Unit - 1
Introduction to Java and Java Programming Environment
Java is a platform as well as a programming language. Java is a high-level programming language that is also robust, object-oriented, and secure.
Java was created in 1995 by Sun Microsystems (which is now a division of Oracle). The founder of Java, James Gosling, is recognized as the "Father of Java." It was known as Oak before Java. Because Oak was already a recognized business, James Gosling and his team decided to alter the name to Java.
Platform - A platform is any hardware or software environment in which a program runs. Java is referred to as a platform because it has a runtime environment (JRE) and API.
Example
Class Simple
{
Public static void main(String args[])
{
System.out.println("Hello Java");
}
}
Application
Java is installed on 3 billion devices, according to Sun. Java is being utilized on a wide range of devices. The following are a few of them:
● Desktop Applications such as acrobat reader, media player, antivirus, etc.
● Enterprise Applications such as banking applications.
● Mobile
● Embedded System
● Smart Card
● Robotics
● Games, etc.
Java Programming Environment
Java is a sophisticated general-purpose programming language that was first released in 1996 and has been around for almost 23 years. The Java programming environment is made up of the following components:
● Java language - programmers use to create the application
● Java virtual machine - The application's execution is controlled by this variable.
● Java ecosystem - delivers added value to programmers who use the programming language.
Java Language
● It's a human-readable language that's quite simple to understand and write (albeit a bit verbose at times).
● It's a class-based, object-oriented system.
● Java is meant to be simple to understand and teach.
● There are numerous distinct Java implementations, both proprietary and open source, available.
● The Java Language Specification (JLS) specifies how a Java application shall behave when it confirms.
Java virtual memory
The Java Virtual Machine is a program that creates a runtime environment for Java programs to run in. If a supporting JVM is not available, Java programs will not run.
When using the command line to launch a Java program, such as —
Java <arguments> <program name>
The operating system would start the JVM as a process, then run the program in the newly started (and empty) Virtual Machine.
Java source files are not accepted as input by JVM. The javac application converts the java source code to bytecode first. Javac accepts source files as input and produces bytecode in the form of class files ending in.class.
The JVM interpreter then steps through these class files one by one, interpreting them and executing the program.
The Just-in-Time (JIT) compiler is a key feature of the JVM. The runtime behavior of programs shows certain fascinating patterns, according to research conducted in the 1970s and 1980s. Some sections of code are executed significantly more frequently than others.
The HotSpot JVM (originally released by Sun in Java 1.3) finds the "hot methods" (often called) and the JIT compiler turns them directly into machine code, avoiding the compilation of source code into bytecode.
Java ecosystem
Java has gained widespread acceptance as a reliable, portable, and high-performing programming language. The enormous amount of third-party libraries and components created in Java is one of the key reasons for Java's success. It's rare nowadays to come across a component that doesn't have a Java connector. There is a Java connector for everything from standard MySQL to NoSQL, monitoring frameworks, and network components.
Key takeaway
Java is a platform as well as a programming language.
Java is a high-level programming language that is also robust, object-oriented, and secure.
Java is a sophisticated general-purpose programming language that was first released in 1996 and has been around for almost 23 years.
Inheritance, data binding, polymorphism, and other notions are all part of the Object-Oriented Programming paradigm.
The object-oriented programming language Simula is regarded as the first. A genuinely object-oriented programming language is a programming paradigm in which everything is represented as an object.
Smalltalk is widely regarded as the first object-oriented programming language.
The popular object-oriented languages are Java, C#, PHP, Python, C++, etc.
Object-oriented programming's fundamental goal is to implement real-world things such as objects, classes, abstraction, inheritance, polymorphism, and so on.
A real-world entity such as a pen, chair, table, computer, watch, and so on is referred to as an object. Object-Oriented Programming (OOP) is a programming methodology or paradigm that uses classes and objects to create a program. It makes software development and maintenance easier by introducing the following concepts:
● Object
● Class
● Inheritance
● Polymorphism
● Abstraction
● Encapsulation
Key takeaway
Object-oriented programming's fundamental goal is to implement real-world things such as objects, classes, abstraction, inheritance, polymorphism, and so on.
The different sizes and values that can be stored in the variable are defined by data types. In Java, there are two types of data types:
● Primitive data types - Boolean, char, byte, short, int, long, float, and double are examples of primitive data types.
● Non - primitives data types - Classes, Interfaces, and Arrays are examples of non-primitive data types.
Primitive data types
Primitive data types are the building blocks of data manipulation in the Java programming language. These are the most basic data types in the Java programming language.
Let's take a closer look at each of the eight primitive data types.
Boolean
Only two potential values are stored in the Boolean data type: true and false. Simple flags that track true/false circumstances are stored in this data type.
The Boolean data type specifies a single bit of data, but its "size" cannot be precisely defined.
Byte
The primitive data type byte is an example of this. It's an 8-bit two-s complement signed integer. It has a value range of -128 to 127. (inclusive). It has a minimum of -128 and a high of 127. It has a value of 0 by default.
The byte data type is used to preserve memory in huge arrays where space is at a premium. Because a byte is four times smaller than an integer, it saves space. It can also be used in place of "int" data type.
Short
A 16-bit signed two's complement integer is the short data type. It has a value range of -32,768 to 32,767. (inclusive). It has a minimum of -32,768 and a maximum of 32,767. It has a value of 0 by default.
The short data type, like the byte data type, can be used to save memory. A short data type is twice the size of an integer.
Int
A 32-bit signed two's complement integer is represented by the int data type. Its range of values is - 2,147,483,648 (-231 -1) to 2,147,483,647 (231 -1). (inclusive). It has a minimum of 2,147,483,648 and a maximum of 2,147,483,647. It has a value of 0 by default.
Unless there is a memory constraint, the int data type is usually chosen as the default data type for integral values.
Long
A 64-bit two's complement integer is the long data type. It has a value range of -9,223,372,036,854,775,808(-263 -1) to 9,223,372,036,854,775,807(263 -1). (inclusive). Its lowest and maximum values are 9,223,372,036,854,775,808 and 9,223,372,036,854,775,807. It has a value of 0 by default. When you need a larger range of values than int can supply, you should utilize the long data type.
Float
The float data type is a 32-bit IEEE 754 floating point with single precision. It has an infinite value range. If you need to preserve memory in big arrays of floating point integers, use a float (rather than a double). For precise numbers, such as currency, the float data type should never be used. 0.0F is the default value.
Double
A double data type is a 64-bit IEEE 754 floating point with double precision. It has an infinite value range. Like float, the double data type is commonly used for decimal values. For precise values, such as currency, the double data type should never be utilized. 0.0d is the default value.
Variables
A variable is a container that holds the value during the execution of a Java program. A data type is assigned to a variable.
The term "variable" refers to the name of a memory region. Local, instance, and static variables are the three types of variables in Java.
A variable is the name of a memory-allocated reserved space. In other words, it is a name of the memory location. It's made out of the words "vary + ability," which signifies that its value can be modified.
Types of variables
There are three types of variables in Java:
Local variable
A local variable is a variable declared within the method's body. This variable can only be used within that method, and the other methods in the class are unaware that it exists.
The keyword "static" cannot be used to declare a local variable.
Instance variable
An instance variable is a variable declared inside the class but outside the method body. It hasn't been declared static.
Because its value is instance-specific and not shared across instances, it's called an instance variable.
Static variable
A static variable is one that has been declared as static. It's not possible for it to be local. You can make a single duplicate of the static variable and share it across all of the class's instances. Static variables are only allocated memory once, when the class is loaded into memory.
Example
Public class A
{
Static int m=100;//static variable
Void method()
{
Int n=90;//local variable
}
Public static void main(String args[])
{
Int data=50;//instance variable
}
}//end of class
Key takeaway
The different sizes and values that can be stored in the variable are defined by data types.
A variable is a container that holds the value during the execution of a Java program. A data type is assigned to a variable.
In Java, type casting is a method or procedure for manually and automatically converting one data type into another. The compiler performs the automatic conversion, whereas the programmer performs the manual conversion.
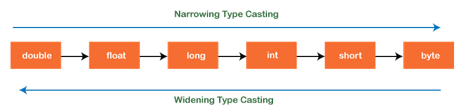
Fig 1: Type casting
The act of allocating a value from one primitive data type to another is known as type casting. When you assign the value of one data type to another, you should consider the data type's compatibility. If they are compatible, Java will convert them automatically (Automatic Type Conversion), but if they aren't, they must be cast or converted directly.
In Java, there are two different methods of casting:
- Widening Casting
When two data types are automatically converted, this type of casting occurs. Implicit Conversion is another name for it. This occurs when the two data types are compatible, as well as when a smaller data type's value is assigned to a larger data type.
This entails converting a smaller data type to a larger data type.
Byte -> short -> char -> int -> long -> float -> double
Example: The numeric data types are interchangeable, however there is no automatic translation from numeric to char or boolean. Furthermore, char and boolean are not interchangeable. To better understand how Implicit type casting works, let's construct a reasoning for it.
Public class Conversion{
Public static void main(String[] args)
{
Int i = 200;
//automatic type conversion
Long l = i;
//automatic type conversion
Float f = l;
System.out.println("Int value "+i);
System.out.println("Long value "+l);
System.out.println("Float value "+f);
}
}
Output
Int value 200
Long value 200
Float value 200.0
2. Narrowing Casting
Narrowing type casting is the process of converting a higher data type to a lower one. It's also known as casting up or explicit conversion. The programmer performs this task manually. The compiler will raise a compile-time error if we do not perform casting.
Converting a larger data type to a smaller data type is what this entails.
Double -> float -> long -> int -> char -> short -> byte
Example
//Java program to illustrate explicit type conversion
Public class Narrowing
{
Public static void main(String[] args)
{
Double d = 200.06;
//explicit type casting
Long l = (long)d;
//explicit type casting
Int i = (int)l;
System.out.println("Double Data type value "+d);
//fractional part lost
System.out.println("Long Data type value "+l);
//fractional part lost
System.out.println("Int Data type value "+i);
}
}
Output
Double Data type value 200.06
Long Data type value 200
Int Data type value 200
Key takeaway
- In Java, type casting is a method or procedure for manually and automatically converting one data type into another.
- The compiler performs the automatic conversion, whereas the programmer performs the manual conversion.
- When you assign the value of one data type to another, you should consider the data type's compatibility.
Java has a large number of operators for manipulating variables. All Java operators can be divided into the following categories:
● Arithmetic Operators
● Relational Operators
● Bitwise Operators
● Logical Operators
● Assignment Operators
● Misc Operators
Arithmetic operators
In the same way as algebraic operators are used in mathematical expressions, arithmetic operators are employed in mathematical expressions. The arithmetic operators are listed in the table below.
Assume integer variable A has a value of 10 and variable B has a value of 20.
Operator | Description | Example |
+ (Addition) | Values are added on both sides of the operator. | A + B will give 30 |
- (Subtraction) | The right-hand operand is subtracted from the left-hand operand. | A - B will give -10 |
* (Multiplication) | Values on both sides of the operator are multiplied. | A * B will give 200 |
/ (Division) | Right-hand operand is divided by left-hand operand. | B / A will give 2 |
% (Modulus) | Returns the remainder after dividing the left-hand operand by the right-hand operand. | B % A will give 0 |
++ (Increment) | Increases the operand's value by one. | B++ gives 21 |
-- (Decrement) | Reduces the operand's value by one.. | B-- gives 19 |
Relational Operators
The Java language supports the following relational operators.
Assume variable A has a value of 10 and variable B has a value of 20.
Operator | Description | Example |
== (equal to) | Checks whether the values of two operands are equal, and if they are, the condition is true. | (A == B) is not true. |
!= (not equal to) | Checks whether the values of two operands are equivalent; if they aren't, the condition is true. | (A != B) is true. |
> (greater than) | If the left operand's value is greater than the right operand's value, then the condition is true. | (A > B) is not true. |
< (less than) | Checks if the left operand's value is less than the right operand's value; if it is, the condition is true. | (A < B) is true. |
>= (greater than or equal to) | If the left operand's value is larger than or equal to the right operand's value, then the condition is true. | (A >= B) is not true. |
<= (less than or equal to) | If the left operand's value is less than or equal to the right operand's value, then the condition is true. | (A <= B) is true. |
Bitwise operators
Long, int, short, char, and byte are all integer types that can be used with Java's bitwise operators.
The bitwise operator works with bits and performs operations bit by bit. Assume a = 60 and b = 13; in binary format, they will look like this:
a = 0011 1100
b = 0000 1101
a&b = 0000 1100
a|b = 0011 1101
a^b = 0011 0001
~a = 1100 0011
Assume integer variable A has a value of 60 and variable B has a value of 13.
Operator | Description | Example |
& (bitwise and) | If a bit exists in both operands, the binary AND operator duplicates it to the result. | (A & B) will give 12 which is 0000 1100 |
| (bitwise or) | If a bit exists in both operands, the binary OR operator copies it. | (A | B) will give 61 which is 0011 1101 |
^ (bitwise XOR) | If a bit is set in one operand but not both, the binary XOR operator replicates it. | (A ^ B) will give 49 which is 0011 0001 |
~ (bitwise compliment) | The Binary Ones Complement Operator is a unary operator that 'flips' bits. | (~A ) will give -61 which is 1100 0011 in 2's complement form due to a signed binary number. |
<< (left shift) | Left Shift Operator in Binary. The value of the left operand is shifted to the left by the number of bits given by the right operand. | A << 2 will give 240 which is 1111 0000 |
>> (right shift) | Right Shift Operator in Binary. The value of the left operand is advanced by the number of bits supplied by the right operand. | A >> 2 will give 15 which is 1111 |
>>> (zero fill right shift) | Zero fill operator with a right shift. The value of the left operand is shifted right by the number of bits supplied in the right operand, and the shifted values are filled with zeros. | A >>>2 will give 15 which is 0000 1111 |
Logical operators
The logical operators are listed in the table below.
Assume that Boolean variable A is true and variable B is false.
Operator | Description | Example |
&& (logical and) | The logical AND operator is what it's called. If both operands are non-zero, the condition is satisfied. | (A && B) is false |
|| (logical or) | The logical OR operator is what it's called. The condition becomes true if any of the two operands is non-zero. | (A || B) is true |
! (logical not) | It's known as the Logical NOT Operator. Its operand's logical state is reversed when it is used. If one of the conditions is true, the Logical NOT operator returns false. | !(A && B) is true |
Assignment operators
The assignment operators supported by the Java language are listed below.
Operator | Description | Example |
= | This is a straightforward assignment operator. Values from the right side operands are assigned to the left side operand. | C = A + B will assign value of A + B into C |
+= | The AND assignment operator is used to combine two variables. It adds the right and left operands together and assigns the result to the left operand. | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator. It subtracts right operand from the left operand and assign the result to left operand. | C -= A is equivalent to C = C – A |
*= | The AND operator multiplies and assigns. It adds the right and left operands together and assigns the result to the left operand. | C *= A is equivalent to C = C * A |
/= | The AND operator divides and assigns. It multiplies the left and right operands and assigns the result to the left operand. | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator. It takes modulus using two operands and assign the result to left operand. | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator. | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator. | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator. | C &= 2 is same as C = C & 2 |
^= | Bitwise exclusive OR and assignment operator. | C ^= 2 is same as C = C ^ 2 |
|= | Bitwise inclusive OR and assignment operator. | C |= 2 is same as C = C | 2 |
Miscellaneous Operators
Only a few more operators are supported by the Java programming language.
Conditional Operator ( ? : )
The ternary operator is another name for the conditional operator. This operator is used to evaluate Boolean expressions and has three operands. The operator's objective is to determine which value should be assigned to a variable. The operator is denoted by the symbol.
Variable x = (expression) ? value if true : value if false
Instance of Operator
Only object reference variables are used with this operator. The operator determines whether or not the item is of a specific type (class type or interface type). The instance of operator is denoted by the symbol.
(Object reference variable) instance of (class/interface type)
The result will be true if the object referred to by the variable on the left side of the operator passes the IS-A check for the class/interface type on the right side.
Precedence of Operators
The order in which terms in an expression are grouped is determined by operator precedence. This has an impact on how an expression is judged. Certain operators take precedence over others; the multiplication operator, for example, takes precedence over the addition operator.
For example, x = 7 + 3 * 2; because operator * has higher precedence than +, x is allocated 13, not 20, and is multiplied by 3 * 2 before being added to 7.
The highest-priority operators appear at the top of the table, while the lowest-priority operators appear at the bottom. Higher precedence operators will be evaluated first within an expression.
Category | Operator | Associativity |
Postfix | Expression ++ expression -- | Left to right |
Unary | ++expression –-expression +expression –expression ~ ! | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | << >> >>> | Left to right |
Relational | < > <= >= instanceof | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %= ^= |= <<= >>= >>>= | Right to left |
Key takeaway
The order in which terms in an expression are grouped is determined by operator precedence. This has an impact on how an expression is judged.
The highest-priority operators appear at the top of the table, while the lowest-priority operators appear at the bottom.
The if statement in Java is used to test the condition. If the condition is true, the if block is executed.
Syntax
If(condition){
//code to be executed
}
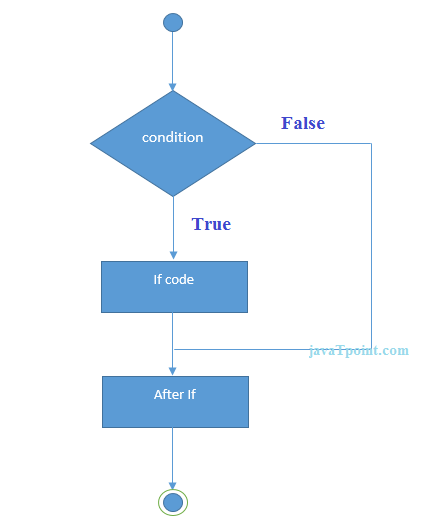
Fig 2: Flow chart of if statements
Example
//Java Program to demonstrate the use of if statement.
Public class IfExample {
Public static void main(String[] args) {
//defining an 'age' variable
Int age=20;
//checking the age
If(age>18){
System.out.print("Age is greater than 18");
}
}
}
Output
Age is greater than 18
Switch
The Java switch statement combines numerous conditions into a single statement. It's similar to an if-else-if conditional statement. The switch statement compares various values to see if they are equal.
● For a switch expression, there can be one or N case values.
● Only switch expressions can be used as case values. The case value must be constant or literal. Variables are not permitted.
● The case values must be one-of-a-kind. It causes a compile-time error if a value is duplicated.
● The byte, short, int, long (with its Wrapper type), enums, and string types must all be present in the Java switch statement.
● A break statement can be included in each case statement, although it is not required. The control jumps after the switch expression when it reaches the break statement. If no break statement is discovered, the next case is executed.
● Optionally, the case value can have a default label.
Syntax
Switch(expression){
Case value1:
//code to be executed;
Break; //optional
Case value2:
//code to be executed;
Break; //optional
......
Default:
Code to be executed if all cases are not matched;
}
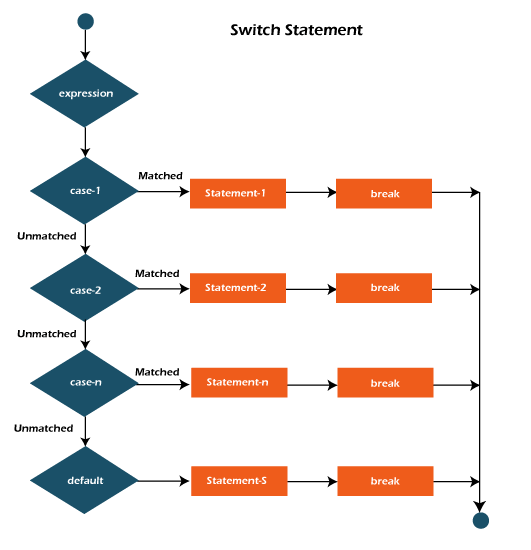
Fig 3: Flow chart
Example
Public class SwitchExample {
Public static void main(String[] args) {
//Declaring a variable for switch expression
Int number=20;
//Switch expression
Switch(number){
//Case statements
Case 10: System.out.println("10");
Break;
Case 20: System.out.println("20");
Break;
Case 30: System.out.println("30");
Break;
//Default case statement
Default:System.out.println("Not in 10, 20 or 30");
}
}
}
Output
20
Iterations
In Java, iteration is a technique used to sequence through a block of code repeatedly until a specific condition either exists or no longer exists. Iterations are a very common approach used with loops.
Common examples include iterating through a list of grades to compute a final grade-point-average, processing a list of email addresses to validate their format, and processing a text file. We can also use iteration as an approach to the name reversal and factorial functions.
Statements
Break statements
When a break statement is reached within a loop, the loop is instantly ended, and program control is resumed at the next statement after the loop.
To break a loop or switch statement in Java, use the break statement. At the stated situation, it interrupts the program's current flow. It only breaks the inner loop in the case of the inner loop.
The Java break statement can be used in all forms of loops, including for loops, while loops, and do-while loops.
Syntax
Break;
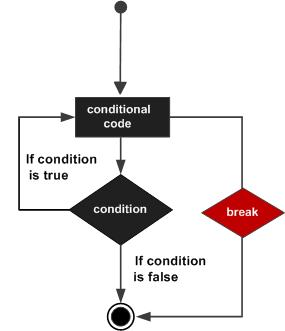
Fig 4: Flow diagram
Example
Public class Test {
Public static void main(String args[]) {
Int [] numbers = {10, 20, 30, 40, 50};
For(int x : numbers ) {
If( x == 30 ) {
Break;
}
System.out.print( x );
System.out.print("\n");
}
}
}
Output
10
20
Continue statements
When you need to jump to the next iteration of the loop right away, you utilize the continue statement in the loop control structure. It can be used in conjunction with a for loop or a while loop.
The loop is continued using the Java continue command. It continues the program's current flow while skipping the remaining code at the specified circumstance. It only continues the inner loop in the case of an inner loop.
The continue statement in Java can be used in all forms of loops, including for loops, while loops, and do-while loops.
Syntax
Continue;
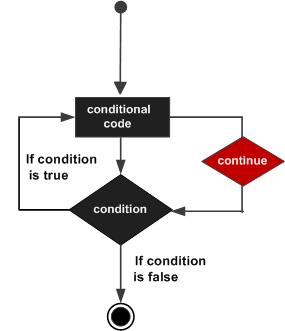
Fig 5: Flow diagram
Example
Public class Test {
Public static void main(String args[]) {
Int [] numbers = {10, 20, 30, 40, 50};
For(int x : numbers ) {
If( x == 30 ) {
Continue;
}
System.out.print( x );
System.out.print("\n");
}
}
}
Output
10
20
40
50
While
The while loop in Java is used to execute a section of code until the provided Boolean condition is true. The loop comes to an end as soon as the Boolean condition is false.
The while loop is a type of if statement that repeats itself. The while loop is recommended if the number of iterations is not fixed.
Syntax
While(Boolean_expression) {
// Statements
}
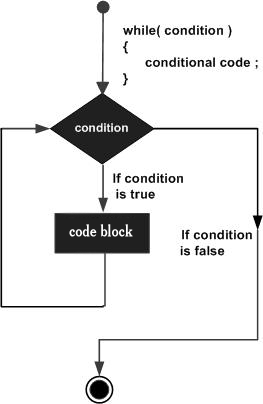
Fig 6: Flow diagram
The while loop's main feature is that it may or may not ever run. The loop body will be skipped and the first statement after the while loop will be executed when the expression is tested and the result is false.
Example
Public class Test {
Public static void main(String args[]) {
Int x = 10;
While( x < 20 ) {
System.out.print("value of x : " + x );
x++;
System.out.print("\n");
}
}
}
Output
Value of x : 10
Value of x : 11
Value of x : 12
Value of x : 13
Value of x : 14
Value of x : 15
Value of x : 16
Value of x : 17
Value of x : 18
Value of x : 19
Do While
The Java do-while loop is used to repeatedly iterate a section of a program until the specified condition is met. A do-while loop is recommended if the number of iterations is not fixed and the loop must be executed at least once.
An exit control loop is a Java do-while loop. In contrast to while and for loops, the do-while loop checks the condition at the end of the loop body. Because the condition is tested after the loop body, the Java do-while loop is executed at least once.
Syntax
Do{
//code to be executed / loop body
//update statement
}while (condition);
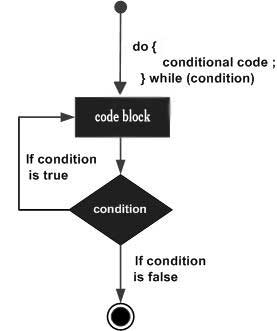
Fig 7: Flow chart
Example
Public class DoWhileExample {
Public static void main(String[] args) {
Int i=1;
Do{
System.out.println(i);
i++;
}while(i<=10);
}
}
Output
1
2
3
4
5
6
7
8
9
10
For
A for loop is a repetition control structure that allows you to design a loop that must be repeated a certain number of times quickly.
When you know how many times a task will be repeated, a for loop comes in handy.
● Initialize - When the loop begins, it executes the starting condition only once. We can either initialize the variable or use one that has already been initialized. It's a condition that can be turned off.
● Condition - It's the second condition that's run every time the loop's condition is tested. It keeps running until the condition is false. It must return either true or false as a boolean value. It's a condition that can be turned off.
● Increment / decrement - It increases or decreases the value of the variable. It's a condition that can be turned off.
● Statements - The loop's statement is performed every time the second condition is false.
Syntax
For(initialization; condition; increment/decrement){
//statement or code to be executed
}
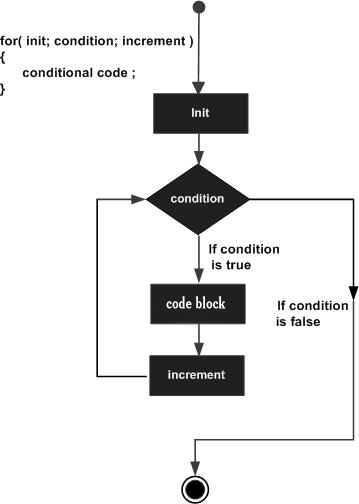
Fig 8: Flow diagram
Example
Public class Test {
Public static void main(String args[]) {
For(int x = 10; x < 20; x = x + 1) {
System.out.print("value of x : " + x );
System.out.print("\n");
}
}
}
Output
Value of x : 10
Value of x : 11
Value of x : 12
Value of x : 13
Value of x : 14
Value of x : 15
Value of x : 16
Value of x : 17
Value of x : 18
Value of x : 19
Nested loops
The term "nested for loop" refers to a for loop that is contained within another loop. When the outer loop executes, the inner loop completes.
Example
Public class NestedForExample {
Public static void main(String[] args) {
//loop of i
For(int i=1;i<=3;i++){
//loop of j
For(int j=1;j<=3;j++){
System.out.println(i+" "+j);
}//end of i
}//end of j
}
}
Output
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
Objects have states and behaviors that they exhibit. A dog, for example, has states such as color, name, and breed, as well as behaviors such as waving the tail, barking, and eating. A class's instance is an object.
Let's take a closer look at what objects are. Many objects, such as vehicles, pets, humans, and other living things, can be found in the actual world. There is a state and a behavior for each of these things.
If we consider a dog, its state includes its name, breed, and color, as well as its activity, which includes barking, wagging the tail, and running.
When you compare a software object to a real-world thing, you'll see that they have a lot in common.
Object and Class Example: main within the class
We've developed a Student class in this example, which has two data members: id and name. The object of the Student class is created using the new keyword, and the object's value is printed.
Inside the class, we're going to create a main() method.
//Java Program to illustrate how to define a class and fields
//Defining a Student class.
Class Student{
//defining fields
Int id;//field or data member or instance variable
String name;
//creating main method inside the Student class
Public static void main(String args[]){
//Creating an object or instance
Student s1=new Student();//creating an object of Student
//Printing values of the object
System.out.println(s1.id);//accessing member through reference variable
System.out.println(s1.name);
}
}
Output
0
Null
Classes
A class is a template/blueprint that outlines the behavior/state that objects of that kind support.
Individual objects are formed from a blueprint called a class.
Here's an example of a class.
Public class Dog {
String breed;
Int age;
String color;
Void barking() {
}
Void hungry() {
}
Void sleeping() {
}
}
Any of the following variable kinds can be found in a class.
Local variables – Local variables are variables specified within methods, constructors, or blocks. The variable will be declared and initialized within the method, and then removed once the method is finished.
Instance variables – Instance variables are variables that exist within a class but are not associated with any methods. When the class is created, these variables are set to their default values. Instance variables can be accessed from within any of the class's methods, constructors, or blocks.
Class variables – Class variables are variables declared with the static keyword within a class, outside of any methods.
A class can have as many methods as it wants to retrieve the value of different types of methods. Barking(), hungry(), and sleeping() are methods in the sample above.
Key takeaway
- An object is a real-world element in an object–oriented environment.
- Objects can be modelled according to the application's needs.
- A class represents a collection of objects having same characteristic properties that exhibit common behavior.
- It provides a blueprint or definition of the objects from which it can be produced.
- As a member of a class, the creation of an entity is called instantiation.
To define a class, we write:
<modifier> class <name>
{
<attributeDeclaration>*
<constructorDeclaration>*
<methodDeclaration>*
}
Where
<modifier> is an access modifier, which may be combined with other types of modifier.
Example
// Java Program to Creating our Own Custom Class
// Importing input output classes
Import java.io.*;
// Class 1
// Helper class
Class Employee {
// Member variables of this class
// first attribute
Int id;
// second attribute
Int salary;
// third attribute
String name;
// Member function of this class
// Method 1
Public void printDetails()
{
// Print and display commands
System.out.println("My id is " + id);
System.out.println("This is my name " + name);
}
// Method 2
Public int getSalary()
{
// Simply returning the salary
Return salary;
}
}
// Class 2
// Main class
Class Custom {
// Main driver method
Public static void main(String[] args)
{
// Display message only
System.out.println("This is the custom class");
// Creating object of custom class in the main()
// method Instantiating a new Employee object
Employee harry = new Employee();
// Again creating object of custom class and
// instantiating a new Employee object
Employee robin = new Employee();
// Initializing values for first object created
// above
Harry.id = 23;
Harry.salary = 100000;
Harry.name = "Ritu bhatiya";
// Initializing values for second object created
// above
Robin.id = 25;
Robin.salary = 150000;
Robin.name = "Amit thripathi";
// Printing object attributes by
// calling the method as defined in our class
Harry.printDetails();
Robin.printDetails();
// Calling the method again of our class and
// storing it in a variable
Int salary = robin.getSalary();
// Print and display the above salary
System.out.println("Salary of robin : " + salary
+ "$");
System.out.println("ID : " + harry.id);
}
}
Output
This is the custom class
My id is 23
This is my name Ritu bhatiya
My id is 25
This is my name Amit tripathi
Salary of robin : 150000$
ID : 23
A constructor is similar to a method in Java, except it does not have a return type. It, like Java methods, can be overloaded.
In Java, constructor overloading refers to the use of multiple constructors with varying parameter lists. They're structured so that each constructor does something distinct. The compiler distinguishes them based on the number of parameters in the list and their kinds.
Example
//Java program to overload constructors
Class Student5{
Int id;
String name;
Int age;
//creating two arg constructor
Student5(int i,String n){
Id = i;
Name = n;
}
//creating three arg constructor
Student5(int i,String n,int a){
Id = i;
Name = n;
Age=a;
}
Void display(){System.out.println(id+" "+name+" "+age);}
Public static void main(String args[]){
Student5 s1 = new Student5(100,"Ka");
Student5 s2 = new Student5(200,"Ki",25);
s1.display();
s2.display();
}
}
Output
100 Ka 0
200 Ki 25
Key takeaway
A constructor is similar to a method in Java, except it does not have a return type. It, like Java methods, can be overloaded.
In Java, constructor overloading refers to the use of multiple constructors with varying parameter lists.
In Java, the static keyword is mostly used for memory management. With variables, methods, blocks, and nested classes, we can use the static keyword. The static keyword refers to a class rather than a specific instance of that class.
The static can take the form of:
● Variable (also known as a class variable)
● Method (also known as a class method)
● Block
● Nested class
Static variable
A static variable is defined as a variable that has been declared as static.
The static variable can be used to refer to a property that is shared by all objects (but not unique to each object), such as an employee's firm name or a student's college name.
The static variable is only stored in memory once in the class area, when the class is loaded.
Example of static variable
//Java Program to demonstrate the use of static variable
Class Student{
Int rollno;//instance variable
String name;
Static String college ="ITS";//static variable
//constructor
Student(int r, String n){
Rollno = r;
Name = n;
}
//method to display the values
Void display (){System.out.println(rollno+" "+name+" "+college);}
}
//Test class to show the values of objects
Public class TestStaticVariable1{
Public static void main(String args[]){
Student s1 = new Student(111,"Karan");
Student s2 = new Student(222,"Aryan");
//we can change the college of all objects by the single line of code
//Student.college="BBDIT";
s1.display();
s2.display();
}
}
Output
111 Karan ITS
222 Aryan ITS
Static method
Any method that uses the static keyword is referred to as a static method.
● A class's static method, rather than the class's object, belongs to the class.
● A static method can be called without having to create a class instance.
● A static method can access and update the value of a static data member.
Example:
//Java Program to demonstrate the use of a static method.
Class Student{
Int rollno;
String name;
Static String college = "ITS";
//static method to change the value of static variable
Static void change(){
College = "BBDIT";
}
//constructor to initialize the variable
Student(int r, String n){
Rollno = r;
Name = n;
}
//method to display values
Void display(){System.out.println(rollno+" "+name+" "+college);}
}
//Test class to create and display the values of object
Public class TestStaticMethod{
Public static void main(String args[]){
Student.change();//calling change method
//creating objects
Student s1 = new Student(111,"Karan");
Student s2 = new Student(222,"Aryan");
Student s3 = new Student(333,"Sonoo");
//calling display method
s1.display();
s2.display();
s3.display();
}
}
Output
111 Karan BBDIT
222 Aryan BBDIT
333 Sonoo BBDIT
Key takeaway
In Java, the static keyword is mostly used for memory management.
With variables, methods, blocks, and nested classes, we can use the static keyword.
The static keyword refers to a class rather than a specific instance of that class.
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
When you want a variable to constantly store the same value, such as PI, the final keyword comes in handy (3.14159...).
The last keyword is referred to as a "modifier."
Example
Set a variable to final, to prevent it from being overridden/modified:
Public class Main {
Final int x = 10;
Public static void main(String[] args) {
Main myObj = new Main();
MyObj.x = 25; // will generate an error: cannot assign a value to a final variable
System.out.println(myObj.x);
}
}
This keyword
A reference variable in Java is a variable that refers to the current object of a method or constructor. The main use of this term in Java is to distinguish between class attributes and arguments with similar names.
The following are some examples of 'this' keyword usage:
● It can be used to refer to current class instance variables.
● It can be used to call or start the constructor of the current class.
● It can be used as a parameter in a method call.
● It's possible to send it as an argument to the constructor.
● It can be used to get the current instance of a class.
This keyword in Java can be used in a variety of ways. This is a reference variable in Java that points to the current object.
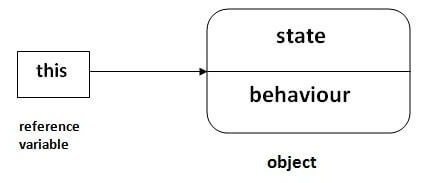
Fig 9: this
Example
Class Student{
Int rollno;
String name;
Float fee;
Student(int rollno,String name,float fee){
This.rollno=rollno;
This.name=name;
This.fee=fee;
}
Void display(){System.out.println(rollno+" "+name+" "+fee);}
}
Class TestThis2{
Public static void main(String args[]){
Student s1=new Student(111,"ankit",5000f);
Student s2=new Student(112,"sumit",6000f);
s1.display();
s2.display();
}
}
Output
111 ankit 5000.0
112 sumit 6000.0
Key takeaway
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
The main use of this term in Java is to distinguish between class attributes and arguments with similar names.
The process through which one class inherits the properties (methods and fields) of another is known as inheritance. The use of inheritance allows for the management of information in a hierarchical way.
A subclass (derived class, child class) is a class that inherits the properties of another, while a superclass is a class that inherits the properties of another (base class, parent class).
Extends keywords
The keyword extends is used to inherit a class's properties. The syntax for the extends keyword is as follows.
Class Super {
.....
.....
}
Class Sub extends Super {
.....
.....
}
When you use the extends keyword, it means you're creating a new class that inherits from an existing one. The word "extends" means "to improve functionality."
In Java, an inherited class is referred to as a parent or superclass, while the new class is referred to as a child or subclass.
Terminology used in inheritance
Class - A class is a collection of objects with similar attributes. It's a blueprint or template from which items are made.
Sub class / child class - A subclass is a class that inherits the properties of another class. A derived class, extended class, or kid class is another name for it.
Superclass / parent class - The features of a subclass are inherited from the superclass. It's also known as a parent class or a base class.
Reusability - Reusability, as the name implies, is a feature that allows you to reuse the fields and methods of an existing class while creating a new one. The fields and methods defined in the preceding class can be reused.
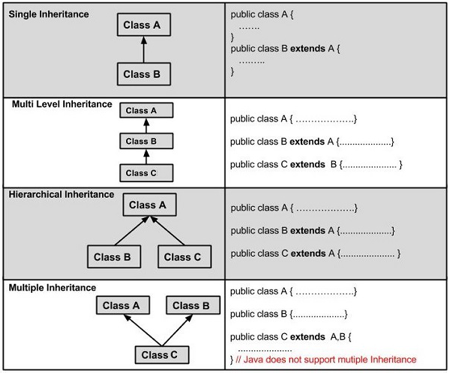
Fig 10: Types of inheritance
Using super to call super class constructor
● Subclasses can have their own private data members, as well as their own constructors.
● Only the subclass's instance variables can be initialized by the subclass's constructors. As a result, when a subclass object is instantiated, the subclass object must additionally perform one of the superclass' constructors.
● The super keyword is used to call a superclass constructor. The following programs show how to utilize the super keyword.
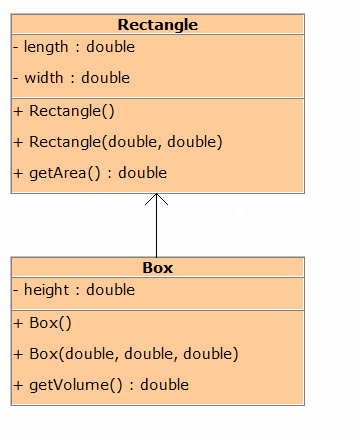
Fig 11: Example
Example
(Rectangle.java)
/**
* This class holds data of a Rectangle.
*/
Public class Rectangle
{
Private double length; // To hold length of rectangle
Private double width; // To hold width of rectangle
/**
* The constructor initialize rectangle's
* length and width with default value
*/
Public Rectangle()
{
Length = 0;
Width = 0;
}
/**
* The constructor accepts the rectangle's
* length and width.
*/
Public Rectangle(double length, double width)
{
This.length = length;
This.width = width;
}
/**
* The getArea method returns the area of
* the rectangle.
*/
Public double getArea()
{
Return length * width;
}
}
(Box.java)
/**
* This class holds data of a Box.
*/
Public class Box extends Rectangle
{
Private double height; // To hold height of the box
/**
* The constructor initialize box's
* length, width and height with default value.
*/
Public Box()
{
// Call the superclass default constructor to
// initialize length and width.
Super();
//Initialize height.
Height = 0;
}
/**
* The constructor accepts the box's
* length, width and height.
*/
Public Box(double length, double width, double height)
{
// Call the superclass constructor to
// initialize length and width.
Super(length, width);
// Initialize height.
This.height = height;
}
/**
* The getVolume method returns the volume of
* the box.
*/
Public double getVolume()
{
Return getArea() * height;
}
}
(BoxDemo.java)
/**
* This program demonstrates calling
* of superclass constructor.
*/
Public class BoxDemo
{
Public static void main(String[] args)
{
// Create a box object.
Box myBox1 = new Box();
// Display the volume of myBox1.
System.out.println("Volume: " + myBox1.getVolume());
// Create a box object.
Box myBox2 = new Box(12.2, 3.5, 2.0);
// Display the volume of myBox2.
System.out.println("Volume: " + myBox1.getVolume());
}
}
Key takeaway
- The process through which one class inherits the properties (methods and fields) of another is known as inheritance.
- The use of inheritance allows for the management of information in a hierarchical way.
In Java, method overriding occurs when a subclass (child class) has the same method as the parent class.
In other words, method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
Usage of Java Method Overriding
● Method overriding is a technique for providing a particular implementation of a method that has already been implemented by its superclass.
● For runtime polymorphism, method overriding is employed.
Rules for method overriding
● The argument list should match that of the overridden method exactly.
● The return type must be the same as or a subtype of the return type stated in the superclass's original overridden method.
● The access level must be lower than the access level of the overridden method. For instance, if the superclass method is marked public, the subclass overriding method cannot be either private or protected.
● Instance methods can only be overridden if the subclass inherits them.
● It is not possible to override a method that has been declared final.
● A static method cannot be overridden, but it can be re-declared.
● A method can't be overridden if it can't be inherited.
● Any superclass function that is not declared private or final can be overridden by a subclass in the same package as the instance's superclass.
● Only non-final methods labeled public or protected can be overridden by a subclass from a different package.
● Regardless of whether the overridden method throws exceptions or not, an overriding method can throw any unchecked exceptions. The overriding method, on the other hand, should not throw any checked exceptions that aren't specified by the overridden method. In comparison to the overridden method, the overriding method can throw fewer or narrower exceptions.
● Overriding constructors is not possible.
Example
//Java Program to illustrate the use of Java Method Overriding
//Creating a parent class.
Class Vehicle{
//defining a method
Void run(){System.out.println("Vehicle is running");}
}
//Creating a child class
Class Bike2 extends Vehicle{
//defining the same method as in the parent class
Void run(){System.out.println("Bike is running safely");}
Public static void main(String args[]){
Bike2 obj = new Bike2();//creating object
Obj.run();//calling method
}
}
Output
Bike is running safely
Key takeaway
Method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
The dynamic dispatch Method that is mechanism resolves a call to an overridden method at runtime. Runtime polymorphism is implemented in Java in this way. When a reference calls an overridden method, Java selects which version of the method to execute based on the type of object being referenced. To put it another way, the kind of object to which it refers decides which version of the overridden method is invoked.
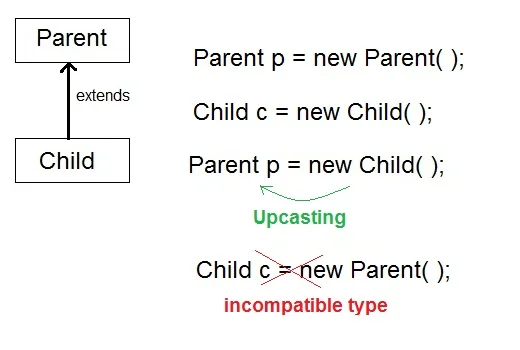
Fig 12: Dynamic method dispatch
Example
Class Animal {
Public void move() {
System.out.println("Animals can move");
}
}
Class Dog extends Animal {
Public void move() {
System.out.println("Dogs can walk and run");
}
}
Public class TestDog {
Public static void main(String args[]) {
Animal a = new Animal(); // Animal reference and object
Animal b = new Dog(); // Animal reference but Dog object
a.move(); // runs the method in Animal class
b.move(); // runs the method in Dog class
}
}
Output
Animals can move
Dogs can walk and run
Even though b is a type of Animal, the move function in the Dog class is called in the above example. The reason for this is that the reference type is checked at compilation time. However, during runtime, JVM determines the object type and executes the method associated with that object.
As a result, because the Animal class has the method move, the program will compile correctly in the example above. The method specific for that object is then executed at runtime.
Key takeaway
The dynamic dispatch Method that is the mechanism resolves a call to an overridden method at runtime. Runtime polymorphism is implemented in Java in this way.
● Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
● It can only be inherited by the concrete classes (descendent classes), where a concrete class can be instantiated.
● Abstract class act as a generic class having attributes and methods which can be commonly shared and accessed by the concrete classes.
● The attributes and methods in the abstract class are non-static, accessible by inheriting classes.
● Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class
● It can be represented as below
Abstract public class RentalCar {
// your code goes here
}
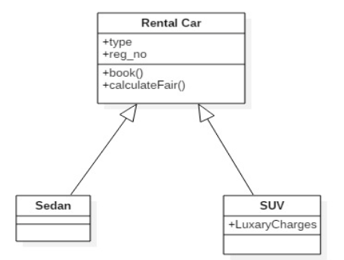
Fig 13: Example of abstract class
Key takeaway
- Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
- Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class.
● The final keyword is final, which means we can't change anything.
● Variables, methods, and classes can all benefit from final keywords.
● If we use the final keyword for inheritance, that is, if we declare any method in the base class with the final keyword, the final method's implementation will be the same as in the derived class.
● If any other class extends this subclass, we can declare the final method in any subclass we wish.
Declare final variable with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final variable pa of string type i.e
The value of this variable is fixed throughout all
The derived classes or not overridden*/
Final String pa = "Hello , We are in parent class variable";
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of variable ch of string type i.e
The value of this variable is not fixed throughout all
The derived classes or overidden*/
String ch = "Hello , We are in child class variable";
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a variable pa by parent object
System.out.println(p.pa);
// Creation of Child class object
Child c = new Child();
// Calling a variable ch by Child object
System.out.println(c.ch);
// Calling a variable pa by Child object
System.out.println(c.pa);
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, We are in parent class variable
Hello, We are in child class variable
Hello, We are in parent class variable
Declare final methods with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final method parent of void type i.e
The implementation of this method is fixed throughout
All the derived classes or not overidden*/
Final void parent() {
System.out.println("Hello , we are in parent method");
}
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of final method child of void type i.e
The implementation of this method is not fixed throughout
All the derived classes or not overidden*/
Void child() {
System.out.println("Hello , we are in child method");
}
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a method parent() by parent object
p.parent();
// Creation of Child class object
Child c = new Child();
// Calling a method child() by Child class object
c.child();
// Calling a method parent() by child object
c.parent();
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, we are in parent method
Hello, we are in child method
Hello, we are in parent method
In Java, packages are used to avoid naming conflicts, limit access, and make searching/locating and using classes, interfaces, enumerations, and annotations easier, among other things.
A Package is a collection of linked types (classes, interfaces, enumerations, and annotations) that provides namespace management and access control.
A Java package is a collection of classes, interfaces, and sub-packages that are similar in nature. In Java, packages are divided into two types: built-in packages and user-defined packages.
Many built-in packages are available, including java, lang, awt, javax, swing, net, io, util, sql, and so on.
Some of the Java packages that are currently available are:
● java.lang - Contains all of the core courses.
● java.io - This package includes classes for input and output functions.
Programmers can create their own packages to gather together classes, interfaces, and other items. It's a good idea to group related classes that you've implemented so that a programmer can quickly see how the classes, interfaces, enumerations, and annotations are connected.
Advantages
1) The Java package is used to organize the classes and interfaces in order to make them easier to maintain.
2) Access control is provided via the Java package.
3) The Java package eliminates naming conflicts.
Access protection
The scope of the class and its members is defined by access modifiers (data and methods). Private members, for example, can be accessed by other members of the same class (methods). Java has several degrees of security that allow members (variables and methods) within classes, subclasses, and packages to be visible.
Packages are used to encapsulate information and act as containers for classes and subpackages. Data and methods are contained in classes. When it comes to the visibility of class members between classes and packages, Java offers four options:
● Subclasses in the same package
● Non-subclasses in the same package
● Subclasses in different packages
● Classes that are neither in the same package nor subclasses
These categories require a variety of access methods, which are provided by the three main access modifiers private, public, and protected.
Simply remember that private can only be seen within its class, public can be seen from anyone, and protected can only be accessed in subclasses within the hierarchy.
There are just two access modifiers that a class can have: default and public. If a class has default access, it can only be accessed by other code inside the same package. However, if the class is public, it can be accessed from anywhere by any code.
Import
The package name is not required if a class wants to utilize another class from the same package. Classes in the same package communicate with one another without the use of any additional syntax.
Example
In this case, a class called Boss is added to the payroll package, which already includes Employee. As seen by the following Boss class, the Boss can refer to the Employee class without requiring the payroll prefix.
Package payroll;
Public class Boss
{
Public void payEmployee(Employee e)
{
e.mailCheck();
}
}
What if the Employee class isn't included in your payroll package? For referring to a class in a separate package, the Boss class must utilize one of the following ways.
The class's fully qualified name can be utilized. As an example,
Payroll.Employee
The import keyword and the wild card (*) can be used to import the package. For example -
Import payroll.*;
The import keyword can be used to import the class itself. For instance,
Import payroll.Employee;
Any number of import statements can be found in a class file. After the package statement and before the class definition, the import statements must exist.
In Java, an interface is a reference type. It's comparable to a class. It consists of a set of abstract methods. When a class implements an interface, it inherits the interface's abstract methods.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods. Method bodies are only available for default and static methods.
The process of creating an interface is identical to that of creating a class. A class, on the other hand, describes an object's features and behaviors. An interface, on the other hand, contains the behaviors that a class implements.
Unless the interface's implementation class is abstract, all of the interface's methods must be declared in the class.
In the following ways, an interface is analogous to a class:
● Any number of methods can be included in an interface.
● An interface is written in a file with the extension.java, with the interface's name matching the file's name.
● A.class file contains the byte code for an interface.
● Packages have interfaces, and the bytecode file for each must be in the same directory structure as the package name.
Why do we use interfaces?
There are three primary reasons to utilize an interface. Below is a list of them.
● It's a technique for achieving abstraction.
● We can offer various inheritance functionality via an interface.
● It's useful for achieving loose coupling.
Declaration
The interface keyword is used to declare an interface. All methods in an interface are declared with an empty body, and all fields are public, static, and final by default. A class that implements an interface is required to implement all of the interface's functions.
Syntax
Interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Key takeaway
- In Java, an interface is a reference type.
- An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods.
When a class implements an interface, it's like the class signing a contract, agreeing to perform the interface's defined actions. If a class doesn't perform all of the interface's actions, it must declare itself abstract.
To implement an interface, a class utilizes the implements keyword. Following the extends component of the declaration, the implements keyword appears in the class declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
Public void eat() {
System.out.println("Mammal eats");
}
Public void travel() {
System.out.println("Mammal travels");
}
Public int noOfLegs() {
Return 0;
}
Public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Output
Mammal eats
Mammal travels
There are a few principles to follow when overriding methods specified in interfaces.
● Checked exceptions should not be declared on implementation methods other than those specified by the interface method or their subclasses.
● When overriding the methods, keep the signature of the interface method and the same return type or subtype.
● Interface methods do not need to be implemented if the implementation class is abstract.
When it comes to implementing interfaces, there are a few guidelines to follow.
● At any given time, a class can implement multiple interfaces.
● A class can only extend one other class while implementing several interfaces.
● In the same manner that a class can extend another class, an interface can extend another interface.
In the same way that a java interface can have methods, an interface can also contain variables such as int, float, and string. Variables in an interface are by default static and final. In Java, all variables in an interface should only have public access.
An interface specifies a behavior protocol rather than how we should be implemented. The protocol described by an interface is followed by a class that implements that interface.
Because java interfaces cannot be instantiated on their own, interface variables are static. The variable's value must be assigned in a static context without any instances.
The final modification assures that the interface variable's value is a real constant that cannot be changed. To put it another way, interfaces can only specify constants and not instance variables.
Templates
Interface interfaceName
{
// Any number of final, static variables
Datatype variableName = value;
// Any number of abstract method declarations
Returntype methodName(list of parameters or no parameters);
}
Example
In the example below, the interface Math contains a collection of constants related to mathematics.
Variables are handled as static and final by default in an interface. However, we write comprehensive declarations in the project for readability reasons, such as public static final double PI = 3.14;
/*
* Interface variables example
*/
Interface Math{
Public static final double PI = 3.14;
Public static final double ELUERNUMBER = 2.718;
Public static final double SQRT = 1.41421;
}
/*
* Test interface variables
*/
Public class Sample {
Public static void main(String[] args) {
//Calculate area of circle
Int radius =2;
//call interface constant Math.PI
Double area = Math.PI*radius*radius;
System.out.println("Area of Circle ="+ area);
}
}
Output
Area of Circle =12.56
Key takeaway
In the same way that a java interface can have methods, an interface can also contain variables such as int, float, and string.
Variables in an interface are by default static and final.
In the same manner that a class can extend another class, an interface can extend another interface. When you use the extends keyword to extend an interface, the new interface inherits the parent interface's methods.
Hockey and Football interfaces are added to the Sports interface below.
Example
// Filename: Sports.java
Public interface Sports {
Public void setHomeTeam(String name);
Public void setVisitingTeam(String name);
}
// Filename: Football.java
Public interface Football extends Sports {
Public void homeTeamScored(int points);
Public void visitingTeamScored(int points);
Public void endOfQuarter(int quarter);
}
// Filename: Hockey.java
Public interface Hockey extends Sports {
Public void homeGoalScored();
Public void visitingGoalScored();
Public void endOfPeriod(int period);
Public void overtimePeriod(int ot);
}
Hockey has four methods, but it inherits two from Sports; as a result, a class that implements Hockey must implement all six methods. Similarly, a class that implements Football must specify the three Football methods as well as the two Sports methods.
Exception Handling in Java is a strong technique for handling runtime failures so that the application's normal flow may be maintained.
We'll learn about Java exceptions, their types, and the difference between checked and unchecked exceptions on this page.
Exception: An exception in Java is an event that causes the program's usual flow to be disrupted. It's a type of object that's thrown at runtime.
Exception Handling: Exception Handling is a framework for dealing with runtime issues like ClassNotFoundException, IOException, SQLException, and RemoteException, among others.
Exception keywords
In Java, there are five keywords that are used to handle exceptions.
Try- The "try" keyword is used to designate a block where exception code should be placed. Either catch or finally must come after the try block. This means that we can't just utilize the try block.
Catch- To handle the exception, the "catch" block is utilized. It must be preceded by a try block, thus we can't just use catch block. It can be followed by a later finally block.
Finally- The "finally" block is used to run the program's most critical code. Whether or not an exception is handled, it is run.
Throw- To throw an exception, use the "throw" keyword.
Throws- Exceptions are declared with the "throws" keyword. There isn't any exception thrown. It specifies that an exception may occur in the method. It's usually used in conjunction with a method signature.
Key takeaway
- Exception Handling in Java is a strong technique for handling runtime failures so that the application's normal flow may be maintained.
- An exception in Java is an event that causes the program's usual flow to be disrupted.
- It's a type of object that's thrown at runtime
Checked exceptions, often known as compile time exceptions, are exceptions that are checked (notified) by the compiler at compilation time. These exceptions cannot simply be ignored, the programmer should take care of (manage) these exceptions.
If you use the FileReader class to read data from a file and the file supplied in its constructor does not exist, a FileNotFoundException is thrown, and the compiler tells the programmer to handle the problem.
Example
Import java.io.File;
Import java.io.FileReader;
Public class FilenotFound_Demo {
Public static void main(String args[]) {
File file = new File("E://file.txt");
FileReader fr = new FileReader(file);
}
}
Output
C:\>javac FilenotFound_Demo.java
FilenotFound_Demo.java:8: error: unreported exception FileNotFoundException; must be caught or declared to be thrown
FileReader fr = new FileReader(file);
^
1 error
Because the FileReader class's read() and shut() methods return IOException, the compiler warns you to handle IOException as well as FileNotFoundException.
Unchecked exceptions
An unchecked exception is one that arises during the execution of a program. Runtime Exceptions are another name for them. Programming errors, such as logic flaws or improper usage of an API, are examples. At the time of compilation, runtime exceptions are ignored.
For example, if you declare an array of size 5 in your application and then try to call the array's 6th element, you'll get an ArrayIndexOutOfBoundsException.
Example
Public class Unchecked_Demo
{
Public static void main(String args[]) {
Int num[] = {1, 2, 3, 4};
System.out.println(num[5]);
}
}
Output
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 5
At Exceptions.Unchecked_Demo.main(Unchecked_Demo.java:8)
Key takeaway
Checked exceptions, often known as compile time exceptions, are exceptions that are checked (notified) by the compiler at compilation time.
An unchecked exception is one that arises during the execution of a program. Runtime Exceptions are another name for them.
The try block in Java is used to contain code that could potentially throw an exception. It has to be utilized as part of the technique.
The rest of the block code will not execute if an exception occurs at a specific statement in the try block. As a result, code that does not throw an exception should not be kept in a try block.
Either a catch or a finally block must be used after a try block in Java.
Syntax of Java try-catch
Try {
// Protected code
} catch (ExceptionName e1) {
// Catch block
}
Syntax of try-finally block
Try{
//code that may throw an exception
}finally{}
By declaring the kind of exception within the parameter, the Java catch block is utilized to handle the Exception. The parent class exception (i.e., Exception) or the created exception type must be defined. However, the good method is to declare the created kind of exception.
Only after the try block should the catch block be utilized. With a single try block, you can utilize many catch blocks.
Example
Public class TryCatchExample
{
Public static void main(String[] args) {
Try
{
Int data=50/0; //may throw exception
}
//handling the exception
Catch(ArithmeticException e)
{
System.out.println(e);
}
System.out.println("rest of the code");
}
}
Output
Java.lang.ArithmeticException: / by zero
Rest of the code
Key takeaway
The try block in Java is used to contain code that could potentially throw an exception. It has to be utilized as part of the technique.
Only after the try block should the catch block be utilized. With a single try block, you can utilize many catch blocks.
Multiple catch blocks can be placed after a try block. The following is the syntax for numerous catch blocks:
Syntax
Try {
// Protected code
} catch (ExceptionType1 e1) {
// Catch block
} catch (ExceptionType2 e2) {
// Catch block
} catch (ExceptionType3 e3) {
// Catch block
}
The above statements show three catch blocks, but after a single try, you can have any number of them. If there is an exception in the protected code, it is thrown to the first catch block in the list. If the thrown exception's data type matches ExceptionType1, it is caught there. If this is not the case, the exception is sent to the second catch statement. This continues until the exception is caught or falls through all catches, at which point the current function is terminated and the exception is passed down the call stack to the preceding method.
Example
This section of code demonstrates how to use numerous try/catch statements.
Try {
File = new FileInputStream(fileName);
x = (byte) file.read();
} catch (IOException i) {
i.printStackTrace();
Return -1;
} catch (FileNotFoundException f) // Not valid! {
f.printStackTrace();
Return -1;
}
Key takeaway
Multiple catch blocks can be placed after a try block.
If a method does not handle a checked exception, the throws keyword must be used to declare it. The keyword throws is found at the end of a method's signature.
The throw keyword can be used to throw an exception, either a newly instantiated one or one that you have just caught.
The exception object that will be thrown is specified. The Exception has a message attached to it that describes the error. These errors could be caused by user inputs, the server, or something else entirely.
The throw keyword in Java can be used to throw either checked or unchecked exceptions. Its primary function is to throw a custom exception.
We may also use the throw keyword to establish our own set of circumstances and explicitly throw an exception. If we divide a number by another number, for example, we can throw an Arithmetic Exception. All we have to do now is set the condition and use the throw keyword to throw an exception.
Syntax
throw new exception_class("error message");
Example
Public class TestThrow1 {
//function to check if person is eligible to vote or not
Public static void validate(int age) {
If(age<18) {
//throw Arithmetic exception if not eligible to vote
Throw new ArithmeticException("Person is not eligible to vote");
}
Else {
System.out.println("Person is eligible to vote!!");
}
}
//main method
Public static void main(String args[]){
//calling the function
Validate(13);
System.out.println("rest of the code...");
}
}
Output

Throws
To define an exception in Java, use the throws keyword. It informs the programmer that an exception may occur. As a result, it is preferable for the programmer to provide exception handling code so that the program's usual flow can be maintained.
Exception Handling is mostly used to deal with exceptions that have been checked. If an unchecked exception arises, such as a Null Pointer Exception, it is the programmers' fault for not inspecting the code before using it.
Syntax
return_type method_name() throws exception_class_name{
//method code
}
Example
Import java.io.IOException;
Class Testthrows1{
Void m()throws IOException{
Throw new IOException("device error");//checked exception
}
Void n()throws IOException{
m();
}
Void p(){
Try{
n();
}catch(Exception e){System.out.println("exception handled");}
}
Public static void main(String args[]){
Testthrows1 obj=new Testthrows1();
Obj.p();
System.out.println("normal flow...");
}
}
Output
Exception handled
Normal flow...
Key takeaway
The throw keyword can be used to throw an exception, either a newly instantiated one or one that you have just caught.
If a method does not handle a checked exception, the throws keyword must be used to declare it. The keyword throws is found at the end of a method's signature.
After a try block or a catch block, the finally block is used. Regardless of whether or not an Exception occurs, a finally block of code is always executed.
No of what occurs in the protected code, using a finally block allows you to run any cleanup-type statements you want.
Finally, at the end of the catch blocks, there is a finally block with the following syntax:
Try {
// Protected code
} catch (ExceptionType1 e1) {
// Catch block
} catch (ExceptionType2 e2) {
// Catch block
} catch (ExceptionType3 e3) {
// Catch block
}finally {
// The finally block always executes.
}
Example
Public class ExcepTest {
Public static void main(String args[]) {
Int a[] = new int[2];
Try {
System.out.println("Access element three :" + a[3]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Exception thrown :" + e);
}finally {
a[0] = 6;
System.out.println("First element value: " + a[0]);
System.out.println("The finally statement is executed");
}
}
}
Output
Exception thrown :java.lang.ArrayIndexOutOfBoundsException: 3
First element value: 6
The finally statement is executed
Take note of the following:
● A try statement is required for a catch clause to occur.
● Finally clauses are not required whenever a try/catch block is present.
● There can't be a try block without a catch clause or a finally clause.
● There can't be any code between the try, catch, and finally blocks.
Built in exceptions
Exceptions that are present in Java libraries are known as built-in exceptions. These exceptions are appropriate for explaining specific mistake scenarios. The following is a list of noteworthy Java built-in exceptions.
Arithmetic Exception
When an unusual circumstance in an arithmetic operation occurs, it is thrown.
Array Index out of Bounds Exception
It's thrown when an array has been accessed with an incorrect index. The index can be negative, or it can be more than or equal to the array's size.
Class Not Found Exception
When we try to access a class whose definition is missing, this Exception is thrown.
File Not Found Exception
When a file is not accessible or does not open, this Exception is thrown.
IO Exception
When an input-output operation fails or is interrupted, this exception is issued.
Interrupted Exception
When a thread is interrupted while waiting, sleeping, or processing, this exception is issued.
No Such Field Exception
It's thrown when a class doesn't have the requested field (or variable).
NoSuchMethodException
It's thrown when you try to call a method that doesn't exist.
NullPointerException
When referring to the members of a null object, this exception is thrown. Nothing is represented by null.
NumberFormatException
When a method fails to convert a string to a numeric representation, this exception is thrown.
RuntimeException
Any exception that happens during runtime is represented by this variable.
StringIndexOutOfBoundsException
It's thrown by String class methods when an index is either negative or larger than the string's length.
Example
// Java program to demonstrate ArithmeticException
Class ArithmeticException_Demo
{
Public static void main(String args[])
{
Try {
Int a = 30, b = 0;
Int c = a/b; // cannot divide by zero
System.out.println ("Result = " + c);
}
Catch(ArithmeticException e) {
System.out.println ("Can't divide a number by 0");
}
}
}
Output
Can't divide a number by 0
Key takeaway
After a try block or a catch block, the finally block is used. Regardless of whether or not an Exception occurs, a finally block of code is always executed.
Exceptions that are present in Java libraries are known as built-in exceptions. These exceptions are appropriate for explaining specific mistake scenarios.
In Java, you can construct your own exceptions. When writing your own exception classes, keep the following factors in mind:
● Throwable must be the parent of all exceptions.
● You must extend the Exception class if you want to build a checked exception that is enforced automatically by the Handle or Declare Rule.
● You must extend the RuntimeNException class if you want to write a runtime exception.
As shown below, we may create our own Exception class.
Class MyException extends Exception
{
}
To construct your own Exception, simply extend the default Exception class. These are regarded as exceptions that have been checked. The Insufficient Funds Exception class is a user-defined exception that extends the Exception class and is therefore examined. An exception class is similar to any other class in that it has properties and methods that are helpful.
Example
// File Name InsufficientFundsException.java
Import java.io.*;
Public class InsufficientFundsException extends Exception {
Private double amount;
Public InsufficientFundsException(double amount) {
This.amount = amount;
}
Public double getAmount() {
Return amount;
}
}
The following Checking Account class has a withdraw() method that throws an Insufficient Funds Exception to demonstrate how to use our user-defined exception.
// File Name CheckingAccount.java
Import java.io.*;
Public class CheckingAccount {
Private double balance;
Private int number;
Public CheckingAccount(int number) {
This.number = number;
}
Public void deposit(double amount) {
Balance += amount;
}
Public void withdraw(double amount) throws InsufficientFundsException {
If(amount <= balance) {
Balance -= amount;
}else {
Double needs = amount - balance;
Throw new InsufficientFundsException(needs);
}
}
Public double getBalance() {
Return balance;
}
Public int getNumber() {
Return number;
}
}
The following BankDemo program shows how to use CheckingAccount's deposit() and withdraw() methods.
// File Name BankDemo.java
Public class BankDemo {
Public static void main(String [] args) {
CheckingAccount c = new CheckingAccount(101);
System.out.println("Depositing $500...");
c.deposit(500.00);
Try {
System.out.println("\nWithdrawing $100...");
c.withdraw(100.00);
System.out.println("\nWithdrawing $600...");
c.withdraw(600.00);
} catch (InsufficientFundsException e) {
System.out.println("Sorry, but you are short $" + e.getAmount());
e.printStackTrace();
}
}
}
Output
Depositing $500...
Withdrawing $100...
Withdrawing $600...
Sorry, but you are short $200.0
InsufficientFundsException
At CheckingAccount.withdraw(CheckingAccount.java:25)
At BankDemo.main(BankDemo.java:13)
References:
- Introduction to Java Programming: Liang, Pearson Education, 7th Edition.
- Balguruswamy, Programming with Java, TMH.
- Programming with Java: Bhave & Patekar, Person Eduction.
- Http://www.beginwithjava.com/java/inheritance/calling-the-superclass-constructor.html
- Https://www.tutorialspoint.com/java/java_basic_operators.htm
- Https://www.w3schools.com/java/ref_keyword_final.asp