UNIT- 2
Basic Introduction to Programming Languages
A programming language defines a set of instructions that are compiled together to perform a specific task by the CPU (Central Processing Unit). The programming language mainly refers to high-level languages such as C, C++, Pascal, Ada, COBOL, etc.
Each programming language contains a unique set of keywords and syntax, which are used to create a set of instructions. Thousands of programming languages have been developed till now, but each language has its specific purpose. These languages vary in the level of abstraction they provide from the hardware. Some programming languages provide less or no abstraction while some provide higher abstraction. Based on the levels of abstraction, they can be classified into two categories:
- Low-level language
- High-level language
The image which is given below describes the abstraction level from hardware. As we can observe from the below image that the machine language provides no abstraction, assembly language provides less abstraction whereas high-level language provides a higher level of abstraction.
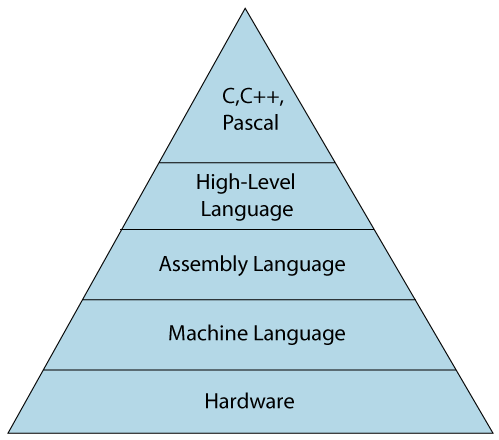
Low-level language
The low-level language is a programming language that provides no abstraction from the hardware, and it is represented in 0 or 1 forms, which are the machine instructions. The languages that come under this category are the Machine level language and Assembly language.
Machine-level language
The machine-level language is a language that consists of a set of instructions that are in the binary form 0 or 1. As we know that computers can understand only machine instructions, which are in binary digits, i.e., 0 and 1, so the instructions given to the computer can be only in binary codes. Creating a program in a machine-level language is a very difficult task as it is not easy for the programmers to write the program in machine instructions. It is error-prone as it is not easy to understand, and its maintenance is also very high. A machine-level language is not portable as each computer has its machine instructions, so if we write a program in one computer will no longer be valid in another computer.
The different processor architectures use different machine codes, for example, a PowerPC processor contains RISC architecture, which requires different code than intel x86 processor, which has a CISC architecture.
Assembly Language
The assembly language contains some human-readable commands such as mov, add, sub, etc. The problems which we were facing in machine-level language are reduced to some extent by using an extended form of machine-level language known as assembly language. Since assembly language instructions are written in English words like mov, add, sub, so it is easier to write and understand.
As we know that computers can only understand the machine-level instructions, so we require a translator that converts the assembly code into machine code. The translator used for translating the code is known as an assembler.
The assembly language code is not portable because the data is stored in computer registers, and the computer has to know the different sets of registers.
The assembly code is not faster than machine code because the assembly language comes above the machine language in the hierarchy, so it means that assembly language has some abstraction from the hardware while machine language has zero abstraction.
Differences between Machine-Level language and Assembly language
The following are the differences between machine-level language and assembly language:
Machine-level language | Assembly language |
The machine-level language comes at the lowest level in the hierarchy, so it has zero abstraction level from the hardware. | The assembly language comes above the machine language means that it has less abstraction level from the hardware. |
It cannot be easily understood by humans. | It is easy to read, write, and maintain. |
The machine-level language is written in binary digits, i.e., 0 and 1. | The assembly language is written in simple English language, so it is easily understandable by the users. |
It does not require any translator as the machine code is directly executed by the computer. | In assembly language, the assembler is used to convert the assembly code into machine code. |
It is a first-generation programming language. | It is a second-generation programming language. |
High-Level Language
The high-level language is a programming language that allows a programmer to write the programs which are independent of a particular type of computer. The high-level languages are considered as high-level because they are closer to human languages than machine-level languages.
When writing a program in a high-level language, then the whole attention needs to be paid to the logic of the problem.
A compiler is required to translate a high-level language into a low-level language.
Advantages of a high-level language
- The high-level language is easy to read, write, and maintain as it is written in English like words.
- The high-level languages are designed to overcome the limitation of low-level language, i.e., portability. The high-level language is portable; i.e., these languages are machine-independent.
Differences between Low-Level language and High-Level language
The following are the differences between low-level language and high-level language:
Low-level language | High-level language |
It is a machine-friendly language, i.e., the computer understands the machine language, which is represented in 0 or 1. | It is a user-friendly language as this language is written in simple English words, which can be easily understood by humans. |
The low-level language takes more time to execute. | It executes at a faster pace. |
It requires the assembler to convert the assembly code into machine code. | It requires the compiler to convert the high-level language instructions into machine code. |
The machine code cannot run on all machines, so it is not a portable language. | The high-level code can run all the platforms, so it is a portable language. |
It is memory efficient. | It is less memory efficient. |
Debugging and maintenance are not easier in a low-level language. | Debugging and maintenance are easier in a high-level language. |
The high-level programming languages can be categorized into different types on the basis of the application area in which they are employed as well as the different design paradigms supported by them. The high-level programming languages are designed for use in a number of areas. Each high-level language is designed by keeping its target application area in mind. Some of the high-level languages are best suited for business domains, while others are apt in the scientific domain only. The high-level language can be categorized on the basis of the various programming paradigms approved by them. The programming paradigms refer to the approach employed by the programming language for solving the different types of problem.
1. Categorisation based on Application
On the basis of application area the high level language can be divided into the following types:
i) Commercial languages
These programming languages are dedicated to the commercial domain and are specially designed for solving business-related problems. These languages can be used in organization for processing handling the data related to payroll, accounts payable and tax building applications. COBOL is the best example of the commercial based high-level programming language employed in the business domain.
Ii) Scientific languages
These programming languages are dedicated to the scientific domain and are specially designed for solving different scientific and mathematical problems. These languages can be used to develop programs for performing complex calculation during scientific research. FORTRAN is the best example of scientific based language.
Iii) Special purpose languages
These programming languages are specially designed for performing some dedicated functions. For example, SQL is a high-level language specially designed to interact with the database programs only. Therefore we can say that the special purpose high-level language is designed to support a particular domain area only.
Iv) General purpose languages
These programming languages are used for developing different types of software application regardless of their application area. The various examples of general purpose high-level programming languages are BASIC, C, C++, and java.
2. Categorisation based on Design paradigm
On the basis of design paradigms the high level programming languages can be categorised into the following types:
i) Procedure-oriented languages
These programming languages are also called an imperative programming language. In this language, a program is written as a sequence of procedures. Each procedure contains a series of instruction for performing a specific task. Each procedure can be called by the other procedures during the program execution. In this type of programming paradigms, a code once written in the form of a procedure can be used any number of times in the program by only specifying the corresponding procedure name. Therefore the procedure-oriented language allows the data to move freely around the system. The various examples of procedure-oriented language are FORTRAN, ALGOL, C, BASIC, and ADA.
Ii) Logic-oriented languages
These languages use logic programming paradigms as the design approach for solving various computational problems. In this programming paradigms predicate logic is used to describe the nature of a problem by defining the relationship between rules and facts. Prolog is the best example of the logic-oriented programming language.
Iii) Object-oriented languages
These languages use object-oriented programming paradigms as the design approach for solving a given problem. In this programming language, a problem is divided into a number of objects which can interact by passing messages to each other. C++ and C# are the examples of object-oriented programming language.
Assembler
In computer science, assembler is a program which converts assembly language into machine code. A computer doesn’t understand human languages like English or french, but it deals in a much simpler language called binary language, but a programmer can not write the whole program with its complexity in a binary language therefore we need a program that can convert the human written language (assembly language) into binary language, these software’s are called assemblers.
In assembler, a programmer can write a program into sequence of assembler instructions, the sequence of assembler instruction is known as source code and source program.
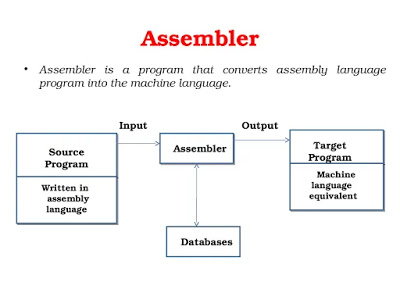
Compiler
A Compiler is a program that converts a number of statements of program into binary language, but it is more intelligent than interpreter because it goes through the entire code at once and can tell the possible errors and limits and ranges. But this makes its operating time a little slower.it is platform-dependent. Its help to detect error and get displayed after reading the entire code by compiler.
In other words we can say that, “Compilers turns the high level language to binary language or machine code at only time once”, it is known as Compiler.
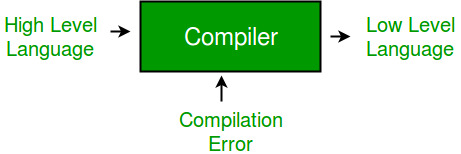
Interpreter
An interpreter is also a program like a compiler that converts assembly language into binary but an interpreter goes through one line of code at a time and executes it and then goes on to the next line of the code and then the next and keeps going on until there is an error in the line or the code has completed.It is 5 to 25 times faster than a compiler but it stops at the line where error occurs and then again if the next line has an error too, where as a compiler gives all the errors in the code at once.if changes are made on that code which is already compiled then the changed code will need to be compiled and added to compiled code or the entire code need to be re-compiled.
Also, a compiler saves the machine codes for future use permanently but an interpreter doesn’t, but an interpreter occupies less memory.
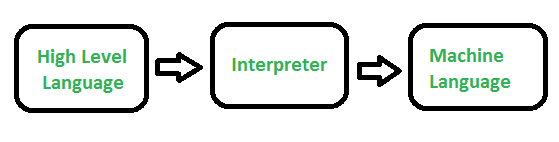
Interpreter is differ from compiler such as,
- Interpreter is faster than compiler.
- It contains less memory.
- Interpreter executes the instructions in to source programming language.
There are several types of interpreter:
- Syntax-directed interpreter
- Threaded interpreter
- Bytecode interpreter
Linker
For a code to run we need to include a header file or a file saved from the library which are pre-defined if they are not included in the beginning of the program then after execution the compiler will generate errors, and the code will not work.
Linker is a program that holds one or more object files which is created by compiler, combines them into one executable file.Linking is implemented at both time,load time and compile time. Compile time is when high level language is turns to machine code and load time is when the code is loaded into the memory by loader.
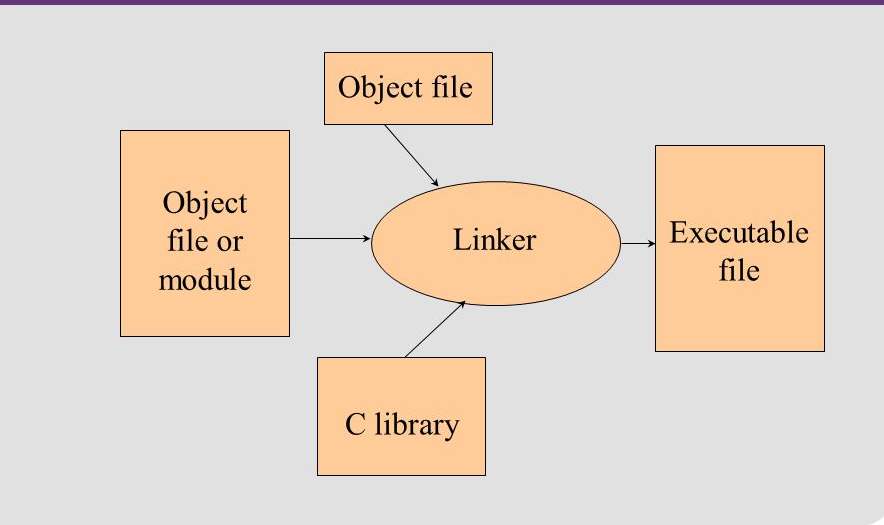
Linker is of two types:
1.Dynamic Linker:-
- It is implemented during run time.
- It requires less memory.
- In dynamic linking there are many chances of error and failure chances.
- Linking stored the program in virtual memory to save RAM,So we have need to shared library
2.Static Linker:-
- It is implemented during compilation of source program.
- It requires more memory.
- Linking is implemented before execution in static linking.
- It is faster and portable.
- In static linking there are less chances to error and No chances to failure.
Loader
Loader is a program that loads machine codes of a program into the system memory.It is part of the OS of the computer that is responsible for loading the program. It is the bare beginning of the execution of a program. Loading a program involves reading the contents of executable file into memory. Only after the program is loaded the operating system starts the program by passing control to the loaded program code. All the OS that support loading have loader and many have loaders permanently in their memory.
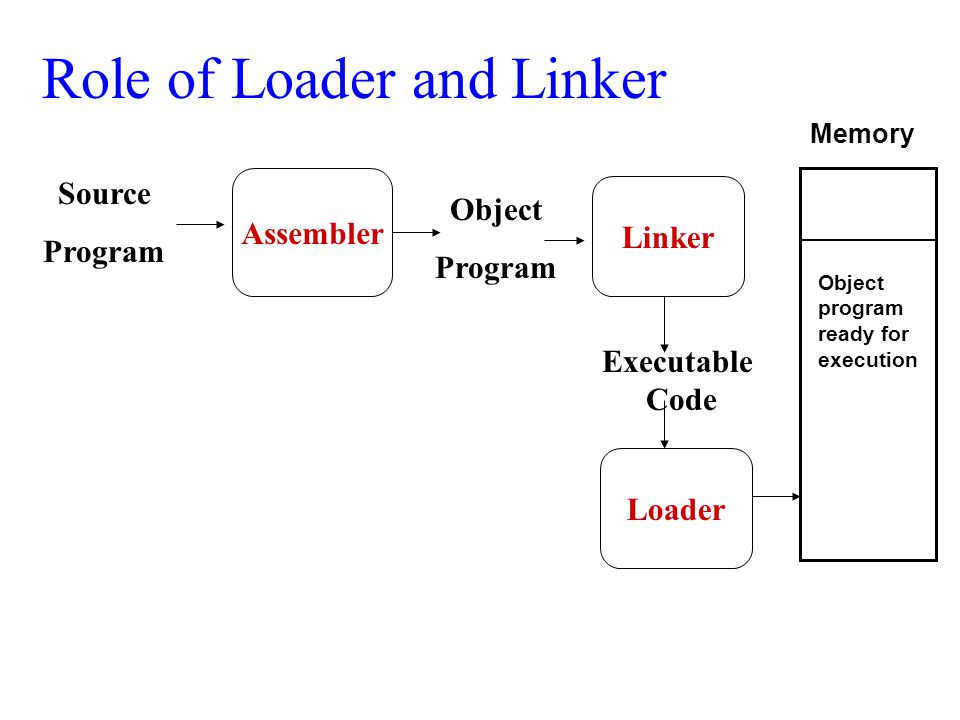
Definition
A linker is a computer utility program that takes one or more object files generated by a compiler and combine them into a single executable file. A loader is a part of an operating system that is responsible for loading programs to memory. A compiler is software that transforms computer code written in one programming language (source code) into another programming language (target code). Thus, this explains the main difference between linker loader and compiler.
Functionality
Furthermore, a linker combines multiple object code and links them with libraries. Meanwhile, a loader prepares the executable file for running while a compiler transforms the source code into object code. Therefore, this is the difference between linker loader and compiler in terms of functionality.
Conclusion
In brief, the difference between linker loader and compiler is that a linker combines one or more object files generated by the compiler to a single executable file and a loader places the programs into memory and prepares them for execution while a compiler converts the source code into object code.
A flowchart is simply a graphical representation of steps. It shows steps in sequential order and is widely used in presenting the flow of algorithms, workflow or processes. Typically, a flowchart shows the steps as boxes of various kinds, and their order by connecting them with arrows.
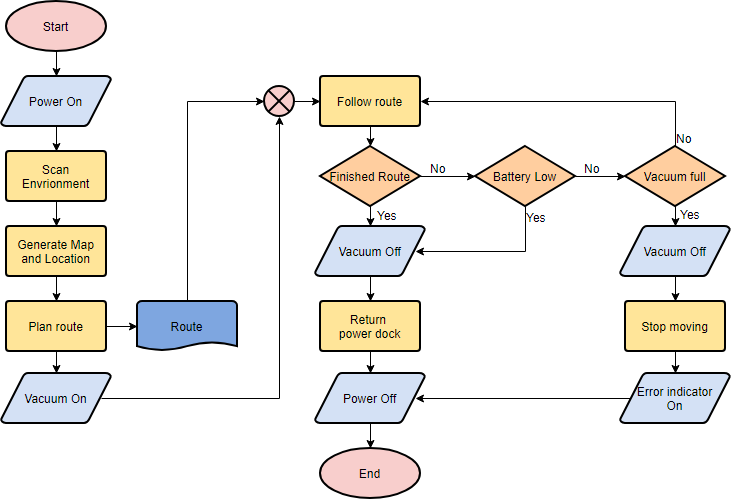
What is a Flowchart?
A flowchart is a graphical representations of steps. It was originated from computer science as a tool for representing algorithms and programming logic but had extended to use in all other kinds of processes. Nowadays, flowcharts play an extremely important role in displaying information and assisting reasoning. They help us visualize complex processes, or make explicit the structure of problems and tasks. A flowchart can also be used to define a process or project to be implemented.
Flowchart Symbols
Different flowchart shapes have different conventional meanings. The meanings of some of the more common shapes are as follows:
Terminator
The terminator symbol represents the starting or ending point of the system.
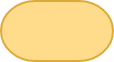
Process
A box indicates some particular operation.
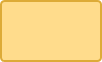
Document
This represents a printout, such as a document or a report.
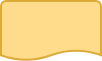
Decision
A diamond represents a decision or branching point. Lines coming out from the diamond indicates different possible situations, leading to different sub-processes.

Data
It represents information entering or leaving the system. An input might be an order from a customer. Output can be a product to be delivered.
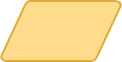
On-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on the same page.

Off-Page Reference
This symbol would contain a letter inside. It indicates that the flow continues on a matching symbol containing the same letter somewhere else on a different page.

Delay or Bottleneck
Identifies a delay or a bottleneck.
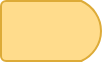
Flow
Lines represent the flow of the sequence and direction of a process.

When to Draw Flowchart?
Using a flowchart has a variety of benefits:
- It helps to clarify complex processes.
- It identifies steps that do not add value to the internal or external customer, including delays; needless storage and transportation; unnecessary work, duplication, and added expense; breakdowns in communication.
- It helps team members gain a shared understanding of the process and use this knowledge to collect data, identify problems, focus discussions, and identify resources.
- It serves as a basis for designing new processes.
Flowchart examples
Here are several flowchart examples. See how you can apply a flowchart practically.
Flowchart Example – Medical Service
This is a hospital flowchart example that shows how clinical cases shall be processed. This flowchart uses decision shapes intensively in representing alternative flows.
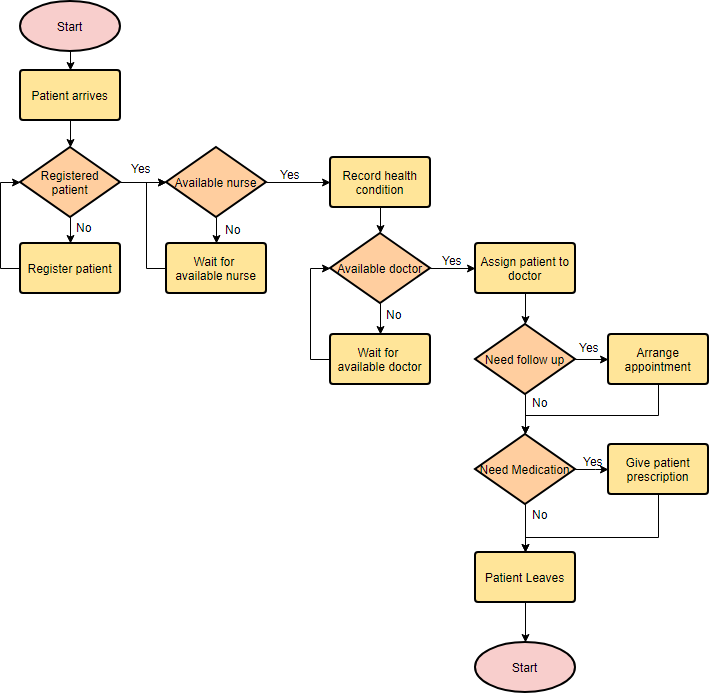
Flowchart Example – Simple Algorithms
A flowchart can also be used in visualizing algorithms, regardless of its complexity. Here is an example that shows how flowchart can be used in showing a simple summation process.
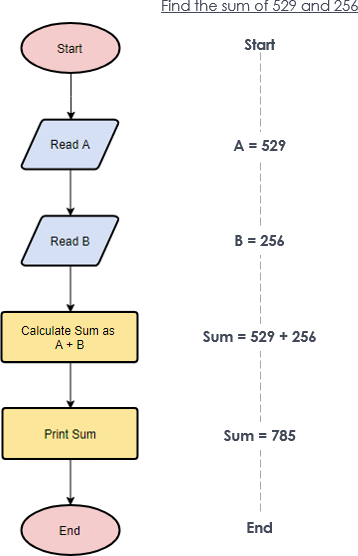
Flowchart Example – Calculate Profit and Loss
The flowchart example below shows how profit and loss can be calculated.
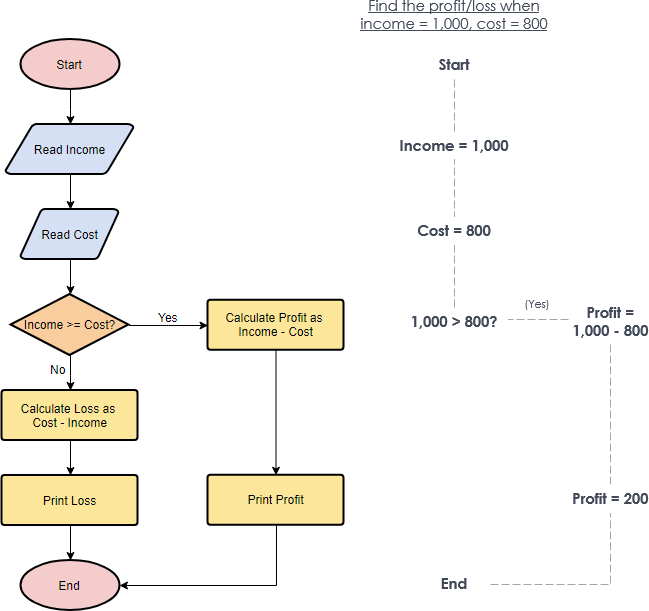
Creating a Flowchart in Visual Paradigm
Let’s see how to draw a flowchart in Visual Paradigm. We will use a very simple flowchart example here. You may expand the example when finished this tutorial.
- Select Diagram > New from the main menu.
- In the New Diagram window, select Flowchart and click Next.
3. You can start from an empty diagram or start from a flowchart template or flowchart example provided. Let’s start from a blank diagram. Select Blank and click Next.
4. Enter the name of the flowchart and click OK.
5. Let’s start by creating a Start symbol. Drag the Start shape from the diagram toolbar and drop it onto the diagram. Name it Start.
6. Create the next shape. Move your mouse pointer over the start shape. Press on the triangular handler on the right and drag it out.
7. Release the mouse button. Select Flow Line > Process from the Resource Catalog.
8. Enter Add items to Cart as the name of the process.
9. Follow the same steps to create two more processes Checkout Shopping Cart and Settle Payment.
10. End the flow by creating a terminator.
Your diagram should look like this:
11. Color the shapes. Select Diagram > Format Panel from the main menu. Select a shape on the diagram and click update its color through the Style setting in the Format Panel.
This is the final flowchart:
Text Books:
1. Fundamental of Information Technology by A.Leon&M.Leon.
2. Let Us C by YashwantKanetkar.
3. Computer Fundamentals and Programming in C by A. K. Sharma, Universities Press.
Reference Books:
1. Programming in C by Schaum Series.
2. Computer Networks (4th Edition) by Andrew S. Tanenbaum
3. Digital Principles and Application by Donald Peach, Albert Paul Malvino
4. Operating System Concepts, (6th Edition) by Abraham Silberschatz, Peter Baer Galvin, Greg Gagne.