UNIT 7
Pointers and Arrays
A void pointer is a general-purpose pointer that can hold the address of any data type, but it is not associated with any data type.
Syntax of void pointer
- Void *ptr;
In C++, we cannot assign the address of a variable to the variable of a different data type. Consider the following example:
- Int *ptr; // integer pointer declaration
- Float a=10.2; // floating variable initialization
- Ptr= &a; // This statement throws an error.
In the above example, we declare a pointer of type integer, i.e., ptr and a float variable, i.e., 'a'. After declaration, we try to store the address of 'a' variable in 'ptr', but this is not possible in C++ as the variable cannot hold the address of different data types.
Let's understand through a simple example.
- #include <iostream.h>
- Using namespace std;
- Int main()
- {
- Int *ptr;
- Float f=10.3;
- Ptr = &f; // error
- Std::cout << "The value of *ptr is : " <<*ptr<< std::endl;
- Return 0;
- }
In the above program, we declare a pointer of integer type and variable of float type. An integer pointer variable cannot point to the float variable, but it can point to an only integer variable.
Output
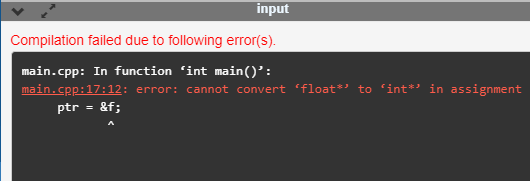
C++ has overcome the above problem by using the C++ void pointer as a void pointer can hold the address of any data type.
Let's look at a simple example of void pointer.
- #include <iostream>
- Using namespace std;
- Int main()
- {
- Void *ptr; // void pointer declaration
- Int a=9; // integer variable initialization
- Ptr=&a; // storing the address of 'a' variable in a void pointer variable.
- Std::cout << &a << std::endl;
- Std::cout << ptr << std::endl;
- Return 0;
- }
In the above program, we declare a void pointer variable and an integer variable where the void pointer contains the address of an integer variable.
Output
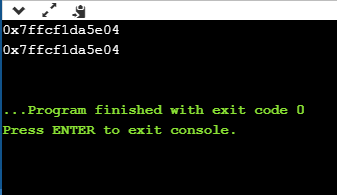
Difference between void pointer in C and C++
In C, we can assign the void pointer to any other pointer type without any typecasting, whereas in C++, we need to typecast when we assign the void pointer type to any other pointer type.
Let's understand through a simple example.
In C,
- #include <stdio.h>
- Int main()
- {
- Void *ptr; // void pointer declaration
- Int *ptr1; // integer pointer declaration
- Int a =90; // integer variable initialization
- Ptr=&a; // storing the address of 'a' in ptr
- Ptr1=ptr; // assigning void pointer to integer pointer type.
- Printf("The value of *ptr1 : %d",*ptr1);
- Return 0;
- }
In the above program, we declare two pointers 'ptr' and 'ptr1' of type void and integer, respectively. We also declare the integer type variable, i.e., 'a'. After declaration, we assign the address of 'a' variable to the pointer 'ptr'. Then, we assign the void pointer to the integer pointer, i.e., ptr1 without any typecasting because in C, we do not need to typecast while assigning the void pointer to any other type of pointer.
Output
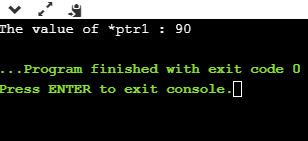
In C++,
- #include <iostream>
- Using namespace std;
- Int main()
- {
- Void *ptr; // void pointer declaration
- Int *ptr1; // integer pointer declaration
- Int data=10; // integer variable initialization
- Ptr=&data; // storing the address of data variable in void pointer variable
- Ptr1=(int *)ptr; // assigning void pointer to integer pointer
- Std::cout << "The value of *ptr1 is : " <<*ptr1<< std::endl;
- Return 0;
- }
In the above program, we declare two pointer variables of type void and int type respectively. We also create another integer type variable, i.e., 'data'. After declaration, we store the address of variable 'data' in a void pointer variable, i.e., ptr. Now, we want to assign the void pointer to integer pointer, in order to do this, we need to apply the cast operator, i.e., (int *) to the void pointer variable. This cast operator tells the compiler which type of value void pointer is holding. For casting, we have to type the data type and * in a bracket like (char *) or (int *).
Output
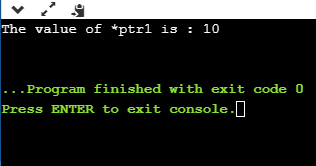
A pointer to a C++ class is done exactly the same way as a pointer to a structure and to access members of a pointer to a class you use the member access operator -> operator, just as you do with pointers to structures. Also as with all pointers, you must initialize the pointer before using it.
Let us try the following example to understand the concept of pointer to a class −
#include <iostream>
Using namespace std;
Class Box {
Public:
// Constructor definition
Box(double l = 2.0, double b = 2.0, double h = 2.0) {
Cout <<"Constructor called." << endl;
Length = l;
Breadth = b;
Height = h;
}
Double Volume() {
Return length * breadth * height;
}
Private:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
};
Int main(void) {
Box Box1(3.3, 1.2, 1.5); // Declare box1
Box Box2(8.5, 6.0, 2.0); // Declare box2
Box *ptrBox; // Declare pointer to a class.
// Save the address of first object
PtrBox = &Box1;
// Now try to access a member using member access operator
Cout << "Volume of Box1: " << ptrBox->Volume() << endl;
// Save the address of second object
PtrBox = &Box2;
// Now try to access a member using member access operator
Cout << "Volume of Box2: " << ptrBox->Volume() << endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Constructor called.
Constructor called.
Volume of Box1: 5.94
Volume of Box2: 102
A variable that holds an address value is called a pointer variable or simply pointer.
Pointer can point to objects as well as to simple data types and arrays.
Sometimes we dont know, at the time that we write the program , how many objects we want to creat. When this is the case we can use new to creat objects while the program is running. New returns a pointer to an unnamed objects. Lets see the example of student that wiil clear your idea about this topic
#include <iostream>
#include <string>
Using namespace std;
Class student
{
Private:
Int rollno;
String name;
Public:
Student():rollno(0),name("")
{}
Student(int r, string n): rollno(r),name (n)
{}
Void get()
{
Cout<<"enter roll no";
Cin>>rollno;
Cout<<"enter name";
Cin>>name;
}
Void print()
{
Cout<<"roll no is "<<rollno;
Cout<<"name is "<<name;
}
};
Void main ()
{
Student *ps=new student;
(*ps).get();
(*ps).print();
Delete ps;
}
Every object in C++ has access to its own address through an important pointer called this pointer. The this pointer is an implicit parameter to all member functions. Therefore, inside a member function, this may be used to refer to the invoking object.
Friend functions do not have a this pointer, because friends are not members of a class. Only member functions have a this pointer.
Let us try the following example to understand the concept of this pointer −
#include <iostream>
Using namespace std;
Class Box {
Public:
// Constructor definition
Box(double l = 2.0, double b = 2.0, double h = 2.0) {
Cout <<"Constructor called." << endl;
Length = l;
Breadth = b;
Height = h;
}
Double Volume() {
Return length * breadth * height;
}
Int compare(Box box) {
Return this->Volume() > box.Volume();
}
Private:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
};
Int main(void) {
Box Box1(3.3, 1.2, 1.5); // Declare box1
Box Box2(8.5, 6.0, 2.0); // Declare box2
If(Box1.compare(Box2)) {
Cout << "Box2 is smaller than Box1" <<endl;
} else {
Cout << "Box2 is equal to or larger than Box1" <<endl;
}
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Constructor called.
Constructor called.
Box2 is equal to or larger than Box1
C++ provides a data structure, the array, which stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type.
Instead of declaring individual variables, such as number0, number1, ..., and number99, you declare one array variable such as numbers and use numbers[0], numbers[1], and ..., numbers[99] to represent individual variables. A specific element in an array is accessed by an index.
All arrays consist of contiguous memory locations. The lowest address corresponds to the first element and the highest address to the last element.
Declaring Arrays
To declare an array in C++, the programmer specifies the type of the elements and the number of elements required by an array as follows −
Type arrayName [ arraySize ];
This is called a single-dimension array. The arraySize must be an integer constant greater than zero and type can be any valid C++ data type. For example, to declare a 10-element array called balance of type double, use this statement −
Double balance[10];
Initializing Arrays
You can initialize C++ array elements either one by one or using a single statement as follows −
Double balance[5] = {1000.0, 2.0, 3.4, 17.0, 50.0};
The number of values between braces { } can not be larger than the number of elements that we declare for the array between square brackets [ ]. Following is an example to assign a single element of the array −
If you omit the size of the array, an array just big enough to hold the initialization is created. Therefore, if you write −
Double balance[] = {1000.0, 2.0, 3.4, 17.0, 50.0};
You will create exactly the same array as you did in the previous example.
Balance[4] = 50.0;
The above statement assigns element number 5th in the array a value of 50.0. Array with 4th index will be 5th, i.e., last element because all arrays have 0 as the index of their first element which is also called base index. Following is the pictorial representaion of the same array we discussed above −

Accessing Array Elements
An element is accessed by indexing the array name. This is done by placing the index of the element within square brackets after the name of the array. For example −
Double salary = balance[9];
The above statement will take 10th element from the array and assign the value to salary variable. Following is an example, which will use all the above-mentioned three concepts viz. Declaration, assignment and accessing arrays −
#include <iostream>
Using namespace std;
#include <iomanip>
Using std::setw;
Int main () {
Int n[ 10 ]; // n is an array of 10 integers
// initialize elements of array n to 0
For ( int i = 0; i < 10; i++ ) {
n[ i ] = i + 100; // set element at location i to i + 100
}
Cout << "Element" << setw( 13 ) << "Value" << endl;
// output each array element's value
For ( int j = 0; j < 10; j++ ) {
Cout << setw( 7 )<< j << setw( 13 ) << n[ j ] << endl;
}
Return 0;
}
This program makes use of setw() function to format the output. When the above code is compiled and executed, it produces the following result −
Element Value
0 100
1 101
2 102
3 103
4 104
5 105
6 106
7 107
8 108
9 109
Arrays in C++
Arrays are important to C++ and should need lots of more detail. There are following few important concepts, which should be clear to a C++ programmer −
Sr.No | Concept & Description |
1 | Multi-dimensional arrays C++ supports multidimensional arrays. The simplest form of the multidimensional array is the two-dimensional array. |
2 | Pointer to an array You can generate a pointer to the first element of an array by simply specifying the array name, without any index. |
3 | Passing arrays to functions You can pass to the function a pointer to an array by specifying the array's name without an index. |
4 | Return array from functions C++ allows a function to return an array. |
Reference Books
1 “Thinking in C++”, Volume 1 and 2 by Bruce Eckel, Chuck Allison, Pearson
Education
2 “Mastering C++”, 1/e by Venugopal, TataMcGraw Hill.
3 “Object Oriented Programming with C++”, 3/e by E. Balaguruswamy, Tata
McGraw Hill.
4 “Starting Out with Object Oriented Programming in C++”, by Tony Gaddis,
Wiley India.