UNIT 2
Introduction to Algorithms
An algorithm is the finite set of English statements which are designed to accomplish the specific task. Study of any algorithm is based on the following four criteria
1. Design of algorithm : This is the first step in the creation of an algorithm. Design of algorithm includes the problem statement which tell user about the area for which algorithm is required. After problem statement next important thing required is the information about available and required resources. The last thing required in design of algorithm phase is information about the expected output.
2. Validation of algorithm : Once an algorithm is designed , it is necessary to validate it for several possible input and make sure that algorithm is providing correct output for every input. This process is known as algorithm validation. The purpose of the validation is to assure us that this algorithm will work correctly independently of the issues concerning the programming language it will eventually be written in.
3. Analysis of algorithm : Analysis of algorithms is also called as performance analysis. This phase refers to the task of determining how much computing time and storage an algorithm requires. The efficiency is measured in best case, worst case and average case analysis.
4. Testing of algorithm : This phase is about to testing of a program which coded as per the algorithm. Testing of such program consists of two phases:
Debugging : It is the process of executing programs on sample data sets to determine whether faulty results occur or not and if occur, to correct them.
Profiling : Profiling or performance measurement is the process of executing a correct program on data sets and measuring the time and space it takes to compute the result
Characteristics of an algorithm
Input: Algorithm must accept zero or more inputs.
Output: Algorithm must provide at least one quantity.
Definiteness: Algorithm must consist of clear and unambiguous instruction.
Finiteness: Algorithm must contain finite set of instruction and algorithm will terminates after a finite number of steps.
Effectiveness: Every instruction in algorithm must be very basic and effective.
Uses of algorithm
Algorithm provide independent layout of the program.
It is easy to develop the program in any desired language with help of layout.
Algorithm representation is very easy to understand.
To design algorithm there is no need of expertise in programming language.
Complexities and Flowchart
There are three ways to represent an algorithm. Consider the following algorithm of addition of two numbers
Step 1 : Start
Step 2 : Read a number, say x and y
Step 3 : Add x and y
Step 4 : Display Addition
Step 5 : Stop
1. Flowcharts: A flow chart is a diagrammatic / pictorial representation of an algorithm. It is the simplest way of representation of algorithm. Initially an algorithm is represented in the form of flowchart and then flowchart is given to programmer to express it in some programming language. Logical error detection is very easy in flowchart as it shows the flow of operations in diagrammatic form. Once flowchart is ready it is very easy to write a program in terms of statements of a programming language. Following are the symbols used in designing the flowcharts.
2. Pseudo code : Pseudo code is the combination of English statements with programming methodology. In pseudo code, there is no restriction of following the syntax of the programming language. Pseudo codes cannot be compiled. It is just a previous step of developing a code for given algorithm.
3. Program : In this way of representation, complete algorithm is represented using some programming language by following the complete syntax of programming language.
Sr. No. | Name of Symbol | Symbol | Meaning / Purpose |
1. | Terminal |
![]() | To indicate START / STOP. Usually it is the first symbol and last symbol used in program logic. |
2. | Input / Output Statement | ![]() | To indicate input / output of Data |
3. | Processing Statement | ![]() | To indicate the processing of instructions. |
4. |
Decision Box | ![]() | To indicate decision making and a branch to one or more alternatives. |
5. | Flow Lines | ![]() | To indicate the direction of flow of information. |
6. | Connector |
![]()
| It is used when flowchart becomes long and need to be continued. Shows the continuity of the algorithm on the next page. |
Computer Programming is defined as the step by step process of designing and developing various sets of computer programs to accomplish a specific computing outcome. The process comprises several tasks like analysis, coding, algorithm generation, checking accuracy and resource consumption of algorithms, etc.
The purpose of computer programming is to find a sequence of instructions that solve a specific problem on a computer.
These basic elements include −
- Programming Environment
- Basic Syntax
- Data Types
- Variables
- Keywords
- Basic Operators
- Decision Making
- Loops
- Numbers
- Characters
- Arrays
- Strings
- Functions
- File I/O
Procedure Oriented Programming
- Procedure Oriented Programming divides program into small procedures.
- A procedure is a set of instructions to perform specific independent task.
- A problem is viewed as a sequence of tasks and a separate procedure is written to perform that task.
- So the main emphasis is on procedure and data is given no/ little importance.
- Mostly data is global data which is shared by functions/procedures.
It employs top down approach.
Fig.: Structure of procedure oriented programming
- Fig. Shows how global data is accessed by all functions in a program while local data can be accessed by only that specific function.
Object Oriented Programming
- Program is divided into number of objects.
- Objects are considered as real life entities that contain data and functions.
- Data is given more importance than functions.
- Data is not accessible to functions of other classes and hence data security is ensured.
- It employs bottom up approach.
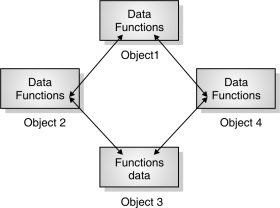
Fig. : Structure of Object Oriented Programming
- Fig. Shows how objects can communicate with other objects with the help of functions. Here data is not accessible to external functions which ensure data security.
Modular Programming
- Modular programming divides program into distinct independent units called as modules.
- Each module is a separate software component that is programmed and tested independently.
- Modular programming leads to effective development and maintenance of large programs.
Generic Programming Technique
- Usually codes or programs are tied to specific types. If we are writing program for stack it is written for a specific data type like stack of integer values.
- If we want to create stack of characters, then we need to rewrite code taking ‘char’ as a data type.
- This rewriting same code for a specific data type can be avoided by generic programming technique.
- Generic programs create software components that can be used for any data type.
- Thus we will write generic program for stack which will work on any data type.
- In C++, class and templates are generic programming components.
C is a procedural programming language. It was initially developed by Dennis Ritchie in the year 1972. It was mainly developed as a system programming language to write an operating system. The main features of C language include low-level access to memory, a simple set of keywords, and clean style, these features make C language suitable for system programming like an operating system or compiler development.
Many later languages have borrowed syntax/features directly or indirectly from C language. Like syntax of Java, PHP, JavaScript, and many other languages are mainly based on C language. C++ is nearly a superset of C language (There are few programs that may compile in C, but not in C++).
Beginning with C programming:
- Structure of a C program
After the above discussion, we can formally assess the structure of a C program. By structure, it is meant that any program can be written in this structure only. Writing a C program in any other structure will hence lead to a Compilation Error.
The structure of a C program is as follows:
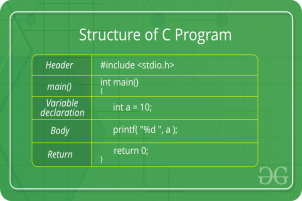
The components of the above structure are:
- Header Files Inclusion: The first and foremost component is the inclusion of the Header files in a C program.
A header file is a file with extension .h which contains C function declarations and macro definitions to be shared between several source files.
Some of C Header files:
- Stddef.h – Defines several useful types and macros.
- Stdint.h – Defines exact width integer types.
- Stdio.h – Defines core input and output functions
- Stdlib.h – Defines numeric conversion functions, pseudo-random network generator, memory allocation
- String.h – Defines string handling functions
- Math.h – Defines common mathematical functions
Syntax to include a header file in C:
#include
2. Main Method Declaration: The next part of a C program is to declare the main() function. The syntax to declare the main function is:
Syntax to Declare main method:
Int main()
{}
3. Variable Declaration: The next part of any C program is the variable declaration. It refers to the variables that are to be used in the function. Please note that in the C program, no variable can be used without being declared. Also in a C program, the variables are to be declared before any operation in the function.
Example:
Int main()
{
Int a;
.
.
4. Body: Body of a function in C program, refers to the operations that are performed in the functions. It can be anything like manipulations, searching, sorting, printing, etc.
Example:
Int main()
{
Int a;
Printf("%d", a);
.
.
5. Return Statement: The last part in any C program is the return statement. The return statement refers to the returning of the values from a function. This return statement and return value depend upon the return type of the function. For example, if the return type is void, then there will be no return statement. In any other case, there will be a return statement and the return value will be of the type of the specified return type.
Example:
Int main()
{
Int a;
Printf("%d", a);
Return 0;
}
2. Writing first program:
Following is first program in C
#include <stdio.h> Int main(void) { Printf("GeeksQuiz"); Return 0; } |
Let us analyze the program line by line.
Line 1: [ #include <stdio.h> ] In a C program, all lines that start with # are processed by preprocessor which is a program invoked by the compiler. In a very basic term, preprocessor takes a C program and produces another C program. The produced program has no lines starting with #, all such lines are processed by the preprocessor. In the above example, preprocessor copies the preprocessed code of stdio.h to our file. The .h files are called header files in C. These header files generally contain declaration of functions. We need stdio.h for the function printf() used in the program.
Line 2 [ int main(void) ] There must to be starting point from where execution of compiled C program begins. In C, the execution typically begins with first line of main(). The void written in brackets indicates that the main doesn’t take any parameter main() can be written to take parameters also. We will be covering that in future posts.
The int written before main indicates return type of main(). The value returned by main indicates status of program termination.
Line 3 and 6: [ { and } ] In C language, a pair of curly brackets define a scope and mainly used in functions and control statements like if, else, loops. All functions must start and end with curly brackets.
Line 4 [ printf(“GeeksQuiz”); ] printf() is a standard library function to print something on standard output. The semicolon at the end of printf indicates line termination. In C, semicolon is always used to indicate end of statement.
Line 5 [ return 0; ] The return statement returns the value from main(). The returned value may be used by operating system to know termination status of your program. The value 0 typically means successful termination.
How to excecute the above program:
Inorder to execute the above program, we need to have a compiler to compile and run our programs.
Paradigm is a method used to solve a problem or to do some task. Though there are many programming languages all of them need to follow some strategy for implementation and this methodology is paradigm.
Programming paradigm is an approach used to solve problem using some programming language or a method to solve problem using tools and techniques.
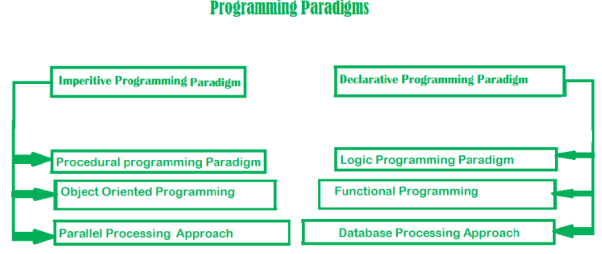
Figure 1. Programming Paradigm
Programming paradigm is classified into
Imperative Paradigm and Declarative Paradigm
Imperative programming paradigm:
- Imperative programming is one of the oldest programming paradigms. It works by changing the program state through assignment statements. It performs step by step task by changing state.
- The focus is how to achieve the goal It consists of several statements and after execution all the result is stored.
Advantage:
- Easy to implement
- It contains loops, variables etc.
Disadvantage:
- Complex problem cannot be solved
- Less efficient and less productive
- Parallel programming is not possible
Example:
// average of five number in C
Int marks [5] = { 12, 32, 45, 13, 19 } int sum = 0;
Float average = 0.0;
For (int i = 0; i < 5; i++) {
Sum = sum + marks[i];
}
Average = sum / 5;
Imperative programming is divided into three broad categories:
- Procedural
- OOP
- Parallel processing.
These paradigms are as follows:
Procedural programming paradigm –
This paradigm emphasizes on procedure in terms of under lying machine model. There is no difference in between procedural and imperative approach. It has the ability to reuse the code.
#include <iostream>
Using namespace std;
Int main ()
{
Int i, fact = 1, num;
Cout << "Enter any Number: ";
Cin >> number.
For (i = 1; i <= num; i++) {
Fact = fact * i;
}
Cout << "Factorial of " << num << " is: " << fact << endl;
Return 0;
}
Object oriented programming –
The program is written as a collection of classes and object which are meant for communication. The smallest and basic entity is the object. All kind of computation is performed on objects only.
Here emphasis is on data rather procedure.
Advantages:
- Data security
- Inheritance
- Code reusability
- Flexible and abstraction is also present
Parallel processing approach –
Parallel processing is the processing of program instructions by dividing them among multiple processors. A parallel- processing system consists of numbers of processor with the objective of running a program in less time by dividing them.
This approach can be like divide and conquer.
Examples are NESL (one of the oldest one) and C/C++ also supports because of some library function.
Declarative programming paradigm:
Declarative is divided as Logic, Functional, Database.
- The declarative programming is a style of building programs that expresses logic of computation but does not provide the control flow.
- Here it considers on theories of some logic. The focus is on what needs to be done rather how it should be done basically emphasize on what code is doing.
- It shows the result rather than showing how it is produced. This is the only difference between imperative (how to do) and declarative (what to do) programming paradigms.
Logic programming paradigms –
Logic programming paradigms can be termed as abstract model of computation. It would solve logical problems like puzzles, series etc.
In normal programming languages, such concept of knowledge base is not available but the concept of artificial intelligence, machine learning we have some models like Perception model mechanism.
In logical programming the main emphasize is on knowledge base and the problem. The execution of the program is very much like proof of mathematical statement,
For example,
Sum of two number in prolog:
Predicates
Sumoftwonumber(integer, integer)
Clauses
Sum(0, 0).
Sum(n, r):-
n1=n-1,
Sum(n1, r1),
r=r1+n
Functional programming paradigms –
In functional programming the paradigms has its origin from mathematics and is language independent. The key principal of this paradigms is the execution of series of mathematical functions.
The central model for the abstraction is the function which is meant for some specific computation and not the data structure. The data is loosely coupled to functions. The function hide their implementation. Function can be replaced with their values without changing the meaning of the program. Some of the languages like perl, javascript are mostly used in this paradigm.
Examples of Functional programming paradigm:
JavaScript: developed by Brendan Eich
Haskwell : developed by Lennart Augustsson, Dave Barton
Scala : developed by Martin Odersky
Erlang : developed by Joe Armstrong, Robert Virding
Lisp : developed by John Mccarthy
ML : developed by Robin Milner
Clojure : developed by Rich Hickey
Database/Data driven programming approach –
This programming methodology is based on data and its movement. Program statements are defined by data rather than hard coding a series of steps.
A database program is the heart of a business information system and provides file creation, data entry, update, query and reporting functions. There are several programming languages that are developed mostly for database application.
For example, SQL. It is applied to streams of structured data, for filtering, transforming, aggregating (such as computing statistics), or calling other programs.
CREATE DATABASE database Addresses.
CREATE TABLE Addr (
PersonID int,
LastName varchar(200),
FirstName varchar(200),
Address varchar(200),
City varchar(200),
State varchar(200)
);
- Program is divided into number of objects.
- Objects are considered as real life entities that contain data and functions.
- Data is given more importance than functions.
- Data is not accessible to functions of other classes and hence data security is ensured.
- It employs bottom up approach.
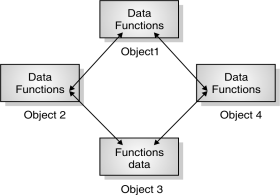
Fig. Structure of Object Oriented Programming
Fig shows how objects can communicate with other objects with the help of functions. Here data is not accessible to external functions which ensure data security.
Advantages of OOP
- Real world complex problems can be modelled very well using OOP as OOP consists of object that represents real life entity.
- Using Inheritance class can reuse code of another class. This leads to faster software development and saves coding efforts and time.
- OOP ensures data security by making members of class private. Private members are accessible to same class hence data is not invaded by external functions.
- We can perform variety of tasks with the help of single function or operator by function or operator overloading.
- OOP provides better software development productivity than traditional programming languages due to data hiding, inheritance, polymorphism and templates.
Sr. No. | Procedure Oriented | Object Oriented |
1. | Employs top down approach. | Employs bottom up approach. |
2. | Program is divided into functions/ procedures. | Program is divided into objects. |
3. | Importance is given to functions/procedures. | Importance is given to data. |
4. | Data can be accessed freely from any function. | Data is hidden and is not accessible to external functions. |
5. | Data is not secured as functions can access data easily. | Data is secured due to data Hiding concept. |
6. | Most of the data is global data. | Data is given various visibility levels with the help of access specifiers private, public and protected. |
7. | C, FORTRAN, PASCAL are procedure oriented languages. | JAVA, C++, Simula are object oriented languages. |
C++ is a middle-level programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. C++ runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX. This C++ tutorial adopts a simple and practical approach to describe the concepts of C++ for beginners to advanced software engineers.
Why to Learn C++
C++ is a MUST for students and working professionals to become a great Software Engineer. I will list down some of the key advantages of learning C++:
- C++ is very close to hardware, so you get a chance to work at a low level which gives you lot of control in terms of memory management, better performance and finally a robust software development.
- C++ programming gives you a clear understanding about Object Oriented Programming. You will understand low level implementation of polymorphism when you will implement virtual tables and virtual table pointers, or dynamic type identification.
- C++ is one of the every green programming languages and loved by millions of software developers. If you are a great C++ programmer then you will never sit without work and more importantly you will get highly paid for your work.
- C++ is the most widely used programming languages in application and system programming. So you can choose your area of interest of software development.
- C++ really teaches you the difference between compiler, linker and loader, different data types, storage classes, variable types their scopes etc.
There are 1000s of good reasons to learn C++ Programming. But one thing for sure, to learn any programming language, not only C++, you just need to code, and code and finally code until you become expert.
Hello World using C++
Just to give you a little excitement about C++ programming, I'm going to give you a small conventional C++ Hello World program, You can try it using Demo link
C++ is a super set of C programming with additional implementation of object-oriented concepts.
#include <iostream>
Using namespace std;
// main() is where program execution begins.
Int main() {
Cout << "Hello World"; // prints Hello World
Return 0;
}
There are many C++ compilers available which you can use to compile and run above mentioned program:
- Apple C++. Xcode
- Bloodshed Dev-C++
- Clang C++
- Cygwin (GNU C++)
- Mentor Graphics
- MINGW - "Minimalist GNU for Windows"
- GNU CC source
- IBM C++
- Intel C++
- Microsoft Visual C++
- Oracle C++
- HP C++
It is really impossible to give a complete list of all the available compilers. The C++ world is just too large and too much new is happening.
Applications of C++ Programming
As mentioned before, C++ is one of the most widely used programming languages. It has it's presence in almost every area of software development. I'm going to list few of them here:
- Application Software Development - C++ programming has been used in developing almost all the major Operating Systems like Windows, Mac OSX and Linux. Apart from the operating systems, the core part of many browsers like Mozilla Firefox and Chrome have been written using C++. C++ also has been used in developing the most popular database system called MySQL.
- Programming Languages Development - C++ has been used extensively in developing new programming languages like C#, Java, JavaScript, Perl, UNIX’s C Shell, PHP and Python, and Verilog etc.
- Computation Programming - C++ is the best friends of scientists because of fast speed and computational efficiencies.
- Games Development - C++ is extremely fast which allows programmers to do procedural programming for CPU intensive functions and provides greater control over hardware, because of which it has been widely used in development of gaming engines.
- Embedded System - C++ is being heavily used in developing Medical and Engineering Applications like softwares for MRI machines, high-end CAD/CAM systems etc.
This list goes on, there are various areas where software developers are happily using C++ to provide great softwares. I highly recommend you to learn C++ and contribute great softwares to the community.
Audience
This C++ tutorial has been prepared for the beginners to help them understand the basic to advanced concepts related to C++.
Prerequisites
Before you start practicing with various types of examples given in this C++ tutorial,we are making an assumption that you are already aware of the basics of computer program and computer programming language.
The character set are set of words, digits, symbols and operators that are valid in C.
There are four types of Character Set:-
Character Set | ||
1. | Letters | Uppercase A-Z |
2. | Digits | All digits 0-9 |
3. | Special Characters | All Symbols: , . : ; ? ' " ! | \ / ~ _$ % # & ^ * - + < > ( ) { } [ ] |
4. | White Spaces | Blank space, Horizintal tab, Carriage return, New line, Form feed |
Tokens are the smallest unit of the program meaningful to the compiler.
Tokens are classified as – Keywords, Identifiers, Contestants, Strings, Operators.
Expression may have one or more operators in it. If expression has multiple operators then these operators are solved according to hierarchy of operators. Hierarchy determines the order in which these operators are executed.
Table: Hierarchy of operators
Functions |
|
Power |
|
Mod |
|
*,/ |
|
+,- |
|
=,<,>,<=,>= |
|
NOT |
|
AND |
|
OR | Least Priority |
Ex.
Find the result of the following operations.
Sr. No. | Operation |
1. | 10/2 |
2. | 15 MOD 2 |
3. | NOT TRUE |
4. | 40 < =35 |
5. | FALSE AND TRUE |
6. | 60 – 15 |
7. | 55>10 |
8. | TRUE OR FALSE |
9. | – 10> 5 |
10. | 10* 0.3 |
Soln.:
Sr. No. | Operation | Solution |
1. | 10/2 | 5 |
2. | 15 MOD 2 | 1 |
3. | NOT TRUE | FALSE |
4. | 40 < =35 | FALSE |
5. | FALSE AND TRUE | FALSE |
6. | 60 – 15 | 45 |
7. | 55>10 | TRUE |
8. | TRUE OR FALSE | TRUE |
9. | – 10> 5 | FALSE |
10. | 10* 0.3 | 3 |
Ex.
Using Hierarchy chart, list the order in which following operations would be processed?
1. +,-,* 2 OR,*,< 3. NOT, AND,*
4. AND,OR,NOT 5. NOT,+,\ 6. MOD,\,< 7. <,AND,>,+
Soln. :
Sr. No. | Given Expression | Solution |
1 | +,-,* | *, +, - |
2 | OR,*,< | *, <, OR |
3 | NOT, AND,* | *, NOT, AND |
4 | AND,OR,NOT | NOT, AND, OR |
5 | NOT,+,\ | \, +, NOT |
6 | MOD,\,< | MOD, \, < |
7 | <,AND,>,+ | +, <,>,AND |
Before we study the basic building blocks of the C programming language, let us look at a bare minimum C program structure so that we can take it as a reference in the upcoming chapters.
Hello World Example
A C program basically consists of the following parts −
- Preprocessor Commands
- Functions
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" −
#include <stdio.h>
Int main() {
/* my first program in C */
Printf("Hello, World! \n");
Return 0;
}
Let us take a look at the various parts of the above program −
- The first line of the program #include <stdio.h> is a preprocessor command, which tells a C compiler to include stdio.h file before going to actual compilation.
- The next line int main() is the main function where the program execution begins.
- The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. So such lines are called comments in the program.
- The next line printf(...) is another function available in C which causes the message "Hello, World!" to be displayed on the screen.
- The next line return 0; terminates the main() function and returns the value 0.
Compile and Execute C Program
Let us see how to save the source code in a file, and how to compile and run it. Following are the simple steps −
- Open a text editor and add the above-mentioned code.
- Save the file as hello.c
- Open a command prompt and go to the directory where you have saved the file.
- Type gcc hello.c and press enter to compile your code.
- If there are no errors in your code, the command prompt will take you to the next line and would generate a.out executable file.
- Now, type a.out to execute your program.
- You will see the output "Hello World" printed on the screen.
$ gcc hello.c
$ ./a.out
Hello, World!
Make sure the gcc compiler is in your path and that you are running it in the directory containing the source file hello.c.
In C++, data types are declarations for variables. This determines the type and size of data associated with variables.
For example,
Int age = 13;
Here, age is a variable of type int. Meaning, the variable can only store integers of either 2 or 4 bytes.
C++ Fundamental Data Types
The table below shows the fundamental data types, their meaning, and their sizes (in bytes):
Data Type Meaning Size (in Bytes)
Int Integer 2 or 4
Float Floating-point 4
Double Double Floating-point 8
Char Character 1
Wchar_t Wide Character 2
Bool Boolean 1
Void Empty 0
1. Int
The int keyword is used to indicate integers.
Its size is usually 4 bytes. Meaning, it can store values from -2147483648 to 214748647.
For example,
Int salary = 85000;
2. float and double
Float and double are used to store floating-point numbers (decimals and exponentials).
The size of float is 4 bytes and the size of double is 8 bytes. Hence, double has two times the precision of float. To learn more, visit C++ float and double.
For example,
Float area = 64.74;
Double volume = 134.64534;
As mentioned above, these two data types are also used for exponentials. For example,
Double distance = 45E12 // 45E12 is equal to 45*10^12
3. Char
Keyword char is used for characters.
Its size is 1 byte.
Characters in C++ are enclosed inside single quotes ' '.
For example,
Char test = 'h';
Note: In C++, an integer value is stored in a char variable rather than the character itself. To learn more, visit C++ characters.
4. wchar_t
Wide character wchar_t is similar to the char data type, except its size is 2 bytes instead of 1.
It is used to represent characters that require more memory to represent them than a single char.
For example,
Wchar_t test = L'ם' // storing Hebrew character;
A variable stores a value in it. This value may change during processing.
- The name given to a variable is called as an identifier.
- Identifier is used as a reference name for a specific value of a variable.
- Computer uses this identifier name to locate a variable in memory.
- Certain rules must be followed while naming an identifier.
It is a symbol performs an action applied to variables ST.
Operands are the symbol on which operation is performed.
Operators are classified as unary and binary operators.
Binary operators are called as Arithmetic, Binary, Logical, Assignment, and Conditional and relational.
An expression is a formula in which operands are linked to each other by the use of operators to compute a value. An operand can be a function reference, a variable, an array element or a constant.
Let's see an example:
- a-b;
In the above expression, minus character (-) is an operator, and a, and b are the two operands.
There are four types of expressions exist in C:
- Arithmetic expressions
- Relational expressions
- Logical expressions
- Conditional expressions
Each type of expression takes certain types of operands and uses a specific set of operators. Evaluation of a particular expression produces a specific value.
For example:
- x = 9/2 + a-b;
The entire above line is a statement, not an expression. The portion after the equal is an expression.
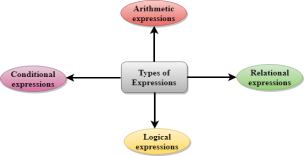
Arithmetic Expressions
An arithmetic expression is an expression that consists of operands and arithmetic operators. An arithmetic expression computes a value of type int, float or double.
When an expression contains only integral operands, then it is known as pure integer expression when it contains only real operands, it is known as pure real expression, and when it contains both integral and real operands, it is known as mixed mode expression.
Evaluation of Arithmetic Expressions
The expressions are evaluated by performing one operation at a time. The precedence and associativity of operators decide the order of the evaluation of individual operations.
When individual operations are performed, the following cases can be happened:
- When both the operands are of type integer, then arithmetic will be performed, and the result of the operation would be an integer value. For example, 3/2 will yield 1 not 1.5 as the fractional part is ignored.
- When both the operands are of type float, then arithmetic will be performed, and the result of the operation would be a real value. For example, 2.0/2.0 will yield 1.0, not 1.
- If one operand is of type integer and another operand is of type real, then the mixed arithmetic will be performed. In this case, the first operand is converted into a real operand, and then arithmetic is performed to produce the real value. For example, 6/2.0 will yield 3.0 as the first value of 6 is converted into 6.0 and then arithmetic is performed to produce 3.0.
Let's understand through an example.
6*2/ (2+1 * 2/3 + 6) + 8 * (8/4)
Evaluation of expression | Description of each operation |
6*2/( 2+1 * 2/3 +6) +8 * (8/4) | An expression is given. |
6*2/(2+2/3 + 6) + 8 * (8/4) | 2 is multiplied by 1, giving value 2. |
6*2/(2+0+6) + 8 * (8/4) | 2 is divided by 3, giving value 0. |
6*2/ 8+ 8 * (8/4) | 2 is added to 6, giving value 8. |
6*2/8 + 8 * 2 | 8 is divided by 4, giving value 2. |
12/8 +8 * 2 | 6 is multiplied by 2, giving value 12. |
1 + 8 * 2 | 12 is divided by 8, giving value 1. |
1 + 16 | 8 is multiplied by 2, giving value 16. |
17 | 1 is added to 16, giving value 17. |
Relational Expressions
- A relational expression is an expression used to compare two operands.
- It is a condition which is used to decide whether the action should be taken or not.
- In relational expressions, a numeric value cannot be compared with the string value.
- The result of the relational expression can be either zero or non-zero value. Here, the zero value is equivalent to a false and non-zero value is equivalent to true.
Relational Expression | Description |
x%2 = = 0 | This condition is used to check whether the x is an even number or not. The relational expression results in value 1 if x is an even number otherwise results in value 0. |
a!=b | It is used to check whether a is not equal to b. This relational expression results in 1 if a is not equal to b otherwise 0. |
a+b = = x+y | It is used to check whether the expression "a+b" is equal to the expression "x+y". |
a>=9 | It is used to check whether the value of a is greater than or equal to 9. |
Let's see a simple example:
- #include <stdio.h>
- Int main()
- {
- Int x=4;
- If(x%2==0)
- {
- Printf("The number x is even");
- }
- Else
- Printf("The number x is not even");
- Return 0;
- }
Output
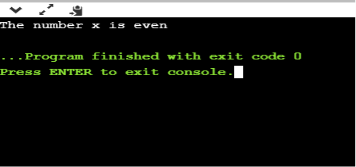
Logical Expressions
- A logical expression is an expression that computes either a zero or non-zero value.
- It is a complex test condition to take a decision.
Let's see some example of the logical expressions.
Logical Expressions | Description |
( x > 4 ) && ( x < 6 ) | It is a test condition to check whether the x is greater than 4 and x is less than 6. The result of the condition is true only when both the conditions are true. |
x > 10 || y <11 | It is a test condition used to check whether x is greater than 10 or y is less than 11. The result of the test condition is true if either of the conditions holds true value. |
! ( x > 10 ) && ( y = = 2 ) | It is a test condition used to check whether x is not greater than 10 and y is equal to 2. The result of the condition is true if both the conditions are true. |
Let's see a simple program of "&&" operator.
- #include <stdio.h>
- Int main()
- {
- Int x = 4;
- Int y = 10;
- If ( (x <10) && (y>5))
- {
- Printf("Condition is true");
- }
- Else
- Printf("Condition is false");
- Return 0;
- }
Output
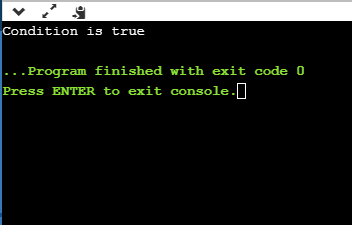
Let's see a simple example of "| |" operator
- #include <stdio.h>
- Int main()
- {
- Int x = 4;
- Int y = 9;
- If ( (x <6) || (y>10))
- {
- Printf("Condition is true");
- }
- Else
- Printf("Condition is false");
- Return 0;
- }
Output
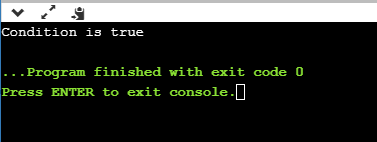
Conditional Expressions
- A conditional expression is an expression that returns 1 if the condition is true otherwise 0.
- A conditional operator is also known as a ternary operator.
The Syntax of Conditional operator
Suppose exp1, exp2 and exp3 are three expressions.
Exp1 ? exp2 : exp3
The above expression is a conditional expression which is evaluated on the basis of the value of the exp1 expression. If the condition of the expression exp1 holds true, then the final conditional expression is represented by exp2 otherwise represented by exp3.
Let's understand through a simple example.
- #include<stdio.h>
- #include<string.h>
- Int main()
- {
- Int age = 25;
- Char status;
- Status = (age>22) ? 'M': 'U';
- If(status == 'M')
- Printf("Married");
- Else
- Printf("Unmarried");
- Return 0;
- }
Output
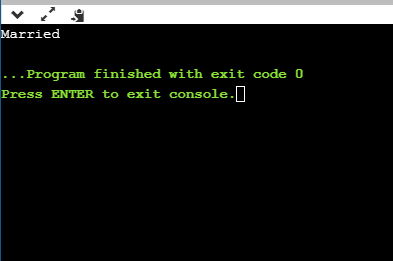
A program is consists of set of instructions. These instructions are not always written in linear sequence. Program is designed to make decisions and to execute set of instructions number of times. Control Structure satisfies these requirements.
1. Decision Making Statements.
Decision making statements consists of two or more conditions specified to execute statement so that any condition becomes true or false another condition executes.
1.1 The if-else statement.
The if else statement consists of if followed by else. Condition specified in if becomes true then statement in if block executes. When condition mentioned in if becomes false then statements in else execute.
Structure of the if – else.
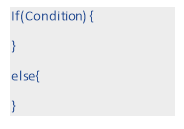
e.g.
1.2 Nested if – else
The Nested if- else consist of multiple if-else statements in another if – else. In this specified conditions are executed from top. While executing nested if else, when condition becomes true statement related with it gets execute and rest conditions are bypassed. When all the conditions become false last else statement gets execute.
Structure of the nested if – else:
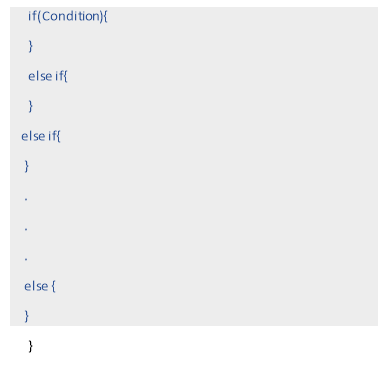
e.g.
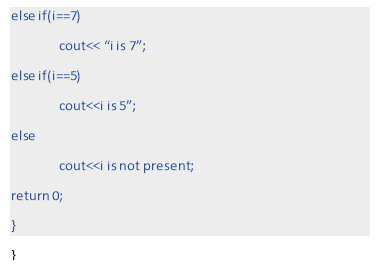
Here output is 5. When i=5 condition will match it will give is 5 output if not then last output will be i is not present.
1.3 The goto
The goto is an unconditional jump type of statement. The goto is used to jump between program from one point to another. The goto can be used to jump out of the nested loop.
Structure of the goto:
While executing program goto provides the information to goto instruction defined as a label. This label is initialized with goto statement before.
e.g.
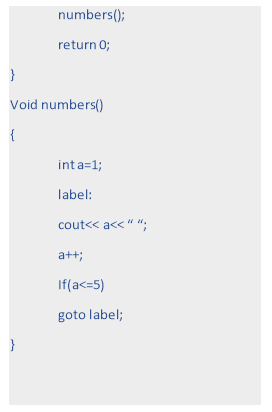
When the compiler will come to goto label then control will goto label defined before the loop and output will be 1 2 3 4 5.
1.4 The break
The break statement is used to terminate the execution where number of iterations is not unknown. It is used in loop to terminate the loop and then control goes to the first statement of the loop. The break statement is also in switch case.
Structure of the break:
e.g.
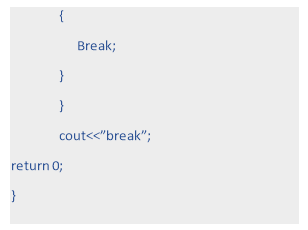
When the compiler will reach to break it will come out and print the output. Output will be x=1,x=9,x=8,x=7,x=6,break.
1.5 The continue
The continue statement work like break statement but it is opposite of break. The Continue statement continue with execution of program by jumping from current code instruction to the next.
Structure of the break:
e.g.
Output is 1 2 3 4 5.
1.6 The switch.
The switch statement is a branch selection statement. The switch statement consists of multiple cases and each case has number of statement. When the condition gets satisfied the statements associated with that case execute. The break statement is used inside switch loop to terminate the execution.
Structure of the switch statement:
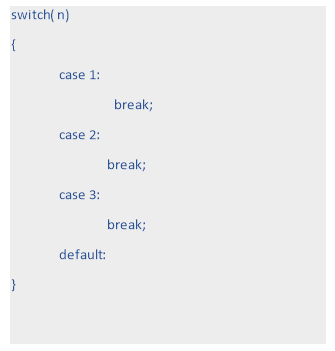
e.g.
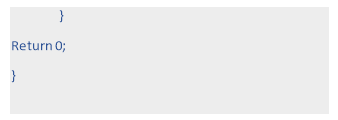
Output is “world”.
2. Loop Statements.
The for loop executes repeatedly until the condition specified in for loop is satisfied. When the condition becomes false it resumes with statement written in for loop.
Structure of the for loop:
Initialization is the assignment to the variable which controls the loop. Condition is an expression, specifies the execution of loop. Increment/ decrement operator changes the variable as the loop executes.
e.g.
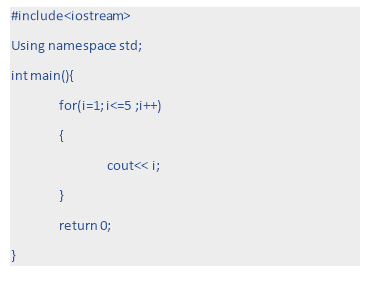
Output is 1 2 3 4 5.
Nested for loop is for within for loop.
Structure of the nested for loop:
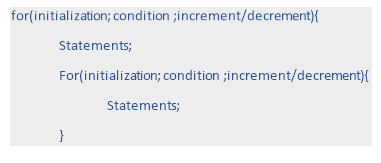
The while loop consists of a condition with set of statements. The Execution of the while loop is done until the condition mentioned in while is true. When the condition becomes false the control comes out of loop.
Structure of the while loop:
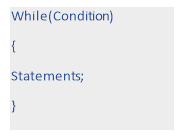
e.g.
X is initialized to 1. The loop will execute till x<=6.
Output will be 1 2 3 4 5 6 7 8 9 10.
The do – while loop consist of consist of condition mentioned at the bottom with while. The do – while executes till condition is true. As the condition is mentioned at bottom do – while loop is executed at least once.
Structure of the do – while:
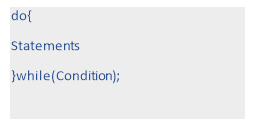
e.g.
Output is: 1 2 3 4 5 6 7 8 9 10.
A file represents a sequence of bytes, regardless of it being a text file or a binary file. C programming language provides access on high level functions as well as low level (OS level) calls to handle file on your storage devices. This chapter will take you through the important calls for file management.
Opening Files
You can use the fopen( ) function to create a new file or to open an existing file. This call will initialize an object of the type FILE, which contains all the information necessary to control the stream. The prototype of this function call is as follows −
FILE *fopen( const char * filename, const char * mode );
Here, filename is a string literal, which you will use to name your file, and access mode can have one of the following values −
Sr.No. | Mode & Description |
1 | R Opens an existing text file for reading purpose. |
2 | W Opens a text file for writing. If it does not exist, then a new file is created. Here your program will start writing content from the beginning of the file. |
3 | A Opens a text file for writing in appending mode. If it does not exist, then a new file is created. Here your program will start appending content in the existing file content. |
4 | r+ Opens a text file for both reading and writing. |
5 | w+ Opens a text file for both reading and writing. It first truncates the file to zero length if it exists, otherwise creates a file if it does not exist. |
6 | a+ Opens a text file for both reading and writing. It creates the file if it does not exist. The reading will start from the beginning but writing can only be appended. |
If you are going to handle binary files, then you will use following access modes instead of the above mentioned ones −
"rb", "wb", "ab", "rb+", "r+b", "wb+", "w+b", "ab+", "a+b"
Closing a File
To close a file, use the fclose( ) function. The prototype of this function is −
Int fclose( FILE *fp );
The fclose(-) function returns zero on success, or EOF if there is an error in closing the file. This function actually flushes any data still pending in the buffer to the file, closes the file, and releases any memory used for the file. The EOF is a constant defined in the header file stdio.h.
There are various functions provided by C standard library to read and write a file, character by character, or in the form of a fixed length string.
Writing a File
Following is the simplest function to write individual characters to a stream −
Int fputc( int c, FILE *fp );
The function fputc() writes the character value of the argument c to the output stream referenced by fp. It returns the written character written on success otherwise EOF if there is an error. You can use the following functions to write a null-terminated string to a stream −
Int fputs( const char *s, FILE *fp );
The function fputs() writes the string s to the output stream referenced by fp. It returns a non-negative value on success, otherwise EOF is returned in case of any error. You can use int fprintf(FILE *fp,const char *format, ...) function as well to write a string into a file. Try the following example.
Make sure you have /tmp directory available. If it is not, then before proceeding, you must create this directory on your machine.
#include <stdio.h>
Main() {
FILE *fp;
Fp = fopen("/tmp/test.txt", "w+");
Fprintf(fp, "This is testing for fprintf...\n");
Fputs("This is testing for fputs...\n", fp);
Fclose(fp);
}
When the above code is compiled and executed, it creates a new file test.txt in /tmp directory and writes two lines using two different functions. Let us read this file in the next section.
Reading a File
Given below is the simplest function to read a single character from a file −
Int fgetc( FILE * fp );
The fgetc() function reads a character from the input file referenced by fp. The return value is the character read, or in case of any error, it returns EOF. The following function allows to read a string from a stream −
Char *fgets( char *buf, int n, FILE *fp );
The functions fgets() reads up to n-1 characters from the input stream referenced by fp. It copies the read string into the buffer buf, appending a null character to terminate the string.
If this function encounters a newline character '\n' or the end of the file EOF before they have read the maximum number of characters, then it returns only the characters read up to that point including the new line character. You can also use int fscanf(FILE *fp, const char *format, ...) function to read strings from a file, but it stops reading after encountering the first space character.
#include <stdio.h>
Main() {
FILE *fp;
Char buff[255];
Fp = fopen("/tmp/test.txt", "r");
Fscanf(fp, "%s", buff);
Printf("1 : %s\n", buff );
Fgets(buff, 255, (FILE*)fp);
Printf("2: %s\n", buff );
Fgets(buff, 255, (FILE*)fp);
Printf("3: %s\n", buff );
Fclose(fp);
}
When the above code is compiled and executed, it reads the file created in the previous section and produces the following result −
1 : This
2: is testing for fprintf...
3: This is testing for fputs...
Let's see a little more in detail about what happened here. First, fscanf() read just This because after that, it encountered a space, second call is for fgets() which reads the remaining line till it encountered end of line. Finally, the last call fgets() reads the second line completely.
Binary I/O Functions
There are two functions, that can be used for binary input and output −
Size_t fread(void *ptr, size_t size_of_elements, size_t number_of_elements, FILE *a_file);
Size_t fwrite(const void *ptr, size_t size_of_elements, size_t number_of_elements, FILE *a_file);
Both of these functions should be used to read or write blocks of memories - usually arrays or structures.
An array is collection of same type of items placed in continuous memory address.
Array helps to represent multiple instances using single variable.
When the variable is used in large number of objects, it becomes difficult to manage.
Array used to store in an instance.
An integer index is used to access an array. Index of array starts with 0 and ends with size of array -1.
Elements should not be initialized more than the size of array.
Example – Different types of array declaration.
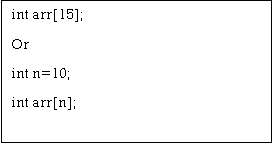
Int arr[] = { 10, 20, 30, 40 } |
A function is the block of statement. An entire program is divided into multiple blocks to perform specific task simultaneously. Every program has one function; is the main().Each function has declaration , function call and definition.
Function Declaration
Function is declared globally to inform the compiler about function name, function parameters and return type.

Example
Function call
Function call has parameter list with function name. Using function call function can be called anywhere in program.

Example
Function definition.
Function definition consists of block of statements which contains actual execution code. When function gets called in program control comes to the function definition.
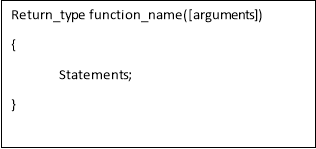
Example
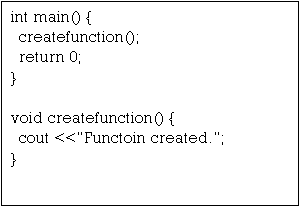
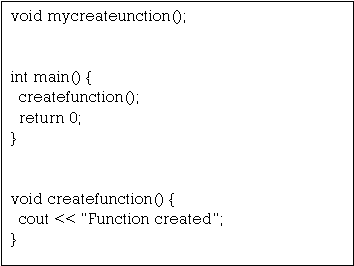
Reference Books:
1. Introduction of Computers: Peter Norton, TMH
2. Object Oriented Programming with C++:E. Balagurusamy, TMH
3. Object Oriented Programming in C++: Rajesh K.Shukla, Wiley India
4. Concepts in Computing: Kenneth Hoganson, Jones & Bartlett.
5. Operating Systems – Silberschatz and Galvin - Wiley India
6. Computer Networks: Andrew Tananbaum, PHI
7. Data Base Management Systems, Korth, TMH
8. Cloud Computing, Kumar, Wiley India