
- For i in a:
- Print(i)
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
Rust Program
- Fn main()
- {
- Let mut temp;
- Let mut a: [i32; 10] = [10, 9, 7, 101, 23, 44, 12, 78, 34, 23];
- For i in 0..10
- {
- For j in (i+1)..10
- {
- If a[j] < a[i]
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Println!("Printing Sorted Element List ...\n");
- For i in &a
- {
- Println!("{} ",i);
- }
- }
Output:
Printing Sorted Element List . . .
7
9
10
12
23
34
34
44
78
101
4
JavaScript Program
- <html>
- <head>
- <title>
- Bubble Sort
- </title>
- </head>
- <body>
- <script>
- Var a = [10, 9, 7, 101, 23, 44, 12, 78, 34, 23];
- For(i=0;i<10;i++)
- {
- For (j=0;j<10;j++)
- {
- If(a[i]<a[j])
- {
- Temp = a[i];
- a[i]=a[j];
- a[j] = temp;
- }
- }
- }
- Txt = "<br>";
- Document.writeln("Printing Sorted Element List ..."+txt);
- For(i=0;i<10;i++)
- {
- Document.writeln(a[i]);
- Document.writeln(txt);
- }
- </script>
- </body>
- </html>
Output:
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
PHP Program
- <html>
- <head>
- <title>Bubble Sort</title>
- </head>
- <body>
- <?php
- $a = array(10, 9, 7, 101, 23, 44, 12, 78, 34, 23);
- For($i=0;$i<10;$i++)
- {
- For ($j=0;$j<10;$j++)
- {
- If($a[$i]<$a[$j])
- {
- $temp = $a[$i];
- $a[$i]=$a[$j];
- $a[$j] = $temp;
- }
- }
- }
- Echo "Printing Sorted Element List ...\n";
- For($i=0;$i<10;$i++)
- {
- Echo $a[$i];
- Echo "\n";
- }
- ?>
- </body>
- </html>
Output:
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
In selection sort, the smallest value among the unsorted elements of the array is selected in every pass and inserted to its appropriate position into the array.
First, find the smallest element of the array and place it on the first position. Then, find the second smallest element of the array and place it on the second position. The process continues until we get the sorted array.
The array with n elements is sorted by using n-1 pass of selection sort algorithm.
- In 1st pass, smallest element of the array is to be found along with its index pos. Then, swap A[0] and A[pos]. Thus A[0] is sorted, we now have n -1 elements which are to be sorted.
- In 2nd pas, position pos of the smallest element present in the sub-array A[n-1] is found. Then, swap, A[1] and A[pos]. Thus A[0] and A[1] are sorted, we now left with n-2 unsorted elements.
- In n-1th pass, position pos of the smaller element between A[n-1] and A[n-2] is to be found. Then, swap, A[pos] and A[n-1].
Therefore, by following the above explained process, the elements A[0], A[1], A[2],...., A[n-1] are sorted.
Example
Consider the following array with 6 elements. Sort the elements of the array by using selection sort.
A = {10, 2, 3, 90, 43, 56}.
Pass | Pos | A[0] | A[1] | A[2] | A[3] | A[4] | A[5] |
1 | 1 | 2 | 10 | 3 | 90 | 43 | 56 |
2 | 2 | 2 | 3 | 10 | 90 | 43 | 56 |
3 | 2 | 2 | 3 | 10 | 90 | 43 | 56 |
4 | 4 | 2 | 3 | 10 | 43 | 90 | 56 |
5 | 5 | 2 | 3 | 10 | 43 | 56 | 90 |
Sorted A = {2, 3, 10, 43, 56, 90}
Complexity
Complexity | Best Case | Average Case | Worst Case |
Time | Ω(n) | θ(n2) | o(n2) |
Space |
|
| o(1) |
Algorithm
SELECTION SORT(ARR, N)
- Step 1: Repeat Steps 2 and 3 for K = 1 to N-1
- Step 2: CALL SMALLEST(ARR, K, N, POS)
- Step 3: SWAP A[K] with ARR[POS]
[END OF LOOP] - Step 4: EXIT
SMALLEST (ARR, K, N, POS)
- Step 1: [INITIALIZE] SET SMALL = ARR[K]
- Step 2: [INITIALIZE] SET POS = K
- Step 3: Repeat for J = K+1 to N -1
IF SMALL > ARR[J]
SET SMALL = ARR[J]
SET POS = J
[END OF IF]
[END OF LOOP] - Step 4: RETURN POS
C Program
- #include<stdio.h>
- Int smallest(int[],int,int);
- Void main ()
- {
- Int a[10] = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Int i,j,k,pos,temp;
- For(i=0;i<10;i++)
- {
- Pos = smallest(a,10,i);
- Temp = a[i];
- a[i]=a[pos];
- a[pos] = temp;
- }
- Printf("\nprinting sorted elements...\n");
- For(i=0;i<10;i++)
- {
- Printf("%d\n",a[i]);
- }
- }
- Int smallest(int a[], int n, int i)
- {
- Int small,pos,j;
- Small = a[i];
- Pos = i;
- For(j=i+1;j<10;j++)
- {
- If(a[j]<small)
- {
- Small = a[j];
- Pos=j;
- }
- }
- Return pos;
- }
Output:
Printing sorted elements...
7
9
10
12
23
23
34
44
78
101
C++ Program
- #include<iostream>
- Using namespace std;
- Int smallest(int[],int,int);
- Int main ()
- {
- Int a[10] = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Int i,j,k,pos,temp;
- For(i=0;i<10;i++)
- {
- Pos = smallest(a,10,i);
- Temp = a[i];
- a[i]=a[pos];
- a[pos] = temp;
- }
- Cout<<"\n printing sorted elements...\n";
- For(i=0;i<10;i++)
- {
- Cout<<a[i]<<"\n";
- }
- Return 0;
- }
- Int smallest(int a[], int n, int i)
- {
- Int small,pos,j;
- Small = a[i];
- Pos = i;
- For(j=i+1;j<10;j++)
- {
- If(a[j]<small)
- {
- Small = a[j];
- Pos=j;
- }
- }
- Return pos;
- }
Output:
Printing sorted elements...
7
9
10
12
23
23
34
44
78
101
Java Program
- Public class SelectionSort {
- Public static void main(String[] args) {
- Int[] a = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Int i,j,k,pos,temp;
- For(i=0;i<10;i++)
- {
- Pos = smallest(a,10,i);
- Temp = a[i];
- a[i]=a[pos];
- a[pos] = temp;
- }
- System.out.println("\nprinting sorted elements...\n");
- For(i=0;i<10;i++)
- {
- System.out.println(a[i]);
- }
- }
- Public static int smallest(int a[], int n, int i)
- {
- Int small,pos,j;
- Small = a[i];
- Pos = i;
- For(j=i+1;j<10;j++)
- {
- If(a[j]<small)
- {
- Small = a[j];
- Pos=j;
- }
- }
- Return pos;
- }
- }
Output:
Printing sorted elements...
7
9
10
12
23
23
34
44
78
101
C# Program
- Using System;
- Public class Program
- {
- Public void Main() {
- Int[] a = {10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Int i,pos,temp;
- For(i=0;i<10;i++)
- {
- Pos = smallest(a,10,i);
- Temp = a[i];
- a[i]=a[pos];
- a[pos] = temp;
- }
- Console.WriteLine("\nprinting sorted elements...\n");
- For(i=0;i<10;i++)
- {
- Console.WriteLine(a[i]);
- }
- }
- Public static int smallest(int[] a, int n, int i)
- {
- Int small,pos,j;
- Small = a[i];
- Pos = i;
- For(j=i+1;j<10;j++)
- {
- If(a[j]<small)
- {
- Small = a[j];
- Pos=j;
- }
- }
- Return pos;
- }
- }
Output:
Printing sorted elements...
7
9
10
12
23
23
34
44
78
101
Python Program
- Def smallest(a,i):
- Small = a[i]
- Pos=i
- For j in range(i+1,10):
- If a[j] < small:
- Small = a[j]
- Pos = j
- Return pos
- a=[10, 9, 7, 101, 23, 44, 12, 78, 34, 23]
- For i in range(0,10):
- Pos = smallest(a,i)
- Temp = a[i]
- a[i]=a[pos]
- a[pos]=temp
- Print("printing sorted elements...")
- For i in a:
- Print(i)
Output:
Printing sorted elements...
7
9
10
12
23
23
34
44
78
101
Rust Program
- Fn main ()
- {
- Let mut a: [i32;10] = [10, 9, 7, 101, 23, 44, 12, 78, 34, 23];
- For i in 0..10
- {
- Let mut small = a[i];
- Let mut pos = i;
- For j in (i+1)..10
- {
- If a[j]<small
- {
- Small = a[j];
- Pos=j;
- }
- }
- Let mut temp = a[i];
- a[i]=a[pos];
- a[pos] = temp;
- }
- Println!("\nprinting sorted elements...\n");
- For i in &a
- {
- Println!("{}",i);
- }
- }
Output:
Printing sorted elements...
7
9
10
12
23
23
34
44
78
101
JavaScript Program
- <html>
- <head>
- <title>
- Selection Sort
- </title>
- </head>
- <body>
- <script>
- Function smallest(a, n, i)
- {
- Var small = a[i];
- Var pos = i;
- For(j=i+1;j<10;j++)
- {
- If(a[j]<small)
- {
- Small = a[j];
- Pos=j;
- }
- }
- Return pos;
- }
- Var a = [10, 9, 7, 101, 23, 44, 12, 78, 34, 23];
- For(i=0;i<10;i++)
- {
- Pos = smallest(a,10,i);
- Temp = a[i];
- a[i]=a[pos];
- a[pos] = temp;
- }
- Document.writeln("printing sorted elements ...\n"+"<br>");
- For(i=0;i<10;i++)
- {
- Document.writeln(a[i]+"<br>");
- }
- </script>
- </body>
- </html>
Output:
Printing sorted elements ...
7
9
10
12
23
23
34
44
78
101
PHP Program
- <html>
- <head>
- <title>Selection sort</title>
- </head>
- <body>
- <?php
- Function smallest($a, $n, $i)
- {
- $small = $a[$i];
- $pos = $i;
- For($j=$i+1;$j<10;$j++)
- {
- If($a[$j]<$small)
- {
- $small = $a[$j];
- $pos=$j;
- }
- }
- Return $pos;
- }
- $a = array(10, 9, 7, 101, 23, 44, 12, 78, 34, 23);
- For($i=0;$i<10;$i++)
- {
- $pos = smallest($a,10,$i);
- $temp = $a[$i];
- $a[$i]=$a[$pos];
- $a[$pos] = $temp;
- }
- Echo "printing sorted elements ...\n";
- For($i=0;$i<10;$i++)
- {
- Echo $a[$i];
- Echo "\n";
- }
- ?>
- </body>
- </html>
Output:
Printing sorted elements ...
7
9
10
12
23
23
34
44
78
101
C Functions
In c, we can divide a large program into the basic building blocks known as function. The function contains the set of programming statements enclosed by {}. A function can be called multiple times to provide reusability and modularity to the C program. In other words, we can say that the collection of functions creates a program. The function is also known as procedure or subroutine in other programming languages.
Advantage of functions in C
There are the following advantages of C functions.
- By using functions, we can avoid rewriting same logic/code again and again in a program.
- We can call C functions any number of times in a program and from any place in a program.
- We can track a large C program easily when it is divided into multiple functions.
- Reusability is the main achievement of C functions.
- However, Function calling is always a overhead in a C program.
Function Aspects
There are three aspects of a C function.
- Function declaration A function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type.
- Function call Function can be called from anywhere in the program. The parameter list must not differ in function calling and function declaration. We must pass the same number of functions as it is declared in the function declaration.
- Function definition It contains the actual statements which are to be executed. It is the most important aspect to which the control comes when the function is called. Here, we must notice that only one value can be returned from the function.
SN | C function aspects | Syntax |
1 | Function declaration | Return_type function_name (argument list); |
2 | Function call | Function_name (argument_list) |
3 | Function definition | Return_type function_name (argument list) {function body;} |
The syntax of creating function in c language is given below:
- Return_type function_name(data_type parameter...){
- //code to be executed
- }
Types of Functions
There are two types of functions in C programming:
- Library Functions: are the functions which are declared in the C header files such as scanf(), printf(), gets(), puts(), ceil(), floor() etc.
- User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times. It reduces the complexity of a big program and optimizes the code.
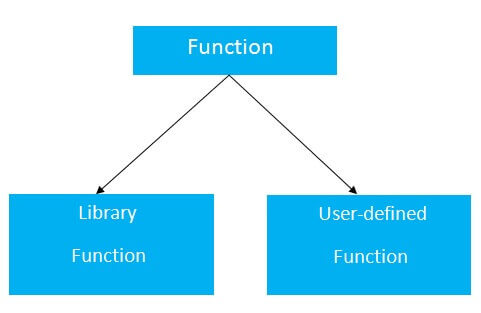
Return Value
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
Let's see a simple example of C function that doesn't return any value from the function.
Example without return value:
- Void hello(){
- Printf("hello c");
- }
If you want to return any value from the function, you need to use any data type such as int, long, char, etc. The return type depends on the value to be returned from the function.
Let's see a simple example of C function that returns int value from the function.
Example with return value:
- Int get(){
- Return 10;
- }
In the above example, we have to return 10 as a value, so the return type is int. If you want to return floating-point value (e.g., 10.2, 3.1, 54.5, etc), you need to use float as the return type of the method.
- Float get(){
- Return 10.2;
- }
Now, you need to call the function, to get the value of the function.
Different aspects of function calling
A function may or may not accept any argument. It may or may not return any value. Based on these facts, There are four different aspects of function calls.
- Function without arguments and without return value
- Function without arguments and with return value
- Function with arguments and without return value
- Function with arguments and with return value
Example for Function without argument and return value
Example 1
- #include<stdio.h>
- Void printName();
- Void main ()
- {
- Printf("Hello ");
- PrintName();
- }
- Void printName()
- {
- Printf("Javatpoint");
- }
Output
Hello Javatpoint
Example 2
- #include<stdio.h>
- Void sum();
- Void main()
- {
- Printf("\nGoing to calculate the sum of two numbers:");
- Sum();
- }
- Void sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Printf("The sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example for Function without argument and with return value
Example 1
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Int result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Result = sum();
- Printf("%d",result);
- }
- Int sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the area of the square
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Printf("Going to calculate the area of the square\n");
- Float area = square();
- Printf("The area of the square: %f\n",area);
- }
- Int square()
- {
- Float side;
- Printf("Enter the length of the side in meters: ");
- Scanf("%f",&side);
- Return side * side;
- }
Output
Going to calculate the area of the square
Enter the length of the side in meters: 10
The area of the square: 100.000000
Example for Function with argument and without return value
Example 1
- #include<stdio.h>
- Void sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Sum(a,b);
- }
- Void sum(int a, int b)
- {
- Printf("\nThe sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the average of five numbers.
- #include<stdio.h>
- Void average(int, int, int, int, int);
- Void main()
- {
- Int a,b,c,d,e;
- Printf("\nGoing to calculate the average of five numbers:");
- Printf("\nEnter five numbers:");
- Scanf("%d %d %d %d %d",&a,&b,&c,&d,&e);
- Average(a,b,c,d,e);
- }
- Void average(int a, int b, int c, int d, int e)
- {
- Float avg;
- Avg = (a+b+c+d+e)/5;
- Printf("The average of given five numbers : %f",avg);
- }
Output
Going to calculate the average of five numbers:
Enter five numbers:10
20
30
40
50
The average of given five numbers : 30.000000
Example for Function with argument and with return value
Example 1
- #include<stdio.h>
- Int sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Result = sum(a,b);
- Printf("\nThe sum is : %d",result);
- }
- Int sum(int a, int b)
- {
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers:10
20
The sum is : 30
Example 2: Program to check whether a number is even or odd
- #include<stdio.h>
- Int even_odd(int);
- Void main()
- {
- Int n,flag=0;
- Printf("\nGoing to check whether a number is even or odd");
- Printf("\nEnter the number: ");
- Scanf("%d",&n);
- Flag = even_odd(n);
- If(flag == 0)
- {
- Printf("\nThe number is odd");
- }
- Else
- {
- Printf("\nThe number is even");
- }
- }
- Int even_odd(int n)
- {
- If(n%2 == 0)
- {
- Return 1;
- }
- Else
- {
- Return 0;
- }
- }
Output
Going to check whether a number is even or odd
Enter the number: 100
The number is even
C Library Functions
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers. All C standard library functions are defined inside the different header files saved with the extension .h. We need to include these header files in our program to make use of the library functions defined in such header files. For example, To use the library functions such as printf/scanf we need to include stdio.h in our program which is a header file that contains all the library functions regarding standard input/output.
The list of mostly used header files is given in the following table.
SN | Header file | Description |
1 | Stdio.h | This is a standard input/output header file. It contains all the library functions regarding standard input/output. |
2 | Conio.h | This is a console input/output header file. |
3 | String.h | It contains all string related library functions like gets(), puts(),etc. |
4 | Stdlib.h | This header file contains all the general library functions like malloc(), calloc(), exit(), etc. |
5 | Math.h | This header file contains all the math operations related functions like sqrt(), pow(), etc. |
6 | Time.h | This header file contains all the time-related functions. |
7 | Ctype.h | This header file contains all character handling functions. |
8 | Stdarg.h | Variable argument functions are defined in this header file. |
9 | Signal.h | All the signal handling functions are defined in this header file. |
10 | Setjmp.h | This file contains all the jump functions. |
11 | Locale.h | This file contains locale functions. |
12 | Errno.h | This file contains error handling functions. |
13 | Assert.h | This file contains diagnostics functions. |
C Standard library functions or simply C Library functions are inbuilt functions in C programming.
The prototype and data definitions of these functions are present in their respective header files. To use these functions we need to include the header file in our program. For example,
If you want to use the printf() function, the header file <stdio.h> should be included.
Int main()
{
Printf("Catch me if you can.");
}
If you try to use printf() without including the stdio.h header file, you will get an error.
Advantages of Using C library functions
1. They work
One of the most important reasons you should use library functions is simply because they work. These functions have gone through multiple rigorous testing and are easy to use.
2. The functions are optimized for performance
Since, the functions are "standard library" functions, a dedicated group of developers constantly make them better. In the process, they are able to create the most efficient code optimized for maximum performance.
3. It saves considerable development time
Since the general functions like printing to a screen, calculating the square root, and many more are already written. You shouldn't worry about creating them once again.
4. The functions are portable
With ever-changing real-world needs, your application is expected to work every time, everywhere. And, these library functions help you in that they do the same thing on every computer.
Example: Square root using sqrt() function
Suppose, you want to find the square root of a number.
To can compute the square root of a number, you can use the sqrt() library function. The function is defined in the math.h header file.
Int main()
{
Float num, root;
Printf("Enter a number: ");
Scanf("%f", &num);
// Computes the square root of num and stores in root.
Root = sqrt(num);
Printf("Square root of %.2f = %.2f", num, root);
Return 0;
}
When you run the program, the output will be:
Enter a number: 12
Square root of 12.00 = 3.46
Library Functions in Different Header Files
C Header Files | |
<assert.h> | Program assertion functions |
<ctype.h> | Character type functions |
<locale.h> | Localization functions |
<math.h> | Mathematics functions |
<setjmp.h> | Jump functions |
<signal.h> | Signal handling functions |
<stdarg.h> | Variable arguments handling functions |
<stdio.h> | Standard Input/Output functions |
<stdlib.h> | Standard Utility functions |
<string.h> | String handling functions |
<time.h> | Date time functions |
User-defined Functions
User can define functions to do a task relevant to their programs. Such functions are called user-defined functions. The main( ) function in which we write all our program is a user-defined function. Every pro0gram execution starts at the main( ) function.
Any function (library or user-defined) has 3 things
- Function declaration
- Function calling
- Function defintion
In case of library functions, the function declaration is in header files. The function is library files and the calling is in the program. But in case of user-defined functions all the 3 things are in your source program. Function declaration:
Syntax:
return data_type function_name(arguments list);
Function Definition:
Syntax:
return data_type function_name(argument list)
{
Body;
}
Function Calling:
Syntax:
Function_name(param_list);
Formal Arguments:
The arguments which are given at the time of function declaration or function definition are called formal arguments.
Actual Arguments:
The arguments which are given at the time of function calling are called actual arguments.
General Form of a Function
Return data type function-name(arglist)
Argument declaration
{
Local variable declaration;
Executable statements;
----------------
----------------
Return(expression);
}
All parts are not essential. Some may be absent.
For eg: The argument list and it’s associated argument declaration part are optional.
We may recall that the main functions discussed so far have not included any arguments.
return: This statement is for returning a value to the calling function. This is an optional statement. It’s absence indicates that no value is being return to the calling function.
Function name: A function must follow the same rules of formation as other variable names in C. Additional care must be taken to avoid duplicating library functions names or operating system commands.
Argument list : It contains valid variable name separated by commas. The list must be surrounded by parenthesis. Note that no semi colon follows the closing parenthesis. The argument variables receive values from the calling function. Thus providing a means of data communication from calling function to the called function.
If the arguments are present before beginning with the statements in the functions it is necessary to declare the type of arguments through the type declaration statements.
Note: There are two methods of declaring the parameters. The older method (known as "classic" method) declares function arguments separately after the definition of function name (known as function header).
The newer method (known as "modern" or ANSI method) combines the function definition and argument declaration in one line. Luckily the modern compilers support both the methods. That is, we can write a function using either of them and execute it successfully.
Eg:
01 | #include < stdio.h> | |
02 | #include < conio.h> | |
03 | Void hai(); /* Function Declaration */ | |
04 | Void main() | |
05 | { | |
06 |
| |
07 | Clrscr(); | |
08 | Hai(); /* Function Calling */ | |
09 | Getch(); | |
10 | } | |
11 |
| |
12 | Void hai() /* Function Definition*/ | |
13 | { | |
14 | Printf("\n WELCOME TO THE WORLD OF C LANGUAGE"); | |
15 | } |
Execution of the program:
The program execution normally starts at the main function. After clearing the screen, the control of the program is taken to the hai( ) function. The executable statements in it i.e, the printf statement is executed. Now the control again is taken to the getch( ) function where it waits for a character to be given by the user.
Output:
WELCOME TO THE WORLD OF C LANGUAGE
Example program that shows the difference between calling and called functions.
01 | #include< stdio.h> |
02 | #include< conio.h> |
03 | Void message(); | |
04 | Void main() | |
05 | { | |
06 | Message(); | |
07 | Printf("calling function is main"); | |
08 | } | |
09 | Void message() | |
10 | { | |
11 | Printf("This function is called function which is called by main"); | |
12 | } | |
OUTPUT: This function is called function which is called by main.
calling function is main.
Note: In the above program main () --------calling function and message () ----called function
Call by value and Call by reference in C
There are two methods to pass the data into the function in C language, i.e., call by value and call by reference.
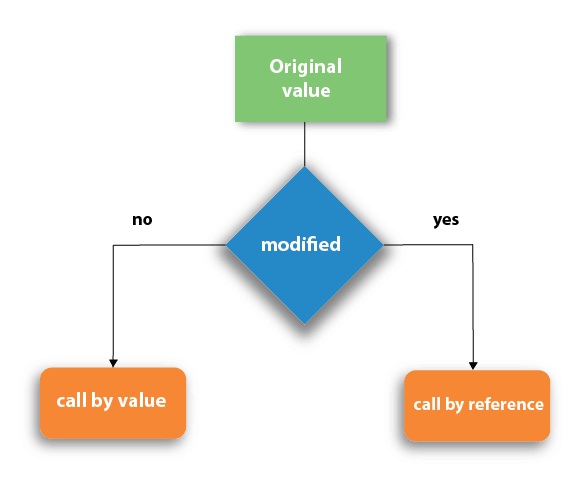
Let's understand call by value and call by reference in c language one by one.
Call by value in C
- In call by value method, the value of the actual parameters is copied into the formal parameters. In other words, we can say that the value of the variable is used in the function call in the call by value method.
- In call by value method, we can not modify the value of the actual parameter by the formal parameter.
- In call by value, different memory is allocated for actual and formal parameters since the value of the actual parameter is copied into the formal parameter.
- The actual parameter is the argument which is used in the function call whereas formal parameter is the argument which is used in the function definition.
Let's try to understand the concept of call by value in c language by the example given below:
- #include<stdio.h>
- Void change(int num) {
- Printf("Before adding value inside function num=%d \n",num);
- Num=num+100;
- Printf("After adding value inside function num=%d \n", num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(x);//passing value in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
Call by Value Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int , int); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(a,b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The value of actual parameters do not change by changing the formal parameters in call by value, a = 10, b = 20
- }
- Void swap (int a, int b)
- {
- Int temp;
- Temp = a;
- a=b;
- b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",a,b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
Call by reference in C
- In call by reference, the address of the variable is passed into the function call as the actual parameter.
- The value of the actual parameters can be modified by changing the formal parameters since the address of the actual parameters is passed.
- In call by reference, the memory allocation is similar for both formal parameters and actual parameters. All the operations in the function are performed on the value stored at the address of the actual parameters, and the modified value gets stored at the same address.
Consider the following example for the call by reference.
- #include<stdio.h>
- Void change(int *num) {
- Printf("Before adding value inside function num=%d \n",*num);
- (*num) += 100;
- Printf("After adding value inside function num=%d \n", *num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(&x);//passing reference in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
Call by reference Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int *, int *); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(&a,&b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The values of actual parameters do change in call by reference, a = 10, b = 20
- }
- Void swap (int *a, int *b)
- {
- Int temp;
- Temp = *a;
- *a=*b;
- *b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",*a,*b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
Difference between call by value and call by reference in c
No. | Call by value | Call by reference |
1 | A copy of the value is passed into the function | An address of value is passed into the function |
2 | Changes made inside the function is limited to the function only. The values of the actual parameters do not change by changing the formal parameters. | Changes made inside the function validate outside of the function also. The values of the actual parameters do change by changing the formal parameters. |
3 | Actual and formal arguments are created at the different memory location | Actual and formal arguments are created at the same memory location |
Recursion is the process which comes into existence when a function calls a copy of itself to work on a smaller problem. Any function which calls itself is called recursive function, and such function calls are called recursive calls. Recursion involves several numbers of recursive calls. However, it is important to impose a termination condition of recursion. Recursion code is shorter than iterative code however it is difficult to understand.
Recursion cannot be applied to all the problem, but it is more useful for the tasks that can be defined in terms of similar subtasks. For Example, recursion may be applied to sorting, searching, and traversal problems.
Generally, iterative solutions are more efficient than recursion since function call is always overhead. Any problem that can be solved recursively, can also be solved iteratively. However, some problems are best suited to be solved by the recursion, for example, tower of Hanoi, Fibonacci series, factorial finding, etc.
In the following example, recursion is used to calculate the factorial of a number.
- #include <stdio.h>
- Int fact (int);
- Int main()
- {
- Int n,f;
- Printf("Enter the number whose factorial you want to calculate?");
- Scanf("%d",&n);
- f = fact(n);
- Printf("factorial = %d",f);
- }
- Int fact(int n)
- {
- If (n==0)
- {
- Return 0;
- }
- Else if ( n == 1)
- {
- Return 1;
- }
- Else
- {
- Return n*fact(n-1);
- }
- }
Output
Enter the number whose factorial you want to calculate?5
Factorial = 120
We can understand the above program of the recursive method call by the figure given below:
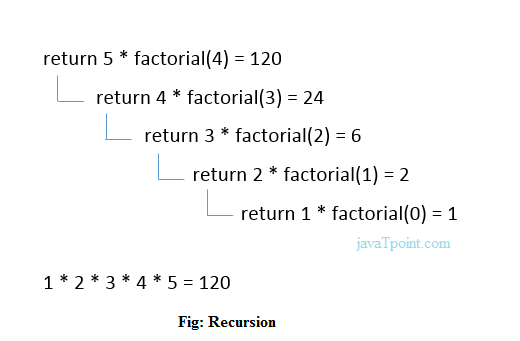
Recursive Function
A recursive function performs the tasks by dividing it into the subtasks. There is a termination condition defined in the function which is satisfied by some specific subtask. After this, the recursion stops and the final result is returned from the function.
The case at which the function doesn't recur is called the base case whereas the instances where the function keeps calling itself to perform a subtask, is called the recursive case. All the recursive functions can be written using this format.
Pseudocode for writing any recursive function is given below.
- If (test_for_base)
- {
- Return some_value;
- }
- Else if (test_for_another_base)
- {
- Return some_another_value;
- }
- Else
- {
- // Statements;
- Recursive call;
- }
Example of recursion in C
Let's see an example to find the nth term of the Fibonacci series.
- #include<stdio.h>
- Int fibonacci(int);
- Void main ()
- {
- Int n,f;
- Printf("Enter the value of n?");
- Scanf("%d",&n);
- f = fibonacci(n);
- Printf("%d",f);
- }
- Int fibonacci (int n)
- {
- If (n==0)
- {
- Return 0;
- }
- Else if (n == 1)
- {
- Return 1;
- }
- Else
- {
- Return fibonacci(n-1)+fibonacci(n-2);
- }
- }
Output
Enter the value of n?12
144
Memory allocation of Recursive method
Each recursive call creates a new copy of that method in the memory. Once some data is returned by the method, the copy is removed from the memory. Since all the variables and other stuff declared inside function get stored in the stack, therefore a separate stack is maintained at each recursive call. Once the value is returned from the corresponding function, the stack gets destroyed. Recursion involves so much complexity in resolving and tracking the values at each recursive call. Therefore we need to maintain the stack and track the values of the variables defined in the stack.
Let us consider the following example to understand the memory allocation of the recursive functions.
- Int display (int n)
- {
- If(n == 0)
- Return 0; // terminating condition
- Else
- {
- Printf("%d",n);
- Return display(n-1); // recursive call
- }
- }
Explanation
Let us examine this recursive function for n = 4. First, all the stacks are maintained which prints the corresponding value of n until n becomes 0, Once the termination condition is reached, the stacks get destroyed one by one by returning 0 to its calling stack. Consider the following image for more information regarding the stack trace for the recursive functions.
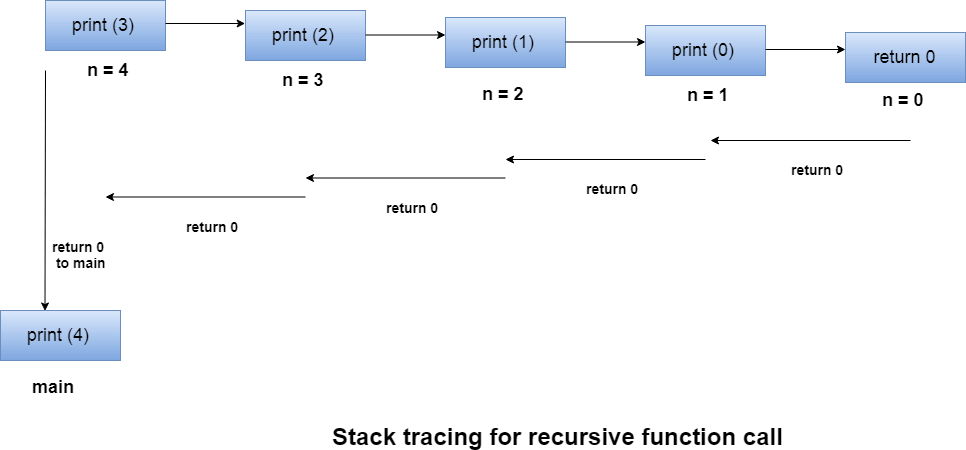
Text Books:
1. V. Rajaraman “Fundamentals of Computers” PHI publication, Fifth Edition.
2. E. Balaguruswamy,” Programming in C”, TMH Publication
3. Wei-Meng Lee” Android™ Application Development” Wiley Publication
Reference Books:
1. Pradeep K. Sinha, Priti Sinha, “Computer Fundamentals”, BPB Publication, Sixth Edition.
2. C.Xavier, Worldwide web Design with HTML, TMH Publication.
3. Yashwant Kanetkar,” Let us C”, Narosa Publication.
4. Kernighan Brian W. And Ritchie Dennis M, ‘The C Programming” Pearson Education.
5. Ed Burnette, “Hello, Android: Introducing Google's Mobile Development Platform”