Unit 6
File Handling and Design Patterns
In programming, we may require some specific input data to be generated several numbers of times. Sometimes, it is not enough to only display the data on the console. The data to be displayed may be very large, and only a limited amount of data can be displayed on the console, and since the memory is volatile, it is impossible to recover the programmatically generated data again and again. However, if we need to do so, we may store it onto the local file system which is volatile and can be accessed every time. Here, comes the need of file handling in C.
File handling in C enables us to create, update, read, and delete the files stored on the local file system through our C program. The following operations can be performed on a file.
- Creation of the new file
- Opening an existing file
- Reading from the file
- Writing to the file
- Deleting the file
Functions for file handling
There are many functions in the C library to open, read, write, search and close the file. A list of file functions are given below:
No. | Function | Description |
1 | Fopen() | Opens new or existing file |
2 | Fprintf() | Write data into the file |
3 | Fscanf() | Reads data from the file |
4 | Fputc() | Writes a character into the file |
5 | Fgetc() | Reads a character from file |
6 | Fclose() | Closes the file |
7 | Fseek() | Sets the file pointer to given position |
8 | Fputw() | Writes an integer to file |
9 | Fgetw() | Reads an integer from file |
10 | Ftell() | Returns current position |
11 | Rewind() | Sets the file pointer to the beginning of the file |
Opening File: fopen()
We must open a file before it can be read, write, or update. The fopen() function is used to open a file. The syntax of the fopen() is given below.
- FILE *fopen( const char * filename, const char * mode );
The fopen() function accepts two parameters:
- The file name (string). If the file is stored at some specific location, then we must mention the path at which the file is stored. For example, a file name can be like "c://some_folder/some_file.ext".
- The mode in which the file is to be opened. It is a string.
We can use one of the following modes in the fopen() function.
Mode | Description |
r | Opens a text file in read mode |
w | Opens a text file in write mode |
a | Opens a text file in append mode |
r+ | Opens a text file in read and write mode |
w+ | Opens a text file in read and write mode |
a+ | Opens a text file in read and write mode |
Rb | Opens a binary file in read mode |
Wb | Opens a binary file in write mode |
Ab | Opens a binary file in append mode |
Rb+ | Opens a binary file in read and write mode |
Wb+ | Opens a binary file in read and write mode |
Ab+ | Opens a binary file in read and write mode |
The fopen function works in the following way.
- Firstly, It searches the file to be opened.
- Then, it loads the file from the disk and place it into the buffer. The buffer is used to provide efficiency for the read operations.
- It sets up a character pointer which points to the first character of the file.
Consider the following example which opens a file in write mode.
- #include<stdio.h>
- Void main( )
- {
- FILE *fp ;
- Char ch ;
- Fp = fopen("file_handle.c","r") ;
- While ( 1 )
- {
- Ch = fgetc ( fp ) ;
- If ( ch == EOF )
- Break ;
- Printf("%c",ch) ;
- }
- Fclose (fp ) ;
- }
Output
The content of the file will be printed.
#include;
Void main( )
{
FILE *fp; // file pointer
Char ch;
Fp = fopen("file_handle.c","r");
While ( 1 )
{
Ch = fgetc ( fp ); //Each character of the file is read and stored in the character file.
If ( ch == EOF )
Break;
Printf("%c",ch);
}
Fclose (fp );
}
Closing File: fclose()
The fclose() function is used to close a file. The file must be closed after performing all the operations on it. The syntax of fclose() function is given below:
- Int fclose( FILE *fp );
C fprintf() and fscanf()
C fputc() and fgetc()
C fputs() and fgets()
C fseek()
We are using the iostream standard library, it provides cin and cout methods for reading from input and writing to output respectively.
To read and write from a file we are using the standard C++ library called fstream. Let us see the data types define in fstream library is:
Data Type | Description |
Fstream | It is used to create files, write information to files, and read information from files. |
Ifstream | It is used to read information from files. |
Ofstream | It is used to create files and write information to the files. |
FileStream example: writing to a file
Let's see the simple example of writing to a text file testout.txt using C++ FileStream programming.
- #include <iostream>
- #include <fstream>
- Using namespace std;
- Int main () {
- Ofstream filestream("testout.txt");
- If (filestream.is_open())
- {
- Filestream << "Welcome to javaTpoint.\n";
- Filestream << "C++ Tutorial.\n";
- Filestream.close();
- }
- Else cout <<"File opening is fail.";
- Return 0;
- }
Output:
The content of a text file testout.txt is set with the data:
Welcome to javaTpoint.
C++ Tutorial.
FileStream example: reading from a file
Let's see the simple example of reading from a text file testout.txt using C++ FileStream programming.
- #include <iostream>
- #include <fstream>
- Using namespace std;
- Int main () {
- String srg;
- Ifstream filestream("testout.txt");
- If (filestream.is_open())
- {
- While ( getline (filestream,srg) )
- {
- Cout << srg <<endl;
- }
- Filestream.close();
- }
- Else {
- Cout << "File opening is fail."<<endl;
- }
- Return 0;
- }
Note: Before running the code a text file named as "testout.txt" is need to be created and the content of a text file is given below:
Welcome to javaTpoint.
C++ Tutorial.
Output:
Welcome to javaTpoint.
C++ Tutorial.
Read and Write Example
Let's see the simple example of writing the data to a text file testout.txt and then reading the data from the file using C++ FileStream programming.
- #include <fstream>
- #include <iostream>
- Using namespace std;
- Int main () {
- Char input[75];
- Ofstream os;
- Os.open("testout.txt");
- Cout <<"Writing to a text file:" << endl;
- Cout << "Please Enter your name: ";
- Cin.getline(input, 100);
- Os << input << endl;
- Cout << "Please Enter your age: ";
- Cin >> input;
- Cin.ignore();
- Os << input << endl;
- Os.close();
- Ifstream is;
- String line;
- Is.open("testout.txt");
- Cout << "Reading from a text file:" << endl;
- While (getline (is,line))
- {
- Cout << line << endl;
- }
- Is.close();
- Return 0;
- }
Output:
Writing to a text file:
Please Enter your name: Nakul Jain
Please Enter your age: 22
Reading from a text file: Nakul Jain
22
In C++ stream refers to the stream of characters that are transferred between the program thread and i/o.
Stream classes in C++ are used to input and output operations on files and io devices. These classes have specific features and to handle input and output of the program.
The iostream.h library holds all the stream classes in the C++ programming language.
Let's see the hierarchy and learn about them,
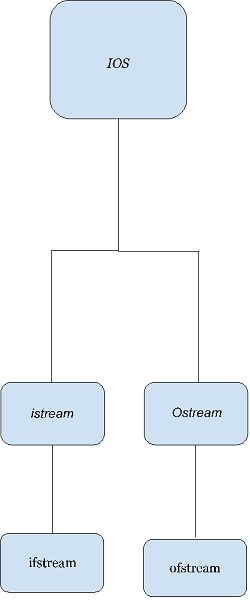
Now, let’s learn about the classes of the iostream library.
Ios class − This class is the base class for all stream classes. The streams can be input or output streams. This class defines members that are independent of how the templates of the class are defined.
Istream Class − The istream class handles the input stream in c++ programming language. These input stream objects are used to read and interpret the input as a sequence of characters. The cin handles the input.
Ostream class − The ostream class handles the output stream in c++ programming language. These output stream objects are used to write data as a sequence of characters on the screen. Cout and puts handle the out streams in c++ programming language.
Example
OUT STREAM
COUT
#include <iostream>
Using namespace std;
Int main(){
Cout<<"This output is printed on screen";
}
Output
This output is printed on screen
PUTS
#include <iostream>
Using namespace std;
Int main(){
Puts("This output is printed using puts");
}
Output
This output is printed using puts
IN STREAM
CIN
#include <iostream>
Using namespace std;
Int main(){
Int no;
Cout<<"Enter a number ";
Cin>>no;
Cout<<"Number entered using cin is "<
Output
Enter a number 3453
Number entered using cin is 3453
Gets
#include <iostream>
Using namespace std;
Int main(){
Char ch[10];
Puts("Enter a character array");
Gets(ch);
Puts("The character array entered using gets is : ");
Puts(ch);
}
Output
Enter a character array
Thdgf
The character array entered using gets is :
Thdgf
These handle data in bytes (8 bits) i.e., the byte stream classes read/write data of 8 bits. Using these you can store characters, videos, audios, images etc.
The InputStream and OutputStream classes (abstract) are the super classes of all the input/output stream classes: classes that are used to read/write a stream of bytes. Following are the byte array stream classes provided by Java −
InputStream | OutputStream |
FIleInputStream | FileOutputStream |
ByteArrayInputStream | ByteArrayOutputStream |
ObjectInputStream | ObjectOutputStream |
PipedInputStream | PipedOutputStream |
FilteredInputStream | FilteredOutputStream |
BufferedInputStream | BufferedOutputStream |
DataInputStream | DataOutputStream |
Example
Following Java program reads data from a particular file using FileInputStream and writes it to another, using FileOutputStream.
Import java.io.File;
Import java.io.FileInputStream;
Import java.io.FileOutputStream;
Import java.io.IOException;
Public class IOStreamsExample {
Public static void main(String args[]) throws IOException {
//Creating FileInputStream object
File file = new File("D:/myFile.txt");
FileInputStream fis = new FileInputStream(file);
Byte bytes[] = new byte[(int) file.length()];
//Reading data from the file
Fis.read(bytes);
//Writing data to another file
File out = new File("D:/CopyOfmyFile.txt");
FileOutputStream outputStream = new FileOutputStream(out);
//Writing data to the file
OutputStream.write(bytes);
OutputStream.flush();
System.out.println("Data successfully written in the specified file");
}
}
Output
Data successfully written in the specified file
Character Streams − These handle data in 16 bit Unicode. Using these you can read and write text data only.
The Reader and Writer classes (abstract) are the super classes of all the character stream classes: classes that are used to read/write character streams. Following are the character array stream classes provided by Java −
Reader | Writer |
BufferedReader | BufferedWriter |
CharacterArrayReader | CharacterArrayWriter |
StringReader | StringWriter |
FileReader | FileWriter |
InputStreamReader | InputStreamWriter |
FileReader | FileWriter |
Example
The following Java program reads data from a particular file using FileReader and writes it to another, using FileWriter.
Import java.io.File;
Import java.io.FileReader;
Import java.io.FileWriter;
Import java.io.IOException;
Public class IOStreamsExample {
Public static void main(String args[]) throws IOException {
//Creating FileReader object
File file = new File("D:/myFile.txt");
FileReader reader = new FileReader(file);
Char chars[] = new char[(int) file.length()];
//Reading data from the file
Reader.read(chars);
//Writing data to another file
File out = new File("D:/CopyOfmyFile.txt");
FileWriter writer = new FileWriter(out);
//Writing data to the file
Writer.write(chars);
Writer.flush();
System.out.println("Data successfully written in the specified file");
}
}
Output
Data successfully written in the specified file
A stream can be defined as a sequence of data. The InputStream is used to read data from a source and the OutputStream is used for writing data to a destination. InputStream and OutputStream are the basic stream classes in Java.
All the other streams just add capabilities to the basics, like the ability to read a whole chunk of data at once for performance reasons (BufferedInputStream) or convert from one kind of character set to Java's native unicode (Reader), or say where the data is coming from (FileInputStream, SocketInputStream and ByteArrayInputStream, etc.)
Why we need Stream Classes?
To perform read and write operation on binary files we need a mechanism to read that binary data on file/to write binary data (i.e. in the form of byte). These classes are capable to read and write one byte on binary files. That’s why we use Stream Classes.
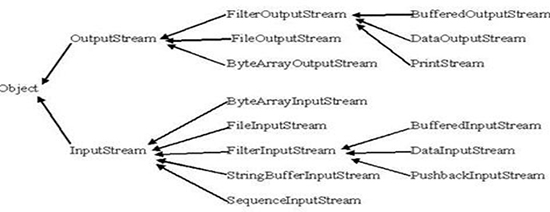
Figure 1: Stream Classes
Stream Classes:
Hierarchy of classes for Input and Output streams.
Here Object is the sequence of character i.e. Stream.
1. FileInputStream and FileOutputStream Classes:
FileInputStream Class:
A FileInputStream is an InputStream to obtain bytes from a file. It is used for reading inputs of raw bytes such as image data. It can read byte oriented data.
Constructor to take file for input:
InputStream f=new FileInputStream(“C:/JavaProg/Demo”);
Here, f is an object of InputStream class and argument of FileInputStream is that path where our file is located.
Methods of FileInputStream:
1. | Public void close () throws IOException {} |
2. | Protected void finalize () throws IOException {} |
3. | Public int read (int r) throws IOException {} |
4. | Public int read (int r) throws IOException {} |
5. | Public int available() throws IOException{} Gives the number of bytes that can be read from this file input stream. Returns an int. |
Listing 1: Demo of FileInputStream Class
Import java.io.*;
Public class fileInputStreamDemo {
Public static void main (String args[]){
Try {
Byte bWrite [] = {10, 20,30,40,50};
OutputStream os = new FileOutputStream("C:/test.txt");
For(int x=0; x < bWrite.length ; x++){
Os.write( bWrite[x] ); // writes the bytes
}
Os.close();
InputStream is = new FileInputStream("C:/test.txt");
Int size = is.available();
For(int i=0; i< size; i++){
System.out.print((char)is.read() + " ");
}
Is.close();
}catch(IOException e){
System.out.print("Exception");
}
}
}
Output of the program is:
10 20 30 40 50
A FileOutputStream is used to create a file and write data into it. The stream would create a file, if it doesn't already exist, before opening it for output.
Constructors for FileOutputStream Class:
Constructor | Description |
FileOutputStream(File file) | Creates a file output stream to write to the file represented by the specified File object. |
FileOutputStream(File file, boolean append) | Creates a file output stream to write to the file represented by the specified File object. |
FileOutputStream(String name) | Creates an output file stream to write to the file with the specified name. |
FileOutputStream(String name, boolean append) | Creates an output file stream to write to the file with the specified name. |
Methods of FileOutStream:
Constructor | Description |
Void close() | This method closes this file output stream and releases any system resources associated with this stream |
Protected void finalize() | This method cleans up the connection to the file, and ensures that the close method of this file output stream is called when there are no more references to this stream. |
FileChannel getChannel() | This method returns the unique FileChannel object associated with this file output stream. |
FileDescriptor getFD() | This method returns the file descriptor associated with this stream. |
Void write(byte[] b) | This method writes b.length bytes from the specified byte array to this file output stream. |
Void write(byte[] b, int off, int len) | This method writes len bytes from the specified byte array starting at offset off to this file output stream. |
Void write(int b) | This method writes the specified byte to this file output stream. |
Example:
Listing 2: Program to demonstrate FileOutputStream Class
Import java.io.FileInputStream;
Import java.io.FileOutputStream;
Import java.io.IOException;
Public class FileOutputStreamDemo {
Public static void main(String[] args) throws IOException {
FileOutputStream fos = null;
FileInputStream fis = null;
Int i=0;
Char c;
Try{
// create new file output stream
Fos=new FileOutputStream("C://test.txt");
// writes byte to the output stream
Fos.write(b, 2, 3);
// flushes the content to the underlying stream
Fos.flush();
// create new file input stream
Fis = new FileInputStream("C://test.txt");
// read till the end of the file
While ((i=fis.read())!=-1)
{
// convert integer to character
c=(char)i;
// prints
System.out.print(c);
}
// if an error occurs
e.printStackTrace ();
} finally {
// closes and releases system resources from stream
If(fos!=null)
Fos.close();
If(fis!=null)
Fis.close();
}
}
}
Output of the Program is:
CDE
2. ByteArrayInputStream and ByteArrayOutputStream Class:
ByteArrayInputStream:
This class contains an internal buffer that contains bytes that may be read from the stream. An internal counter keeps track of the next byte to be supplied by the read method. The methods in this class can be called after the stream has been closed without generating an IOException.
Constructors for ByteArrayInputStream:
Constructor | Description |
ByteArrayInputStream(byte[] buf) | This creates a ByteArrayInputStream so that it uses buf as its buffer array. |
ByteArrayInputStream(byte[] buf, int offset, int length) | This creates ByteArrayInputStream that uses buf as its buffer array. |
Methods of ByteArrayInputStream:
Methods | Description |
Int available() | This method returns the number of remaining bytes that can be read (or skipped over) from this input stream. |
Void close() | Closing a ByteArrayInputStream has no effect. |
Void mark(int readAheadLimit) | This method set the current marked position in the stream. |
Boolean markSupported() | This method tests if this InputStream supports mark/reset. |
Int read() | This method reads the next byte of data from this input stream. |
Int read(byte[] b, int off, int len) | This method reads up to len bytes of data into an array of bytes from this input stream. |
Void reset() | This method resets the buffer to the marked position. |
Long skip(long n) | This method skips n bytes of input from this input stream. |
ByteArrayOutputStream:
This class implements an output stream in which the data is written into a byte array. The buffer automatically grows as data is written to it. The methods in this class can be called after the stream has been closed without generating an IOException.
Constructors for ByteArrayInputStream:
Constructor | Description |
ByteArrayOutputStream() | This creates a new byte array output stream. |
ByteArrayOutputStream (int size) | This creates a new byte array output stream, with a buffer capacity of the specified size, in bytes. |
Methods of ByteArrayInputStream:
Methods | Description |
Int size() | This method returns the current size of the buffer. |
Void close() | Closing a ByteArrayInputStream has no effect. |
Void reset() | This method resets the count field of this byte array output stream to zero, so that all currently accumulated output in the output stream is discarded. |
String toString(String charsetName) | This method converts the buffer's contents into a string decoding bytes using the platform's default character set. |
Byte[] toByteArray() | This method creates a newly allocated byte array. |
Void write(byte[] b, int off, int len) | This method writes len bytes from the specified byte array starting at offset off to this byte array output stream. |
Void write(int b) | This method Writes the specified byte to this byte array output stream. |
Void writeTo(OutputStream out) | This method writes the complete contents of this byte array output stream to the specified output stream argument, as if by calling the output stream's write method using out.write(buf, 0, count). |
Example:
Listing 3: Program to demonstrate ByteArrayInputStream and ByteArrayOutputStream:
Import java.io.*;
Public class ByteStreamTest {
Public static void main(String args[])throws IOException {
ByteArrayOutputStream bOutput = new ByteArrayOutputStream(12);
While( bOutput.size()!= 10 ) {
// Gets the inputs from the user
BOutput.write(System.in.read());
}
Byte b [] = bOutput.toByteArray();
System.out.println("Print the content");
For(int x= 0 ; x < b.length; x++) {
// printing the characters
System.out.print((char)b[x] + " ");
}
System.out.println(" ");
Int c;
ByteArrayInputStream bInput = new ByteArrayInputStream(b);
System.out.println("Converting characters to Upper case " );
For(int y = 0 ; y < 1; y++ ) {
While(( c= bInput.read())!= -1) {
System.out.println(Character.toUpperCase((char)c));
}
BInput.reset();
}
}
}
Output of the Program is:
Asdfghjkly
Print the content
a s d f g h j k l y
Converting characters to Upper case
A
S
D
F
G
H
J
K
L
Y
Conclusion
FileInput and FileOutput streams are the streams that are used to read/write byte of data to a file. While ByteInputArray and ByteOutputArray read/write bytes of data at a time because it deals with array of byte and works faster than FileInput and FileOutput Stream.
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Stream
A stream can be defined as a sequence of data. There are two kinds of Streams −
- InPutStream − The InputStream is used to read data from a source.
- OutPutStream − The OutputStream is used for writing data to a destination.

Java provides strong but flexible support for I/O related to files and networks but this tutorial covers very basic functionality related to streams and I/O. We will see the most commonly used examples one by one −
Byte Streams
Java byte streams are used to perform input and output of 8-bit bytes. Though there are many classes related to byte streams but the most frequently used classes are, FileInputStream and FileOutputStream. Following is an example which makes use of these two classes to copy an input file into an output file −
Example
Import java.io.*;
Public class CopyFile {
Public static void main(String args[]) throws IOException {
FileInputStream in = null;
FileOutputStream out = null;
Try {
In = new FileInputStream("input.txt");
Out = new FileOutputStream("output.txt");
Int c;
While ((c = in.read()) != -1) {
Out.write(c);
}
}finally {
If (in != null) {
In.close();
}
If (out != null) {
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Character Streams
Java Byte streams are used to perform input and output of 8-bit bytes, whereas Java Character streams are used to perform input and output for 16-bit unicode. Though there are many classes related to character streams but the most frequently used classes are, FileReader and FileWriter. Though internally FileReader uses FileInputStream and FileWriter uses FileOutputStream but here the major difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
We can re-write the above example, which makes the use of these two classes to copy an input file (having unicode characters) into an output file −
Example
Import java.io.*;
Public class CopyFile {
Public static void main(String args[]) throws IOException {
FileReader in = null;
FileWriter out = null;
Try {
In = new FileReader("input.txt");
Out = new FileWriter("output.txt");
Int c;
While ((c = in.read()) != -1) {
Out.write(c);
}
}finally {
If (in != null) {
In.close();
}
If (out != null) {
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Standard Streams
All the programming languages provide support for standard I/O where the user's program can take input from a keyboard and then produce an output on the computer screen. If you are aware of C or C++ programming languages, then you must be aware of three standard devices STDIN, STDOUT and STDERR. Similarly, Java provides the following three standard streams −
- Standard Input − This is used to feed the data to user's program and usually a keyboard is used as standard input stream and represented as System.in.
- Standard Output − This is used to output the data produced by the user's program and usually a computer screen is used for standard output stream and represented as System.out.
- Standard Error − This is used to output the error data produced by the user's program and usually a computer screen is used for standard error stream and represented as System.err.
Following is a simple program, which creates InputStreamReader to read standard input stream until the user types a "q" −
Example
Import java.io.*;
Public class ReadConsole {
Public static void main(String args[]) throws IOException {
InputStreamReader cin = null;
Try {
Cin = new InputStreamReader(System.in);
System.out.println("Enter characters, 'q' to quit.");
Char c;
Do {
c = (char) cin.read();
System.out.print(c);
} while(c != 'q');
}finally {
If (cin != null) {
Cin.close();
}
}
}
}
Let's keep the above code in ReadConsole.java file and try to compile and execute it as shown in the following program. This program continues to read and output the same character until we press 'q' −
$javac ReadConsole.java
$java ReadConsole
Enter characters, 'q' to quit.
1
1
e
e
q
q
Reading and Writing Files
As described earlier, a stream can be defined as a sequence of data. The InputStream is used to read data from a source and the OutputStream is used for writing data to a destination.
Here is a hierarchy of classes to deal with Input and Output streams.
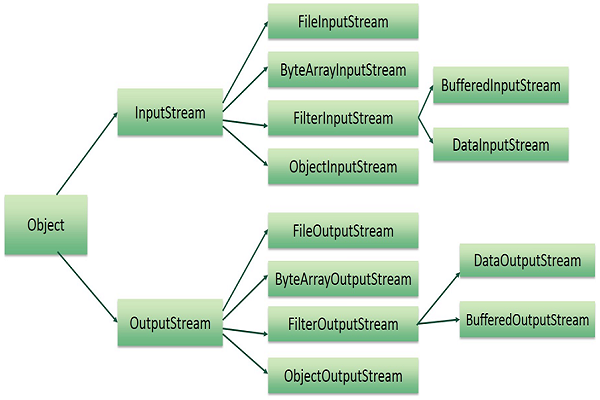
The two important streams are FileInputStream and FileOutputStream, which would be discussed in this tutorial.
FileInputStream
This stream is used for reading data from the files. Objects can be created using the keyword new and there are several types of constructors available.
Following constructor takes a file name as a string to create an input stream object to read the file −
InputStream f = new FileInputStream("C:/java/hello");
Following constructor takes a file object to create an input stream object to read the file. First we create a file object using File() method as follows −
File f = new File("C:/java/hello");
InputStream f = new FileInputStream(f);
Once you have InputStream object in hand, then there is a list of helper methods which can be used to read to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public int read(int r)throws IOException{} This method reads the specified byte of data from the InputStream. Returns an int. Returns the next byte of data and -1 will be returned if it's the end of the file. |
4 | Public int read(byte[] r) throws IOException{} This method reads r.length bytes from the input stream into an array. Returns the total number of bytes read. If it is the end of the file, -1 will be returned. |
5 | Public int available() throws IOException{} Gives the number of bytes that can be read from this file input stream. Returns an int. |
There are other important input streams available, for more detail you can refer to the following links −
- ByteArrayInputStream
- DataInputStream
FileOutputStream
FileOutputStream is used to create a file and write data into it. The stream would create a file, if it doesn't already exist, before opening it for output.
Here are two constructors which can be used to create a FileOutputStream object.
Following constructor takes a file name as a string to create an input stream object to write the file −
OutputStream f = new FileOutputStream("C:/java/hello")
Following constructor takes a file object to create an output stream object to write the file. First, we create a file object using File() method as follows −
File f = new File("C:/java/hello");
OutputStream f = new FileOutputStream(f);
Once you have OutputStream object in hand, then there is a list of helper methods, which can be used to write to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public void write(int w)throws IOException{} This methods writes the specified byte to the output stream. |
4 | Public void write(byte[] w) Writes w.length bytes from the mentioned byte array to the OutputStream. |
There are other important output streams available, for more detail you can refer to the following links −
- ByteArrayOutputStream
- DataOutputStream
Example
Following is the example to demonstrate InputStream and OutputStream −
Import java.io.*;
Public class fileStreamTest {
Public static void main(String args[]) {
Try {
Byte bWrite [] = {11,21,3,40,5};
OutputStream os = new FileOutputStream("test.txt");
For(int x = 0; x < bWrite.length ; x++) {
Os.write( bWrite[x] ); // writes the bytes
}
Os.close();
InputStream is = new FileInputStream("test.txt");
Int size = is.available();
For(int i = 0; i < size; i++) {
System.out.print((char)is.read() + " ");
}
Is.close();
} catch (IOException e) {
System.out.print("Exception");
}
}
}
The above code would create file test.txt and would write given numbers in binary format. Same would be the output on the stdout screen.
File Navigation and I/O
There are several other classes that we would be going through to get to know the basics of File Navigation and I/O.
- File Class
- FileReader Class
- FileWriter Class
Directories in Java
A directory is a File which can contain a list of other files and directories. You use File object to create directories, to list down files available in a directory. For complete detail, check a list of all the methods which you can call on File object and what are related to directories.
Creating Directories
There are two useful File utility methods, which can be used to create directories −
- The mkdir( ) method creates a directory, returning true on success and false on failure. Failure indicates that the path specified in the File object already exists, or that the directory cannot be created because the entire path does not exist yet.
- The mkdirs() method creates both a directory and all the parents of the directory.
Following example creates "/tmp/user/java/bin" directory −
Example
Import java.io.File;
Public class CreateDir {
Public static void main(String args[]) {
String dirname = "/tmp/user/java/bin";
File d = new File(dirname);
// Create directory now.
d.mkdirs();
}
}
Compile and execute the above code to create "/tmp/user/java/bin".
Note − Java automatically takes care of path separators on UNIX and Windows as per conventions. If you use a forward slash (/) on a Windows version of Java, the path will still resolve correctly.
Listing Directories
You can use list( ) method provided by File object to list down all the files and directories available in a directory as follows −
Example
Import java.io.File;
Public class ReadDir {
Public static void main(String[] args) {
File file = null;
String[] paths;
Try {
// create new file object
File = new File("/tmp");
// array of files and directory
Paths = file.list();
// for each name in the path array
For(String path:paths) {
// prints filename and directory name
System.out.println(path);
}
} catch (Exception e) {
// if any error occurs
e.printStackTrace();
}
}
}
This will produce the following result based on the directories and files available in your /tmp directory −
Output
Test1.txt
Test2.txt
ReadDir.java
ReadDir.class
The File class is an abstract representation of file and directory pathname. A pathname can be either absolute or relative.
The File class have several methods for working with directories and files such as creating new directories or files, deleting and renaming directories or files, listing the contents of a directory etc.
Fields
Modifier | Type | Field | Description |
Static | String | PathSeparator | It is system-dependent path-separator character, represented as a string for convenience. |
Static | Char | PathSeparatorChar | It is system-dependent path-separator character. |
Static | String | Separator | It is system-dependent default name-separator character, represented as a string for convenience. |
Static | Char | SeparatorChar | It is system-dependent default name-separator character. |
Constructors
Constructor | Description |
File(File parent, String child) | It creates a new File instance from a parent abstract pathname and a child pathname string. |
File(String pathname) | It creates a new File instance by converting the given pathname string into an abstract pathname. |
File(String parent, String child) | It creates a new File instance from a parent pathname string and a child pathname string. |
File(URI uri) | It creates a new File instance by converting the given file: URI into an abstract pathname. |
Useful Methods
Modifier and Type | Method | Description |
Static File | CreateTempFile(String prefix, String suffix) | It creates an empty file in the default temporary-file directory, using the given prefix and suffix to generate its name. |
Boolean | CreateNewFile() | It atomically creates a new, empty file named by this abstract pathname if and only if a file with this name does not yet exist. |
Boolean | CanWrite() | It tests whether the application can modify the file denoted by this abstract pathname.String[] |
Boolean | CanExecute() | It tests whether the application can execute the file denoted by this abstract pathname. |
Boolean | CanRead() | It tests whether the application can read the file denoted by this abstract pathname. |
Boolean | IsAbsolute() | It tests whether this abstract pathname is absolute. |
Boolean | IsDirectory() | It tests whether the file denoted by this abstract pathname is a directory. |
Boolean | IsFile() | It tests whether the file denoted by this abstract pathname is a normal file. |
String | GetName() | It returns the name of the file or directory denoted by this abstract pathname. |
String | GetParent() | It returns the pathname string of this abstract pathname's parent, or null if this pathname does not name a parent directory. |
Path | ToPath() | It returns a java.nio.file.Path object constructed from the this abstract path. |
URI | ToURI() | It constructs a file: URI that represents this abstract pathname. |
File[] | ListFiles() | It returns an array of abstract pathnames denoting the files in the directory denoted by this abstract pathname |
Long | GetFreeSpace() | It returns the number of unallocated bytes in the partition named by this abstract path name. |
String[] | List(FilenameFilter filter) | It returns an array of strings naming the files and directories in the directory denoted by this abstract pathname that satisfy the specified filter. |
Boolean | Mkdir() | It creates the directory named by this abstract pathname. |
Java File Example 1
- Import java.io.*;
- Public class FileDemo {
- Public static void main(String[] args) {
- Try {
- File file = new File("javaFile123.txt");
- If (file.createNewFile()) {
- System.out.println("New File is created!");
- } else {
- System.out.println("File already exists.");
- }
- } catch (IOException e) {
- e.printStackTrace();
- }
- }
- }
Output:
New File is created!
Java File Example 2
- Import java.io.*;
- Public class FileDemo2 {
- Public static void main(String[] args) {
- String path = "";
- Boolean bool = false;
- Try {
- // createing new files
- File file = new File("testFile1.txt");
- File.createNewFile();
- System.out.println(file);
- // createing new canonical from file object
- File file2 = file.getCanonicalFile();
- // returns true if the file exists
- System.out.println(file2);
- Bool = file2.exists();
- // returns absolute pathname
- Path = file2.getAbsolutePath();
- System.out.println(bool);
- // if file exists
- If (bool) {
- // prints
- System.out.print(path + " Exists? " + bool);
- }
- } catch (Exception e) {
- // if any error occurs
- e.printStackTrace();
- }
- }
- }
Output:
TestFile1.txt
/home/Work/Project/File/testFile1.txt
True
/home/Work/Project/File/testFile1.txt Exists? true
Java File Example 3
- Import java.io.*;
- Public class FileExample {
- Public static void main(String[] args) {
- File f=new File("/Users/sonoojaiswal/Documents");
- String filenames[]=f.list();
- For(String filename:filenames){
- System.out.println(filename);
- }
- }
- }
Output:
"info.properties"
"info.properties".rtf
.DS_Store
.localized
Alok news
Apache-tomcat-9.0.0.M19
Apache-tomcat-9.0.0.M19.tar
Bestreturn_org.rtf
BIODATA.pages
BIODATA.pdf
BIODATA.png
Struts2jars.zip
Workspace
Java File Example 4
- Import java.io.*;
- Public class FileExample {
- Public static void main(String[] args) {
- File dir=new File("/Users/sonoojaiswal/Documents");
- File files[]=dir.listFiles();
- For(File file:files){
- System.out.println(file.getName()+" Can Write: "+file.canWrite()+"
- Is Hidden: "+file.isHidden()+" Length: "+file.length()+" bytes");
- }
- }
- }
Output:
"info.properties" Can Write: true Is Hidden: false Length: 15 bytes
"info.properties".rtf Can Write: true Is Hidden: false Length: 385 bytes
.DS_Store Can Write: true Is Hidden: true Length: 36868 bytes
.localized Can Write: true Is Hidden: true Length: 0 bytes
Alok news Can Write: true Is Hidden: false Length: 850 bytes
Apache-tomcat-9.0.0.M19 Can Write: true Is Hidden: false Length: 476 bytes
Apache-tomcat-9.0.0.M19.tar Can Write: true Is Hidden: false Length: 13711360 bytes
Bestreturn_org.rtf Can Write: true Is Hidden: false Length: 389 bytes
BIODATA.pages Can Write: true Is Hidden: false Length: 707985 bytes
BIODATA.pdf Can Write: true Is Hidden: false Length: 69681 bytes
BIODATA.png Can Write: true Is Hidden: false Length: 282125 bytes
Workspace Can Write: true Is Hidden: false Length: 1972 bytes
Introduction
The java.io.Exceptions provides for system input and output through data streams, serialization and the file system.
Interface Summary
Sr.No. | Interface & Description |
1 | CharConversionException This is a base class for character conversion exceptions. |
2 | EOFException These are signals that an end of file or end of stream has been reached unexpectedly during input. |
3 | FileNotFoundException These are the signals that an attempt to open the file denoted by a specified pathname has failed. |
4 | InterruptedIOException This is signals that an I/O operation has been interrupted. |
5 | InvalidClassException This is thrown when the Serialization runtime detects one of the following problems with a Class. |
6 | InvalidObjectException This indicates that one or more deserialized objects failed validation tests. |
7 | IOException These are the signals that an I/O exception of some sort has occurred. |
8 | NotActiveException This is thrown when serialization or deserialization is not active. |
9 | NotSerializableException This is thrown when an instance is required to have a Serializable interface. |
10 | ObjectStreamException This is a superclass of all exceptions specific to Object Stream classes. |
11 | OptionalDataException This is an exception indicating the failure of an object read operation due to unread primitive data, or the end of data belonging to a serialized object in the stream. |
12 | StreamCorruptedException This is thrown when control information that was read from an object stream violates internal consistency checks. |
13 | SyncFailedException These are the signals that a sync operation has failed. |
14 | UnsupportedEncodingException This character encoding is not supported. |
15 | UTFDataFormatException This are signals that a malformed string in modified UTF-8 format has been read in a data input stream or by any class that implements the data input interface. |
16 | WriteAbortedException This are signals that one of the ObjectStreamExceptions was thrown during a write operation. |
Creating a file is easy by using pre-defined classes and packages. There are three ways to create a file.
- Using File.createNewFile() method
- Using FileOutputStream class
- Using File.createFile() method
Java File.createNewFile() method
The File.createNewFile() is a method of File class which belongs to a java.io package. It does not accept any argument. The method automatically creates a new, empty file. The method returns a boolean value:
- True, if the file created successfully.
- False, if the file already exists.
When we initialize File class object, we provide the file name and then we can call createNewFile() method of the File class to create a new file in Java.
The File.createNewFile() method throws java.io.IOException if an I/O error occurred. It also throws SecurityException if a security manager exists and its SecurityManager.checkWriter(java.lang.String) method denies write access to the file. The signature of the method is:
- Public boolean createNewFile() throws IOException
We can pass the file name or absolute path or relative path as an argument in the File class object. For a non-absolute path, File object tries to locate the file in the current directory.
Example
The following example creates a new, empty text file. The first run creates music.txt successfully while on the second run it failed. We can create any type of file by changing the file extension only.
- Import java.io.File;
- Import java.io.IOException;
- Public class CreateFileExample1
- {
- Public static void main(String[] args)
- {
- File file = new File("C:\\demo\\music.txt"); //initialize File object and passing path as argument
- Boolean result;
- Try
- {
- Result = file.createNewFile(); //creates a new file
- If(result) // test if successfully created a new file
- {
- System.out.println("file created "+file.getCanonicalPath()); //returns the path string
- }
- Else
- {
- System.out.println("File already exist at location: "+file.getCanonicalPath());
- }
- }
- Catch (IOException e)
- {
- e.printStackTrace(); //prints exception if any
- }
- }
- }
Output
When file does not exists.

When file already exists.

Java FileOutputStream
A file Output stream writes data to a file. Java FileOutputStream class also provide support for files. It belongs to the java.io package. It stores the data into bytes. We use FileOutputStream class when we need to write some data into the created file. The FileOutputStream class provides a constructor to create a file. The signature of the constructor is:
- Public FileOutputStream(String name, boolean append) throws FileNotFoundException
Parameters
Name: is the file name
Append: if true, byte will be written to the end of the file, not in the beginning.
Example
In the following example, we have created a file using FileOutputStream.
- Import java.io.FileOutputStream;
- Import java.util.Scanner;
- Public class CreateFileExample
- {
- Public static void main(String args[])
- {
- Try
- {
- Scanner sc=new Scanner(System.in); //object of Scanner class
- System.out.print("Enter the file name: ");
- String name=sc.nextLine(); //variable name to store the file name
- FileOutputStream fos=new FileOutputStream(name, true); // true for append mode
- System.out.print("Enter file content: ");
- String str=sc.nextLine()+"\n"; //str stores the string which we have entered
- Byte[] b= str.getBytes(); //converts string into bytes
- Fos.write(b); //writes bytes into file
- Fos.close(); //close the file
- System.out.println("file saved.");
- }
- Catch(Exception e)
- {
- e.printStackTrace();
- }
- }
- }
Output
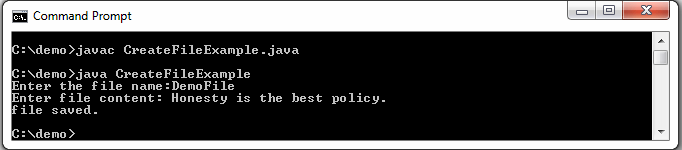
Java File.createFile() method
The File.createFile() is a method of File class which belongs to java.nio.file package. It also provides support for files. The nio package is buffer-oriented. The createFile() method is also used to create a new, empty file. We don't need to close the resources when using this method. It is an advantage. The signature of the method is:
- Public static Path createFile(Path, Attribute) throws IOException
Path: The path of the file.
Attribute: An optional list of file attributes.
The method returns the file.
The following example also creates a new, empty file. We create a Path instance using a static method in the Paths class (java.nio.file.Paths) named Paths.get(). Notice the following statement:
Path path = Paths.get("C:\\demo\\javaprogram.txt");
In the above line Path is an interface and Paths is a class. Both belongs to same package. The Paths.get() method creates the Path Instance.
- Import java.io.IOException;
- Import java.nio.file.*;
- Public class CreateFileExample3
- {
- Public static void main(String[] args)
- {
- Path path = Paths.get("C:\\demo\\javaprogram.txt"); //creates Path instance
- Try
- {
- Path p= Files.createFile(path); //creates file at specified location
- System.out.println("File Created at Path: "+p);
- }
- Catch (IOException e)
- {
- e.printStackTrace();
- }
- }
- }
Output

Character Streams − These handle data in 16 bit Unicode. Using these you can read and write text data only.
The Reader and Writer classes (abstract) are the super classes of all the character stream classes: classes that are used to read/write character streams. Following are the character array stream classes provided by Java −
Reader | Writer |
BufferedReader | BufferedWriter |
CharacterArrayReader | CharacterArrayWriter |
StringReader | StringWriter |
FileReader | FileWriter |
InputStreamReader | InputStreamWriter |
FileReader | FileWriter |
Example
The following Java program reads data from a particular file using FileReader and writes it to another, using FileWriter.
Import java.io.File;
Import java.io.FileReader;
Import java.io.FileWriter;
Import java.io.IOException;
Public class IOStreamsExample {
Public static void main(String args[]) throws IOException {
//Creating FileReader object
File file = new File("D:/myFile.txt");
FileReader reader = new FileReader(file);
Char chars[] = new char[(int) file.length()];
//Reading data from the file
Reader.read(chars);
//Writing data to another file
File out = new File("D:/CopyOfmyFile.txt");
FileWriter writer = new FileWriter(out);
//Writing data to the file
Writer.write(chars);
Writer.flush();
System.out.println("Data successfully written in the specified file");
}
}
Output
Data successfully written in the specified file
Usually data is much more complicated than the data for the example program. But the program shows the outer logic that is needed to read most files. Sometimes the data in a file represents something other than a primitive Java type. For example, word processor files contain various arrangements of bytes that encode fonts, page formats, special symbols, and graphics.
The DataOutputStream and DataInputStream classes have several methods for reading and writing single bytes.
WriteByte() writes the least significant byte of its int argument to the output stream. ReadUnsignedByte() reads a byte from the input stream and puts it in the least significant byte of its return value.
DataOutputStream:
Public final void writeByte(int b) throws IOException
DataInputStream:
Public final int readUnsignedByte() throws IOException
Not everything in Java is an object. There is a special group of data types (also known as primitive types) that will be used quite often in programming. For performance reasons, the designers of the Java language decided to include these primitive types. Java determines the size of each primitive type. These sizes do not change from one operating system to another. This is one of the key features of the language that makes Java so portable. Java defines eight primitive types of data: byte, short, int, long, char, float, double, and boolean. The primitive types are also commonly referred to as simple types which can be put in four groups
- Integers: This group includes byte, short, int, and long, which are for whole-valued signed numbers.
- Floating-point numbers: This group includes float and double, which represent numbers with fractional precision.
- Characters: This group includes char, which represents symbols in a character set, like letters and numbers.
- Boolean: This group includes boolean, which is a special type for representing true/false values.
Let’s discuss each in details:
Byte
The smallest integer type is byte. It has a minimum value of -128 and a maximum value of 127 (inclusive). The byte data type can be useful for saving memory in large arrays, where the memory savings actually matters. Byte variables are declared by use of the byte keyword. For example, the following declares and initialize byte variables called b:
Byte b =100;
Short:
The short data type is a 16-bit signed two's complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). As with byte, the same guidelines apply: you can use a short to save memory in large arrays, in situations where the memory savings actually matters. Following example declares and initialize short variable called s:
Short s =123;
Int:
The most commonly used integer type is int. It is a signed 32-bit type that has a range from –2,147,483,648 to 2,147,483,647. In addition to other uses, variables of type int are commonly employed to control loops and to index arrays. This data type will most likely be large enough for the numbers your program will use, but if you need a wider range of values, use long instead.
Int v = 123543;
Int calc = -9876345;
Long:
Long is a signed 64-bit type and is useful for those occasions where an int type is not large enough to hold the desired value. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use of this data type might be in banking application when large amount is to be calculated and stored.
Long amountVal = 1234567891;
Float:
Floating-point numbers, also known as real numbers, are used when evaluating expressions that require fractional precision. For example interest rate calculation or calculating square root. The float data type is a single-precision 32-bit IEEE 754 floating point. As with the recommendations for byte and short, use a float (instead of double) if you need to save memory in large arrays of floating point numbers. The type float specifies a single-precision value that uses 32 bits of storage. Single precision is faster on some processors and takes half as much space as double precision. The declaration and initialization syntax for float variables given below, please note “f” after value initialization.
Float intrestRate = 12.25f;
Double:
Double precision, as denoted by the double keyword, uses 64 bits to store a value. Double precision is actually faster than single precision on some modern processors that have been optimized for high-speed mathematical calculations. All transcendental math functions, such as sin( ), cos( ), and sqrt( ), return double values. The declaration and initialization syntax for double variables given below, please note “d” after value initialization.
Double sineVal = 12345.234d;
Boolean:
The boolean data type has only two possible values: true and false. Use this data type for simple flags that track true/false conditions. This is the type returned by all relational operators, as in the case of a < b. Boolean is also the type required by the conditional expressions that govern the control statements such as if or while.
Boolean flag = true;
Booleanval = false;
Char:
In Java, the data type used to store characters is char. The char data type is a single 16-bit Unicode character. It has a minimum value of '\u0000' (or 0) and a maximum value of '\uffff' (or 65,535 inclusive). There are no negative chars.
Char ch1 = 88; // code for X
Char ch2 = 'Y';
Primitive Variables can be of two types
(1) Class level (instance) variable:
It's not mandatory to initialize Class level (instance) variable. If we do not initialize instance variable compiler will assign default value to it. Generally speaking, this default will be zero or null, depending on the data type. Relying on such default values, however, is generally considered bad coding practice.The following chart summarizes the default values for the above data types.
Primitive Data Type | Default Value 3.6 |
Byte | 0 |
Short | 0 |
Int | 0 |
Long | 0L |
Float | 0.0f |
Double | 0.0d |
Char | '\u0000' |
Boolean | False |
(2) Method local variable:
Method local variables have to be initialized before using it. The compiler never assigns a default value to an un-initialized local variable. If you cannot initialize your local variable where it is declared, make sure to assign it a value before you attempt to use it. Accessing an un-initialized local variable will result in a compile-time error.
Let’s See simple java program which declares, initialize and print all of primitive types.
Java class PrimitiveDemo as below:
Package primitive;
Public class PrimitiveDemo {
Public static void main(String[] args) {
Byte b =100;
Short s =123;
Int v = 123543;
Int calc = -9876345;
Long amountVal = 1234567891;
Float intrestRate = 12.25f;
Double sineVal = 12345.234d;
Boolean flag = true;
Boolean val = false;
Char ch1 = 88; // code for X
Char ch2 = 'Y';
System.out.println("byte Value = "+ b);
System.out.println("short Value = "+ s);
System.out.println("int Value = "+ v);
System.out.println("int second Value = "+ calc);
System.out.println("long Value = "+ amountVal);
System.out.println("float Value = "+ intrestRate);
System.out.println("double Value = "+ sineVal);
System.out.println("boolean Value = "+ flag);
System.out.println("boolean Value = "+ val);
System.out.println("char Value = "+ ch1);
System.out.println("char Value = "+ ch2);
}
}
Copy
Output of above PrimitiveDemo class as below:
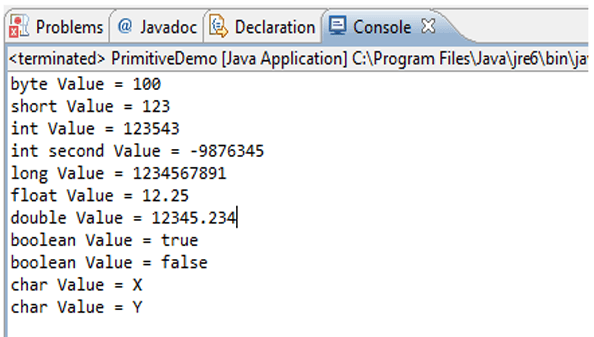
Summary of Data Types
Primitive Type | Size | Minimum Value | Maximum Value | Wrapper Type |
Char | 16-bit | Unicode 0 | Unicode 216-1 | Character |
Byte | 8-bit | -128 | +127 | Byte |
Short | 16-bit | -215 | +215-1 | Short |
Int | 32-bit | -231 | +231-1 | Integer |
Long | 64-bit | -263 | +263-1 | Long |
Float | 32-bit | Approx range 1.4e-045 to 3.4e+038 | Float | |
Double | 64-bit | Approx range 4.9e-324 to 1.8e+308 | Double | |
Boolean | 1-bit | True or false | Boolean |
Summary
- Java has group of variable types called primitive data type which are not object.
- Primitive types are categorized as Integer, Floating point, characters and boolean.
- Primitive types help for better performance of the application.
Concatenate: It is possible to concatenate two or more files and save in a different file.
In java, by using SequenceInputStream class we can concatenate two or more files.
Buffer Files: In java, we can create a buffer to store temporary data that is read from & written to a stream and this process known as i/o buffer operation.
Buffer is sit between programmer and source/destination file.
Buffer can be created by using following classes:
- BufferedInputClass
- BufferedOutputClass
Example: write a program to concatenate two file A & B and concatenated data print on output screen.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | Import java.io.*; Public class FileConcat { Public static void main(String arg[]) throws IOException { FileInputStream I1 = null; FileInputStream I2 = null; I1 = new FileInputStream("A.txt"); //open file A for concatenate I2 = new FileInputStream("B.txt"); //open file B for concatenate // declare sis to store combined file SequenceInputStream sis = null; Sis = new SequenceInputStream(I1, I2); // create buffer for input output BufferedInputStream ibs = new BufferedInputStream(sis); BufferedOutputStream obs = new BufferedOutputStream(System.out); Int c; While ((c = ibs.read()) != -1) // read& write till the end of buffer { Obs.write((char) c); } Ibs.close(); Obs.close(); I1.close(); I2.close(); } } |
Suppose we have a File “A.txt”
Hello students I am aditya. |
And File “B.txt”
Hello |
Output: Hello students I am aditya. Hello
Example: Write a program to copy the content of one file to another file using buffer/BufferReader.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | Import java.io.*; Public class FileCopy1 { Public static void main(String[] args) throws IOException { PrintWriter pw = new PrintWriter("B.txt"); // PrintWriter for write on file B BufferedReader br = new BufferedReader(new FileReader("A.txt")); String line = br.readLine(); // read first line from file A While (line != null) // loop to copy each line of A.txt to C.txt { Pw.println(line); Line = br.readLine(); } Pw.flush(); // closing resources Br.close(); Pw.close(); } } |
Suppose we have a File “A.txt”
Hello students I am aditya. |
Output: File “B”
Hello students I am aditya. |
Note: If there is no file “B” it will be create automatically.
Example: Write a program to concatenate two file A & B and save the concatenated data on a file C.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 | Import java.io.*; Public class FileMerge { Public static void main(String[] args) throws IOException { PrintWriter pw = new PrintWriter("C.txt"); // PrintWriter for write on C.txt
// BufferedReader object for A.txt BufferedReader br = new BufferedReader(new FileReader("A.txt"));
String line = br.readLine(); // read first line from file A While (line != null) // loop to copy each line of A.txt to C.txt { Pw.println(line); Line = br.readLine(); }
Br = new BufferedReader(new FileReader("B.txt"));
Line = br.readLine(); // read first line from file B While (line != null) // loop to copy each line of B.txt to C.txt { Pw.println(line); Line = br.readLine(); }
Pw.flush();
// closing resources Br.close(); Pw.close(); } } |
Suppose we have a File “A.txt” and File “B.txt”
Hello students I am aditya. |
Hello |
Output: File “C”
Hello students I am aditya. Hello |
Note: Ifthere is no file “C” it will be create automatically.
This class is used for reading and writing to random access file. A random access file behaves like a large array of bytes. There is a cursor implied to the array called file pointer, by moving the cursor we do the read write operations. If end-of-file is reached before the desired number of byte has been read than EOFException is thrown. It is a type of IOException.
Constructor
Constructor | Description |
RandomAccessFile(File file, String mode) | Creates a random access file stream to read from, and optionally to write to, the file specified by the File argument. |
RandomAccessFile(String name, String mode) | Creates a random access file stream to read from, and optionally to write to, a file with the specified name. |
Method
Modifier and Type | Method | Method |
Void | Close() | It closes this random access file stream and releases any system resources associated with the stream. |
FileChannel | GetChannel() | It returns the unique FileChannel object associated with this file. |
Int | ReadInt() | It reads a signed 32-bit integer from this file. |
String | ReadUTF() | It reads in a string from this file. |
Void | Seek(long pos) | It sets the file-pointer offset, measured from the beginning of this file, at which the next read or write occurs. |
Void | WriteDouble(double v) | It converts the double argument to a long using the doubleToLongBits method in class Double, and then writes that long value to the file as an eight-byte quantity, high byte first. |
Void | WriteFloat(float v) | It converts the float argument to an int using the floatToIntBits method in class Float, and then writes that int value to the file as a four-byte quantity, high byte first. |
Void | Write(int b) | It writes the specified byte to this file. |
Int | Read() | It reads a byte of data from this file. |
Long | Length() | It returns the length of this file. |
Void | Seek(long pos) | It sets the file-pointer offset, measured from the beginning of this file, at which the next read or write occurs. |
Example
- Import java.io.IOException;
- Import java.io.RandomAccessFile;
- Public class RandomAccessFileExample {
- Static final String FILEPATH ="myFile.TXT";
- Public static void main(String[] args) {
- Try {
- System.out.println(new String(readFromFile(FILEPATH, 0, 18)));
- WriteToFile(FILEPATH, "I love my country and my people", 31);
- } catch (IOException e) {
- e.printStackTrace();
- }
- }
- Private static byte[] readFromFile(String filePath, int position, int size)
- Throws IOException {
- RandomAccessFile file = new RandomAccessFile(filePath, "r");
- File.seek(position);
- Byte[] bytes = new byte[size];
- File.read(bytes);
- File.close();
- Return bytes;
- }
- Private static void writeToFile(String filePath, String data, int position)
- Throws IOException {
- RandomAccessFile file = new RandomAccessFile(filePath, "rw");
- File.seek(position);
- File.write(data.getBytes());
- File.close();
- }
- }
The myFile.TXT contains text "This class is used for reading and writing to random access file."
After running the program it will contains
This class is used for reading I love my country and my peoplele.
A design patterns are well-proved solution for solving the specific problem/task.
Now, a question will be arising in your mind what kind of specific problem? Let me explain by taking an example.
Problem Given:
Suppose you want to create a class for which only a single instance (or object) should be created and that single object can be used by all other classes.
Solution:
Singleton design pattern is the best solution of above specific problem. So, every design pattern has some specification or set of rules for solving the problems. What are those specifications, you will see later in the types of design patterns.
But remember one-thing, design patterns are programming language independent strategies for solving the common object-oriented design problems. That means, a design pattern represents an idea, not a particular implementation.
By using the design patterns you can make your code more flexible, reusable and maintainable. It is the most important part because java internally follows design patterns.
To become a professional software developer, you must know at least some popular solutions (i.e. design patterns) to the coding problems.
Advantage of design pattern:
- They are reusable in multiple projects.
- They provide the solutions that help to define the system architecture.
- They capture the software engineering experiences.
- They provide transparency to the design of an application.
- They are well-proved and testified solutions since they have been built upon the knowledge and experience of expert software developers.
- Design patterns don?t guarantee an absolute solution to a problem. They provide clarity to the system architecture and the possibility of building a better system.
When should we use the design patterns?
We must use the design patterns during the analysis and requirement phase of SDLC(Software Development Life Cycle).
Design patterns ease the analysis and requirement phase of SDLC by providing information based on prior hands-on experiences.
Categorization of design patterns:
Basically, design patterns are categorized into two parts:
- Core Java (or JSE) Design Patterns.
- JEE Design Patterns.
Core Java Design Patterns
In core java, there are mainly three types of design patterns, which are further divided into their sub-parts:
1. Creational Design Pattern
- Factory Pattern
- Abstract Factory Pattern
- Singleton Pattern
- Prototype Pattern
- Builder Pattern.
2. Structural Design Pattern
- Adapter Pattern
- Bridge Pattern
- Composite Pattern
- Decorator Pattern
- Facade Pattern
- Flyweight Pattern
- Proxy Pattern
3. Behavioural Design Pattern
- Chain Of Responsibility Pattern
- Command Pattern
- Interpreter Pattern
- Iterator Pattern
- Mediator Pattern
- Memento Pattern
- Observer Pattern
- State Pattern
- Strategy Pattern
- Template Pattern
- Visitor Pattern
An Adapter Pattern says that just "converts the interface of a class into another interface that a client wants".
In other words, to provide the interface according to client requirement while using the services of a class with a different interface.
The Adapter Pattern is also known as Wrapper.
Advantage of Adapter Pattern
- It allows two or more previously incompatible objects to interact.
- It allows reusability of existing functionality.
Usage of Adapter pattern:
It is used:
- When an object needs to utilize an existing class with an incompatible interface.
- When you want to create a reusable class that cooperates with classes which don't have compatible interfaces.
- When you want to create a reusable class that cooperates with classes which don't have compatible interfaces.
Example of Adapter Pattern
Let's understand the example of adapter design pattern by the above UML diagram.
UML for Adapter Pattern:
There are the following specifications for the adapter pattern:
- Target Interface: This is the desired interface class which will be used by the clients.
- Adapter class: This class is a wrapper class which implements the desired target interface and modifies the specific request available from the Adaptee class.
- Adaptee class: This is the class which is used by the Adapter class to reuse the existing functionality and modify them for desired use.
- Client: This class will interact with the Adapter class.
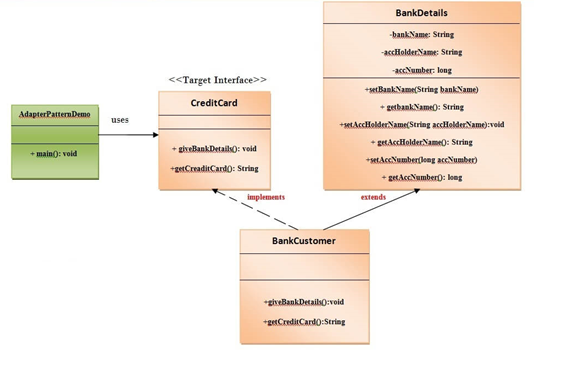
Implementation of above UML:
Step 1
Create a CreditCard interface (Target interface).
- Public interface CreditCard {
- Public void giveBankDetails();
- Public String getCreditCard();
- }// End of the CreditCard interface.
Step 2
Create a BankDetails class (Adaptee class).
File: BankDetails.java
- // This is the adapter class.
- Public class BankDetails{
- Private String bankName;
- Private String accHolderName;
- Private long accNumber;
- Public String getBankName() {
- Return bankName;
- }
- Public void setBankName(String bankName) {
- This.bankName = bankName;
- }
- Public String getAccHolderName() {
- Return accHolderName;
- }
- Public void setAccHolderName(String accHolderName) {
- This.accHolderName = accHolderName;
- }
- Public long getAccNumber() {
- Return accNumber;
- }
- Public void setAccNumber(long accNumber) {
- This.accNumber = accNumber;
- }
- }// End of the BankDetails class.
Step 3
Create a BankCustomer class (Adapter class).
File: BankCustomer.java
- // This is the adapter class
- Import java.io.BufferedReader;
- Import java.io.InputStreamReader;
- Public class BankCustomer extends BankDetails implements CreditCard {
- Public void giveBankDetails(){
- Try{
- BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
- System.out.print("Enter the account holder name :");
- String customername=br.readLine();
- System.out.print("\n");
- System.out.print("Enter the account number:");
- Long accno=Long.parseLong(br.readLine());
- System.out.print("\n");
- System.out.print("Enter the bank name :");
- String bankname=br.readLine();
- SetAccHolderName(customername);
- SetAccNumber(accno);
- SetBankName(bankname);
- }catch(Exception e){
- e.printStackTrace();
- }
- }
- @Override
- Public String getCreditCard() {
- Long accno=getAccNumber();
- String accholdername=getAccHolderName();
- String bname=getBankName();
- Return ("The Account number "+accno+" of "+accholdername+" in "+bname+ "
- Bank is valid and authenticated for issuing the credit card. ");
- }
- }//End of the BankCustomer class.
Step 4
Create a AdapterPatternDemo class (client class).
File: AdapterPatternDemo.java
- //This is the client class.
- Public class AdapterPatternDemo {
- Public static void main(String args[]){
- CreditCard targetInterface=new BankCustomer();
- TargetInterface.giveBankDetails();
- System.out.print(targetInterface.getCreditCard());
- }
- }//End of the BankCustomer class.
Output
- Enter the account holder name :Sonoo Jaiswal
- Enter the account number:10001
- Enter the bank name :State Bank of India
- The Account number 10001 of Sonoo Jaiswal in State Bank of India bank is valid
- And authenticated for issuing the credit card.
Singleton Pattern says that just" define a class that has only one instance and provides a global point of access to it".
In other words, a class must ensure that only single instance should be created and single object can be used by all other classes.
There are two forms of singleton design pattern
- Early Instantiation: creation of instance at load time.
- Lazy Instantiation: creation of instance when required.
Advantage of Singleton design pattern
- Saves memory because object is not created at each request. Only single instance is reused again and again.
Usage of Singleton design pattern
- Singleton pattern is mostly used in multi-threaded and database applications. It is used in logging, caching, thread pools, configuration settings etc.
Uml of Singleton design pattern
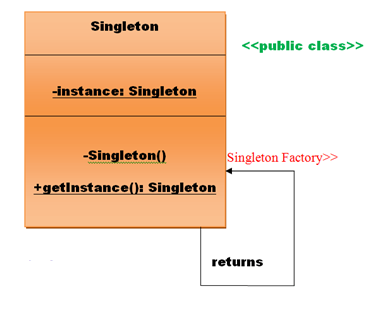
How to create Singleton design pattern?
To create the singleton class, we need to have static member of class, private constructor and static factory method.
- Static member: It gets memory only once because of static, itcontains the instance of the Singleton class.
- Private constructor: It will prevent to instantiate the Singleton class from outside the class.
- Static factory method: This provides the global point of access to the Singleton object and returns the instance to the caller.
Understanding early Instantiation of Singleton Pattern
In such case, we create the instance of the class at the time of declaring the static data member, so instance of the class is created at the time of classloading.
Let's see the example of singleton design pattern using early instantiation.
File: A.java
- Class A{
- Private static A obj=new A();//Early, instance will be created at load time
- Private A(){}
- Public static A getA(){
- Return obj;
- }
- Public void doSomething(){
- //write your code
- }
- }
Understanding lazy Instantiation of Singleton Pattern
In such case, we create the instance of the class in synchronized method or synchronized block, so instance of the class is created when required.
Let's see the simple example of singleton design pattern using lazy instantiation.
File: A.java
- Class A{
- Private static A obj;
- Private A(){}
- Public static A getA(){
- If (obj == null){
- Synchronized(Singleton.class){
- If (obj == null){
- Obj = new Singleton();//instance will be created at request time
- }
- }
- }
- Return obj;
- }
- Public void doSomething(){
- //write your code
- }
- }
Significance of Classloader in Singleton Pattern
If singleton class is loaded by two classloaders, two instance of singleton class will be created, one for each classloader.
Significance of Serialization in Singleton Pattern
If singleton class is Serializable, you can serialize the singleton instance. Once it is serialized, you can deserialize it but it will not return the singleton object.
To resolve this issue, you need to override the readResolve() method that enforces the singleton. It is called just after the object is deserialized. It returns the singleton object.
- Public class A implements Serializable {
- //your code of singleton
- Protected Object readResolve() {
- Return getA();
- }
- }
Understanding Real Example of Singleton Pattern
- We are going to create a JDBCSingleton class. This JDBCSingleton class contains its constructor as private and a private static instance jdbc of itself.
- JDBCSingleton class provides a static method to get its static instance to the outside world. Now, JDBCSingletonDemo class will use JDBCSingleton class to get the JDBCSingleton object.
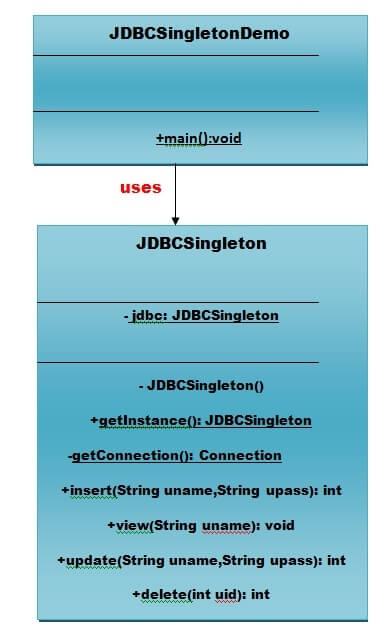
Assumption: you have created a table userdata that has three fields uid, uname and upassword in mysql database. Database name is ashwinirajput, username is root, password is ashwini.
File: JDBCSingleton.java
- Import java.io.BufferedReader;
- Import java.io.IOException;
- Import java.io.InputStreamReader;
- Import java.sql.Connection;
- Import java.sql.DriverManager;
- Import java.sql.PreparedStatement;
- Import java.sql.ResultSet;
- Import java.sql.SQLException;
- Class JDBCSingleton {
- //Step 1
- // create a JDBCSingleton class.
- //static member holds only one instance of the JDBCSingleton class.
- Private static JDBCSingleton jdbc;
- //JDBCSingleton prevents the instantiation from any other class.
- Private JDBCSingleton() { }
- //Now we are providing gloabal point of access.
- Public static JDBCSingleton getInstance() {
- If (jdbc==null)
- {
- Jdbc=new JDBCSingleton();
- }
- Return jdbc;
- }
- // to get the connection from methods like insert, view etc.
- Private static Connection getConnection()throws ClassNotFoundException, SQLException
- {
- Connection con=null;
- Class.forName("com.mysql.jdbc.Driver");
- Con= DriverManager.getConnection("jdbc:mysql://localhost:3306/ashwanirajput", "root", "ashwani");
- Return con;
- }
- //to insert the record into the database
- Public int insert(String name, String pass) throws SQLException
- {
- Connection c=null;
- PreparedStatement ps=null;
- Int recordCounter=0;
- Try {
- c=this.getConnection();
- Ps=c.prepareStatement("insert into userdata(uname,upassword)values(?,?)");
- Ps.setString(1, name);
- Ps.setString(2, pass);
- RecordCounter=ps.executeUpdate();
- } catch (Exception e) { e.printStackTrace(); } finally{
- If (ps!=null){
- Ps.close();
- }if(c!=null){
- c.close();
- }
- }
- Return recordCounter;
- }
- //to view the data from the database
- Public void view(String name) throws SQLException
- {
- Connection con = null;
- PreparedStatement ps = null;
- ResultSet rs = null;
- Try {
- Con=this.getConnection();
- Ps=con.prepareStatement("select * from userdata where uname=?");
- Ps.setString(1, name);
- Rs=ps.executeQuery();
- While (rs.next()) {
- System.out.println("Name= "+rs.getString(2)+"\t"+"Paasword= "+rs.getString(3));
- }
- } catch (Exception e) { System.out.println(e);}
- Finally{
- If(rs!=null){
- Rs.close();
- }if (ps!=null){
- Ps.close();
- }if(con!=null){
- Con.close();
- }
- }
- }
- // to update the password for the given username
- Public int update(String name, String password) throws SQLException {
- Connection c=null;
- PreparedStatement ps=null;
- Int recordCounter=0;
- Try {
- c=this.getConnection();
- Ps=c.prepareStatement(" update userdata set upassword=? where uname='"+name+"' ");
- Ps.setString(1, password);
- RecordCounter=ps.executeUpdate();
- } catch (Exception e) { e.printStackTrace(); } finally{
- If (ps!=null){
- Ps.close();
- }if(c!=null){
- c.close();
- }
- }
- Return recordCounter;
- }
- // to delete the data from the database
- Public int delete(int userid) throws SQLException{
- Connection c=null;
- PreparedStatement ps=null;
- Int recordCounter=0;
- Try {
- c=this.getConnection();
- Ps=c.prepareStatement(" delete from userdata where uid='"+userid+"' ");
- RecordCounter=ps.executeUpdate();
- } catch (Exception e) { e.printStackTrace(); }
- Finally{
- If (ps!=null){
- Ps.close();
- }if(c!=null){
- c.close();
- }
- }
- Return recordCounter;
- }
- }// End of JDBCSingleton class
File: JDBCSingletonDemo.java
- Import java.io.BufferedReader;
- Import java.io.IOException;
- Import java.io.InputStreamReader;
- Import java.sql.Connection;
- Import java.sql.DriverManager;
- Import java.sql.PreparedStatement;
- Import java.sql.ResultSet;
- Import java.sql.SQLException;
- Class JDBCSingletonDemo{
- Static int count=1;
- Static int choice;
- Public static void main(String[] args) throws IOException {
- JDBCSingleton jdbc= JDBCSingleton.getInstance();
- BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
- Do{
- System.out.println("DATABASE OPERATIONS");
- System.out.println(" --------------------- ");
- System.out.println(" 1. Insertion ");
- System.out.println(" 2. View ");
- System.out.println(" 3. Delete ");
- System.out.println(" 4. Update ");
- System.out.println(" 5. Exit ");
- System.out.print("\n");
- System.out.print("Please enter the choice what you want to perform in the database: ");
- Choice=Integer.parseInt(br.readLine());
- Switch(choice) {
- Case 1:{
- System.out.print("Enter the username you want to insert data into the database: ");
- String username=br.readLine();
- System.out.print("Enter the password you want to insert data into the database: ");
- String password=br.readLine();
- Try {
- Int i= jdbc.insert(username, password);
- If (i>0) {
- System.out.println((count++) + " Data has been inserted successfully");
- }else{
- System.out.println("Data has not been inserted ");
- }
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Press Enter key to continue...");
- System.in.read();
- }//End of case 1
- Break;
- Case 2:{
- System.out.print("Enter the username : ");
- String username=br.readLine();
- Try {
- Jdbc.view(username);
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Press Enter key to continue...");
- System.in.read();
- }//End of case 2
- Break;
- Case 3:{
- System.out.print("Enter the userid, you want to delete: ");
- Int userid=Integer.parseInt(br.readLine());
- Try {
- Int i= jdbc.delete(userid);
- If (i>0) {
- System.out.println((count++) + " Data has been deleted successfully");
- }else{
- System.out.println("Data has not been deleted");
- }
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Press Enter key to continue...");
- System.in.read();
- }//End of case 3
- Break;
- Case 4:{
- System.out.print("Enter the username, you want to update: ");
- String username=br.readLine();
- System.out.print("Enter the new password ");
- String password=br.readLine();
- Try {
- Int i= jdbc.update(username, password);
- If (i>0) {
- System.out.println((count++) + " Data has been updated successfully");
- }
- } catch (Exception e) {
- System.out.println(e);
- }
- System.out.println("Press Enter key to continue...");
- System.in.read();
- }// end of case 4
- Break;
- Default:
- Return;
- }
- } while (choice!=4);
- }
- }
Output
According to GoF, Iterator Pattern is used "to access the elements of an aggregate object sequentially without exposing its underlying implementation".
The Iterator pattern is also known as Cursor.
In collection framework, we are now using Iterator that is preferred over Enumeration.
java.util.Iterator interface uses Iterator Design Pattern.
Advantage of Iterator Pattern
- It supports variations in the traversal of a collection.
- It simplifies the interface to the collection.
Usage of Iterator Pattern:
It is used:
- When you want to access a collection of objects without exposing its internal representation.
- When there are multiple traversals of objects need to be supported in the collection.
Example of Iterator Pattern
Let's understand the example of iterator pattern pattern by the above UML diagram.
UML for Iterator Pattern:
Implementation of above UML
Step 1
Create a Iterartor interface.
- Public interface Iterator {
- Public boolean hasNext();
- Public Object next();
- }
Step 2
Create a Container interface.
- Public interface Container {
- Public Iterator getIterator();
- }// End of the Iterator interface.
Step 3
Create a CollectionofNames class that will implement Container interface.
File: CollectionofNames.java
- Public class CollectionofNames implements Container {
- Public String name[]={"Ashwani Rajput", "Soono Jaiswal","Rishi Kumar","Rahul Mehta","Hemant Mishra"};
- @Override
- Public Iterator getIterator() {
- Return new CollectionofNamesIterate() ;
- }
- Private class CollectionofNamesIterate implements Iterator{
- Int i;
- @Override
- Public boolean hasNext() {
- If (i<name.length){
- Return true;
- }
- Return false;
- }
- @Override
- Public Object next() {
- If(this.hasNext()){
- Return name[i++];
- }
- Return null;
- }
- }
- }
- }
Step 4
Create a IteratorPatternDemo class.
File: IteratorPatternDemo.java
- Public class IteratorPatternDemo {
- Public static void main(String[] args) {
- CollectionofNames cmpnyRepository = new CollectionofNames();
- For(Iterator iter = cmpnyRepository.getIterator(); iter.hasNext();){
- String name = (String)iter.next();
- System.out.println("Name : " + name);
- }
- }
- }
Output
- Name : Ashwani Rajput
- Name : Soono Jaiswal
- Name : Rishi Kumar
- Name : Rahul Mehta
- Name : Hemant Mishra
References:
1. Object-Oriented Programming and Java by Danny Poo (Author), Derek Kiong (Author), Swarnalatha Ashok (Author)Springer; 2nd ed. 2008 edition (12 October 2007), ISBN-10: 1846289629, ISBN-13: 978-1846289620,2007
2. Java The complete reference, 9th edition, Herbert Schildt, McGraw Hill Education (India) Pvt. Ltd.
3. Object-Oriented Design Using Java, Dale Skrien, McGraw-Hill Publishing, 2008, ISBN - 0077423097, 9780077423094. 4. UML for Java Programmers by Robert C. Martin, Prentice Hall, ISBN 0131428489,2003.