Unit 2
Numbers
Algorithm
What is an Algorithm?
An algorithm is a process or a set of rules required to perform calculations or some other problem-solving operations especially by a computer. The formal definition of an algorithm is that it contains the finite set of instructions which are being carried in a specific order to perform the specific task. It is not the complete program or code; it is just a solution (logic) of a problem, which can be represented either as an informal description using a Flowchart or Pseudocode.
Characteristics of an Algorithm
The following are the characteristics of an algorithm:
- Input: An algorithm has some input values. We can pass 0 or some input value to an algorithm.
- Output: We will get 1 or more output at the end of an algorithm.
- Unambiguity: An algorithm should be unambiguous which means that the instructions in an algorithm should be clear and simple.
- Finiteness: An algorithm should have finiteness. Here, finiteness means that the algorithm should contain a limited number of instructions, i.e., the instructions should be countable.
- Effectiveness: An algorithm should be effective as each instruction in an algorithm affects the overall process.
- Language independent: An algorithm must be language-independent so that the instructions in an algorithm can be implemented in any of the languages with the same output.
Dataflow of an Algorithm
- Problem: A problem can be a real-world problem or any instance from the real-world problem for which we need to create a program or the set of instructions. The set of instructions is known as an algorithm.
- Algorithm: An algorithm will be designed for a problem which is a step by step procedure.
- Input: After designing an algorithm, the required and the desired inputs are provided to the algorithm.
- Processing unit: The input will be given to the processing unit, and the processing unit will produce the desired output.
- Output: The output is the outcome or the result of the program.
Why do we need Algorithms?
We need algorithms because of the following reasons:
- Scalability: It helps us to understand the scalability. When we have a big real-world problem, we need to scale it down into small-small steps to easily analyze the problem.
- Performance: The real-world is not easily broken down into smaller steps. If the problem can be easily broken into smaller steps means that the problem is feasible.
Let's understand the algorithm through a real-world example. Suppose we want to make a lemon juice, so following are the steps required to make a lemon juice:
Step 1: First, we will cut the lemon into half.
Step 2: Squeeze the lemon as much you can and take out its juice in a container.
Step 3: Add two tablespoon sugar in it.
Step 4: Stir the container until the sugar gets dissolved.
Step 5: When sugar gets dissolved, add some water and ice in it.
Step 6: Store the juice in a fridge for 5 to minutes.
Step 7: Now, it's ready to drink.
The above real-world can be directly compared to the definition of the algorithm. We cannot perform the step 3 before the step 2, we need to follow the specific order to make lemon juice. An algorithm also says that each and every instruction should be followed in a specific order to perform a specific task.
Now we will look an example of an algorithm in programming.
We will write an algorithm to add two numbers entered by the user.
The following are the steps required to add two numbers entered by the user:
Step 1: Start
Step 2: Declare three variables a, b, and sum.
Step 3: Enter the values of a and b.
Step 4: Add the values of a and b and store the result in the sum variable, i.e., sum=a+b.
Step 5: Print sum
Step 6: Stop
Factors of an Algorithm
The following are the factors that we need to consider for designing an algorithm:
- Modularity: If any problem is given and we can break that problem into small-small modules or small-small steps, which is a basic definition of an algorithm, it means that this feature has been perfectly designed for the algorithm.
- Correctness: The correctness of an algorithm is defined as when the given inputs produce the desired output, which means that the algorithm has been designed algorithm. The analysis of an algorithm has been done correctly.
- Maintainability: Here, maintainability means that the algorithm should be designed in a very simple structured way so that when we redefine the algorithm, no major change will be done in the algorithm.
- Functionality: It considers various logical steps to solve the real-world problem.
- Robustness: Robustness means that how an algorithm can clearly define our problem.
- User-friendly: If the algorithm is not user-friendly, then the designer will not be able to explain it to the programmer.
- Simplicity: If the algorithm is simple then it is easy to understand.
- Extensibility: If any other algorithm designer or programmer wants to use your algorithm then it should be extensible.
Importance of Algorithms
- Theoretical importance: When any real-world problem is given to us and we break the problem into small-small modules. To break down the problem, we should know all the theoretical aspects.
- Practical importance: As we know that theory cannot be completed without the practical implementation. So, the importance of algorithm can be considered as both theoretical and practical.
Issues of Algorithms
The following are the issues that come while designing an algorithm:
- How to design algorithms: As we know that an algorithm is a step-by-step procedure so we must follow some steps to design an algorithm.
- How to analyze algorithm efficiency
Approaches of Algorithm
The following are the approaches used after considering both the theoretical and practical importance of designing an algorithm:
- Brute force algorithm: The general logic structure is applied to design an algorithm. It is also known as an exhaustive search algorithm that searches all the possibilities to provide the required solution. Such algorithms are of two types:
- Optimizing: Finding all the solutions of a problem and then take out the best solution or if the value of the best solution is known then it will terminate if the best solution is known.
- Sacrificing: As soon as the best solution is found, then it will stop.
- Divide and conquer: It is a very implementation of an algorithm. It allows you to design an algorithm in a step-by-step variation. It breaks down the algorithm to solve the problem in different methods. It allows you to break down the problem into different methods, and valid output is produced for the valid input. This valid output is passed to some other function.
- Greedy algorithm: It is an algorithm paradigm that makes an optimal choice on each iteration with the hope of getting the best solution. It is easy to implement and has a faster execution time. But, there are very rare cases in which it provides the optimal solution.
- Dynamic programming: It makes the algorithm more efficient by storing the intermediate results. It follows five different steps to find the optimal solution for the problem:
- It breaks down the problem into a subproblem to find the optimal solution.
- After breaking down the problem, it finds the optimal solution out of these subproblems.
- Stores the result of the subproblems is known as memorization.
- Reuse the result so that it cannot be recomputed for the same subproblems.
- Finally, it computes the result of the complex program.
- Branch and Bound Algorithm: The branch and bound algorithm can be applied to only integer programming problems. This approach divides all the sets of feasible solutions into smaller subsets. These subsets are further evaluated to find the best solution.
- Randomized Algorithm: As we have seen in a regular algorithm, we have predefined input and required output. Those algorithms that have some defined set of inputs and required output, and follow some described steps are known as deterministic algorithms. What happens that when the random variable is introduced in the randomized algorithm?. In a randomized algorithm, some random bits are introduced by the algorithm and added in the input to produce the output, which is random in nature. Randomized algorithms are simpler and efficient than the deterministic algorithm.
- Backtracking: Backtracking is an algorithmic technique that solves the problem recursively and removes the solution if it does not satisfy the constraints of a problem.
The major categories of algorithms are given below:
- Sort: Algorithm developed for sorting the items in a certain order.
- Search: Algorithm developed for searching the items inside a data structure.
- Delete: Algorithm developed for deleting the existing element from the data structure.
- Insert: Algorithm developed for inserting an item inside a data structure.
- Update: Algorithm developed for updating the existing element inside a data structure.
Algorithm Analysis
The algorithm can be analyzed in two levels, i.e., first is before creating the algorithm, and second is after creating the algorithm. The following are the two analysis of an algorithm:
- Priori Analysis: Here, priori analysis is the theoretical analysis of an algorithm which is done before implementing the algorithm. Various factors can be considered before implementing the algorithm like processor speed, which has no effect on the implementation part.
- Posterior Analysis: Here, posterior analysis is a practical analysis of an algorithm. The practical analysis is achieved by implementing the algorithm using any programming language. This analysis basically evaluate that how much running time and space taken by the algorithm.
Algorithm Complexity
The performance of the algorithm can be measured in two factors:
- Time complexity: The time complexity of an algorithm is the amount of time required to complete the execution. The time complexity of an algorithm is denoted by the big O notation. Here, big O notation is the asymptotic notation to represent the time complexity. The time complexity is mainly calculated by counting the number of steps to finish the execution. Let's understand the time complexity through an example.
- Sum=0;
- // Suppose we have to calculate the sum of n numbers.
- For i=1 to n
- Sum=sum+i;
- // when the loop ends then sum holds the sum of the n numbers
- Return sum;
In the above code, the time complexity of the loop statement will be atleast n, and if the value of n increases, then the time complexity also increases. While the complexity of the code, i.e., return sum will be constant as its value is not dependent on the value of n and will provide the result in one step only. We generally consider the worst-time complexity as it is the maximum time taken for any given input size.
- Space complexity: An algorithm's space complexity is the amount of space required to solve a problem and produce an output. Similar to the time complexity, space complexity is also expressed in big O notation.
For an algorithm, the space is required for the following purposes:
- To store program instructions
- To store constant values
- To store variable values
- To track the function calls, jumping statements, etc.
Auxiliary space: The extra space required by the algorithm, excluding the input size, is known as an auxiliary space. The space complexity considers both the spaces, i.e., auxiliary space, and space used by the input.
So,
Space complexity = Auxiliary space + Input size.
Types of Algorithms
The following are the types of algorithm:
- Search Algorithm
- Sort Algorithm
Search Algorithm
On each day, we search for something in our day to day life. Similarly, with the case of computer, huge data is stored in a computer that whenever the user asks for any data then the computer searches for that data in the memory and provides that data to the user. There are mainly two techniques available to search the data in an array:
- Linear search
- Binary search
Linear Search
Linear search is a very simple algorithm that starts searching for an element or a value from the beginning of an array until the required element is not found. It compares the element to be searched with all the elements in an array, if the match is found, then it returns the index of the element else it returns -1. This algorithm can be implemented on the unsorted list.
Binary Search
A Binary algorithm is the simplest algorithm that searches the element very quickly. It is used to search the element from the sorted list. The elements must be stored in sequential order or the sorted manner to implement the binary algorithm. Binary search cannot be implemented if the elements are stored in a random manner. It is used to find the middle element of the list.
Sorting Algorithms
Sorting algorithms are used to rearrange the elements in an array or a given data structure either in an ascending or descending order. The comparison operator decides the new order of the elements.
Why do we need a sorting algorithm?
- An efficient sorting algorithm is required for optimizing the efficiency of other algorithms like binary search algorithm as a binary search algorithm requires an array to be sorted in a particular order, mainly in ascending order.
- It produces information in a sorted order, which is a human-readable format.
- Searching a particular element in a sorted list is faster than the unsorted list.
Flowchart
Flowchart could be a delineate illustration of sequence of logical steps of a program. Flowcharts use easy geometric shapes to depict processes and arrows to indicate relationships and process/data flow.
Flowchart Symbols
Here is a chart for some of the common symbols used in drawing flowcharts.
Symbol | Symbol Name | Purpose |
![]() | Start/Stop | Used at the beginning and end of the algorithm to show start and end of the program. |
![]() | Process | Indicates processes like mathematical operations. |
![]() | Input/ Output | Used for denoting program inputs and outputs. |
![]() | Decision | Stands for decision statements in a program, where answer is usually Yes or No. |
![]() | Arrow | Shows relationships between different shapes. |
![]() | On-page Connector | Connects two or more parts of a flowchart, which are on the same page. |
![]() | Off-page Connector | Connects two parts of a flowchart which are spread over different pages. |
Guidelines for Developing Flowcharts
These area unit some points to stay in mind whereas developing a flow chart flow chart
• Flowchart will have only 1 begin and one stop image
• On-page connectors area unit documented victimization numbers
• Off-page connectors area unit documented victimization alphabets
• General flow of processes is prime to bottom or left to right
• Arrows mustn't cross one another
Example Flowcharts
Here is the flowchart for going to the market to purchase a pen.
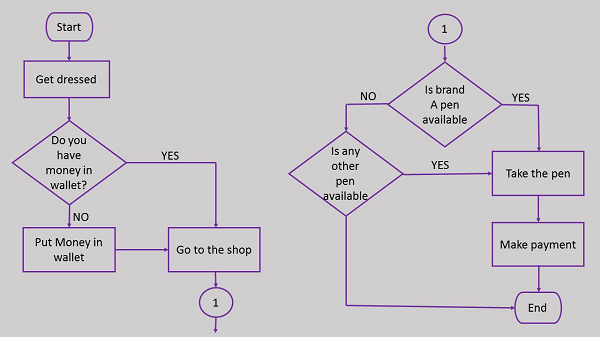
Here is a flowchart to calculate the average of two numbers.
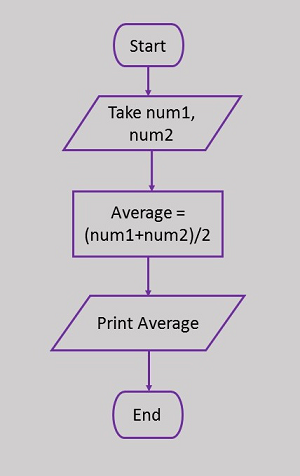
What is Python?
Python may be a programing language that's straightforward to find out, nonetheless powerful and versatile. Several skilled code engineers use Python daily to try and do their job, for each giant comes and little ones. Python is free, open supply, and it runs on Windows, Mac, and Linux, in addition as several different operational systems. It is a nice language if you would like to start out learning the way to write pc programs.
What Do I Need?
To effectively use Python on Windows, you would like some items of software:
• Python
• Cygwin
• Sublime Text or another text editor
Let's handle them one at a time.
Installing Python
Download the version of Python that you simply wish. In these examples, i am downloading Python three.2.3.
Run the installer, and let it install to the default location: in this case, C:\Python32\.
That was easy!
Installing Cygwin
This is a bit more complicated. First, go to the Cygwin homepage, download setup.exe, and run it.
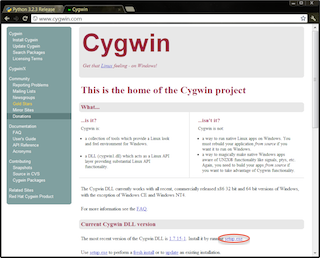
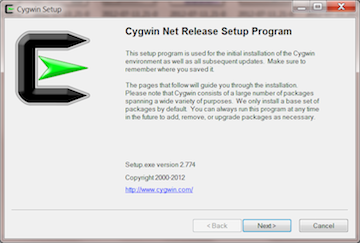
Cygwin may be a program that may transfer and install different programs from the web for you. You'll have to relinquish it some info regarding your web association, though most of the time you'll simply settle for the defaults and keep going.
Next, Cygwin can show you a protracted list of transfer sites. Each is precisely the same: you'll decide one randomly. You are doing not ought to decide an equivalent transfer web site shown within the screenshot. Once you have picked one and clicked the "next" button, you will see Associate in Nursing upgrade warning, however that solely applies to folks upgrading from Associate in Nursing older version of Cygwin. Since this is often a replacement installation, we will ignore it.
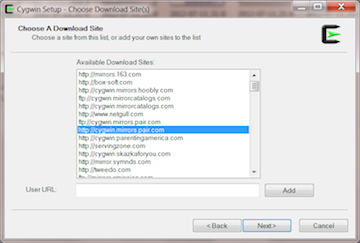
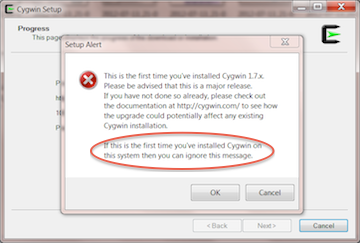
Now, we have a tendency to get to pick out what code we would like to put in from the transfer web site. We would like to put in 3 code packages: openssh, git, and curl. For every one, use the search box to search out the package, so click on the word "Skip" in order that it changes to a version range. Install the newest on the market version for every of those packages.
Next, Cygwin can tell you that you simply ought to install sure different packages as dependencies. Basically, this suggests that if you would like to use an explicit package named A, and A depends on another package named B so as to run properly, then Cygwin can discover this and raise to put in package B in addition. You'll simply hit "Next". At that time, Cygwin can begin downloading and putting in all the packages that you've got requested, in addition as all their dependencies. Counting on the speed of your web association, this might take a bit whereas. Once the center of the 3 progress bars (the one marked "Total") is full, Cygwin is finished.
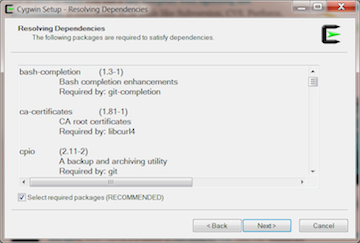
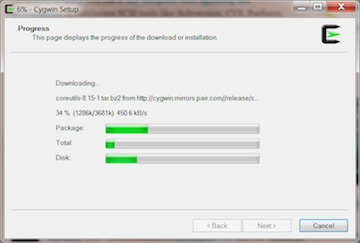
If you raise Cygwin to put in Associate in Nursing icon on your desktop, it'll do thus, making a road named "Cygwin Terminal". You will use this icon to run your Python code, in addition on access the openssh, git, and curl packages that you simply put in. Double-click the icon to run Cygwin Terminal, and you will see a window like this pop up:
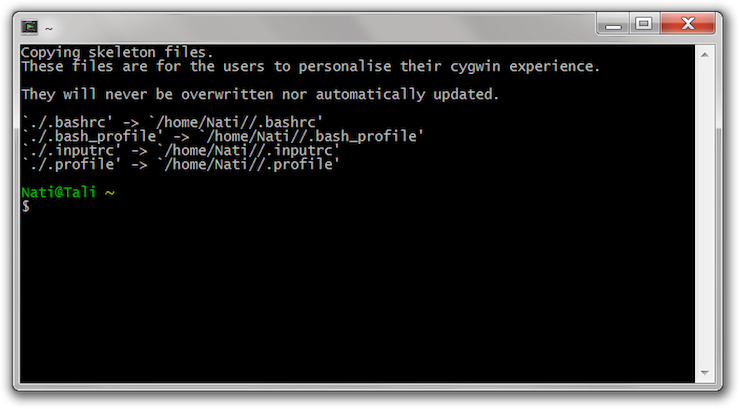
The exact text in your terminal window are a bit completely different, since it depends on the name of your pc, and also the name of your user account. Currently we will begin victimisation the terminal: if you do not knowledge. First, let's use the that command to search out out wherever those packages area unit that we have a tendency to installed:
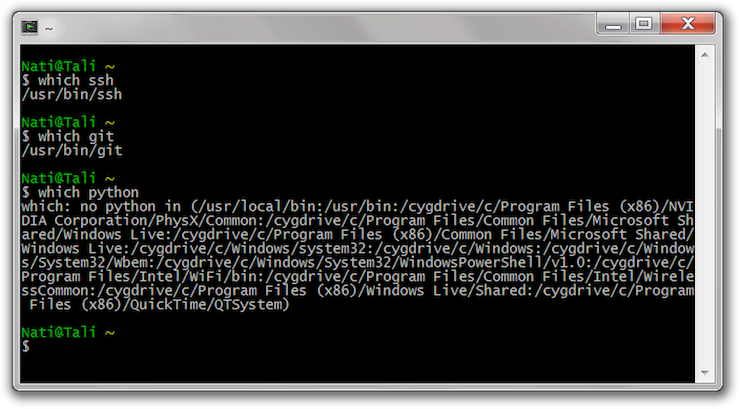
So it's just like the terminal will realize ssh and so-and-so, however not python. That is apprehensible, since we have a tendency to did not use Cygwin to put in Python. To inform Cygwin the way to realize Python, run the subsequent command:
Echo "PATH=$PATH:/cygdrive/c/Python32" >> .bash_profile
Note that you simply're victimisation an equivalent directory that you put in Python into: if you are putting in a unique version of Python, then replace Python32 with the version of Python you have got put in. You ought to solely ought to do that once: once you have run that command, the terminal can invariably be able to realize Python once more.
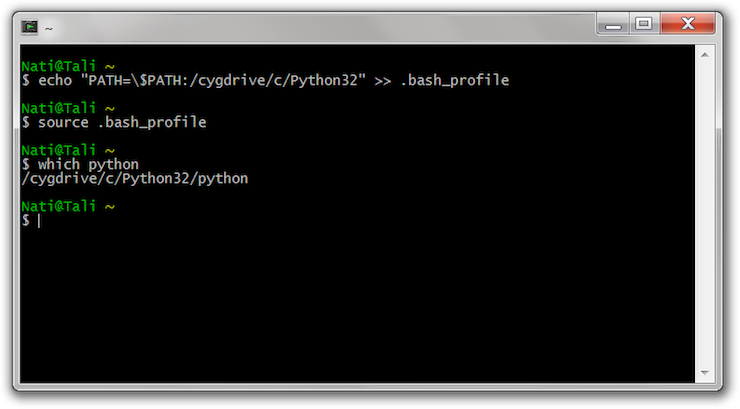
You're finally done installing and setting up Cygwin!
Installing Sublime Text
Lastly, you'll have a text editor. There area unit several smart choices, however I counsel chic Text it's totally powerful and helpful, and it's an infinite free trial. Please note that Microsoft Word may be a application, not a text editor: you can not use Microsoft Word for writing pc programs.
Installing chic Text is easy: simply visit grandeur Text homepage, transfer the installer, and install it. Chic Text isn't free code, however you'll strive it for as long as you wish before you get it. If you finish up writing several programs victimisation chic Text, I encourage you to shop for a license.
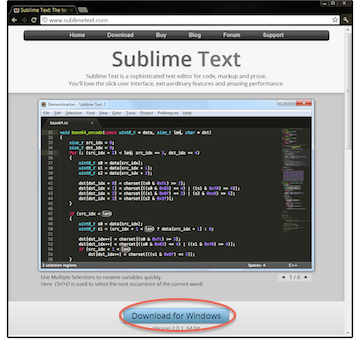
Verify that it all works
Now that you are equipped up, let's verify that everything is functioning properly! the primary program that any coder writes is termed "Hello, World!", and every one it will is write that text bent the terminal. So, let's write "Hello, World!" in Python! If your pc is ready up properly, this could work properly:
$ python -c 'print("Hello, World!")'
Hello, World!
If that works, lets advance to writing a program into a file. Open up chic Text, and sort within the following:
#!/usr/bin/env python
Print("Hello, World!")
Save the move into your home directory (inside of C:cygwinhome) as hullo.py. Then open up the Terminal, cd to it directory, and run:
$ chmoda+x hullo.py
$ ./hello.py
Hello, World!
And you ought to see an equivalent hullo, World! output that you simply saw before.
The Python language has several similarities to Perl, C, and Java. However, there square measure some definite variations between the languages.
First Python Program
Let us execute programs in several modes of programming.
Interactive Mode Programming
Invoking the interpreter while not passing a script file as a parameter brings up the subsequent the subsequent
$ python
Python 2.4.3 (#1, Nov eleven 2010, 13:34:43)
[GCC 4.1.2 a pair of0080704 (Red Hat four.1.2-48)] on linux2
Type "help", "copyright", "credits" or "license" for a lot of info.
>>>
Type the subsequent text at the Python prompt and press the Enter −
>>> print "Hello, Python!"
If you're running new edition of Python, then you'd have to be compelled to use print statement with parenthesis as in print ("Hello, Python!");.but in Python version a pair of.4.3, this produces the subsequent the subsequent
Hello, Python!
Script Mode Programming
Invoking the interpreter with a script parameter begins execution of the script and continues till the script is finished. Once the script is finished, the interpreter isn't any longer active.
Let us write a straightforward Python program during a script. Python files have extension .py. Kind the subsequent ASCII text file during a take a look at.py file −
Print "Hello, Python!"
We assume that you just have Python interpreter set in PATH variable. Now, attempt to run this program as follows −
$ python take a look at.py
This produces the subsequent the subsequent
Hello, Python!
Let us attempt otherwise to execute a Python script. Here is that the changed take a look at.py file −
#!/usr/bin/python
Print "Hello, Python!"
We assume that you just have Python interpreter obtainable in /usr/bin directory. Now, attempt to run this program as follows −
$ chmod +x take a look at.py # this is often to create file practicable
$./test.py
This produces the subsequent the subsequent
Hello, Python!
Python Identifiers
A Python symbol may be a name wont to determine a variable, function, class, module or different object. Associate degree symbol starts with a letter A to Z or a to z or associate degree underscore (_) followed by zero or a lot of letters, underscores and digits (0 to 9).
Python doesn't permit punctuation characters like @, $, and a pair of among identifiers. Python may be a case sensitive artificial language. Thus, force and force square measure 2 completely different identifiers in Python.
Here square measure naming conventions for Python identifiers −
• Class names begin with associate degree capital letter. All different identifiers begin with a lowercase letter.
• Starting associate degree symbol with one leading underscore indicates that the symbol is non-public.
• Starting associate degree symbol with 2 leading underscores indicates a powerfully non-public symbol.
• If the symbol additionally ends with 2 trailing underscores, the symbol may be a language-defined special name.
Reserved Words
The following list shows the Python keywords. These are reserved words and you cannot use them as constant or variable or any other identifier names. All the Python keywords contain lowercase letters only.
And | Exec | Not |
Assert | Finally | Or |
Break | For | Pass |
Class | From | |
Continue | Global | Raise |
Def | If | Return |
Del | Import | Try |
Elif | In | While |
Else | Is | With |
Except | Lambda | Yield |
Lines and Indentation
Python provides no braces to indicate blocks of code for class and function definitions or flow control. Blocks of code are denoted by line indentation, which is rigidly enforced.
The number of spaces in the indentation is variable, but all statements within the block must be indented the same amount. For example −
If True:
Print "True"
Else:
Print "False"
However, the following block generates an error −
If True:
Print "Answer"
Print "True"
Else:
Print "Answer"
Print "False"
Thus, in Python all the continuous lines indented with same number of spaces would form a block. The following example has various statement blocks −
Note− Do not try to understand the logic at this point of time. Just make sure you understood various blocks even if they are without braces.
#!/usr/bin/python
Import sys
Try:
# open file stream
File = open(file_name, "w")
ExceptIOError:
Print "There was an error writing to", file_name
Sys.exit()
Print "Enter '", file_finish,
Print "' When finished"
Whilefile_text != file_finish:
File_text = raw_input("Enter text: ")
Iffile_text == file_finish:
# close the file
File.close
Break
File.write(file_text)
File.write("\n")
File.close()
File_name = raw_input("Enter filename: ")
Iflen(file_name) == 0:
Print "Next time please enter something"
Sys.exit()
Try:
File = open(file_name, "r")
ExceptIOError:
Print "There was an error reading file"
Sys.exit()
File_text = file.read()
File.close()
Printfile_text
Multi-Line Statements
Statements in Python typically end with a new line. Python does, however, allow the use of the line continuation character (\) to denote that the line should continue. For example −
Total = item_one + \
Item_two + \
Item_three
Statements contained within the [], {}, or () brackets do not need to use the line continuation character. For example −
Days = ['Monday', 'Tuesday', 'Wednesday',
'Thursday', 'Friday']
Quotation in Python
Python accepts single ('), double (") and triple (''' or """) quotes to denote string literals, as long as the same type of quote starts and ends the string.
The triple quotes are used to span the string across multiple lines. For example, all the following are legal −
Word = 'word'
Sentence = "This is a sentence."
Paragraph = """This is a paragraph. It is
Made up of multiple lines and sentences."""
Comments in Python
A hash sign (#) that is not inside a string literal begins a comment. All characters after the # and up to the end of the physical line are part of the comment and the Python interpreter ignores them.
#!/usr/bin/python
# First comment
Print "Hello, Python!" # second comment
This produces the following result −
Hello, Python!
You can type a comment on the same line after a statement or expression −
Name = "Madisetti" # This is again comment
You can comment multiple lines as follows −
# This is a comment.
# This is a comment, too.
# This is a comment, too.
# I said that already.
Following triple-quoted string is also ignored by Python interpreter and can be used as a multiline comments:
'''
This is a multiline
Comment.
'''
Using Blank Lines
A line containing only whitespace, possibly with a comment, is known as a blank line and Python totally ignores it.
In an interactive interpreter session, you must enter an empty physical line to terminate a multiline statement.
Waiting for the User
The following line of the program displays the prompt, the statement saying “Press the enter key to exit”, and waits for the user to take action −
#!/usr/bin/python
Raw_input("\n\nPress the enter key to exit.")
Here, "\n\n" is used to create two new lines before displaying the actual line. Once the user presses the key, the program ends. This is a nice trick to keep a console window open until the user is done with an application.
Multiple Statements on a Single Line
The semicolon ( ; ) allows multiple statements on the single line given that neither statement starts a new code block. Here is a sample snip using the semicolon −
Import sys; x = 'foo'; sys.stdout.write(x + '\n')
Multiple Statement Groups as Suites
A group of individual statements, which make a single code block are called suites in Python. Compound or complex statements, such as if, while, def, and class require a header line and a suite.
Header lines begin the statement (with the keyword) and terminate with a colon ( : ) and are followed by one or more lines which make up the suite. For example −
If expression :
Suite
Elif expression :
Suite
Else :
Suite
Command Line Arguments
Many programs can be run to provide you with some basic information about how they should be run. Python enables you to do this with -h −
$ python -h
Usage: python [option] ... [-c cmd | -m mod | file | -] [arg] ...
Options and arguments (and corresponding environment variables):
-c cmd : program passed in as string (terminates option list)
-d : debug output from parser (also PYTHONDEBUG=x)
-E : ignore environment variables (such as PYTHONPATH)
-h : print this help message and exit
[ etc. ]
You can also program your script in such a way that it should accept various options. Command Line Arguments is an advanced topic and should be studied a bit later once you have gone through rest of the Python concepts.
Run Command
You can use run command in the input prompt to run a Python script. The run command is actually line magic command and should actually be written as %run. However, the %automagic mode is always on by default, so you can omit this.
In [1]: run hello.py
Hello IPython
Edit Command
IPython also provides edit magic command. It invokes default editor of the operating system. You can open it through Windows Notepad editor and the script can be edited. Once you close it after saving its input, the output of modified script will be displayed.
In [2]: edit hello.py
Editing... Done. Executing edited code...
Hello IPython
Welcome to interactive computing
Note that hello.py initially contained only one statement and after editing one more statement was added. If no file name is given to edit command, a temporary file is created. Observe the following code that shows the same.
In [7]: edit
IPython will make a temporary file named:
C:\Users\acer\AppData\Local\Temp\ipython_edit_4aa4vx8f\ipython_edit_t7i6s_er.py
Editing... Done. Executing edited code...
Magic of IPython
Out[7]: 'print ("magic of IPython")'
The Terms 'Interactive' and 'Shell'
The term "interactive" traces back to the Latin expression "inter agere". The verb "agere" means that amongst different things "to do something" and "to act", whereas "inter" denotes the abstraction and temporal position to things and events, i.e. "between" or "among" objects, persons, and events. Thus "inter agere" means that "to act between" or "to act among" these.
With this in mind, we will say that the interactive shell is between the user and also the software system (e.g. Linux, Unix, Windows or others). Rather than associate degree software system associate degree interpreter are often used for a artificial language like Python still. The Python interpreter are often used from associate degree interactive shell.
The interactive shell is additionally interactive within the means that it stands between the commands or actions and their execution. In different words, the shell waits for commands from the user, that it executes and returns the results of the execution. Afterwards, the shell waits for consequent input.
A shell in biology may be a carbonate "wall" that protects snails or mussels from its setting or its enemies. Similarly, a shell in software systems lies between the kernel of the operative system and also the user. It is a "protection" in each directions. The user does not ought to use the sophisticated basic functions of the OS however is capable of mistreatment the interactive shell that is comparatively straightforward and simple to grasp. The kernel is protected against unintentional and incorrect usages of system functions.
Python offers a snug instruction interface with the Python Shell, that is additionally referred to as the "Python Interactive Shell". It's just like the term "Interactive Shell" may be a tautology, as a result of "shell" is interactive on its own, a minimum of the sort of shells we've represented within the previous paragraphs.
Using the Python Interactive Shell
With the Python interactive interpreter it is easy to check Python commands. The Python interpreter can be invoked by typing the command "python" without any parameter followed by the "return" key at the shell prompt:
Python
Python comes back with the following information:
$ python
Python 2.7.11 (default, Mar 27 2019, 22:11:17)
[GCC 7.3.0] :: Anaconda, Inc. On linux
Type "help", "copyright", "credits" or "license" for more information.
A closer look at the output above reveals that we have the wrong Python version. We wanted to use Python 3.x, but what we got is the installed standard of the operating system, i.e. version 2.7.11+.
The easiest way to check if a Python 3.x version is installed: Open a Terminal. Type in python but no return. Instead, type the "Tab" key. You will see possible extensions and other installed versions, if there are some:
Bernd@venus:~$ $ python
Python python3 python3.7-config python-config
Python2 python3.6 python3.7m pythontex
Python2.7 python3.6-config python3.7m-config pythontex3
Python2.7-config python3.6m python3-config python-whiteboard
Python2-config python3.6m-config python3m
Python2-pbr python3.7 python3m-config
Bernd@venus:~$ python
If no other Python version shows up, python3.x has to be installed. Afterwards, we can start the newly installed version by typing python3:
$ python3
Python 3.6.7 (default, Oct 22 2018, 11:32:17)
[GCC 8.2.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
Once the Python interpreter is started, you can issue any command at the command prompt ">>>". Let's see, what happens, if we type in the word "hello":
Hello
---------------------------------------------------------------------------
NameErrorTraceback (most recent call last)
<ipython-input-1-f572d396fae9> in <module>
----> 1 hello
NameError: name 'hello' is not defined
Of course, "hello" is not a proper Python command, so the interactive shell returns ("raises") an error.
The first real command we will use is the print command. We will create the mandatory "Hello World" statement:
Print("Hello World")
Hello World
It couldn't have been easier, could it? Oh yes, it can be written in a even simpler way. In the Interactive Python Interpreter - but not in a program - the print is not necessary. We can just type in a string or a number and it will be "echoed"
"Hello World"
Output::
'Hello World'
3
Output::
3
How to Quit the Python Shell
So, we have just started, and we are already talking about quitting the shell. We do this, because we know, how annoying it can be, if you don't know how to properly quit a program.
It's easy to end the interactive session: You can either use exit() or Ctrl-D (i.e. EOF) to exit. The brackets behind the exit function are crucial. (Warning: Exit without brackets works in Python2.x but doesn't work anymore in Python3.x)
The Shell as a Simple Calculator
In the following example we use the interpreter as a simple calculator by typing an arithmetic expression:
4.567 * 8.323 * 17
Output::
646.189397
Python follows the usual order of operations in expressions. The standard order of operations is expressed in the following enumeration:
- Exponents and roots
- Multiplication and division
- Addition and subtraction
In other words, we don't need parentheses in the expression "3 + (2 * 4)":
3 + 2 * 4
Output::
11
The most recent output value is automatically stored by the interpreter in a special variable with the name "_". So we can print the output from the recent example again by typing an underscore after the prompt:
_
Output::
11
The underscore can be used in other expressions like any other variable:
_ * 3
Output::
33
The underscore variable is only available in the Python shell. It's NOT available in Python scripts or programs.
Using Variables
It's simple to use variables in the Python shell. If you are an absolute beginner and if you don't know anything about variables. Values can be saved in variables. Variable names don't require any special tagging, like they do in Perl, where you have to use dollar signs, percentage signs and at signs to tag variables:
Maximal = 124
Width = 94
Print(maximal - width)
30
Multiline Statements
We haven't introduced multiline statements so far. So beginners can skip the rest of this chapter and can continue with the following chapters.
We will show how the interactive prompt deals with multiline statements like for loops.
l = ["A", 42, 78, "Just a String"]
For character in l:
Print(character)
A
42
78
Just a String
After having input "for character in l: " the interpretor expects the input of the next line to be indented. In other words: the interpretor expects an indented block, which is the body of the loop. This indented block will be iterated. The interpretor shows this "expectation" by displaying three dots "..." instead of the standard Python interactive prompt ">>>". Another special feature of the interactive shell: when we are done with the indented lines, i.e. the block, we have to enter an empty line to indicate that the block is finished. Attention: the additional empty line is only necessary in the interactive shell! In a Python program, it is enough to return to the indentation level of the "for" line, the one with the colon ":" at the end.
Strings
Strings are created by putting a sequence of characters in quotes. Strings can be surrounded by single quotes, double quotes or triple quotes, which are made up of three single or three double quotes. Strings are immutable. In other words, once defined, they cannot be changed. We will cover this topic in detail in another chapter.
"Hello" + " " + "World"
Output::
'Hello World'
A string in triple quotes can span several lines without using the escape character:
City = """
... Toronto is the largest city in Canada
... And the provincial capital of Ontario.
... It is located in Southern Ontario on the
... Northwestern shore of Lake Ontario.
... """
Print(city)
Toronto is the largest city in Canada
And the provincial capital of Ontario.
It is located in Southern Ontario on the
Northwestern shore of Lake Ontario.
Multiplication on strings is defined, which is essentially a multiple concatenation:
".-." * 4
Output::
'.-..-..-..-.'
Variables can hold values, and every value has a data-type. Python is a dynamically typed language; hence we do not need to define the type of the variable while declaring it. The interpreter implicitly binds the value with its type.
- a = 5
The variable a holds integer value five and we did not define its type. Python interpreter will automatically interpret variables a as an integer type.
Python enables us to check the type of the variable used in the program. Python provides us the type() function, which returns the type of the variable passed.
Consider the following example to define the values of different data types and checking its type.
- a=10
- b="Hi Python"
- c = 10.5
- Print(type(a))
- Print(type(b))
- Print(type(c))
Output:
<type 'int'>
<type 'str'>
<type 'float'>
Standard data types
A variable can hold different types of values. For example, a person's name must be stored as a string whereas its id must be stored as an integer.
Python provides various standard data types that define the storage method on each of them. The data types defined in Python are given below.
- Numbers
- Sequence Type
- Boolean
- Set
- Dictionary
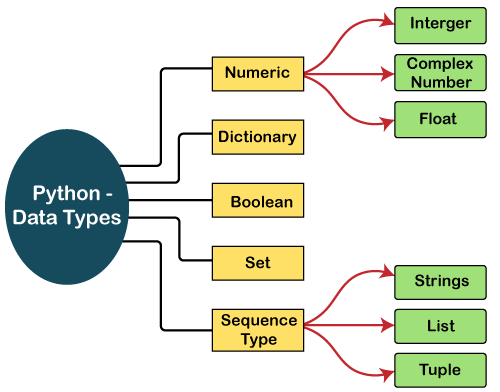
In this section of the tutorial, we will give a brief introduction of the above data-types. We will discuss each one of them in detail later in this tutorial.
Numbers
Number stores numeric values. The integer, float, and complex values belong to a Python Numbers data-type. Python provides the type() function to know the data-type of the variable. Similarly, the isinstance() function is used to check an object belongs to a particular class.
Python creates Number objects when a number is assigned to a variable. For example;
- a = 5
- Print("The type of a", type(a))
- b = 40.5
- Print("The type of b", type(b))
- c = 1+3j
- Print("The type of c", type(c))
- Print(" c is a complex number", isinstance(1+3j,complex))
Output:
The type of a <class 'int'>
The type of b <class 'float'>
The type of c <class 'complex'>
c is complex number: True
Python supports three types of numeric data.
- Int - Integer value can be any length such as integers 10, 2, 29, -20, -150 etc. Python has no restriction on the length of an integer. Its value belongs to int
- Float - Float is used to store floating-point numbers like 1.9, 9.902, 15.2, etc. It is accurate upto 15 decimal points.
- Complex - A complex number contains an ordered pair, i.e., x + iy where x and y denote the real and imaginary parts, respectively. The complex numbers like 2.14j, 2.0 + 2.3j, etc.
Sequence Type
String
The string can be defined as the sequence of characters represented in the quotation marks. In Python, we can use single, double, or triple quotes to define a string.
String handling in Python is a straightforward task since Python provides built-in functions and operators to perform operations in the string.
In the case of string handling, the operator + is used to concatenate two strings as the operation "hello"+" python" returns "hello python".
The operator * is known as a repetition operator as the operation "Python" *2 returns 'Python Python'.
The following example illustrates the string in Python.
Example - 1
- Str = "string using double quotes"
- Print(str)
- s = '''''A multiline
- String'''
- Print(s)
Output:
String using double quotes
A multiline
String
Consider the following example of string handling.
Example - 2
- Str1 = 'hello javatpoint' #string str1
- Str2 = ' how are you' #string str2
- Print (str1[0:2]) #printing first two character using slice operator
- Print (str1[4]) #printing 4th character of the string
- Print (str1*2) #printing the string twice
- Print (str1 + str2) #printing the concatenation of str1 and str2
Output:
He
o
Hellojavatpointhellojavatpoint
Hellojavatpoint how are you
List
Python Lists are similar to arrays in C. However, the list can contain data of different types. The items stored in the list are separated with a comma (,) and enclosed within square brackets [].
We can use slice [:] operators to access the data of the list. The concatenation operator (+) and repetition operator (*) works with the list in the same way as they were working with the strings.
Consider the following example.
- List1 = [1, "hi", "Python", 2]
- #Checking type of given list
- Print(type(list1))
- #Printing the list1
- Print (list1)
- # List slicing
- Print (list1[3:])
- # List slicing
- Print (list1[0:2])
- # List Concatenation using + operator
- Print (list1 + list1)
- # List repetation using * operator
- Print (list1 * 3)
Output:
[1, 'hi', 'Python', 2]
[2]
[1, 'hi']
[1, 'hi', 'Python', 2, 1, 'hi', 'Python', 2]
[1, 'hi', 'Python', 2, 1, 'hi', 'Python', 2, 1, 'hi', 'Python', 2]
Tuple
A tuple is similar to the list in many ways. Like lists, tuples also contain the collection of the items of different data types. The items of the tuple are separated with a comma (,) and enclosed in parentheses ().
A tuple is a read-only data structure as we can't modify the size and value of the items of a tuple.
Let's see a simple example of the tuple.
- Tup = ("hi", "Python", 2)
- # Checking type of tup
- Print (type(tup))
- #Printing the tuple
- Print (tup)
- # Tuple slicing
- Print (tup[1:])
- Print (tup[0:1])
- # Tuple concatenation using + operator
- Print (tup + tup)
- # Tuple repatation using * operator
- Print (tup * 3)
- # Adding value to tup. It will throw an error.
- t[2] = "hi"
Output:
<class 'tuple'>
('hi', 'Python', 2)
('Python', 2)
('hi',)
('hi', 'Python', 2, 'hi', 'Python', 2)
('hi', 'Python', 2, 'hi', 'Python', 2, 'hi', 'Python', 2)
Traceback (most recent call last):
File "main.py", line 14, in <module>
t[2] = "hi";
TypeError: 'tuple' object does not support item assignment
Dictionary
Dictionary is an unordered set of a key-value pair of items. It is like an associative array or a hash table where each key stores a specific value. Key can hold any primitive data type, whereas value is an arbitrary Python object.
The items in the dictionary are separated with the comma (,) and enclosed in the curly braces {}.
Consider the following example.
- d = {1:'Jimmy', 2:'Alex', 3:'john', 4:'mike'}
- # Printing dictionary
- Print (d)
- # Accesing value using keys
- Print("1st name is "+d[1])
- Print("2nd name is "+ d[4])
- Print (d.keys())
- Print (d.values())
Output:
1st name is Jimmy
2nd name is mike
{1: 'Jimmy', 2: 'Alex', 3: 'john', 4: 'mike'}
Dict_keys([1, 2, 3, 4])
Dict_values(['Jimmy', 'Alex', 'john', 'mike'])
Boolean
Boolean type provides two built-in values, True and False. These values are used to determine the given statement true or false. It denotes by the class bool. True can be represented by any non-zero value or 'T' whereas false can be represented by the 0 or 'F'. Consider the following example.
- # Python program to check the boolean type
- Print(type(True))
- Print(type(False))
- Print(false)
Output:
<class 'bool'>
<class 'bool'>
NameError: name 'false' is not defined
Set
Python Set is the unordered collection of the data type. It is iterable, mutable(can modify after creation), and has unique elements. In set, the order of the elements is undefined; it may return the changed sequence of the element. The set is created by using a built-in function set(), or a sequence of elements is passed in the curly braces and separated by the comma. It can contain various types of values. Consider the following example.
- # Creating Empty set
- Set1 = set()
- Set2 = {'James', 2, 3,'Python'}
- #Printing Set value
- Print(set2)
- # Adding element to the set
- Set2.add(10)
- Print(set2)
- #Removing element from the set
- Set2.remove(2)
- Print(set2)
Output:
{3, 'Python', 'James', 2}
{'Python', 'James', 3, 2, 10}
{'Python', 'James', 3, 10}
There is a need to generate random numbers when studying a model or behavior of a program for different range of values. Python can generate such random numbers by using the random module. In the below examples we will first see how to generate a single random number and then extend it to generate a list of random numbers.
Generating a Single Random Number
The random() method in random module generates a float number between 0 and 1.
Example
Import random
n =random.random()
Print(n)
Output
Running the above code gives us the following result −
0.2112200
Generating Number in a Range
The randint() method generates a integer between a given range of numbers.
Example
Import random
n =random.randint(0,22)
Print(n)
Output
Running the above code gives us the following result −
2
Generating a List of numbers Using For Loop
We can use the above randint() method along with a for loop to generate a list of numbers. We first create an empty list and then append the random numbers generated to the empty list one by one.
Example
Import random
Randomlist=[]
Foriin range(0,5):
n =random.randint(1,30)
Randomlist.append(n)
Print(randomlist)
Output
Running the above code gives us the following result −
[10, 5, 21, 1, 17]
Using random.sample()
We can also use the sample() method available in random module to directly generate a list of random numbers.Here we specify a range and give how many random numbers we need to generate.
Example
Import random
#Generate 5 random numbers between 10 and 30
Randomlist=random.sample(range(10,30),5)
Print(randomlist)
Output
Running the above code gives us the following result −
[16, 19, 13, 18, 15]
Python – Numbers
Number data types store numeric values. They are immutable data types, means that changing the value of a number data type results in a newly allocated object.
Number objects are created when you assign a value to them. For example −
Var1 = 1
Var2 = 10
You can also delete the reference to a number object by using the del statement. The syntax of the del statement is −
Del var1[,var2[,var3[....,varN]]]]
You can delete a single object or multiple objects by using the del statement. For example −
Delvar
Delvar_a, var_b
Python supports four different numerical types −
- Int (signed integers)− They are often called just integers or ints, are positive or negative whole numbers with no decimal point.
- Long (long integers )− Also called longs, they are integers of unlimited size, written like integers and followed by an uppercase or lowercase L.
- Float (floating point real values)− Also called floats, they represent real numbers and are written with a decimal point dividing the integer and fractional parts. Floats may also be in scientific notation, with E or e indicating the power of 10 (2.5e2 = 2.5 x 102 = 250).
- Complex (complex numbers)− are of the form a + bJ, where a and b are floats and J (or j) represents the square root of -1 (which is an imaginary number). The real part of the number is a, and the imaginary part is b. Complex numbers are not used much in Python programming.
Examples
Here are some examples of numbers
Int | Long | Float | Complex |
10 | 51924361L | 0.0 | 3.14j |
100 | -0x19323L | 15.20 | 45.j |
-786 | 0122L | -21.9 | 9.322e-36j |
080 | 0xDEFABCECBDAECBFBAEL | 32.3+e18 | .876j |
-0490 | 535633629843L | -90. | -.6545+0J |
-0x260 | -052318172735L | -32.54e100 | 3e+26J |
0x69 | -4721885298529L | 70.2-E12 | 4.53e-7j |
- Python allows you to use a lowercase L with long, but it is recommended that you use only an uppercase L to avoid confusion with the number 1. Python displays long integers with an uppercase L.
- A complex number consists of an ordered pair of real floating point numbers denoted by a + bj, where a is the real part and b is the imaginary part of the complex number.
Number Type Conversion
Python converts numbers internally in an expression containing mixed types to a common type for evaluation. But sometimes, you need to coerce a number explicitly from one type to another to satisfy the requirements of an operator or function parameter.
- Type int(x) to convert x to a plain integer.
- Type long(x) to convert x to a long integer.
- Type float(x) to convert x to a floating-point number.
- Type complex(x) to convert x to a complex number with real part x and imaginary part zero.
- Type complex(x, y) to convert x and y to a complex number with real part x and imaginary part y. x and y are numeric expressions
Mathematical Functions
Python includes following functions that perform mathematical calculations.
Sr.No. | Function & Returns ( description ) |
1 | Abs(x) The absolute value of x: the (positive) distance between x and zero. |
2 | Ceil(x) The ceiling of x: the smallest integer not less than x |
3 | Cmp(x,y) -1 if x < y, 0 if x == y, or 1 if x > y |
4 | Exp(x) The exponential of x: ex |
5 | Fabs(x) The absolute value of x. |
6 | Floor(x) The floor of x: the largest integer not greater than x |
7 | Log(x) The natural logarithm of x, for x> 0 |
8 | Log10(x) The base-10 logarithm of x for x> 0. |
9 | Max(x1, x2,...) The largest of its arguments: the value closest to positive infinity |
10 | Min(x1, x2,...) The smallest of its arguments: the value closest to negative infinity |
11 | Modf(x) The fractional and integer parts of x in a two-item tuple. Both parts have the same sign as x. The integer part is returned as a float. |
12 | Pow(x,y) The value of x**y. |
13 | Round(x [,n]) x rounded to n digits from the decimal point. Python rounds away from zero as a tie-breaker: round(0.5) is 1.0 and round(-0.5) is -1.0. |
14 | Sqrt(x) The square root of x for x > 0 |
Random Number Functions
Random numbers are used for games, simulations, testing, security, and privacy applications. Python includes following functions that are commonly used.
Sr.No. | Function & Description |
1 | Choice(seq) A random item from a list, tuple, or string. |
2 | Randrange ([start,] stop [,step]) A randomly selected element from range(start, stop, step) |
3 | Random() A random float r, such that 0 is less than or equal to r and r is less than 1 |
4 | Seed([x]) Sets the integer starting value used in generating random numbers. Call this function before calling any other random module function. Returns None. |
5 | Shuffle(1st) Randomizes the items of a list in place. Returns None. |
6 | Uniform(x,y) A random float r, such that x is less than or equal to r and r is less than y |
Trigonometric Functions
Python includes following functions that perform trigonometric calculations.
Sr.No. | Function & Description |
1 | Acos(x) Return the arc cosine of x, in radians. |
2 | Asin(x) Return the arc sine of x, in radians. |
3 | Atan(x) Return the arc tangent of x, in radians. |
4 | Atan2(y,x) Return atan(y / x), in radians. |
5 | Cos(x) Return the cosine of x radians. |
6 | Hypot(X,Y) Return the Euclidean norm, sqrt(x*x + y*y). |
7 | Sin(X) Return the sine of x radians. |
8 | Tan(x) Return the tangent of x radians. |
9 | Degrees(X) Converts angle x from radians to degrees. |
10 | Radians(X) Converts angle x from degrees to radians. |
Mathematical Constants
The module also defines two mathematical constants −
Sr.No. | Constants & Description |
1 | Pi The mathematical constant pi. |
2 | E The mathematical constant e. |
Every variable in python holds an instance of an object. There are two types of objects in python i.e. Mutable and Immutable objects. Whenever an object is instantiated, it is assigned a unique object id. The type of the object is defined at the runtime and it can’t be changed afterwards. However, it’s state can be changed if it is a mutable object.
To summarise the difference, mutable objects can change their state or contents and immutable objects can’t change their state or content.
- Immutable Objects : These are of in-built types like int, float, bool, string, unicode, tuple. In simple words, an immutable object can’t be changed after it is created.
# Python code to test that # tuples are immutable
Tuple1 =(0, 1, 2, 3) Tuple1[0] =4 Print(tuple1) |
Error :
Traceback (most recent call last):
File "e0eaddff843a8695575daec34506f126.py", line 3, in
Tuple1[0]=4
TypeError: 'tuple' object does not support item assignment
# Python code to test that # strings are immutable
Message ="Welcome to GeeksforGeeks" Message[0] ='p' Print(message) |
Error :
Traceback (most recent call last):
File "/home/ff856d3c5411909530c4d328eeca165b.py", line 3, in
Message[0] = 'p'
TypeError: 'str' object does not support item assignment
- Mutable Objects:These are of type list,dict,set. Custom classes are generally mutable.
# Python code to test that # lists are mutable Color =["red", "blue", "green"] Print(color)
Color[0] ="pink" Color[-1] ="orange" Print(color) |
Output
['red', 'blue', 'green']
['pink', 'blue', 'orange']
Conclusion
- Mutable and immutable objects are handled differently in python. Immutable objects are quicker to access and are expensive to change because it involves the creation of a copy.
Whereas mutable objects are easy to change. - Use of mutable objects is recommended when there is a need to change the size or content of the object.
- Exception : However, there is an exception in immutability as well. We know that tuple in python is immutable. But the tuple consists of a sequence of names with unchangeable bindings to objects.
Consider a tuple
Tup = ([3, 4, 5], 'myname')
The tuple consists of a string and a list. Strings are immutable so we can’t change its value. But the contents of the list can change. The tuple itself isn’t mutable but contain items that are mutable.
Till now, we were taking the input from the console and writing it back to the console to interact with the user.
Sometimes, it is not enough to only display the data on the console. The data to be displayed may be very large, and only a limited amount of data can be displayed on the console since the memory is volatile, it is impossible to recover the programmatically generated data again and again.
The file handling plays an important role when the data needs to be stored permanently into the file. A file is a named location on disk to store related information. We can access the stored information (non-volatile) after the program termination.
The file-handling implementation is slightly lengthy or complicated in the other programming language, but it is easier and shorter in Python.
In Python, files are treated in two modes as text or binary. The file may be in the text or binary format, and each line of a file is ended with the special character.
Hence, a file operation can be done in the following order.
- Open a file
- Read or write - Performing operation
- Close the file
Opening a file
Python provides an open() function that accepts two arguments, file name and access mode in which the file is accessed. The function returns a file object which can be used to perform various operations like reading, writing, etc.
Syntax:
- File object = open(<file-name>, <access-mode>, <buffering>)
The files can be accessed using various modes like read, write, or append. The following are the details about the access mode to open a file.
SN | Access mode | Description |
1 | r | It opens the file to read-only mode. The file pointer exists at the beginning. The file is by default open in this mode if no access mode is passed. |
2 | Rb | It opens the file to read-only in binary format. The file pointer exists at the beginning of the file. |
3 | r+ | It opens the file to read and write both. The file pointer exists at the beginning of the file. |
4 | Rb+ | It opens the file to read and write both in binary format. The file pointer exists at the beginning of the file. |
5 | w | It opens the file to write only. It overwrites the file if previously exists or creates a new one if no file exists with the same name. The file pointer exists at the beginning of the file. |
6 | Wb | It opens the file to write only in binary format. It overwrites the file if it exists previously or creates a new one if no file exists. The file pointer exists at the beginning of the file. |
7 | w+ | It opens the file to write and read both. It is different from r+ in the sense that it overwrites the previous file if one exists whereas r+ doesn't overwrite the previously written file. It creates a new file if no file exists. The file pointer exists at the beginning of the file. |
8 | Wb+ | It opens the file to write and read both in binary format. The file pointer exists at the beginning of the file. |
9 | a | It opens the file in the append mode. The file pointer exists at the end of the previously written file if exists any. It creates a new file if no file exists with the same name. |
10 | Ab | It opens the file in the append mode in binary format. The pointer exists at the end of the previously written file. It creates a new file in binary format if no file exists with the same name. |
11 | a+ | It opens a file to append and read both. The file pointer remains at the end of the file if a file exists. It creates a new file if no file exists with the same name. |
12 | Ab+ | It opens a file to append and read both in binary format. The file pointer remains at the end of the file. |
Let's look at the simple example to open a file named "file.txt" (stored in the same directory) in read mode and printing its content on the console.
Example
- #opens the file file.txt in read mode
- Fileptr = open("file.txt","r")
- If fileptr:
- Print("file is opened successfully")
Output:
<class '_io.TextIOWrapper'>
File is opened successfully
In the above code, we have passed filename as a first argument and opened file in read mode as we mentioned r as the second argument. The fileptr holds the file object and if the file is opened successfully, it will execute the print statement
The close() method
Once all the operations are done on the file, we must close it through our Python script using the close() method. Any unwritten information gets destroyed once the close() method is called on a file object.
We can perform any operation on the file externally using the file system which is the currently opened in Python; hence it is good practice to close the file once all the operations are done.
The syntax to use the close() method is given below.
Syntax
- Fileobject.close()
Consider the following example.
- # opens the file file.txt in read mode
- Fileptr = open("file.txt","r")
- If fileptr:
- Print("file is opened successfully")
- #closes the opened file
- Fileptr.close()
After closing the file, we cannot perform any operation in the file. The file needs to be properly closed. If any exception occurs while performing some operations in the file then the program terminates without closing the file.
We should use the following method to overcome such type of problem.
- Try:
- Fileptr = open("file.txt")
- # perform file operations
- Finally:
- Fileptr.close()
The with statement
The with statement was introduced in python 2.5. The with statement is useful in the case of manipulating the files. It is used in the scenario where a pair of statements is to be executed with a block of code in between.
The syntax to open a file using with the statement is given below.
- With open(<file name>, <access mode>) as <file-pointer>:
- #statement suite
The advantage of using with statement is that it provides the guarantee to close the file regardless of how the nested block exits.
It is always suggestible to use the with statement in the case of files because, if the break, return, or exception occurs in the nested block of code then it automatically closes the file, we don't need to write the close() function. It doesn't let the file to corrupt.
Consider the following example.
Example
- With open("file.txt",'r') as f:
- Content = f.read();
- Print(content)
Writing the file
To write some text to a file, we need to open the file using the open method with one of the following access modes.
w: It will overwrite the file if any file exists. The file pointer is at the beginning of the file.
a: It will append the existing file. The file pointer is at the end of the file. It creates a new file if no file exists.
Consider the following example.
Example
- # open the file.txt in append mode. Create a new file if no such file exists.
- Fileptr = open("file2.txt", "w")
- # appending the content to the file
- Fileptr.write('''''Python is the modern day language. It makes things so simple.
- It is the fastest-growing programing language''')
- # closing the opened the file
- Fileptr.close()
Output:
File2.txt
Python is the modern-day language. It makes things so simple. It is the fastest growing programming language.
Snapshot of the file2.txt
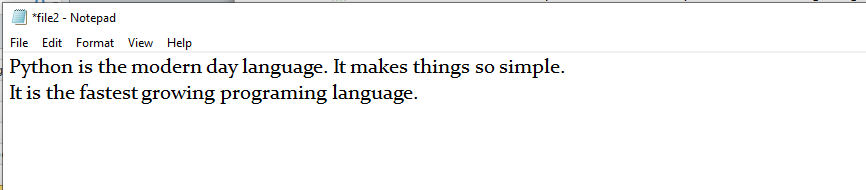
We have opened the file in w mode. The file1.txt file doesn't exist, it created a new file and we have written the content in the file using the write() function.
Example 2
- #open the file.txt in write mode.
- Fileptr = open("file2.txt","a")
- #overwriting the content of the file
- Fileptr.write(" Python has an easy syntax and user-friendly interaction.")
- #closing the opened file
- Fileptr.close()
Output:
Python is the modern day language. It makes things so simple.
It is the fastest growing programing language Python has an easy syntax and user-friendly interaction.
Snapshot of the file2.txt
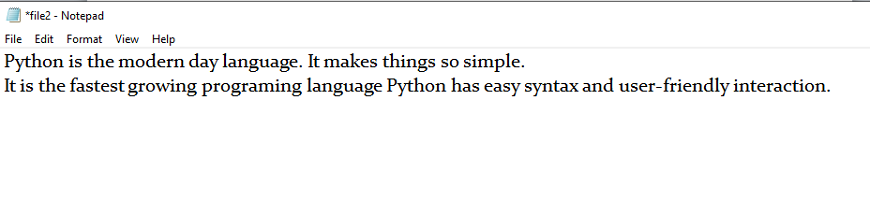
We can see that the content of the file is modified. We have opened the file in a mode and it appended the content in the existing file2.txt.
To read a file using the Python script, the Python provides the read() method. The read() method reads a string from the file. It can read the data in the text as well as a binary format.
The syntax of the read() method is given below.
Syntax:
- Fileobj.read(<count>)
Here, the count is the number of bytes to be read from the file starting from the beginning of the file. If the count is not specified, then it may read the content of the file until the end.
Consider the following example.
Example
- #open the file.txt in read mode. causes error if no such file exists.
- Fileptr = open("file2.txt","r")
- #stores all the data of the file into the variable content
- Content = fileptr.read(10)
- # prints the type of the data stored in the file
- Print(type(content))
- #prints the content of the file
- Print(content)
- #closes the opened file
- Fileptr.close()
Output:
<class 'str'>
Python is
In the above code, we have read the content of file2.txt by using the read() function. We have passed count value as ten which means it will read the first ten characters from the file.
If we use the following line, then it will print all content of the file.
- Content = fileptr.read()
- Print(content)
Output:
Python is the modern-day language. It makes things so simple.
It is the fastest-growing programing language Python has easy an syntax and user-friendly interaction.
Read file through for loop
We can read the file using for loop. Consider the following example.
- #open the file.txt in read mode. causes an error if no such file exists.
- Fileptr = open("file2.txt","r");
- #running a for loop
- For i in fileptr:
- Print(i) # i contains each line of the file
Output:
Python is the modern day language.
It makes things so simple.
Python has easy syntax and user-friendly interaction.
Read Lines of the file
Python facilitates to read the file line by line by using a function readline() method. The readline() method reads the lines of the file from the beginning, i.e., if we use the readline() method two times, then we can get the first two lines of the file.
Consider the following example which contains a function readline() that reads the first line of our file "file2.txt" containing three lines. Consider the following example.
Example 1: Reading lines using readline() function
- #open the file.txt in read mode. causes error if no such file exists.
- Fileptr = open("file2.txt","r");
- #stores all the data of the file into the variable content
- Content = fileptr.readline()
- Content1 = fileptr.readline()
- #prints the content of the file
- Print(content)
- Print(content1)
- #closes the opened file
- Fileptr.close()
Output:
Python is the modern day language.
It makes things so simple.
We called the readline() function two times that's why it read two lines from the file.
Python provides also the readlines() method which is used for the reading lines. It returns the list of the lines till the end of file(EOF) is reached.
Example 2: Reading Lines Using readlines() function
- #open the file.txt in read mode. causes error if no such file exists.
- Fileptr = open("file2.txt","r");
- #stores all the data of the file into the variable content
- Content = fileptr.readlines()
- #prints the content of the file
- Print(content)
- #closes the opened file
- Fileptr.close()
Output:
['Python is the modern day language.\n', 'It makes things so simple.\n', 'Python has easy syntax and user-friendly interaction.']
Creating a new file
The new file can be created by using one of the following access modes with the function open().
x: it creates a new file with the specified name. It causes an error a file exists with the same name.
a: It creates a new file with the specified name if no such file exists. It appends the content to the file if the file already exists with the specified name.
w: It creates a new file with the specified name if no such file exists. It overwrites the existing file.
Consider the following example.
Example 1
- #open the file.txt in read mode. causes error if no such file exists.
- Fileptr = open("file2.txt","x")
- Print(fileptr)
- If fileptr:
- Print("File created successfully")
Output:
<_io.TextIOWrapper name='file2.txt' mode='x' encoding='cp1252'>
File created successfully
File Pointer positions
Python provides the tell() method which is used to print the byte number at which the file pointer currently exists. Consider the following example.
- # open the file file2.txt in read mode
- Fileptr = open("file2.txt","r")
- #initially the filepointer is at 0
- Print("The filepointer is at byte :",fileptr.tell())
- #reading the content of the file
- Content = fileptr.read();
- #after the read operation file pointer modifies. tell() returns the location of the fileptr.
- Print("After reading, the filepointer is at:",fileptr.tell())
Output:
The filepointer is at byte : 0
After reading, the filepointer is at: 117
Modifying file pointer position
In real-world applications, sometimes we need to change the file pointer location externally since we may need to read or write the content at various locations.
For this purpose, the Python provides us the seek() method which enables us to modify the file pointer position externally.
The syntax to use the seek() method is given below.
Syntax:
- <file-ptr>.seek(offset[, from)
The seek() method accepts two parameters:
Offset: It refers to the new position of the file pointer within the file.
From: It indicates the reference position from where the bytes are to be moved. If it is set to 0, the beginning of the file is used as the reference position. If it is set to 1, the current position of the file pointer is used as the reference position. If it is set to 2, the end of the file pointer is used as the reference position.
Consider the following example.
Example
- # open the file file2.txt in read mode
- Fileptr = open("file2.txt","r")
- #initially the filepointer is at 0
- Print("The filepointer is at byte :",fileptr.tell())
- #changing the file pointer location to 10.
- Fileptr.seek(10);
- #tell() returns the location of the fileptr.
- Print("After reading, the filepointer is at:",fileptr.tell())
Output:
The filepointer is at byte : 0
After reading, the filepointer is at: 10
Python OS module
Renaming the file
The Python os module enables interaction with the operating system. The os module provides the functions that are involved in file processing operations like renaming, deleting, etc. It provides us the rename() method to rename the specified file to a new name. The syntax to use the rename() method is given below.
Syntax:
- Rename(current-name, new-name)
The first argument is the current file name and the second argument is the modified name. We can change the file name bypassing these two arguments.
Example 1:
- Import os
- #rename file2.txt to file3.txt
- Os.rename("file2.txt","file3.txt")
Output:
The above code renamed current file2.txt to file3.txt
Removing the file
The os module provides the remove() method which is used to remove the specified file. The syntax to use the remove() method is given below.
- Remove(file-name)
Example 1
- Import os;
- #deleting the file named file3.txt
- Os.remove("file3.txt")
Creating the new directory
The mkdir() method is used to create the directories in the current working directory. The syntax to create the new directory is given below.
Syntax:
- Mkdir(directory name)
Example 1
- Import os
- #creating a new directory with the name new
- Os.mkdir("new")
The getcwd() method
This method returns the current working directory.
The syntax to use the getcwd() method is given below.
Syntax
- Os.getcwd()
Example
- Import os
- Os.getcwd()
Output:
'C:\\Users\\DEVANSH SHARMA'
Changing the current working directory
The chdir() method is used to change the current working directory to a specified directory.
The syntax to use the chdir() method is given below.
Syntax
- Chdir("new-directory")
Example
- Import os
- # Changing current directory with the new directiory
- Os.chdir("C:\\Users\\DEVANSH SHARMA\\Documents")
- #It will display the current working directory
- Os.getcwd()
Output:
'C:\\Users\\DEVANSH SHARMA\\Documents'
Deleting directory
The rmdir() method is used to delete the specified directory.
The syntax to use the rmdir() method is given below.
Syntax
- Os.rmdir(directory name)
Example 1
- Import os
- #removing the new directory
- Os.rmdir("directory_name")
It will remove the specified directory.
Writing Python output to the files
In Python, there are the requirements to write the output of a Python script to a file.
The check_call() method of module subprocess is used to execute a Python script and write the output of that script to a file.
The following example contains two python scripts. The script file1.py executes the script file.py and writes its output to the text file output.txt.
Example
File.py
- Temperatures=[10,-20,-289,100]
- Def c_to_f(c):
- If c< -273.15:
- Return "That temperature doesn't make sense!"
- Else:
- f=c*9/5+32
- Return f
- For t in temperatures:
- Print(c_to_f(t))
File.py
- Import subprocess
- With open("output.txt", "wb") as f:
- Subprocess.check_call(["python", "file.py"], stdout=f)
The file related methods
The file object provides the following methods to manipulate the files on various operating systems.
SN | Method | Description |
1 | File.close() | It closes the opened file. The file once closed, it can't be read or write anymore. |
2 | File.fush() | It flushes the internal buffer. |
3 | File.fileno() | It returns the file descriptor used by the underlying implementation to request I/O from the OS. |
4 | File.isatty() | It returns true if the file is connected to a TTY device, otherwise returns false. |
5 | File.next() | It returns the next line from the file. |
6 | File.read([size]) | It reads the file for the specified size. |
7 | File.readline([size]) | It reads one line from the file and places the file pointer to the beginning of the new line. |
8 | File.readlines([sizehint]) | It returns a list containing all the lines of the file. It reads the file until the EOF occurs using readline() function. |
9 | File.seek(offset[,from) | It modifies the position of the file pointer to a specified offset with the specified reference. |
10 | File.tell() | It returns the current position of the file pointer within the file. |
11 | File.truncate([size]) | It truncates the file to the optional specified size. |
12 | File.write(str) | It writes the specified string to a file |
13 | File.writelines(seq) | It writes a sequence of the strings to a file. |
Python Operators
The operator can be defined as a symbol which is responsible for a particular operation between two operands. Operators are the pillars of a program on which the logic is built in a specific programming language. Python provides a variety of operators, which are described as follows.
- Arithmetic operators
- Comparison operators
- Assignment Operators
- Logical Operators
- Bitwise Operators
- Membership Operators
- Identity Operators
Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations between two operands. It includes + (addition), - (subtraction), *(multiplication), /(divide), %(reminder), //(floor division), and exponent (**) operators.
Consider the following table for a detailed explanation of arithmetic operators.
Operator | Description |
+ (Addition) | It is used to add two operands. For example, if a = 20, b = 10 =>a+b = 30 |
- (Subtraction) | It is used to subtract the second operand from the first operand. If the first operand is less than the second operand, the value results negative. For example, if a = 20, b = 10 => a - b = 10 |
/ (divide) | It returns the quotient after dividing the first operand by the second operand. For example, if a = 20, b = 10 => a/b = 2.0 |
* (Multiplication) | It is used to multiply one operand with the other. For example, if a = 20, b = 10 => a * b = 200 |
% (reminder) | It returns the reminder after dividing the first operand by the second operand. For example, if a = 20, b = 10 =>a%b = 0 |
** (Exponent) | It is an exponent operator represented as it calculates the first operand power to the second operand. |
// (Floor division) | It gives the floor value of the quotient produced by dividing the two operands. |
Comparison operator
Comparison operators are used to comparing the value of the two operands and returns Boolean true or false accordingly. The comparison operators are described in the following table.
Operator | Description |
== | If the value of two operands is equal, then the condition becomes true. |
!= | If the value of two operands is not equal, then the condition becomes true. |
<= | If the first operand is less than or equal to the second operand, then the condition becomes true. |
>= | If the first operand is greater than or equal to the second operand, then the condition becomes true. |
> | If the first operand is greater than the second operand, then the condition becomes true. |
< | If the first operand is less than the second operand, then the condition becomes true. |
Assignment Operators
The assignment operators are used to assign the value of the right expression to the left operand. The assignment operators are described in the following table.
Operator | Description |
= | It assigns the value of the right expression to the left operand. |
+= | It increases the value of the left operand by the value of the right operand and assigns the modified value back to left operand. For example, if a = 10, b = 20 => a+ = b will be equal to a = a+ b and therefore, a = 30. |
-= | It decreases the value of the left operand by the value of the right operand and assigns the modified value back to left operand. For example, if a = 20, b = 10 => a- = b will be equal to a = a- b and therefore, a = 10. |
*= | It multiplies the value of the left operand by the value of the right operand and assigns the modified value back to then the left operand. For example, if a = 10, b = 20 => a* = b will be equal to a = a* b and therefore, a = 200. |
%= | It divides the value of the left operand by the value of the right operand and assigns the reminder back to the left operand. For example, if a = 20, b = 10 => a % = b will be equal to a = a % b and therefore, a = 0. |
**= | a**=b will be equal to a=a**b, for example, if a = 4, b =2, a**=b will assign 4**2 = 16 to a. |
//= | A//=b will be equal to a = a// b, for example, if a = 4, b = 3, a//=b will assign 4//3 = 1 to a. |
Bitwise Operators
The bitwise operators perform bit by bit operation on the values of the two operands. Consider the following example.
For example,
- If a = 7
- b = 6
- Then, binary (a) = 0111
- Binary (b) = 0011
- Hence, a & b = 0011
- a | b = 0111
- a ^ b = 0100
- ~ a = 1000
Operator | Description |
& (binary and) | If both the bits at the same place in two operands are 1, then 1 is copied to the result. Otherwise, 0 is copied. |
| (binary or) | The resulting bit will be 0 if both the bits are zero; otherwise, the resulting bit will be 1. |
^ (binary xor) | The resulting bit will be 1 if both the bits are different; otherwise, the resulting bit will be 0. |
~ (negation) | It calculates the negation of each bit of the operand, i.e., if the bit is 0, the resulting bit will be 1 and vice versa. |
<< (left shift) | The left operand value is moved left by the number of bits present in the right operand. |
>> (right shift) | The left operand is moved right by the number of bits present in the right operand. |
Logical Operators
The logical operators are used primarily in the expression evaluation to make a decision. Python supports the following logical operators.
Operator | Description |
And | If both the expression are true, then the condition will be true. If a and b are the two expressions, a → true, b → true => a and b → true. |
Or | If one of the expressions is true, then the condition will be true. If a and b are the two expressions, a → true, b → false => a or b → true. |
Not | If an expression a is true, then not (a) will be false and vice versa. |
Membership Operators
Python membership operators are used to check the membership of value inside a Python data structure. If the value is present in the data structure, then the resulting value is true otherwise it returns false.
Operator | Description |
In | It is evaluated to be true if the first operand is found in the second operand (list, tuple, or dictionary). |
Not in | It is evaluated to be true if the first operand is not found in the second operand (list, tuple, or dictionary). |
Identity Operators
The identity operators are used to decide whether an element certain class or type.
Operator | Description |
Is | It is evaluated to be true if the reference present at both sides point to the same object. |
Is not | It is evaluated to be true if the reference present at both sides do not point to the same object. |
Operator Precedence
The precedence of the operators is essential to find out since it enables us to know which operator should be evaluated first. The precedence table of the operators in Python is given below.
Operator | Description |
** | The exponent operator is given priority over all the others used in the expression. |
~ + - | The negation, unary plus, and minus. |
* / % // | The multiplication, divide, modules, reminder, and floor division. |
+ - | Binary plus, and minus |
>><< | Left shift. And right shift |
& | Binary and. |
^ | | Binary xor, and or |
<= <>>= | Comparison operators (less than, less than equal to, greater than, greater then equal to). |
<> == != | Equality operators. |
= %= /= //= -= += | Assignment operators |
Is is not | Identity operators |
In not in | Membership operators |
Not or and | Logical operators |
Python - Regular Expressions
A regular expression is a special sequence of characters that helps you match or find other strings or sets of strings, using a specialized syntax held in a pattern. Regular expressions are widely used in UNIX world.
The Python module re provides full support for Perl-like regular expressions in Python. The re module raises the exception re.error if an error occurs while compiling or using a regular expression.
We would cover two important functions, which would be used to handle regular expressions. But a small thing first: There are various characters, which would have special meaning when they are used in regular expression. To avoid any confusion while dealing with regular expressions, we would use Raw Strings as r'expression'.
The match Function
This function attempts to match RE pattern to string with optional flags.
Here is the syntax for this function −
Re.match(pattern, string, flags=0)
Here is the description of the parameters −
Sr.No. | Parameter & Description |
1 | Pattern This is the regular expression to be matched. |
2 | String This is the string, which would be searched to match the pattern at the beginning of string. |
3 | Flags You can specify different flags using bitwise OR (|). These are modifiers, which are listed in the table below. |
The re.match function returns a match object on success, None on failure. We usegroup(num) or groups() function of match object to get matched expression.
Sr.No. | Match Object Method & Description |
1 | Group(num=0) This method returns entire match (or specific subgroup num) |
2 | Groups() This method returns all matching subgroups in a tuple (empty if there weren't any) |
Example
#!/usr/bin/python
Import re
Line="Cats are smarter than dogs"
MatchObj=re.match( r'(.*) are (.*?) .*', line,re.M|re.I)
IfmatchObj:
Print"matchObj.group() : ",matchObj.group()
Print"matchObj.group(1) : ",matchObj.group(1)
Print"matchObj.group(2) : ",matchObj.group(2)
Else:
Print"No match!!"
When the above code is executed, it produces following result −
MatchObj.group() : Cats are smarter than dogs
MatchObj.group(1) : Cats
MatchObj.group(2) : smarter
The search Function
This function searches for first occurrence of RE pattern within string with optional flags.
Here is the syntax for this function −
Re.search(pattern, string, flags=0)
Here is the description of the parameters −
Sr.No. | Parameter & Description |
1 | Pattern This is the regular expression to be matched. |
2 | String This is the string, which would be searched to match the pattern anywhere in the string. |
3 | Flags You can specify different flags using bitwise OR (|). These are modifiers, which are listed in the table below. |
The re.search function returns a match object on success, none on failure. We use group(num) or groups() function of match object to get matched expression.
Sr.No. | Match Object Methods & Description |
1 | Group(num=0) This method returns entire match (or specific subgroup num) |
2 | Groups() This method returns all matching subgroups in a tuple (empty if there weren't any) |
Example
#!/usr/bin/python
Import re
Line="Cats are smarter than dogs";
SearchObj=re.search( r'(.*) are (.*?) .*', line,re.M|re.I)
IfsearchObj:
Print"searchObj.group() : ",searchObj.group()
Print"searchObj.group(1) : ",searchObj.group(1)
Print"searchObj.group(2) : ",searchObj.group(2)
Else:
Print"Nothing found!!"
When the above code is executed, it produces following result −
SearchObj.group() : Cats are smarter than dogs
SearchObj.group(1) : Cats
SearchObj.group(2) : smarter
Matching Versus Searching
Python offers two different primitive operations based on regular expressions: match checks for a match only at the beginning of the string, while search checks for a match anywhere in the string (this is what Perl does by default).
Example
#!/usr/bin/python
Import re
Line="Cats are smarter than dogs";
MatchObj=re.match(r'dogs', line,re.M|re.I)
IfmatchObj:
Print"match -->matchObj.group() : ",matchObj.group()
Else:
Print"No match!!"
SearchObj=re.search(r'dogs', line,re.M|re.I)
IfsearchObj:
Print"search -->searchObj.group() : ",searchObj.group()
Else:
Print"Nothing found!!"
When the above code is executed, it produces the following result −
No match!!
Search -->searchObj.group() : dogs
Search and Replace
One of the most important re methods that use regular expressions is sub.
Syntax
Re.sub(pattern, repl, string, max=0)
This method replaces all occurrences of the RE pattern in string with repl, substituting all occurrences unless max provided. This method returns modified string.
Example
#!/usr/bin/python
Import re
Phone="2004-959-559 # This is Phone Number"
# Delete Python-style comments
Num=re.sub(r'#.*$',"", phone)
Print"Phone Num : ",num
# Remove anything other than digits
Num=re.sub(r'\D',"", phone)
Print"Phone Num : ",num
When the above code is executed, it produces the following result −
Phone Num : 2004-959-559
Phone Num : 2004959559
Regular Expression Modifiers: Option Flags
Regular expression literals may include an optional modifier to control various aspects of matching. The modifiers are specified as an optional flag. You can provide multiple modifiers using exclusive OR (|), as shown previously and may be represented by one of these −
Sr.No. | Modifier & Description |
1 | Re.I Performs case-insensitive matching. |
2 | Re.L Interprets words according to the current locale. This interpretation affects the alphabetic group (\w and \W), as well as word boundary behavior(\b and \B). |
3 | Re.M Makes $ match the end of a line (not just the end of the string) and makes ^ match the start of any line (not just the start of the string). |
4 | Re.S Makes a period (dot) match any character, including a newline. |
5 | Re.U Interprets letters according to the Unicode character set. This flag affects the behavior of \w, \W, \b, \B. |
6 | Re.X Permits "cuter" regular expression syntax. It ignores whitespace (except inside a set [] or when escaped by a backslash) and treats unescaped # as a comment marker. |
Regular Expression Patterns
Except for control characters, (+ ? . * ^ $ ( ) [ ] { } | \), all characters match themselves. You can escape a control character by preceding it with a backslash.
Following table lists the regular expression syntax that is available in Python −
Sr.No. | Pattern & Description |
1 | ^ Matches beginning of line. |
2 | $ Matches end of line. |
3 | . Matches any single character except newline. Using m option allows it to match newline as well. |
4 | [...] Matches any single character in brackets. |
5 | [^...] Matches any single character not in brackets |
6 | Re* Matches 0 or more occurrences of preceding expression. |
7 | Re+ Matches 1 or more occurrence of preceding expression. |
8 | Re? Matches 0 or 1 occurrence of preceding expression. |
9 | Re{ n} Matches exactly n number of occurrences of preceding expression. |
10 | Re{ n,} Matches n or more occurrences of preceding expression. |
11 | Re{ n, m} Matches at least n and at most m occurrences of preceding expression. |
12 | a| b Matches either a or b. |
13 | (re) Groups regular expressions and remembers matched text. |
14 | (?imx) Temporarily toggles on i, m, or x options within a regular expression. If in parentheses, only that area is affected. |
15 | (?-imx) Temporarily toggles off i, m, or x options within a regular expression. If in parentheses, only that area is affected. |
16 | (?: re) Groups regular expressions without remembering matched text. |
17 | (?imx: re) Temporarily toggles on i, m, or x options within parentheses. |
18 | (?-imx: re) Temporarily toggles off i, m, or x options within parentheses. |
19 | (?#...) Comment. |
20 | (?= re) Specifies position using a pattern. Doesn't have a range. |
21 | (?! re) Specifies position using pattern negation. Doesn't have a range. |
22 | (?> re) Matches independent pattern without backtracking. |
23 | \w Matches word characters. |
24 | \W Matches nonword characters. |
25 | \s Matches whitespace. Equivalent to [\t\n\r\f]. |
26 | \S Matches nonwhitespace. |
27 | \d Matches digits. Equivalent to [0-9]. |
28 | \D Matches nondigits. |
29 | \A Matches beginning of string. |
30 | \Z Matches end of string. If a newline exists, it matches just before newline. |
31 | \z Matches end of string. |
32 | \G Matches point where last match finished. |
33 | \b Matches word boundaries when outside brackets. Matches backspace (0x08) when inside brackets. |
34 | \B Matches nonword boundaries. |
35 | \n, \t, etc. Matches newlines, carriage returns, tabs, etc. |
36 | \1...\9 Matches nth grouped subexpression. |
37 | \10 Matches nth grouped subexpression if it matched already. Otherwise refers to the octal representation of a character code. |
Regular Expression Examples
Literal characters
Sr.No. | Example & Description |
1 | Python Match "python". |
Character classes
Sr.No. | Example & Description |
1 | [Pp]ython Match "Python" or "python" |
2 | Rub[ye] Match "ruby" or "rube" |
3 | [aeiou] Match any one lowercase vowel |
4 | [0-9] Match any digit; same as [0123456789] |
5 | [a-z] Match any lowercase ASCII letter |
6 | [A-Z] Match any uppercase ASCII letter |
7 | [a-zA-Z0-9] Match any of the above |
8 | [^aeiou] Match anything other than a lowercase vowel |
9 | [^0-9] Match anything other than a digit |
Special Character Classes
Sr.No. | Example & Description |
1 | . Match any character except newline |
2 | \d Match a digit: [0-9] |
3 | \D Match a nondigit: [^0-9] |
4 | \s Match a whitespace character: [ \t\r\n\f] |
5 | \S Match nonwhitespace: [^ \t\r\n\f] |
6 | \w Match a single word character: [A-Za-z0-9_] |
7 | \W Match a nonword character: [^A-Za-z0-9_] |
Repetition Cases
Sr.No. | Example & Description |
1 | Ruby? Match "rub" or "ruby": the y is optional |
2 | Ruby* Match "rub" plus 0 or more ys |
3 | Ruby+ Match "rub" plus 1 or more ys |
4 | \d{3} Match exactly 3 digits |
5 | \d{3,} Match 3 or more digits |
6 | \d{3,5} Match 3, 4, or 5 digits |
Nongreedy repetition
This matches the smallest number of repetitions −
Sr.No. | Example & Description |
1 | <.*> Greedy repetition: matches "<python>perl>" |
2 | <.*?> Nongreedy: matches "<python>" in "<python>perl>" |
Grouping with Parentheses
Sr.No. | Example & Description |
1 | \D\d+ No group: + repeats \d |
2 | (\D\d)+ Grouped: + repeats \D\d pair |
3 | ([Pp]ython(, )?)+ Match "Python", "Python, python, python", etc. |
Backreferences
This matches a previously matched group again −
Sr.No. | Example & Description |
1 | ([Pp])ython&\1ails Match python&pails or Python&Pails |
2 | (['"])[^\1]*\1 Single or double-quoted string. \1 matches whatever the 1st group matched. \2 matches whatever the 2nd group matched, etc. |
Alternatives
Sr.No. | Example & Description |
1 | Python|perl Match "python" or "perl" |
2 | Rub(y|le)) Match "ruby" or "ruble" |
3 | Python(!+|\?) "Python" followed by one or more !or one ? |
Anchors
This needs to specify match position.
Sr.No. | Example & Description |
1 | ^Python Match "Python" at the start of a string or internal line |
2 | Python$ Match "Python" at the end of a string or line |
3 | \APython Match "Python" at the start of a string |
4 | Python\Z Match "Python" at the end of a string |
5 | \bPython\b Match "Python" at a word boundary |
6 | \brub\B \B is nonword boundary: match "rub" in "rube" and "ruby" but not alone |
7 | Python(?=!) Match "Python", if followed by an exclamation point. |
8 | Python(?!!) Match "Python", if not followed by an exclamation point. |
Special Syntax with Parentheses
Sr.No. | Example & Description |
1 | R(?#comment) Matches "R". All the rest is a comment |
2 | R(?i)uby Case-insensitive while matching "uby" |
3 | R(?i:uby) Same as above |
4 | Rub(?:y|le)) Group only without creating \1 backreference |
Membership operators are operators used to validate the membership of a value. It test for membership in a sequence, such as strings, lists, or tuples.
- In operator : The ‘in’ operator is used to check if a value exists in a sequence or not. Evaluates to true if it finds a variable in the specified sequence and false otherwise.
# Python program to illustrate # Finding common member in list # using 'in' operator List1=[1,2,3,4,5] List2=[6,7,8,9] Foritem inlist1: Ifitem inlist2: Print("overlapping") Else: Print("not overlapping") |
Output:
Not overlapping
Same example without using in operator:
# Python program to illustrate # Finding common member in list # without using 'in' operator
# Define a function() that takes two lists Defoverlapping(list1,list2):
c=0 d=0 Fori inlist1: c+=1 Fori inlist2: d+=1 Fori inrange(0,c): Forj inrange(0,d): If(list1[i]==list2[j]): Return1 Return0 List1=[1,2,3,4,5] List2=[6,7,8,9] If(overlapping(list1,list2)): Print("overlapping") Else: Print("not overlapping") |
Output:
Not overlapping
2. ‘not in’ operator- Evaluates to true if it does not finds a variable in the specified sequence and false otherwise.
# Python program to illustrate # not 'in' operator x =24 y =20 List=[10, 20, 30, 40, 50];
If( x notinlist): Print("x is NOT present in given list") Else: Print("x is present in given list")
If( y inlist): Print("y is present in given list") Else: Print("y is NOT present in given list") |
Identity operators
In Python are used to determine whether a value is of a certain class or type. They are usually used to determine the type of data a certain variable contains.
There are different identity operators such as
- ‘is’ operator – Evaluates to true if the variables on either side of the operator point to the same object and false otherwise.
# Python program to illustrate the use # of 'is' identity operator x =5 If(type(x) isint): Print("true") Else: Print("false") |
Output:
True
2. ‘is not’ operator – Evaluates to false if the variables on either side of the operator point to the same object and true otherwise.
# Python program to illustrate the # use of 'is not' identity operator x =5.2 If(type(x) isnotint): Print("true") Else: Print("false") |
Output:
True
Python Loops
The flow of the programs written in any programming language is sequential by default. Sometimes we may need to alter the flow of the program. The execution of a specific code may need to be repeated several numbers of times.
For this purpose, The programming languages provide various types of loops which are capable of repeating some specific code several numbers of times. Consider the following diagram to understand the working of a loop statement.
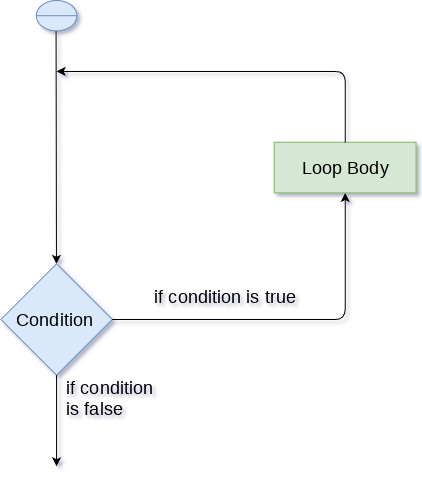
Why we use loops in python?
The looping simplifies the complex problems into the easy ones. It enables us to alter the flow of the program so that instead of writing the same code again and again, we can repeat the same code for a finite number of times. For example, if we need to print the first 10 natural numbers then, instead of using the print statement 10 times, we can print inside a loop which runs up to 10 iterations.
Advantages of loops
There are the following advantages of loops in Python.
- It provides code re-usability.
- Using loops, we do not need to write the same code again and again.
- Using loops, we can traverse over the elements of data structures (array or linked lists).
There are the following loop statements in Python.
Loop Statement | Description |
For loop | The for loop is used in the case where we need to execute some part of the code until the given condition is satisfied. The for loop is also called as a per-tested loop. It is better to use for loop if the number of iteration is known in advance. |
While loop | The while loop is to be used in the scenario where we don't know the number of iterations in advance. The block of statements is executed in the while loop until the condition specified in the while loop is satisfied. It is also called a pre-tested loop. |
Do-while loop | The do-while loop continues until a given condition satisfies. It is also called post tested loop. It is used when it is necessary to execute the loop at least once (mostly menu driven programs). |
Python for loop
The forloop in Python is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like list, tuple, or dictionary.
The syntax of for loop in python is given below.
- For iterating_var in sequence:
- Statement(s)
The for loop flowchart
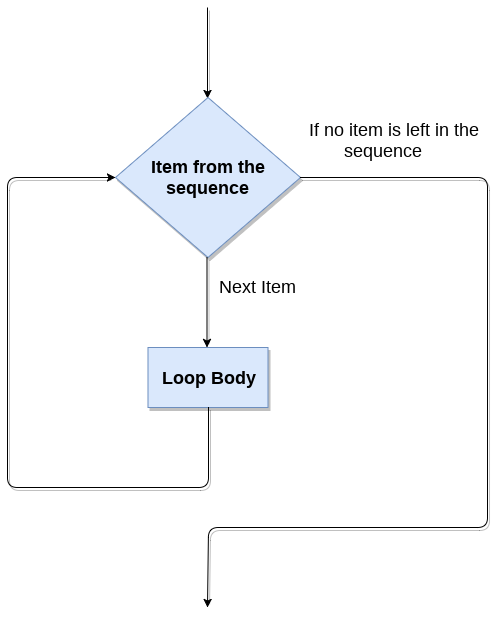
For loop Using Sequence
Example-1: Iterating string using for loop
- Str = "Python"
- For i in str:
- Print(i)
Output:
P
y
t
h
o
n
Example- 2: Program to print the table of the given number .
- List = [1,2,3,4,5,6,7,8,9,10]
- n = 5
- For i in list:
- c = n*i
- Print(c)
Output:
5
10
15
20
25
30
35
40
45
50s
Example-4: Program to print the sum of the given list.
- List = [10,30,23,43,65,12]
- Sum = 0
- For i in list:
- Sum = sum+i
- Print("The sum is:",sum)
Output:
The sum is: 183
For loop Using range() function
The range() function
The range() function is used to generate the sequence of the numbers. If we pass the range(10), it will generate the numbers from 0 to 9. The syntax of the range() function is given below.
Syntax:
- Range(start,stop,step size)
- The start represents the beginning of the iteration.
- The stop represents that the loop will iterate till stop-1. The range(1,5) will generate numbers 1 to 4 iterations. It is optional.
- The step size is used to skip the specific numbers from the iteration. It is optional to use. By default, the step size is 1. It is optional.
Consider the following examples:
Example-1: Program to print numbers in sequence.
- For i in range(10):
- Print(i,end = ' ')
Output:
0 1 2 3 4 5 6 7 8 9
Example - 2: Program to print table of given number.
- n = int(input("Enter the number "))
- For i in range(1,11):
- c = n*i
- Print(n,"*",i,"=",c)
Output:
Enter the number 10
10 * 1 = 10
10 * 2 = 20
10 * 3 = 30
10 * 4 = 40
10 * 5 = 50
10 * 6 = 60
10 * 7 = 70
10 * 8 = 80
10 * 9 = 90
10 * 10 = 100
Example-3: Program to print even number using step size in range().
- n = int(input("Enter the number "))
- For i in range(2,n,2):
- Print(i)
Output:
Enter the number 20
2
4
6
8
10
12
14
16
18
We can also use the range() function with sequence of numbers. The len() function is combined with range() function which iterate through a sequence using indexing. Consider the following example.
- List = ['Peter','Joseph','Ricky','Devansh']
- For i in range(len(list)):
- Print("Hello",list[i])
Output:
Hello Peter
Hello Joseph
Hello Ricky
Hello Devansh
Nested for loop in python
Python allows us to nest any number of for loops inside a for loop. The inner loop is executed n number of times for every iteration of the outer loop. The syntax is given below.
Syntax
- For iterating_var1 in sequence: #outer loop
- For iterating_var2 in sequence: #inner loop
- #block of statements
- #Other statements
Example- 1: Nested for loop
- # User input for number of rows
- Rows = int(input("Enter the rows:"))
- # Outer loop will print number of rows
- For i in range(0,rows+1):
- # Inner loop will print number of Astrisk
- For j in range(i):
- Print("*",end = '')
- Print()
Output:
Enter the rows:5
*
**
***
****
*****
Example-2: Program to number pyramid.
- Rows = int(input("Enter the rows"))
- For i in range(0,rows+1):
- For j in range(i):
- Print(i,end = '')
- Print()
Output:
1
22
333
4444
55555
Using else statement with for loop
Unlike other languages like C, C++, or Java, Python allows us to use the else statement with the for loop which can be executed only when all the iterations are exhausted. Here, we must notice that if the loop contains any of the break statement then the else statement will not be executed.
Example 1
- For i in range(0,5):
- Print(i)
- Else:
- Print("for loop completely exhausted, since there is no break.")
Output:
0
1
2
3
4
For loop completely exhausted, since there is no break.
The for loop completely exhausted, since there is no break.
Example 2
- For i in range(0,5):
- Print(i)
- Break;
- Else:print("for loop is exhausted");
- Print("The loop is broken due to break statement...came out of the loop")
In the above example, the loop is broken due to the break statement; therefore, the else statement will not be executed. The statement present immediate next to else block will be executed.
Output:
0
The loop is broken due to the break statement...came out of the loop.
Python While loop
The Python while loop allows a part of the code to be executed until the given condition returns false. It is also known as a pre-tested loop.
It can be viewed as a repeating if statement. When we don't know the number of iterations then the while loop is most effective to use.
The syntax is given below.
- While expression:
- Statements
Here, the statements can be a single statement or a group of statements. The expression should be any valid Python expression resulting in true or false. The true is any non-zero value and false is 0.
While loop Flowchart
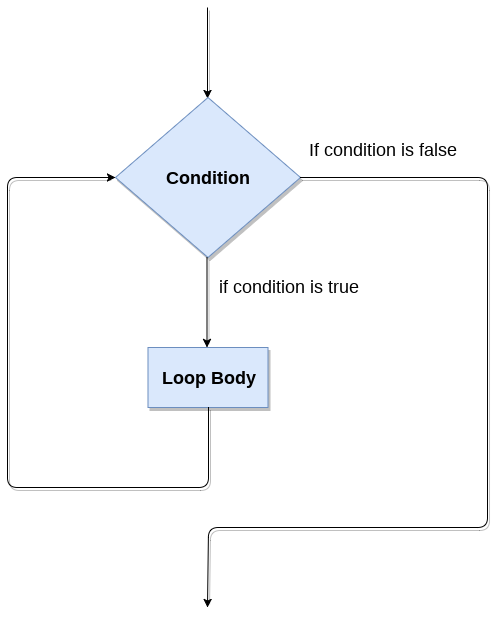
Loop Control Statements
We can change the normal sequence of while loop's execution using the loop control statement. When the while loop's execution is completed, all automatic objects defined in that scope are demolished. Python offers the following control statement to use within the while loop.
1. Continue Statement - When the continue statement is encountered, the control transfer to the beginning of the loop. Let's understand the following example.
Example:
- # prints all letters except 'a' and 't'
- i = 0
- Str1 = 'javatpoint'
- While i < len(str1):
- If str1[i] == 'a' or str1[i] == 't':
- i += 1
- Continue
- Print('Current Letter :', a[i])
- i += 1
Output:
Current Letter : j
Current Letter : v
Current Letter : p
Current Letter : o
Current Letter :i
Current Letter : n
2. Break Statement - When the break statement is encountered, it brings control out of the loop.
Example:
- # The control transfer is transfered
- # when break statement soon it sees t
- i = 0
- Str1 = 'javatpoint'
- While i < len(str1):
- If str1[i] == 't':
- i += 1
- Break
- Print('Current Letter :', str1[i])
- i += 1
Output:
Current Letter : j
Current Letter : a
Current Letter : v
Current Letter : a
3. Pass Statement - The pass statement is used to declare the empty loop. It is also used to define empty class, function, and control statement. Let's understand the following example.
Example -
- # An empty loop
- Str1 = 'javatpoint'
- i = 0
- While i < len(str1):
- i += 1
- Pass
- Print('Value of i :', i)
Output:
Value of i : 10
Example-1: Program to print 1 to 10 using while loop
- i=1
- #The while loop will iterate until condition becomes false.
- While(i<=10):
- Print(i)
- i=i+1
Output:
1
2
3
4
5
6
7
8
9
10
Example -2: Program to print table of given numbers.
- i=1
- Number=0
- b=9
- Number = int(input("Enter the number:"))
- While i<=10:
- Print("%d X %d = %d \n"%(number,i,number*i))
- i = i+1
Output:
Enter the number:10
10 X 1 = 10
10 X 2 = 20
10 X 3 = 30
10 X 4 = 40
10 X 5 = 50
10 X 6 = 60
10 X 7 = 70
10 X 8 = 80
10 X 9 = 90
10 X 10 = 100
Infinite while loop
If the condition is given in the while loop never becomes false, then the while loop will never terminate, and it turns into the infinite while loop.
Any non-zero value in the while loop indicates an always-true condition, whereas zero indicates the always-false condition. This type of approach is useful if we want our program to run continuously in the loop without any disturbance.
Example 1
- While (1):
- Print("Hi! we are inside the infinite while loop")
Output:
Hi! we are inside the infinite while loop
Hi! we are inside the infinite while loop
Example 2
- Var = 1
- While(var != 2):
- i = int(input("Enter the number:"))
- Print("Entered value is %d"%(i))
Output:
Enter the number:10
Entered value is 10
Enter the number:10
Entered value is 10
Enter the number:10
Entered value is 10
Infinite time
Using else with while loop
Python allows us to use the else statement with the while loop also. The else block is executed when the condition given in the while statement becomes false. Like for loop, if the while loop is broken using break statement, then the else block will not be executed, and the statement present after else block will be executed. The else statement is optional to use with the while loop. Consider the following example.
Example 1
- i=1
- While(i<=5):
- Print(i)
- i=i+1
- Else:
- Print("The while loop exhausted")
Example 2
- i=1
- While(i<=5):
- Print(i)
- i=i+1
- If(i==3):
- Break
- Else:
- Print("The while loop exhausted")
Output:
1
2
In the above code, when the break statement encountered, then while loop stopped its execution and skipped the else statement.
Example-3 Program to print Fibonacci numbers to given limit
- Terms = int(input("Enter the terms "))
- # first two intial terms
- a = 0
- b = 1
- Count = 0
- # check if the number of terms is Zero or negative
- If (terms <= 0):
- Print("Please enter a valid integer")
- Elif (terms == 1):
- Print("Fibonacci sequence upto",limit,":")
- Print(a)
- Else:
- Print("Fibonacci sequence:")
- While (count < terms) :
- Print(a, end = ' ')
- c = a + b
- # updateing values
- a = b
- b = c
- Count += 1
Output:
Enter the terms 10
Fibonacci sequence:
0 1 1 2 3 5 8 13 21 34
Python break statement
The break is a keyword in python which is used to bring the program control out of the loop. The break statement breaks the loops one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops. In other words, we can say that break is used to abort the current execution of the program and the control goes to the next line after the loop.
The break is commonly used in the cases where we need to break the loop for a given condition.
The syntax of the break is given below.
- #loop statements
- Break;
Example 1
- List =[1,2,3,4]
- Count = 1;
- For i in list:
- If i == 4:
- Print("item matched")
- Count = count + 1;
- Break
- Print("found at",count,"location");
Output:
Item matched
Found at 2 location
Example 2
- Str = "python"
- For i in str:
- If i == 'o':
- Break
- Print(i);
Output:
p
y
t
h
Example 3: break statement with while loop
- i = 0;
- While 1:
- Print(i," ",end=""),
- i=i+1;
- If i == 10:
- Break;
- Print("came out of while loop");
Output:
0 1 2 3 4 5 6 7 8 9 came out of while loop
Example 3
- n=2
- While 1:
- i=1;
- While i<=10:
- Print("%d X %d = %d\n"%(n,i,n*i));
- i = i+1;
- Choice = int(input("Do you want to continue printing the table, press 0 for no?"))
- If choice == 0:
- Break;
- n=n+1
Output:
2 X 1 = 2
2 X 2 = 4
2 X 3 = 6
2 X 4 = 8
2 X 5 = 10
2 X 6 = 12
2 X 7 = 14
2 X 8 = 16
2 X 9 = 18
2 X 10 = 20
Do you want to continue printing the table, press 0 for no?1
3 X 1 = 3
3 X 2 = 6
3 X 3 = 9
3 X 4 = 12
3 X 5 = 15
3 X 6 = 18
3 X 7 = 21
3 X 8 = 24
3 X 9 = 27
3 X 10 = 30
Do you want to continue printing the table, press 0 for no?0
Python continue Statement
The continue statement in Python is used to bring the program control to the beginning of the loop. The continue statement skips the remaining lines of code inside the loop and start with the next iteration. It is mainly used for a particular condition inside the loop so that we can skip some specific code for a particular condition.The continue statement in Python is used to bring the program control to the beginning of the loop. The continue statement skips the remaining lines of code inside the loop and start with the next iteration. It is mainly used for a particular condition inside the loop so that we can skip some specific code for a particular condition.
Syntax
- #loop statements
- Continue
- #the code to be skipped
Flow Diagram
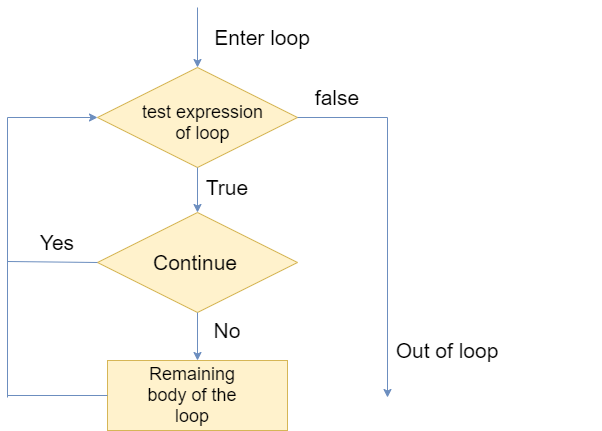
Consider the following examples.
Example 1
- i = 0
- While(i < 10):
- i = i+1
- If(i == 5):
- Continue
- Print(i)
Output:
1
2
3
4
6
7
8
9
10
Observe the output of above code, the value 5 is skipped because we have provided the if condition using with continue statement in while loop. When it matched with the given condition then control transferred to the beginning of the while loop and it skipped the value 5 from the code.
Let's have a look at another example:
Example 2
- Str = "JavaTpoint"
- For i in str:
- If(i == 'T'):
- Continue
- Print(i)
Output:
J
a
v
a
p
o
i
n
t
Pass Statement
The pass statement is a null operation since nothing happens when it is executed. It is used in the cases where a statement is syntactically needed but we don't want to use any executable statement at its place.
For example, it can be used while overriding a parent class method in the subclass but don't want to give its specific implementation in the subclass.
Pass is also used where the code will be written somewhere but not yet written in the program file. Consider the following example.
Example
- List = [1,2,3,4,5]
- Flag = 0
- For i in list:
- Print("Current element:",i,end=" ");
- If i==3:
- Pass
- Print("\nWe are inside pass block\n");
- Flag = 1
- If flag==1:
- Print("\nCame out of pass\n");
- Flag=0
Output:
Current element: 1 Current element: 2 Current element: 3
We are inside pass block
Came out of pass
Current element: 4 Current element: 5
Reference Books:
1 Computers Today by Sanders.
2 Fundamentals of Computers TTTI Publication.
3 Learning Python by Mark Lutz, 5th edition
4 Python cookbook, by David Beazley , 3rd Edition
5 Python Essential Reference, by David Beazley , 4th edition
6 Python in a Nutshell, by Alex Mortelli, 2nd Edition.
7 Python programming: An Introduction to computer science, by John Zelle, 2nd Edition.