UNIT 3
Arrays
An array is defined as the collection of similar type of data items stored at contiguous memory locations. Arrays are the derived data type in C programming language which can store the primitive type of data such as int, char, double, float, etc. It also has the capability to store the collection of derived data types, such as pointers, structure, etc. The array is the simplest data structure where each data element can be randomly accessed by using its index number.
C array is beneficial if you have to store similar elements. For example, if we want to store the marks of a student in 6 subjects, then we don't need to define different variables for the marks in the different subject. Instead of that, we can define an array which can store the marks in each subject at the contiguous memory locations.
By using the array, we can access the elements easily. Only a few lines of code are required to access the elements of the array.
Properties of Array
The array contains the following properties.
- Each element of an array is of same data type and carries the same size, i.e., int = 4 bytes.
- Elements of the array are stored at contiguous memory locations where the first element is stored at the smallest memory location.
- Elements of the array can be randomly accessed since we can calculate the address of each element of the array with the given base address and the size of the data element.
Advantage of C Array
1) Code Optimization: Less code to the access the data.
2) Ease of traversing: By using the for loop, we can retrieve the elements of an array easily.
3) Ease of sorting: To sort the elements of the array, we need a few lines of code only.
4) Random Access: We can access any element randomly using the array.
Disadvantage of C Array
1) Fixed Size: Whatever size, we define at the time of declaration of the array, we can't exceed the limit. So, it doesn't grow the size dynamically like LinkedList which we will learn later.
Declaration of C Array
We can declare an array in the c language in the following way.
- Data_type array_name[array_size];
Now, let us see the example to declare the array.
- Int marks[5];
Here, int is the data_type, marks are the array_name, and 5 is the array_size.
Initialization of C Array
The simplest way to initialize an array is by using the index of each element. We can initialize each element of the array by using the index. Consider the following example.
- Marks[0]=80;//initialization of array
- Marks[1]=60;
- Marks[2]=70;
- Marks[3]=85;
- Marks[4]=75;
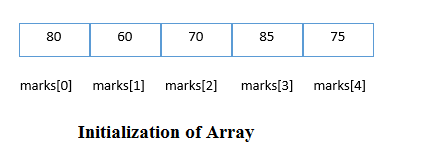
C array example
- #include<stdio.h>
- Int main(){
- Int i=0;
- Int marks[5];//declaration of array
- Marks[0]=80;//initialization of array
- Marks[1]=60;
- Marks[2]=70;
- Marks[3]=85;
- Marks[4]=75;
- //traversal of array
- For(i=0;i<5;i++){
- Printf("%d \n",marks[i]);
- }//end of for loop
- Return 0;
- }
Output
80
60
70
85
75
C Array: Declaration with Initialization
We can initialize the c array at the time of declaration. Let's see the code.
- Int marks[5]={20,30,40,50,60};
In such case, there is no requirement to define the size. So it may also be written as the following code.
- Int marks[]={20,30,40,50,60};
Let's see the C program to declare and initialize the array in C.
- #include<stdio.h>
- Int main(){
- Int i=0;
- Int marks[5]={20,30,40,50,60};//declaration and initialization of array
- //traversal of array
- For(i=0;i<5;i++){
- Printf("%d \n",marks[i]);
- }
- Return 0;
- }
Output
20
30
40
50
60
C Array Example: Sorting an array
In the following program, we are using bubble sort method to sort the array in ascending order.
- #include<stdio.h>
- Void main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Program to print the largest and second largest element of the array.
- #include<stdio.h>
- Void main ()
- {
- Int arr[100],i,n,largest,sec_largest;
- Printf("Enter the size of the array?");
- Scanf("%d",&n);
- Printf("Enter the elements of the array?");
- For(i = 0; i<n; i++)
- {
- Scanf("%d",&arr[i]);
- }
- Largest = arr[0];
- Sec_largest = arr[1];
- For(i=0;i<n;i++)
- {
- If(arr[i]>largest)
- {
- Sec_largest = largest;
- Largest = arr[i];
- }
- Else if (arr[i]>sec_largest && arr[i]!=largest)
- {
- Sec_largest=arr[i];
- }
- }
- Printf("largest = %d, second largest = %d",largest,sec_largest);
- }
One dimensional Array
A one-dimensional array is a group of elements having the same datatype and same name. Individual elements are referred to using common name and unique index of the elements.
The simplest form of an array is one-dimensional-array. The array itself is given name and its elements are referred to by their subscripts. In , an array is denoted as follows:
Array_name[array_size]
Where size specifies the number of elements in the array and the subscript (also called index) value ranges from 0 through size-1.
Declare One Dimensional Array
Here is the general form to declare one dimensional array in
Data_typearray_name[array_size];
Here, data_type is any valid data type, array_name is the name of the array, and array_size is the size of array. Here is an example, declaring an array named arr of int type, having maximum element size of 10 elements
Intarr[10];
Initialize One Dimensional Array
Here is the general form to initialize values to one dimensional array
Data_typearray_name[array_size] = {comma_separated_element_list};
Here is an example, declaring and initializing values to the array name arr of type int, containing 10 elements
Intarr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
One Dimensional Array Example
Here are some example program, demonstrating one dimensional array
/*One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[5] = {1, 2, 3, 4, 5};
Inti;
For(i=0; i<5; i++)
{
Cout<<"arr["<<i<<"] = "<<arr[i]<<"\n";
}
Getch();
}
Here is the sample output of this program:
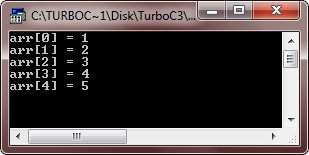
Here is another example, also demonstrating one dimension array in
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[10];
Inti;
Int sum=0, avg=0;
Cout<<"Enter 10 array elements: ";
For(i=0; i<10; i++)
{
Cin>>arr[i];
Sum = sum + arr[i];
}
Cout<<"\nThe array elements are: \n";
For(i=0; i<10; i++)
{
Cout<<arr[i]<<" ";
}
Cout<<"\n\nSum of all elements is: "<<sum;
Avg = sum/10;
Cout<<"\nAnd average is: "<<avg;
Getch();
}
Here is the sample run of the above program:
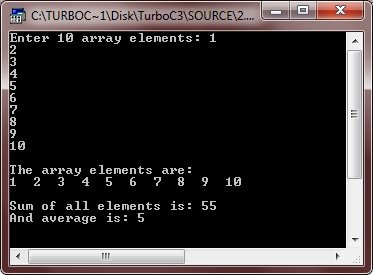
Below is another program on one-dimensional array in
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[5];
Inti, position, index;
Cout<<"Enter 5 array elements: ";
For(i=0; i<5; i++)
{
Cin>>arr[i];
}
Cout<<"\nIndex\t\tPosition";
For(i=0; i<5; i++)
{
Cout<<"\n";
Cout<<i<<" = "<<arr[i]<<"\t\t"<<i+1<<" = "<<arr[i+1];
}
Cout<<" (Garbage value/not of array)";
Cout<<"\n\nImportant - Array element can only be accessed by indexing the array\n";
Cout<<"Note - Array index always starts from 0";
Getch();
}
Below is the sample run of this program:
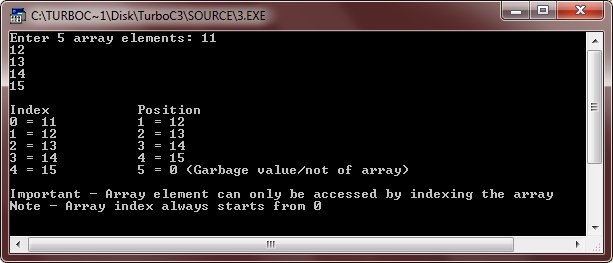
Let's take one more program, on single or one dimensional array
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[10];
Inti, position, index;
Cout<<"Enter 10 array elements: ";
For(i=0; i<10; i++)
{
Cin>>arr[i];
}
Cout<<"Accessing element at position...Enter position...";
Cin>>position;
Cout<<"\nElement present at position "<<position<<" is "<<arr[position+1];
Cout<<"\n\nAccessing element at index..Enter index..";
Cin>>index;
Cout<<"\nElement present at index "<<index<<" is "<<arr[index];
Getch();
}
Here is the sample run of the above program:
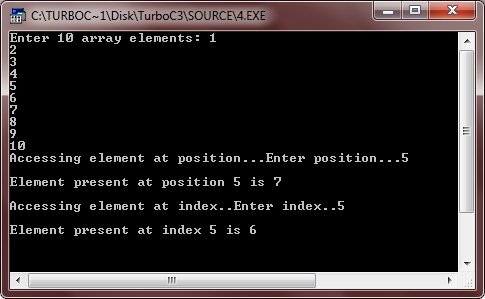
Two Dimensional Array
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns. However, 2D arrays are created to implement a relational database lookalike data structure. It provides ease of holding the bulk of data at once which can be passed to any number of functions wherever required.
Declaration of two dimensional Array in C
The syntax to declare the 2D array is given below.
- Data_type array_name[rows][columns];
Consider the following example.
- Int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Initialization of 2D Array in C
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array. The two-dimensional array can be declared and defined in the following way.
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Two-dimensional array example in C
- #include<stdio.h>
- Int main(){
- Int i=0,j=0;
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
- //traversing 2D array
- For(i=0;i<4;i++){
- For(j=0;j<3;j++){
- Printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
- }//end of j
- }//end of i
- Return 0;
- }
Output
Arr[0][0] = 1
Arr[0][1] = 2
Arr[0][2] = 3
Arr[1][0] = 2
Arr[1][1] = 3
Arr[1][2] = 4
Arr[2][0] = 3
Arr[2][1] = 4
Arr[2][2] = 5
Arr[3][0] = 4
Arr[3][1] = 5
Arr[3][2] = 6
C 2D array example: Storing elements in a matrix and printing it.
- #include <stdio.h>
- Void main ()
- {
- Int arr[3][3],i,j;
- For (i=0;i<3;i++)
- {
- For (j=0;j<3;j++)
- {
- Printf("Enter a[%d][%d]: ",i,j);
- Scanf("%d",&arr[i][j]);
- }
- }
- Printf("\n printing the elements ....\n");
- For(i=0;i<3;i++)
- {
- Printf("\n");
- For (j=0;j<3;j++)
- {
- Printf("%d\t",arr[i][j]);
- }
- }
- }
Output
Enter a[0][0]: 56
Enter a[0][1]: 10
Enter a[0][2]: 30
Enter a[1][0]: 34
Enter a[1][1]: 21
Enter a[1][2]: 34
Enter a[2][0]: 45
Enter a[2][1]: 56
Enter a[2][2]: 78
Printing the elements ....
56 10 30
34 21 34
45 56 78
The string can be defined as the one-dimensional array of characters terminated by a null ('\0'). The character array or the string is used to manipulate text such as word or sentences. Each character in the array occupies one byte of memory, and the last character must always be 0. The termination character ('\0') is important in a string since it is the only way to identify where the string ends. When we define a string as char s[10], the character s[10] is implicitly initialized with the null in the memory.
There are two ways to declare a string in c language.
- By char array
- By string literal
Let's see the example of declaring string by char array in C language.
- Char ch[10]={'j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't', '\0'};
As we know, array index starts from 0, so it will be represented as in the figure given below.
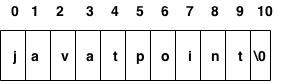
While declaring string, size is not mandatory. So we can write the above code as given below:
- Char ch[]={'j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't', '\0'};
We can also define the string by the string literal in C language. For example:
- Char ch[]="javatpoint";
In such case, '\0' will be appended at the end of the string by the compiler.
Difference between char array and string literal
There are two main differences between char array and literal.
- We need to add the null character '\0' at the end of the array by ourselfwhereas, it is appended internally by the compiler in the case of the character array.
- The string literal cannot be reassigned to another set of characters whereas, we can reassign the characters of the array.
String Example in C
Let's see a simple example where a string is declared and being printed. The '%s' is used as a format specifier for the string in c language.
- #include<stdio.h>
- #include <string.h>
- Int main(){
- Char ch[11]={'j', 'a', 'v', 'a', 't', 'p', 'o', 'i', 'n', 't', '\0'};
- Char ch2[11]="javatpoint";
- Printf("Char Array Value is: %s\n", ch);
- Printf("String Literal Value is: %s\n", ch2);
- Return 0;
- }
Output
Char Array Value is: javatpoint
String Literal Value is: javatpoint
Traversing String
Traversing the string is one of the most important aspects in any of the programming languages. We may need to manipulate a very large text which can be done by traversing the text. Traversing string is somewhat different from the traversing an integer array. We need to know the length of the array to traverse an integer array, whereas we may use the null character in the case of string to identify the end the string and terminate the loop.
Hence, there are two ways to traverse a string.
- By using the length of string
- By using the null character.
Let's discuss each one of them.
Using the length of string
Let's see an example of counting the number of vowels in a string.
- #include<stdio.h>
- Void main ()
- {
- Char s[11] = "javatpoint";
- Int i = 0;
- Int count = 0;
- While(i<11)
- {
- If(s[i]=='a' || s[i] == 'e' || s[i] == 'i' || s[i] == 'u' || s[i] == 'o')
- {
- Count ++;
- }
- i++;
- }
- Printf("The number of vowels %d",count);
- }
Output
The number of vowels 4
Using the null character
Let's see the same example of counting the number of vowels by using the null character.
- #include<stdio.h>
- Void main ()
- {
- Char s[11] = "javatpoint";
- Int i = 0;
- Int count = 0;
- While(s[i] != NULL)
- {
- If(s[i]=='a' || s[i] == 'e' || s[i] == 'i' || s[i] == 'u' || s[i] == 'o')
- {
- Count ++;
- }
- i++;
- }
- Printf("The number of vowels %d",count);
- }
Output
The number of vowels 4
Accepting string as the input
Till now, we have used scanf to accept the input from the user. However, it can also be used in the case of strings but with a different scenario. Consider the below code which stores the string while space is encountered.
- #include<stdio.h>
- Void main ()
- {
- Char s[20];
- Printf("Enter the string?");
- Scanf("%s",s);
- Printf("You entered %s",s);
- }
Output
Enter the string?javatpoint is the best
You entered javatpoint
It is clear from the output that, the above code will not work for space separated strings. To make this code working for the space separated strings, the minor changed required in the scanf function, i.e., instead of writing scanf("%s",s), we must write: scanf("%[^\n]s",s) which instructs the compiler to store the string s while the new line (\n) is encountered. Let's consider the following example to store the space-separated strings.
- #include<stdio.h>
- Void main ()
- {
- Char s[20];
- Printf("Enter the string?");
- Scanf("%[^\n]s",s);
- Printf("You entered %s",s);
- }
Output
Enter the string?javatpoint is the best
You entered javatpoint is the best
Here we must also notice that we do not need to use address of (&) operator in scanf to store a string since string s is an array of characters and the name of the array, i.e., s indicates the base address of the string (character array) therefore we need not use & with it.
Some important points
However, there are the following points which must be noticed while entering the strings by using scanf.
- The compiler doesn't perform bounds checking on the character array. Hence, there can be a case where the length of the string can exceed the dimension of the character array which may always overwrite some important data.
- Instead of using scanf, we may use gets() which is an inbuilt function defined in a header file string.h. The gets() is capable of receiving only one string at a time.
Pointers with strings
We have used pointers with the array, functions, and primitive data types so far. However, pointers can be used to point to the strings. There are various advantages of using pointers to point strings. Let us consider the following example to access the string via the pointer.
- #include<stdio.h>
- Void main ()
- {
- Char s[11] = "javatpoint";
- Char *p = s; // pointer p is pointing to string s.
- Printf("%s",p); // the string javatpoint is printed if we print p.
- }
Output
Javatpoint
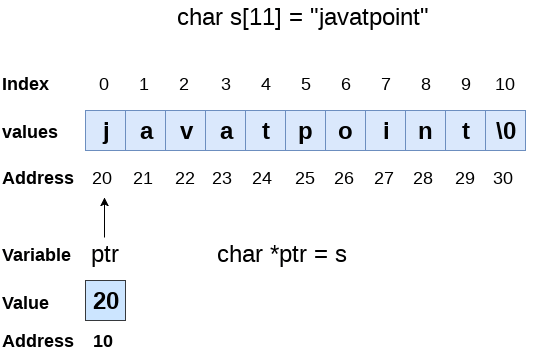
As we know that string is an array of characters, the pointers can be used in the same way they were used with arrays. In the above example, p is declared as a pointer to the array of characters s. P affects similar to s since s is the base address of the string and treated as a pointer internally. However, we can not change the content of s or copy the content of s into another string directly. For this purpose, we need to use the pointers to store the strings. In the following example, we have shown the use of pointers to copy the content of a string into another.
- #include<stdio.h>
- Void main ()
- {
- Char *p = "hello javatpoint";
- Printf("String p: %s\n",p);
- Char *q;
- Printf("copying the content of p into q...\n");
- q = p;
- Printf("String q: %s\n",q);
- }
Output
String p: hello javatpoint
Copying the content of p into q...
String q: hello javatpoint
Once a string is defined, it cannot be reassigned to another set of characters. However, using pointers, we can assign the set of characters to the string. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Char *p = "hello javatpoint";
- Printf("Before assigning: %s\n",p);
- p = "hello";
- Printf("After assigning: %s\n",p);
- }
Output
Before assigning: hello javatpoint
After assigning: hello
Handling strings as array of characters
Strings are actually one-dimensional array of characters terminated by a null character '\0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word "Hello". To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word "Hello."
Char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
If you follow the rule of array initialization then you can write the above statement as follows −
Char greeting[] = "Hello";
Following is the memory presentation of the above defined string in C/C++ −
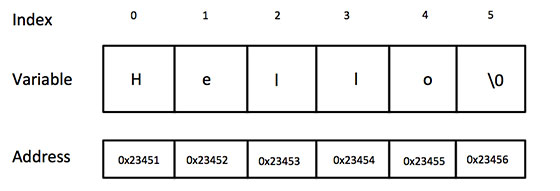
Actually, you do not place the null character at the end of a string constant. The C compiler automatically places the '\0' at the end of the string when it initializes the array. Let us try to print the above mentioned string −
#include <stdio.h>
Int main () {
Char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
Printf("Greeting message: %s\n", greeting );
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Greeting message: Hello
C supports a wide range of functions that manipulate null-terminated strings −
Sr.No. | Function & Purpose |
1 | Strcpy(s1, s2); Copies string s2 into string s1. |
2 | Strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | Strlen(s1); Returns the length of string s1. |
4 | Strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | Strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | Strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
The following example uses some of the above-mentioned functions −
#include <stdio.h>
#include <string.h>
Int main () {
Char str1[12] = "Hello";
Char str2[12] = "World";
Char str3[12];
Intlen ;
/* copy str1 into str3 */
Strcpy(str3, str1);
Printf("strcpy( str3, str1) : %s\n", str3 );
/* concatenates str1 and str2 */
Strcat( str1, str2);
Printf("strcat( str1, str2): %s\n", str1 );
/* total lenghth of str1 after concatenation */
Len = strlen(str1);
Printf("strlen(str1) : %d\n", len );
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Strcpy( str3, str1) : Hello
Strcat( str1, str2): HelloWorld
Strlen(str1) : 10
Suggested Text Books
Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill
E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
Suggested Reference Books
Brian W. Kernighan and Dennis M. Ritchie, The C Programming Language, Prentice Hall of India