UNIT 3
FUNCTIONS
A function is a group of statements that together perform a task. Every C++ program has at least one function, which is main(), and all the most trivial programs can define additional functions.
You can divide up your code into separate functions. How you divide up your code among different functions is up to you, but logically the division usually is such that each function performs a specific task.
A function declaration tells the compiler about a function's name, return type, and parameters. A function definition provides the actual body of the function.
The C++ standard library provides numerous built-in functions that your program can call. For example, function strcat() to concatenate two strings, function memcpy() to copy one memory location to another location and many more functions.
A function is known with various names like a method or a sub-routine or a procedure etc.
Defining a Function
The general form of a C++ function definition is as follows −
Return_type function_name( parameter list ) {
Body of the function
}
A C++ function definition consists of a function header and a function body. Here are all the parts of a function −
- Return Type − A function may return a value. The return_type is the data type of the value the function returns. Some functions perform the desired operations without returning a value. In this case, the return_type is the keyword void.
- Function Name − This is the actual name of the function. The function name and the parameter list together constitute the function signature.
- Parameters − A parameter is like a placeholder. When a function is invoked, you pass a value to the parameter. This value is referred to as actual parameter or argument. The parameter list refers to the type, order, and number of the parameters of a function. Parameters are optional; that is, a function may contain no parameters.
- Function Body − The function body contains a collection of statements that define what the function does.
Example
Following is the source code for a function called max(). This function takes two parameters num1 and num2 and return the biggest of both −
// function returning the max between two numbers
Int max(int num1, int num2) {
// local variable declaration
Int result;
If (num1 > num2)
Result = num1;
Else
Result = num2;
Return result;
}
Function Declarations
A function declaration tells the compiler about a function name and how to call the function. The actual body of the function can be defined separately.
A function declaration has the following parts −
Return_type function_name( parameter list );
For the above defined function max(), following is the function declaration −
Int max(int num1, int num2);
Parameter names are not important in function declaration only their type is required, so following is also valid declaration −
Int max(int, int);
Function declaration is required when you define a function in one source file and you call that function in another file. In such case, you should declare the function at the top of the file calling the function.
Calling a Function
While creating a C++ function, you give a definition of what the function has to do. To use a function, you will have to call or invoke that function.
When a program calls a function, program control is transferred to the called function. A called function performs defined task and when it’s return statement is executed or when its function-ending closing brace is reached, it returns program control back to the main program.
To call a function, you simply need to pass the required parameters along with function name, and if function returns a value, then you can store returned value. For example −
#include <iostream>
Using namespace std;
// function declaration
Int max(int num1, int num2);
Int main () {
// local variable declaration:
Int a = 100;
Int b = 200;
Int ret;
// calling a function to get max value.
Ret = max(a, b);
Cout << "Max value is : " << ret << endl;
Return 0;
}
// function returning the max between two numbers
Int max(int num1, int num2) {
// local variable declaration
Int result;
If (num1 > num2)
Result = num1;
Else
Result = num2;
Return result;
}
I kept max() function along with main() function and compiled the source code. While running final executable, it would produce the following result −
Max value is : 200
Function Arguments
If a function is to use arguments, it must declare variables that accept the values of the arguments. These variables are called the formal parameters of the function.
The formal parameters behave like other local variables inside the function and are created upon entry into the function and destroyed upon exit.
While calling a function, there are two ways that arguments can be passed to a function −
Sr.No | Call Type & Description |
1 | Call by value This method copies the actual value of an argument into the formal parameter of the function. In this case, changes made to the parameter inside the function have no effect on the argument. |
2 | Call by pointer This method copies the address of an argument into the formal parameter. Inside the function, the address is used to access the actual argument used in the call. This means that changes made to the parameter affect the argument. |
3 | Call by reference This method copies the reference of an argument into the formal parameter. Inside the function, the reference is used to access the actual argument used in the call. This means that changes made to the parameter affect the argument. |
By default, C++ uses call by value to pass arguments. In general, this means that code within a function cannot alter the arguments used to call the function and above mentioned example while calling max() function used the same method.
Default Values for Parameters
When you define a function, you can specify a default value for each of the last parameters. This value will be used if the corresponding argument is left blank when calling to the function.
This is done by using the assignment operator and assigning values for the arguments in the function definition. If a value for that parameter is not passed when the function is called, the default given value is used, but if a value is specified, this default value is ignored and the passed value is used instead. Consider the following example −
#include <iostream>
Using namespace std;
Int sum(int a, int b = 20) {
Int result;
Result = a + b;
Return (result);
}
Int main () {
// local variable declaration:
Int a = 100;
Int b = 200;
Int result;
// calling a function to add the values.
Result = sum(a, b);
Cout << "Total value is :" << result << endl;
// calling a function again as follows.
Result = sum(a);
Cout << "Total value is :" << result << endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Total value is :300
Total value is :120
C++ allows programmer to define their own function.
A user-defined function group’s code to perform a specific task and that group of code is given a name (identifier).
When the function is invoked from any part of program, it all executes the codes defined in the body of function.
Example of User-defined functions
Using namespace std;
// Function prototype (declaration)
Int add(int, int);
Int main()
{
Int num1, num2, sum;
Cout<<"Enters two numbers to add: ";
Cin >> num1 >> num2;
// Function call
Sum = add(num1, num2);
Cout << "Sum = " << sum;
Return 0;
}
// Function definition
Int add(int a, int b)
{
Int add;
Add = a + b;
// Return statement
Return add;
}
C++
Copy
Output
Enters two integers: 8
-4
Sum = 4
Types of User-defined functions
There can be 4 different types of user-defined functions, they are:
- Function with no arguments and no return value
- Function with no arguments and a return value
- Function with arguments and no return value
- Function with arguments and a return value
Below, we will discuss about all these types, along with program examples.
1. Function with no arguments and no return value :
Such functions can either be used to display information or they are completely dependent on user inputs.
Example :
In the following program, prime() is called from the main() with 0no arguments.
prime() takes the positive number from the user and checks whether the number is a prime number or not.
Since, return type of prime() is void, no value is returned from the function.
Using namespace std;
Void prime();
Int main()
{
// No argument is passed to prime()
Prime();
Return 0;
}
// Return type of function is void because value is not returned.
Void prime()
{
Int num, i, flag = 0;
Cout << "Enter a positive integer enter to check: ";
Cin >> num;
For(i = 2; i <= num/2; ++i)
{
If(num % i == 0)
{
Flag = 1;
Break;
}
}
If (flag == 1)
{
Cout << num << " is not a prime number.";
}
Else
{
Cout << num << " is a prime number.";
}
}
C++
Copy
2. Function with no arguments and a return value :
In the following program, prime() function is called from the main() with no arguments.
prime() takes a positive integer from the user. Since, return type of the function is an int, it returns the inputted number from the user back to the calling main() function.
Then, whether the number is prime or not is checked in the main() itself and printed onto the screen.
Example
Using namespace std;
Int prime();
Int main()
{
Int num, i, flag = 0;
// No argument is passed to prime()
Num = prime();
For (i = 2; i <= num/2; ++i)
{
If (num%i == 0)
{
Flag = 1;
Break;
}
}
If (flag == 1)
{
Cout<<num<<" is not a prime number.";
}
Else
{
Cout<<num<<" is a prime number.";
}
Return 0;
}
// Return type of function is int
Int prime()
{
Int n;
Printf("Enter a positive integer to check: ");
Cin >> n;
Return n;
}
C++
Copy
3. Function with arguments and no return value :
We are using the same function as example again and again, to demonstrate that to solve a problem there can be many different ways.
This time, we have modified the above example to make the function prime() take one int value as argument, but it will not be returning anything.
In the program, positive number is first asked from the user which is stored in the variable num.
Then, num is passed to the prime() function where, whether the number is prime or not is checked and printed.
Since, the return type of prime() is a void, no value is returned from the function.
Example
Using namespace std;
Void prime(int n);
Int main()
{
Int num;
Cout << "Enter a positive integer to check: ";
Cin >> num;
// Argument num is passed to the function prime()
Prime(num);
Return 0;
}
// There is no return value to calling function. Hence, return type of function is void. */
Void prime(int n)
{ int i, flag = 0;
For (i = 2; i <= n/2; ++i)
{
If (n%i == 0)
{
Flag = 1;
Break;
}
}
If (flag == 1)
{
Cout << n << " is not a prime number.";
}
Else {
Cout << n << " is a prime number.";
}
}
C++
Copy
4. Function with arguments and a return value:
This is the best type, as this makes the function completely independent of inputs and outputs, and only the logic is defined inside the function body.
In the following program, a positive integer is asked from the user and stored in the variable num.
Then, num is passed to the function prime() where, whether the number is prime or not is checked.
Since, the return type of prime() is an int, 1 or 0 is returned to the main() calling function. If the number is a prime number, 1 is returned. If not, 0 is returned.
Back in the main() function, the returned 1 or 0 is stored in the variable flag, and the corresponding text is printed onto the screen.
Example
Using namespace std;
Int prime(int n);
Int main()
{
Int num, flag = 0;
Cout << "Enter positive integer to check: ";
Cin >> num;
// Argument num is passed to check() function
Flag = prime(num);
If(flag == 1)
Cout << num << " is not a prime number.";
Else
Cout<< num << " is a prime number.";
Return 0;
}
/* This function returns integer value. */
Int prime(int n)
{
Int i;
For(i = 2; i <= n/2; ++i)
{
If(n % i == 0)
Return 1;
}
Return 0;
}
The return statement returns the flow of the execution to the function from where it is called. This statement does not mandatorily need any conditional statements. As soon as the statement is executed, the flow of the program stops immediately and returns the control from where it was called. The return statement may or may not return anything for a void function, but for a non-void function, a return value is must be returned.
Syntax:
Return[expression];
There are various ways to use return statements. Few are mentioned below:
- Methods not returning a value: In C/C++ one cannot skip the return statement, when the methods are of return type. The return statement can be skipped only for void types.
- Not using return statement in void return type function: When a function does not return anything, the void return type is used. So if there is a void return type in the function definition, then there will be no return statement inside that function (generally).
Syntax:
Void func()
{
.
.
.
}
Example:
// C code to show not using return // statement in void return type function
#include <stdio.h>
// void method Void Print() { Printf("Welcome to GeeksforGeeks"); }
// Driver method Int main() {
// Calling print Print();
Return 0; } |
Output:
Welcome to GeeksforGeeks
Using return statement in void return type function: Now the question arises, what if there is a return statement inside a void return type function? Since we know that, if there is a void return type in the function definition, then there will be no return statement inside that function. But if there is a return statement inside it, then also there will be no problem if the syntax of it will be:
Correct Syntax:
Void func()
{
Return;
}
This syntax is used in function just as a jump statement in order to break the flow of the function and jump out of it. One can think of it as an alternative to “break statement” to use in functions.
Example:
// C code to show using return // statement in void return type function
#include <stdio.h>
// void method Void Print() { Printf("Welcome to GeeksforGeeks");
// void method using the return statement Return; }
// Driver method Int main() {
// Calling print Print(); Return 0; } |
Output:
Welcome to GeeksforGeeks
But if the return statement tries to return a value in a void return type function, that will lead to errors.
Incorrect Syntax:
Void func()
{
Return value;
}
Warnings:
Warning: 'return' with a value, in function returning void
Example:
// C code to show using return statement // with a value in void return type function
#include <stdio.h>
// void method Void Print() { Printf("Welcome to GeeksforGeeks");
// void method using the return // statement to return a value Return 10; }
// Driver method Int main() {
// Calling print Print(); Return 0; } |
Warnings:
Prog.c: In function 'Print':
Prog.c:12:9: warning: 'return' with a value, in function returning void
Return 10;
^
Methods returning a value: For methods that define a return type, the return statement must be immediately followed by the return value of that specified return type.
Syntax:
Return-type func()
{
Return value;
}
Example:
// C code to illustrate Methods returning // a value using return statement
#include <stdio.h>
// non-void return type // function to calculate sum Int SUM(int a, int b) { Int s1 = a + b;
// method using the return // statement to return a value Return s1; }
// Driver method Int main() { Int num1 = 10; Int num2 = 10; Int sum_of = SUM(num1, num2); Printf("The sum is %d", sum_of); Return 0; } |
Output:
The sum is 20
Void functions are “void” due to the fact that they are not supposed to return values. True, but not completely. We cannot return values but there is something we can surely return from void functions. Some of cases are listed below.
A void function can do return
We can simply write return statement in a void fun(). In-fact it is considered a good practice (for readability of code) to write return; statement to indicate end of function.
#include <iostream> Using namespace std;
Void fun() { Cout << "Hello";
// We can write return in void Return; }
Int main() { Fun(); Return 0; } |
Output :
Hello
A void fun() can return another void function
// C++ code to demonstrate void() // returning void() #include<iostream> Using namespace std;
// A sample void function Void work() { Cout << "The void function has returned " " a void() !!! \n"; }
// Driver void() returning void work() Void test() { // Returning void function Return work(); }
Int main() { // Calling void function Test(); Return 0; } |
Output:
The void function has returned a void() !!!
The above code explains how void() can actually be useful to return void functions without giving error.
A void() can return a void value.
A void() cannot return a value that can be used. But it can return a value which is void without giving an error.
// C++ code to demonstrate void() // returning a void value #include<iostream> Using namespace std;
// Driver void() returning a void value Void test() { Cout << "Hello";
// Returning a void value Return (void)"Doesn't Print"; } Int main() { Test(); Return 0; } |
Output:
Hello
There are different ways in which parameter data can be passed into and out of methods and functions. Let us assume that a function B() is called from another function A(). In this case A is called the “caller function” and B is called the “called function or callee function”. Also, the arguments which A sends to B are called actual arguments and the parameters of B are called formal arguments.
Terminology
- Formal Parameter : A variable and its type as they appear in the prototype of the function or method.
- Actual Parameter : The variable or expression corresponding to a formal parameter that appears in the function or method call in the calling environment.
- Modes:
- IN: Passes info from caller to calle.
- OUT: Callee writes values in caller.
- IN/OUT: Caller tells callee value of variable, which may be updated by callee.
Important methods of Parameter Passing
- Pass By Value : This method uses in-mode semantics. Changes made to formal parameter do not get transmitted back to the caller. Any modifications to the formal parameter variable inside the called function or method affect only the separate storage location and will not be reflected in the actual parameter in the calling environment. This method is also called as call by value.
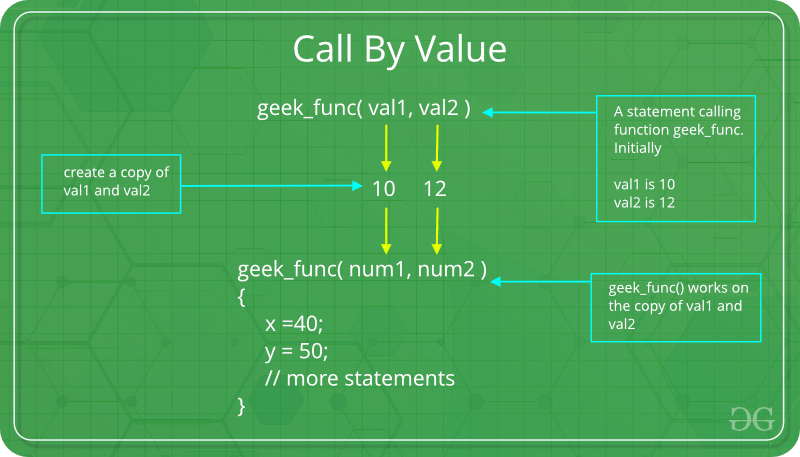
// C program to illustrate // call by value #include <stdio.h>
Void func(int a, int b) { a += b; Printf("In func, a = %d b = %d\n", a, b); } Int main(void) { Int x = 5, y = 7;
// Passing parameters Func(x, y); Printf("In main, x = %d y = %d\n", x, y); Return 0; } |
Output:
In func, a = 12 b = 7
In main, x = 5 y = 7
- Inefficiency in storage allocation
- For objects and arrays, the copy semantics are costly
- Pass by reference(aliasing) : This technique uses in/out-mode semantics. Changes made to formal parameter do get transmitted back to the caller through parameter passing. Any changes to the formal parameter are reflected in the actual parameter in the calling environment as formal parameter receives a reference (or pointer) to the actual data. This method is also called as <em>call by reference. This method is efficient in both time and space.
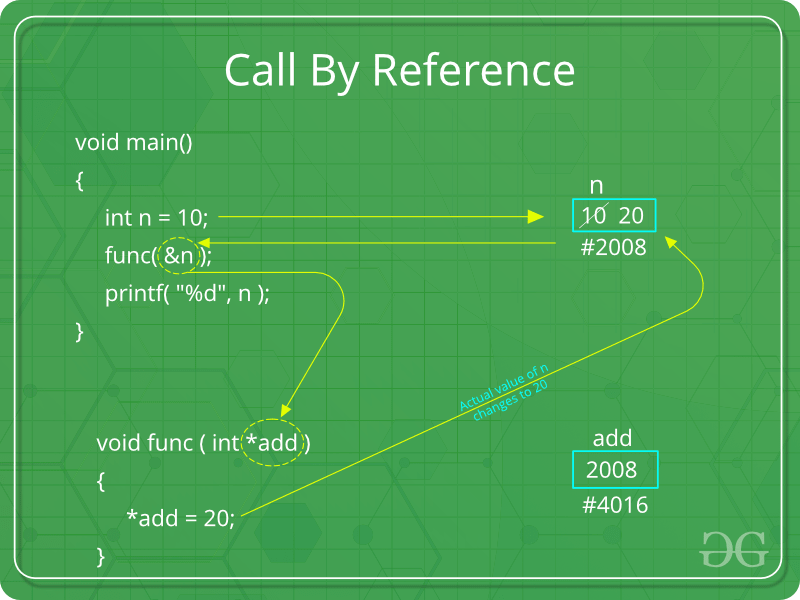
// C program to illustrate // call by reference #include <stdio.h>
Void swapnum(int* i, int* j) { Int temp = *i; *i = *j; *j = temp; }
Int main(void) { Int a = 10, b = 20;
// passing parameters Swapnum(&a, &b);
Printf("a is %d and b is %d\n", a, b); Return 0; } |
Output:
a is 20 and b is 10
C and C++ both support call by value as well as call by reference whereas Java does’nt support call by reference.
Shortcomings:
- Many potential scenarios can occur
- Programs are difficult to understand sometimes
Other methods of Parameter Passing
These techniques are older and were used in earlier programming languages like Pascal, Algol and Fortran. These techniques are not applicable in high level languages.
- Pass by Result : This method uses out-mode semantics. Just before control is transfered back to the caller, the value of the formal parameter is transmitted back to the actual parameter.T his method is sometimes called call by result. In general, pass by result technique is implemented by copy.
- Pass by Value-Result : This method uses in/out-mode semantics. It is a combination of Pass-by-Value and Pass-by-result. Just before the control is transferred back to the caller, the value of the formal parameter is transmitted back to the actual parameter. This method is sometimes called as call by value-result
- Pass by name : This technique is used in programming language such as Algol. In this technique, symbolic “name” of a variable is passed, which allows it both to be accessed and update.
Example:
To double the value of C[j], you can pass its name (not its value) into the following procedure. - Procedure double(x);
- Real x;
- Begin
- x:=x*2
- End;
In general, the effect of pass-by-name is to textually substitute the argument in a procedure call for the corresponding parameter in the body of the procedure.
Implications of Pass-by-Name mechanism:
- The argument expression is re-evaluated each time the formal parameter is passed.
- The procedure can change the values of variables used in the argument expression and hence change the expression’s value.
If we create two or more members having the same name but different in number or type of parameter, it is known as C++ overloading. In C++, we can overload:
- Methods,
- Constructors, and
- Indexed properties
It is because these members have parameters only.
Types of overloading in C++ are:
- Function overloading
- Operator overloading
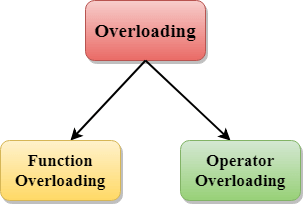
C++ Function Overloading
Function Overloading is defined as the process of having two or more function with the same name, but different in parameters is known as function overloading in C++. In function overloading, the function is redefined by using either different types of arguments or a different number of arguments. It is only through these differences compiler can differentiate between the functions.
The advantage of Function overloading is that it increases the readability of the program because you don't need to use different names for the same action.
C++ Function Overloading Example
Let's see the simple example of function overloading where we are changing number of arguments of add() method.
// program of function overloading when number of arguments vary.
- #include <iostream>
- Using namespace std;
- Class Cal {
- Public:
- Static int add(int a,int b){
- Return a + b;
- }
- Static int add(int a, int b, int c)
- {
- Return a + b + c;
- }
- };
- Int main(void) {
- Cal C; // class object declaration.
- Cout<<C.add(10, 20)<<endl;
- Cout<<C.add(12, 20, 23);
- Return 0;
- }
Output:
30
55
Let's see the simple example when the type of the arguments vary.
// Program of function overloading with different types of arguments.
- #include<iostream>
- Using namespace std;
- Int mul(int,int);
- Float mul(float,int);
- Int mul(int a,int b)
- {
- Return a*b;
- }
- Float mul(double x, int y)
- {
- Return x*y;
- }
- Int main()
- {
- Int r1 = mul(6,7);
- Float r2 = mul(0.2,3);
- Std::cout << "r1 is : " <<r1<< std::endl;
- Std::cout <<"r2 is : " <<r2<< std::endl;
- Return 0;
- }
Output:
r1 is : 42
r2 is : 0.6
Function Overloading and Ambiguity
When the compiler is unable to decide which function is to be invoked among the overloaded function, this situation is known as function overloading.
When the compiler shows the ambiguity error, the compiler does not run the program.
Causes of Function Overloading:
- Type Conversion.
- Function with default arguments.
- Function with pass by reference.
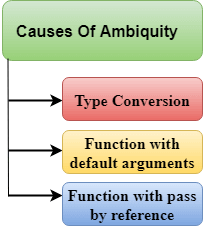
- Type Conversion:
Let's see a simple example.
- #include<iostream>
- Using namespace std;
- Void fun(int);
- Void fun(float);
- Void fun(int i)
- {
- Std::cout << "Value of i is : " <<i<< std::endl;
- }
- Void fun(float j)
- {
- Std::cout << "Value of j is : " <<j<< std::endl;
- }
- Int main()
- {
- Fun(12);
- Fun(1.2);
- Return 0;
- }
The above example shows an error "call of overloaded 'fun(double)' is ambiguous". The fun(10) will call the first function. The fun(1.2) calls the second function according to our prediction. But, this does not refer to any function as in C++, all the floating point constants are treated as double not as a float. If we replace float to double, the program works. Therefore, this is a type conversion from float to double.
- Function with Default Arguments
Let's see a simple example.
- #include<iostream>
- Using namespace std;
- Void fun(int);
- Void fun(int,int);
- Void fun(int i)
- {
- Std::cout << "Value of i is : " <<i<< std::endl;
- }
- Void fun(int a,int b=9)
- {
- Std::cout << "Value of a is : " <<a<< std::endl;
- Std::cout << "Value of b is : " <<b<< std::endl;
- }
- Int main()
- {
- Fun(12);
- Return 0;
- }
The above example shows an error "call of overloaded 'fun(int)' is ambiguous". The fun(int a, int b=9) can be called in two ways: first is by calling the function with one argument, i.e., fun(12) and another way is calling the function with two arguments, i.e., fun(4,5). The fun(int i) function is invoked with one argument. Therefore, the compiler could not be able to select among fun(int i) and fun(int a,int b=9).
- Function with pass by reference
Let's see a simple example.
- #include <iostream>
- Using namespace std;
- Void fun(int);
- Void fun(int &);
- Int main()
- {
- Int a=10;
- Fun(a); // error, which f()?
- Return 0;
- }
- Void fun(int x)
- {
- Std::cout << "Value of x is : " <<x<< std::endl;
- }
- Void fun(int &b)
- {
- Std::cout << "Value of b is : " <<b<< std::endl;
- }
The above example shows an error "call of overloaded 'fun(int&)' is ambiguous". The first function takes one integer argument and the second function takes a reference parameter as an argument. In this case, the compiler does not know which function is needed by the user as there is no syntactical difference between the fun(int) and fun(int &).
C++ Operators Overloading
Operator overloading is a compile-time polymorphism in which the operator is overloaded to provide the special meaning to the user-defined data type. Operator overloading is used to overload or redefines most of the operators available in C++. It is used to perform the operation on the user-defined data type. For example, C++ provides the ability to add the variables of the user-defined data type that is applied to the built-in data types.
The advantage of Operators overloading is to perform different operations on the same operand.
Operator that cannot be overloaded are as follows:
- Scope operator (::)
- Sizeof
- Member selector(.)
- Member pointer selector(*)
- Ternary operator(?:)
Syntax of Operator Overloading
- Return_type class_name : : operator op(argument_list)
- {
- // body of the function.
- }
Where the return type is the type of value returned by the function.
Class_name is the name of the class.
Operator op is an operator function where op is the operator being overloaded, and the operator is the keyword.
Rules for Operator Overloading
- Existing operators can only be overloaded, but the new operators cannot be overloaded.
- The overloaded operator contains atleast one operand of the user-defined data type.
- We cannot use friend function to overload certain operators. However, the member function can be used to overload those operators.
- When unary operators are overloaded through a member function take no explicit arguments, but, if they are overloaded by a friend function, takes one argument.
- When binary operators are overloaded through a member function takes one explicit argument, and if they are overloaded through a friend function takes two explicit arguments.
C++ Operators Overloading Example
Let's see the simple example of operator overloading in C++. In this example, void operator ++ () operator function is defined (inside Test class).
// program to overload the unary operator ++.
- #include <iostream>
- Using namespace std;
- Class Test
- {
- Private:
- Int num;
- Public:
- Test(): num(8){}
- Void operator ++() {
- Num = num+2;
- }
- Void Print() {
- Cout<<"The Count is: "<<num;
- }
- };
- Int main()
- {
- Test tt;
- ++tt; // calling of a function "void operator ++()"
- Tt.Print();
- Return 0;
- }
Output:
The Count is: 10
Let's see a simple example of overloading the binary operators.
// program to overload the binary operators.
- #include <iostream>
- Using namespace std;
- Class A
- {
- Int x;
- Public:
- A(){}
- A(int i)
- {
- x=i;
- }
- Void operator+(A);
- Void display();
- };
- Void A :: operator+(A a)
- {
- Int m = x+a.x;
- Cout<<"The result of the addition of two objects is : "<<m;
- }
- Int main()
- {
- A a1(5);
- A a2(4);
- a1+a2;
- Return 0;
- }
Output:
The result of the addition of two objects is : 9
- A C++ virtual function is a member function in the base class that you redefine in a derived class. It is declared using the virtual keyword.
- It is used to tell the compiler to perform dynamic linkage or late binding on the function.
- There is a necessity to use the single pointer to refer to all the objects of the different classes. So, we create the pointer to the base class that refers to all the derived objects. But, when base class pointer contains the address of the derived class object, always executes the base class function. This issue can only be resolved by using the 'virtual' function.
- A 'virtual' is a keyword preceding the normal declaration of a function.
- When the function is made virtual, C++ determines which function is to be invoked at the runtime based on the type of the object pointed by the base class pointer.
Late binding or Dynamic linkage
In late binding function call is resolved during runtime. Therefore compiler determines the type of object at runtime, and then binds the function call.
Rules of Virtual Function
- Virtual functions must be members of some class.
- Virtual functions cannot be static members.
- They are accessed through object pointers.
- They can be a friend of another class.
- A virtual function must be defined in the base class, even though it is not used.
- The prototypes of a virtual function of the base class and all the derived classes must be identical. If the two functions with the same name but different prototypes, C++ will consider them as the overloaded functions.
- We cannot have a virtual constructor, but we can have a virtual destructor
- Consider the situation when we don't use the virtual keyword.
- #include <iostream>
- Using namespace std;
- Class A
- {
- Int x=5;
- Public:
- Void display()
- {
- Std::cout << "Value of x is : " << x<<std::endl;
- }
- };
- Class B: public A
- {
- Int y = 10;
- Public:
- Void display()
- {
- Std::cout << "Value of y is : " <<y<< std::endl;
- }
- };
- Int main()
- {
- A *a;
- B b;
- a = &b;
- a->display();
- Return 0;
- }
Output:
Value of x is : 5
In the above example, * a is the base class pointer. The pointer can only access the base class members but not the members of the derived class. Although C++ permits the base pointer to point to any object derived from the base class, it cannot directly access the members of the derived class. Therefore, there is a need for virtual function which allows the base pointer to access the members of the derived class.
C++ virtual function Example
Let's see the simple example of C++ virtual function used to invoked the derived class in a program.
- #include <iostream>
- {
- Public:
- Virtual void display()
- {
- Cout << "Base class is invoked"<<endl;
- }
- };
- Class B:public A
- {
- Public:
- Void display()
- {
- Cout << "Derived Class is invoked"<<endl;
- }
- };
- Int main()
- {
- A* a; //pointer of base class
- B b; //object of derived class
- a = &b;
- a->display(); //Late Binding occurs
- }
Output:
Derived Class is invoked
Pure Virtual Function
- A virtual function is not used for performing any task. It only serves as a placeholder.
- When the function has no definition, such function is known as "do-nothing" function.
- The "do-nothing" function is known as a pure virtual function. A pure virtual function is a function declared in the base class that has no definition relative to the base class.
- A class containing the pure virtual function cannot be used to declare the objects of its own, such classes are known as abstract base classes.
- The main objective of the base class is to provide the traits to the derived classes and to create the base pointer used for achieving the runtime polymorphism.
Pure virtual function can be defined as:
- Virtual void display() = 0;
Let's see a simple example:
- #include <iostream>
- Using namespace std;
- Class Base
- {
- Public:
- Virtual void show() = 0;
- };
- Class Derived : public Base
- {
- Public:
- Void show()
- {
- Std::cout << "Derived class is derived from the base class." << std::endl;
- }
- };
- Int main()
- {
- Base *bptr;
- //Base b;
- Derived d;
- Bptr = &d;
- Bptr->show();
- Return 0;
- }
Output:
Derived class is derived from the base class.
Reference Books
1 “Thinking in C++”, Volume 1 and 2 by Bruce Eckel, Chuck Allison, Pearson
Education
2 “Mastering C++”, 1/e by Venugopal, TataMcGraw Hill.
3 “Object Oriented Programming with C++”, 3/e by E. Balaguruswamy, Tata
McGraw Hill.
4 “Starting Out with Object Oriented Programming in C++”, by Tony Gaddis,
Wiley India.