Unit 3
Arrays
One dimensional Array
A one-dimensional array is a group of elements having the same datatype and same name. Individual elements are referred to using common name and unique index of the elements.
The simplest form of an array is one-dimensional-array. The array itself is given name and its elements are referred to by their subscripts. In , an array is denoted as follows:
Array_name[array_size]
Where size specifies the number of elements in the array and the subscript (also called index) value ranges from 0 through size-1.
Declare One Dimensional Array
Here is the general form to declare one dimensional array in
Data_type array_name[array_size];
Here, data_type is any valid data type, array_name is the name of the array, and array_size is the size of array. Here is an example, declaring an array named arr of int type, having maximum element size of 10 elements
Int arr[10];
Initialize One Dimensional Array
Here is the general form to initialize values to one dimensional array
Data_type array_name[array_size] = {comma_separated_element_list};
Here is an example, declaring and initializing values to the array name arr of type int, containing 10 elements
Int arr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
One Dimensional Array Example
Here are some example programs, demonstrating one dimensional array
/*One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[5] = {1, 2, 3, 4, 5};
Inti;
For(i=0; i<5; i++)
{
Cout<<"arr["<<i<<"] = "<<arr[i]<<"\n";
}
Getch();
}
Here is the sample output of this program:
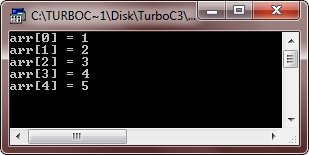
Here is another example, also demonstrating one dimension array in
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[10];
Inti;
Int sum=0, avg=0;
Cout<<"Enter 10 array elements: ";
For(i=0; i<10; i++)
{
Cin>>arr[i];
Sum = sum + arr[i];
}
Cout<<"\nThe array elements are: \n";
For(i=0; i<10; i++)
{
Cout<<arr[i]<<" ";
}
Cout<<"\n\nSum of all elements is: "<<sum;
Avg = sum/10;
Cout<<"\nAnd average is: "<<avg;
Getch();
}
Here is the sample run of the above program:
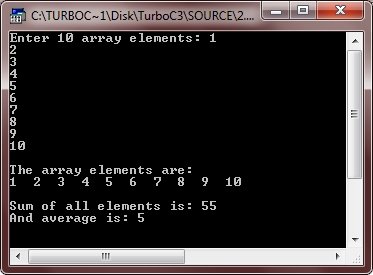
Below is another program on one-dimensional array in
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[5];
Inti, position, index;
Cout<<"Enter 5 array elements: ";
For(i=0; i<5; i++)
{
Cin>>arr[i];
}
Cout<<"\nIndex\t\tPosition";
For(i=0; i<5; i++)
{
Cout<<"\n";
Cout<<i<<" = "<<arr[i]<<"\t\t"<<i+1<<" = "<<arr[i+1];
}
Cout<<" (Garbage value/not of array)";
Cout<<"\n\nImportant - Array element can only be accessed by indexing the array\n";
Cout<<"Note - Array index always starts from 0";
Getch();
}
Below is the sample run of this program:
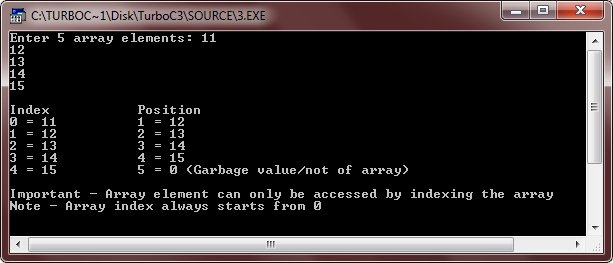
Let's take one more program, on single or one dimensional array
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[10];
Inti, position, index;
Cout<<"Enter 10 array elements: ";
For(i=0; i<10; i++)
{
Cin>>arr[i];
}
Cout<<"Accessing element at position...Enter position...";
Cin>>position;
Cout<<"\nElement present at position "<<position<<" is "<<arr[position+1];
Cout<<"\n\nAccessing element at index..Enter index..";
Cin>>index;
Cout<<"\nElement present at index "<<index<<" is "<<arr[index];
Getch();
}
Here is the sample run of the above program:
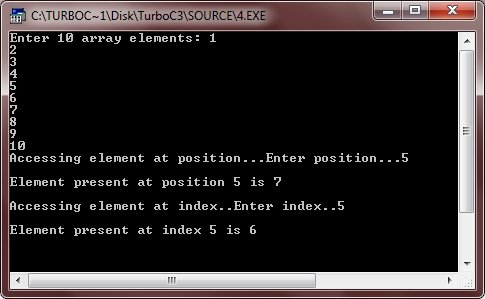
Two Dimensional Array
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns. However, 2D arrays are created to implement a relational database lookalike data structure. It provides ease of holding the bulk of data at once which can be passed to any number of functions wherever required.
Declaration of two dimensional Array in C
The syntax to declare the 2D array is given below.
- Data_type array_name[rows][columns];
Consider the following example.
- Int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Initialization of 2D Array in C
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array. The two-dimensional array can be declared and defined in the following way.
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Two-dimensional array example in C
- #include<stdio.h>
- Int main(){
- Int i=0,j=0;
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
- //traversing 2D array
- For(i=0;i<4;i++){
- For(j=0;j<3;j++){
- Printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
- }//end of j
- }//end of i
- Return 0;
- }
Output
Arr[0][0] = 1
Arr[0][1] = 2
Arr[0][2] = 3
Arr[1][0] = 2
Arr[1][1] = 3
Arr[1][2] = 4
Arr[2][0] = 3
Arr[2][1] = 4
Arr[2][2] = 5
Arr[3][0] = 4
Arr[3][1] = 5
Arr[3][2] = 6
C 2D array example: Storing elements in a matrix and printing it.
- #include <stdio.h>
- Void main ()
- {
- Int arr[3][3],i,j;
- For (i=0;i<3;i++)
- {
- For (j=0;j<3;j++)
- {
- Printf("Enter a[%d][%d]: ",i,j);
- Scanf("%d",&arr[i][j]);
- }
- }
- Printf("\n printing the elements ....\n");
- For(i=0;i<3;i++)
- {
- Printf("\n");
- For (j=0;j<3;j++)
- {
- Printf("%d\t",arr[i][j]);
- }
- }
- }
Output
Enter a[0][0]: 56
Enter a[0][1]: 10
Enter a[0][2]: 30
Enter a[1][0]: 34
Enter a[1][1]: 21
Enter a[1][2]: 34
Enter a[2][0]: 45
Enter a[2][1]: 56
Enter a[2][2]: 78
Printing the elements ....
56 10 30
34 21 34
45 56 78
Program to Add Two Matrices
Intmain(){
Int r, c, a[100][100], b[100][100], sum[100][100], i, j;
Printf("Enter the number of rows (between 1 and 100): ");
Scanf("%d", &r);
Printf("Enter the number of columns (between 1 and 100): ");
Scanf("%d", &c);
Printf("\nEnter elements of 1st matrix:\n");
For (i = 0; i< r; ++i)
For (j = 0; j < c; ++j) {
Printf("Enter element a%d%d: ", i + 1, j + 1);
Scanf("%d", &a[i][j]);
}
Printf("Enter elements of 2nd matrix:\n");
For (i = 0; i< r; ++i)
For (j = 0; j < c; ++j) {
Printf("Enter element a%d%d: ", i + 1, j + 1);
Scanf("%d", &b[i][j]);
}
// adding two matrices
For (i = 0; i< r; ++i)
For (j = 0; j < c; ++j) {
Sum[i][j] = a[i][j] + b[i][j];
}
// printing the result
Printf("\nSum of two matrices: \n");
For (i = 0; i< r; ++i)
For (j = 0; j < c; ++j) {
Printf("%d ", sum[i][j]);
If (j == c - 1) {
Printf("\n\n");
}
}
Return0;
}
Output
Enter the number of rows (between 1 and 100): 2
Enter the number of columns (between 1 and 100): 3
Enter elements of 1st matrix:
Enter element a11: 2
Enter element a12: 3
Enter element a13: 4
Enter element a21: 5
Enter element a22: 2
Enter element a23: 3
Enter elements of 2nd matrix:
Enter element a11: -4
Enter element a12: 5
Enter element a13: 3
Enter element a21: 5
Enter element a22: 6
Enter element a23: 3
Sum of two matrices:
-2 8 7
10 8 6
In this program, the user is asked to enter the number of rows r and columns c. Then, the user is asked to enter the elements of the two matrices (of order r*c).
We then added corresponding elements of two matrices and saved it in another matrix (two-dimensional array). Finally, the result is printed on the screen.
A Subprogram is a program inside any larger program that can be reused any number of times.
Characteristics of a Subprogram:
(1) A Subprogram is implemented using the Call & Return instructions in Assembly Language.
(2) The Call Instruction is present in the Main Program and the Return(Ret) Instruction is present in the subprogram itself.
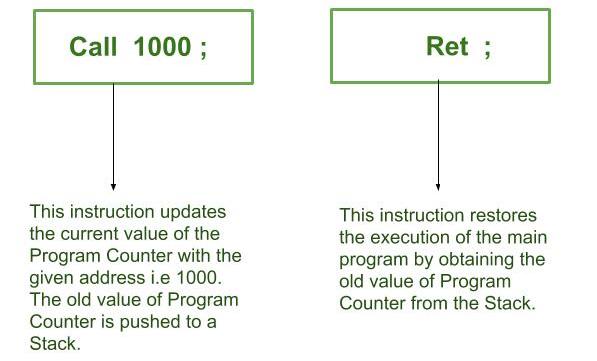
(3) It is important to note that the Main Program is suspended during the execution of any subprogram. Moreover, after the completion of the subprogram the main program executes from the next sequential address present in the Program Counter.
(4) For the implementation of any subprogram, a “Stack” is used to store the “Return Address” to the Main Program. Here, Return Address means the immediately next instruction address after the Call Instruction in the Main program. This Return Address is present inside the Program Counter. Thus during the execution of the Call Instruction, the Program Counter value is first pushed to the Stack as the Return Address and then the Program Counter value is updated to the given address in the Call Instruction . Similarly, during the execution of Return(Ret) Instruction, the value present in the stack is popped and the Program Counter value is restored for further execution of the Main Program .
(5) The Main advantage of Subprogram is that it avoids repetition of Code and allows us to reuse the same code again and again.
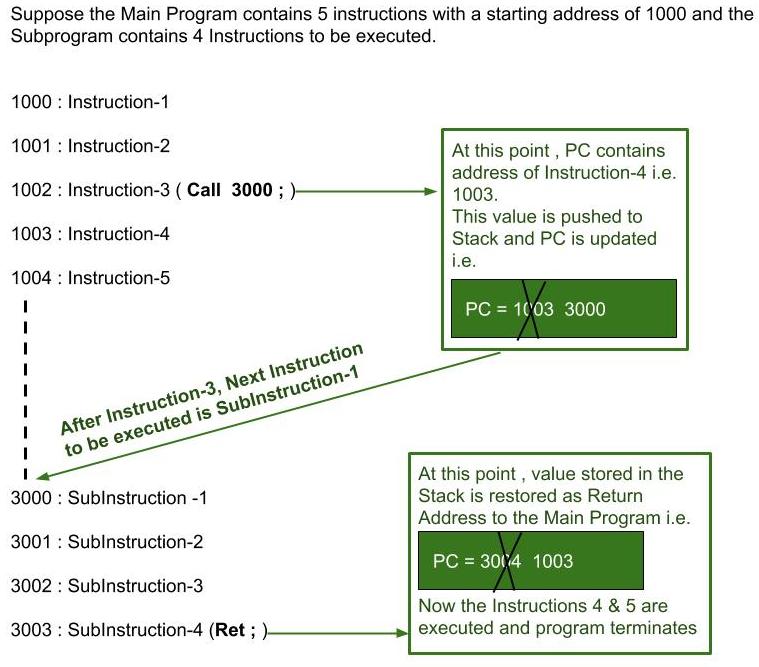
In c, we can divide a large program into the basic building blocks known as function. The function contains the set of programming statements enclosed by {}. A function can be called multiple times to provide reusability and modularity to the C program. In other words, we can say that the collection of functions creates a program. The function is also known as procedure or subroutine in other programming languages.
Advantage of functions in C
There are the following advantages of C functions.
- By using functions, we can avoid rewriting same logic/code again and again in a program.
- We can call C functions any number of times in a program and from any place in a program.
- We can track a large C program easily when it is divided into multiple functions.
- Reusability is the main achievement of C functions.
- However, Function calling is always a overhead in a C program.
Function Aspects
There are three aspects of a C function.
- Function declarationA function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type.
- Function call Function can be called from anywhere in the program. The parameter list must not differ in function calling and function declaration. We must pass the same number of functions as it is declared in the function declaration.
- Function definition It contains the actual statements which are to be executed. It is the most important aspect to which the control comes when the function is called. Here, we must notice that only one value can be returned from the function.
SN | C function aspects | Syntax |
1 | Function declaration | Return_typefunction_name (argument list); |
2 | Function call | Function_name (argument_list) |
3 | Function definition | Return_typefunction_name (argument list) {function body;} |
The syntax of creating function in c language is given below:
- Return_type function_name(data_type parameter...){
- //code to be executed
- }
Types of Functions
There are two types of functions in C programming:
- Library Functions: are the functions which are declared in the C header files such as scanf(), printf(), gets(), puts(), ceil(), floor() etc.
- User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times. It reduces the complexity of a big program and optimizes the code.
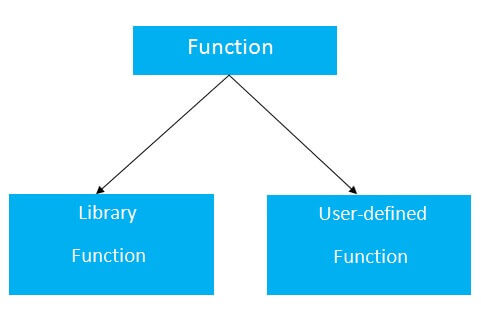
Return Value
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
Let's see a simple example of C function that doesn't return any value from the function.
Example without return value:
- Void hello(){
- Printf("hello c");
- }
If you want to return any value from the function, you need to use any data type such as int, long, char, etc. The return type depends on the value to be returned from the function.
Let's see a simple example of C function that returns int value from the function.
Example with return value:
- Int get(){
- Return 10;
- }
In the above example, we have to return 10 as a value, so the return type is int. If you want to return floating-point value (e.g., 10.2, 3.1, 54.5, etc), you need to use float as the return type of the method.
- Float get(){
- Return 10.2;
- }
Now, you need to call the function, to get the value of the function.
Different aspects of function calling
A function may or may not accept any argument. It may or may not return any value. Based on these facts, There are four different aspects of function calls.
- Function without arguments and without return value
- Function without arguments and with return value
- Function with arguments and without return value
- Function with arguments and with return value
Example for Function without argument and return value
Example 1
- #include<stdio.h>
- Void printName();
- Void main ()
- {
- Printf("Hello ");
- PrintName();
- }
- Void printName()
- {
- Printf("Javatpoint");
- }
Output
Hello Javatpoint
Example 2
- #include<stdio.h>
- Void sum();
- Void main()
- {
- Printf("\nGoing to calculate the sum of two numbers:");
- Sum();
- }
- Void sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Printf("The sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example for Function without argument and with return value
Example 1
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Int result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Result = sum();
- Printf("%d",result);
- }
- Int sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the area of the square
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Printf("Going to calculate the area of the square\n");
- Float area = square();
- Printf("The area of the square: %f\n",area);
- }
- Int square()
- {
- Float side;
- Printf("Enter the length of the side in meters: ");
- Scanf("%f",&side);
- Return side * side;
- }
Output
Going to calculate the area of the square
Enter the length of the side in meters: 10
The area of the square: 100.000000
Example for Function with argument and without return value
Example 1
- #include<stdio.h>
- Void sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Sum(a,b);
- }
- Void sum(int a, int b)
- {
- Printf("\nThe sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the average of five numbers.
- #include<stdio.h>
- Void average(int, int, int, int, int);
- Void main()
- {
- Int a,b,c,d,e;
- Printf("\nGoing to calculate the average of five numbers:");
- Printf("\nEnter five numbers:");
- Scanf("%d %d %d %d %d",&a,&b,&c,&d,&e);
- Average(a,b,c,d,e);
- }
- Void average(int a, int b, int c, int d, int e)
- {
- Float avg;
- Avg = (a+b+c+d+e)/5;
- Printf("The average of given five numbers : %f",avg);
- }
Output
Going to calculate the average of five numbers:
Enter five numbers:10
20
30
40
50
The average of given five numbers: 30.000000
Example for Function with argument and with return value
Example 1
- #include<stdio.h>
- Int sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Result = sum(a,b);
- Printf("\nThe sum is : %d",result);
- }
- Int sum(int a, int b)
- {
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers:10
20
The sum is : 30
Example 2: Program to check whether a number is even or odd
- #include<stdio.h>
- Int even_odd(int);
- Void main()
- {
- Int n,flag=0;
- Printf("\nGoing to check whether a number is even or odd");
- Printf("\nEnter the number: ");
- Scanf("%d",&n);
- Flag = even_odd(n);
- If(flag == 0)
- {
- Printf("\nThe number is odd");
- }
- Else
- {
- Printf("\nThe number is even");
- }
- }
- Int even_odd(int n)
- {
- If(n%2 == 0)
- {
- Return 1;
- }
- Else
- {
- Return 0;
- }
- }
Output
Going to check whether a number is even or odd
Enter the number: 100
The number is even
C Library Functions
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers. All C standard library functions are defined inside the different header files saved with the extension .h. We need to include these header files in our program to make use of the library functions defined in such header files. For example, To use the library functions such as printf/scanf we need to include stdio.h in our program which is a header file that contains all the library functions regarding standard input/output.
The list of mostly used header files is given in the following table.
SN | Header file | Description |
1 | Stdio.h | This is a standard input/output header file. It contains all the library functions regarding standard input/output. |
2 | Conio.h | This is a console input/output header file. |
3 | String.h | It contains all string related library functions like gets(), puts(),etc. |
4 | Stdlib.h | This header file contains all the general library functions like malloc(), calloc(), exit(), etc. |
5 | Math.h | This header file contains all the math operations related functions like sqrt(), pow(), etc. |
6 | Time.h | This header file contains all the time-related functions. |
7 | Ctype.h | This header file contains all character handling functions. |
8 | Stdarg.h | Variable argument functions are defined in this header file. |
9 | Signal.h | All the signal handling functions are defined in this header file. |
10 | Setjmp.h | This file contains all the jump functions. |
11 | Locale.h | This file contains locale functions. |
12 | Errno.h | This file contains error handling functions. |
13 | Assert.h | This file contains diagnostics functions. |
There are two methods to pass the data into the function in C language, i.e., call by value and call by reference.
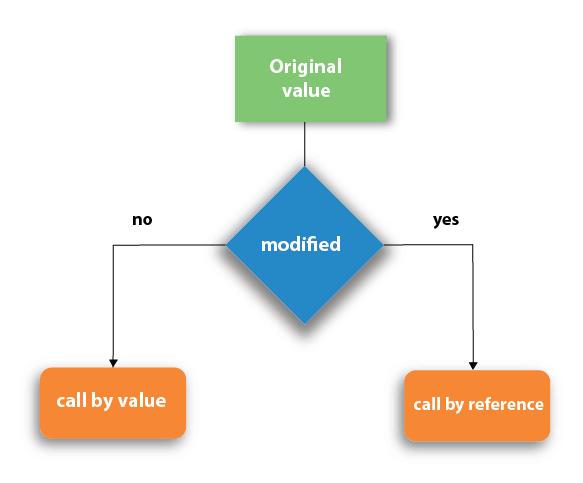
Let's understand call by value and call by reference in c language one by one.
Call by value in C
- In call by value method, the value of the actual parameters is copied into the formal parameters. In other words, we can say that the value of the variable is used in the function call in the call by value method.
- In call by value method, we can not modify the value of the actual parameter by the formal parameter.
- In call by value, different memory is allocated for actual and formal parameters since the value of the actual parameter is copied into the formal parameter.
- The actual parameter is the argument which is used in the function call whereas formal parameter is the argument which is used in the function definition.
Let's try to understand the concept of call by value in c language by the example given below:
- #include<stdio.h>
- Void change(int num) {
- Printf("Before adding value inside function num=%d \n",num);
- Num=num+100;
- Printf("After adding value inside function num=%d \n", num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(x);//passing value in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=100
Call by Value Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int , int); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(a,b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The value of actual parameters do not change by changing the formal parameters in call by value, a = 10, b = 20
- }
- Void swap (int a, int b)
- {
- Int temp;
- Temp = a;
- a=b;
- b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",a,b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
Call by reference in C
- In call by reference, the address of the variable is passed into the function call as the actual parameter.
- The value of the actual parameters can be modified by changing the formal parameters since the address of the actual parameters is passed.
- In call by reference, the memory allocation is similar for both formal parameters and actual parameters. All the operations in the function are performed on the value stored at the address of the actual parameters, and the modified value gets stored at the same address.
Consider the following example for the call by reference.
- #include<stdio.h>
- Void change(int *num) {
- Printf("Before adding value inside function num=%d \n",*num);
- (*num) += 100;
- Printf("After adding value inside function num=%d \n", *num);
- }
- Int main() {
- Int x=100;
- Printf("Before function call x=%d \n", x);
- Change(&x);//passing reference in function
- Printf("After function call x=%d \n", x);
- Return 0;
- }
Output
Before function call x=100
Before adding value inside function num=100
After adding value inside function num=200
After function call x=200
Call by reference Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int *, int *); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(&a,&b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The values of actual parameters do change in call by reference, a = 10, b = 20
- }
- Void swap (int *a, int *b)
- {
- Int temp;
- Temp = *a;
- *a=*b;
- *b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",*a,*b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 20, b = 10
Difference between call by value and call by reference in c
No. | Call by value | Call by reference |
1 | A copy of the value is passed into the function | An address of value is passed into the function |
2 | Changes made inside the function is limited to the function only. The values of the actual parameters do not change by changing the formal parameters. | Changes made inside the function validate outside of the function also. The values of the actual parameters do change by changing the formal parameters. |
3 | Actual and formal arguments are created at the different memory location | Actual and formal arguments are created at the same memory location |
The pointer in C language is a variable which stores the address of another variable. This variable can be of type int, char, array, function, or any other pointer. The size of the pointer depends on the architecture. However, in 32-bit architecture the size of a pointer is 2 byte.
Consider the following example to define a pointer which stores the address of an integer.
- Int n = 10;
- Int* p = &n; // Variable p of type pointer is pointing to the address of the variable n of type integer.
Declaring a pointer
The pointer in c language can be declared using * (asterisk symbol). It is also known as indirection pointer used to dereference a pointer.
- Int *a;//pointer to int
- Char *c;//pointer to char
Pointer Example
An example of using pointers to print the address and value is given below.
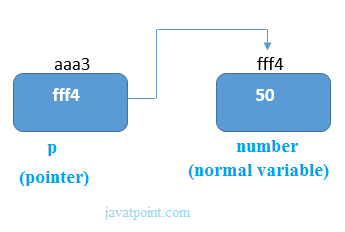
As you can see in the above figure, pointer variable stores the address of number variable, i.e., fff4. The value of number variable is 50. But the address of pointer variable p is aaa3.
By the help of * (indirection operator), we can print the value of pointer variable p.
Let's see the pointer example as explained for the above figure.
- #include<stdio.h>
- Int main(){
- Int number=50;
- Int *p;
- p=&number;//stores the address of number variable
- Printf("Address of p variable is %x \n",p); // p contains the address of the number therefore printing p gives the address of number.
- Printf("Value of p variable is %d \n",*p); // As we know that * is used to dereference a pointer therefore if we print *p, we will get the value stored at the address contained by p.
- Return 0;
- }
Output
Address of number variable is fff4
Address of p variable is fff4
Value of p variable is 50
Pointer to array
- Int arr[10];
- Int *p[10]=&arr; // Variable p of type pointer is pointing to the address of an integer array arr.
Pointer to a function
- Void show (int);
- Void(*p)(int) = &display; // Pointer p is pointing to the address of a function
Pointer to structure
- Struct st {
- Int i;
- Float f;
- }ref;
- Struct st *p = &ref;
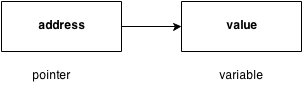
Advantage of pointer
1) Pointer reduces the code and improves the performance, it is used to retrieving strings, trees, etc. and used with arrays, structures, and functions.
2) We can return multiple values from a function using the pointer.
3) It makes you able to access any memory location in the computer's memory.
Usage of pointer
There are many applications of pointers in c language.
1) Dynamic memory allocation
In c language, we can dynamically allocate memory using malloc() and calloc() functions where the pointer is used.
2) Arrays, Functions, and Structures
Pointers in c language are widely used in arrays, functions, and structures. It reduces the code and improves the performance.
Address Of (&) Operator
The address of operator '&' returns the address of a variable. But, we need to use %u to display the address of a variable.
- #include<stdio.h>
- Int main(){
- Int number=50;
- Printf("value of number is %d, address of number is %u",number,&number);
- Return 0;
- }
Output
Value of number is 50, address of number is fff4
NULL Pointer
A pointer that is not assigned any value but NULL is known as the NULL pointer. If you don't have any address to be specified in the pointer at the time of declaration, you can assign NULL value. It will provide a better approach.
Int *p=NULL;
In the most libraries, the value of the pointer is 0 (zero).
Pointer Program to swap two numbers without using the 3rd variable.
- #include<stdio.h>
- Int main(){
- Int a=10,b=20,*p1=&a,*p2=&b;
- Printf("Before swap: *p1=%d *p2=%d",*p1,*p2);
- *p1=*p1+*p2;
- *p2=*p1-*p2;
- *p1=*p1-*p2;
- Printf("\nAfter swap: *p1=%d *p2=%d",*p1,*p2);
- Return 0;
- }
Output
Before swap: *p1=10 *p2=20
After swap: *p1=20 *p2=10
Reading complex pointers
There are several things which must be taken into the consideration while reading the complex pointers in C. Lets see the precedence and associativity of the operators which are used regarding pointers.
Operator | Precedence | Associativity |
(), [] | 1 | Left to right |
*, identifier | 2 | Right to left |
Data type | 3 | - |
Here,we must notice that,
- (): This operator is a bracket operator used to declare and define the function.
- []: This operator is an array subscript operator
- * : This operator is a pointer operator.
- Identifier: It is the name of the pointer. The priority will always be assigned to this.
- Data type: Data type is the type of the variable to which the pointer is intended to point. It also includes the modifier like signed int, long, etc).
How to read the pointer: int (*p)[10].
To read the pointer, we must see that () and [] have the equal precedence. Therefore, their associativity must be considered here. The associativity is left to right, so the priority goes to ().
Inside the bracket (), pointer operator * and pointer name (identifier) p have the same precedence. Therefore, their associativity must be considered here which is right to left, so the priority goes to p, and the second priority goes to *.
Assign the 3rd priority to [] since the data type has the last precedence. Therefore the pointer will look like following.
- Char -> 4
- * -> 2
- p -> 1
- [10] -> 3
The pointer will be read as p is a pointer to an array of integers of size 10.
Example
How to read the following pointer?
- Int (*p)(int (*)[2], int (*)void))
Explanation
This pointer will be read as p is a pointer to such function which accepts the first parameter as the pointer to a one-dimensional array of integers of size two and the second parameter as the pointer to a function which parameter is void and return type is the integer.
Relationship between Arrays and Pointers
An array is a block of sequential data. Let's write a program to print addresses of array elements.
Intmain(){
Int x[4];
Inti;
For(i = 0; i<4; ++i) {
Printf("&x[%d] = %p\n", i, &x[i]);
}
Printf("Address of array x: %p", x);
Return0;
}
Output
&x[0] = 1450734448
&x[1] = 1450734452
&x[2] = 1450734456
&x[3] = 1450734460
Address of array x: 1450734448
There is a difference of 4 bytes between two consecutive elements of array x. It is because the size of int is 4 bytes (on our compiler).
Notice that, the address of &x[0] and x is the same. It's because the variable name x points to the first element of the array.
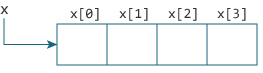
From the above example, it is clear that &x[0] is equivalent to x. And, x[0] is equivalent to *x.
Similarly,
- &x[1] is equivalent to x+1 and x[1] is equivalent to *(x+1).
- &x[2] is equivalent to x+2 and x[2] is equivalent to *(x+2).
- ...
- Basically, &x[i] is equivalent to x+i and x[i] is equivalent to *(x+i).
Example 1: Pointers and Arrays
Intmain(){
Inti, x[6], sum = 0;
Printf("Enter 6 numbers: ");
For(i = 0; i<6; ++i) {
// Equivalent to scanf("%d", &x[i]);
Scanf("%d", x+i);
// Equivalent to sum += x[i]
Sum += *(x+i);
}
Printf("Sum = %d", sum);
Return0;
}
When you run the program, the output will be:
Enter 6 numbers: 2
3
4
4
12
4
Sum = 29
Here, we have declared an array x of 6 elements. To access elements of the array, we have used pointers.
In most contexts, array names decay to pointers. In simple words, array names are converted to pointers. That's the reason why you can use pointers to access elements of arrays. However, you should remember that pointers and arrays are not the same.
There are a few cases where array names don't decay to pointers.
Example 2: Arrays and Pointers
Intmain(){
Int x[5] = {1, 2, 3, 4, 5};
Int* ptr;
// ptr is assigned the address of the third element
Ptr = &x[2];
Printf("*ptr = %d \n", *ptr); // 3
Printf("*(ptr+1) = %d \n", *(ptr+1)); // 4
Printf("*(ptr-1) = %d", *(ptr-1)); // 2
Return0;
}
When you run the program, the output will be:
*ptr = 3
*(ptr+1) = 4
*(ptr-1) = 2
In this example, &x[2], the address of the third element, is assigned to the ptr pointer. Hence, 3 was displayed when we printed *ptr.
And, printing *(ptr+1) gives us the fourth element. Similarly, printing *(ptr-1) gives us the second element.
C programming allows passing a pointer to a function. To do so, simply declare the function parameter as a pointer type.
Following is a simple example where we pass an unsigned long pointer to a function and change the value inside the function which reflects back in the calling function −
#include <stdio.h>
#include <time.h>
VoidgetSeconds(unsigned long *par);
Int main () {
Unsigned long sec;
GetSeconds(&sec );
/* print the actual value */
Printf("Number of seconds: %ld\n", sec );
Return 0;
}
VoidgetSeconds(unsigned long *par) {
/* get the current number of seconds */
*par = time( NULL );
Return;
}
When the above code is compiled and executed, it produces the following result −
Number of seconds :1294450468
The function, which can accept a pointer, can also accept an array as shown in the following example −
#include <stdio.h>
/* function declaration */
DoublegetAverage(int *arr, int size);
Int main () {
/* an int array with 5 elements */
Int balance[5] = {1000, 2, 3, 17, 50};
Doubleavg;
/* pass pointer to the array as an argument */
Avg = getAverage( balance, 5 ) ;
/* output the returned value */
Printf("Average value is: %f\n", avg );
Return 0;
}
DoublegetAverage(int *arr, int size) {
Inti, sum = 0;
Doubleavg;
For (i = 0; i< size; ++i) {
Sum += arr[i];
}
Avg = (double)sum / size;
Returnavg;
}
When the above code is compiled together and executed, it produces the following result −
Average value is: 214.40000
Array and pointers are closely related to each other. In C++, the name of an array is considered às a pointer, i.e., the name of an array contains the address of an element. C++ considers the array name as the address of the first element. For example, if we create an array, i.e., marks which hold the 20 values of integer type, then marks will contain the address of first element, i.e., marks[0]. Therefore, we can say that array name (marks) is a pointer which is holding the address of the first element of an array.
Let's understand this scenario through an example.
- #include <iostream>
- Using namespace std;
- Int main()
- {
- Int *ptr; // integer pointer declaration
- Int marks[10]; // marks array declaration
- Std::cout << "Enter the elements of an array :" << std::endl;
- For(int i=0;i<10;i++)
- {
- Cin>>marks[i];
- }
- Ptr=marks; // both marks and ptr pointing to the same element..
- Std::cout << "The value of *ptr is :" <<*ptr<< std::endl;
- Std::cout << "The value of *marks is :" <<*marks<<std::endl;
- }
In the above code, we declare an integer pointer and an array of integer type. We assign the address of marks to the ptr by using the statement ptr=marks; it means that both the variables 'marks' and 'ptr' point to the same element, i.e., marks[0]. When we try to print the values of *ptr and *marks, then it comes out to be same. Hence, it is proved that the array name stores the address of the first element of an array.
Output
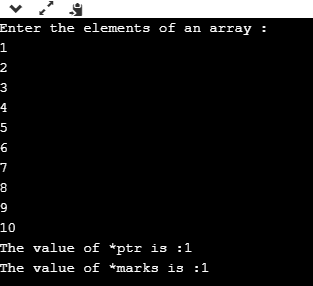
Array of Pointers
An array of pointers is an array that consists of variables of pointer type, which means that the variable is a pointer addressing to some other element. Suppose we create an array of pointer holding 5 integer pointers; then its declaration would look like:
- Int *ptr[5]; // array of 5 integer pointer.
In the above declaration, we declare an array of pointer named as ptr, and it allocates 5 integer pointers in memory.
The element of an array of a pointer can also be initialized by assigning the address of some other element. Let's observe this case through an example.
- Int a; // variable declaration.
- Ptr[2] = &a;
In the above code, we are assigning the address of 'a' variable to the third element of an array 'ptr'.
We can also retrieve the value of 'a' be dereferencing the pointer.
- *ptr[2];
Let's understand through an example.
- #include <iostream>
- Using namespace std;
- Int main()
- {
- Int ptr1[5]; // integer array declaration
- Int *ptr2[5]; // integer array of pointer declaration
- Std::cout << "Enter five numbers :" << std::endl;
- For(int i=0;i<5;i++)
- {
- Std::cin >> ptr1[i];
- }
- For(int i=0;i<5;i++)
- {
- Ptr2[i]=&ptr1[i];
- }
- // printing the values of ptr1 array
- Std::cout << "The values are" << std::endl;
- For(int i=0;i<5;i++)
- {
- Std::cout << *ptr2[i] << std::endl;
- }
- }
In the above code, we declare an array of integer type and an array of integer pointers. We have defined the 'for' loop, which iterates through the elements of an array 'ptr1', and on each iteration, the address of element of ptr1 at index 'i' gets stored in the ptr2 at index 'i'.
Output
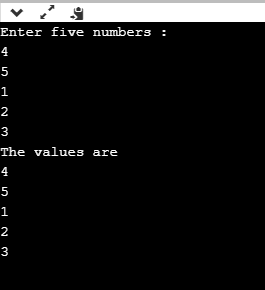
Till now, we have learnt the array of pointers to an integer. Now, we will see how to create the array of pointers to strings.
Array of Pointer to Strings
An array of pointer to strings is an array of character pointers that holds the address of the first character of a string or we can say the base address of a string.
The following are the differences between an array of pointers to string and two-dimensional array of characters:
- An array of pointers to string is more efficient than the two-dimensional array of characters in case of memory consumption because an array of pointer to strings consumes less memory than the two-dimensional array of characters to store the strings.
- In an array of pointers, the manipulation of strings is comparatively easier than in the case of 2d array. We can also easily change the position of the strings by using the pointers.
Let's see how to declare the array of pointers to string.
First, we declare the array of pointer to string:
- Char *names[5] = {"john",
- "Peter",
- "Marco",
- "Devin",
- "Ronan"};
In the above code, we declared an array of pointer names as 'names' of size 5. In the above case, we have done the initialization at the time of declaration, so we do not need to mention the size of the array of a pointer. The above code can be re-written as:
- Char *names[ ] = {"john",
- "Peter",
- "Marco",
- "Devin",
- "Ronan"};
In the above case, each element of the 'names' array is a string literal, and each string literal would hold the base address of the first character of a string. For example, names[0] contains the base address of "john", names[1] contains the base address of "Peter", and so on. It is not guaranteed that all the string literals will be stored in the contiguous memory location, but the characters of a string literal are stored in a contiguous memory location.
Let's create a simple example.
- #include <iostream>
- Using namespace std;
- Int main()
- {
- Char *names[5] = {"john",
- "Peter",
- "Marco",
- "Devin",
- "Ronan"};
- For(int i=0;i<5;i++)
- {
- Std::cout << names[i] << std::endl;
- }
- Return 0;
- }
In the above code, we have declared an array of char pointer holding 5 string literals, and the first character of each string is holding the base address of the string.
Output
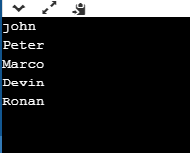
In C, there are various general problems which requires passing more than one variable of the same type to a function. For example, consider a function which sorts the 10 elements in ascending order. Such a function requires 10 numbers to be passed as the actual parameters from the main function. Here, instead of declaring 10 different numbers and then passing into the function, we can declare and initialize an array and pass that into the function. This will resolve all the complexity since the function will now work for any number of values.
As we know that the array_name contains the address of the first element. Here, we must notice that we need to pass only the name of the array in the function which is intended to accept an array. The array defined as the formal parameter will automatically refer to the array specified by the array name defined as an actual parameter.
Consider the following syntax to pass an array to the function.
- Functionname(arrayname);//passing array
Methods to declare a function that receives an array as an argument
There are 3 ways to declare the function which is intended to receive an array as an argument.
First way:
- Return_type function(type arrayname[])
Declaring blank subscript notation [] is the widely used technique.
Second way:
- Return_type function(type arrayname[SIZE])
Optionally, we can define size in subscript notation [].
Third way:
- Return_type function(type *arrayname)
You can also use the concept of a pointer. In pointer chapter, we will learn about it.
C language passing an array to function example
- #include<stdio.h>
- Int minarray(int arr[],int size){
- Int min=arr[0];
- Int i=0;
- For(i=1;i<size;i++){
- If(min>arr[i]){
- Min=arr[i];
- }
- }//end of for
- Return min;
- }//end of function
- Int main(){
- Int i=0,min=0;
- Int numbers[]={4,5,7,3,8,9};//declaration of array
- Min=minarray(numbers,6);//passing array with size
- Printf("minimum number is %d \n",min);
- Return 0;
- }
Output
Minimum number is 3
C function to sort the array
- #include<stdio.h>
- Void Bubble_Sort(int[]);
- Void main ()
- {
- Int arr[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Bubble_Sort(arr);
- }
- Void Bubble_Sort(int a[]) //array a[] points to arr.
- {
- Int i, j,temp;
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] < a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Output
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Returning array from the function
As we know that, a function can not return more than one value. However, if we try to write the return statement as return a, b, c; to return three values (a,b,c), the function will return the last mentioned value which is c in our case. In some problems, we may need to return multiple values from a function. In such cases, an array is returned from the function.
Returning an array is similar to passing the array into the function. The name of the array is returned from the function. To make a function returning an array, the following syntax is used.
- Int * Function_name() {
- //some statements;
- Return array_type;
- }
To store the array returned from the function, we can define a pointer which points to that array. We can traverse the array by increasing that pointer since pointer initially points to the base address of the array. Consider the following example that contains a function returning the sorted array.
- #include<stdio.h>
- Int* Bubble_Sort(int[]);
- Void main ()
- {
- Int arr[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- Int *p = Bubble_Sort(arr), i;
- Printf("printing sorted elements ...\n");
- For(i=0;i<10;i++)
- {
- Printf("%d\n",*(p+i));
- }
- }
- Int* Bubble_Sort(int a[]) //array a[] points to arr.
- {
- Int i, j,temp;
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] < a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Return a;
- }
Output
Printing Sorted Element List ...
7
9
10
12
23
23
34
44
78
101
Text Books:
[T1] Herbert Schildt, ―C: The Complete Reference‖, OsbourneMcgraw Hill, 4th Edition, 2002.
[T2] Forouzan Behrouz A. ―Computer Science: A Structured Programming Approach Using C, Cengage Learning 2/e
Reference Books:
[R1] Kernighan & Ritchie, ―C Programming Language‖, The (Ansi C version), PHI, 2/e
[R2] K.R Venugopal, ―Mastering C ‖, TMH
[R3] R.S. Salaria "Application Programming in C " Khanna Publishers4/e
[R4] YashwantKanetkar― Test your C Skills ‖ , BPB Publications
[R5] http://www.codeblocks.org/
[R6] http://gcc.gnu.org/
[R7] Programming in ANSI C, E. Balagurusamy; Mc Graw Hill, 6th Edition.