UNIT 2
Control Structures, Arrays
Arithmetic expressions
An arithmetic expression contains only arithmetic operators and operands. We know that the arithmetic operators in C language include unary operators (+ – ++ — ), multiplicative operators (* / %) and additive operators (+ – ). The arithmetic operands include integral operands (various int and char types) and floating-type operands (float, double and long double).
Example Simple arithmetic expressions
a) Several valid arithmetic expressions are given below. Assume that variables a and b are of type int.
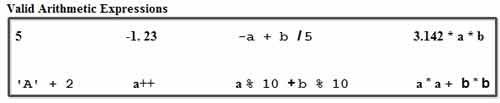
Observe that the operators are used correctly. The unary operators in expressions -1.23, a++ and -a + b /5 correctly operate on a single operand. The unary minus (-) is used as a prefix operator whereas the increment operator (++) is used in the postfix form. All other operators (multiplicative and additive) are used as infix operators and they operate on two operands. Also, the modulo-arithmetic(s) operator operates on integer operands as required. The expression ‘A’ + 2 is valid and its value is equal to 67, as the ASCII value of character ‘A’ is 65. Note that the operands and operators need not be separated by spaces. For example, the last two expressions in the first row can also be written as -a+b/5 and 3 .142*a*b. However, the spaces are often used to improve readability.
b) The expressions given below are invalid
The first two expressions are invalid because the operands a and b are not connected by any operator. This is true even for the third expression (a++ –b) as the++ and — operators operate on operands a and b, respectively, but there is no operator connecting these operands.
The last two expressions are invalid as they contain two binary operators in succession. These operators operate on a single operand instead of two. Note that the – operator in last expression cannot be interpreted as a unary operator as it does not precede an operand.
c) Can you tell which of the following expressions are valid?

Surprisingly, all are valid C expressions although they do not appear to be so. In expression +a +b, the first+ operator is a unary operator that operates on variable a, whereas the second+ is a binary operator that connects variables a and b.
Now observe the space between the two’-‘ symbols in the second expression. They cannot
Be interpreted as a decrement operator. As a result, the second – symbol is interpreted as a unary minus operator, whereas the first – is interpreted as a subtraction operator that operates on two operands a and -b. ·
The two consecutive operators in expression a % 10 I – b does not pose a problem as the –
Operator is interpreted as a unary operator and the I operator operates on two operands (a % 10 and -b). However, the space between – and b is misleading.
In the last expression, observe the spaces separating the middle + symbol from the increment operators that operate on variables a and b. The middle + is interpreted as a binary operator that operates on a and b.
d) Several simple mathematical equations are given below along with equivalent C expressions.
Assume that the symbolic constant PI has been defined as
#define PI 3.1415927
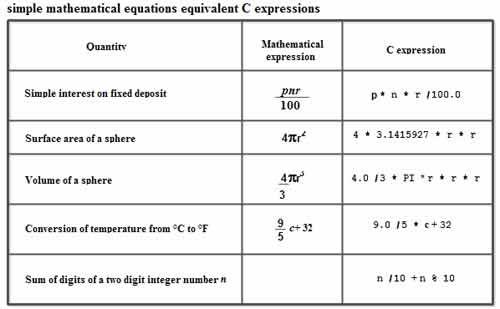
Observe that the literal 4 in expression 3 is written as floating constant 4.0 to avoid the integer division of 4 and 3. Similarly, constant 9 in expression 4 is also written as a floating constant. The sum of digits of a two-digit integer number is written as n I 10 + n % 10.Since n is assumed to be an integer variable, the expression n I 1o performs integer division and determines the digit at the 10’s place, whereas the expression n % 10 determines the digit at the unit’s place.
Evaluation of Simple Arithmetic Expressions
We use the operator precedence and associativity rules to determine the meaning and value of an expression in an unambiguous manner. Recall that the operators in an expression are bound to their operands in the order of their precedence. If the expression contains more than one operator at the same precedence level, they are associated with their operands using the associativity rules. Table summarizes these rules for arithmetic and assignment operators.
If the given expression is simple, we can often directly convert it to its mathematical form and evaluate it. However, if the given expression is complex, i. e., it contains several operators at different precedence levels, we need a systematic approach to convert it to a mathematical equation and evaluate it. The steps to convert a given valid C expression to its mathematical form (if possible) and to evaluate it are as follows:
1. First determine the order in which the operators are bound to their operands by applying the precedence and associativity rules. Note that after an operator is bound to its operand(s), that sub-expression is considered as a single operand for the adjacent operators.
2. Obtain the equivalent mathematical equation for given C expression (if possible) by following the operator binding sequence (obtained in step 1).
3. Determine the value of the given expression by evaluating operators in the binding sequence.
The steps to determine operator binding in an arithmetic expression are explained below with the help of the expression -a+ b * c – d I e + f.
1. The unary operators (unary +, unary – , ++ and – -) have the highest precedence and right-to-left associativity. Thus, the given expression is first scanned from right to left and unary operators, if any, are bound to their operands. The order is indicated below the expression as follows:
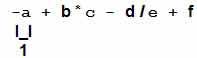
2. The multiplicative operators (*, I and %) have the next highest precedence and left to- right associativity. Thus, the expression is scanned from left-to-right and the multiplicative operators, if any, are bound to their operands as shown below. (Observe that after completion of the above step, sub-expressions -a, b * c and d I e will be operands for the remaining operator bindings.)
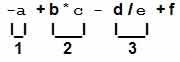
3. The additive operators (+ and -) have the next highest precedence and left-to-right associativity. Hence, the expression is scanned from left-to-tight and the additive operators, if any, are bound to their operands as shown below. Observe that the operands for the first + operator are the sub-expressions -a and b * c. Similarly, the operands for the – operator are -a+ b * c and d /e.
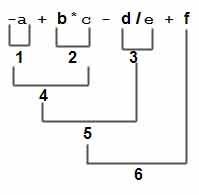
Now we can write the mathematical equation for the given C expression by following the operator binding sequence as shown below:
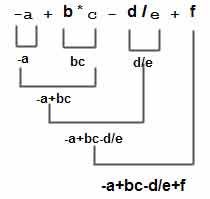
Now the given expression can be evaluated, again by following the operator binding sequence, as shown below. Assume that the variables a, b, c, ct, e and f are of type float and are initialized with values as a= 1. 5, b = 2. 0, c = 3. 5, ct= 5.0, e = 2. 5 and f = 1. 25.
Remember that except for some operators (& & || ? : and the comma operator), the C language does not specify the order of evaluation of sub-expressions. Thus, the sub expressions at the same level can be evaluated in any order. For example, the sub expressions -a, b * c and ct I e in the above expression can be evaluated in any order.
The procedure explained above can also be used to check the validity of an expression. The given expression is valid if we arrive at a single operand (or value) after all the operators in the given expression are considered. Consider a more complex arithmetic expression: -a–+ -b++ * –c. This expression appears to be invalid due to the excessive use of operators. It contains three operands a, b and c and seven operators, five of which are unary (-, ++ and –) and the other two are binary operators (+ and *). However, using the operator binding steps, we can easily verify that it is a valid expression:
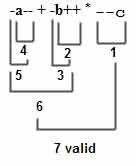
Precedence
Operator precedence determines which operator is performed first in an expression with more than one operators with different precedence.
For example: Solve
10 + 20 * 30
10 + 20 * 30 is calculated as 10 + (20 * 30)
And not as (10 + 20) * 30
Operators Associativity is used when two operators of same precedence appear in an expression. Associativity can be either Left to Right or Right to Left.
For example: ‘*’ and ‘/’ have same precedence and their associativity is Left to Right, so the expression “100 / 10 * 10” is treated as “(100 / 10) * 10”.
Operators Precedence and Associativity are two characteristics of operators that determine the evaluation order of sub-expressions in absence of brackets
For example: Solve
100 + 200 / 10 - 3 * 10
1) Associativity is only used when there are two or more operators of same precedence.
The point to note is associativity doesn’t define the order in which operands of a single operator are evaluated. For example, consider the following program, associativity of the + operator is left to right, but it doesn’t mean f1() is always called before f2(). The output of the following program is in-fact compiler dependent.
// Associativity is not used in the below program. // Output is compiler dependent.
#include <stdio.h>
Int x = 0; Int f1() { x = 5; Return x; } Int f2() { x = 10; Return x; } Int main() { Int p = f1() + f2(); Printf("%d ", x); Return 0; } |
2) All operators with the same precedence have same associativity
This is necessary, otherwise, there won’t be any way for the compiler to decide evaluation order of expressions which have two operators of same precedence and different associativity. For example + and – have the same associativity.
3) Precedence and associativity of postfix ++ and prefix ++ are different
Precedence of postfix ++ is more than prefix ++, their associativity is also different. Associativity of postfix ++ is left to right and associativity of prefix ++ is right to left.
4) Comma has the least precedence among all operators and should be used carefully For example consider the following program, the output is 1.
#include <stdio.h> Int main() { Int a; a = 1, 2, 3; // Evaluated as (a = 1), 2, 3 Printf("%d", a); Return 0; } |
5) There is no chaining of comparison operators in C
In Python, expression like “c > b > a” is treated as “c > b and b > a”, but this type of chaining doesn’t happen in C. For example consider the following program. The output of following program is “FALSE”.
#include <stdio.h> Int main() { Int a = 10, b = 20, c = 30;
// (c > b > a) is treated as ((c > b) > a), associativity of '>' // is left to right. Therefore the value becomes ((30 > 20) > 10) // which becomes (1 > 20) If (c > b > a) Printf("TRUE"); Else Printf("FALSE"); Return 0; } |
Please see the following precedence and associativity table for reference.
Operator | Description |
Associativity |
( ) | Parentheses (function call) (see Note 1) | Left-to-right |
++ — | Prefix increment/decrement | Right-to-left |
* / % | Multiplication/division/modulus | Left-to-right |
+ – | Addition/subtraction | Left-to-right |
<< >> | Bitwise shift left, Bitwise shift right | Left-to-right |
< <= | Relational less than/less than or equal to | Left-to-right |
== != | Relational is equal to/is not equal to | Left-to-right |
& | Bitwise AND | Left-to-right |
^ | Bitwise exclusive OR | Left-to-right |
| | Bitwise inclusive OR | Left-to-right |
&& | Logical AND | Left-to-right |
| | | Logical OR | Left-to-right |
? : | Ternary conditional | Right-to-left |
= | Assignment | Right-to-left |
, | Comma (separate expressions) | Left-to-right |
It is good to know precedence and associativity rules, but the best thing is to use brackets, especially for less commonly used operators (operators other than +, -, *.. Etc). Brackets increase the readability of the code as the reader doesn’t have to see the table to find out the order.
Conditional Branching
If else Statement
The if-else statement in C is used to perform the operations based on some specific condition. The operations specified in if block are executed if and only if the given condition is true.
There are the following variants of if statement in C language.
- If statement
- If-else statement
- If else-if ladder
- Nested if
If Statement
The if statement is used to check some given condition and perform some operations depending upon the correctness of that condition. It is mostly used in the scenario where we need to perform the different operations for the different conditions. The syntax of the if statement is given below.
- If(expression){
- //code to be executed
- }
Flowchart of if statement in C
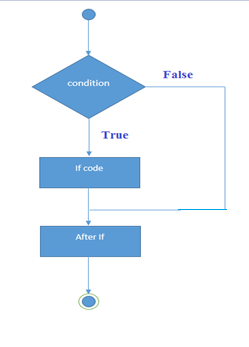
Let's see a simple example of C language if statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("Enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
Program to find the largest number of the three.
- #include <stdio.h>
- Int main()
- {
- Int a, b, c;
- Printf("Enter three numbers?");
- Scanf("%d %d %d",&a,&b,&c);
- If(a>b && a>c)
- {
- Printf("%d is largest",a);
- }
- If(b>a && b > c)
- {
- Printf("%d is largest",b);
- }
- If(c>a && c>b)
- {
- Printf("%d is largest",c);
- }
- If(a == b && a == c)
- {
- Printf("All are equal");
- }
- }
Output
Enter three numbers?
12 23 34
34 is largest
If-else Statement
The if-else statement is used to perform two operations for a single condition. The if-else statement is an extension to the if statement using which, we can perform two different operations, i.e., one is for the correctness of that condition, and the other is for the incorrectness of the condition. Here, we must notice that if and else block cannot be executed simultaneously. Using if-else statement is always preferable since it always invokes an otherwise case with every if condition. The syntax of the if-else statement is given below.
- If(expression){
- //code to be executed if condition is true
- }else{
- //code to be executed if condition is false
- }
Flowchart of the if-else statement in C
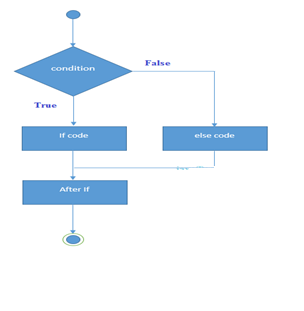
Let's see the simple example to check whether a number is even or odd using if-else statement in C language.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number%2==0){
- Printf("%d is even number",number);
- }
- Else{
- Printf("%d is odd number",number);
- }
- Return 0;
- }
Output
Enter a number:4
4 is even number
Enter a number:5
5 is odd number
Program to check whether a person is eligible to vote or not.
- #include <stdio.h>
- Int main()
- {
- Int age;
- Printf("Enter your age?");
- Scanf("%d",&age);
- If(age>=18)
- {
- Printf("You are eligible to vote...");
- }
- Else
- {
- Printf("Sorry ... you can't vote");
- }
- }
Output
Enter your age?18
You are eligible to vote...
Enter your age?13
Sorry ... You can't vote
If else-if ladder Statement
The if-else-if ladder statement is an extension to the if-else statement. It is used in the scenario where there are multiple cases to be performed for different conditions. In if-else-if ladder statement, if a condition is true then the statements defined in the if block will be executed, otherwise if some other condition is true then the statements defined in the else-if block will be executed, at the last if none of the condition is true then the statements defined in the else block will be executed. There are multiple else-if blocks possible. It is similar to the switch case statement where the default is executed instead of else block if none of the cases is matched.
- If(condition1){
- //code to be executed if condition1 is true
- }else if(condition2){
- //code to be executed if condition2 is true
- }
- Else if(condition3){
- //code to be executed if condition3 is true
- }
- ...
- Else{
- //code to be executed if all the conditions are false
- }
Flowchart of else-if ladder statement in C
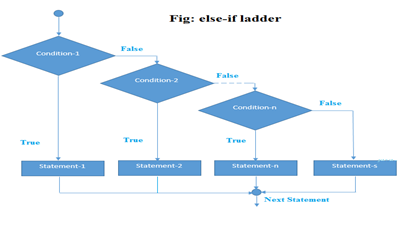
The example of an if-else-if statement in C language is given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- If(number==10){
- Printf("number is equals to 10");
- }
- Else if(number==50){
- Printf("number is equal to 50");
- }
- Else if(number==100){
- Printf("number is equal to 100");
- }
- Else{
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Program to calculate the grade of the student according to the specified marks.
- #include <stdio.h>
- Int main()
- {
- Int marks;
- Printf("Enter your marks?");
- Scanf("%d",&marks);
- If(marks > 85 && marks <= 100)
- {
- Printf("Congrats ! you scored grade A ...");
- }
- Else if (marks > 60 && marks <= 85)
- {
- Printf("You scored grade B + ...");
- }
- Else if (marks > 40 && marks <= 60)
- {
- Printf("You scored grade B ...");
- }
- Else if (marks > 30 && marks <= 40)
- {
- Printf("You scored grade C ...");
- }
- Else
- {
- Printf("Sorry you are fail ...");
- }
- }
Output
Enter your marks?10
Sorry you are fail ...
Enter your marks?40
You scored grade C ...
Enter your marks?90
Congrats ! you scored grade A ...
Switch Statement
The switch statement in C is an alternate to if-else-if ladder statement which allows us to execute multiple operations for the different possibles values of a single variable called switch variable. Here, We can define various statements in the multiple cases for the different values of a single variable.
The syntax of switch statement is given below:
- Switch(expression){
- Case value1:
- //code to be executed;
- Break; //optional
- Case value2:
- //code to be executed;
- Break; //optional
- ......
- Default:
- Code to be executed if all cases are not matched;
- }
Rules for switch statement in C language
1) The switch expression must be of an integer or character type.
2) The case value must be an integer or character constant.
3) The case value can be used only inside the switch statement.
4) The break statement in switch case is not must. It is optional. If there is no break statement found in the case, all the cases will be executed present after the matched case. It is known as fall through the state of C switch statement.
Let's try to understand it by the examples. We are assuming that there are following variables.
- Int x,y,z;
- Char a,b;
- Float f;
Valid Switch | Invalid Switch | Valid Case | Invalid Case |
Switch(x) | Switch(f) | Case 3; | Case 2.5; |
Switch(x>y) | Switch(x+2.5) | Case 'a'; | Case x; |
Switch(a+b-2) |
| Case 1+2; | Case x+2; |
Switch(func(x,y)) |
| Case 'x'>'y'; | Case 1,2,3; |
Flowchart of switch statement in C
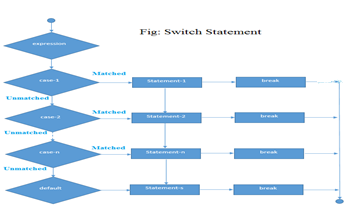
Functioning of switch case statement
First, the integer expression specified in the switch statement is evaluated. This value is then matched one by one with the constant values given in the different cases. If a match is found, then all the statements specified in that case are executed along with the all the cases present after that case including the default statement. No two cases can have similar values. If the matched case contains a break statement, then all the cases present after that will be skipped, and the control comes out of the switch. Otherwise, all the cases following the matched case will be executed.
Let's see a simple example of c language switch statement.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equals to 10");
- Break;
- Case 50:
- Printf("number is equal to 50");
- Break;
- Case 100:
- Printf("number is equal to 100");
- Break;
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:4
Number is not equal to 10, 50 or 100
Enter a number:50
Number is equal to 50
Switch case example 2
- #include <stdio.h>
- Int main()
- {
- Int x = 10, y = 5;
- Switch(x>y && x+y>0)
- {
- Case 1:
- Printf("hi");
- Break;
- Case 0:
- Printf("bye");
- Break;
- Default:
- Printf(" Hello bye ");
- }
- }
Output
Hi
C Switch statement is fall-through
In C language, the switch statement is fall through; it means if you don't use a break statement in the switch case, all the cases after the matching case will be executed.
Let's try to understand the fall through state of switch statement by the example given below.
- #include<stdio.h>
- Int main(){
- Int number=0;
- Printf("enter a number:");
- Scanf("%d",&number);
- Switch(number){
- Case 10:
- Printf("number is equal to 10\n");
- Case 50:
- Printf("number is equal to 50\n");
- Case 100:
- Printf("number is equal to 100\n");
- Default:
- Printf("number is not equal to 10, 50 or 100");
- }
- Return 0;
- }
Output
Enter a number:10
Number is equal to 10
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Output
Enter a number:50
Number is equal to 50
Number is equal to 100
Number is not equal to 10, 50 or 100
Nested switch case statement
We can use as many switch statement as we want inside a switch statement. Such type of statements is called nested switch case statements. Consider the following example.
- #include <stdio.h>
- Int main () {
- Int i = 10;
- Int j = 20;
- Switch(i) {
- Case 10:
- Printf("the value of i evaluated in outer switch: %d\n",i);
- Case 20:
- Switch(j) {
- Case 20:
- Printf("The value of j evaluated in nested switch: %d\n",j);
- }
- }
- Printf("Exact value of i is : %d\n", i );
- Printf("Exact value of j is : %d\n", j );
- Return 0;
- }
Output
The value of i evaluated in outer switch: 10
The value of j evaluated in nested switch: 20
Exact value of i is : 10
Exact value of j is : 20
If-else vs switch
What is an if-else statement?
An if-else statement in C programming is a conditional statement that executes a different set of statements based on the condition that is true or false. The 'if' block will be executed only when the specified condition is true, and if the specified condition is false, then the else block will be executed.
Syntax of if-else statement is given below:
- If(expression)
- {
- // statements;
- }
- Else
- {
- // statements;
- }
What is a switch statement?
A switch statement is a conditional statement used in C programming to check the value of a variable and compare it with all the cases. If the value is matched with any case, then its corresponding statements will be executed. Each case has some name or number known as the identifier. The value entered by the user will be compared with all the cases until the case is found. If the value entered by the user is not matched with any case, then the default statement will be executed.
Syntax of the switch statement is given below:
- Switch(expression)
- {
- Case constant 1:
- // statements;
- Break;
- Case constant 2:
- // statements;
- Break;
- Case constant n:
- // statements;
- Break;
- Default:
- // statements;
- }
Similarity b/w if-else and switch
Both the if-else and switch are the decision-making statements. Here, decision-making statements mean that the output of the expression will decide which statements are to be executed.
Differences b/w if-else and switch statement
The following are the differences between if-else and switch statement are:
- Definition
If-else
Based on the result of the expression in the 'if-else' statement, the block of statements will be executed. If the condition is true, then the 'if' block will be executed otherwise 'else' block will execute.
Switch statement
The switch statement contains multiple cases or choices. The user will decide the case, which is to execute.
- Expression
If-else
It can contain a single expression or multiple expressions for multiple choices. In this, an expression is evaluated based on the range of values or conditions. It checks both equality and logical expressions.
Switch
It contains only a single expression, and this expression is either a single integer object or a string object. It checks only equality expression.
- Evaluation
If-else
An if-else statement can evaluate almost all the types of data such as integer, floating-point, character, pointer, or Boolean.
Switch
A switch statement can evaluate either an integer or a character.
- Sequence of Execution
If-else
In the case of 'if-else' statement, either the 'if' block or the 'else' block will be executed based on the condition.
Switch
In the case of the 'switch' statement, one case after another will be executed until the break keyword is not found, or the default statement is executed.
- Default Execution
If-else
If the condition is not true within the 'if' statement, then by default, the else block statements will be executed.
Switch
If the expression specified within the switch statement is not matched with any of the cases, then the default statement, if defined, will be executed.
- Values
If-else
Values are based on the condition specified inside the 'if' statement. The value will decide either the 'if' or 'else' block is to be executed.
Switch
In this case, value is decided by the user. Based on the choice of the user, the case will be executed.
- Use
If-else
It evaluates a condition to be true or false.
Switch
A switch statement compares the value of the variable with multiple cases. If the value is matched with any of the cases, then the block of statements associated with this case will be executed.
- Editing
If-else
Editing in 'if-else' statement is not easy as if we remove the 'else' statement, then it will create the havoc.
Switch
Editing in switch statement is easier as compared to the 'if-else' statement. If we remove any of the cases from the switch, then it will not interrupt the execution of other cases. Therefore, we can say that the switch statement is easy to modify and maintain.
- Speed
If-else
If the choices are multiple, then the speed of the execution of 'if-else' statements is slow.
Switch
The case constants in the switch statement create a jump table at the compile time. This jump table chooses the path of the execution based on the value of the expression. If we have a multiple choice, then the execution of the switch statement will be much faster than the equivalent logic of 'if-else' statement.
Let's summarize the above differences in a tabular form.
| If-else | Switch |
Definition | Depending on the condition in the 'if' statement, 'if' and 'else' blocks are executed. | The user will decide which statement is to be executed. |
Expression | It contains either logical or equality expression. | It contains a single expression which can be either a character or integer variable. |
Evaluation | It evaluates all types of data, such as integer, floating-point, character or Boolean. | It evaluates either an integer, or character. |
Sequence of execution | First, the condition is checked. If the condition is true then 'if' block is executed otherwise 'else' block | It executes one case after another till the break keyword is not found, or the default statement is executed. |
Default execution | If the condition is not true, then by default, else block will be executed. | If the value does not match with any case, then by default, default statement is executed. |
Editing | Editing is not easy in the 'if-else' statement. | Cases in a switch statement are easy to maintain and modify. Therefore, we can say that the removal or editing of any case will not interrupt the execution of other cases. |
Speed | If there are multiple choices implemented through 'if-else', then the speed of the execution will be slow. | If we have multiple choices then the switch statement is the best option as the speed of the execution will be much higher than 'if-else'. |
Loops
The looping can be defined as repeating the same process multiple times until a specific condition satisfies. There are three types of loops used in the C language. In this part of the tutorial, we are going to learn all the aspects of C loops.
Why use loops in C language?
The looping simplifies the complex problems into the easy ones. It enables us to alter the flow of the program so that instead of writing the same code again and again, we can repeat the same code for a finite number of times. For example, if we need to print the first 10 natural numbers then, instead of using the printf statement 10 times, we can print inside a loop which runs up to 10 iterations.
Advantage of loops in C
1) It provides code reusability.
2) Using loops, we do not need to write the same code again and again.
3) Using loops, we can traverse over the elements of data structures (array or linked lists).
Types of C Loops
There are three types of loops in C language that is given below:
- Do while
- While
- For
Do-while loop in C
The do-while loop continues until a given condition satisfies. It is also called post tested loop. It is used when it is necessary to execute the loop at least once (mostly menu driven programs).
The syntax of do-while loop in c language is given below:
- Do{
- //code to be executed
- }while(condition);
While loop in C
The while loop in c is to be used in the scenario where we don't know the number of iterations in advance. The block of statements is executed in the while loop until the condition specified in the while loop is satisfied. It is also called a pre-tested loop.
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
For loop in C
The for loop is used in the case where we need to execute some part of the code until the given condition is satisfied. The for loop is also called as a per-tested loop. It is better to use for loop if the number of iteration is known in advance.
The syntax of for loop in c language is given below:
- For(initialization;condition;incr/decr){
- //code to be executed
- }
Do while loop
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
Do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example 1
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Flowchart of do while loop
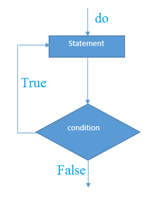
do while example
There is given the simple program of c language do while loop where we are printing the table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- Do{
- Printf("%d \n",i);
- i++;
- }while(i<=10);
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loop
The do-while loop will run infinite times if we pass any non-zero value as the conditional expression.
- Do{
- //statement
- }while(1);
While loop
While loop is also known as a pre-tested loop. In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition. It can be viewed as a repeating if statement. The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax of while loop in C language
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
Flowchart of while loop in C
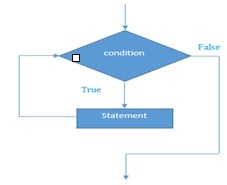
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- While(i<=10){
- Printf("%d \n",i);
- i++;
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
- #include<stdio.h>
- Int main(){
- Int i=1,number=0,b=9;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- While(i<=10){
- Printf("%d \n",(number*i));
- i++;
- }
- Return 0;
- }
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
Properties of while loop
- A conditional expression is used to check the condition. The statements defined inside the while loop will repeatedly execute until the given condition fails.
- The condition will be true if it returns 0. The condition will be false if it returns any non-zero number.
- In while loop, the condition expression is compulsory.
- Running a while loop without a body is possible.
- We can have more than one conditional expression in while loop.
- If the loop body contains only one statement, then the braces are optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int j = 1;
- While(j+=2,j<=10)
- {
- Printf("%d ",j);
- }
- Printf("%d",j);
- }
Output
3 5 7 9 11
Example 2
- #include<stdio.h>
- Void main ()
- {
- While()
- {
- Printf("hello Javatpoint");
- }
- }
Output
Compile time error: while loop can't be empty
Example 3
- #include<stdio.h>
- Void main ()
- {
- Int x = 10, y = 2;
- While(x+y-1)
- {
- Printf("%d %d",x--,y--);
- }
- }
Output
Infinite loop
Infinitive while loop in C
If the expression passed in while loop results in any non-zero value then the loop will run the infinite number of times.
- While(1){
- //statement
- }
For loop
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
- For(Expression 1; Expression 2; Expression 3){
- //code to be executed
- }
Flowchart of for loop in C
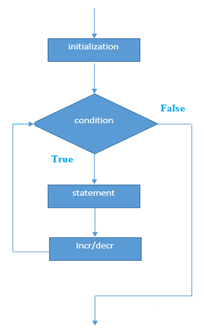
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
- The expression represents the initialization of the loop variable.
- We can initialize more than one variable in Expression 1.
- Expression 1 is optional.
- In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int a,b,c;
- For(a=0,b=12,c=23;a<2;a++)
- {
- Printf("%d ",a+b+c);
- }
- }
Output
35 36
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- For(;i<5;i++)
- {
- Printf("%d ",i);
- }
- }
Output
1 2 3 4
Properties of Expression 2
- Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
- Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
- Expression 2 is optional.
- Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
- We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;i<=4;i++)
- {
- Printf("%d ",i);
- }
- }
Output
0 1 2 3 4
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i,j,k;
- For(i=0,j=0,k=0;i<4,k<8,j<10;i++)
- {
- Printf("%d %d %d\n",i,j,k);
- j+=2;
- k+=3;
- }
- }
Output
0 0 0
1 2 3
2 4 6
3 6 9
4 8 12
Example 3
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;;i++)
- {
- Printf("%d",i);
- }
- }
Output
Infinite loop
Properties of Expression 3
- Expression 3 is used to update the loop variable.
- We can update more than one variable at the same time.
- Expression 3 is optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int i=0,j=2;
- For(i = 0;i<5;i++,j=j+2)
- {
- Printf("%d %d\n",i,j);
- }
- }
Output
0 2
1 4
2 6
3 8
4 10
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Int i;
- For(i=0;i<10;i++)
- {
- Int i = 20;
- Printf("%d ",i);
- }
- }
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
- #include<stdio.h>
- Void main ()
- {
- For(;;)
- {
- Printf("welcome to javatpoint");
- }
- }
If you run this program, you will see above statement infinite times.
Nested Loops in C
C supports nesting of loops in C. Nesting of loops is the feature in C that allows the looping of statements inside another loop. Let's observe an example of nesting loops in C.
Any number of loops can be defined inside another loop, i.e., there is no restriction for defining any number of loops. The nesting level can be defined at n times. You can define any type of loop inside another loop; for example, you can define 'while' loop inside a 'for' loop.
Syntax of Nested loop
- Outer_loop
- {
- Inner_loop
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Outer_loop and Inner_loop are the valid loops that can be a 'for' loop, 'while' loop or 'do-while' loop.
Nested for loop
The nested for loop means any type of loop which is defined inside the 'for' loop.
- For (initialization; condition; update)
- {
- For(initialization; condition; update)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested for loop
- #include <stdio.h>
- Int main()
- {
- Int n;// variable declaration
- Printf("Enter the value of n :");
- // Displaying the n tables.
- For(int i=1;i<=n;i++) // outer loop
- {
- For(int j=1;j<=10;j++) // inner loop
- {
- Printf("%d\t",(i*j)); // printing the value.
- }
- Printf("\n");
- }
Explanation of the above code
- First, the 'i' variable is initialized to 1 and then program control passes to the i<=n.
- The program control checks whether the condition 'i<=n' is true or not.
- If the condition is true, then the program control passes to the inner loop.
- The inner loop will get executed until the condition is true.
- After the execution of the inner loop, the control moves back to the update of the outer loop, i.e., i++.
- After incrementing the value of the loop counter, the condition is checked again, i.e., i<=n.
- If the condition is true, then the inner loop will be executed again.
- This process will continue until the condition of the outer loop is true.
Output:
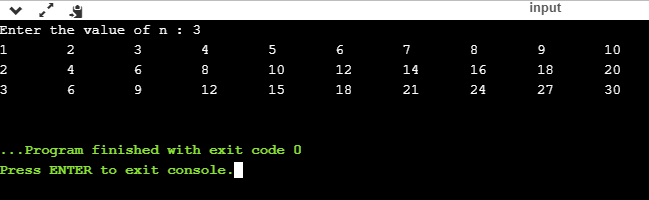
Nested while loop
The nested while loop means any type of loop which is defined inside the 'while' loop.
- While(condition)
- {
- While(condition)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested while loop
- #include <stdio.h>
- Int main()
- {
- Int rows; // variable declaration
- Int columns; // variable declaration
- Int k=1; // variable initialization
- Printf("Enter the number of rows :"); // input the number of rows.
- Scanf("%d",&rows);
- Printf("\nEnter the number of columns :"); // input the number of columns.
- Scanf("%d",&columns);
- Int a[rows][columns]; //2d array declaration
- Int i=1;
- While(i<=rows) // outer loop
- {
- Int j=1;
- While(j<=columns) // inner loop
- {
- Printf("%d\t",k); // printing the value of k.
- k++; // increment counter
- j++;
- }
- i++;
- Printf("\n");
- }
- }
Explanation of the above code.
- We have created the 2d array, i.e., int a[rows][columns].
- The program initializes the 'i' variable by 1.
- Now, control moves to the while loop, and this loop checks whether the condition is true, then the program control moves to the inner loop.
- After the execution of the inner loop, the control moves to the update of the outer loop, i.e., i++.
- After incrementing the value of 'i', the condition (i<=rows) is checked.
- If the condition is true, the control then again moves to the inner loop.
- This process continues until the condition of the outer loop is true.
Output:
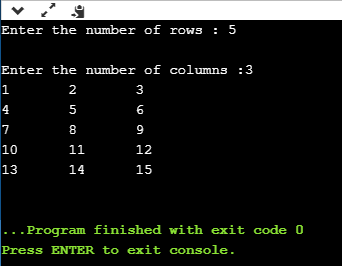
Nested do..while loop
The nested do..while loop means any type of loop which is defined inside the 'do..while' loop.
- Do
- {
- Do
- {
- // inner loop statements.
- }while(condition);
- // outer loop statements.
- }while(condition);
Example of nested do..while loop.
- #include <stdio.h>
- Int main()
- {
- /*printing the pattern
- ********
- ********
- ********
- ******** */
- Int i=1;
- Do // outer loop
- {
- Int j=1;
- Do // inner loop
- {
- Printf("*");
- j++;
- }while(j<=8);
- Printf("\n");
- i++;
- }while(i<=4);
- }
Output:
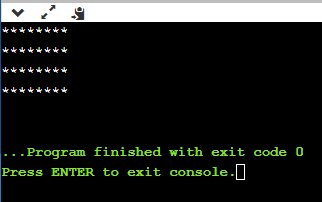
Explanation of the above code.
- First, we initialize the outer loop counter variable, i.e., 'i' by 1.
- As we know that the do..while loop executes once without checking the condition, so the inner loop is executed without checking the condition in the outer loop.
- After the execution of the inner loop, the control moves to the update of the i++.
- When the loop counter value is incremented, the condition is checked. If the condition in the outer loop is true, then the inner loop is executed.
- This process will continue until the condition in the outer loop is true.
Infinite Loop in C
What is infinite loop?
An infinite loop is a looping construct that does not terminate the loop and executes the loop forever. It is also called an indefinite loop or an endless loop. It either produces a continuous output or no output.
When to use an infinite loop
An infinite loop is useful for those applications that accept the user input and generate the output continuously until the user exits from the application manually. In the following situations, this type of loop can be used:
- All the operating systems run in an infinite loop as it does not exist after performing some task. It comes out of an infinite loop only when the user manually shuts down the system.
- All the servers run in an infinite loop as the server responds to all the client requests. It comes out of an indefinite loop only when the administrator shuts down the server manually.
- All the games also run in an infinite loop. The game will accept the user requests until the user exits from the game.
We can create an infinite loop through various loop structures. The following are the loop structures through which we will define the infinite loop:
- For loop
- While loop
- Do-while loop
- Go to statement
- C macros
For loop
Let's see the infinite 'for' loop. The following is the definition for the infinite for loop:
- For(; ;)
- {
- // body of the for loop.
- }
As we know that all the parts of the 'for' loop are optional, and in the above for loop, we have not mentioned any condition; so, this loop will execute infinite times.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- For(;;)
- {
- Printf("Hello javatpoint");
- }
- Return 0;
- }
In the above code, we run the 'for' loop infinite times, so "Hello javatpoint" will be displayed infinitely.
Output
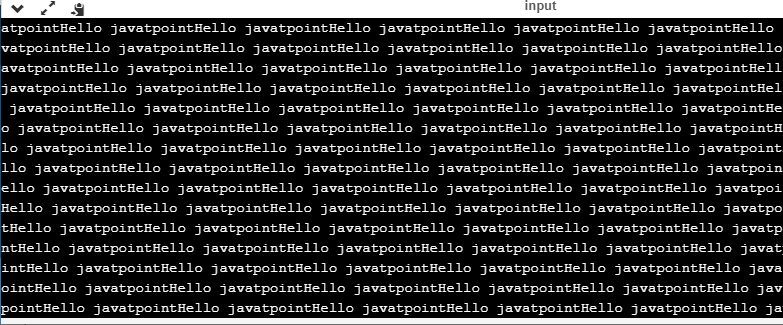
While loop
Now, we will see how to create an infinite loop using a while loop. The following is the definition for the infinite while loop:
- While(1)
- {
- // body of the loop..
- }
In the above while loop, we put '1' inside the loop condition. As we know that any non-zero integer represents the true condition while '0' represents the false condition.
Let's look at a simple example.
- #include <stdio.h>
- Int main()
- {
- Int i=0;
- While(1)
- {
- i++;
- Printf("i is :%d",i);
- }
- Return 0;
- }
In the above code, we have defined a while loop, which runs infinite times as it does not contain any condition. The value of 'i' will be updated an infinite number of times.
Output
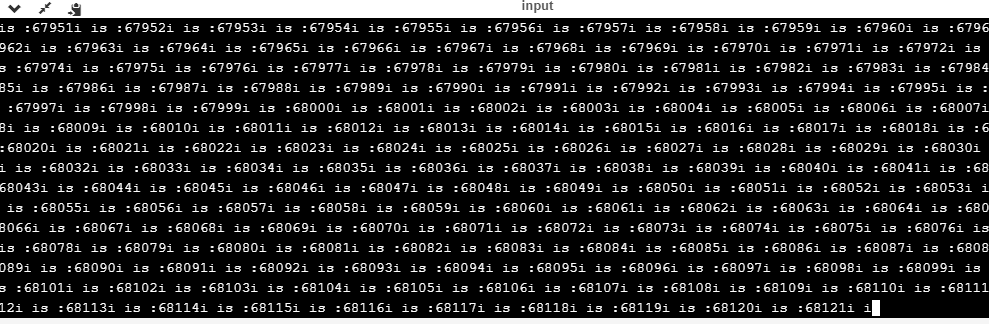
Do..while loop
The do..while loop can also be used to create the infinite loop. The following is the syntax to create the infinite do..while loop.
- Do
- {
- // body of the loop..
- }while(1);
The above do..while loop represents the infinite condition as we provide the '1' value inside the loop condition. As we already know that non-zero integer represents the true condition, so this loop will run infinite times.
Goto statement
We can also use the goto statement to define the infinite loop.
- Infinite_loop;
- // body statements.
- Goto infinite_loop;
In the above code, the goto statement transfers the control to the infinite loop.
Macros
We can also create the infinite loop with the help of a macro constant. Let's understand through an example.
- #include <stdio.h>
- #define infinite for(;;)
- Int main()
- {
- Infinite
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined a macro named as 'infinite', and its value is 'for(;;)'. Whenever the word 'infinite' comes in a program then it will be replaced with a 'for(;;)'.
Output
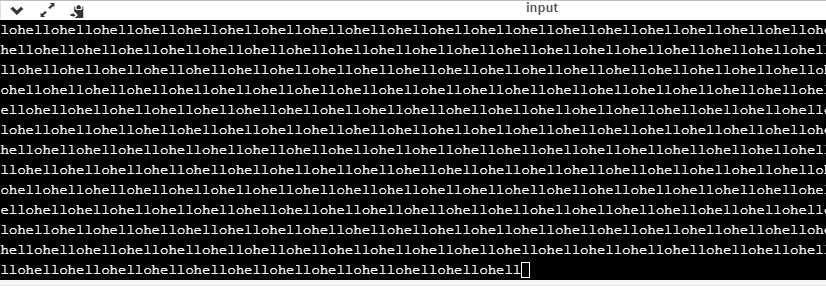
Till now, we have seen various ways to define an infinite loop. However, we need some approach to come out of the infinite loop. In order to come out of the infinite loop, we can use the break statement.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- Char ch;
- While(1)
- {
- Ch=getchar();
- If(ch=='n')
- {
- Break;
- }
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined the while loop, which will execute an infinite number of times until we press the key 'n'. We have added the 'if' statement inside the while loop. The 'if' statement contains the break keyword, and the break keyword brings control out of the loop.
Unintentional infinite loops
Sometimes the situation arises where unintentional infinite loops occur due to the bug in the code. If we are the beginners, then it becomes very difficult to trace them. Below are some measures to trace an unintentional infinite loop:
- We should examine the semicolons carefully. Sometimes we put the semicolon at the wrong place, which leads to the infinite loop.
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- While(i<=10);
- {
- Printf("%d", i);
- i++;
- }
- Return 0;
- }
In the above code, we put the semicolon after the condition of the while loop which leads to the infinite loop. Due to this semicolon, the internal body of the while loop will not execute.
- We should check the logical conditions carefully. Sometimes by mistake, we place the assignment operator (=) instead of a relational operator (= =).
- #include <stdio.h>
- Int main()
- {
- Char ch='n';
- While(ch='y')
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we use the assignment operator (ch='y') which leads to the execution of loop infinite number of times.
- We use the wrong loop condition which causes the loop to be executed indefinitely.
- #include <stdio.h>
- Int main()
- {
- For(int i=1;i>=1;i++)
- {
- Printf("hello");
- }
- Return 0;
- }
The above code will execute the 'for loop' infinite number of times. As we put the condition (i>=1), which will always be true for every condition, it means that "hello" will be printed infinitely.
- We should be careful when we are using the break keyword in the nested loop because it will terminate the execution of the nearest loop, not the entire loop.
- #include <stdio.h>
- Int main()
- {
- While(1)
- {
- For(int i=1;i<=10;i++)
- {
- If(i%2==0)
- {
- Break;
- }
- }
- }
- Return 0;
- }
In the above code, the while loop will be executed an infinite number of times as we use the break keyword in an inner loop. This break keyword will bring the control out of the inner loop, not from the outer loop.
- We should be very careful when we are using the floating-point value inside the loop as we cannot underestimate the floating-point errors.
- #include <stdio.h>
- Int main()
- {
- Float x = 3.0;
- While (x != 4.0) {
- Printf("x = %f\n", x);
- x += 0.1;
- }
- Return 0;
- }
In the above code, the loop will run infinite times as the computer represents a floating-point value as a real value. The computer will represent the value of 4.0 as 3.999999 or 4.000001, so the condition (x !=4.0) will never be false. The solution to this problem is to write the condition as (k<=4.0).
One dimensional Array
The variable allows us to store a single value at a time, what if we want to store roll no. Of 100 students? For this task, we have to declare 100 variables, then assign values to each of them. What if there are 10000 students or more? As you can see declaring that many variables for a single entity (i.e student) is not a good idea. In a situation like these arrays provide a better way to store data.
What is an Array?
An array is a collection of one or more values of the same type. Each value is called an element of the array. The elements of the array share the same variable name but each element has its own unique index number (also known as a subscript). An array can be of any type, For example: int, float, char etc. If an array is of type int then it's elements must be of type int only.
To store roll no. Of 100 students, we have to declare an array of size 100 i.e roll_no[100]. Here size of the array is 100 , so it is capable of storing 100 values. In C, index or subscript starts from 0, so roll_no[0] is the first element, roll_no[1] is the second element and so on. Note that the last element of the array will be at roll_no[99] not at roll_no[100] because the index starts at 0.
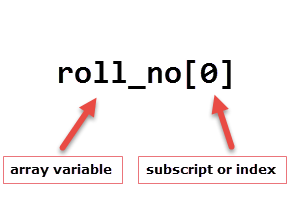
Arrays can be single or multidimensional. The number of subscript or index determines the dimensions of the array. An array of one dimension is known as a one-dimensional array or 1-D array, while an array of two dimensions is known as a two-dimensional array or 2-D array.
Let's start with a one-dimensional array.
One-dimensional array
Conceptually you can think of a one-dimensional array as a row, where elements are stored one after another.
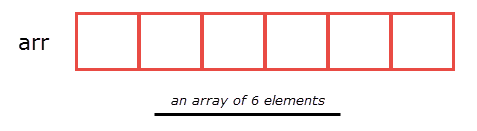
Syntax: datatype array_name[size];
Datatype: It denotes the type of the elements in the array.
Array_name: Name of the array. It must be a valid identifier.
Size: Number of elements an array can hold. Here are some example of array declarations:
1 2 3 | Int num[100]; Float temp[20]; Char ch[50]; |
Num is an array of type int, which can only store 100 elements of type int.
temp is an array of type float, which can only store 20 elements of type float.
ch is an array of type char, which can only store 50 elements of type char.
Note: When an array is declared it contains garbage values.
The individual elements in the array:
1 2 3 | Num[0], num[1], num[2], ....., num[99] Temp[0], temp[1], temp[2], ....., temp[19] Ch[0], ch[1], ch[2], ....., ch[49] |
We can also use variables and symbolic constants to specify the size of the array.
1 2 3 4 5 6 7 8 9 10 | #define SIZE 10
Int main() { Int size = 10;
Int my_arr1[SIZE]; // ok Int my_arr2[size]; // not allowed until C99 // ... } |
Note: Until C99 standard, we were not allowed to use variables to specify the size of the array. If you are using a compiler which supports C99 standard, the above code would compile successfully. However, If you're using an older version of C compiler like Turbo C++, then you will get an error.
The use of symbolic constants makes the program maintainable, because later if you want to change the size of the array you need to modify it at once place only i.e in the #define directive.
Accessing elements of an array
The elements of an array can be accessed by specifying array name followed by subscript or index inside square brackets (i.e []). Array subscript or index starts at 0. If the size of an array is 10 then the first element is at index 0, while the last element is at index 9. The first valid subscript (i.e 0) is known as the lower bound, while last valid subscript is known as the upper bound.
Int my_arr[5];
Then elements of this array are;
First element – my_arr[0]
Second element – my_arr[1]
Third element – my_arr[2]
Fourth element – my_arr[3]
Fifth element – my_arr[4]
Array subscript or index can be any expression that yields an integer value. For example:
1 2 3 4 | Int i = 0, j = 2; My_arr[i]; // 1st element My_arr[i+1]; // 2nd element My_arr[i+j]; // 3rd element |
In the array my_arr, the last element is at my_arr[4], What if you try to access elements beyond the last valid index of the array?
1 2 3 | Printf("%d", my_arr[5]); // 6th element Printf("%d", my_arr[10]); // 11th element Printf("%d", my_arr[-1]); // element just before 0 |
Sure indexes 5, 10 and -1 are not valid but C compiler will not show any error message instead some garbage value will be printed. The C language doesn't check bounds of the array. It is the responsibility of the programmer to check array bounds whenever required.
Processing 1-D arrays
The following program uses for loop to take input and print elements of a 1-D array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include<stdio.h>
Int main() { Int arr[5], i;
For(i = 0; i < 5; i++) { Printf("Enter a[%d]: ", i); Scanf("%d", &arr[i]); }
Printf("\nPrinting elements of the array: \n\n");
For(i = 0; i < 5; i++) { Printf("%d ", arr[i]); }
// signal to operating system program ran fine Return 0; } |
Expected Output:
1 2 3 4 5 6 7 8 9 | Enter a[0]: 11 Enter a[1]: 22 Enter a[2]: 34 Enter a[3]: 4 Enter a[4]: 34
Printing elements of the array:
11 22 34 4 34 |
How it works:
In Line 5, we have declared an array of 5 integers and variable i of type int. Then a for loop is used to enter five elements into an array. In scanf() we have used & operator (also known as the address of operator) on element arr[i] of an array, just like we had done with variables of type int, float, char etc. Line 13 prints "Printing elements of the array" to the console. The second for loop prints all the elements of an array one by one.
The following program prints the sum of elements of an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #include<stdio.h>
Int main() { Int arr[5], i, s = 0;
For(i = 0; i < 5; i++) { Printf("Enter a[%d]: ", i); Scanf("%d", &arr[i]); }
For(i = 0; i < 5; i++) { s += arr[i]; }
Printf("\nSum of elements = %d ", s);
// signal to operating system program ran fine Return 0; } |
Expected Output:
1 2 3 4 5 6 7 | Enter a[0]: 22 Enter a[1]: 33 Enter a[2]: 56 Enter a[3]: 73 Enter a[4]: 23
Sum of elements = 207 |
How it works: The first for loop asks the user to enter five elements into the array. The second for loop reads all the elements of an array one by one and accumulate the sum of all the elements in the variable s. Note that it is necessary to initialize the variable s to 0, otherwise, we will get the wrong answer because of the garbage value of s.
Initializing Array
When an array is declared inside a function the elements of the array have garbage value. If an array is global or static, then its elements are automatically initialized to 0. We can explicitly initialize elements of an array at the time of declaration using the following syntax:
Syntax: datatype array_name[size] = { val1, val2, val3, ..... ValN };
Datatype is the type of elements of an array.
Array_name is the variable name, which must be any valid identifier.
Size is the size of the array.
Val1, val2 ... Are the constants known as initializers. Each value is separated by a comma(,) and then there is a semi-colon (;) after the closing curly brace (}).
Here is are some examples:
1 2 3 | Float temp[5] = {12.3, 4.1, 3.8, 9.5, 4.5}; // an array of 5 floats
Int arr[9] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; // an array of 9 ints |
While initializing 1-D array it is optional to specify the size of the array, so you can also write the above statements as:
1 2 3 | Float temp[] = {12.3, 4.1, 3.8, 9.5, 4.5}; // an array of 5 floats
Int arr[] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; // an array of 9 ints |
If the number of initializers is less than the specified size then the remaining elements of the array are assigned a value of 0.
Float temp[5] = {12.3, 4.1};
Here the size of temp array is 5 but there are only two initializers. After this initialization the elements of the array are as follows:
Temp[0] is 12.3
temp[1] is 4.1
temp[2] is 0
temp[3] is 0
temp[4] is 0
If the number of initializers is greater than the size of the array then, the compiler will report an error. For example:
Int num[5] = {1, 2, 3, 4, 5, 6, 7, 8} // error
The following program finds the highest and lowest elements in an array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 | #include<stdio.h> #define SIZE 10
Int main() { Int my_arr[SIZE] = {34,56,78,15,43,71,89,34,70,91}; Int i, max, min;
Max = min = my_arr[0];
For(i = 0; i < SIZE; i++) { // if value of current element is greater than previous value // then assign new value to max If(my_arr[i] > max) { Max = my_arr[i]; }
// if the value of current element is less than previous element // then assign new value to min If(my_arr[i] < min) { Min = my_arr[i]; } }
Printf("Lowest value = %d\n", min); Printf("Highest value = %d", max);
// signal to operating system everything works fine Return 0; } |
Expected Output:
1 2 | Lowest value = 15 Highest value = 91 |
How it works: In line 6, first, we have declared and initialized an array of 10 integers. In the next line, we have declared three more variables of type int namely: i, max and min. In line 9, we have assigned the value of the first element of my_arr to max and min. A for loop is used to iterate through all the elements of an array. Inside the for loop, the first if condition (my_arr[i] > max) checks whether the current element is greater than max, if it is, we assign the value of the current element to max.
The second if statement checks whether the value of the current element is smaller than the value of min. If it is, we assign the value of the current element to min. This process continues until there are elements in the array left to iterate.
When the process is finished, max and min variables will have maximum and minimum values respectively.
Two dimensional Array
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns. However, 2D arrays are created to implement a relational database lookalike data structure. It provides ease of holding the bulk of data at once which can be passed to any number of functions wherever required.
Declaration of two dimensional Array in C
The syntax to declare the 2D array is given below.
- Data_type array_name[rows][columns];
Consider the following example.
- Int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Initialization of 2D Array in C
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array. The two-dimensional array can be declared and defined in the following way.
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Two-dimensional array example in C
- #include<stdio.h>
- Int main(){
- Int i=0,j=0;
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
- //traversing 2D array
- For(i=0;i<4;i++){
- For(j=0;j<3;j++){
- Printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
- }//end of j
- }//end of i
- Return 0;
- }
Output
Arr[0][0] = 1
Arr[0][1] = 2
Arr[0][2] = 3
Arr[1][0] = 2
Arr[1][1] = 3
Arr[1][2] = 4
Arr[2][0] = 3
Arr[2][1] = 4
Arr[2][2] = 5
Arr[3][0] = 4
Arr[3][1] = 5
Arr[3][2] = 6
C 2D array example: Storing elements in a matrix and printing it.
- #include <stdio.h>
- Void main ()
- {
- Int arr[3][3],i,j;
- For (i=0;i<3;i++)
- {
- For (j=0;j<3;j++)
- {
- Printf("Enter a[%d][%d]: ",i,j);
- Scanf("%d",&arr[i][j]);
- }
- }
- Printf("\n printing the elements ....\n");
- For(i=0;i<3;i++)
- {
- Printf("\n");
- For (j=0;j<3;j++)
- {
- Printf("%d\t",arr[i][j]);
- }
- }
- }
Output
Enter a[0][0]: 56
Enter a[0][1]: 10
Enter a[0][2]: 30
Enter a[1][0]: 34
Enter a[1][1]: 21
Enter a[1][2]: 34
Enter a[2][0]: 45
Enter a[2][1]: 56
Enter a[2][2]: 78
Printing the elements ....
56 10 30
34 21 34
45 56 78
A string is a collection of characters, stored in an array followed by null ('\0') character. Null character represents the end of string.
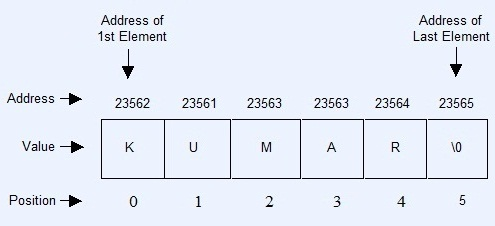
Address of first element is random, address of next element depend upon the type of array. Here, the type is character and character takes one byte in memory, therefore every next address will increment by one.
Index of array will always starts with zero.
Declaration of String or Character array
Declaration of array means creating sequential bolcks of memory to hold fixed number of values.
Syntax for string declaration :
Char String-name[size of String ];
Example for string declaration :
Char String [25]; //Statement 1
In the above example we have declared a character array which can hold twenty-five characters at a time.
Initialization of String
Initialization of string means assigning value to declared character array or string.
Examples 1 :Using sequence of characters.
Char String [ ] = {'H','e','l','l','o',' ','W','o','r','l','d','\0'};
In the above example, we are declaring and initializing a string at same time. When we declare and initialize a string at same time, giving the size of array is optional and it is programmer's job to specify the null character at the end to terminate the string.
Example 2 : Using assignment operator
Char String [ ] = "Hello World";
In this approch, programmer doesn't need to provide null character, compiler will automatically add null character at the end of string.
Input string using getche() function
The getche() read characters from console (output window) one by one and put these characters into string using loop.
Examples for input string with getche() function
#include<stdio.h>
Void main()
{
Char String [50];
Char ch;
Int i;
Printf("\n\n\tEnter your name : ");
For(i=0;i<50;i++)
{
Ch = getche(); //Statement 1
If(ch==13) //Statement 2
Break;
String [i] = ch;
}
String [i] = '\0'; //Statement 3
Printf("\n\n\tHello, ");
For(i=0; String [i]!='\0';i++)
Printf("%c", String [i]);
}
Output :
Enter your name : Kumar
Hello Kumar
In the above example, A for loop will execute upto 50 time and getche() which inside for loop will read single character from console and put the character into ch. If user press the enter key, condition given in statement 2 will satisfy and terminate the loop otherwise every character will assign to String. Statement 4 is assigning null character to String.
Input string using scanf() function
The scanf() can read the entire word at a time. The format specifier for a string is "%s". The scanf() ignores the any occurrence of leading whitespace characters and terminate the string at the first occurrence of whitespace after any input.
Examples for input string with scanf() function
#include<stdio.h>
Void main()
{
Char String [50];
Printf("\n\n\tEnter your name : ");
Scanf("%s", String );
Printf("\n\n\tHello %s", String );
}
Output :
Enter your name : Kumar Wadhwa
Hello Kumar
In the output of above example there is five leading whitespaces in the input which is ignored by the compiler.
Input string using gets() function
The gets() can read the entire line at a time and the string will not terminate until user press the enter key. The gets() will put all the leading and trailing whitespaces into str.
Examples for input string with gets() function
#include<stdio.h>
Void main()
{
Char String [50];
Printf("\n\n\tEnter your name : ");
Gets( String );
Printf("\n\n\tHello %s", String );
}
Output :
Enter your name : Kumar Wadhwa
Hello Kumar Wadhwa
In the output of above example there is five leading whitespaces in the input which is not ignored by the compiler.
Reference:
1. Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill
2. A.K. Sharma, Computer Fundamentals and Programming in C, Universities Press, 2nd Edition, 2018.
3. E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
4. Brian W. Kernighan and Dennis M. Ritchie, the C Programming Language, Prentice Hall of India.