Unit - 4
I/O Streams
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Stream
A stream can be defined as a sequence of data. There are two kinds of Streams −
● InPut Stream − The Input Stream is used to read data from a source.
● OutPut Stream − The Output Stream is used for writing data to a destination.

Fig 1: Stream
Java provides strong but flexible support for I/O related to files and networks but this tutorial covers very basic functionality related to streams and I/O. We will see the most commonly used examples one by one −
Byte Streams
Java byte streams are used to perform input and output of 8-bit bytes. Though there are many classes related to byte streams but the most frequently used classes are, FileInputStream and FileOutputStream. Following is an example which makes use of these two classes to copy an input file into an output file −
Example
Import java.io.*;
Public class CopyFile {
Public static void main(String args[]) throws IOException {
FileInputStream in = null;
FileOutputStream out = null;
Try {
In = new FileInputStream("input.txt");
Out = new FileOutputStream("output.txt");
Int c;
While ((c = in.read()) != -1) {
Out.write(c);
}
}finally {
If (in != null) {
In.close();
}
If (out != null) {
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Character Streams
Java Byte streams are used to perform input and output of 8-bit bytes, whereas Java Character streams are used to perform input and output for 16-bit unicode. Though there are many classes related to character streams but the most frequently used classes are, FileReader and FileWriter. Though internally FileReader uses FileInputStream and FileWriter uses FileOutputStream but here the major difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
We can rewrite the above example, which makes the use of these two classes to copy an input file (having unicode characters) into an output file −
Example
Import java.io.*;
Public class CopyFile {
Public static void main(String args[]) throws IOException {
FileReader in = null;
FileWriter out = null;
Try {
In = new FileReader("input.txt");
Out = new FileWriter("output.txt");
Int c;
While ((c = in.read()) != -1) {
Out.write(c);
}
}finally {
If (in != null) {
In.close();
}
If (out != null) {
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Standard Streams
All the programming languages provide support for standard I/O where the user's program can take input from a keyboard and then produce an output on the computer screen. If you are aware of C or C++ programming languages, then you must be aware of three standard devices STDIN, STDOUT and STDERR. Similarly, Java provides the following three standard streams −
● Standard Input − This is used to feed the data to user's program and usually a keyboard is used as standard input stream and represented as System.in.
● Standard Output − This is used to output the data produced by the user's program and usually a computer screen is used for standard output stream and represented as System.out.
● Standard Error − This is used to output the error data produced by the user's program and usually a computer screen is used for standard error stream and represented as System.err.
Following is a simple program, which creates InputStreamReader to read standard input stream until the user types a "q" −
Example
Import java.io.*;
Public class ReadConsole {
Public static void main(String args[]) throws IOException {
InputStreamReader cin = null;
Try {
Cin = new InputStreamReader(System.in);
System.out.println("Enter characters, 'q' to quit.");
Char c;
Do {
c = (char) cin.read();
System.out.print(c);
} while(c != 'q');
}finally {
If (cin != null) {
Cin.close();
}
}
}
}
Let's keep the above code in ReadConsole.java file and try to compile and execute it as shown in the following program. This program continues to read and output the same character until we press 'q' −
$javac ReadConsole.java
$java ReadConsole
Enter characters, 'q' to quit.
1
1
e
e
q
q
Reading and Writing Files
As described earlier, a stream can be defined as a sequence of data. The InputStream is used to read data from a source and the OutputStream is used for writing data to a destination.
Here is a hierarchy of classes to deal with Input and Output streams.
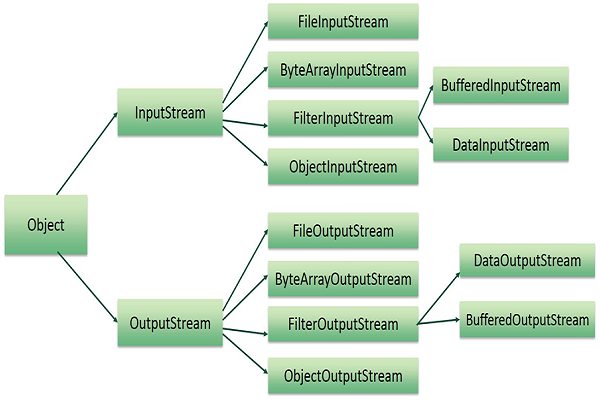
Fig 2: Hierarchy of classes to deal with Input and Output streams
File Input Stream
This stream is used for reading data from the files. Objects can be created using the keyword new and there are several types of constructors available.
Following constructor takes a file name as a string to create an input stream object to read the file −
InputStream f = new FileInputStream("C:/java/hello");
Following constructor takes a file object to create an input stream object to read the file. First we create a file object using File() method as follows −
File f = new File("C:/java/hello");
InputStream f = new FileInputStream(f);
Once you have InputStream object in hand, then there is a list of helper methods which can be used to read to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public int read(int r)throws IOException{} This method reads the specified byte of data from the InputStream. Returns an int. Returns the next byte of data and -1 will be returned if it's the end of the file. |
4 | Public int read(byte[] r) throws IOException{} This method reads r.length bytes from the input stream into an array. Returns the total number of bytes read. If it is the end of the file, -1 will be returned. |
5 | Public int available() throws IOException{} Gives the number of bytes that can be read from this file input stream. Returns an int. |
There are other important input streams available, for more detail you can refer to the following links –
Byte Array Input Stream
Data Input Stream
File Output Stream
File Output Stream is used to create a file and write data into it. The stream would create a file, if it doesn't already exist, before opening it for output.
Here are two constructors which can be used to create a File Output Stream object.
Following constructor takes a file name as a string to create an input stream object to write the file −
OutputStream f = new FileOutputStream("C:/java/hello")
Following constructor takes a file object to create an output stream object to write the file. First, we create a file object using File() method as follows −
File f = new File("C:/java/hello");
OutputStream f = new FileOutputStream(f);
Once you have OutputStream object in hand, then there is a list of helper methods, which can be used to write to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public void write(int w)throws IOException{} This methods writes the specified byte to the output stream. |
4 | Public void write(byte[] w) Writes w.length bytes from the mentioned byte array to the OutputStream. |
There are other important output streams available, for more detail you can refer to the following links –
Byte Array Output Stream
Data Output Stream
Example
Following is the example to demonstrate Input Stream and Output Stream −
Import java.io.*;
Public class fileStreamTest {
Public static void main(String args[]) {
Try {
Byte bWrite [] = {11,21,3,40,5};
OutputStream os = new FileOutputStream("test.txt");
For(int x = 0; x < bWrite.length ; x++) {
Os.write( bWrite[x] ); // writes the bytes
}
Os.close();
InputStream is = new FileInputStream("test.txt");
Int size = is.available();
For(int i = 0; i < size; i++) {
System.out.print((char)is.read() + " ");
}
Is.close();
} catch (IOException e) {
System.out.print("Exception");
}
}
}
The above code would create file test.txt and would write given numbers in binary format. Same would be the output on the stdout screen.
File Navigation and I/O
There are several other classes that we would be going through to get to know the basics of File Navigation and I/O.
● File Class
● FileReader Class
● FileWriter Class
Directories in Java
A directory is a File which can contain a list of other files and directories. You use File object to create directories, to list down files available in a directory. For complete detail, check a list of all the methods which you can call on File object and what are related to directories.
Creating Directories
There are two useful File utility methods, which can be used to create directories:
● The mkdir( ) method creates a directory, returning true on success and false on failure. Failure indicates that the path specified in the File object already exists, or that the directory cannot be created because the entire path does not exist yet.
● The mkdirs() method creates both a directory and all the parents of the directory.
Following example creates "/tmp/user/java/bin" directory −
Example
Import java.io.File;
Public class CreateDir {
Public static void main(String args[]) {
String dirname = "/tmp/user/java/bin";
File d = new File(dirname);
// Create directory now.
d.mkdirs();
}
}
Compile and execute the above code to create "/tmp/user/java/bin".
Note − Java automatically takes care of path separators on UNIX and Windows as per conventions. If you use a forward slash (/) on a Windows version of Java, the path will still resolve correctly.
Listing Directories
You can use list( ) method provided by File object to list down all the files and directories available in a directory as follows −
Example
Import java.io.File;
Public class ReadDir {
Public static void main(String[] args) {
File file = null;
String[] paths;
Try {
// create new file object
File = new File("/tmp");
// array of files and directory
Paths = file.list();
// for each name in the path array
For(String path:paths) {
// prints filename and directory name
System.out.println(path);
}
} catch (Exception e) {
// if any error occurs
e.printStackTrace();
}
}
}
This will produce the following result based on the directories and files available in your /tmp directory −
Output
Test1.txt
Test2.txt
ReadDir.java
ReadDir.class
Key takeaway
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Java Console Class
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
Let's see a simple example to read text from console.
- String text=System.console().readLine();
- System.out.println("Text is: "+text);
Java Console class declaration
Let's see the declaration for Java.io.Console class:
Public final class Console extends Object implements Flushable
Java Console class methods
Method | Description |
Reader reader() | It is used to retrieve the reader object associated with the console |
String readLine() | It is used to read a single line of text from the console. |
String readLine(String fmt, Object... Args) | It provides a formatted prompt then reads the single line of text from the console. |
Char[] readPassword() | It is used to read password that is not being displayed on the console. |
Char[] readPassword(String fmt, Object... Args) | It provides a formatted prompt then reads the password that is not being displayed on the console. |
Console format(String fmt, Object... Args) | It is used to write a formatted string to the console output stream. |
Console printf(String format, Object... Args) | It is used to write a string to the console output stream. |
PrintWriter writer() | It is used to retrieve the PrintWriter object associated with the console. |
Void flush() | It is used to flushes the console. |
How to get the object of Console
System class provides a static method console() that returns the singleton instance of Console class.
- Public static Console console(){}
Let's see the code to get the instance of Console class.
- Console c=System.console();
Java Console Example
- Import java.io.Console;
- Class ReadStringTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter your name: ");
- String n=c.readLine();
- System.out.println("Welcome "+n);
- }
- }
Output
Enter your name: Nakul Jain
Welcome Nakul Jain
Java Console Example to read password
- Import java.io.Console;
- Class ReadPasswordTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter password: ");
- Char[] ch=c.readPassword();
- String pass=String.valueOf(ch);//converting char array into string
- System.out.println("Password is: "+pass);
- }
- }
Output
Enter password:
Password is: 123
Key takeaway
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
We can work with files in Java using the File Class. The java.io package contains this File Class. To use the File class, first create an object of the class and then specify the file's name.
Why is File Handling Necessary?
File handling is an essential aspect of any programming language since it allows us to save the output of any programme in a file and execute operations on it.
To put it another way, file handling is the process of reading and writing data to a file.
Example
// Import the File class
Import java.io.File
// Specify the filename
File obj = new File("filename.txt");
To perform I/O operations on a file, Java employs the concept of a stream. So, let's look at what a Stream is in Java.
What is a Stream?
A stream in Java is a collection of data that can be of two types.
Byte Stream
This primarily applies to byte data. The file handling process with a byte stream is when an input is provided and executed using byte data.
Character Stream
A character stream is a stream in which characters are incorporated. The file handling process with a character stream is the processing of incoming data using characters.
Now that you understand what a stream is, continue reading this article on File Handling in Java to learn about the many methods that may be used to perform actions on files such as generating, reading, and writing.
Java File Methods
The methods for conducting operations on Java files are shown in the table below.
Method | Type | Description |
CanRead() | Boolean | It tests whether the file is readable or not |
CanWrite() | Boolean | It tests whether the file is writable or not |
CreateNewFile() | Boolean | This method creates an empty file |
Delete() | Boolean | Deletes a file |
Exists() | Boolean | It tests whether the file exists |
GetName() | String | Returns the name of the file |
GetAbsolutePath() | String | Returns the absolute pathname of the file |
Length() | Long | Returns the size of the file in bytes |
List() | String[] | Returns an array of the files in the directory |
Mkdir() | Boolean | Creates a directory |
File Operations in Java
A file can be processed in four different ways. They are as follows:
● Create a File
● Get File Information
● Write To a File
● Read from a File
Let's look at the specifics of each of these operations now.
Create a File
The createNewFile() method can be used to create a file in this instance. If the file was successfully created, this method returns true; if the file already exists, it returns false.
Let's have a look at how to make a file in Java.
Package FileHandling;
// Import the File class
Import java.io.File;
// Import the IOException class to handle errors
Import java.io.IOException;
Public class CreateFile {
Public static void main(String[] args) {
Try {
// Creating an object of a file
File myObj = new File("D:FileHandlingNewFilef1.txt");
If (myObj.createNewFile()) {
System.out.println("File created: " + myObj.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
A file named NewFilef1 is created in the specified location by the code above. If an error occurs, it is handled in the catch block. Let's see what happens when we run the aforementioned code:
Output:
File created: NewFilef1.txt
Now that you've grasped this, let's look at how to retrieve File information.
Get File information
With the help of the example code below, let's look at how to get file information using various methods.
Package FileHandling;
Import java.io.File; // Import the File class
Public class FileInformation {
Public static void main(String[] args) {
// Creating an object of a file
File myObj = new File("NewFilef1.txt");
If (myObj.exists()) {
// Returning the file name
System.out.println("File name: " + myObj.getName());
// Returning the path of the file
System.out.println("Absolute path: " + myObj.getAbsolutePath());
// Displaying whether the file is writable
System.out.println("Writeable: " + myObj.canWrite());
// Displaying whether the file is readable or not
System.out.println("Readable " + myObj.canRead());
// Returning the length of the file in bytes
System.out.println("File size in bytes " + myObj.length());
} else {
System.out.println("The file does not exist.");
}
}
}
When you run the aforementioned application, you'll obtain the following file information in the output:
Output:
File name: NewFilef1.txt
Absolute path: D:FileHandlingNewFilef1.txt
Writable: true
Readable true
File size in bytes 52
This is how you construct a programme to get the information from a particular file. Let's have a look at two additional actions on the file now. In other words, read and write operations.
Write to a File
I've written some text into a file using the FileWriter class and its write() function in the following example. Let's have a look at this with the help of some code.
Package FileHandling;
// Import the FileWriter class
Import java.io.FileWriter;
// Import the IOException class to handle errors
Import java.io.IOException;
Public class WriteToFile {
Public static void main(String[] args) {
Try {
FileWriter myWriter = new FileWriter("D:FileHandlingNewFilef1.txt");
// Writes this content into the specified file
MyWriter.write(Java is the prominent programming language of the millenium!");
// Closing is necessary to retrieve the resources allocated
MyWriter.close();
System.out.println("Successfully wrote to the file.");
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Output:
Successfully wrote to the file
When you run the file, the text "Java is the millennium's most popular programming language!" will be added to the file you've created. By opening the file in the provided place, you may double-check it.
Now let's look at the last operation on the file, which is to read a file.
Read from a File
To read the contents of the text file, I used the Scanner class in the following example.
Package FileHandling;
// Import the File class
Import java.io.File;
// Import this class to handle errors
Import java.io.FileNotFoundException;
// Import the Scanner class to read text files
Import java.util.Scanner;
Public class ReadFromFile {
Public static void main(String[] args) {
Try {
// Creating an object of the file for reading the data
File myObj = new File("D:FileHandlingNewFilef1.txt");
Scanner myReader = new Scanner(myObj);
While (myReader.hasNextLine()) {
String data = myReader.nextLine();
System.out.println(data);
}
MyReader.close();
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
When you run the aforesaid software, the contents of the supplied file will be shown.
Output:
Java is the prominent programming language of the millennium!
Key takeaway
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
References:
1. T. Budd (2009), An Introduction to Object Oriented Programming, 3rd edition, Pearson Education, India.
2. J. Nino, F. A. Hosch (2002), An Introduction to programming and OO design using Java, John Wiley & sons, New Jersey.
3. Y. Daniel Liang (2010), Introduction to Java programming, 7th edition, Pearson education, India.
4. Object Oriented Programming with C++ by E Balagurusamy, Fifth Edition, TMH.
Unit - 4
I/O Streams
Unit - 4
I/O Streams
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Stream
A stream can be defined as a sequence of data. There are two kinds of Streams −
● InPut Stream − The Input Stream is used to read data from a source.
● OutPut Stream − The Output Stream is used for writing data to a destination.

Fig 1: Stream
Java provides strong but flexible support for I/O related to files and networks but this tutorial covers very basic functionality related to streams and I/O. We will see the most commonly used examples one by one −
Byte Streams
Java byte streams are used to perform input and output of 8-bit bytes. Though there are many classes related to byte streams but the most frequently used classes are, FileInputStream and FileOutputStream. Following is an example which makes use of these two classes to copy an input file into an output file −
Example
Import java.io.*;
Public class CopyFile {
Public static void main(String args[]) throws IOException {
FileInputStream in = null;
FileOutputStream out = null;
Try {
In = new FileInputStream("input.txt");
Out = new FileOutputStream("output.txt");
Int c;
While ((c = in.read()) != -1) {
Out.write(c);
}
}finally {
If (in != null) {
In.close();
}
If (out != null) {
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Character Streams
Java Byte streams are used to perform input and output of 8-bit bytes, whereas Java Character streams are used to perform input and output for 16-bit unicode. Though there are many classes related to character streams but the most frequently used classes are, FileReader and FileWriter. Though internally FileReader uses FileInputStream and FileWriter uses FileOutputStream but here the major difference is that FileReader reads two bytes at a time and FileWriter writes two bytes at a time.
We can rewrite the above example, which makes the use of these two classes to copy an input file (having unicode characters) into an output file −
Example
Import java.io.*;
Public class CopyFile {
Public static void main(String args[]) throws IOException {
FileReader in = null;
FileWriter out = null;
Try {
In = new FileReader("input.txt");
Out = new FileWriter("output.txt");
Int c;
While ((c = in.read()) != -1) {
Out.write(c);
}
}finally {
If (in != null) {
In.close();
}
If (out != null) {
Out.close();
}
}
}
}
Now let's have a file input.txt with the following content −
This is test for copy file.
As a next step, compile the above program and execute it, which will result in creating output.txt file with the same content as we have in input.txt. So let's put the above code in CopyFile.java file and do the following −
$javac CopyFile.java
$java CopyFile
Standard Streams
All the programming languages provide support for standard I/O where the user's program can take input from a keyboard and then produce an output on the computer screen. If you are aware of C or C++ programming languages, then you must be aware of three standard devices STDIN, STDOUT and STDERR. Similarly, Java provides the following three standard streams −
● Standard Input − This is used to feed the data to user's program and usually a keyboard is used as standard input stream and represented as System.in.
● Standard Output − This is used to output the data produced by the user's program and usually a computer screen is used for standard output stream and represented as System.out.
● Standard Error − This is used to output the error data produced by the user's program and usually a computer screen is used for standard error stream and represented as System.err.
Following is a simple program, which creates InputStreamReader to read standard input stream until the user types a "q" −
Example
Import java.io.*;
Public class ReadConsole {
Public static void main(String args[]) throws IOException {
InputStreamReader cin = null;
Try {
Cin = new InputStreamReader(System.in);
System.out.println("Enter characters, 'q' to quit.");
Char c;
Do {
c = (char) cin.read();
System.out.print(c);
} while(c != 'q');
}finally {
If (cin != null) {
Cin.close();
}
}
}
}
Let's keep the above code in ReadConsole.java file and try to compile and execute it as shown in the following program. This program continues to read and output the same character until we press 'q' −
$javac ReadConsole.java
$java ReadConsole
Enter characters, 'q' to quit.
1
1
e
e
q
q
Reading and Writing Files
As described earlier, a stream can be defined as a sequence of data. The InputStream is used to read data from a source and the OutputStream is used for writing data to a destination.
Here is a hierarchy of classes to deal with Input and Output streams.
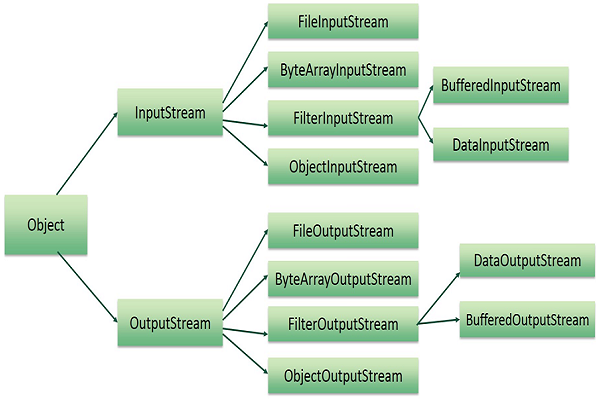
Fig 2: Hierarchy of classes to deal with Input and Output streams
File Input Stream
This stream is used for reading data from the files. Objects can be created using the keyword new and there are several types of constructors available.
Following constructor takes a file name as a string to create an input stream object to read the file −
InputStream f = new FileInputStream("C:/java/hello");
Following constructor takes a file object to create an input stream object to read the file. First we create a file object using File() method as follows −
File f = new File("C:/java/hello");
InputStream f = new FileInputStream(f);
Once you have InputStream object in hand, then there is a list of helper methods which can be used to read to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public int read(int r)throws IOException{} This method reads the specified byte of data from the InputStream. Returns an int. Returns the next byte of data and -1 will be returned if it's the end of the file. |
4 | Public int read(byte[] r) throws IOException{} This method reads r.length bytes from the input stream into an array. Returns the total number of bytes read. If it is the end of the file, -1 will be returned. |
5 | Public int available() throws IOException{} Gives the number of bytes that can be read from this file input stream. Returns an int. |
There are other important input streams available, for more detail you can refer to the following links –
Byte Array Input Stream
Data Input Stream
File Output Stream
File Output Stream is used to create a file and write data into it. The stream would create a file, if it doesn't already exist, before opening it for output.
Here are two constructors which can be used to create a File Output Stream object.
Following constructor takes a file name as a string to create an input stream object to write the file −
OutputStream f = new FileOutputStream("C:/java/hello")
Following constructor takes a file object to create an output stream object to write the file. First, we create a file object using File() method as follows −
File f = new File("C:/java/hello");
OutputStream f = new FileOutputStream(f);
Once you have OutputStream object in hand, then there is a list of helper methods, which can be used to write to stream or to do other operations on the stream.
Sr.No. | Method & Description |
1 | Public void close() throws IOException{} This method closes the file output stream. Releases any system resources associated with the file. Throws an IOException. |
2 | Protected void finalize()throws IOException {} This method cleans up the connection to the file. Ensures that the close method of this file output stream is called when there are no more references to this stream. Throws an IOException. |
3 | Public void write(int w)throws IOException{} This methods writes the specified byte to the output stream. |
4 | Public void write(byte[] w) Writes w.length bytes from the mentioned byte array to the OutputStream. |
There are other important output streams available, for more detail you can refer to the following links –
Byte Array Output Stream
Data Output Stream
Example
Following is the example to demonstrate Input Stream and Output Stream −
Import java.io.*;
Public class fileStreamTest {
Public static void main(String args[]) {
Try {
Byte bWrite [] = {11,21,3,40,5};
OutputStream os = new FileOutputStream("test.txt");
For(int x = 0; x < bWrite.length ; x++) {
Os.write( bWrite[x] ); // writes the bytes
}
Os.close();
InputStream is = new FileInputStream("test.txt");
Int size = is.available();
For(int i = 0; i < size; i++) {
System.out.print((char)is.read() + " ");
}
Is.close();
} catch (IOException e) {
System.out.print("Exception");
}
}
}
The above code would create file test.txt and would write given numbers in binary format. Same would be the output on the stdout screen.
File Navigation and I/O
There are several other classes that we would be going through to get to know the basics of File Navigation and I/O.
● File Class
● FileReader Class
● FileWriter Class
Directories in Java
A directory is a File which can contain a list of other files and directories. You use File object to create directories, to list down files available in a directory. For complete detail, check a list of all the methods which you can call on File object and what are related to directories.
Creating Directories
There are two useful File utility methods, which can be used to create directories:
● The mkdir( ) method creates a directory, returning true on success and false on failure. Failure indicates that the path specified in the File object already exists, or that the directory cannot be created because the entire path does not exist yet.
● The mkdirs() method creates both a directory and all the parents of the directory.
Following example creates "/tmp/user/java/bin" directory −
Example
Import java.io.File;
Public class CreateDir {
Public static void main(String args[]) {
String dirname = "/tmp/user/java/bin";
File d = new File(dirname);
// Create directory now.
d.mkdirs();
}
}
Compile and execute the above code to create "/tmp/user/java/bin".
Note − Java automatically takes care of path separators on UNIX and Windows as per conventions. If you use a forward slash (/) on a Windows version of Java, the path will still resolve correctly.
Listing Directories
You can use list( ) method provided by File object to list down all the files and directories available in a directory as follows −
Example
Import java.io.File;
Public class ReadDir {
Public static void main(String[] args) {
File file = null;
String[] paths;
Try {
// create new file object
File = new File("/tmp");
// array of files and directory
Paths = file.list();
// for each name in the path array
For(String path:paths) {
// prints filename and directory name
System.out.println(path);
}
} catch (Exception e) {
// if any error occurs
e.printStackTrace();
}
}
}
This will produce the following result based on the directories and files available in your /tmp directory −
Output
Test1.txt
Test2.txt
ReadDir.java
ReadDir.class
Key takeaway
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Java Console Class
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
Let's see a simple example to read text from console.
- String text=System.console().readLine();
- System.out.println("Text is: "+text);
Java Console class declaration
Let's see the declaration for Java.io.Console class:
Public final class Console extends Object implements Flushable
Java Console class methods
Method | Description |
Reader reader() | It is used to retrieve the reader object associated with the console |
String readLine() | It is used to read a single line of text from the console. |
String readLine(String fmt, Object... Args) | It provides a formatted prompt then reads the single line of text from the console. |
Char[] readPassword() | It is used to read password that is not being displayed on the console. |
Char[] readPassword(String fmt, Object... Args) | It provides a formatted prompt then reads the password that is not being displayed on the console. |
Console format(String fmt, Object... Args) | It is used to write a formatted string to the console output stream. |
Console printf(String format, Object... Args) | It is used to write a string to the console output stream. |
PrintWriter writer() | It is used to retrieve the PrintWriter object associated with the console. |
Void flush() | It is used to flushes the console. |
How to get the object of Console
System class provides a static method console() that returns the singleton instance of Console class.
- Public static Console console(){}
Let's see the code to get the instance of Console class.
- Console c=System.console();
Java Console Example
- Import java.io.Console;
- Class ReadStringTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter your name: ");
- String n=c.readLine();
- System.out.println("Welcome "+n);
- }
- }
Output
Enter your name: Nakul Jain
Welcome Nakul Jain
Java Console Example to read password
- Import java.io.Console;
- Class ReadPasswordTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter password: ");
- Char[] ch=c.readPassword();
- String pass=String.valueOf(ch);//converting char array into string
- System.out.println("Password is: "+pass);
- }
- }
Output
Enter password:
Password is: 123
Key takeaway
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
We can work with files in Java using the File Class. The java.io package contains this File Class. To use the File class, first create an object of the class and then specify the file's name.
Why is File Handling Necessary?
File handling is an essential aspect of any programming language since it allows us to save the output of any programme in a file and execute operations on it.
To put it another way, file handling is the process of reading and writing data to a file.
Example
// Import the File class
Import java.io.File
// Specify the filename
File obj = new File("filename.txt");
To perform I/O operations on a file, Java employs the concept of a stream. So, let's look at what a Stream is in Java.
What is a Stream?
A stream in Java is a collection of data that can be of two types.
Byte Stream
This primarily applies to byte data. The file handling process with a byte stream is when an input is provided and executed using byte data.
Character Stream
A character stream is a stream in which characters are incorporated. The file handling process with a character stream is the processing of incoming data using characters.
Now that you understand what a stream is, continue reading this article on File Handling in Java to learn about the many methods that may be used to perform actions on files such as generating, reading, and writing.
Java File Methods
The methods for conducting operations on Java files are shown in the table below.
Method | Type | Description |
CanRead() | Boolean | It tests whether the file is readable or not |
CanWrite() | Boolean | It tests whether the file is writable or not |
CreateNewFile() | Boolean | This method creates an empty file |
Delete() | Boolean | Deletes a file |
Exists() | Boolean | It tests whether the file exists |
GetName() | String | Returns the name of the file |
GetAbsolutePath() | String | Returns the absolute pathname of the file |
Length() | Long | Returns the size of the file in bytes |
List() | String[] | Returns an array of the files in the directory |
Mkdir() | Boolean | Creates a directory |
File Operations in Java
A file can be processed in four different ways. They are as follows:
● Create a File
● Get File Information
● Write To a File
● Read from a File
Let's look at the specifics of each of these operations now.
Create a File
The createNewFile() method can be used to create a file in this instance. If the file was successfully created, this method returns true; if the file already exists, it returns false.
Let's have a look at how to make a file in Java.
Package FileHandling;
// Import the File class
Import java.io.File;
// Import the IOException class to handle errors
Import java.io.IOException;
Public class CreateFile {
Public static void main(String[] args) {
Try {
// Creating an object of a file
File myObj = new File("D:FileHandlingNewFilef1.txt");
If (myObj.createNewFile()) {
System.out.println("File created: " + myObj.getName());
} else {
System.out.println("File already exists.");
}
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
A file named NewFilef1 is created in the specified location by the code above. If an error occurs, it is handled in the catch block. Let's see what happens when we run the aforementioned code:
Output:
File created: NewFilef1.txt
Now that you've grasped this, let's look at how to retrieve File information.
Get File information
With the help of the example code below, let's look at how to get file information using various methods.
Package FileHandling;
Import java.io.File; // Import the File class
Public class FileInformation {
Public static void main(String[] args) {
// Creating an object of a file
File myObj = new File("NewFilef1.txt");
If (myObj.exists()) {
// Returning the file name
System.out.println("File name: " + myObj.getName());
// Returning the path of the file
System.out.println("Absolute path: " + myObj.getAbsolutePath());
// Displaying whether the file is writable
System.out.println("Writeable: " + myObj.canWrite());
// Displaying whether the file is readable or not
System.out.println("Readable " + myObj.canRead());
// Returning the length of the file in bytes
System.out.println("File size in bytes " + myObj.length());
} else {
System.out.println("The file does not exist.");
}
}
}
When you run the aforementioned application, you'll obtain the following file information in the output:
Output:
File name: NewFilef1.txt
Absolute path: D:FileHandlingNewFilef1.txt
Writable: true
Readable true
File size in bytes 52
This is how you construct a programme to get the information from a particular file. Let's have a look at two additional actions on the file now. In other words, read and write operations.
Write to a File
I've written some text into a file using the FileWriter class and its write() function in the following example. Let's have a look at this with the help of some code.
Package FileHandling;
// Import the FileWriter class
Import java.io.FileWriter;
// Import the IOException class to handle errors
Import java.io.IOException;
Public class WriteToFile {
Public static void main(String[] args) {
Try {
FileWriter myWriter = new FileWriter("D:FileHandlingNewFilef1.txt");
// Writes this content into the specified file
MyWriter.write(Java is the prominent programming language of the millenium!");
// Closing is necessary to retrieve the resources allocated
MyWriter.close();
System.out.println("Successfully wrote to the file.");
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
Output:
Successfully wrote to the file
When you run the file, the text "Java is the millennium's most popular programming language!" will be added to the file you've created. By opening the file in the provided place, you may double-check it.
Now let's look at the last operation on the file, which is to read a file.
Read from a File
To read the contents of the text file, I used the Scanner class in the following example.
Package FileHandling;
// Import the File class
Import java.io.File;
// Import this class to handle errors
Import java.io.FileNotFoundException;
// Import the Scanner class to read text files
Import java.util.Scanner;
Public class ReadFromFile {
Public static void main(String[] args) {
Try {
// Creating an object of the file for reading the data
File myObj = new File("D:FileHandlingNewFilef1.txt");
Scanner myReader = new Scanner(myObj);
While (myReader.hasNextLine()) {
String data = myReader.nextLine();
System.out.println(data);
}
MyReader.close();
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
}
When you run the aforesaid software, the contents of the supplied file will be shown.
Output:
Java is the prominent programming language of the millennium!
Key takeaway
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
References:
1. T. Budd (2009), An Introduction to Object Oriented Programming, 3rd edition, Pearson Education, India.
2. J. Nino, F. A. Hosch (2002), An Introduction to programming and OO design using Java, John Wiley & sons, New Jersey.
3. Y. Daniel Liang (2010), Introduction to Java programming, 7th edition, Pearson education, India.
4. Object Oriented Programming with C++ by E Balagurusamy, Fifth Edition, TMH.