Unit - 1
Object Oriented Programming features
Features of Object Oriented Programming
The most important features of OOP language which make it different from other programming language are discussed below.
1. Classes: In OOP the real world entity can be represented with the help of class which depicts its framework for all the objects.
A class can consist of as many objects as a programmer want.
2. Objects: Objects are used to store data and values. The data is stored by using variables and methods are used to define the behaviour or function of that object.
By using a single class, we can create as many objects as we want and all these objects will have all the variables and behaviour of a class.
3. Encapsulation: It is the way toward restricting object’s state (fields) and behaviours (methods) together in a single entity called "Class".
Since it envelops the two fields and methods by a class, it will be secured from the external access.
We can limit the access to the methods of a class utilizing access modifiers, for example, private, protected and public keywords.
The object deals with its own state by means of these methods and no other class can modify it except if external permission is provided. To use the object, you should use the methods provided.
Whenever a class is created in Java, it implies we are doing encapsulation.
It also helps in accomplishing the reusability of code without compromising its security.
4. Abstraction: Abstraction is an enhancement of encapsulation. It is used to show only required data and hide rest of the data of an object from the client.
5. Inheritance: By using the concept of inheritance we can inherit few/all properties of another object. For instance, a child acquires the qualities of his/her parents.
Reusability is a significant benefit of using inheritance. You can reuse the fields and methods of the already created class.
With the help of inheritance, we can create a new class by inheriting the features and methods of any existing class.
Java provides us different types of inheritance such as single, multiple, multilevel, hierarchical, and hybrid.
6. Polymorphism: Polymorphism provides us the flexibility to define an object in multiple forms. The main use of polymorphism in OOP is when reference of a parent class is made to a child class object.
Here if a message is sent to multiple class objects, then every objects gives response according to their fields and methods. So, response of every class object will be different for a single request.
Concept of Objects and Classes
Objects have states and behaviors that they exhibit. A dog, for example, has states such as color, name, and breed, as well as behaviors such as waving the tail, barking, and eating. A class's instance is an object.
Let's take a closer look at what objects are. Many objects, such as vehicles, pets, humans, and other living things, can be found in the actual world. There is a state and a behavior for each of these things.
If we consider a dog, its state includes its name, breed, and color, as well as its activity, which includes barking, wagging the tail, and running.
When you compare a software object to a real-world thing, you'll see that they have a lot in common.
Object and Class Example: main within the class
We've developed a Student class in this example, which has two data members: id and name. The object of the Student class is created using the new keyword, and the object's value is printed.
Inside the class, we're going to create a main() method.
//Java Program to illustrate how to define a class and fields
//Defining a Student class.
Class Student{
//defining fields
int id;//field or data member or instance variable
String name;
//creating main method inside the Student class
public static void main(String args[]){
//Creating an object or instance
Student s1=new Student();//creating an object of Student
//Printing values of the object
System.out.println(s1.id);//accessing member through reference variable
System.out.println(s1.name);
}
}
Output
0
Null
Classes
A class is a template/blueprint that outlines the behavior/state that objects of that kind support.
Individual objects are formed from a blueprint called a class.
Here's an example of a class.
Public class Dog {
String breed;
int age;
String color;
void barking() {
}
void hungry() {
}
void sleeping() {
}
}
Any of the following variable kinds can be found in a class.
Local variables – Local variables are variables specified within methods, constructors, or blocks. The variable will be declared and initialized within the method, and then removed once the method is finished.
Instance variables – Instance variables are variables that exist within a class but are not associated with any methods. When the class is created, these variables are set to their default values. Instance variables can be accessed from within any of the class's methods, constructors, or blocks.
Class variables – Class variables are variables declared with the static keyword within a class, outside of any methods.
A class can have as many methods as it wants to retrieve the value of different types of methods. Barking(), hungry(), and sleeping() are methods in the sample above.
- Abstraction is a feature of OOPs.
- The feature allows us to hide the implementation detail from the user and shows only the functionality of the programming to the user. Because the user is not interested to know the implementation.
- It is also safe from the security point of view.
- The best example of abstraction is a car.
- Eg:- When we derive a car, we do not know how is the car moving or how internal components are working? But we know how to derive a car.
- It means it is not necessary to know how the car is working, but it is important how to derive a car. The same is an abstraction.
- The same principle (as we have explained in the above example) also applied in Java programming
- In the language of programming, the code implementation is hidden from the user and only the necessary functionality is shown or provided to the user. We can achieve abstraction in two ways:
- Using Abstract Class
- Using Interface
Encapsulation in Java represents a way of hiding the data (variables) and the methods as a single unit.
In encapsulation, the variables of a class will not be visible to other classes; they can only be accessed by the methods of their own class.
Data encapsulation sometimes is also known as data hiding.
To implement the concept of data encapsulation, declare all the variables of the class as private and declare methods as public to set and get the values of variable.
Example
Public class Demoencap {
Private String name;
Private int age;
Public int getAge() {
Return age;
}
Public String getName() {
Return name;
}
Public void setAge( int newAge) {
Age = newAge;
}
Public void setName(String newName) {
Name = newName;
}
}
Public class Usedemo{
Public static void main(String args[]) {
Demoencap cap = new Demoencap();
Cap.setName("Riya");
Cap.setAge(15);
System.out.print("Student Name : " + cap.getName() + “/n” + " Student Age : " + cap.getAge());
}
}
In the above example get() and set() methods are used to access the variables of the class. These methods are referred to as getter and setters.
Hence, if any class want to access their variable they have to use getter and setter for the same purpose.
The output of above program is:
Output
Student Name: Riya
Student Age: 15
Advantages of Encapsulation
- The members of a class can be declared as read-only or write-only.
- A class have complete control on the data stored inside its variables.
Inheritance is one of the main principles of OOP language. By using this principle one can inherit all the properties of other class. Inheritance allows us to manage information in a hierarchal manner.
Inheritance use two types of class: Superclass and subclass. Superclass also known as parent or base class is the class whose properties are inherited while subclass also known as derived or child class is the class who inherits the properties.
To inherit a class we simply use the extend keyword which allows to inherit the definition of class to another as shown below:
Class subclass-name extends superclass-name {
// body of class
}
Lets look at a simple example of inheritance:
Class First {// Superclass.
Int x, y;
Void showxy() {
System.out.println("x and y: " + x + " " + y);
}
}
Class Second extends First {// Subclass Second by extending class First.
Int z;
Void showz() {
System.out.println("z: " + z);
}
Void sum() {
System.out.println("x+y+z: " + (x+y+z));
}
}
Class Example {
Public static void main(String args[]) {
First superOb = new First();
Second subOb = new Second();
SuperOb.x = 100;
SuperOb.y = 200;
System.out.println("Data of superclass: ");
SuperOb.showxy();
System.out.println();
/* The subclass can access all public members of its superclass. */
SubOb.x = 45;
SubOb.y = 10;
SubOb.z = 5;
System.out.println("Contents of subOb: ");
SubOb.showxy();
SubOb.showz();
System.out.println();
System.out.println("Sum of i, j and k in subOb:");
SubOb.sum();
}
}
Output
Data of superclass:
x and y: 100 200
Contents of subOb:
x and y: 45 10
z: 5
Sum of x, y and z in subOb:
x+y+z: 60
Polymorphism can be defined as the ability to take different forms. It occurs when inheritance is used to have many classes that are related to each other.
Polymorphism most commonly used when a parent class reference is made to refer to a child class object.
Being one of the most important features of OOP, it must be supported by all languages based on OOP. Polymorphism in Java gives us the flexibility to use all the inherited properties of superclass to perform a task.
In real life, the simplest example to explain the concept of polymorphism is a person class. A single person can have different relationship with other person, like a mother, a daughter, a sister, a friend, an employee etc.
To better understand the concept of polymorphism we have to take a look at an example.
Consider a superclass named “Shapes” having a method name area(). The subclasses of this superclass can be Square, Rectangle, Triangle or any other shape. Using inheritance the subclass inherits the area() method also, but each shape has its own way of calculating area. Hence we can see that method name is same but it is used in different forms.
Class Shapes {
public void area() {
System.out.println("Area of shape can be calculated as: \n");
}
}
Class Square extends Shapes {
public void area() {
System.out.println("Area of Square is side * side ");
}
}
Class Rectangle extends Shapes {
public void area() {
System.out.println("Area of Rectangle is base * height ");
}
}
Class Main {
public static void main(String[] args) {
Shapes myShape = new Shapes(); // Create a Shapes object
Shapes mySquare = new Square(); // Create a Triangle object
Shapes myRectangle = new Rectangle(); // Create a Circle object
myShape.area();
mySquare.area();
myRectangle.area();
}
}
Output
Area of shape can be calculated as:
Area of Square is side * side
Area of Rectangle is base * height
Java is a platform as well as a programming language. Java is a high-level programming language that is also robust, object-oriented, and secure.
Java was created in 1995 by Sun Microsystems (which is now a division of Oracle). The founder of Java, James Gosling, is recognized as the "Father of Java." It was known as Oak before Java. Because Oak was already a recognized business, James Gosling and his team decided to alter the name to Java.
Platform - A platform is any hardware or software environment in which a program runs. Java is referred to as a platform because it has a runtime environment (JRE) and API.
Features of Java
● Object Oriented: Java is a complete object oriented language. This means that everything in Java revolves around the use of Objects.
● Secure: Java is a very secure language; one of the main reasons is the lack of pointers. Virus free software’s can be developed using Java programming constructs.
● Robust: Java is a very robust language because of features like automatic garbage collection, memory management, exception handling, etc.
● Portable: One of the very important features of Java is that can be used on any platform without any problems. This means that it allows you to run the Java Bytecode on any platform.
● Simple: Java is a simple language because of its ease of use and less complicated features.
● Platform Independent: Java is a ‘write’ once, ‘run’ anywhere language, which means that once written, it has the capability to be executed on any machine that has Java Runtime Environment (JRE).
Compiling the program
Compiling a Java program is easy. Given are the steps to compile and run a program.
Step 1: To compile a Java program you need to open command prompt by following steps start menu -> All Programs -> Accessories -> command prompt.
Step 2: Next set the directory path where you saved your Java program. Let us consider my current directory is c:\dis. Following needs to be done to change the directory.
C:\> cd dis (press enter key)
Step 3: Compile the program by running following command.
C:\dis> javac FirstProgram.java (press enter key)
This step will inform the compiler to compile the program. The compiler will look for any error, if there is no error it will tell you to perform next step.
After successful compilation the system will generate a FirstProgram.class file in the same directory.
How Java program compiles?
After successful compilation, the Java compiler will convert the java source code into machine independent code known as bytecode.
This bytecode file will be stored with the extension .class in the same directory where your program is saved.
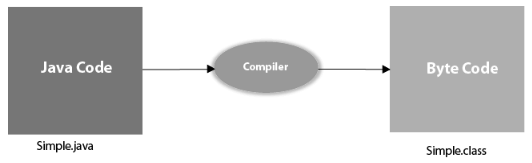
First Java Program
Java is an object oriented programming language, so the complete program must be written inside a class.
Let’s consider an example
Class FirstProgram
{
Public static void main(String[] args)
{
System.out.println(“My first program”);
}
}
Save the above file as FirstProgram.java
Prerequisites Before start writing a java program
Before writing java program one must make sure that following software or applications are properly installed.
- First of all, download JDK and install it.
- Set path of the jdk/bin directory.
- Create the Java program.
- Compile and run the program.
Besides this learning Java is easy doesn’t require any previous knowledge of other programming language but required some logics for writing programs.
The syntax of writing a Java program is similar to that of C, so if you know C language little bit then it will be easy for you to write Java Program.
Also, if you have any idea of C++ then it will easy to understand the concept of object oriented programming which in turn helps to understand Java better.
Writing the program
To write a program, first of all open the notepad by following the sequence start menu -> All Programs -> Accessories -> Notepad and then write the below code.
Class FirstProgram
{
Public static void main(String[] args)
{
System.out.println(“My first program”);
}
}
After writing the code save the file as FirstProgram.java.
Different ways to write a Java program
- Sequence of the modifiers doesn’t matter, prototype of method will be same.
Static public void main(String args[])
2. The string array can be written in many ways all have the same meaning.
Public static void main(String[] args)
Public static void main(String []args)
Public static void main(String args[])
3. By using three ellipses we can provide var-args to the main().
Public static void main(String...args)
4. Placing a semicolon at the end of class is not necessary its optional.
Class FirstProgram
{
Static public void main(String...args)
{
System.out.println(“My first program”);
}
};
Executing the program
Now to run the program as shown below
C:\dis> java FirstProgram
You will be able to see the desired output on the command prompt.
How Java program executes?
The compiler will generate the class files which are machine independent or OS, which allows them to execute or run on any system.
At the time of execution, the main class file is passed to JVM (Java Virtual Machine) which the goes through following stages:
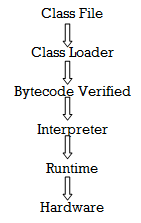
● Class loader: It is a sub part of JVM which is used to load class files.
● Bytecode Verifier: It checks part of code which contain access verifier to see any illegal access to the code.
● Interpreter: It read the Bytecode in stream and then run the instructions.
JVM (Java Virtual Machine) is a program that gives us the flexibility to execute Java programs. It provides a runtime environment to execute the Java code or applications.
This is the reason why Java is said to platform independent language as it requires JVM on the system it runs its Java code.
JVM is also used to execute programs of other languages that are compiled to Bytecode.
JVM can also be describe as:
- A specification where JVM working is pre defined.
- An implementation like JRE (Java Runtime Environment) is required.
- Runtime Instance means wherever a program is run on command prompt using java command, an instance of JVM is created.
Significance of JVM
JVM is used for two primary functions
- It allows Java programs to use the concept of “Write Once and Run Anywhere”.
- It is utilized to manage and optimize memory usage for programs.
Architecture of JVM
Java applications require a run-time engine which is provided by JVM. The main method of Java program is called by JVM. JVM is a subsystem of JRE (Java Runtime Environment).
Let’s see the internal architecture of JVM as shown in the figure below:
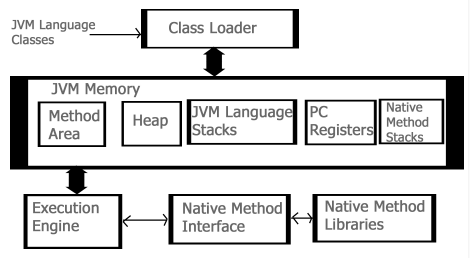
Fig: JVM architecture
- Class Loader: This part is used to load class files. There are three major functions of class loader as Loading, Linking and Initialization.
- Method Area: Structure of class like metadata, the constant runtime pool, and the code for methods are stored method area.
- Heap: Heap is the memory where all the objects, instance variables and arrays are stored. This is a shared memory which is accessed by multiple threads.
- JVM Language Stacks: These hold the local data variables and their results. Each thread has their own JVM stack used to store data.
- PC Registers: The currently running IVM instructions are stored in PC Registers. In Java, every thread has its their own PC register.
- Native Method Stacks: It hold the instruction of native code depending on their native library.
- Execution Engine: This engine is used to test the software, hardware or complete system. It doesn’t contain any information about the tested product.
- Native Method Interface: It is a programming interface. It allows Java code to call by libraries and native applications.
- Native Method Libraries: It consists of native libraries which are required by execution engine.
Constructor
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Properties of a constructor:
1. Name of the constructor is the same as its class name.
2. A default constructor is always present in a class implicitly.
3. There are three main types of constructors namely default, parameterized and copy constructors.
4. A constructor in Java can not be abstract, final, static and Synchronized.
Public class NewClass{
//This is the constructor
NewClass(){
}}
The new keyword here creates the object of class NewClass and invokes the constructor to initialize this newly created object.
Default constructor:
A default constructor is a constructor that is created implicitly by the JVM.
Program to demonstrate default constructors:
Class student
{
Int id;
String name;
Student()
{
System.out.println("Student id is 20 and name is Amruta");
}
Public static void main(String args[])
{
Student s = new student();
}
}
Parameterized constructor:
It is a constructor that has to be created explicitly by the programmer. This constructor has parameters that can be passed when a constructor is called at the time of object creation.
Program:
Class Student4
{
Int id;
String name;
Student4(int i,String n)
{
Id = i;
Name = n;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student4 s1 = new Student4(20,"Rema");
Student4 s2 = new Student4(23,"Sadhna");
s1.display();
s2.display();
}
}
Copy constructor:
A content of one constructor can be copied into another constructor using the objects created. Such a constructor is called as a copy constructor.
Program:
Class Student6
{
Int id;
String name;
Student6(int i,String n)
{
Id = i;
Name = n;
}
Student6(Student6 s)
{
Id = s.id;
Name =s.name;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student6 s1 = new Student6(20,"AMRUTA");
Student6 s2 = new Student6(s1);
s1.display();
s2.display();
}
}
Output:
They are used to control the visibility of members like classes, variables and methods.
- Public: Accessible to other classes defined in the same package as well as those defined in other packages.
- Default: Accessible to other classes in the same package but will be inaccessible to classes outside the package.
- Private: Access is restricted to class only.
- Protected: Accessed only by the subclasses in other package or any class within the package of the class of the protected member
Access specifiers are used for the following:
- Access specifiers for classes
- Access specifiers for instance variables
- Access specifiers for methods
- Access specifiers for constructors
1. Access specifiers for classes
A class can be declared public. Private access specifier cannot be applied to a class, and it’s the same for protected. If there is no access specifier mentioned, default access restrictions will be applied.
2. Access specifiers for Instance variables
All four specifiers- public, private, default and protected will be applicable for instance variables. Public variable can be modified from all classes, in or outside the package. Variables are accessible from classes in the same package only is access specifier is not stated. For private variables, access is restricted to class.
3. Access specifiers for methods
All four specifiers- public, private, default and protected will be applicable for methods as well.
4. Access specifiers for constructors
All four specifiers may be applied to the constructor as well. For a public declare constructor, an object can be created for any other class. If no access specifier mentioned, the object could be created from any class inside the same package. For private access specifiers, object maybe created in that class only.
In Java, the static keyword is mostly used for memory management. With variables, methods, blocks, and nested classes, we can use the static keyword. The static keyword refers to a class rather than a specific instance of that class.
The static can take the form of:
- Variable (also known as a class variable)
- Method (also known as a class method)
- Block
- Nested class
Static variable
A static variable is defined as a variable that has been declared as static.
The static variable can be used to refer to a property that is shared by all objects (but not unique to each object), such as an employee's firm name or a student's college name.
The static variable is only stored in memory once in the class area, when the class is loaded.
Example of static variable
//Java Program to demonstrate the use of static variable
Class Student{
Int rollno;//instance variable
String name;
Static String college ="ITS";//static variable
//constructor
Student(int r, String n){
Rollno = r;
Name = n;
}
//method to display the values
Void display (){System.out.println(rollno+" "+name+" "+college);}
}
//Test class to show the values of objects
Public class TestStaticVariable1{
Public static void main(String args[]){
Student s1 = new Student(111,"Karan");
Student s2 = new Student(222,"Aryan");
//we can change the college of all objects by the single line of code
//Student.college="BBDIT";
s1.display();
s2.display();
}
}
Output
111 Karan ITS
222 Aryan ITS
Static method
Any method that uses the static keyword is referred to as a static method.
- A class's static method, rather than the class's object, belongs to the class.
- A static method can be called without having to create a class instance.
- A static method can access and update the value of a static data member.
Example:
//Java Program to demonstrate the use of a static method.
Class Student{
Int rollno;
String name;
Static String college = "ITS";
//static method to change the value of static variable
Static void change(){
College = "BBDIT";
}
//constructor to initialize the variable
Student(int r, String n){
Rollno = r;
Name = n;
}
//method to display values
Void display(){System.out.println(rollno+" "+name+" "+college);}
}
//Test class to create and display the values of object
Public class TestStaticMethod{
Public static void main(String args[]){
Student.change();//calling change method
//creating objects
Student s1 = new Student(111,"Karan");
Student s2 = new Student(222,"Aryan");
Student s3 = new Student(333,"Sonoo");
//calling display method
s1.display();
s2.display();
s3.display();
}
}
Output
111 Karan BBDIT
222 Aryan BBDIT
333 Sonoo BBDIT
Key takeaway
In Java, the static keyword is mostly used for memory management.
With variables, methods, blocks, and nested classes, we can use the static keyword.
The static keyword refers to a class rather than a specific instance of that class.
The different sizes and values that can be stored in the variable are defined by data types. In Java, there are two types of data types:
● Primitive data types - Boolean, char, byte, short, int, long, float, and double are examples of primitive data types.
● Non - primitives data types - Classes, Interfaces, and Arrays are examples of non-primitive data types.
Primitive data types
Primitive data types are the building blocks of data manipulation in the Java programming language. These are the most basic data types in the Java programming language.
Let's take a closer look at each of the eight primitive data types.
Boolean
Only two potential values are stored in the Boolean data type: true and false. Simple flags that track true/false circumstances are stored in this data type.
The Boolean data type specifies a single bit of data, but its "size" cannot be precisely defined.
Byte
The primitive data type byte is an example of this. It's an 8-bit two-s complement signed integer. It has a value range of -128 to 127. (inclusive). It has a minimum of -128 and a high of 127. It has a value of 0 by default.
The byte data type is used to preserve memory in huge arrays where space is at a premium. Because a byte is four times smaller than an integer, it saves space. It can also be used in place of "int" data type.
Short
A 16-bit signed two's complement integer is the short data type. It has a value range of -32,768 to 32,767. (inclusive). It has a minimum of -32,768 and a maximum of 32,767. It has a value of 0 by default.
The short data type, like the byte data type, can be used to save memory. A short data type is twice the size of an integer.
Int
A 32-bit signed two's complement integer is represented by the int data type. Its range of values is - 2,147,483,648 (-231 -1) to 2,147,483,647 (231 -1). (inclusive). It has a minimum of 2,147,483,648 and a maximum of 2,147,483,647. It has a value of 0 by default.
Unless there is a memory constraint, the int data type is usually chosen as the default data type for integral values.
Long
A 64-bit two's complement integer is the long data type. It has a value range of -9,223,372,036,854,775,808(-263 -1) to 9,223,372,036,854,775,807(263 -1). (inclusive).
Its lowest and maximum values are 9,223,372,036,854,775,808 and 9,223,372,036,854,775,807. It has a value of 0 by default. When you need a larger range of values than int can supply, you should utilize the long data type.
Float
The float data type is a 32-bit IEEE 754 floating point with single precision. It has an infinite value range. If you need to preserve memory in big arrays of floating point integers, use a float (rather than a double). For precise numbers, such as currency, the float data type should never be used. 0.0F is the default value.
Double
A double data type is a 64-bit IEEE 754 floating point with double precision. It has an infinite value range. Like float, the double data type is commonly used for decimal values. For precise values, such as currency, the double data type should never be utilized. 0.0d is the default value.
Key takeaway
The different sizes and values that can be stored in the variable are defined by data types.
References:
1. Sun Certified Java Programmer for Java 6 by Kathy Sierra.
2. The Java TM Programming Language (3rd Edition) by Arnold, Holmes, Gosling, Goteti.
3. Core Java for Beginners by Rashmi Kanta Das(III Edition) Vikas Publication.
4. Java A Beginner's Guide, Fifth Edition, Tata McGra.