Unit – 4
Java Servlet
Servlets are server-side components that provide a flexible framework for building server-side web applications. CGI was originally created to provide server-side functionality to web applications. Although CGI was crucial in the Internet's growth, its performance, scalability, and reusability concerns made it a less than ideal alternative. All of that changes with Java Servlets. Java servlets are a server-side processing framework built from the ground up utilizing Sun's write once, run anywhere technology.
Web developers can use servlets to create quick and efficient server-side applications that can be launched on any servlet-capable web server. Servlet is completely contained within the Java Virtual Machine. Because the servlet operates on the server, it is not affected by browser compatibility.
In comparison to CGI, Servlets have a lot of advantages. They are as follows:
- Platform Independent
Because servlets are built entirely in Java, they are platform agnostic. Servlets can run on any web server that supports Servlets. Assuming you construct a web application on a Windows PC using the Java web server, you can easily run it on an Apache web server (if Apache Serve is installed) without having to modify or compile the code. Servlets' platform independence gives them a significant edge over other servlet alternative.
2. Performance
Java programs are slow due to the interpreted nature of the language. The java servlets, on the other hand, are extremely fast. These issues arise as a result of the way servlets operate on a web server. The initialization of any software takes a large amount of time. However, when a servlet receives a request, it is initialized the first time it receives it and remains in memory until it times out or the server shuts down. To handle a new request, the servlet simply spawns a new thread and calls the servlet's service function. Traditional CGI scripts, on the other hand, launch a new process to serve the request.
3. Extensibility
Java Servlets are written in Java, an object-oriented programming language that may be expanded or polymorphed to create new objects. As a result, java servlets take advantage of all of these benefits and can be extended from existing classes to deliver the best possible solutions.
4. Safety
Memory management, exception handling, and other security features are all available in Java. Servlets inherited all of these characteristics and has grown to be a very powerful web server extension.
5. Secure
Because servlets are server-side components, they inherit the web server's security. Java Security Manager is also beneficial to servlets.
Key takeaway
- Servlets are server-side components that provide a flexible framework for building server-side web applications.
- CGI was originally created to provide server-side functionality to web applications.
The Java Enterprise Platform's fundamental web specification is Java Servlet. Developers can use the Servlet API to engage with the request/response cycle in web applications. This project provides information about the Java Servlet specification's ongoing development.
On the Java EE platform, Java Servlets is a JCP Standard technology for interacting with the web. The official Servlet standard problem tracker is hosted on this GitHub repository.
The following Java API Specifications are heavily referenced throughout this specification:
● Java2 Platform Enterprise Edition, v1.2 (J2EE)
● JavaServer PagesTM, v1.1 (JSP)
● Java Naming and Directory Interface (JNDI)
Servlets are Java classes that handle HTTP requests and follow the javax.servlet specification. Interface for servlets. Servlets that extend javax.servlet are commonly written by web application developers. HTTP queries are handled by http.HttpServlet, an abstract class that implements the Servlet interface and is specifically built to handle HTTP requests.
The sample source code structure of a servlet example to demonstrate Hello World is shown below.
// Import required java libraries
Import java.io.*;
Import javax.servlet.*;
Import javax.servlet.http.*;
// Extend HttpServlet class
Public class HelloWorld extends HttpServlet {
private String message;
public void init() throws ServletException {
// Do required initialization
message = "Hello World";
}
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// Set response content type
response.setContentType("text/html");
// Actual logic goes here.
PrintWriter out = response.getWriter();
out.println("<h1>" + message + "</h1>");
}
public void destroy() {
// do nothing.
}
}
Compiling servlet
Let's make a file called HelloWorld.java with the preceding code in it. This file should be saved to C:ServletDevel (in Windows) or /usr/ServletDevel (in Linux) (in Unix). Before continuing, this path location must be added to CLASSPATH.
Assuming your environment is set up correctly, build HelloWorld.java in the ServletDevel directory as follows:
$ javac HelloWorld.java
If the servlet requires other libraries, you must also add those JAR files to your CLASSPATH. Because I'm not utilizing any additional libraries in the Hello World program, I've only included the servlet-api.jar JAR file.
This command line makes use of the Sun Microsystems Java Software Development Kit's built-in javac compiler (JDK). You must include the location of the Java SDK that you are using in the PATH environment variable for this command to work properly.
If everything goes well, the HelloWorld.class file will be created in the same directory.
Key takeaway
- Servlets are Java classes that handle HTTP requests and follow the javax.servlet specification. Interface for servlets.
- Servlets that extend javax.servlet are commonly written by web application developers.
Web Container takes information about the servet to be executed from the deployment descriptor, which is an xml file.
The Parser is used by the web container to extract data from the web.xml file. Many xml parsers exist, including SAX, DOM, and Pull.
The web.xml file contains numerous elements. Some of the components required to run the simple servlet program are listed below.
<web-app>
<servlet>
<servlet-name>ram</servlet-name>
<servlet-class>DemoServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>ram</servlet-name>
<url-pattern>/welcome</url-pattern>
</servlet-mapping>
</web-app>
Description of the Elements
The web.xml file contains an excessive number of components. Here's an example of some of the elements found in the aforesaid web.xml file. The following are the components:
<web-app> reflects the entirety of the application
<servlet> represents the servlet and is a sub-element of <web-app>.
<servlet-name> The name of the servlet is represented by the sub element of <servlet>.
<servlet-class> The class of the servlet is represented by the sub element of <servlet>.
<servlet-mapping> is a sub-element of the <web-app> element. It's used to map the servlet's location.
<url-pattern> is a sub-element of the <servlet-mapping> element. This pattern is used to invoke the servlet on the client side.
Key takeaway
- Web Container takes information about the servet to be executed from the deployment descriptor, which is an xml file.
- The Parser is used by the web container to extract data from the web.xml file. Many xml parsers exist, including SAX, DOM, and Pull.
The term "servlet collaboration" refers to the ability of one servlet to communicate with another. Servlets are sometimes used to convey common information from one servlet to another via various method invocations.
What are the two types of servlet collaboration methods?
Servlet cooperation is a means for two servlets to communicate with each other. There are three ways to collaborate using servlets. Include() and forward() methods are available in RequestDispatchers. Using the Response object's sendRedirect() method.
As a result, what is the purpose of the RequestDispatcher interface? The RequestDispatcher interface allows you to send a request to another resource, which may be html, servlet, or jsp. The content of another resource can likewise be included via this interface. It is one of the servlet cooperation methods.
What is the best way to achieve servlet collaboration?
Servlets can be loaded using the ServletContext access methods or a servlet can be redirected from another servlet. This can also be accomplished using RequestDispatcher's forward() and include() methods, as well as the sendRedirect() method. Java classes that execute on a web server are known as servlets.
RequestDispatcher
The RequestDispatcher interface allows you to send a request to another resource, which may be html, servlet, or jsp. The content of another resource can likewise be included via this interface. It is one of the servlet cooperation methods.
Methods of RequestDispatcher interface
The RequestDispatcher interface has two methods specified.
- Public void forward(ServletRequest request,ServletResponse
Response)throws ServletException,java.io.IOException: Forwards a servlet request to another server resource (servlet, JSP file, or HTML page).
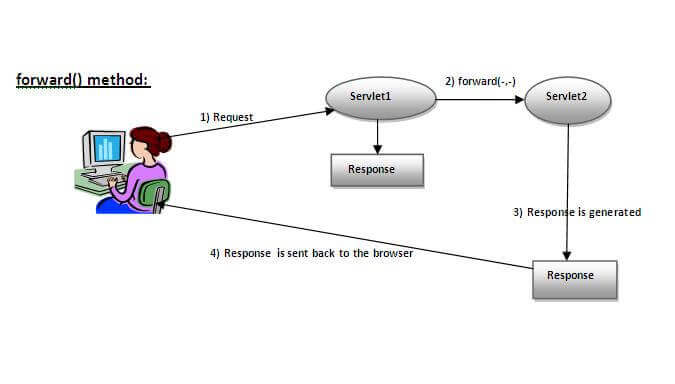
Fig 1: forward()
The response of the second servlet is sent to the client, as shown in the diagram above. The user does not see the first servlet's response.
2. public void include(ServletRequest request,ServletResponse
Response)throws ServletException,java.io.IOException: The answer includes the content of a resource (servlet, JSP page, or HTML file).
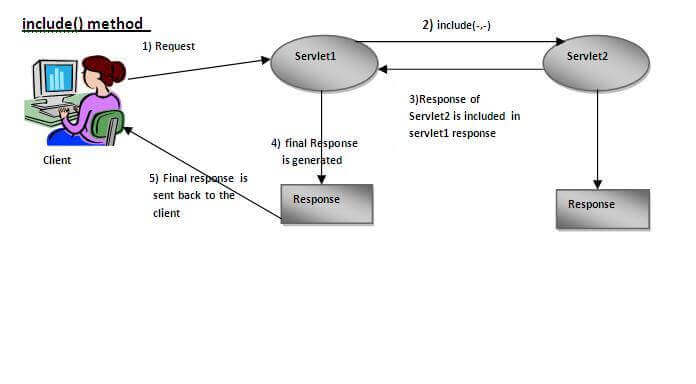
Fig 2: include()
As you can see in the above figure, the response of the second servlet is included in the response of the first servlet that is being sent to the client.
SendRedirect
The HttpServletResponse interface's sendRedirect() function can be used to redirect a response to another resource, such as a servlet, jsp, or html file.
It accepts both relative and absolute URLs.
It works on the client side because it makes another request using the browser's url bar. As a result, it can be used both inside and outside the server.
Syntax
Public void sendRedirect(String URL)throws IOException;
Key takeaway
- The term "servlet collaboration" refers to the ability of one servlet to communicate with another.
- Servlets are sometimes used to convey common information from one servlet to another via various method invocations.
- A session is essentially a time frame, and tracking refers to keeping user data for a set length of time.
- Web servers and the HTTP protocol are stateless.
- All requests and responses are distinct from one another.
- Each request to the web server is considered a separate request.
- Session tracking is a method used by the web container to keep track of a user's session information. It's used to identify a certain user.
Methods of Session Tracking
Session Tracking employs four different techniques:
1) Cookies
2) Hidden Form Field
3) URL Rewriting
4) HttpSession
Cookies
Cookies are little pieces of data sent by the web server in the response header and kept by the browser. Each web client can be assigned a unique session ID by a web server. Cookies are used to keep the session going. Cookies can be turned off by the client.
Hidden From Field
The information is inserted into the web pages via the hidden form field, which is then transferred to the server. These fields are hidden from the user's view.
For example:
<input type = hidden' name = 'session' value = '12345' >
URL Rewriting
With each request and return, append some extra data via URL as request parameters. URL rewriting is a better technique to keep session management and browser work in sync.
HttpSession
A user session is represented by the HttpSession object. A session is established between an HTTP client and an HTTP server using the HttpSession interface. A user session is a collection of data about a user that spans many HTTP requests.
For example:
HttpSession session = request.getSession( );
Session.setAttribute("username", "password");
Key takeaway
- A session is essentially a time frame, and tracking refers to keeping user data for a set length of time.
- Web servers and the HTTP protocol are stateless.
References:
- Elliotte Rusty Harold, “Java Network Programming”, O’Reilly publisher.
- Patrick Naughton, “COMPLETE REFERENCE: JAVA2”, Tata McGraw - Hill.
- Web references: http://java.sun.com.
- Https://www.tutorialride.com/
- Https://javaee.github.io/