UNIT 5
Pointers
The pointer in C language is a variable which stores the address of another variable. This variable can be of type int, char, array, function, or any other pointer. The size of the pointer depends on the architecture. However, in 32-bit architecture the size of a pointer is 2 byte.
Consider the following example to define a pointer which stores the address of an integer.
- Int n = 10;
- Int* p = &n; // Variable p of type pointer is pointing to the address of the variable n of type integer.
Declaring a pointer
The pointer in c language can be declared using * (asterisk symbol). It is also known as indirection pointer used to dereference a pointer.
- Int *a;//pointer to int
- Char *c;//pointer to char
Pointer Example
An example of using pointers to print the address and value is given below.
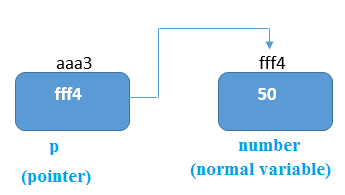
As you can see in the above figure, pointer variable stores the address of number variable, i.e., fff4. The value of number variable is 50. But the address of pointer variable p is aaa3.
By the help of * (indirection operator), we can print the value of pointer variable p.
Let's see the pointer example as explained for the above figure.
- #include<stdio.h>
- Int main(){
- Int number=50;
- Int *p;
- p=&number;//stores the address of number variable
- Printf("Address of p variable is %x \n",p); // p contains the address of the number therefore printing p gives the address of number.
- Printf("Value of p variable is %d \n",*p); // As we know that * is used to dereference a pointer therefore if we print *p, we will get the value stored at the address contained by p.
- Return 0;
- }
Output
Address of number variable is fff4
Address of p variable is fff4
Value of p variable is 50
Pointer to array
- Int arr[10];
- Int *p[10]=&arr; // Variable p of type pointer is pointing to the address of an integer array arr.
Pointer to a function
- Void show (int);
- Void(*p)(int) = &display; // Pointer p is pointing to the address of a function
Pointer to structure
- Struct st {
- Int i;
- Float f;
- }ref;
- Struct st *p = &ref;
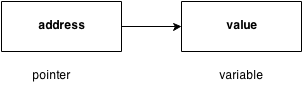
Advantage of pointer
1) Pointer reduces the code and improves the performance, it is used to retrieving strings, trees, etc. and used with arrays, structures, and functions.
2) We can return multiple values from a function using the pointer.
3) It makes you able to access any memory location in the computer's memory.
Usage of pointer
There are many applications of pointers in c language.
1) Dynamic memory allocation
In c language, we can dynamically allocate memory using malloc() and calloc() functions where the pointer is used.
2) Arrays, Functions, and Structures
Pointers in c language are widely used in arrays, functions, and structures. It reduces the code and improves the performance.
Address Of (&) Operator
The address of operator '&' returns the address of a variable. But, we need to use %u to display the address of a variable.
- #include<stdio.h>
- Int main(){
- Int number=50;
- Printf("value of number is %d, address of number is %u",number,&number);
- Return 0;
- }
Output
Value of number is 50, address of number is fff4
NULL Pointer
A pointer that is not assigned any value but NULL is known as the NULL pointer. If you don't have any address to be specified in the pointer at the time of declaration, you can assign NULL value. It will provide a better approach.
Int *p=NULL;
In the most libraries, the value of the pointer is 0 (zero).
Pointer Program to swap two numbers without using the 3rd variable.
- #include<stdio.h>
- Int main(){
- Int a=10,b=20,*p1=&a,*p2=&b;
- Printf("Before swap: *p1=%d *p2=%d",*p1,*p2);
- *p1=*p1+*p2;
- *p2=*p1-*p2;
- *p1=*p1-*p2;
- Printf("\nAfter swap: *p1=%d *p2=%d",*p1,*p2);
- Return 0;
- }
Output
Before swap: *p1=10 *p2=20
After swap: *p1=20 *p2=10
Reading complex pointers
There are several things which must be taken into the consideration while reading the complex pointers in C. Lets see the precedence and associativity of the operators which are used regarding pointers.
Operator | Precedence | Associativity |
(), [] | 1 | Left to right |
*, identifier | 2 | Right to left |
Data type | 3 | - |
Here,we must notice that,
- (): This operator is a bracket operator used to declare and define the function.
- []: This operator is an array subscript operator
- * : This operator is a pointer operator.
- Identifier: It is the name of the pointer. The priority will always be assigned to this.
- Data type: Data type is the type of the variable to which the pointer is intended to point. It also includes the modifier like signed int, long, etc).
How to read the pointer: int (*p)[10].
To read the pointer, we must see that () and [] have the equal precedence. Therefore, their associativity must be considered here. The associativity is left to right, so the priority goes to ().
Inside the bracket (), pointer operator * and pointer name (identifier) p have the same precedence. Therefore, their associativity must be considered here which is right to left, so the priority goes to p, and the second priority goes to *.
Assign the 3rd priority to [] since the data type has the last precedence. Therefore the pointer will look like following.
- Char -> 4
- * -> 2
- p -> 1
- [10] -> 3
The pointer will be read as p is a pointer to an array of integers of size 10.
Example
How to read the following pointer?
- Int (*p)(int (*)[2], int (*)void))
Explanation
This pointer will be read as p is a pointer to such function which accepts the first parameter as the pointer to a one-dimensional array of integers of size two and the second parameter as the pointer to a function which parameter is void and return type is the integer.
POINTER is a variable that stores address of another variable. A pointer can also be used to refer to another pointer function. A pointer can be incremented/decremented, i.e., to point to the next/ previous memory location. The purpose of pointer is to save memory space and achieve faster execution time.
How does Pointer Work?
If we declare a variable v of type int, v will actually store a value.
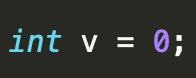
v is equal to zero now.
However, each variable, apart from value, also has its address (or, simply put, where it is located in the memory). The address can be retrieved by putting an ampersand (&) before the variable name.

If you print the address of a variable on the screen, it will look like a totally random number (moreover, it can be different from run to run).
Let's try this in practice.
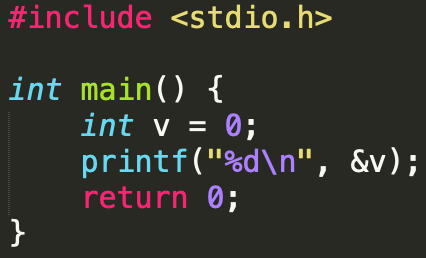
The output of this program is -480613588.
Now, what is a pointer? Instead of storing a value, a pointer will y store the address of a variable.
Int *y = &v;
VARIABLE | POINTER |
A value stored in a named storage/memory address | A variable that points to the storage/memory address of another variable |
What You Will Learn: [show]
Declaring a pointer
Like variables, pointers have to be declared before they can be used in your program. Pointers can be named anything you want as long as they obey C's naming rules. A pointer declaration has the following form.
Data_type * pointer_variable_name;
Here,
- Data_type is the pointer's base type of C's variable types and indicates the type of the variable that the pointer points to.
- The asterisk (*: the same asterisk used for multiplication) which is indirection operator, declares a pointer.
Let's see some valid pointer declarations
Int *ptr_thing; /* pointer to an integer */
Int *ptr1,thing;/* ptr1 is a pointer to type integer and thing is an integer variable */
Double *ptr2; /* pointer to a double */
Float *ptr3; /* pointer to a float */
Char *ch1 ; /* pointer to a character */
Float *ptr, variable;/*ptr is a pointer to type float and variable is an ordinary float variable */
Initialize a pointer
After declaring a pointer, we initialize it like standard variables with a variable address. If pointers are not uninitialized and used in the program, the results are unpredictable and potentially disastrous.
To get the address of a variable, we use the ampersand (&)operator, placed before the name of a variable whose address we need. Pointer initialization is done with the following syntax.
Pointer = &variable;
A simple program for pointer illustration is given below:
#include <stdio.h>
Int main()
{
Int a=10; //variable declaration
Int *p; //pointer variable declaration
p=&a; //store address of variable a in pointer p
Printf("Address stored in a variable p is:%x\n",p); //accessing the address
Printf("Value stored in a variable p is:%d\n",*p); //accessing the value
Return 0;
}
Output:
Address stored in a variable p is:60ff08
Value stored in a variable p is:10
Operator | Meaning |
* | Serves 2 purpose
|
& | Serves only 1 purpose
|
Types of a pointer
Null pointer
We can create a null pointer by assigning null value during the pointer declaration. This method is useful when you do not have any address assigned to the pointer. A null pointer always contains value 0.
Following program illustrates the use of a null pointer:
#include <stdio.h>
Int main()
{
Int *p = NULL; //null pointer
Printf(“The value inside variable p is:\n%x”,p);
Return 0;
}
Output:
The value inside variable p is:
0
Void Pointer
In C programming, a void pointer is also called as a generic pointer. It does not have any standard data type. A void pointer is created by using the keyword void. It can be used to store an address of any variable.
Following program illustrates the use of a void pointer:
#include <stdio.h>
Int main()
{
Void *p = NULL; //void pointer
Printf("The size of pointer is:%d\n",sizeof(p));
Return 0;
}
Output:
The size of pointer is:4
Wild pointer
A pointer is said to be a wild pointer if it is not being initialized to anything. These types of pointers are not efficient because they may point to some unknown memory location which may cause problems in our program and it may lead to crashing of the program. One should always be careful while working with wild pointers.
Following program illustrates the use of wild pointer:
#include <stdio.h>
Int main()
{
Int *p; //wild pointer
Printf("\n%d",*p);
Return 0;
}
Output
Timeout: the monitored command dumped core
Sh: line 1: 95298 Segmentation fault timeout 10s main
Other types of pointers in 'c' are as follows:
- Dangling pointer
- Complex pointer
- Near pointer
- Far pointer
- Huge pointer
Direct and Indirect Access Pointers
In C, there are two equivalent ways to access and manipulate a variable content
- Direct access: we use directly the variable name
- Indirect access: we use a pointer to the variable
Let's understand this with the help of program below
#include <stdio.h>
/* Declare and initialize an int variable */
Int var = 1;
/* Declare a pointer to int */
Int *ptr;
Int main( void )
{
/* Initialize ptr to point to var */
Ptr = &var;
/* Access var directly and indirectly */
Printf("\nDirect access, var = %d", var);
Printf("\nIndirect access, var = %d", *ptr);
/* Display the address of var two ways */
Printf("\n\nThe address of var = %d", &var);
Printf("\nThe address of var = %d\n", ptr);
/*change the content of var through the pointer*/
*ptr=48;
Printf("\nIndirect access, var = %d", *ptr);
Return 0;}
After compiling the program without any errors, the result is:
Direct access, var = 1
Indirect access, var = 1
The address of var = 4202496
The address of var = 4202496
Indirect access, var = 48
Pointers Arithmetic
The pointer operations are summarized in the following figure
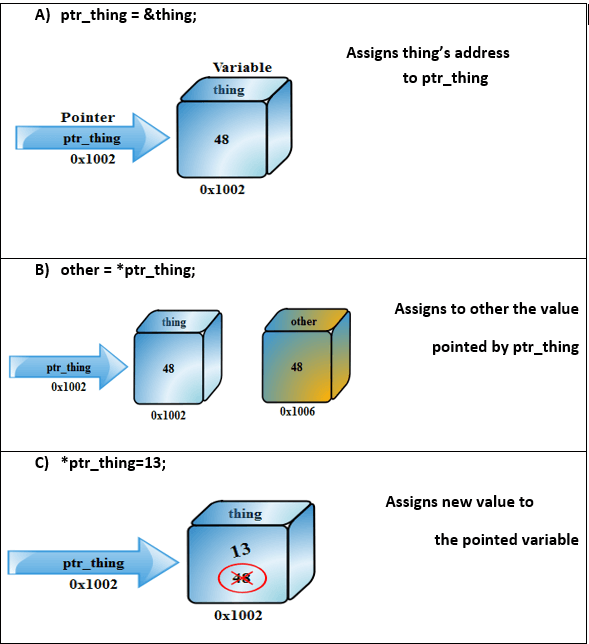
Pointer Operations
Priority operation (precedence)
When working with pointers, we must observe the following priority rules:
- The operators * and & have the same priority as the unary operators (the negation!, the incrementation++, decrement--).
- In the same expression, the unary operators *, &,!, ++, - are evaluated from right to left.
If a P pointer points to an X variable, then * P can be used wherever X can be written.
The following expressions are equivalent:
Int X =10 | |
Expression | Equivalent Expression |
Y=*P+1 | Y=X+1 |
In the latter case, parentheses are needed: as the unary operators * and ++ are evaluated from right to left, without the parentheses the pointer P would be incremented, not the object on which P points.
Below table shows the arithmetic and basic operation that can be used when dealing with pointers
Operation | Explanation |
Assignment | Int *P1,*P2 P1=P2; P1 and P2 point to the same integer variable |
Incrementation and decrementation | Int *P1; P1++;P1-- ; |
Adding an offset (Constant) | This allows the pointer to move N elements in a table. The pointer will be increased or decreased by N times the number of byte (s) of the type of the variable. P1+5; |
Pointers and Arrays
Traditionally, we access the array elements using its index, but this method can be eliminated by using pointers. Pointers make it easy to access each array element.
#include <stdio.h>
Int main()
{
Int a[5]={1,2,3,4,5}; //array initialization
Int *p; //pointer declaration
/*the ptr points to the first element of the array*/
p=a; /*We can also type simply ptr==&a[0] */
Printf("Printing the array elements using pointer\n");
For(int i=0;i<5;i++) //loop for traversing array elements
{
Printf("\n%x",*p); //printing array elements
p++; //incrementing to the next element, you can also write p=p+1
}
Return 0;
}
Output
1
2
3
4
5
Adding a particular number to a pointer will move the pointer location to the value obtained by an addition operation. Suppose p is a pointer that currently points to the memory location 0 if we perform following addition operation, p+1 then it will execute in this manner:

Pointer Addition/Increment
Since p currently points to the location 0 after adding 1, the value will become 1, and hence the pointer will point to the memory location 1.
Pointers and Strings
A string is an array of char objects, ending with a null character '\ 0'. We can manipulate strings using pointers. Here is an example that explains this section
#include <stdio.h>
#include <string.h>
Int main()
{
Char str[]="Hello Guru99!";
Char *p;
p=str;
Printf("First character is:%c\n",*p);
p =p+1;
Printf("Next character is:%c\n",*p);
Printf("Printing all the characters in a string\n");
p=str; //reset the pointer
For(int i=0;i<strlen(str);i++)
{
Printf("%c\n",*p);
p++;
}
Return 0;
}
Output
First character is:H
Next character is:e
Printing all the characters in a string
H
e
l
l
o
G
u
r
u
9
9
!
Another way to deal strings is with an array of pointers like in the following program:
#include <stdio.h>
Int main(){
Char *materials[ ] = { "iron", "copper", "gold"};
Printf("Please remember these materials :\n");
Int i ;
For (i = 0; i < 3; i++) {
Printf("%s\n", materials[ i ]);}
Return 0;}
Output:
Please remember these materials:
Iron
Copper
Gold
Advantages of Pointers
- Pointers are useful for accessing memory locations.
- Pointers provide an efficient way for accessing the elements of an array structure.
- Pointers are used for dynamic memory allocation as well as deallocation.
- Pointers are used to form complex data structures such as linked list, graph, tree, etc.
Disadvantages of Pointers
- Pointers are a little complex to understand.
- Pointers can lead to various errors such as segmentation faults or can access a memory location which is not required at all.
- If an incorrect value is provided to a pointer, it may cause memory corruption.
- Pointers are also responsible for memory leakage.
- Pointers are comparatively slower than that of the variables.
- Programmers find it very difficult to work with the pointers; therefore it is programmer's responsibility to manipulate a pointer carefully.
Summary
- A pointer is nothing but a memory location where data is stored.
- A pointer is used to access the memory location.
- There are various types of pointers such as a null pointer, wild pointer, void pointer and other types of pointers.
- Pointers can be used with array and string to access elements more efficiently.
- We can create function pointers to invoke a function dynamically.
- Arithmetic operations can be done on a pointer which is known as pointer arithmetic.
- Pointers can also point to function which make it easy to call different functions in the case of defining an array of pointers.
- When you want to deal different variable data type, you can use a typecast void pointer.
Self-Referential structures are those structures that have one or more pointers which point to the same type of structure, as their member.
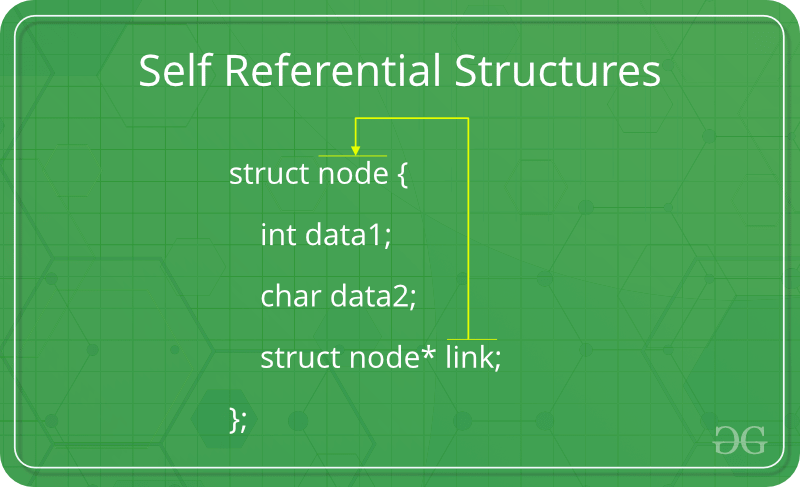
In other words, structures pointing to the same type of structures are self-referential in nature.
Example:
Struct node { Int data1; Char data2; Struct node* link; };
Int main() { Struct node ob; Return 0; } |
In the above example ‘link’ is a pointer to a structure of type ‘node’. Hence, the structure ‘node’ is a self-referential structure with ‘link’ as the referencing pointer.
An important point to consider is that the pointer should be initialized properly before accessing, as by default it contains garbage value.
Types of Self Referential Structures
- Self-Referential Structure with Single Link
- Self-Referential Structure with Multiple Links
Self-Referential Structure with Single Link: These structures can have only one self-pointer as their member. The following example will show us how to connect the objects of a self-referential structure with the single link and access the corresponding data members. The connection formed is shown in the following figure.
#include <stdio.h>
Struct node { Int data1; Char data2; Struct node* link; };
Int main() { Struct node ob1; // Node1
// Initialization Ob1.link = NULL; Ob1.data1 = 10; Ob1.data2 = 20;
Struct node ob2; // Node2
// Initialization Ob2.link = NULL; Ob2.data1 = 30; Ob2.data2 = 40;
// Linking ob1 and ob2 Ob1.link = &ob2;
// Accessing data members of ob2 using ob1 Printf("%d", ob1.link->data1); Printf("\n%d", ob1.link->data2); Return 0; } |
Output:
30
40
Self-Referential Structure with Multiple Links: Self-referential structures with multiple links can have more than one self-pointers. Many complicated data structures can be easily constructed using these structures. Such structures can easily connect to more than one nodes at a time. The following example shows one such structure with more than one links.
The connections made in the above example can be understood using the following figure.
#include <stdio.h>
Struct node { Int data; Struct node* prev_link; Struct node* next_link; };
Int main() { Struct node ob1; // Node1
// Initialization Ob1.prev_link = NULL; Ob1.next_link = NULL; Ob1.data = 10;
Struct node ob2; // Node2
// Initialization Ob2.prev_link = NULL; Ob2.next_link = NULL; Ob2.data = 20;
Struct node ob3; // Node3
// Initialization Ob3.prev_link = NULL; Ob3.next_link = NULL; Ob3.data = 30;
// Forward links Ob1.next_link = &ob2; Ob2.next_link = &ob3;
// Backward links Ob2.prev_link = &ob1; Ob3.prev_link = &ob2;
// Accessing data of ob1, ob2 and ob3 by ob1 Printf("%d\t", ob1.data); Printf("%d\t", ob1.next_link->data); Printf("%d\n", ob1.next_link->next_link->data);
// Accessing data of ob1, ob2 and ob3 by ob2 Printf("%d\t", ob2.prev_link->data); Printf("%d\t", ob2.data); Printf("%d\n", ob2.next_link->data);
// Accessing data of ob1, ob2 and ob3 by ob3 Printf("%d\t", ob3.prev_link->prev_link->data); Printf("%d\t", ob3.prev_link->data); Printf("%d", ob3.data); Return 0; } |
Output:
10 20 30
10 20 30
10 20 30
In the above example we can see that ‘ob1’, ‘ob2’ and ‘ob3’ are three objects of the self-referential structure ‘node’. And they are connected using their links in such a way that any of them can easily access each other’s data. This is the beauty of the self-referential structures. The connections can be manipulated according to the requirements of the programmer.
Applications:
Self-referential structures are very useful in creation of other complex data structures like:
- Linked lists
- Stacks
- Queues
- Trees
- Graphs etc.
A linked list is a sequence of data structures, which are connected together via links. Linked List is a sequence of links which contains items. Each link contains a connection to another link. Linked list is the second most-used data structure after array.
Implementation in C
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <stdbool.h>
Struct node {
Int data;
Int key;
Struct node *next;
};
Struct node *head = NULL;
Struct node *current = NULL;
//display the list
Void printList() {
Struct node *ptr = head;
Printf("\n[ ");
//start from the beginning
While(ptr != NULL) {
Printf("(%d,%d) ",ptr->key,ptr->data);
Ptr = ptr->next;
}
Printf(" ]");
}
//insert link at the first location
Void insertFirst(int key, int data) {
//create a link
Struct node *link = (struct node*) malloc(sizeof(struct node));
Link->key = key;
Link->data = data;
//point it to old first node
Link->next = head;
//point first to new first node
Head = link;
}
//delete first item
Struct node* deleteFirst() {
//save reference to first link
Struct node *tempLink = head;
//mark next to first link as first
Head = head->next;
//return the deleted link
Return tempLink;
}
//is list empty
Bool isEmpty() {
Return head == NULL;
}
Int length() {
Int length = 0;
Struct node *current;
For(current = head; current != NULL; current = current->next) {
Length++;
}
Return length;
}
//find a link with given key
Struct node* find(int key) {
//start from the first link
Struct node* current = head;
//if list is empty
If(head == NULL) {
Return NULL;
}
//navigate through list
While(current->key != key) {
//if it is last node
If(current->next == NULL) {
Return NULL;
} else {
//go to next link
Current = current->next;
}
}
//if data found, return the current Link
Return current;
}
//delete a link with given key
Struct node* delete(int key) {
//start from the first link
Struct node* current = head;
Struct node* previous = NULL;
//if list is empty
If(head == NULL) {
Return NULL;
}
//navigate through list
While(current->key != key) {
//if it is last node
If(current->next == NULL) {
Return NULL;
} else {
//store reference to current link
Previous = current;
//move to next link
Current = current->next;
}
}
//found a match, update the link
If(current == head) {
//change first to point to next link
Head = head->next;
} else {
//bypass the current link
Previous->next = current->next;
}
Return current;
}
Void sort() {
Int i, j, k, tempKey, tempData;
Struct node *current;
Struct node *next;
Int size = length();
k = size ;
For ( i = 0 ; i < size - 1 ; i++, k-- ) {
Current = head;
Next = head->next;
For ( j = 1 ; j < k ; j++ ) {
If ( current->data > next->data ) {
TempData = current->data;
Current->data = next->data;
Next->data = tempData;
TempKey = current->key;
Current->key = next->key;
Next->key = tempKey;
}
Current = current->next;
Next = next->next;
}
}
}
Void reverse(struct node** head_ref) {
Struct node* prev = NULL;
Struct node* current = *head_ref;
Struct node* next;
While (current != NULL) {
Next = current->next;
Current->next = prev;
Prev = current;
Current = next;
}
*head_ref = prev;
}
Void main() {
InsertFirst(1,10);
InsertFirst(2,20);
InsertFirst(3,30);
InsertFirst(4,1);
InsertFirst(5,40);
InsertFirst(6,56);
Printf("Original List: ");
//print list
PrintList();
While(!isEmpty()) {
Struct node *temp = deleteFirst();
Printf("\nDeleted value:");
Printf("(%d,%d) ",temp->key,temp->data);
}
Printf("\nList after deleting all items: ");
PrintList();
InsertFirst(1,10);
InsertFirst(2,20);
InsertFirst(3,30);
InsertFirst(4,1);
InsertFirst(5,40);
InsertFirst(6,56);
Printf("\nRestored List: ");
PrintList();
Printf("\n");
Struct node *foundLink = find(4);
If(foundLink != NULL) {
Printf("Element found: ");
Printf("(%d,%d) ",foundLink->key,foundLink->data);
Printf("\n");
} else {
Printf("Element not found.");
}
Delete(4);
Printf("List after deleting an item: ");
PrintList();
Printf("\n");
FoundLink = find(4);
If(foundLink != NULL) {
Printf("Element found: ");
Printf("(%d,%d) ",foundLink->key,foundLink->data);
Printf("\n");
} else {
Printf("Element not found.");
}
Printf("\n");
Sort();
Printf("List after sorting the data: ");
PrintList();
Reverse(&head);
Printf("\nList after reversing the data: ");
PrintList();
}
If we compile and run the above program, it will produce the following result −
Output
Original List:
[ (6,56) (5,40) (4,1) (3,30) (2,20) (1,10) ]
Deleted value:(6,56)
Deleted value:(5,40)
Deleted value:(4,1)
Deleted value:(3,30)
Deleted value:(2,20)
Deleted value:(1,10)
List after deleting all items:
[ ]
Restored List:
[ (6,56) (5,40) (4,1) (3,30) (2,20) (1,10) ]
Element found: (4,1)
List after deleting an item:
[ (6,56) (5,40) (3,30) (2,20) (1,10) ]
Element not found.
List after sorting the data:
[ (1,10) (2,20) (3,30) (5,40) (6,56) ]
List after reversing the data:
[ (6,56) (5,40) (3,30) (2,20) (1,10) ]
In programming, we may require some specific input data to be generated several numbers of times. Sometimes, it is not enough to only display the data on the console. The data to be displayed may be very large, and only a limited amount of data can be displayed on the console, and since the memory is volatile, it is impossible to recover the programmatically generated data again and again. However, if we need to do so, we may store it onto the local file system which is volatile and can be accessed every time. Here, comes the need of file handling in C.
File handling in C enables us to create, update, read, and delete the files stored on the local file system through our C program. The following operations can be performed on a file.
- Creation of the new file
- Opening an existing file
- Reading from the file
- Writing to the file
- Deleting the file
Functions for file handling
There are many functions in the C library to open, read, write, search and close the file. A list of file functions are given below:
No. | Function | Description |
1 | Fopen() | Opens new or existing file |
2 | Fprintf() | Write data into the file |
3 | Fscanf() | Reads data from the file |
4 | Fputc() | Writes a character into the file |
5 | Fgetc() | Reads a character from file |
6 | Fclose() | Closes the file |
7 | Fseek() | Sets the file pointer to given position |
8 | Fputw() | Writes an integer to file |
9 | Fgetw() | Reads an integer from file |
10 | Ftell() | Returns current position |
11 | Rewind() | Sets the file pointer to the beginning of the file |
Opening File: fopen()
We must open a file before it can be read, write, or update. The fopen() function is used to open a file. The syntax of the fopen() is given below.
- FILE *fopen( const char * filename, const char * mode );
The fopen() function accepts two parameters:
- The file name (string). If the file is stored at some specific location, then we must mention the path at which the file is stored. For example, a file name can be like "c://some_folder/some_file.ext".
- The mode in which the file is to be opened. It is a string.
We can use one of the following modes in the fopen() function.
Mode | Description |
r | Opens a text file in read mode |
w | Opens a text file in write mode |
a | Opens a text file in append mode |
r+ | Opens a text file in read and write mode |
w+ | Opens a text file in read and write mode |
a+ | Opens a text file in read and write mode |
Rb | Opens a binary file in read mode |
Wb | Opens a binary file in write mode |
Ab | Opens a binary file in append mode |
Rb+ | Opens a binary file in read and write mode |
Wb+ | Opens a binary file in read and write mode |
Ab+ | Opens a binary file in read and write mode |
The fopen function works in the following way.
- Firstly, It searches the file to be opened.
- Then, it loads the file from the disk and place it into the buffer. The buffer is used to provide efficiency for the read operations.
- It sets up a character pointer which points to the first character of the file.
Consider the following example which opens a file in write mode.
- #include<stdio.h>
- Void main( )
- {
- FILE *fp ;
- Char ch ;
- Fp = fopen("file_handle.c","r") ;
- While ( 1 )
- {
- Ch = fgetc ( fp ) ;
- If ( ch == EOF )
- Break ;
- Printf("%c",ch) ;
- }
- Fclose (fp ) ;
- }
Output
The content of the file will be printed.
#include;
Void main( )
{
FILE *fp; // file pointer
Char ch;
Fp = fopen("file_handle.c","r");
While ( 1 )
{
Ch = fgetc ( fp ); //Each character of the file is read and stored in the character file.
If ( ch == EOF )
Break;
Printf("%c",ch);
}
Fclose (fp );
}
Closing File: fclose()
The fclose() function is used to close a file. The file must be closed after performing all the operations on it. The syntax of fclose() function is given below:
- Int fclose( FILE *fp );
C fprintf() and fscanf()
C fprintf() and fscanf() example
Writing File : fprintf() function
The fprintf() function is used to write set of characters into file. It sends formatted output to a stream.
Syntax:
- Int fprintf(FILE *stream, const char *format [, argument, ...])
Example:
- #include <stdio.h>
- Main(){
- FILE *fp;
- Fp = fopen("file.txt", "w");//opening file
- Fprintf(fp, "Hello file by fprintf...\n");//writing data into file
- Fclose(fp);//closing file
- }
Reading File : fscanf() function
The fscanf() function is used to read set of characters from file. It reads a word from the file and returns EOF at the end of file.
Syntax:
- Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
- #include <stdio.h>
- Main(){
- FILE *fp;
- Char buff[255];//creating char array to store data of file
- Fp = fopen("file.txt", "r");
- While(fscanf(fp, "%s", buff)!=EOF){
- Printf("%s ", buff );
- }
- Fclose(fp);
- }
Output:
Hello file by fprintf...
C File Example: Storing employee information
Let's see a file handling example to store employee information as entered by user from console. We are going to store id, name and salary of the employee.
- #include <stdio.h>
- Void main()
- {
- FILE *fptr;
- Int id;
- Char name[30];
- Float salary;
- Fptr = fopen("emp.txt", "w+");/* open for writing */
- If (fptr == NULL)
- {
- Printf("File does not exists \n");
- Return;
- }
- Printf("Enter the id\n");
- Scanf("%d", &id);
- Fprintf(fptr, "Id= %d\n", id);
- Printf("Enter the name \n");
- Scanf("%s", name);
- Fprintf(fptr, "Name= %s\n", name);
- Printf("Enter the salary\n");
- Scanf("%f", &salary);
- Fprintf(fptr, "Salary= %.2f\n", salary);
- Fclose(fptr);
- }
Output:
Enter the id
1
Enter the name
Sonoo
Enter the salary
120000
Now open file from current directory. For windows operating system, go to TC\bin directory, you will see emp.txt file. It will have following information.
Emp.txt
Id= 1
Name= sonoo
Salary= 120000
C fputc() and fgetc()
C fputc() and fgetc() example
Writing File : fputc() function
The fputc() function is used to write a single character into file. It outputs a character to a stream.
Syntax:
- Int fputc(int c, FILE *stream)
Example:
- #include <stdio.h>
- Main(){
- FILE *fp;
- Fp = fopen("file1.txt", "w");//opening file
- Fputc('a',fp);//writing single character into file
- Fclose(fp);//closing file
- }
File1.txt
a
Reading File : fgetc() function
The fgetc() function returns a single character from the file. It gets a character from the stream. It returns EOF at the end of file.
Syntax:
- Int fgetc(FILE *stream)
Example:
- #include<stdio.h>
- #include<conio.h>
- Void main(){
- FILE *fp;
- Char c;
- Clrscr();
- Fp=fopen("myfile.txt","r");
- While((c=fgetc(fp))!=EOF){
- Printf("%c",c);
- }
- Fclose(fp);
- Getch();
- }
Myfile.txt
This is simple text message
C fputs() and fgets()
C fputs() and fgets() example
The fputs() and fgets() in C programming are used to write and read string from stream. Let's see examples of writing and reading file using fgets() and fgets() functions.
Writing File : fputs() function
The fputs() function writes a line of characters into file. It outputs string to a stream.
Syntax:
- Int fputs(const char *s, FILE *stream)
Example:
- #include<stdio.h>
- #include<conio.h>
- Void main(){
- FILE *fp;
- Clrscr();
- Fp=fopen("myfile2.txt","w");
- Fputs("hello c programming",fp);
- Fclose(fp);
- Getch();
- }
Myfile2.txt
Hello c programming
Reading File : fgets() function
The fgets() function reads a line of characters from file. It gets string from a stream.
Syntax:
- Char* fgets(char *s, int n, FILE *stream)
Example:
- #include<stdio.h>
- #include<conio.h>
- Void main(){
- FILE *fp;
- Char text[300];
- Clrscr();
- Fp=fopen("myfile2.txt","r");
- Printf("%s",fgets(text,200,fp));
- Fclose(fp);
- Getch();
- }
Output:
Hello c programming
C fseek()
C fseek() example
The fseek() function is used to set the file pointer to the specified offset. It is used to write data into file at desired location.
Syntax:
- Int fseek(FILE *stream, long int offset, int whence)
There are 3 constants used in the fseek() function for whence: SEEK_SET, SEEK_CUR and SEEK_END.
Example:
- #include <stdio.h>
- Void main(){
- FILE *fp;
- Fp = fopen("myfile.txt","w+");
- Fputs("This is javatpoint", fp);
- Fseek( fp, 7, SEEK_SET );
- Fputs("sonoo jaiswal", fp);
- Fclose(fp);
- }
Myfile.txt
This is sonoo jaiswal
Reference:
1. Byron Gottfried, Schaum's Outline of Programming with C, McGraw-Hill
2. A.K. Sharma, Computer Fundamentals and Programming in C, Universities Press, 2nd Edition, 2018.
3. E. Balaguruswamy, Programming in ANSI C, Tata McGraw-Hill
4. Brian W. Kernighan and Dennis M. Ritchie, the C Programming Language, Prentice Hall of India.