UNIT- 2
Arithmetic expressions and precedence
Arithmetic Expressions consist of numeric literals, arithmetic operators, and numeric variables. They simplify to a single value, when evaluated.
Here is an example of an arithmetic expression with no variables:
3.14*10*10
This expression evaluates to 314, the approximate area of a circle with radius 10. Similarly, the expression
3.14*radius*radius
Would also evaluate to 314, if the variable radius stored the value 10.
Note that the parentheses in the last expression helps dictate which order to evaluate the expression.
Multiplication and division have a higher order of precedence than addition and subtraction. In an arithmetic expression, it is evaluated left to right, only performing the multiplications and divisions.
After doing this, process the expression again from left to right, doing all the additions and subtractions.
So,
3+4*5
First evaluates to
3+20 which then evaluates to 23.
Consider this expression:
3 + 4*5 - 6/3*4/8 + 2*6 - 4*3*2
First go through and do all the multiplications and divisions:
3 + 20 - 1 + 12 - 24
Integer Division: In particular, if the division has a leftover remainder or fraction, this is simply discarded.
For example:
13/4 evaluates to 3
19/3 evaluates to 6 but
Similarly, if you have an expression with integer variables part of a division, this evaluates to an integer as well.
For example, in this segment of code, y gets set to 2.
Int x = 8;
Int y = x/3;
However, if we did the following,
Double x = 8;
Double y = x/3;
y would equal 2.66666666 (approximately).
The way C decides whether it will do an integer division (as in the first example), or a real number division (as in the second example), is based on the TYPE of the operands.
If both operands are ints, an integer division is done.
If either operand is a float or a double, then a real division is done. The compiler will treat constants without the decimal point as integers, and constants with the decimal point as a float. Thus, the expressions 13/4 and 13/4.0 will evaluate to 3 and 3.25 respectively.
The mod operator (%)
The one new operator is the mod operator, which is denoted by the percent sign(%). This operator is ONLY defined for integer operands. It is defined as follows:
a%b evaluates to the remainder of a divided by b.
For example,
12%5 = 2
19%6 = 1
14%7 = 0
19%200 = 19
The precedence of the mod operator is the same as the precedence of multiplication and division.
Every expression in C language contains set of operators and operands. Operators are the special symbols which are used to indicate the type of operation whereas operands are the data member or variables operating as per operation specified by operator. One expression contains one or more set of operators and operands. So to avoid the ambiguity in execution of expression, C compiler fixed the precedence of operator. Depending on the operation, operators are classified into following category.
- Arithmetic Operator: These are the operators which are useful in performing mathematical calculations. Following are the list of arithmetic operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
+ | Used to add two operands | Result of A+B is 15 | |
2. | - | Used to subtract two operands | Result of A-B is 5 |
3. | * | Used to multiply two operands | Result of A*B is 50 |
4. | / | Used to divide two operands | Result of A/B is 2 |
5. | % | Find reminder of the division of two operand | Result of A%B is 0 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of addition of %d and %d is %d",a,b,a+b);
Printf("\n Result of subtraction of %d and %d is %d",a,b,a-b);
Printf("\n Result of multiplication %d and %d is %d",a,b,a*b);
Printf("\n Result of division of %d and %d is %d",a,b,a/b);
Printf("\n Result of modulus of %d and %d is %d",a,b,a%b);
Getch();
}
Output:
Enter two numbers
5
3
Result of addition of 5 and 3 is 8
Result of subtraction of 5 and 3 is 2
Result of multiplication of 5 and 3 is 15
Result of division of 5 and 3 is 1
Result of modulus of 5 and 3 is 2
2. Relational Operators: Relational operator used to compare two operands. Relational operator produce result in terms of binary values. It returns 1 when the result is true and 0 when result is false. Following are the list of relational operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | < | This is less than operator which is used to check whether the value of left operand is less than the value of right operand or not | Result of A<B is false
|
2. | > | This is greater than operator which is used to check whether the value of left operand is greater than the value of right operand or not | Result of A>B is true |
3. | <= | This is less than or equal to operator | Result of A<=B is false |
4. | >= | This is greater than or equal to operator | Result of A>=B is true |
5. | == | This is equal to operator which is used to check value of both operands are equal or not | Result of A==B is false |
6. | != | This is not equal to operator which is used to check value of both operands are equal or not | Result of A!=B is true |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of less than operator of %d and %d is %d",a,b,a<b);
Printf("\n Result of greater than operator of %d and %d is %d",a,b,a>b);
Printf("\n Result of leass than or equal to operator %d and %d is %d",a,b,a<=b);
Printf("\n Result of greater than or equal to operator of %d and %d is %d", a,b,a>=b);
Printf("\n Result of double equal to operator of %d and %d is %d",a,b,a==b);
Printf("\n Result of not equal to operator of %d and %d is %d",a,b,a!=b);
Getch();
}
Output:
Enter two numbers
5
3
Result of less than operator of 5 and 3 is 0
Result of greater than operator of 5 and 3 is 1
Result of less than or equal to operator of 5 and 3 is 0
Result of greater than or equal to operator of 5 and 3 is 1
Result of double equal to operator of 5 and 3 is 0
Result of not equal to operator of 5 and 3 is 1
Precedence and Associativity
Operator precedence determines the grouping of terms in an expression and decides how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has a higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has a higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | <<>> | Left to right |
Relational | <<= >>= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
Example Code
#include <stdio.h>
Main() {
Int a = 20;
Int b = 10;
Int c = 15;
Int d = 5;
Int e;
e = (a + b) * c / d; // ( 30 * 15 ) / 5
Printf("Value of (a + b) * c / d is : %d\n", e );
e = ((a + b) * c) / d; // (30 * 15 ) / 5
Printf("Value of ((a + b) * c) / d is : %d\n" , e );
e = (a + b) * (c / d); // (30) * (15/5)
Printf("Value of (a + b) * (c / d) is : %d\n", e );
e = a + (b * c) / d; // 20 + (150/5)
Printf("Value of a + (b * c) / d is : %d\n" , e );
Return 0;
}
Output
Value of (a + b) * c / d is : 90
Value of ((a + b) * c) / d is : 90
Value of (a + b) * (c / d) is : 90
Value of a + (b * c) / d is : 50
Conditional Branching
If else Statement
The if-else statement in C is used to perform the operations based on some specific condition. The operations specified in if block are executed if and only if the given condition is true.
There are the following variants of if statement in C language.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
- If(expression){
- //code to be executed
- }
Flowchart of if statement in C
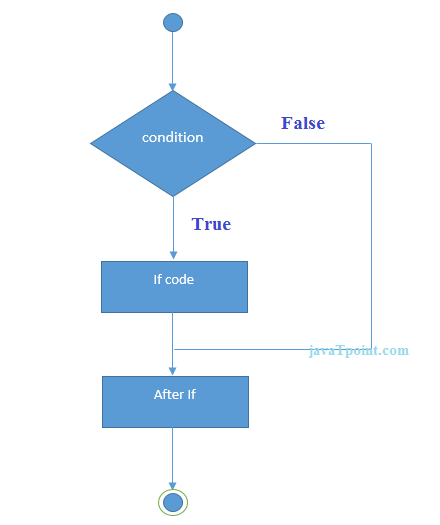
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 is largest
If-else Statement
The if-else statement is used to perform two operations for a single condition. The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition. Here, we must notice that if and else block cannot be executed simiulteneously. Using if-else statement is always preferable since it always invokes an otherwise case with every if condition. The syntax of the if-else statement is given below.
- If(expression){
- //code to be executed if condition is true
- }else{
- //code to be executed if condition is false
- }
Flowchart of the if-else statement in C
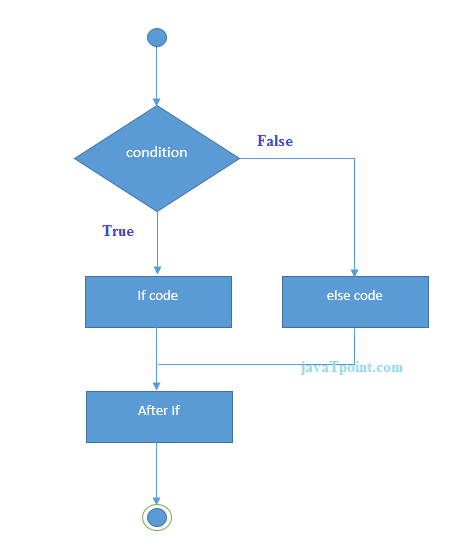
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Else{
- Printf("%d is odd number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
- #include <stdio.h>
- Int main()
- {
- Int age;
- Printf("Enter your age?");
- Scanf("%d",&age);
- If(age>=18)
- {
- Printf("You are eligible to vote...");
- }
- Else
- {
- Printf("Sorry ... you can't vote");
- }
- }
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... You can't vote
If else-if ladder Statement
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
- If(condition1){
- //code to be executed if condition1 is true
- }else if(condition2){
- //code to be executed if condition2 is true
- }
- Else if(condition3){
- //code to be executed if condition3 is true
- }
- ...
- Else{
- //code to be executed if all the conditions are false
- }
Flowchart of else-if ladder statement in C
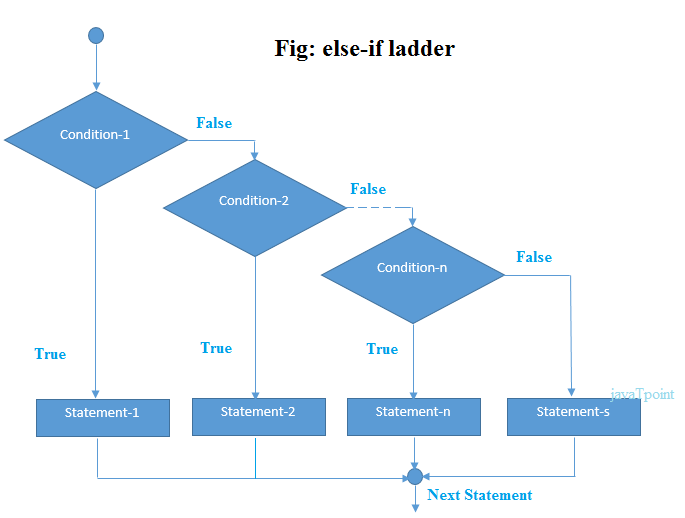
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats ! you scored grade A ...
Switch Statement
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement is given below:
- Switch(expression){
- Case value1:
- //code to be executed;
- Break; //optional
- Case value2:
- //code to be executed;
- Break; //optional
- ......
- Default:
- Code to be executed if all cases are not matched;
- }
Rules for switch statement in C language
1) The switch expression must be of an integer or character type.
2) The case value must be an integer or character constant.
3) The case value can be used only inside the switch statement.
4) The break statement in switch case is not must. It is optional. If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
Let's try to understand it by the examples. We are assuming that there are following variables.
- Int x,y,z;
- Char a,b;
- Float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
Switch(x) | Switch(f) | Case 3; | Case 2.5; |
Switch(x>y) | Switch(x+2.5) | Case 'a'; | Case x; |
Switch(a+b-2) |
| Case 1+2; | Case x+2; |
Switch(func(x,y)) |
| Case 'x'>'y'; | Case 1,2,3; |
Flowchart of switch statement in C
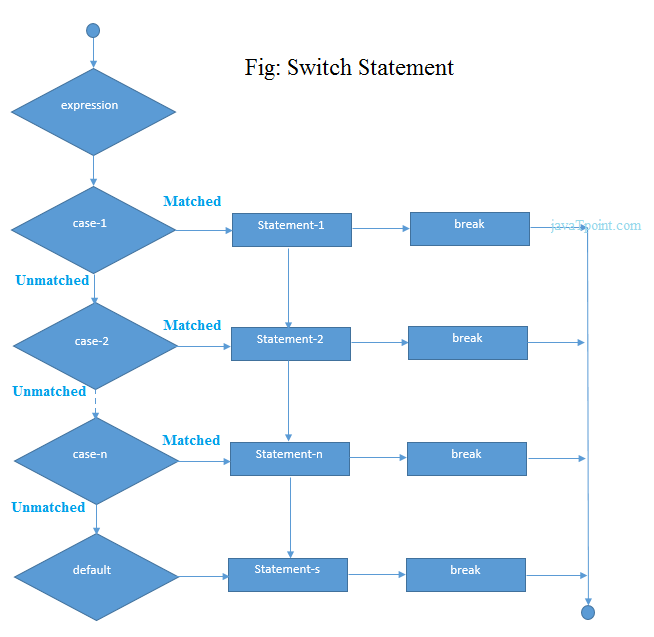
Functioning of switch case statement
First, the integer expression specified in the switch statement is evaluated. This value is then matched one by one with the constant values given in the different cases. If a match is found, then all the statements specified in that case are executed along with the all the cases present after that case including the default statement. No two cases can have similar values. If the matched case contains a break statement, then all the cases present after that will be skipped, and the control comes out of the switch. Otherwise, all the cases following the matched case will be executed.
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example 2
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
C Switch statement is fall-through
In C language, the switch statement is fall through; it means if you don't use a break statement in the switch case, all the cases after the matching case will be executed.
Let's try to understand the fall through state of switch statement by the example given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equal to 10\n");
- Case 50:
- Printf("number is equal to 50\n");
- Case 100:
- Printf("number is equal to 100\n");
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:10
Number is equal to 10
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Output
Enter a number:50
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Nested switch case statement
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
- #include <stdio.h>
- Int main () {
- Int i = 10;
- Int j = 20;
- Switch(i) {
- Case 10:
- Printf("the value of i evaluated in outer switch: %d\n",i);
- Case 20:
- Switch(j) {
- Case 20:
- Printf("The value of j evaluated in nested switch: %d\n",j);
- }
- }
- Printf("Exact value of i is : %d\n", i );
- Printf("Exact value of j is : %d\n", j );
- Return 0;
- }
Output
The value of i evaluated in outer switch: 10
The value of j evaluated in nested switch: 20
Exact value of i is : 10
Exact value of j is : 20
If-else vs switch
What is an if-else statement?
An if-else statement in C programming is a conditional statement that executes a different set of statements based on the condition that is true or false. The 'if' block will be executed only when the specified condition is true, and if the specified condition is false, then the else block will be executed.
Syntax of if-else statement is given below:
- If(expression)
- {
- // statements;
- }
- Else
- {
- // statements;
- }
What is a switch statement?
A switch statement is a conditional statement used in C programming to check the value of a variable and compare it with all the cases. If the value is matched with any case, then its corresponding statements will be executed. Each case has some name or number known as the identifier. The value entered by the user will be compared with all the cases until the case is found. If the value entered by the user is not matched with any case, then the default statement will be executed.
Syntax of the switch statement is given below:
- Switch(expression)
- {
- Case constant 1:
- // statements;
- Break;
- Case constant 2:
- // statements;
- Break;
- Case constant n:
- // statements;
- Break;
- Default:
- // statements;
- }
Similarity b/w if-else and switch
Both the if-else and switch are the decision-making statements. Here, decision-making statements mean that the output of the expression will decide which statements are to be executed.
Differences b/w if-else and switch statement
The following are the differences between if-else and switch statement are:
- Definition
If-else
Based on the result of the expression in the 'if-else' statement, the block of statements will be executed. If the condition is true, then the 'if' block will be executed otherwise 'else' block will execute.
Switch statement
The switch statement contains multiple cases or choices. The user will decide the case, which is to execute.
- Expression
If-else
It can contain a single expression or multiple expressions for multiple choices. In this, an expression is evaluated based on the range of values or conditions. It checks both equality and logical expressions.
Switch
It contains only a single expression, and this expression is either a single integer object or a string object. It checks only equality expression.
- Evaluation
If-else
An if-else statement can evaluate almost all the types of data such as integer, floating-point, character, pointer, or Boolean.
Switch
A switch statement can evaluate either an integer or a character.
- Sequence of Execution
If-else
In the case of 'if-else' statement, either the 'if' block or the 'else' block will be executed based on the condition.
Switch
In the case of the 'switch' statement, one case after another will be executed until the break keyword is not found, or the default statement is executed.
- Default Execution
If-else
If the condition is not true within the 'if' statement, then by default, the else block statements will be executed.
Switch
If the expression specified within the switch statement is not matched with any of the cases, then the default statement, if defined, will be executed.
- Values
If-else
Values are based on the condition specified inside the 'if' statement. The value will decide either the 'if' or 'else' block is to be executed.
Switch
In this case, value is decided by the user. Based on the choice of the user, the case will be executed.
- Use
If-else
It evaluates a condition to be true or false.
Switch
A switch statement compares the value of the variable with multiple cases. If the value is matched with any of the cases, then the block of statements associated with this case will be executed.
- Editing
If-else
Editing in 'if-else' statement is not easy as if we remove the 'else' statement, then it will create the havoc.
Switch
Editing in switch statement is easier as compared to the 'if-else' statement. If we remove any of the cases from the switch, then it will not interrupt the execution of other cases. Therefore, we can say that the switch statement is easy to modify and maintain.
- Speed
If-else
If the choices are multiple, then the speed of the execution of 'if-else' statements is slow.
Switch
The case constants in the switch statement create a jump table at the compile time. This jump table chooses the path of the execution based on the value of the expression. If we have a multiple choice, then the execution of the switch statement will be much faster than the equivalent logic of 'if-else' statement.
Let's summarize the above differences in a tabular form.
| If-else | Switch |
Definition | Depending on the condition in the 'if' statement, 'if' and 'else' blocks are executed. | The user will decide which statement is to be executed. |
Expression | It contains either logical or equality expression. | It contains a single expression which can be either a character or integer variable. |
Evaluation | It evaluates all types of data, such as integer, floating-point, character or Boolean. | It evaluates either an integer, or character. |
Sequence of execution | First, the condition is checked. If the condition is true then 'if' block is executed otherwise 'else' block | It executes one case after another till the break keyword is not found, or the default statement is executed. |
Default execution | If the condition is not true, then by default, else block will be executed. | If the value does not match with any case, then by default, default statement is executed. |
Editing | Editing is not easy in the 'if-else' statement. | Cases in a switch statement are easy to maintain and modify. Therefore, we can say that the removal or editing of any case will not interrupt the execution of other cases. |
Speed | If there are multiple choices implemented through 'if-else', then the speed of the execution will be slow. | If we have multiple choices then the switch statement is the best option as the speed of the execution will be much higher than 'if-else'. |
Loops
The looping can be defined as repeating the same process multiple times until a specific condition satisfies. There are three types of loops used in the C language. In this part of the tutorial, we are going to learn all the aspects of C loops.
Why use loops in C language?
The looping simplifies the complex problems into the easy ones. It enables us to alter the flow of the program so that instead of writing the same code again and again, we can repeat the same code for a finite number of times. For example, if we need to print the first 10 natural numbers then, instead of using the printf statement 10 times, we can print inside a loop which runs up to 10 iterations.
Advantage of loops in C
1) It provides code reusability.
2) Using loops, we do not need to write the same code again and again.
3) Using loops, we can traverse over the elements of data structures (array or linked lists).
Types of C Loops
There are three types of loops in C language that is given below:
- Do while
- While
- For
Do-while loop in C
The do-while loop continues until a given condition satisfies. It is also called post tested loop. It is used when it is necessary to execute the loop at least once (mostly menu driven programs).
The syntax of do-while loop in c language is given below:
- Do{
- //code to be executed
- }while(condition);
While loop in C
The while loop in c is to be used in the scenario where we don't know the number of iterations in advance. The block of statements is executed in the while loop until the condition specified in the while loop is satisfied. It is also called a pre-tested loop.
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
For loop in C
The for loop is used in the case where we need to execute some part of the code until the given condition is satisfied. The for loop is also called as a per-tested loop. It is better to use for loop if the number of iteration is known in advance.
The syntax of for loop in c language is given below:
- For(initialization;condition;incr/decr){
- //code to be executed
- }
Do while loop
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
Do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example 1
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Flowchart of do while loop
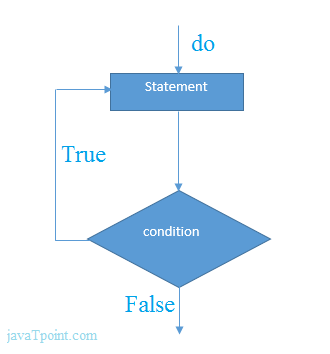
do while example
There is given the simple program of c language do while loop where we are printing the table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- Do{
- Printf("%d \n",i);
- i++;
- }while(i<=10);
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loop
The do-while loop will run infinite times if we pass any non-zero value as the conditional expression.
- Do{
- //statement
- }while(1);
While loop
While loop is also known as a pre-tested loop. In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition. It can be viewed as a repeating if statement. The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax of while loop in C language
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
Flowchart of while loop in C
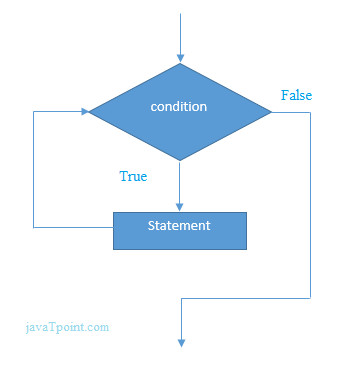
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- While(i<=10){
- Printf("%d \n",i);
- i++;
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
- #include<stdio.h>
- Int main(){
- Int i=1,number=0,b=9;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- While(i<=10){
- Printf("%d \n",(number*i));
- i++;
- }
- Return 0;
- }
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
Properties of while loop
- A conditional expression is used to check the condition. The statements defined inside the while loop will repeatedly execute until the given condition fails.
- The condition will be true if it returns 0. The condition will be false if it returns any non-zero number.
- In while loop, the condition expression is compulsory.
- Running a while loop without a body is possible.
- We can have more than one conditional expression in while loop.
- If the loop body contains only one statement, then the braces are optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int j = 1;
- While(j+=2,j<=10)
- {
- Printf("%d ",j);
- }
- Printf("%d",j);
- }
Output
3 5 7 9 11
Example 2
- #include<stdio.h>
- Void main ()
- {
- While()
- {
- Printf("hello Javatpoint");
- }
- }
Output
Compile time error: while loop can't be empty
Example 3
- #include<stdio.h>
- Void main ()
- {
- Int x = 10, y = 2;
- While(x+y-1)
- {
- Printf("%d %d",x--,y--);
- }
- }
Output
Infinite loop
Infinitive while loop in C
If the expression passed in while loop results in any non-zero value then the loop will run the infinite number of times.
- While(1){
- //statement
- }
For loop
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
- For(Expression 1; Expression 2; Expression 3){
- //code to be executed
- }
Flowchart of for loop in C
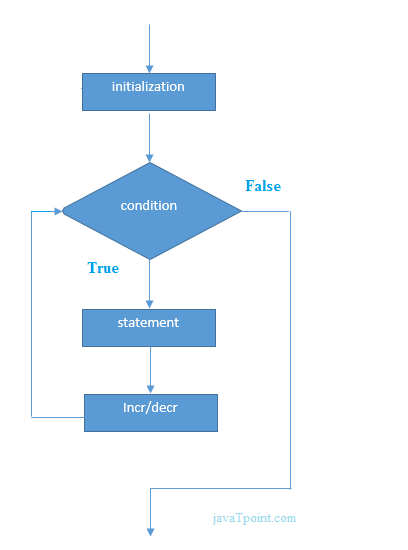
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
- The expression represents the initialization of the loop variable.
- We can initialize more than one variable in Expression 1.
- Expression 1 is optional.
- In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int a,b,c;
- For(a=0,b=12,c=23;a<2;a++)
- {
- Printf("%d ",a+b+c);
- }
- }
Output
35 36
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- For(;i<5;i++)
- {
- Printf("%d ",i);
- }
- }
Output
1 2 3 4
Properties of Expression 2
- Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
- Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
- Expression 2 is optional.
- Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
- We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;i<=4;i++)
- {
- Printf("%d ",i);
- }
- }
Output
0 1 2 3 4
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i,j,k;
- For(i=0,j=0,k=0;i<4,k<8,j<10;i++)
- {
- Printf("%d %d %d\n",i,j,k);
- j+=2;
- k+=3;
- }
- }
Output
0 0 0
1 2 3
2 4 6
3 6 9
4 8 12
Example 3
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;;i++)
- {
- Printf("%d",i);
- }
- }
Output
Infinite loop
Properties of Expression 3
- Expression 3 is used to update the loop variable.
- We can update more than one variable at the same time.
- Expression 3 is optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int i=0,j=2;
- For(i = 0;i<5;i++,j=j+2)
- {
- Printf("%d %d\n",i,j);
- }
- }
Output
0 2
1 4
2 6
3 8
4 10
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Int i;
- For(i=0;i<10;i++)
- {
- Int i = 20;
- Printf("%d ",i);
- }
- }
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
- #include<stdio.h>
- Void main ()
- {
- For(;;)
- {
- Printf("welcome to javatpoint");
- }
- }
If you run this program, you will see above statement infinite times.
Nested Loops in C
C supports nesting of loops in C. Nesting of loops is the feature in C that allows the looping of statements inside another loop. Let's observe an example of nesting loops in C.
Any number of loops can be defined inside another loop, i.e., there is no restriction for defining any number of loops. The nesting level can be defined at n times. You can define any type of loop inside another loop; for example, you can define 'while' loop inside a 'for' loop.
Syntax of Nested loop
- Outer_loop
- {
- Inner_loop
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Outer_loop and Inner_loop are the valid loops that can be a 'for' loop, 'while' loop or 'do-while' loop.
Nested for loop
The nested for loop means any type of loop which is defined inside the 'for' loop.
- For (initialization; condition; update)
- {
- For(initialization; condition; update)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested for loop
- #include <stdio.h>
- Int main()
- {
- Int n;// variable declaration
- Printf("Enter the value of n :");
- // Displaying the n tables.
- For(int i=1;i<=n;i++) // outer loop
- {
- For(int j=1;j<=10;j++) // inner loop
- {
- Printf("%d\t",(i*j)); // printing the value.
- }
- Printf("\n");
- }
Explanation of the above code
- First, the 'i' variable is initialized to 1 and then program control passes to the i<=n.
- The program control checks whether the condition 'i<=n' is true or not.
- If the condition is true, then the program control passes to the inner loop.
- The inner loop will get executed until the condition is true.
- After the execution of the inner loop, the control moves back to the update of the outer loop, i.e., i++.
- After incrementing the value of the loop counter, the condition is checked again, i.e., i<=n.
- If the condition is true, then the inner loop will be executed again.
- This process will continue until the condition of the outer loop is true.
Output:
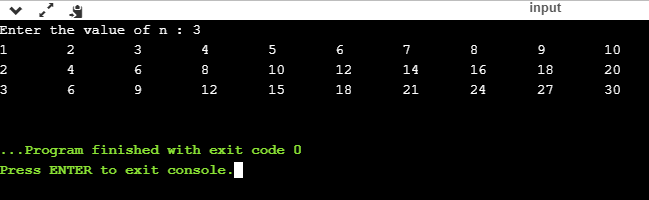
Nested while loop
The nested while loop means any type of loop which is defined inside the 'while' loop.
- While(condition)
- {
- While(condition)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested while loop
- #include <stdio.h>
- Int main()
- {
- Int rows; // variable declaration
- Int columns; // variable declaration
- Int k=1; // variable initialization
- Printf("Enter the number of rows :"); // input the number of rows.
- Scanf("%d",&rows);
- Printf("\nEnter the number of columns :"); // input the number of columns.
- Scanf("%d",&columns);
- Int a[rows][columns]; //2d array declaration
- Int i=1;
- While(i<=rows) // outer loop
- {
- Int j=1;
- While(j<=columns) // inner loop
- {
- Printf("%d\t",k); // printing the value of k.
- k++; // increment counter
- j++;
- }
- i++;
- Printf("\n");
- }
- }
Explanation of the above code.
- We have created the 2d array, i.e., int a[rows][columns].
- The program initializes the 'i' variable by 1.
- Now, control moves to the while loop, and this loop checks whether the condition is true, then the program control moves to the inner loop.
- After the execution of the inner loop, the control moves to the update of the outer loop, i.e., i++.
- After incrementing the value of 'i', the condition (i<=rows) is checked.
- If the condition is true, the control then again moves to the inner loop.
- This process continues until the condition of the outer loop is true.
Output:
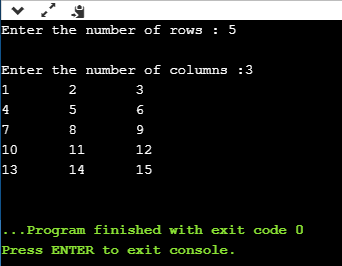
Nested do..while loop
The nested do..while loop means any type of loop which is defined inside the 'do..while' loop.
- Do
- {
- Do
- {
- // inner loop statements.
- }while(condition);
- // outer loop statements.
- }while(condition);
Example of nested do..while loop.
- #include <stdio.h>
- Int main()
- {
- /*printing the pattern
- ********
- ********
- ********
- ******** */
- Int i=1;
- Do // outer loop
- {
- Int j=1;
- Do // inner loop
- {
- Printf("*");
- j++;
- }while(j<=8);
- Printf("\n");
- i++;
- }while(i<=4);
- }
Output:
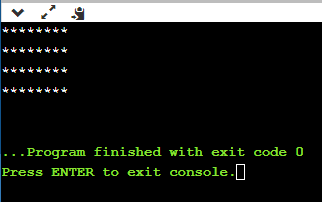
Explanation of the above code.
- First, we initialize the outer loop counter variable, i.e., 'i' by 1.
- As we know that the do..while loop executes once without checking the condition, so the inner loop is executed without checking the condition in the outer loop.
- After the execution of the inner loop, the control moves to the update of the i++.
- When the loop counter value is incremented, the condition is checked. If the condition in the outer loop is true, then the inner loop is executed.
- This process will continue until the condition in the outer loop is true.
Infinite Loop in C
What is infinite loop?
An infinite loop is a looping construct that does not terminate the loop and executes the loop forever. It is also called an indefinite loop or an endless loop. It either produces a continuous output or no output.
When to use an infinite loop
An infinite loop is useful for those applications that accept the user input and generate the output continuously until the user exits from the application manually. In the following situations, this type of loop can be used:
- All the operating systems run in an infinite loop as it does not exist after performing some task. It comes out of an infinite loop only when the user manually shuts down the system.
- All the servers run in an infinite loop as the server responds to all the client requests. It comes out of an indefinite loop only when the administrator shuts down the server manually.
- All the games also run in an infinite loop. The game will accept the user requests until the user exits from the game.
We can create an infinite loop through various loop structures. The following are the loop structures through which we will define the infinite loop:
- For loop
- While loop
- Do-while loop
- Go to statement
- C macros
For loop
Let's see the infinite 'for' loop. The following is the definition for the infinite for loop:
- For(; ;)
- {
- // body of the for loop.
- }
As we know that all the parts of the 'for' loop are optional, and in the above for loop, we have not mentioned any condition; so, this loop will execute infinite times.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- For(;;)
- {
- Printf("Hello javatpoint");
- }
- Return 0;
- }
In the above code, we run the 'for' loop infinite times, so "Hello javatpoint" will be displayed infinitely.
Output
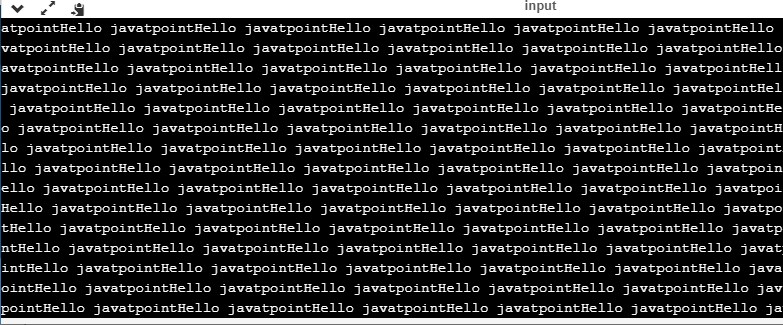
While loop
Now, we will see how to create an infinite loop using a while loop. The following is the definition for the infinite while loop:
- While(1)
- {
- // body of the loop..
- }
In the above while loop, we put '1' inside the loop condition. As we know that any non-zero integer represents the true condition while '0' represents the false condition.
Let's look at a simple example.
- #include <stdio.h>
- Int main()
- {
- Int i=0;
- While(1)
- {
- i++;
- Printf("i is :%d",i);
- }
- Return 0;
- }
In the above code, we have defined a while loop, which runs infinite times as it does not contain any condition. The value of 'i' will be updated an infinite number of times.
Output
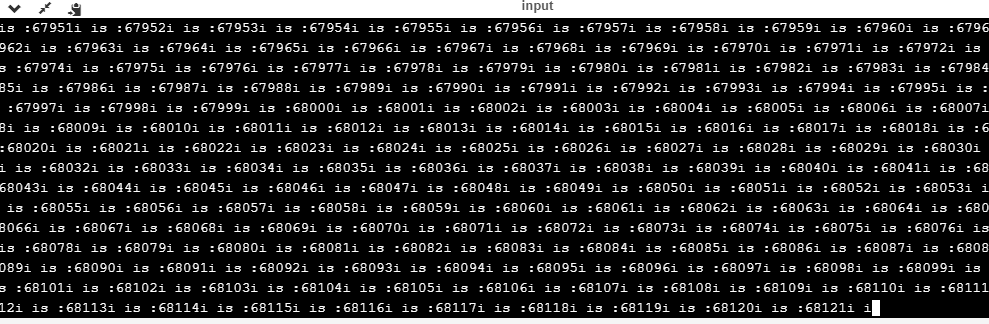
Do..while loop
The do..while loop can also be used to create the infinite loop. The following is the syntax to create the infinite do..while loop.
- Do
- {
- // body of the loop..
- }while(1);
The above do..while loop represents the infinite condition as we provide the '1' value inside the loop condition. As we already know that non-zero integer represents the true condition, so this loop will run infinite times.
Goto statement
We can also use the goto statement to define the infinite loop.
- Infinite_loop;
- // body statements.
- Goto infinite_loop;
In the above code, the goto statement transfers the control to the infinite loop.
Macros
We can also create the infinite loop with the help of a macro constant. Let's understand through an example.
- #include <stdio.h>
- #define infinite for(;;)
- Int main()
- {
- Infinite
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined a macro named as 'infinite', and its value is 'for(;;)'. Whenever the word 'infinite' comes in a program then it will be replaced with a 'for(;;)'.
Output
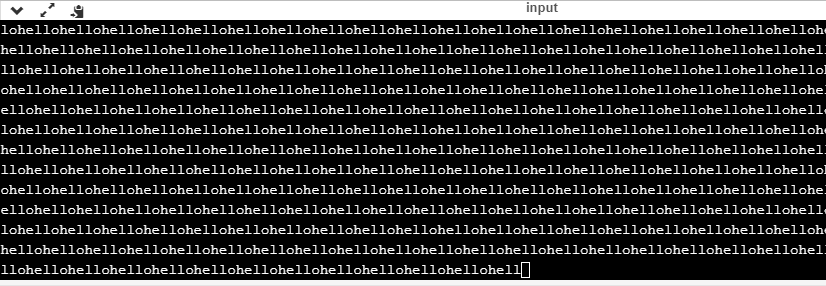
Till now, we have seen various ways to define an infinite loop. However, we need some approach to come out of the infinite loop. In order to come out of the infinite loop, we can use the break statement.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- Char ch;
- While(1)
- {
- Ch=getchar();
- If(ch=='n')
- {
- Break;
- }
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined the while loop, which will execute an infinite number of times until we press the key 'n'. We have added the 'if' statement inside the while loop. The 'if' statement contains the break keyword, and the break keyword brings control out of the loop.
Unintentional infinite loops
Sometimes the situation arises where unintentional infinite loops occur due to the bug in the code. If we are the beginners, then it becomes very difficult to trace them. Below are some measures to trace an unintentional infinite loop:
- We should examine the semicolons carefully. Sometimes we put the semicolon at the wrong place, which leads to the infinite loop.
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- While(i<=10);
- {
- Printf("%d", i);
- i++;
- }
- Return 0;
- }
In the above code, we put the semicolon after the condition of the while loop which leads to the infinite loop. Due to this semicolon, the internal body of the while loop will not execute.
- We should check the logical conditions carefully. Sometimes by mistake, we place the assignment operator (=) instead of a relational operator (= =).
- #include <stdio.h>
- Int main()
- {
- Char ch='n';
- While(ch='y')
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we use the assignment operator (ch='y') which leads to the execution of loop infinite number of times.
- We use the wrong loop condition which causes the loop to be executed indefinitely.
- #include <stdio.h>
- Int main()
- {
- For(int i=1;i>=1;i++)
- {
- Printf("hello");
- }
- Return 0;
- }
The above code will execute the 'for loop' infinite number of times. As we put the condition (i>=1), which will always be true for every condition, it means that "hello" will be printed infinitely.
- We should be careful when we are using the break keyword in the nested loop because it will terminate the execution of the nearest loop, not the entire loop.
- #include <stdio.h>
- Int main()
- {
- While(1)
- {
- For(int i=1;i<=10;i++)
- {
- If(i%2==0)
- {
- Break;
- }
- }
- }
- Return 0;
- }
In the above code, the while loop will be executed an infinite number of times as we use the break keyword in an inner loop. This break keyword will bring the control out of the inner loop, not from the outer loop.
- We should be very careful when we are using the floating-point value inside the loop as we cannot underestimate the floating-point errors.
- #include <stdio.h>
- Int main()
- {
- Float x = 3.0;
- While (x != 4.0) {
- Printf("x = %f\n", x);
- x += 0.1;
- }
- Return 0;
- }
In the above code, the loop will run infinite times as the computer represents a floating-point value as a real value. The computer will represent the value of 4.0 as 3.999999 or 4.000001, so the condition (x !=4.0) will never be false. The solution to this problem is to write the condition as (k<=4.0).
In conditional branching change in sequence of statement execution is depends upon the specified condition. Conditional statements allow programmer to check a condition and execute certain parts of code depending on the result of conditional expression. Conditional expression must be resulted into Boolean value. In C language, there are two forms of conditional statements:
1. If-----else statement: It is used to select one option between two alternatives
2. Switch statement: It is used to select one option between multiple alternative
- If Statements
This statement permits the programmer to allocate condition on the execution of a statement. If the evaluated condition found to be true, the single statement following the "if" is execute. If the condition is found to be false, the following statement is skipped. Syntax of the if statement is as follows
if (condition)
statement1;
statement2;
In the above syntax, “if” is the keyword and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and then statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
if-----else statement
This statement permits the programmer to execute a statement out of the two statements. If the evaluated condition is found to be true, the single statement following the "if" is executed and statement following else is skipped. If the condition is found to be false, statement following the "if" is skipped and statement following else is executed.
In this statement “if” part is compulsory whereas “else” is the optional part. For every “if” statement there may be or may not be “else” statement but for every “else” statement there must be “if” part otherwise compiler will gives “Misplaced else” error.
Syntax of the “if----else” statement is as follows
If (condition)
Statement1;
Else
Statement2;
In the above syntax, “if” and “else” are the keywords and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and skip statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
Nested if-----else statements :
Nested “if-----else” statements are used when programmer wants to check multiple conditions. Nested “if---else” contains several “if---else” a statement out of which only one statement is executed. Number of “if----else” statements is equal to the number of conditions to be checked. Following is the syntax for nested “if---else” statements for three conditions
If (condition1)
Statement1;
Else if (condition2)
Statement2;
Else if (condition3)
Statement3;
Else
Statement4;
In the above syntax, compiler first check condition1, if it trues then it will execute statement1 and skip all the remaining statements. If condition1 is false then compiler directly checks condition2, if it is true then compiler execute statement2 and skip all the remaining statements. If condition2 is also false then compiler directly checks condition3, if it is true then compiler execute statement3 otherwise it will execute statement 4.
Note:
If the test expression is evaluated to true,
• Statements inside the body of if are executed.
• Statements inside the body of else are skipped from execution.
If the test expression is evaluated to false,
• Statements inside the body of else are executed
• Statements inside the body of if are skipped from execution.
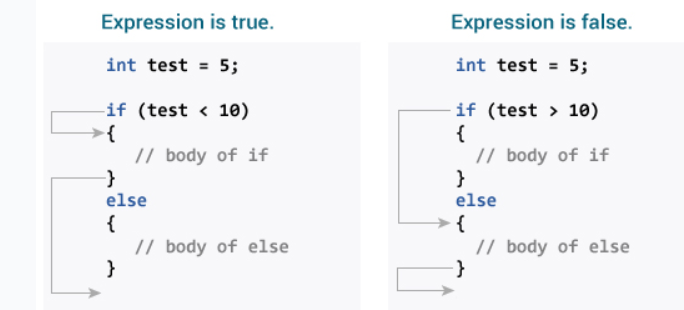
// Check whether an integer is odd or even
#include <stdio.h>
Int main() {
Int number;
Printf("Enter an integer: ");
Scanf("%d", &number);
// True if the remainder is 0
If (number%2 == 0) {
Printf("%d is an even integer.",number);
}
Else {
Printf("%d is an odd integer.",number);
}
Return 0;
}
Program to relate two integers using =, > or < symbol
#include <stdio.h>
Int main() {
Int number1, number2;
Printf("Enter two integers: ");
Scanf("%d %d", &number1, &number2);
//checks if the two integers are equal.
If(number1 == number2) {
Printf("Result: %d = %d",number1,number2);
}
//checks if number1 is greater than number2.
Else if (number1 > number2) {
Printf("Result: %d > %d", number1, number2);
}
//checks if both test expressions are false
Else {
Printf("Result: %d < %d",number1, number2);
}
Return 0;
}
- Switch Statements
This statement permits the programmer to choose one option out of several options depending on one condition. When the “switch” statement is executed, the expression in the switch statement is evaluated and the control is transferred directly to the group of statements whose “case” label value matches with the value of the expression. Syntax for switch statement is as follows:
Switch(expression)
{
Case constant1:
Statements 1;
Break;
Case constant2:
Statements 2;
Break;
…………………..
Default:
Statements n;
Break;
}
In the above, “switch”, “case”, “break” and “default” are keywords. Out of which “switch” and “case” are the compulsory keywords whereas “break” and “default” is optional keywords.
- “switch” keyword is used to start switch statement with conditional expression.
- “case” is the compulsory keyword which labeled with a constant value. If the value of expression matches with the case value then statement followed by “case” statement is executed.
- “break” is the optional keyword in switch statement. The execution of “break” statement causes the transfer of flow of execution outside the “switch” statements scope. Absence of “break” statement causes the execution of all the following “case” statements without concerning value of the expression.
- “default” is the optional keyword in “switch” statement. When the value of expression is not match with the any of the “case” statement then the statement following “default” keyword is executed.
Example: Program to spell user entered single digit number in English is as follows
#include<stdio.h>
#include<conio.h>
Void main()
{
Int n;
Clrscr();
Printf("Enter a number");
Scanf("%d",&n);
Switch(n)
{
Case 0: printf("\n Zero");
Break;
Case 1: printf("\n One");
Break;
Case 2: printf("\n Two");
Break;
Case 3: printf("\n Three");
Break;
Case 4: printf("\n Four");
Break;
Case 5: printf("\n Five");
Break;
Case 6: printf("\n Six");
Break;
Case 7: printf("\n Seven");
Break;
Case 8: printf("\n Eight");
Break;
Case 9: printf("\n Nine");
Break;
Default: printf("Given number is not single digit number");
}
Getch();
}
Output
Enter a number
5
Five
Loops are a form of iteration (recursion being the other form). Proper use of recursion depends on viewing the problem in a certain way to extract the recursion. Loop-based iteration is no different. They all come down to the same process.
- View the task as a sequence identical or nearly identical sub-tasks
- Figure out what changes each iteration (if anything)
- Express that change in programming terms
- Figure out when the program should stop performing the task
- Express that end condition as a conditional that asks - under what condition should the task continue repeating (not when should it stop)
While Loops
The while loop is the universal loop - all loops can be constructed using while loops. All other loops are created for specific circumstances.
While( condition ) BB; | ![]() |
Int i = 0; While( i < 5 ) { Printf( "i = %d\n", i); i++; } Printf( "After loop, i = %d\n", i ); | ![]() |
How it works:
The condition is checked prior to executing the body every time. It will only exit once the condition evaluates to false. Make sure you don't forget to have something change so that it eventually terminates the loop.
When to use this construct: This is appropriate for any loop - unless your loop follows the special circumstances for the loops below. In general, this is for loops for which the exit condition is not simply a counter that increments (as you see above). Instead, it's perfect for loops such as menus asking for user input.
Do-while
If you know you want to run the body of a loop at least once, then a do-while loop is appropriate.
Do{ BB; } while( condition ) | ![]() |
Int stop = 0; Do { Stop = Menu(); } while (!stop); | ![]() |
How it works:
The BB will run once no matter what, then the condition will be evaluated. Once that BB is run once, it is equivalent to the while loop.
When to use this construct: When you want the body to run at least once.
For
The for loop was created based on a common mistake when implementing loops - forgetting the update step. That is, forgetting to increment your counter or some such action. If you forget the action that gets you closer to loop termination, then your loop will not terminate.
The for loop is designed so that
- Programmers will remember the lines that are crucial to loop termination
- Others reading the loop will be able to quickly read what controls the loop
For (init; condition; update) { BB; } | ![]() |
Int i; For( i = 0; i < 5; i++ ) Printf( "i = %d\n", i ); Printf( "After loop\n"); | ![]() |
How it works:
The init code runs before the loop. Then it evaluates the condition. Next, it runs the loop body. It finishes with the update step, looping back to the condition again. The most common mistake in terms of understanding is forgetting that the condition is evaluated prior to the first loop iteration.
When to use this construct:
When your loop control is very simple and can be expressed easily inside the for loop syntax.
The comma operator
- C has a comma operator, that basically combines two statements so that they can be considered as a single statement.
- About the only place this is ever used is in for loops, to either provide multiple initializations or to allow for multiple incrementations.
- For example:
Int i, j = 10, sum;
For( i = 0, sum = 0; i < 5; i++, j-- )
Sum += i * j;
Break and continue
- Break and continue are two C/C++ statements that allow us to further control flow within and out of loops.
- Break causes execution to immediately jump out of the current loop, and proceed with the code following the loop.
- Continue causes the remainder of the current iteration of the loop to be skipped, and for execution to recommence with the next iteration.
- In the case of for loops, the incrementation step will be executed next, followed by the condition test to start the next loop iteration.
- In the case of while and do-while loops, execution jumps to the next loop condition test.
Suggested Text Books
Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill
E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
Suggested Reference Books
Brian W. Kernighan and Dennis M. Ritchie, The C Programming Language, Prentice Hall of India
UNIT- 2
Arithmetic expressions and precedence
Arithmetic Expressions consist of numeric literals, arithmetic operators, and numeric variables. They simplify to a single value, when evaluated.
Here is an example of an arithmetic expression with no variables:
3.14*10*10
This expression evaluates to 314, the approximate area of a circle with radius 10. Similarly, the expression
3.14*radius*radius
Would also evaluate to 314, if the variable radius stored the value 10.
Note that the parentheses in the last expression helps dictate which order to evaluate the expression.
Multiplication and division have a higher order of precedence than addition and subtraction. In an arithmetic expression, it is evaluated left to right, only performing the multiplications and divisions.
After doing this, process the expression again from left to right, doing all the additions and subtractions.
So,
3+4*5
First evaluates to
3+20 which then evaluates to 23.
Consider this expression:
3 + 4*5 - 6/3*4/8 + 2*6 - 4*3*2
First go through and do all the multiplications and divisions:
3 + 20 - 1 + 12 - 24
Integer Division: In particular, if the division has a leftover remainder or fraction, this is simply discarded.
For example:
13/4 evaluates to 3
19/3 evaluates to 6 but
Similarly, if you have an expression with integer variables part of a division, this evaluates to an integer as well.
For example, in this segment of code, y gets set to 2.
Int x = 8;
Int y = x/3;
However, if we did the following,
Double x = 8;
Double y = x/3;
y would equal 2.66666666 (approximately).
The way C decides whether it will do an integer division (as in the first example), or a real number division (as in the second example), is based on the TYPE of the operands.
If both operands are ints, an integer division is done.
If either operand is a float or a double, then a real division is done. The compiler will treat constants without the decimal point as integers, and constants with the decimal point as a float. Thus, the expressions 13/4 and 13/4.0 will evaluate to 3 and 3.25 respectively.
The mod operator (%)
The one new operator is the mod operator, which is denoted by the percent sign(%). This operator is ONLY defined for integer operands. It is defined as follows:
a%b evaluates to the remainder of a divided by b.
For example,
12%5 = 2
19%6 = 1
14%7 = 0
19%200 = 19
The precedence of the mod operator is the same as the precedence of multiplication and division.
Every expression in C language contains set of operators and operands. Operators are the special symbols which are used to indicate the type of operation whereas operands are the data member or variables operating as per operation specified by operator. One expression contains one or more set of operators and operands. So to avoid the ambiguity in execution of expression, C compiler fixed the precedence of operator. Depending on the operation, operators are classified into following category.
- Arithmetic Operator: These are the operators which are useful in performing mathematical calculations. Following are the list of arithmetic operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
+ | Used to add two operands | Result of A+B is 15 | |
2. | - | Used to subtract two operands | Result of A-B is 5 |
3. | * | Used to multiply two operands | Result of A*B is 50 |
4. | / | Used to divide two operands | Result of A/B is 2 |
5. | % | Find reminder of the division of two operand | Result of A%B is 0 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of addition of %d and %d is %d",a,b,a+b);
Printf("\n Result of subtraction of %d and %d is %d",a,b,a-b);
Printf("\n Result of multiplication %d and %d is %d",a,b,a*b);
Printf("\n Result of division of %d and %d is %d",a,b,a/b);
Printf("\n Result of modulus of %d and %d is %d",a,b,a%b);
Getch();
}
Output:
Enter two numbers
5
3
Result of addition of 5 and 3 is 8
Result of subtraction of 5 and 3 is 2
Result of multiplication of 5 and 3 is 15
Result of division of 5 and 3 is 1
Result of modulus of 5 and 3 is 2
2. Relational Operators: Relational operator used to compare two operands. Relational operator produce result in terms of binary values. It returns 1 when the result is true and 0 when result is false. Following are the list of relational operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | < | This is less than operator which is used to check whether the value of left operand is less than the value of right operand or not | Result of A<B is false
|
2. | > | This is greater than operator which is used to check whether the value of left operand is greater than the value of right operand or not | Result of A>B is true |
3. | <= | This is less than or equal to operator | Result of A<=B is false |
4. | >= | This is greater than or equal to operator | Result of A>=B is true |
5. | == | This is equal to operator which is used to check value of both operands are equal or not | Result of A==B is false |
6. | != | This is not equal to operator which is used to check value of both operands are equal or not | Result of A!=B is true |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of less than operator of %d and %d is %d",a,b,a<b);
Printf("\n Result of greater than operator of %d and %d is %d",a,b,a>b);
Printf("\n Result of leass than or equal to operator %d and %d is %d",a,b,a<=b);
Printf("\n Result of greater than or equal to operator of %d and %d is %d", a,b,a>=b);
Printf("\n Result of double equal to operator of %d and %d is %d",a,b,a==b);
Printf("\n Result of not equal to operator of %d and %d is %d",a,b,a!=b);
Getch();
}
Output:
Enter two numbers
5
3
Result of less than operator of 5 and 3 is 0
Result of greater than operator of 5 and 3 is 1
Result of less than or equal to operator of 5 and 3 is 0
Result of greater than or equal to operator of 5 and 3 is 1
Result of double equal to operator of 5 and 3 is 0
Result of not equal to operator of 5 and 3 is 1
Precedence and Associativity
Operator precedence determines the grouping of terms in an expression and decides how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has a higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has a higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | <<>> | Left to right |
Relational | <<= >>= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
Example Code
#include <stdio.h>
Main() {
Int a = 20;
Int b = 10;
Int c = 15;
Int d = 5;
Int e;
e = (a + b) * c / d; // ( 30 * 15 ) / 5
Printf("Value of (a + b) * c / d is : %d\n", e );
e = ((a + b) * c) / d; // (30 * 15 ) / 5
Printf("Value of ((a + b) * c) / d is : %d\n" , e );
e = (a + b) * (c / d); // (30) * (15/5)
Printf("Value of (a + b) * (c / d) is : %d\n", e );
e = a + (b * c) / d; // 20 + (150/5)
Printf("Value of a + (b * c) / d is : %d\n" , e );
Return 0;
}
Output
Value of (a + b) * c / d is : 90
Value of ((a + b) * c) / d is : 90
Value of (a + b) * (c / d) is : 90
Value of a + (b * c) / d is : 50
Conditional Branching
If else Statement
The if-else statement in C is used to perform the operations based on some specific condition. The operations specified in if block are executed if and only if the given condition is true.
There are the following variants of if statement in C language.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
- If(expression){
- //code to be executed
- }
Flowchart of if statement in C
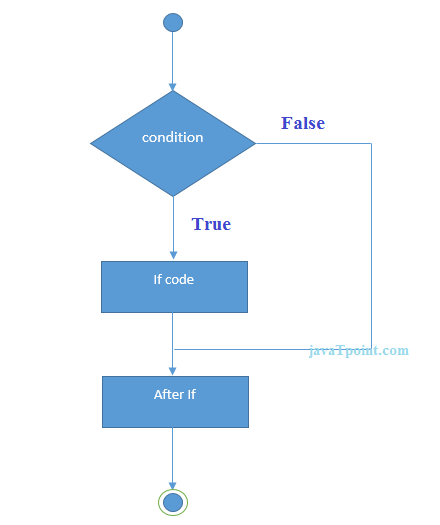
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 is largest
If-else Statement
The if-else statement is used to perform two operations for a single condition. The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition. Here, we must notice that if and else block cannot be executed simiulteneously. Using if-else statement is always preferable since it always invokes an otherwise case with every if condition. The syntax of the if-else statement is given below.
- If(expression){
- //code to be executed if condition is true
- }else{
- //code to be executed if condition is false
- }
Flowchart of the if-else statement in C
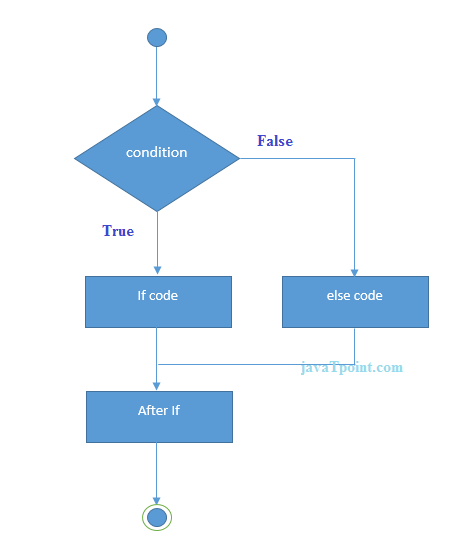
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Else{
- Printf("%d is odd number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
- #include <stdio.h>
- Int main()
- {
- Int age;
- Printf("Enter your age?");
- Scanf("%d",&age);
- If(age>=18)
- {
- Printf("You are eligible to vote...");
- }
- Else
- {
- Printf("Sorry ... you can't vote");
- }
- }
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... You can't vote
If else-if ladder Statement
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
- If(condition1){
- //code to be executed if condition1 is true
- }else if(condition2){
- //code to be executed if condition2 is true
- }
- Else if(condition3){
- //code to be executed if condition3 is true
- }
- ...
- Else{
- //code to be executed if all the conditions are false
- }
Flowchart of else-if ladder statement in C
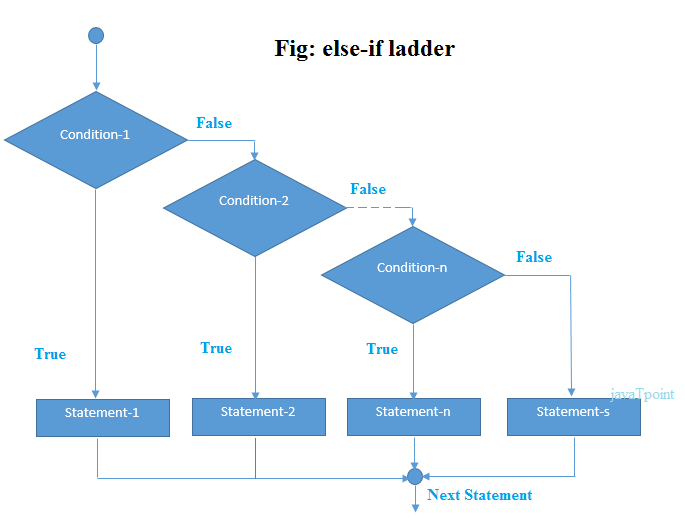
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats ! you scored grade A ...
Switch Statement
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement is given below:
- Switch(expression){
- Case value1:
- //code to be executed;
- Break; //optional
- Case value2:
- //code to be executed;
- Break; //optional
- ......
- Default:
- Code to be executed if all cases are not matched;
- }
Rules for switch statement in C language
1) The switch expression must be of an integer or character type.
2) The case value must be an integer or character constant.
3) The case value can be used only inside the switch statement.
4) The break statement in switch case is not must. It is optional. If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
Let's try to understand it by the examples. We are assuming that there are following variables.
- Int x,y,z;
- Char a,b;
- Float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
Switch(x) | Switch(f) | Case 3; | Case 2.5; |
Switch(x>y) | Switch(x+2.5) | Case 'a'; | Case x; |
Switch(a+b-2) |
| Case 1+2; | Case x+2; |
Switch(func(x,y)) |
| Case 'x'>'y'; | Case 1,2,3; |
Flowchart of switch statement in C
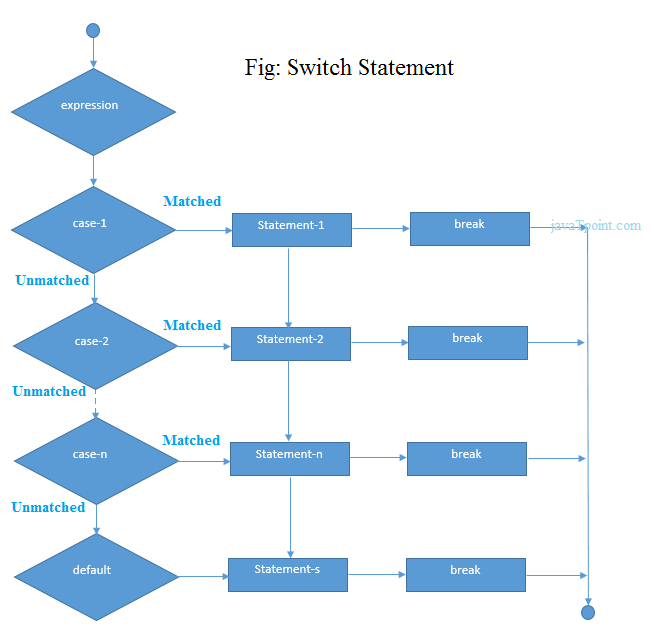
Functioning of switch case statement
First, the integer expression specified in the switch statement is evaluated. This value is then matched one by one with the constant values given in the different cases. If a match is found, then all the statements specified in that case are executed along with the all the cases present after that case including the default statement. No two cases can have similar values. If the matched case contains a break statement, then all the cases present after that will be skipped, and the control comes out of the switch. Otherwise, all the cases following the matched case will be executed.
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example 2
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
C Switch statement is fall-through
In C language, the switch statement is fall through; it means if you don't use a break statement in the switch case, all the cases after the matching case will be executed.
Let's try to understand the fall through state of switch statement by the example given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equal to 10\n");
- Case 50:
- Printf("number is equal to 50\n");
- Case 100:
- Printf("number is equal to 100\n");
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:10
Number is equal to 10
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Output
Enter a number:50
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Nested switch case statement
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
- #include <stdio.h>
- Int main () {
- Int i = 10;
- Int j = 20;
- Switch(i) {
- Case 10:
- Printf("the value of i evaluated in outer switch: %d\n",i);
- Case 20:
- Switch(j) {
- Case 20:
- Printf("The value of j evaluated in nested switch: %d\n",j);
- }
- }
- Printf("Exact value of i is : %d\n", i );
- Printf("Exact value of j is : %d\n", j );
- Return 0;
- }
Output
The value of i evaluated in outer switch: 10
The value of j evaluated in nested switch: 20
Exact value of i is : 10
Exact value of j is : 20
If-else vs switch
What is an if-else statement?
An if-else statement in C programming is a conditional statement that executes a different set of statements based on the condition that is true or false. The 'if' block will be executed only when the specified condition is true, and if the specified condition is false, then the else block will be executed.
Syntax of if-else statement is given below:
- If(expression)
- {
- // statements;
- }
- Else
- {
- // statements;
- }
What is a switch statement?
A switch statement is a conditional statement used in C programming to check the value of a variable and compare it with all the cases. If the value is matched with any case, then its corresponding statements will be executed. Each case has some name or number known as the identifier. The value entered by the user will be compared with all the cases until the case is found. If the value entered by the user is not matched with any case, then the default statement will be executed.
Syntax of the switch statement is given below:
- Switch(expression)
- {
- Case constant 1:
- // statements;
- Break;
- Case constant 2:
- // statements;
- Break;
- Case constant n:
- // statements;
- Break;
- Default:
- // statements;
- }
Similarity b/w if-else and switch
Both the if-else and switch are the decision-making statements. Here, decision-making statements mean that the output of the expression will decide which statements are to be executed.
Differences b/w if-else and switch statement
The following are the differences between if-else and switch statement are:
- Definition
If-else
Based on the result of the expression in the 'if-else' statement, the block of statements will be executed. If the condition is true, then the 'if' block will be executed otherwise 'else' block will execute.
Switch statement
The switch statement contains multiple cases or choices. The user will decide the case, which is to execute.
- Expression
If-else
It can contain a single expression or multiple expressions for multiple choices. In this, an expression is evaluated based on the range of values or conditions. It checks both equality and logical expressions.
Switch
It contains only a single expression, and this expression is either a single integer object or a string object. It checks only equality expression.
- Evaluation
If-else
An if-else statement can evaluate almost all the types of data such as integer, floating-point, character, pointer, or Boolean.
Switch
A switch statement can evaluate either an integer or a character.
- Sequence of Execution
If-else
In the case of 'if-else' statement, either the 'if' block or the 'else' block will be executed based on the condition.
Switch
In the case of the 'switch' statement, one case after another will be executed until the break keyword is not found, or the default statement is executed.
- Default Execution
If-else
If the condition is not true within the 'if' statement, then by default, the else block statements will be executed.
Switch
If the expression specified within the switch statement is not matched with any of the cases, then the default statement, if defined, will be executed.
- Values
If-else
Values are based on the condition specified inside the 'if' statement. The value will decide either the 'if' or 'else' block is to be executed.
Switch
In this case, value is decided by the user. Based on the choice of the user, the case will be executed.
- Use
If-else
It evaluates a condition to be true or false.
Switch
A switch statement compares the value of the variable with multiple cases. If the value is matched with any of the cases, then the block of statements associated with this case will be executed.
- Editing
If-else
Editing in 'if-else' statement is not easy as if we remove the 'else' statement, then it will create the havoc.
Switch
Editing in switch statement is easier as compared to the 'if-else' statement. If we remove any of the cases from the switch, then it will not interrupt the execution of other cases. Therefore, we can say that the switch statement is easy to modify and maintain.
- Speed
If-else
If the choices are multiple, then the speed of the execution of 'if-else' statements is slow.
Switch
The case constants in the switch statement create a jump table at the compile time. This jump table chooses the path of the execution based on the value of the expression. If we have a multiple choice, then the execution of the switch statement will be much faster than the equivalent logic of 'if-else' statement.
Let's summarize the above differences in a tabular form.
| If-else | Switch |
Definition | Depending on the condition in the 'if' statement, 'if' and 'else' blocks are executed. | The user will decide which statement is to be executed. |
Expression | It contains either logical or equality expression. | It contains a single expression which can be either a character or integer variable. |
Evaluation | It evaluates all types of data, such as integer, floating-point, character or Boolean. | It evaluates either an integer, or character. |
Sequence of execution | First, the condition is checked. If the condition is true then 'if' block is executed otherwise 'else' block | It executes one case after another till the break keyword is not found, or the default statement is executed. |
Default execution | If the condition is not true, then by default, else block will be executed. | If the value does not match with any case, then by default, default statement is executed. |
Editing | Editing is not easy in the 'if-else' statement. | Cases in a switch statement are easy to maintain and modify. Therefore, we can say that the removal or editing of any case will not interrupt the execution of other cases. |
Speed | If there are multiple choices implemented through 'if-else', then the speed of the execution will be slow. | If we have multiple choices then the switch statement is the best option as the speed of the execution will be much higher than 'if-else'. |
Loops
The looping can be defined as repeating the same process multiple times until a specific condition satisfies. There are three types of loops used in the C language. In this part of the tutorial, we are going to learn all the aspects of C loops.
Why use loops in C language?
The looping simplifies the complex problems into the easy ones. It enables us to alter the flow of the program so that instead of writing the same code again and again, we can repeat the same code for a finite number of times. For example, if we need to print the first 10 natural numbers then, instead of using the printf statement 10 times, we can print inside a loop which runs up to 10 iterations.
Advantage of loops in C
1) It provides code reusability.
2) Using loops, we do not need to write the same code again and again.
3) Using loops, we can traverse over the elements of data structures (array or linked lists).
Types of C Loops
There are three types of loops in C language that is given below:
- Do while
- While
- For
Do-while loop in C
The do-while loop continues until a given condition satisfies. It is also called post tested loop. It is used when it is necessary to execute the loop at least once (mostly menu driven programs).
The syntax of do-while loop in c language is given below:
- Do{
- //code to be executed
- }while(condition);
While loop in C
The while loop in c is to be used in the scenario where we don't know the number of iterations in advance. The block of statements is executed in the while loop until the condition specified in the while loop is satisfied. It is also called a pre-tested loop.
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
For loop in C
The for loop is used in the case where we need to execute some part of the code until the given condition is satisfied. The for loop is also called as a per-tested loop. It is better to use for loop if the number of iteration is known in advance.
The syntax of for loop in c language is given below:
- For(initialization;condition;incr/decr){
- //code to be executed
- }
Do while loop
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
Do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example 1
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Flowchart of do while loop
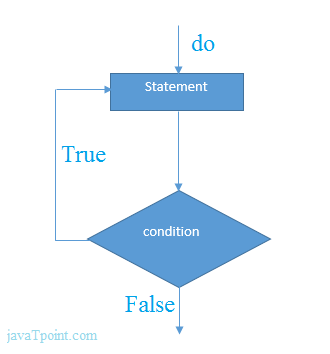
do while example
There is given the simple program of c language do while loop where we are printing the table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- Do{
- Printf("%d \n",i);
- i++;
- }while(i<=10);
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loop
The do-while loop will run infinite times if we pass any non-zero value as the conditional expression.
- Do{
- //statement
- }while(1);
While loop
While loop is also known as a pre-tested loop. In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition. It can be viewed as a repeating if statement. The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax of while loop in C language
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
Flowchart of while loop in C
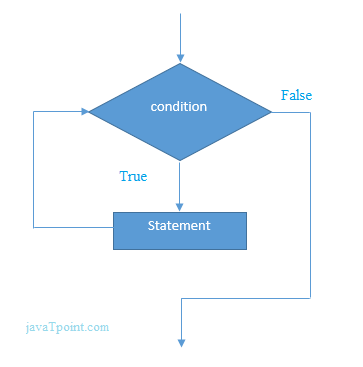
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- While(i<=10){
- Printf("%d \n",i);
- i++;
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
- #include<stdio.h>
- Int main(){
- Int i=1,number=0,b=9;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- While(i<=10){
- Printf("%d \n",(number*i));
- i++;
- }
- Return 0;
- }
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
Properties of while loop
- A conditional expression is used to check the condition. The statements defined inside the while loop will repeatedly execute until the given condition fails.
- The condition will be true if it returns 0. The condition will be false if it returns any non-zero number.
- In while loop, the condition expression is compulsory.
- Running a while loop without a body is possible.
- We can have more than one conditional expression in while loop.
- If the loop body contains only one statement, then the braces are optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int j = 1;
- While(j+=2,j<=10)
- {
- Printf("%d ",j);
- }
- Printf("%d",j);
- }
Output
3 5 7 9 11
Example 2
- #include<stdio.h>
- Void main ()
- {
- While()
- {
- Printf("hello Javatpoint");
- }
- }
Output
Compile time error: while loop can't be empty
Example 3
- #include<stdio.h>
- Void main ()
- {
- Int x = 10, y = 2;
- While(x+y-1)
- {
- Printf("%d %d",x--,y--);
- }
- }
Output
Infinite loop
Infinitive while loop in C
If the expression passed in while loop results in any non-zero value then the loop will run the infinite number of times.
- While(1){
- //statement
- }
For loop
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
- For(Expression 1; Expression 2; Expression 3){
- //code to be executed
- }
Flowchart of for loop in C
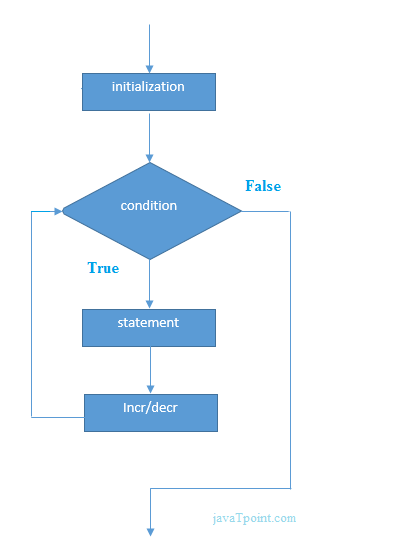
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
- The expression represents the initialization of the loop variable.
- We can initialize more than one variable in Expression 1.
- Expression 1 is optional.
- In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int a,b,c;
- For(a=0,b=12,c=23;a<2;a++)
- {
- Printf("%d ",a+b+c);
- }
- }
Output
35 36
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- For(;i<5;i++)
- {
- Printf("%d ",i);
- }
- }
Output
1 2 3 4
Properties of Expression 2
- Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
- Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
- Expression 2 is optional.
- Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
- We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;i<=4;i++)
- {
- Printf("%d ",i);
- }
- }
Output
0 1 2 3 4
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i,j,k;
- For(i=0,j=0,k=0;i<4,k<8,j<10;i++)
- {
- Printf("%d %d %d\n",i,j,k);
- j+=2;
- k+=3;
- }
- }
Output
0 0 0
1 2 3
2 4 6
3 6 9
4 8 12
Example 3
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;;i++)
- {
- Printf("%d",i);
- }
- }
Output
Infinite loop
Properties of Expression 3
- Expression 3 is used to update the loop variable.
- We can update more than one variable at the same time.
- Expression 3 is optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int i=0,j=2;
- For(i = 0;i<5;i++,j=j+2)
- {
- Printf("%d %d\n",i,j);
- }
- }
Output
0 2
1 4
2 6
3 8
4 10
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Int i;
- For(i=0;i<10;i++)
- {
- Int i = 20;
- Printf("%d ",i);
- }
- }
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
- #include<stdio.h>
- Void main ()
- {
- For(;;)
- {
- Printf("welcome to javatpoint");
- }
- }
If you run this program, you will see above statement infinite times.
Nested Loops in C
C supports nesting of loops in C. Nesting of loops is the feature in C that allows the looping of statements inside another loop. Let's observe an example of nesting loops in C.
Any number of loops can be defined inside another loop, i.e., there is no restriction for defining any number of loops. The nesting level can be defined at n times. You can define any type of loop inside another loop; for example, you can define 'while' loop inside a 'for' loop.
Syntax of Nested loop
- Outer_loop
- {
- Inner_loop
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Outer_loop and Inner_loop are the valid loops that can be a 'for' loop, 'while' loop or 'do-while' loop.
Nested for loop
The nested for loop means any type of loop which is defined inside the 'for' loop.
- For (initialization; condition; update)
- {
- For(initialization; condition; update)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested for loop
- #include <stdio.h>
- Int main()
- {
- Int n;// variable declaration
- Printf("Enter the value of n :");
- // Displaying the n tables.
- For(int i=1;i<=n;i++) // outer loop
- {
- For(int j=1;j<=10;j++) // inner loop
- {
- Printf("%d\t",(i*j)); // printing the value.
- }
- Printf("\n");
- }
Explanation of the above code
- First, the 'i' variable is initialized to 1 and then program control passes to the i<=n.
- The program control checks whether the condition 'i<=n' is true or not.
- If the condition is true, then the program control passes to the inner loop.
- The inner loop will get executed until the condition is true.
- After the execution of the inner loop, the control moves back to the update of the outer loop, i.e., i++.
- After incrementing the value of the loop counter, the condition is checked again, i.e., i<=n.
- If the condition is true, then the inner loop will be executed again.
- This process will continue until the condition of the outer loop is true.
Output:
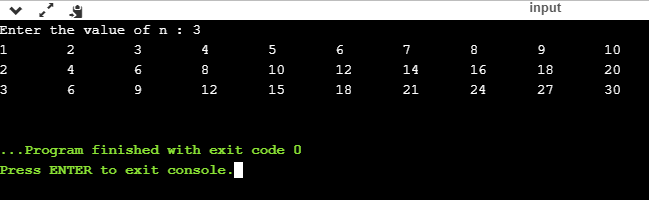
Nested while loop
The nested while loop means any type of loop which is defined inside the 'while' loop.
- While(condition)
- {
- While(condition)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested while loop
- #include <stdio.h>
- Int main()
- {
- Int rows; // variable declaration
- Int columns; // variable declaration
- Int k=1; // variable initialization
- Printf("Enter the number of rows :"); // input the number of rows.
- Scanf("%d",&rows);
- Printf("\nEnter the number of columns :"); // input the number of columns.
- Scanf("%d",&columns);
- Int a[rows][columns]; //2d array declaration
- Int i=1;
- While(i<=rows) // outer loop
- {
- Int j=1;
- While(j<=columns) // inner loop
- {
- Printf("%d\t",k); // printing the value of k.
- k++; // increment counter
- j++;
- }
- i++;
- Printf("\n");
- }
- }
Explanation of the above code.
- We have created the 2d array, i.e., int a[rows][columns].
- The program initializes the 'i' variable by 1.
- Now, control moves to the while loop, and this loop checks whether the condition is true, then the program control moves to the inner loop.
- After the execution of the inner loop, the control moves to the update of the outer loop, i.e., i++.
- After incrementing the value of 'i', the condition (i<=rows) is checked.
- If the condition is true, the control then again moves to the inner loop.
- This process continues until the condition of the outer loop is true.
Output:
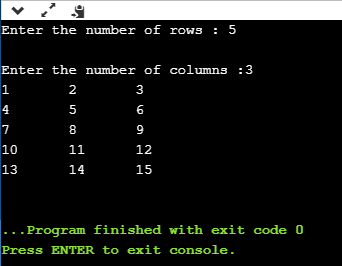
Nested do..while loop
The nested do..while loop means any type of loop which is defined inside the 'do..while' loop.
- Do
- {
- Do
- {
- // inner loop statements.
- }while(condition);
- // outer loop statements.
- }while(condition);
Example of nested do..while loop.
- #include <stdio.h>
- Int main()
- {
- /*printing the pattern
- ********
- ********
- ********
- ******** */
- Int i=1;
- Do // outer loop
- {
- Int j=1;
- Do // inner loop
- {
- Printf("*");
- j++;
- }while(j<=8);
- Printf("\n");
- i++;
- }while(i<=4);
- }
Output:
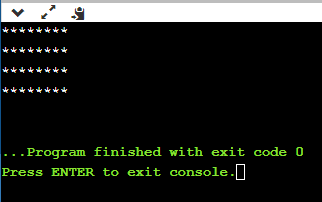
Explanation of the above code.
- First, we initialize the outer loop counter variable, i.e., 'i' by 1.
- As we know that the do..while loop executes once without checking the condition, so the inner loop is executed without checking the condition in the outer loop.
- After the execution of the inner loop, the control moves to the update of the i++.
- When the loop counter value is incremented, the condition is checked. If the condition in the outer loop is true, then the inner loop is executed.
- This process will continue until the condition in the outer loop is true.
Infinite Loop in C
What is infinite loop?
An infinite loop is a looping construct that does not terminate the loop and executes the loop forever. It is also called an indefinite loop or an endless loop. It either produces a continuous output or no output.
When to use an infinite loop
An infinite loop is useful for those applications that accept the user input and generate the output continuously until the user exits from the application manually. In the following situations, this type of loop can be used:
- All the operating systems run in an infinite loop as it does not exist after performing some task. It comes out of an infinite loop only when the user manually shuts down the system.
- All the servers run in an infinite loop as the server responds to all the client requests. It comes out of an indefinite loop only when the administrator shuts down the server manually.
- All the games also run in an infinite loop. The game will accept the user requests until the user exits from the game.
We can create an infinite loop through various loop structures. The following are the loop structures through which we will define the infinite loop:
- For loop
- While loop
- Do-while loop
- Go to statement
- C macros
For loop
Let's see the infinite 'for' loop. The following is the definition for the infinite for loop:
- For(; ;)
- {
- // body of the for loop.
- }
As we know that all the parts of the 'for' loop are optional, and in the above for loop, we have not mentioned any condition; so, this loop will execute infinite times.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- For(;;)
- {
- Printf("Hello javatpoint");
- }
- Return 0;
- }
In the above code, we run the 'for' loop infinite times, so "Hello javatpoint" will be displayed infinitely.
Output
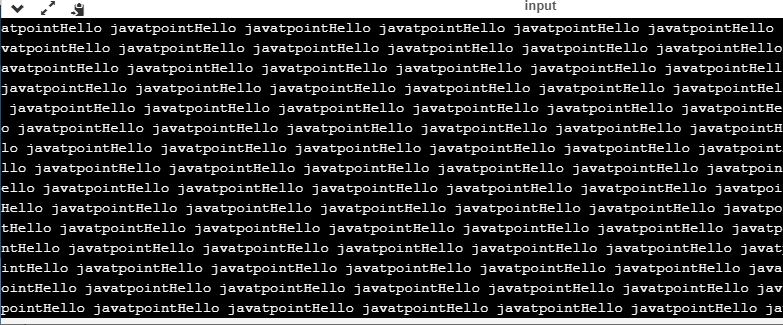
While loop
Now, we will see how to create an infinite loop using a while loop. The following is the definition for the infinite while loop:
- While(1)
- {
- // body of the loop..
- }
In the above while loop, we put '1' inside the loop condition. As we know that any non-zero integer represents the true condition while '0' represents the false condition.
Let's look at a simple example.
- #include <stdio.h>
- Int main()
- {
- Int i=0;
- While(1)
- {
- i++;
- Printf("i is :%d",i);
- }
- Return 0;
- }
In the above code, we have defined a while loop, which runs infinite times as it does not contain any condition. The value of 'i' will be updated an infinite number of times.
Output
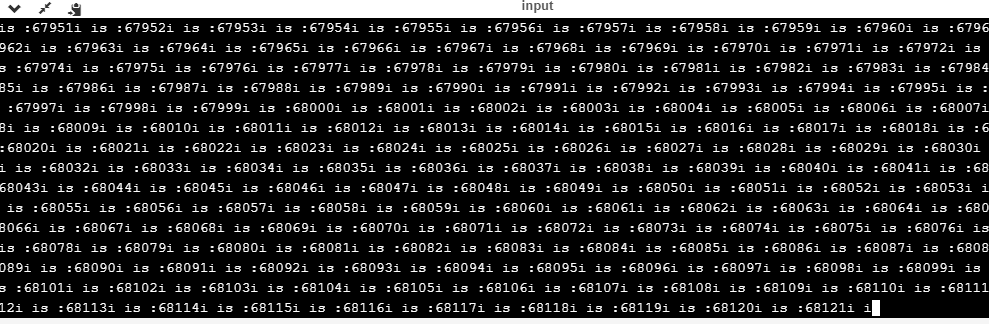
Do..while loop
The do..while loop can also be used to create the infinite loop. The following is the syntax to create the infinite do..while loop.
- Do
- {
- // body of the loop..
- }while(1);
The above do..while loop represents the infinite condition as we provide the '1' value inside the loop condition. As we already know that non-zero integer represents the true condition, so this loop will run infinite times.
Goto statement
We can also use the goto statement to define the infinite loop.
- Infinite_loop;
- // body statements.
- Goto infinite_loop;
In the above code, the goto statement transfers the control to the infinite loop.
Macros
We can also create the infinite loop with the help of a macro constant. Let's understand through an example.
- #include <stdio.h>
- #define infinite for(;;)
- Int main()
- {
- Infinite
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined a macro named as 'infinite', and its value is 'for(;;)'. Whenever the word 'infinite' comes in a program then it will be replaced with a 'for(;;)'.
Output
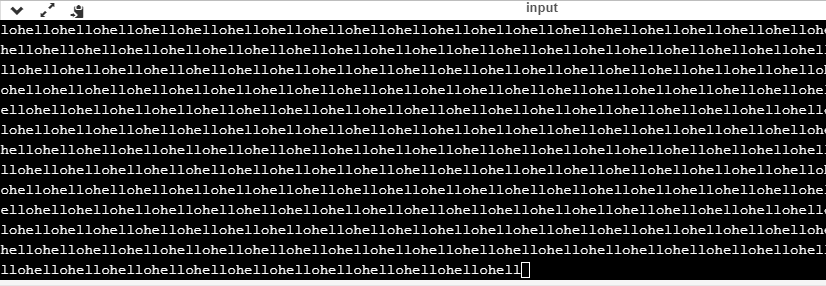
Till now, we have seen various ways to define an infinite loop. However, we need some approach to come out of the infinite loop. In order to come out of the infinite loop, we can use the break statement.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- Char ch;
- While(1)
- {
- Ch=getchar();
- If(ch=='n')
- {
- Break;
- }
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined the while loop, which will execute an infinite number of times until we press the key 'n'. We have added the 'if' statement inside the while loop. The 'if' statement contains the break keyword, and the break keyword brings control out of the loop.
Unintentional infinite loops
Sometimes the situation arises where unintentional infinite loops occur due to the bug in the code. If we are the beginners, then it becomes very difficult to trace them. Below are some measures to trace an unintentional infinite loop:
- We should examine the semicolons carefully. Sometimes we put the semicolon at the wrong place, which leads to the infinite loop.
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- While(i<=10);
- {
- Printf("%d", i);
- i++;
- }
- Return 0;
- }
In the above code, we put the semicolon after the condition of the while loop which leads to the infinite loop. Due to this semicolon, the internal body of the while loop will not execute.
- We should check the logical conditions carefully. Sometimes by mistake, we place the assignment operator (=) instead of a relational operator (= =).
- #include <stdio.h>
- Int main()
- {
- Char ch='n';
- While(ch='y')
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we use the assignment operator (ch='y') which leads to the execution of loop infinite number of times.
- We use the wrong loop condition which causes the loop to be executed indefinitely.
- #include <stdio.h>
- Int main()
- {
- For(int i=1;i>=1;i++)
- {
- Printf("hello");
- }
- Return 0;
- }
The above code will execute the 'for loop' infinite number of times. As we put the condition (i>=1), which will always be true for every condition, it means that "hello" will be printed infinitely.
- We should be careful when we are using the break keyword in the nested loop because it will terminate the execution of the nearest loop, not the entire loop.
- #include <stdio.h>
- Int main()
- {
- While(1)
- {
- For(int i=1;i<=10;i++)
- {
- If(i%2==0)
- {
- Break;
- }
- }
- }
- Return 0;
- }
In the above code, the while loop will be executed an infinite number of times as we use the break keyword in an inner loop. This break keyword will bring the control out of the inner loop, not from the outer loop.
- We should be very careful when we are using the floating-point value inside the loop as we cannot underestimate the floating-point errors.
- #include <stdio.h>
- Int main()
- {
- Float x = 3.0;
- While (x != 4.0) {
- Printf("x = %f\n", x);
- x += 0.1;
- }
- Return 0;
- }
In the above code, the loop will run infinite times as the computer represents a floating-point value as a real value. The computer will represent the value of 4.0 as 3.999999 or 4.000001, so the condition (x !=4.0) will never be false. The solution to this problem is to write the condition as (k<=4.0).
In conditional branching change in sequence of statement execution is depends upon the specified condition. Conditional statements allow programmer to check a condition and execute certain parts of code depending on the result of conditional expression. Conditional expression must be resulted into Boolean value. In C language, there are two forms of conditional statements:
1. If-----else statement: It is used to select one option between two alternatives
2. Switch statement: It is used to select one option between multiple alternative
- If Statements
This statement permits the programmer to allocate condition on the execution of a statement. If the evaluated condition found to be true, the single statement following the "if" is execute. If the condition is found to be false, the following statement is skipped. Syntax of the if statement is as follows
if (condition)
statement1;
statement2;
In the above syntax, “if” is the keyword and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and then statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
if-----else statement
This statement permits the programmer to execute a statement out of the two statements. If the evaluated condition is found to be true, the single statement following the "if" is executed and statement following else is skipped. If the condition is found to be false, statement following the "if" is skipped and statement following else is executed.
In this statement “if” part is compulsory whereas “else” is the optional part. For every “if” statement there may be or may not be “else” statement but for every “else” statement there must be “if” part otherwise compiler will gives “Misplaced else” error.
Syntax of the “if----else” statement is as follows
If (condition)
Statement1;
Else
Statement2;
In the above syntax, “if” and “else” are the keywords and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and skip statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
Nested if-----else statements :
Nested “if-----else” statements are used when programmer wants to check multiple conditions. Nested “if---else” contains several “if---else” a statement out of which only one statement is executed. Number of “if----else” statements is equal to the number of conditions to be checked. Following is the syntax for nested “if---else” statements for three conditions
If (condition1)
Statement1;
Else if (condition2)
Statement2;
Else if (condition3)
Statement3;
Else
Statement4;
In the above syntax, compiler first check condition1, if it trues then it will execute statement1 and skip all the remaining statements. If condition1 is false then compiler directly checks condition2, if it is true then compiler execute statement2 and skip all the remaining statements. If condition2 is also false then compiler directly checks condition3, if it is true then compiler execute statement3 otherwise it will execute statement 4.
Note:
If the test expression is evaluated to true,
• Statements inside the body of if are executed.
• Statements inside the body of else are skipped from execution.
If the test expression is evaluated to false,
• Statements inside the body of else are executed
• Statements inside the body of if are skipped from execution.
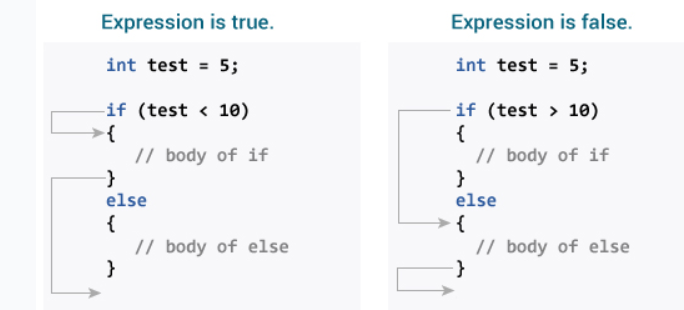
// Check whether an integer is odd or even
#include <stdio.h>
Int main() {
Int number;
Printf("Enter an integer: ");
Scanf("%d", &number);
// True if the remainder is 0
If (number%2 == 0) {
Printf("%d is an even integer.",number);
}
Else {
Printf("%d is an odd integer.",number);
}
Return 0;
}
Program to relate two integers using =, > or < symbol
#include <stdio.h>
Int main() {
Int number1, number2;
Printf("Enter two integers: ");
Scanf("%d %d", &number1, &number2);
//checks if the two integers are equal.
If(number1 == number2) {
Printf("Result: %d = %d",number1,number2);
}
//checks if number1 is greater than number2.
Else if (number1 > number2) {
Printf("Result: %d > %d", number1, number2);
}
//checks if both test expressions are false
Else {
Printf("Result: %d < %d",number1, number2);
}
Return 0;
}
- Switch Statements
This statement permits the programmer to choose one option out of several options depending on one condition. When the “switch” statement is executed, the expression in the switch statement is evaluated and the control is transferred directly to the group of statements whose “case” label value matches with the value of the expression. Syntax for switch statement is as follows:
Switch(expression)
{
Case constant1:
Statements 1;
Break;
Case constant2:
Statements 2;
Break;
…………………..
Default:
Statements n;
Break;
}
In the above, “switch”, “case”, “break” and “default” are keywords. Out of which “switch” and “case” are the compulsory keywords whereas “break” and “default” is optional keywords.
- “switch” keyword is used to start switch statement with conditional expression.
- “case” is the compulsory keyword which labeled with a constant value. If the value of expression matches with the case value then statement followed by “case” statement is executed.
- “break” is the optional keyword in switch statement. The execution of “break” statement causes the transfer of flow of execution outside the “switch” statements scope. Absence of “break” statement causes the execution of all the following “case” statements without concerning value of the expression.
- “default” is the optional keyword in “switch” statement. When the value of expression is not match with the any of the “case” statement then the statement following “default” keyword is executed.
Example: Program to spell user entered single digit number in English is as follows
#include<stdio.h>
#include<conio.h>
Void main()
{
Int n;
Clrscr();
Printf("Enter a number");
Scanf("%d",&n);
Switch(n)
{
Case 0: printf("\n Zero");
Break;
Case 1: printf("\n One");
Break;
Case 2: printf("\n Two");
Break;
Case 3: printf("\n Three");
Break;
Case 4: printf("\n Four");
Break;
Case 5: printf("\n Five");
Break;
Case 6: printf("\n Six");
Break;
Case 7: printf("\n Seven");
Break;
Case 8: printf("\n Eight");
Break;
Case 9: printf("\n Nine");
Break;
Default: printf("Given number is not single digit number");
}
Getch();
}
Output
Enter a number
5
Five
Loops are a form of iteration (recursion being the other form). Proper use of recursion depends on viewing the problem in a certain way to extract the recursion. Loop-based iteration is no different. They all come down to the same process.
- View the task as a sequence identical or nearly identical sub-tasks
- Figure out what changes each iteration (if anything)
- Express that change in programming terms
- Figure out when the program should stop performing the task
- Express that end condition as a conditional that asks - under what condition should the task continue repeating (not when should it stop)
While Loops
The while loop is the universal loop - all loops can be constructed using while loops. All other loops are created for specific circumstances.
While( condition ) BB; | ![]() |
Int i = 0; While( i < 5 ) { Printf( "i = %d\n", i); i++; } Printf( "After loop, i = %d\n", i ); | ![]() |
How it works:
The condition is checked prior to executing the body every time. It will only exit once the condition evaluates to false. Make sure you don't forget to have something change so that it eventually terminates the loop.
When to use this construct: This is appropriate for any loop - unless your loop follows the special circumstances for the loops below. In general, this is for loops for which the exit condition is not simply a counter that increments (as you see above). Instead, it's perfect for loops such as menus asking for user input.
Do-while
If you know you want to run the body of a loop at least once, then a do-while loop is appropriate.
Do{ BB; } while( condition ) | ![]() |
Int stop = 0; Do { Stop = Menu(); } while (!stop); | ![]() |
How it works:
The BB will run once no matter what, then the condition will be evaluated. Once that BB is run once, it is equivalent to the while loop.
When to use this construct: When you want the body to run at least once.
For
The for loop was created based on a common mistake when implementing loops - forgetting the update step. That is, forgetting to increment your counter or some such action. If you forget the action that gets you closer to loop termination, then your loop will not terminate.
The for loop is designed so that
- Programmers will remember the lines that are crucial to loop termination
- Others reading the loop will be able to quickly read what controls the loop
For (init; condition; update) { BB; } | ![]() |
Int i; For( i = 0; i < 5; i++ ) Printf( "i = %d\n", i ); Printf( "After loop\n"); | ![]() |
How it works:
The init code runs before the loop. Then it evaluates the condition. Next, it runs the loop body. It finishes with the update step, looping back to the condition again. The most common mistake in terms of understanding is forgetting that the condition is evaluated prior to the first loop iteration.
When to use this construct:
When your loop control is very simple and can be expressed easily inside the for loop syntax.
The comma operator
- C has a comma operator, that basically combines two statements so that they can be considered as a single statement.
- About the only place this is ever used is in for loops, to either provide multiple initializations or to allow for multiple incrementations.
- For example:
Int i, j = 10, sum;
For( i = 0, sum = 0; i < 5; i++, j-- )
Sum += i * j;
Break and continue
- Break and continue are two C/C++ statements that allow us to further control flow within and out of loops.
- Break causes execution to immediately jump out of the current loop, and proceed with the code following the loop.
- Continue causes the remainder of the current iteration of the loop to be skipped, and for execution to recommence with the next iteration.
- In the case of for loops, the incrementation step will be executed next, followed by the condition test to start the next loop iteration.
- In the case of while and do-while loops, execution jumps to the next loop condition test.
Suggested Text Books
Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill
E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
Suggested Reference Books
Brian W. Kernighan and Dennis M. Ritchie, The C Programming Language, Prentice Hall of India