Unit - 2
Inheritance and Polymorphism
Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Why use inheritance in java
● For Method overriding (so runtime polymorphism can be achieved).
● For Code Reusability.
Terms used in Inheritance
● Class: A class is a group of objects which have common properties. It is a template or blueprint from which objects are created.
● Sub Class/Child Class: Subclass is a class which inherits the other class. It is also called a derived class, extended class, or child class.
● Super Class/Parent Class: Superclass is the class from where a subclass inherits the features. It is also called a base class or a parent class.
● Reusability: As the name specifies, reusability is a mechanism which facilitates you to reuse the fields and methods of the existing class when you create a new class. You can use the same fields and methods already defined in the previous class.
The syntax of Java Inheritance
- Class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
The extends keyword indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
In the terminology of Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass.
Java Inheritance Example
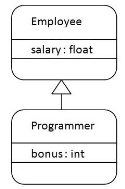
As displayed in the above figure, Programmer is the subclass and Employee is the superclass. The relationship between the two classes is Programmer IS-A Employee. It means that Programmer is a type of Employee.
- Class Employee{
- Float salary=40000;
- }
- Class Programmer extends Employee{
- Int bonus=10000;
- Public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
Programmer salary is:40000.0
Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Key takeaway
Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
Types of Inheritance
1. Single Inheritance
Also called as single level inheritance it is the mechanism of deriving a single sub class from a super class. The subclass will be defined by the keyword extends which signifies that the properties of the super class are extended to the sub class. The sub class will contain its own variables and methods as well as it can access methods and variables of the super class.
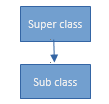
Syntax:
Class Subclass-name extends Superclass-name
{
//methods and fields
}
Program:
Class x
{
Void one()
{
System.out.println("Printing one");
}
}
Class y extends x
{
Void two()
{
System.out.println("Printing two");
}
}
Public class test
{
Public static void main(String args[])
{
y t= new y();
t.two();
t.one();
}
}
Output:
Printing two
Printing one
2. Multi- level Inheritance
It is the mechanism of deriving a sub class from a super class and again a sub class is derived from this derived subclass which acts as a base class for the newly derived class. It includes a multi level base class which allows building a chain of classes where a derived class acts as super class.
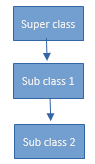
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}class three extends two
{
Void print_three()
{
System.out.println("Printing three");}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
t.print_three();
t.print_two(); t.print_one();
}
}
Output:
Printing three
Printing two
Printing one
3. Hierarchical Inheritance
In Hierarchical Inheritance, one class serves as a super class (base class) for more than one sub class.
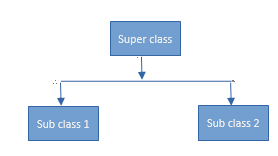
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}
Class three extends two
{
Void print_three()
{
System.out.println("Printing three");
}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
Two n= new two();t.print_three();
n.print_two();
t.print_one();
}}
Output:
Printing three
Printing two
Printing one
4. Hybrid Inheritance
It is the combination of Single inheritance and multi level inheritance.
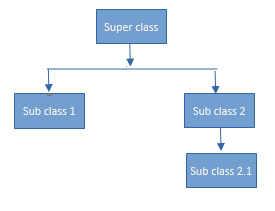
Key takeaway
In Java, there are three types of inheritance based on class: single, multilayer, and hierarchical inheritance.
Multiple and hybrid inheritance are only enabled through interfaces in Java programming.
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers. They are as follows:
● public - A public modifier's access level is visible everywhere. It can be accessible from within and outside the class, from within and outside the package, and from within and outside the package.
● protected - A protected modifier's access level is both within the package and outside the package via a child class. The child class cannot be accessed from outside the package unless it is created.
● default - A default modifier's access level is limited to the package. It's not possible to get to it from outside the package. If you don't indicate an access level, the default will be used.
● private - A private modifier's access level is limited to the class. It isn't accessible outside of the class.
The access modifier for that member (variable or method) will be default if it is not declared as public, protected, or private. Access modifiers can also be applied to classes.
Private is the most restrictive of the four access modifiers, whereas public is the least restrictive. The following is the syntax for declaring an access modifier:
Access-modifier data-type variable-name;
Java Access Modifiers
Let's look at a basic table to see how access modifiers work in Java.
Access Modifier | Within class | Within package | Outside package by subclass only | Outside package |
Private | Y | N | N | N |
Default | Y | Y | N | N |
Protected | Y | Y | Y | N |
Public | Y | Y | Y | Y |
Private
Only members of the class have access to the private access modifier.
An example of a private access modifier in action
We've built two classes in this example: A and Simple. Private data members and private methods are found in a class. There is a compile-time problem since we are accessing these secret members from outside the class.
Class A{
Private int data=40;
Private void msg(){System.out.println("Hello java");}
}
Public class Simple{
Public static void main(String args[]){
A obj=new A();
System.out.println(obj.data);//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Default
If you don't specify a modifier, it will be treated as default. The default modifier is only available within the package. It's not possible to get to it from outside the package. It allows for more accessibility than a private setting. It is, nevertheless, more limited than protected and open.
A default access modifier is one example.
We've produced two packages in this example: pack and mypack. We're accessing A from outside its package because A isn't public and so can't be accessed from outside the package.
//save by A.java
Package pack;
Class A{
Void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Protected
The protected access modifier can be used both inside and outside of the package, but only through inheritance.
The data member, method, and function Object() { [native code] } can all have the protected access modifier applied to them. It is not applicable to the class.
It is more accessible than the standard modifier.
A protected access modifier is an example.
We've produced two packages in this example: pack and mypack. The public A class of the pack package can be accessed from outside the package. However, because this package's msg function is protected, it can only be accessible from outside the class through inheritance.
//save by A.java
Package pack;
Public class A{
Protected void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B extends A{
Public static void main(String args[]){
B obj = new B();
Obj.msg();
}
}
Output:Hello
Public
The public access modifier can be used everywhere. Among all the modifiers, it has the broadest application.
A public access modifier is an example.
//save by A.java
Package pack;
Public class A{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
Output: Hello
Key takeaway
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers.
A reference variable in Java is a variable that refers to the current object of a method or constructor. The main use of this term in Java is to distinguish between class attributes and arguments with similar names.
The following are some examples of 'this' keyword usage:
● It can be used to refer to current class instance variables.
● It can be used to call or start the constructor of the current class.
● It can be used as a parameter in a method call.
● It's possible to send it as an argument to the constructor.
● It can be used to get the current instance of a class.
This keyword in Java can be used in a variety of ways. This is a reference variable in Java that points to the current object.
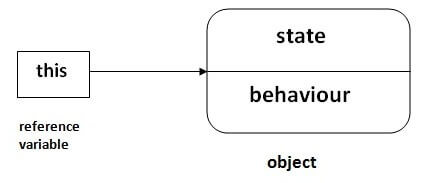
Fig: this
Example
Class Student{
Int rollno;
String name;
Float fee;
Student(int rollno,String name,float fee){
This.rollno=rollno;
This.name=name;
This.fee=fee;
}
Void display(){System.out.println(rollno+" "+name+" "+fee);}
}
Class TestThis2{
Public static void main(String args[]){
Student s1=new Student(111,"ankit",5000f);
Student s2=new Student(112,"sumit",6000f);
s1.display();
s2.display();
}
}
Output
111 ankit 5000.0
112 sumit 6000.0
Key takeaway
The main use of this term in Java is to distinguish between class attributes and arguments with similar names.
It refers to an immediate parent class object. There are 3 ways to use the ‘super’ keyword:
- To refer immediate parent class instance variable.
- To invoke immediate parent class method.
- To invoke immediate parent class constructor.
Program to refer immediate parent class instance variable using super keyword
Class transport
{
Int maxspeed = 200;
}
Class vehicle extends transport
{
Int maxspeed =100;
}
Class car extends vehicle
{
Int maxspeed = 120;
Void disp()
{System.out.println("Max speed= "+super.maxspeed);
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.disp();
}
}
Output:
Max speed= 100
Program to invoke immediate parent class method
Class transport
{
Void message()
{
System.out.println("transport");
}
}
Class vehicle extends transport
{
Void message()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Int maxspeed = 120;
Void message()
{
System.out.println("car");
}
Void display()
{
Message();
Super.message();
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.display();}
}
Output:
Car
Vehicle
Program to invoke immediate parent class constructor
Class vehicle
{
Vehicle()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Car()
{
Super();
System.out.println("car");
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
}
}
Output:
Vehicle
Car
Key takeaway
In Java, the super keyword is a reference variable that refers to an immediate parent class object.
When you create a subclass instance, you're also creating an instance of the parent class, which is referenced to by the super reference variable.
Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
Method overloading is an implementation of polymorphism in a code which is “One interface, multiple methods” paradigm.
To invoke an overloaded method, it is important to pass the right number or arguments or parameters; since this will determine which version of method to actually call.
All the overloaded methods have different return type but we cant distinguish method based on the type of data they return.
Consider a simple example of method overloading:
Class Addition {
// Overloaded method takes two int parameters
Public int add(int a, int b)
{
Return (a + b);
}
// Overloaded method takes three int parameters
Public int add(int a, int b, int c)
{
Return (a + b + c);
}
// Overloaded method takes two double parameters
Public double add(double a, double b)
{
Return (a + b);
}
Public static void main(String args[])
{
Addition a = new Addition();
System.out.println(a.add(100, 20));
System.out.println(a.add(11, 2, 3));
System.out.println(a.add(0.5, 2.5));
}
}
Output:
120
16
3.0
While creating a overloaded method it s not necessary that all the methods must relate to one another. For example one add() method can do addition, while other add() method can concatenate string; since each version of a method perform a separate activity you desire.
However, it is preferable to see a relationship between overloaded method as shown in the above example, where all add() method doing addition.
Benefits of Method Overloading
● Technique over-burdening builds the coherence of the program.
● This gives adaptability to developers with the goal that they can call similar strategy for various sorts of information.
● This makes the code look spotless.
● This lessens the execution time in light of the fact that the limiting is done in accumulation time itself.
● Technique over-burdening limits the intricacy of the code.
● With this, we can utilize the code once more, which saves memory.
Key takeaway
Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
In Java, method overriding occurs when a subclass (child class) has the same method as the parent class.
In other words, method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
Usage of Java Method Overriding
● Method overriding is a technique for providing a particular implementation of a method that has already been implemented by its superclass.
● For runtime polymorphism, method overriding is employed.
Rules for method overriding
● The argument list should match that of the overridden method exactly.
● The return type must be the same as or a subtype of the return type stated in the superclass's original overridden method.
● The access level must be lower than the access level of the overridden method. For instance, if the superclass method is marked public, the subclass overriding method cannot be either private or protected.
● Instance methods can only be overridden if the subclass inherits them.
● It is not possible to override a method that has been declared final.
● A static method cannot be overridden, but it can be re-declared.
● A method can't be overridden if it can't be inherited.
● Any superclass function that is not declared private or final can be overridden by a subclass in the same package as the instance's superclass.
● Only non-final methods labeled public or protected can be overridden by a subclass from a different package.
● Regardless of whether the overridden method throws exceptions or not, an overriding method can throw any unchecked exceptions. The overriding method, on the other hand, should not throw any checked exceptions that aren't specified by the overridden method. In comparison to the overridden method, the overriding method can throw fewer or narrower exceptions.
● Overriding constructors is not possible.
Example
//Java Program to illustrate the use of Java Method Overriding
//Creating a parent class.
Class Vehicle{
//defining a method
Void run(){System.out.println("Vehicle is running");}
}
//Creating a child class
Class Bike2 extends Vehicle{
//defining the same method as in the parent class
Void run(){System.out.println("Bike is running safely");}
Public static void main(String args[]){
Bike2 obj = new Bike2();//creating object
Obj.run();//calling method
}
}
Output
Bike is running safely
Key takeaway
Method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
● Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
● It can only be inherited by the concrete classes (descendent classes), where a concrete class can be instantiated.
● Abstract class act as a generic class having attributes and methods which can be commonly shared and accessed by the concrete classes.
● The attributes and methods in the abstract class are non-static, accessible by inheriting classes.
● Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class
● It can be represented as below
Abstract public class RentalCar {
// your code goes here
}
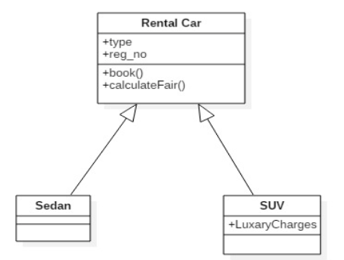
Fig: Example of abstract class
Key takeaway
- Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
- Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class.
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
When you want a variable to constantly store the same value, such as PI, the final keyword comes in handy (3.14159...).
The last keyword is referred to as a "modifier."
Example
Set a variable to final, to prevent it from being overridden/modified:
Public class Main {
Final int x = 10;
Public static void main(String[] args) {
Main myObj = new Main();
MyObj.x = 25; // will generate an error: cannot assign a value to a final variable
System.out.println(myObj.x);
}
}
Using Final with Inheritance
● The final keyword is final, which means we can't change anything.
● Variables, methods, and classes can all benefit from final keywords.
● If we use the final keyword for inheritance, that is, if we declare any method in the base class with the final keyword, the final method's implementation will be the same as in the derived class.
● If any other class extends this subclass, we can declare the final method in any subclass we wish.
Declare final variable with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final variable pa of string type i.e
The value of this variable is fixed throughout all
The derived classes or not overridden*/
Final String pa = "Hello , We are in parent class variable";
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of variable ch of string type i.e
The value of this variable is not fixed throughout all
The derived classes or overridden*/
String ch = "Hello , We are in child class variable";
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a variable pa by parent object
System.out.println(p.pa);
// Creation of Child class object
Child c = new Child();
// Calling a variable ch by Child object
System.out.println(c.ch);
// Calling a variable pa by Child object
System.out.println(c.pa);
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, We are in parent class variable
Hello, We are in child class variable
Hello, We are in parent class variable
Declare final methods with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final method parent of void type i.e
The implementation of this method is fixed throughout
All the derived classes or not overridden*/
Final void parent() {
System.out.println("Hello , we are in parent method");
}
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of final method child of void type i.e
The implementation of this method is not fixed throughout
All the derived classes or not overridden*/
Void child() {
System.out.println("Hello , we are in child method");
}
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a method parent() by parent object
p.parent();
// Creation of Child class object
Child c = new Child();
// Calling a method child() by Child class object
c.child();
// Calling a method parent() by child object
c.parent();
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, we are in parent method
Hello, we are in child method
Hello, we are in parent method
Key takeaway
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
References:
1. T. Budd (2009), An Introduction to Object Oriented Programming, 3rd edition, Pearson Education, India.
2. J. Nino, F. A. Hosch (2002), An Introduction to programming and OO design using Java, John Wiley & sons, New Jersey.
3. Y. Daniel Liang (2010), Introduction to Java programming, 7th edition, Pearson education, India.
4. Object Oriented Programming with C++ by E Balagurusamy, Fifth Edition, TMH.
Unit - 2
Inheritance and Polymorphism
Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Why use inheritance in java
● For Method overriding (so runtime polymorphism can be achieved).
● For Code Reusability.
Terms used in Inheritance
● Class: A class is a group of objects which have common properties. It is a template or blueprint from which objects are created.
● Sub Class/Child Class: Subclass is a class which inherits the other class. It is also called a derived class, extended class, or child class.
● Super Class/Parent Class: Superclass is the class from where a subclass inherits the features. It is also called a base class or a parent class.
● Reusability: As the name specifies, reusability is a mechanism which facilitates you to reuse the fields and methods of the existing class when you create a new class. You can use the same fields and methods already defined in the previous class.
The syntax of Java Inheritance
- Class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
The extends keyword indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
In the terminology of Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass.
Java Inheritance Example
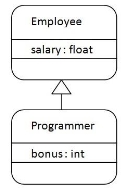
As displayed in the above figure, Programmer is the subclass and Employee is the superclass. The relationship between the two classes is Programmer IS-A Employee. It means that Programmer is a type of Employee.
- Class Employee{
- Float salary=40000;
- }
- Class Programmer extends Employee{
- Int bonus=10000;
- Public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
Programmer salary is:40000.0
Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Key takeaway
Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
Types of Inheritance
1. Single Inheritance
Also called as single level inheritance it is the mechanism of deriving a single sub class from a super class. The subclass will be defined by the keyword extends which signifies that the properties of the super class are extended to the sub class. The sub class will contain its own variables and methods as well as it can access methods and variables of the super class.
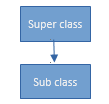
Syntax:
Class Subclass-name extends Superclass-name
{
//methods and fields
}
Program:
Class x
{
Void one()
{
System.out.println("Printing one");
}
}
Class y extends x
{
Void two()
{
System.out.println("Printing two");
}
}
Public class test
{
Public static void main(String args[])
{
y t= new y();
t.two();
t.one();
}
}
Output:
Printing two
Printing one
2. Multi- level Inheritance
It is the mechanism of deriving a sub class from a super class and again a sub class is derived from this derived subclass which acts as a base class for the newly derived class. It includes a multi level base class which allows building a chain of classes where a derived class acts as super class.
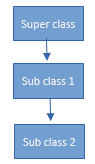
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}class three extends two
{
Void print_three()
{
System.out.println("Printing three");}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
t.print_three();
t.print_two(); t.print_one();
}
}
Output:
Printing three
Printing two
Printing one
3. Hierarchical Inheritance
In Hierarchical Inheritance, one class serves as a super class (base class) for more than one sub class.
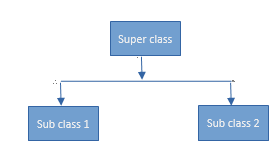
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}
Class three extends two
{
Void print_three()
{
System.out.println("Printing three");
}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
Two n= new two();t.print_three();
n.print_two();
t.print_one();
}}
Output:
Printing three
Printing two
Printing one
4. Hybrid Inheritance
It is the combination of Single inheritance and multi level inheritance.
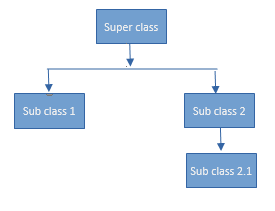
Key takeaway
In Java, there are three types of inheritance based on class: single, multilayer, and hierarchical inheritance.
Multiple and hybrid inheritance are only enabled through interfaces in Java programming.
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers. They are as follows:
● public - A public modifier's access level is visible everywhere. It can be accessible from within and outside the class, from within and outside the package, and from within and outside the package.
● protected - A protected modifier's access level is both within the package and outside the package via a child class. The child class cannot be accessed from outside the package unless it is created.
● default - A default modifier's access level is limited to the package. It's not possible to get to it from outside the package. If you don't indicate an access level, the default will be used.
● private - A private modifier's access level is limited to the class. It isn't accessible outside of the class.
The access modifier for that member (variable or method) will be default if it is not declared as public, protected, or private. Access modifiers can also be applied to classes.
Private is the most restrictive of the four access modifiers, whereas public is the least restrictive. The following is the syntax for declaring an access modifier:
Access-modifier data-type variable-name;
Java Access Modifiers
Let's look at a basic table to see how access modifiers work in Java.
Access Modifier | Within class | Within package | Outside package by subclass only | Outside package |
Private | Y | N | N | N |
Default | Y | Y | N | N |
Protected | Y | Y | Y | N |
Public | Y | Y | Y | Y |
Private
Only members of the class have access to the private access modifier.
An example of a private access modifier in action
We've built two classes in this example: A and Simple. Private data members and private methods are found in a class. There is a compile-time problem since we are accessing these secret members from outside the class.
Class A{
Private int data=40;
Private void msg(){System.out.println("Hello java");}
}
Public class Simple{
Public static void main(String args[]){
A obj=new A();
System.out.println(obj.data);//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Default
If you don't specify a modifier, it will be treated as default. The default modifier is only available within the package. It's not possible to get to it from outside the package. It allows for more accessibility than a private setting. It is, nevertheless, more limited than protected and open.
A default access modifier is one example.
We've produced two packages in this example: pack and mypack. We're accessing A from outside its package because A isn't public and so can't be accessed from outside the package.
//save by A.java
Package pack;
Class A{
Void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Protected
The protected access modifier can be used both inside and outside of the package, but only through inheritance.
The data member, method, and function Object() { [native code] } can all have the protected access modifier applied to them. It is not applicable to the class.
It is more accessible than the standard modifier.
A protected access modifier is an example.
We've produced two packages in this example: pack and mypack. The public A class of the pack package can be accessed from outside the package. However, because this package's msg function is protected, it can only be accessible from outside the class through inheritance.
//save by A.java
Package pack;
Public class A{
Protected void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B extends A{
Public static void main(String args[]){
B obj = new B();
Obj.msg();
}
}
Output:Hello
Public
The public access modifier can be used everywhere. Among all the modifiers, it has the broadest application.
A public access modifier is an example.
//save by A.java
Package pack;
Public class A{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
Output: Hello
Key takeaway
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers.
A reference variable in Java is a variable that refers to the current object of a method or constructor. The main use of this term in Java is to distinguish between class attributes and arguments with similar names.
The following are some examples of 'this' keyword usage:
● It can be used to refer to current class instance variables.
● It can be used to call or start the constructor of the current class.
● It can be used as a parameter in a method call.
● It's possible to send it as an argument to the constructor.
● It can be used to get the current instance of a class.
This keyword in Java can be used in a variety of ways. This is a reference variable in Java that points to the current object.
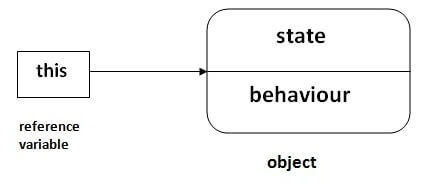
Fig: this
Example
Class Student{
Int rollno;
String name;
Float fee;
Student(int rollno,String name,float fee){
This.rollno=rollno;
This.name=name;
This.fee=fee;
}
Void display(){System.out.println(rollno+" "+name+" "+fee);}
}
Class TestThis2{
Public static void main(String args[]){
Student s1=new Student(111,"ankit",5000f);
Student s2=new Student(112,"sumit",6000f);
s1.display();
s2.display();
}
}
Output
111 ankit 5000.0
112 sumit 6000.0
Key takeaway
The main use of this term in Java is to distinguish between class attributes and arguments with similar names.
It refers to an immediate parent class object. There are 3 ways to use the ‘super’ keyword:
- To refer immediate parent class instance variable.
- To invoke immediate parent class method.
- To invoke immediate parent class constructor.
Program to refer immediate parent class instance variable using super keyword
Class transport
{
Int maxspeed = 200;
}
Class vehicle extends transport
{
Int maxspeed =100;
}
Class car extends vehicle
{
Int maxspeed = 120;
Void disp()
{System.out.println("Max speed= "+super.maxspeed);
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.disp();
}
}
Output:
Max speed= 100
Program to invoke immediate parent class method
Class transport
{
Void message()
{
System.out.println("transport");
}
}
Class vehicle extends transport
{
Void message()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Int maxspeed = 120;
Void message()
{
System.out.println("car");
}
Void display()
{
Message();
Super.message();
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.display();}
}
Output:
Car
Vehicle
Program to invoke immediate parent class constructor
Class vehicle
{
Vehicle()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Car()
{
Super();
System.out.println("car");
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
}
}
Output:
Vehicle
Car
Key takeaway
In Java, the super keyword is a reference variable that refers to an immediate parent class object.
When you create a subclass instance, you're also creating an instance of the parent class, which is referenced to by the super reference variable.
Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
Method overloading is an implementation of polymorphism in a code which is “One interface, multiple methods” paradigm.
To invoke an overloaded method, it is important to pass the right number or arguments or parameters; since this will determine which version of method to actually call.
All the overloaded methods have different return type but we cant distinguish method based on the type of data they return.
Consider a simple example of method overloading:
Class Addition {
// Overloaded method takes two int parameters
Public int add(int a, int b)
{
Return (a + b);
}
// Overloaded method takes three int parameters
Public int add(int a, int b, int c)
{
Return (a + b + c);
}
// Overloaded method takes two double parameters
Public double add(double a, double b)
{
Return (a + b);
}
Public static void main(String args[])
{
Addition a = new Addition();
System.out.println(a.add(100, 20));
System.out.println(a.add(11, 2, 3));
System.out.println(a.add(0.5, 2.5));
}
}
Output:
120
16
3.0
While creating a overloaded method it s not necessary that all the methods must relate to one another. For example one add() method can do addition, while other add() method can concatenate string; since each version of a method perform a separate activity you desire.
However, it is preferable to see a relationship between overloaded method as shown in the above example, where all add() method doing addition.
Benefits of Method Overloading
● Technique over-burdening builds the coherence of the program.
● This gives adaptability to developers with the goal that they can call similar strategy for various sorts of information.
● This makes the code look spotless.
● This lessens the execution time in light of the fact that the limiting is done in accumulation time itself.
● Technique over-burdening limits the intricacy of the code.
● With this, we can utilize the code once more, which saves memory.
Key takeaway
Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
In Java, method overriding occurs when a subclass (child class) has the same method as the parent class.
In other words, method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
Usage of Java Method Overriding
● Method overriding is a technique for providing a particular implementation of a method that has already been implemented by its superclass.
● For runtime polymorphism, method overriding is employed.
Rules for method overriding
● The argument list should match that of the overridden method exactly.
● The return type must be the same as or a subtype of the return type stated in the superclass's original overridden method.
● The access level must be lower than the access level of the overridden method. For instance, if the superclass method is marked public, the subclass overriding method cannot be either private or protected.
● Instance methods can only be overridden if the subclass inherits them.
● It is not possible to override a method that has been declared final.
● A static method cannot be overridden, but it can be re-declared.
● A method can't be overridden if it can't be inherited.
● Any superclass function that is not declared private or final can be overridden by a subclass in the same package as the instance's superclass.
● Only non-final methods labeled public or protected can be overridden by a subclass from a different package.
● Regardless of whether the overridden method throws exceptions or not, an overriding method can throw any unchecked exceptions. The overriding method, on the other hand, should not throw any checked exceptions that aren't specified by the overridden method. In comparison to the overridden method, the overriding method can throw fewer or narrower exceptions.
● Overriding constructors is not possible.
Example
//Java Program to illustrate the use of Java Method Overriding
//Creating a parent class.
Class Vehicle{
//defining a method
Void run(){System.out.println("Vehicle is running");}
}
//Creating a child class
Class Bike2 extends Vehicle{
//defining the same method as in the parent class
Void run(){System.out.println("Bike is running safely");}
Public static void main(String args[]){
Bike2 obj = new Bike2();//creating object
Obj.run();//calling method
}
}
Output
Bike is running safely
Key takeaway
Method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
● Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
● It can only be inherited by the concrete classes (descendent classes), where a concrete class can be instantiated.
● Abstract class act as a generic class having attributes and methods which can be commonly shared and accessed by the concrete classes.
● The attributes and methods in the abstract class are non-static, accessible by inheriting classes.
● Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class
● It can be represented as below
Abstract public class RentalCar {
// your code goes here
}
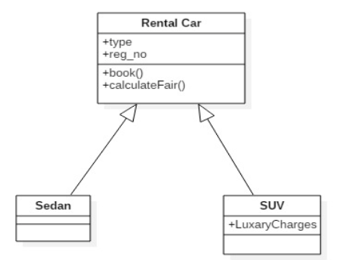
Fig: Example of abstract class
Key takeaway
- Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
- Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class.
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
When you want a variable to constantly store the same value, such as PI, the final keyword comes in handy (3.14159...).
The last keyword is referred to as a "modifier."
Example
Set a variable to final, to prevent it from being overridden/modified:
Public class Main {
Final int x = 10;
Public static void main(String[] args) {
Main myObj = new Main();
MyObj.x = 25; // will generate an error: cannot assign a value to a final variable
System.out.println(myObj.x);
}
}
Using Final with Inheritance
● The final keyword is final, which means we can't change anything.
● Variables, methods, and classes can all benefit from final keywords.
● If we use the final keyword for inheritance, that is, if we declare any method in the base class with the final keyword, the final method's implementation will be the same as in the derived class.
● If any other class extends this subclass, we can declare the final method in any subclass we wish.
Declare final variable with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final variable pa of string type i.e
The value of this variable is fixed throughout all
The derived classes or not overridden*/
Final String pa = "Hello , We are in parent class variable";
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of variable ch of string type i.e
The value of this variable is not fixed throughout all
The derived classes or overridden*/
String ch = "Hello , We are in child class variable";
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a variable pa by parent object
System.out.println(p.pa);
// Creation of Child class object
Child c = new Child();
// Calling a variable ch by Child object
System.out.println(c.ch);
// Calling a variable pa by Child object
System.out.println(c.pa);
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, We are in parent class variable
Hello, We are in child class variable
Hello, We are in parent class variable
Declare final methods with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final method parent of void type i.e
The implementation of this method is fixed throughout
All the derived classes or not overridden*/
Final void parent() {
System.out.println("Hello , we are in parent method");
}
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of final method child of void type i.e
The implementation of this method is not fixed throughout
All the derived classes or not overridden*/
Void child() {
System.out.println("Hello , we are in child method");
}
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a method parent() by parent object
p.parent();
// Creation of Child class object
Child c = new Child();
// Calling a method child() by Child class object
c.child();
// Calling a method parent() by child object
c.parent();
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, we are in parent method
Hello, we are in child method
Hello, we are in parent method
Key takeaway
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
References:
1. T. Budd (2009), An Introduction to Object Oriented Programming, 3rd edition, Pearson Education, India.
2. J. Nino, F. A. Hosch (2002), An Introduction to programming and OO design using Java, John Wiley & sons, New Jersey.
3. Y. Daniel Liang (2010), Introduction to Java programming, 7th edition, Pearson education, India.
4. Object Oriented Programming with C++ by E Balagurusamy, Fifth Edition, TMH.