Unit - 2
Operators
Java has a large number of operators for manipulating variables. All Java operators can be divided into the following categories:
● Arithmetic Operators
● Relational Operators
● Bitwise Operators
● Logical Operators
● Assignment Operators
● Misc Operators
Arithmetic operators
In the same way as algebraic operators are used in mathematical expressions, arithmetic operators are employed in mathematical expressions. The arithmetic operators are listed in the table below.
Assume integer variable A has a value of 10 and variable B has a value of 20.
Operator | Description | Example |
+ (Addition) | Values are added on both sides of the operator. | A + B will give 30 |
- (Subtraction) | The right-hand operand is subtracted from the left-hand operand. | A - B will give -10 |
* (Multiplication) | Values on both sides of the operator are multiplied. | A * B will give 200 |
/ (Division) | Right-hand operand is divided by left-hand operand. | B / A will give 2 |
% (Modulus) | Returns the remainder after dividing the left-hand operand by the right-hand operand. | B % A will give 0 |
++ (Increment) | Increases the operand's value by one. | B++ gives 21 |
-- (Decrement) | Reduces the operand's value by one.. | B-- gives 19 |
Relational Operators
The Java language supports the following relational operators.
Assume variable A has a value of 10 and variable B has a value of 20.
Operator | Description | Example |
== (equal to) | Checks whether the values of two operands are equal, and if they are, the condition is true. | (A == B) is not true. |
!= (not equal to) | Checks whether the values of two operands are equivalent; if they aren't, the condition is true. | (A != B) is true. |
> (greater than) | If the left operand's value is greater than the right operand's value, then the condition is true. | (A > B) is not true. |
< (less than) | Checks if the left operand's value is less than the right operand's value; if it is, the condition is true. | (A < B) is true. |
>= (greater than or equal to) | If the left operand's value is larger than or equal to the right operand's value, then the condition is true. | (A >= B) is not true. |
<= (less than or equal to) | If the left operand's value is less than or equal to the right operand's value, then the condition is true. | (A <= B) is true. |
Bitwise operators
Long, int, short, char, and byte are all integer types that can be used with Java's bitwise operators.
The bitwise operator works with bits and performs operations bit by bit. Assume a = 60 and b = 13; in binary format, they will look like this:
a = 0011 1100
b = 0000 1101
a&b = 0000 1100
a|b = 0011 1101
a^b = 0011 0001
~a = 1100 0011
Assume integer variable A has a value of 60 and variable B has a value of 13.
Operator | Description | Example |
& (bitwise and) | If a bit exists in both operands, the binary AND operator duplicates it to the result. | (A & B) will give 12 which is 0000 1100 |
| (bitwise or) | If a bit exists in both operands, the binary OR operator copies it. | (A | B) will give 61 which is 0011 1101 |
^ (bitwise XOR) | If a bit is set in one operand but not both, the binary XOR operator replicates it. | (A ^ B) will give 49 which is 0011 0001 |
~ (bitwise compliment) | The Binary Ones Complement Operator is a unary operator that 'flips' bits. | (~A ) will give -61 which is 1100 0011 in 2's complement form due to a signed binary number. |
<< (left shift) | Left Shift Operator in Binary. The value of the left operand is shifted to the left by the number of bits given by the right operand. | A << 2 will give 240 which is 1111 0000 |
>> (right shift) | Right Shift Operator in Binary. The value of the left operand is advanced by the number of bits supplied by the right operand. | A >> 2 will give 15 which is 1111 |
>>> (zero fill right shift) | Zero fill operator with a right shift. The value of the left operand is shifted right by the number of bits supplied in the right operand, and the shifted values are filled with zeros. | A >>>2 will give 15 which is 0000 1111 |
Logical operators
The logical operators are listed in the table below.
Assume that Boolean variable A is true and variable B is false.
Operator | Description | Example |
&& (logical and) | The logical AND operator is what it's called. If both operands are non-zero, the condition is satisfied. | (A && B) is false |
|| (logical or) | The logical OR operator is what it's called. The condition becomes true if any of the two operands is non-zero. | (A || B) is true |
! (logical not) | It's known as the Logical NOT Operator. Its operand's logical state is reversed when it is used. If one of the conditions is true, the Logical NOT operator returns false. | !(A && B) is true |
Assignment operators
The assignment operators supported by the Java language are listed below.
Operator | Description | Example |
= | This is a straightforward assignment operator. Values from the right side operands are assigned to the left side operand. | C = A + B will assign value of A + B into C |
+= | The AND assignment operator is used to combine two variables. It adds the right and left operands together and assigns the result to the left operand. | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator. It subtracts right operand from the left operand and assign the result to left operand. | C -= A is equivalent to C = C – A |
*= | The AND operator multiplies and assigns. It adds the right and left operands together and assigns the result to the left operand. | C *= A is equivalent to C = C * A |
/= | The AND operator divides and assigns. It multiplies the left and right operands and assigns the result to the left operand. | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator. It takes modulus using two operands and assign the result to left operand. | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator. | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator. | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator. | C &= 2 is same as C = C & 2 |
^= | Bitwise exclusive OR and assignment operator. | C ^= 2 is same as C = C ^ 2 |
|= | Bitwise inclusive OR and assignment operator. | C |= 2 is same as C = C | 2 |
Miscellaneous Operators
Only a few more operators are supported by the Java programming language.
Conditional Operator ( ? : )
The ternary operator is another name for the conditional operator. This operator is used to evaluate Boolean expressions and has three operands. The operator's objective is to determine which value should be assigned to a variable. The operator is denoted by the symbol.
Variable x = (expression) ? value if true : value if false
Instance of Operator
Only object reference variables are used with this operator. The operator determines whether or not the item is of a specific type (class type or interface type).
The instance of operator is denoted by the symbol.
(Object reference variable) instance of (class/interface type)
The result will be true if the object referred to by the variable on the left side of the operator passes the IS-A check for the class/interface type on the right side.
Key takeaway
The order in which terms in an expression are grouped is determined by operator precedence. This has an impact on how an expression is judged.
The highest-priority operators appear at the top of the table, while the lowest-priority operators appear at the bottom.
Java provides us three types of control statements:
● Conditional statements: These are used when we want to choose among two or more paths. Conditional statements are of three types in java: if/else/else if, ternary operator and switch.
● Loop/Iterators: Loops are used when we want to repeatedly run some part of the code. Java provides three types of loop: for, while and do while.
● Branching statements: These statements are used to alter the flow of control in loops. Branching statements are of two types: break and continue.
Conditional Statements
Conditional statements are used to choose the path for execution. These are of three types: if/else/else if, ternary and switch.
If statement
The if statement in Java is used to test the condition. If the condition is true, the if block is executed.
Syntax
If(condition){
//code to be executed
}
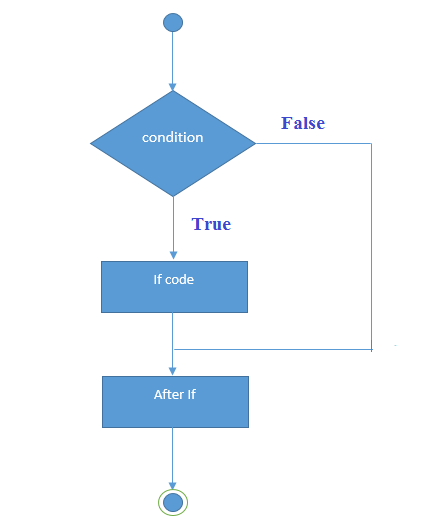
Fig: Flowchart of if statements
Example
//Java Program to demonstrate the use of if statement.
Public class IfExample {
Public static void main(String[] args) {
//defining an 'age' variable
int age=20;
//checking the age
if(age>18){
System.out.print("Age is greater than 18");
}
}
}
Output
Age is greater than 18
If-else statement
An extended version of if statement, which use a second block of code which is known as else block in the situation when if condition fails.
Syntax of if else statement:
If(Boolean condition)
{
Line 1;// it will execute only when if statement holds true
}
Else
{
Line 2;// it will execute only when if statement holds false
}
Example
Public class Program {
Public static void main(String[] args)
{
Int a = 5;
Int b = 15;
If(a+b>=20)
{
System.out.println(“if condition is true”);
}
Else
{
System.out.println(“if condition is false”);
}
}
}
Output:
If condition is true
If-else-if ladder or Nested if-else condition
In nested if-else condition first if condition is followed by many else-if conditions. In other way we can define it as a decision tree of if-else-if statement where we have to make multiple choices based on combination of conditions.
Syntax of nested if-else condition:
If(Boolean condition 1) {
Statement 1; //it will executes when condition 1 is true
}
Else if(Boolean condition 2) {
Statement 2; // it will executes when condition 2 is true
}
Else {
Statement 2; //it will executes when all the conditions are false
}
Example:
Public class Example {
Public static void main(String[] args) {
Int a = 15;
If(a < 10) {
System.out.println("a is less than 10");
}else if (a = 12) {
System.out.println("a is equal to 12");
}else if(a > 10) {
System.out.println("a is greater than 10");
}else {
System.out.println(a);
}
}
}
Output:
a is greater than 10
Ternary Condition
Ternary condition is an enhancement or shorthand to the if-else condition. The working of ternary and if-else condition is same.
Consider an example of if-else:
If (a > 2) {
System.out.println("a is higher than 2");
} else {
System.out.println("a is lower or equal to 2");
}
Now see the same code in ternary condition
System.out.println(a > 2 ? "a is higher than 2" : "a is lower or equal to 2");
Switch
The Java switch statement combines numerous conditions into a single statement. It's similar to an if-else-if conditional statement. The switch statement compares various values to see if they are equal.
● For a switch expression, there can be one or N case values.
● Only switch expressions can be used as case values. The case value must be constant or literal. Variables are not permitted.
● The case values must be one-of-a-kind. It causes a compile-time error if a value is duplicated.
● The byte, short, int, long (with its Wrapper type), enums, and string types must all be present in the Java switch statement.
● A break statement can be included in each case statement, although it is not required. The control jumps after the switch expression when it reaches the break statement. If no break statement is discovered, the next case is executed.
● Optionally, the case value can have a default label.
Syntax
Switch(expression){
Case value1:
//code to be executed;
Break; //optional
Case value2:
//code to be executed;
Break; //optional
......
Default:
code to be executed if all cases are not matched;
}
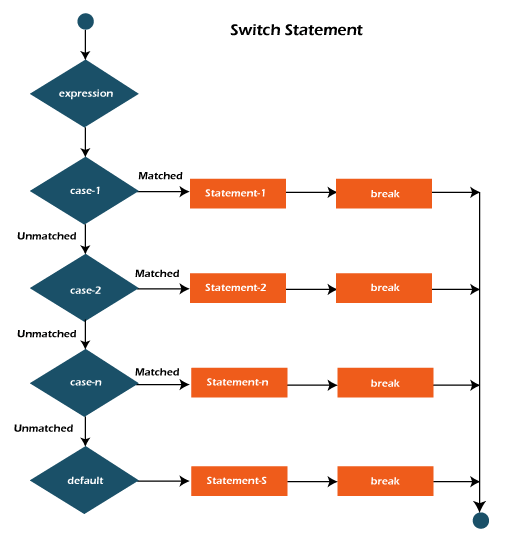
Fig: Flow chart
Example
Public class SwitchExample {
Public static void main(String[] args) {
//Declaring a variable for switch expression
int number=20;
//Switch expression
switch(number){
//Case statements
case 10: System.out.println("10");
break;
case 20: System.out.println("20");
break;
case 30: System.out.println("30");
break;
//Default case statement
default:System.out.println("Not in 10, 20 or 30");
}
}
}
Output
20
Statements
Break statements
When a break statement is reached within a loop, the loop is instantly ended, and program control is resumed at the next statement after the loop.
To break a loop or switch statement in Java, use the break statement. At the stated situation, it interrupts the program's current flow. It only breaks the inner loop in the case of the inner loop.
The Java break statement can be used in all forms of loops, including for loops, while loops, and do-while loops.
Syntax
Break;
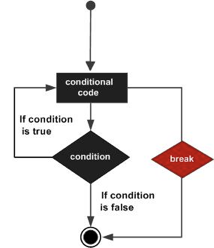
Fig: Flow diagram
Example
Public class Test {
public static void main(String args[]) {
int [] numbers = {10, 20, 30, 40, 50};
for(int x : numbers ) {
if( x == 30 ) {
break;
}
System.out.print( x );
System.out.print("\n");
}
}
}
Output
10
20
Continue statements
When you need to jump to the next iteration of the loop right away, you utilize the continue statement in the loop control structure. It can be used in conjunction with a for loop or a while loop.
The loop is continued using the Java continue command. It continues the program's current flow while skipping the remaining code at the specified circumstance. It only continues the inner loop in the case of an inner loop.
The continue statement in Java can be used in all forms of loops, including for loops, while loops, and do-while loops.
Syntax
Continue;
Fig: Flow diagram
Example
Public class Test {
public static void main(String args[]) {
int [] numbers = {10, 20, 30, 40, 50};
for(int x : numbers ) {
if( x == 30 ) {
continue;
}
System.out.print( x );
System.out.print("\n");
}
}
}
Output
10
20
40
50
While
The while loop in Java is used to execute a section of code until the provided Boolean condition is true. The loop comes to an end as soon as the Boolean condition is false.
The while loop is a type of if statement that repeats itself. The while loop is recommended if the number of iterations is not fixed.
Syntax
While(Boolean_expression) {
// Statements
}
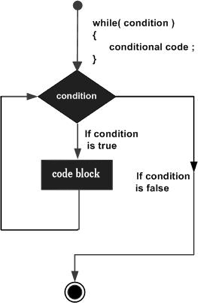
Fig: Flow diagram
The while loop's main feature is that it may or may not ever run. The loop body will be skipped and the first statement after the while loop will be executed when the expression is tested and the result is false.
Example
Public class Test {
public static void main(String args[]) {
int x = 10;
while( x < 20 ) {
System.out.print("value of x : " + x );
x++;
System.out.print("\n");
}
}
}
Output
Value of x : 10
Value of x : 11
Value of x : 12
Value of x : 13
Value of x : 14
Value of x : 15
Value of x : 16
Value of x : 17
Value of x : 18
Value of x : 19
Do While
The Java do-while loop is used to repeatedly iterate a section of a program until the specified condition is met. A do-while loop is recommended if the number of iterations is not fixed and the loop must be executed at least once.
An exit control loop is a Java do-while loop. In contrast to while and for loops, the do-while loop checks the condition at the end of the loop body. Because the condition is tested after the loop body, the Java do-while loop is executed at least once.
Syntax
Do{
//code to be executed / loop body
//update statement
}while (condition);
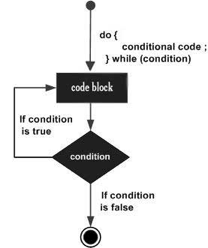
Fig: Flow chart
Example
Public class DoWhileExample {
Public static void main(String[] args) {
int i=1;
do{
System.out.println(i);
i++;
}while(i<=10);
}
}
Output
1
2
3
4
5
6
7
8
9
10
For
A for loop is a repetition control structure that allows you to design a loop that must be repeated a certain number of times quickly.
When you know how many times a task will be repeated, a for loop comes in handy.
● Initialize - When the loop begins, it executes the starting condition only once. We can either initialize the variable or use one that has already been initialized. It's a condition that can be turned off.
● Condition - It's the second condition that's run every time the loop's condition is tested. It keeps running until the condition is false. It must return either true or false as a boolean value. It's a condition that can be turned off.
● Increment / decrement - It increases or decreases the value of the variable. It's a condition that can be turned off.
● Statements - The loop's statement is performed every time the second condition is false.
Syntax
For(initialization; condition; increment/decrement){
//statement or code to be executed
}
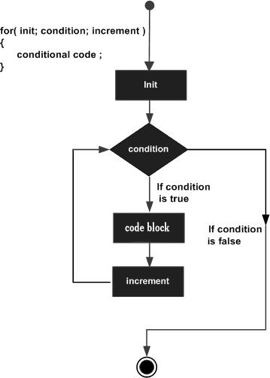
Fig: Flow diagram
Example
Public class Test {
public static void main(String args[]) {
for(int x = 10; x < 20; x = x + 1) {
System.out.print("value of x : " + x );
System.out.print("\n");
}
}
}
Output
Value of x : 10
Value of x : 11
Value of x : 12
Value of x : 13
Value of x : 14
Value of x : 15
Value of x : 16
Value of x : 17
Value of x : 18
Value of x : 19
Nested loops
The term "nested for loop" refers to a for loop that is contained within another loop. When the outer loop executes, the inner loop completes.
Example
Public class NestedForExample {
Public static void main(String[] args) {
//loop of i
For(int i=1;i<=3;i++){
//loop of j
For(int j=1;j<=3;j++){
System.out.println(i+" "+j);
}//end of i
}//end of j
}
}
Output
1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
In Java we use primitive data type which is nothing but simple data types like int or double. They are used to store the basic data types supported by the language.
To increase the performance primitive data types are the preferred choice rather than objects. If we use objects for these values it will always create an extra burden on the simplest calculations, that is why, primitive types are used.
Besides performance parameter, there are chances when object reference will be needed. For example, primitive types cannot make reference to methods we need objects.
To handle these, Java provides type wrappers classes. Wrapper classes are those classes which are used to encapsulate a primitive data type inside an object.
The use of wrapper class in Java is to convert primitive types into object and object into primitive type. They are needed because Java is designed on the concept of object.
Need of Wrapper classes
- To convert the primitive data type into objects.
- Java provides the package name java.util.package which consists of all classes used to handle wrapper class.
- There are such data structures like ArrayList and Vector which doesn’t store primitive type, they only store Objects. Here we need wrapper class.
- It helps in synchronization of multithreading.
Different predefined wrapper classes
In Java, the java.lang package consist of total eight wrapper classes. These classes are:
Primitive Type | Wrapper class |
Boolean | Boolean |
Char | Character |
Byte | Byte |
Short | Short |
Int | Integer |
Long | Long |
Float | Float |
Double | Double |
Example of wrapper class
Public class Wrapper Demo {
public static void main(String[] args) {
Integer myInt = 7;
Double myDouble = 8.1;
Character myChar = 'D';
System.out.println(myInt.intValue());
System.out.println(myDouble.doubleValue());
System.out.println(myChar.charValue());
}
}
Output
7
8.1
D
Predefined Constructors for the wrapper classes
In Java, there are two types of constructor available for wrapper class:
- In first constructor, the primitive data type is passed as an argument.
- In second constructor, string is passed as an argument.
Example of both the constructor is:
Public class Demo
{
public static void main(String[] args)
{
Byte b1 = new Byte((byte) 20); //Constructor which takes byte value as an argument
Byte b2 = new Byte("20"); //Constructor which takes String as an argument
Short s1 = new Short((short) 10); //Constructor which takes short value as an argument
Short s2 = new Short("5"); //Constructor which takes String as an argument
Integer i1 = new Integer(10); //Constructor which takes int value as an argument
Integer i2 = new Integer("10"); //Constructor which takes String as an argument
Long l1 = new Long(20); //Constructor which takes long value as an argument
Long l2 = new Long("20"); //Constructor which takes String as an argument
Float f1 = new Float(14.2f); //Constructor which takes float value as an argument
Float f2 = new Float("8.6"); //Constructor which takes String as an argument
Float f3 = new Float(8.6d); //Constructor which takes double value as an argument
Double d1 = new Double(7.8d); //Constructor which takes double value as an argument
Double d2 = new Double("7.8"); //Constructor which takes String as an argument
Boolean bl1 = new Boolean(true); //Constructor which takes boolean value as an argument
Boolean bl2 = new Boolean("false"); //Constructor which takes String as an argument
Character c1 = new Character('E'); //Constructor which takes char value as an argument
Character c2 = new Character("xyz"); //Compile time error : String abc can not be converted to character
}
}
Input through command line arguments are stored in the string format which further than converted into integer using parseInt method of the integer class. Same thing is done for float and other data type during execution.
In this input form we use args[]. Information is passed during the runtime of program.
Example
Class Demoinput {
public static void main(String[] args)
{
if (args.length > 0) {
System.out.println("The command line arguments are:");
// iterating the args array and printing the command line arguments
for (String val : args)
System.out.println(val);
}
else
System.out.println("No command line " + "arguments found.");
}
}
Output
The command line arguments are:
Good
Day
There can be a lot of usage of java this keyword. In java, this is a reference variable that refers to the current object.
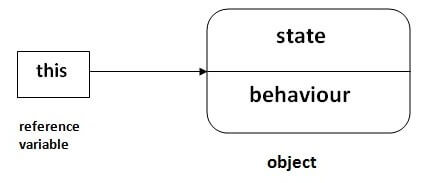
Fig – This keyword
Usage of java this keyword
Here is given the 6 usage of java this keyword.
- This can be used to refer current class instance variable.
- This can be used to invoke current class method (implicitly)
- This() can be used to invoke current class constructor.
- This can be passed as an argument in the method call.
- This can be passed as argument in the constructor call.
- This can be used to return the current class instance from the method.
Suggestion: If you are beginner to java, lookup only three usage of this keyword.
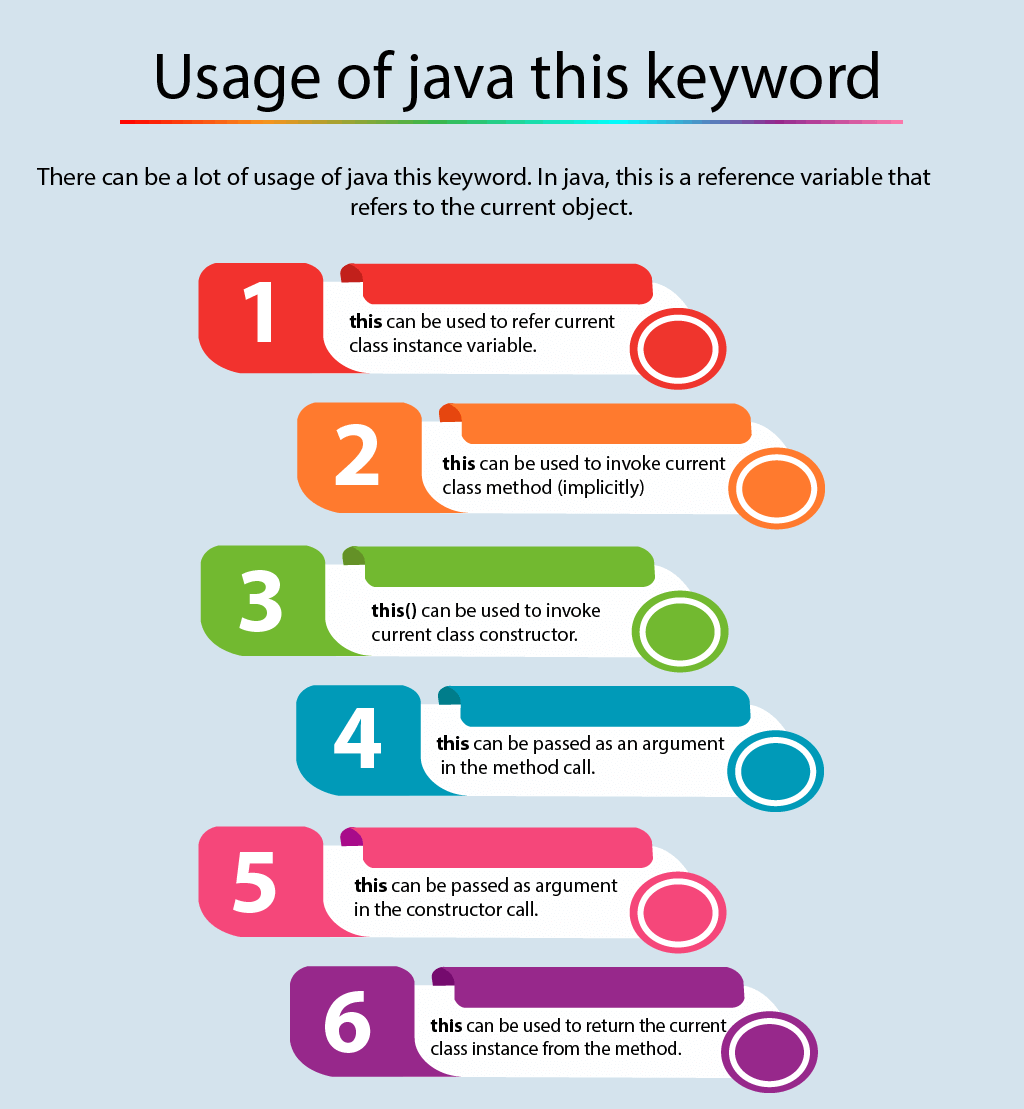
Fig – Usage
1) this: to refer current class instance variable
The this keyword can be used to refer current class instance variable. If there is ambiguity between the instance variables and parameters, this keyword resolves the problem of ambiguity.
Understanding the problem without this keyword
Let's understand the problem if we don't use this keyword by the example given below:
- Class Student{
- Int rollno;
- String name;
- Float fee;
- Student(int rollno,String name,float fee){
- Rollno=rollno;
- Name=name;
- Fee=fee;
- }
- Void display(){System.out.println(rollno+" "+name+" "+fee);}
- }
- Class TestThis1{
- Public static void main(String args[]){
- Student s1=new Student(111,"ankit",5000f);
- Student s2=new Student(112,"sumit",6000f);
- s1.display();
- s2.display();
- }}
Output:
0 null 0.0
0 null 0.0
In the above example, parameters (formal arguments) and instance variables are same. So, we are using this keyword to distinguish local variable and instance variable.
Solution of the above problem by this keyword
- Class Student{
- Int rollno;
- String name;
- Float fee;
- Student(int rollno,String name,float fee){
- This.rollno=rollno;
- This.name=name;
- This.fee=fee;
- }
- Void display(){System.out.println(rollno+" "+name+" "+fee);}
- }
- Class TestThis2{
- Public static void main(String args[]){
- Student s1=new Student(111,"ankit",5000f);
- Student s2=new Student(112,"sumit",6000f);
- s1.display();
- s2.display();
- }}
Output:
111 ankit 5000
112 sumit 6000
If local variables(formal arguments) and instance variables are different, there is no need to use this keyword like in the following program:
Program where this keyword is not required
- Class Student{
- Int rollno;
- String name;
- Float fee;
- Student(int r,String n,float f){
- Rollno=r;
- Name=n;
- Fee=f;
- }
- Void display(){System.out.println(rollno+" "+name+" "+fee);}
- }
- Class TestThis3{
- Public static void main(String args[]){
- Student s1=new Student(111,"ankit",5000f);
- Student s2=new Student(112,"sumit",6000f);
- s1.display();
- s2.display();
- }}
Output:
111 ankit 5000
112 sumit 6000
It is better approach to use meaningful names for variables. So we use same name for instance variables and parameters in real time, and always use this keyword.
2) this: to invoke current class method
You may invoke the method of the current class by using the this keyword. If you don't use the this keyword, compiler automatically adds this keyword while invoking the method. Let's see the example
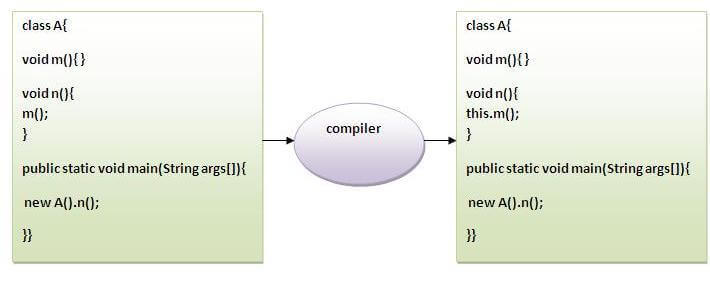
Fig – Example
- Class A{
- Void m(){System.out.println("hello m");}
- Void n(){
- System.out.println("hello n");
- //m();//same as this.m()
- This.m();
- }
- }
- Class TestThis4{
- Public static void main(String args[]){
- A a=new A();
- a.n();
- }}
Output:
Hello n
Hello m
3) this() : to invoke current class constructor
The this() constructor call can be used to invoke the current class constructor. It is used to reuse the constructor. In other words, it is used for constructor chaining.
Calling default constructor from parameterized constructor:
- Class A{
- A(){System.out.println("hello a");}
- A(int x){
- This();
- System.out.println(x);
- }
- }
- Class TestThis5{
- Public static void main(String args[]){
- A a=new A(10);
- }}
Output:
Hello a
10
Calling parameterized constructor from default constructor:
- Class A{
- A(){
- This(5);
- System.out.println("hello a");
- }
- A(int x){
- System.out.println(x);
- }
- }
- Class TestThis6{
- Public static void main(String args[]){
- A a=new A();
- }}
Output:
5
Hello a
Real usage of this() constructor call
The this() constructor call should be used to reuse the constructor from the constructor. It maintains the chain between the constructors i.e. it is used for constructor chaining. Let's see the example given below that displays the actual use of this keyword.
- Class Student{
- Int rollno;
- String name,course;
- Float fee;
- Student(int rollno,String name,String course){
- This.rollno=rollno;
- This.name=name;
- This.course=course;
- }
- Student(int rollno,String name,String course,float fee){
- This(rollno,name,course);//reusing constructor
- This.fee=fee;
- }
- Void display(){System.out.println(rollno+" "+name+" "+course+" "+fee);}
- }
- Class TestThis7{
- Public static void main(String args[]){
- Student s1=new Student(111,"ankit","java");
- Student s2=new Student(112,"sumit","java",6000f);
- s1.display();
- s2.display();
- }}
Output:
111 ankit java null
112 sumit java 6000
Rule: Call to this() must be the first statement in constructor.
- Class Student{
- Int rollno;
- String name,course;
- Float fee;
- Student(int rollno,String name,String course){
- This.rollno=rollno;
- This.name=name;
- This.course=course;
- }
- Student(int rollno,String name,String course,float fee){
- This.fee=fee;
- This(rollno,name,course);//C.T.Error
- }
- Void display(){System.out.println(rollno+" "+name+" "+course+" "+fee);}
- }
- Class TestThis8{
- Public static void main(String args[]){
- Student s1=new Student(111,"ankit","java");
- Student s2=new Student(112,"sumit","java",6000f);
- s1.display();
- s2.display();
- }}
Compile Time Error: Call to this must be first statement in constructor
4) this: to pass as an argument in the method
The this keyword can also be passed as an argument in the method. It is mainly used in the event handling. Let's see the example:
- Class S2{
- Void m(S2 obj){
- System.out.println("method is invoked");
- }
- Void p(){
- m(this);
- }
- Public static void main(String args[]){
- S2 s1 = new S2();
- s1.p();
- }
- }
Output:
Method is invoked
Application of this that can be passed as an argument:
In event handling (or) in a situation where we have to provide reference of a class to another one. It is used to reuse one object in many methods.
5) this: to pass as argument in the constructor call
We can pass the this keyword in the constructor also. It is useful if we have to use one object in multiple classes. Let's see the example:
- Class B{
- A4 obj;
- B(A4 obj){
- This.obj=obj;
- }
- Void display(){
- System.out.println(obj.data);//using data member of A4 class
- }
- }
- Class A4{
- Int data=10;
- A4(){
- B b=new B(this);
- b.display();
- }
- Public static void main(String args[]){
- A4 a=new A4();
- }
- }
Output:10
6) this keyword can be used to return current class instance
We can return this keyword as an statement from the method. In such case, return type of the method must be the class type (non-primitive). Let's see the example:
Syntax of this that can be returned as a statement
- Return_type method_name(){
- Return this;
- }
Example of this keyword that you return as a statement from the method
- Class A{
- A getA(){
- Return this;
- }
- Void msg(){System.out.println("Hello java");}
- }
- Class Test1{
- Public static void main(String args[]){
- New A().getA().msg();
- }
- }
Output:
Hello java
Proving this keyword
Let's prove that this keyword refers to the current class instance variable. In this program, we are printing the reference variable and this, output of both variables are same.
- Class A5{
- Void m(){
- System.out.println(this);//prints same reference ID
- }
- Public static void main(String args[]){
- A5 obj=new A5();
- System.out.println(obj);//prints the reference ID
- Obj.m();
- }
- }
Output:
A5@22b3ea59
A5@22b3ea59
Key takeaway
Here is given the 6 usage of java this keyword.
- This can be used to refer current class instance variable.
- This can be used to invoke current class method (implicitly)
- This() can be used to invoke current class constructor.
- This can be passed as an argument in the method call.
- This can be passed as argument in the constructor call.
- This can be used to return the current class instance from the method.
Suggestion: If you are beginner to java, lookup only three usage of this keyword.
In java, garbage means unreferenced objects.
Garbage Collection is process of reclaiming the runtime unused memory automatically. In other words, it is a way to destroy the unused objects.
To do so, we were using free() function in C language and delete() in C++. But, in java it is performed automatically. So, java provides better memory management.
Advantage of Garbage Collection
- It makes java memory efficient because garbage collector removes the unreferenced objects from heap memory.
- It is automatically done by the garbage collector(a part of JVM) so we don't need to make extra efforts.
How can an object be unreferenced?
There are many ways:
- By nulling the reference
- By assigning a reference to another
- By anonymous object etc.
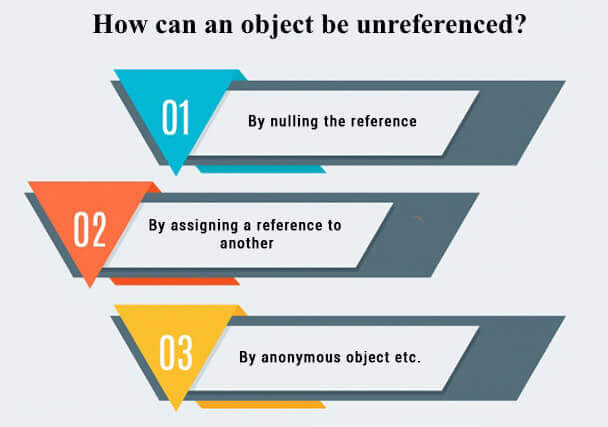
Fig – How can an object be unreferenced?
1) By nulling a reference:
- Employee e=new Employee();
- e=null;
2) By assigning a reference to another:
- Employee e1=new Employee();
- Employee e2=new Employee();
- e1=e2;//now the first object referred by e1 is available for garbage collection
3) By anonymous object:
- New Employee();
Finalize() method
The finalize() method is invoked each time before the object is garbage collected. This method can be used to perform cleanup processing. This method is defined in Object class as:
- Protected void finalize(){}
Note: The Garbage collector of JVM collects only those objects that are created by new keyword. So if you have created any object without new, you can use finalize method to perform cleanup processing (destroying remaining objects).
Gc() method
The gc() method is used to invoke the garbage collector to perform cleanup processing. The gc() is found in System and Runtime classes.
- Public static void gc(){}
Note: Garbage collection is performed by a daemon thread called Garbage Collector(GC). This thread calls the finalize() method before object is garbage collected.
Simple Example of garbage collection in java
- Public class TestGarbage1{
- Public void finalize(){System.out.println("object is garbage collected");}
- Public static void main(String args[]){
- TestGarbage1 s1=new TestGarbage1();
- TestGarbage1 s2=new TestGarbage1();
- s1=null;
- s2=null;
- System.gc();
- }
- }
object is garbage collected
object is garbage collected
Note: Neither finalization nor garbage collection is guaranteed.
Key takeaway
Garbage Collection is process of reclaiming the runtime unused memory automatically. In other words, it is a way to destroy the unused objects.
To do so, we were using free() function in C language and delete() in C++. But, in java it is performed automatically. So, java provides better memory management.
Normally, an array is a collection of similar type of elements which has contiguous memory location.
Java array is an object which contains elements of a similar data type. Additionally, The elements of an array are stored in a contiguous memory location. It is a data structure where we store similar elements. We can store only a fixed set of elements in a Java array.
Array in Java is index-based, the first element of the array is stored at the 0th index, 2nd element is stored on 1st index and so on.
Unlike C/C++, we can get the length of the array using the length member. In C/C++, we need to use the sizeof operator.
In Java, array is an object of a dynamically generated class. Java array inherits the Object class, and implements the Serializable as well as Cloneable interfaces. We can store primitive values or objects in an array in Java. Like C/C++, we can also create single dimentional or multidimentional arrays in Java.
Moreover, Java provides the feature of anonymous arrays which is not available in C/C++.
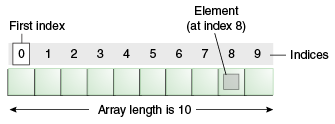
Fig – Example
Advantages
- Code Optimization: It makes the code optimized, we can retrieve or sort the data efficiently.
- Random access: We can get any data located at an index position.
Disadvantages
- Size Limit: We can store only the fixed size of elements in the array. It doesn't grow its size at runtime. To solve this problem, collection framework is used in Java which grows automatically.
Types of Array in java
There are two types of array.
- Single Dimensional Array
- Multidimensional Array
Single Dimensional Array in Java
Syntax to Declare an Array in Java
- DataType[] arr; (or)
- DataType []arr; (or)
- DataType arr[];
Instantiation of an Array in Java
ArrayRefVar=new datatype[size];
Example of Java Array
Let's see the simple example of java array, where we are going to declare, instantiate, initialize and traverse an array.
- //Java Program to illustrate how to declare, instantiate, initialize
- //and traverse the Java array.
- Class Testarray{
- Public static void main(String args[]){
- Int a[]=new int[5];//declaration and instantiation
- a[0]=10;//initialization
- a[1]=20;
- a[2]=70;
- a[3]=40;
- a[4]=50;
- //traversing array
- For(int i=0;i<a.length;i++)//length is the property of array
- System.out.println(a[i]);
- }}
Output:
10
20
70
40
50
Declaration, Instantiation and Initialization of Java Array
We can declare, instantiate and initialize the java array together by:
Int a[]={33,3,4,5};//declaration, instantiation and initialization
Let's see the simple example to print this array.
- //Java Program to illustrate the use of declaration, instantiation
- //and initialization of Java array in a single line
- Class Testarray1{
- Public static void main(String args[]){
- Int a[]={33,3,4,5};//declaration, instantiation and initialization
- //printing array
- For(int i=0;i<a.length;i++)//length is the property of array
- System.out.println(a[i]);
- }}
Output:
33
3
4
5
For-each Loop for Java Array
We can also print the Java array using for-each loop. The Java for-each loop prints the array elements one by one. It holds an array element in a variable, then executes the body of the loop.
The syntax of the for-each loop is given below:
- For(data_type variable:array){
- //body of the loop
- }
Let us see the example of print the elements of Java array using the for-each loop.
- //Java Program to print the array elements using for-each loop
- Class Testarray1{
- Public static void main(String args[]){
- Int arr[]={33,3,4,5};
- //printing array using for-each loop
- For(int i:arr)
- System.out.println(i);
- }}
Output:
33
3
4
5
Passing Array to a Method in Java
We can pass the java array to method so that we can reuse the same logic on any array.
Let's see the simple example to get the minimum number of an array using a method.
- //Java Program to demonstrate the way of passing an array
- //to method.
- Class Testarray2{
- //creating a method which receives an array as a parameter
- Static void min(int arr[]){
- Int min=arr[0];
- For(int i=1;i<arr.length;i++)
- If(min>arr[i])
- Min=arr[i];
- System.out.println(min);
- }
- Public static void main(String args[]){
- Int a[]={33,3,4,5};//declaring and initializing an array
- Min(a);//passing array to method
- }}
Output:
3
Anonymous Array in Java
Java supports the feature of an anonymous array, so you don't need to declare the array while passing an array to the method.
- //Java Program to demonstrate the way of passing an anonymous array
- //to method.
- Public class TestAnonymousArray{
- //creating a method which receives an array as a parameter
- Static void printArray(int arr[]){
- For(int i=0;i<arr.length;i++)
- System.out.println(arr[i]);
- }
- Public static void main(String args[]){
- PrintArray(new int[]{10,22,44,66});//passing anonymous array to method
- }}
Output:
10
22
44
66
Returning Array from the Method
We can also return an array from the method in Java.
- //Java Program to return an array from the method
- Class TestReturnArray{
- //creating method which returns an array
- Static int[] get(){
- Return new int[]{10,30,50,90,60};
- }
- Public static void main(String args[]){
- //calling method which returns an array
- Int arr[]=get();
- //printing the values of an array
- For(int i=0;i<arr.length;i++)
- System.out.println(arr[i]);
- }}
Output:
10
30
50
90
60
Array Index Out Of Bounds Exception
The Java Virtual Machine (JVM) throws an ArrayIndexOutOfBoundsException if length of the array in negative, equal to the array size or greater than the array size while traversing the array.
- //Java Program to demonstrate the case of
- //ArrayIndexOutOfBoundsException in a Java Array.
- Public class TestArrayException{
- Public static void main(String args[]){
- Int arr[]={50,60,70,80};
- For(int i=0;i<=arr.length;i++){
- System.out.println(arr[i]);
- }
- }}
Output:
Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 4
At TestArrayException.main(TestArrayException.java:5)
50
60
70
80
Multidimensional Array in Java
In such case, data is stored in row and column based index (also known as matrix form).
Syntax to Declare Multidimensional Array in Java
- DataType[][] arrayRefVar; (or)
- DataType [][]arrayRefVar; (or)
- DataType arrayRefVar[][]; (or)
- DataType []arrayRefVar[];
Example to instantiate Multidimensional Array in Java
Int[][] arr=new int[3][3];//3 row and 3 column
Example to initialize Multidimensional Array in Java
- Arr[0][0]=1;
- Arr[0][1]=2;
- Arr[0][2]=3;
- Arr[1][0]=4;
- Arr[1][1]=5;
- Arr[1][2]=6;
- Arr[2][0]=7;
- Arr[2][1]=8;
- Arr[2][2]=9;
Example of Multidimensional Java Array
Let's see the simple example to declare, instantiate, initialize and print the 2Dimensional array.
- //Java Program to illustrate the use of multidimensional array
- Class Testarray3{
- Public static void main(String args[]){
- //declaring and initializing 2D array
- Int arr[][]={{1,2,3},{2,4,5},{4,4,5}};
- //printing 2D array
- For(int i=0;i<3;i++){
- For(int j=0;j<3;j++){
- System.out.print(arr[i][j]+" ");
- }
- System.out.println();
- }
- }}
Output:
1 2 3
2 4 5
4 4 5
In Java, string is basically an object that represents sequence of char values. An array of characters works same as Java string. For example:
- Char[] ch={'j','a','v','a','t','p','o','i','n','t'};
- String s=new String(ch);
Is same as:
- String s="javatpoint";
Java String class provides a lot of methods to perform operations on strings such as compare(), concat(), equals(), split(), length(), replace(), compareTo(), intern(), substring() etc.
The java.lang.String class implements Serializable, Comparable and CharSequence interfaces.
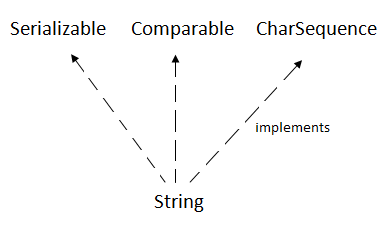
Fig – String
Char Sequence Interface
The Char Sequence interface is used to represent the sequence of characters. String, String Buffer and StringBuilder classes implement it. It means, we can create strings in java by using these three classes.
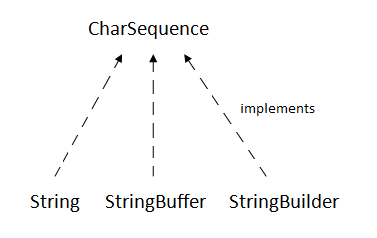
Fig – Char Sequence
The Java String is immutable which means it cannot be changed. Whenever we change any string, a new instance is created. For mutable strings, you can use String Buffer and StringBuilder classes.
We will discuss immutable string later. Let's first understand what is String in Java and how to create the String object.
What is String in java
Generally, String is a sequence of characters. But in Java, string is an object that represents a sequence of characters. The java.lang.String class is used to create a string object.
How to create a string object?
There are two ways to create String object:
- By string literal
- By new keyword
1) String Literal
Java String literal is created by using double quotes. For Example:
String s="welcome";
Each time you create a string literal, the JVM checks the "string constant pool" first. If the string already exists in the pool, a reference to the pooled instance is returned. If the string doesn't exist in the pool, a new string instance is created and placed in the pool. For example:
- String s1="Welcome";
- String s2="Welcome";//It doesn't create a new instance
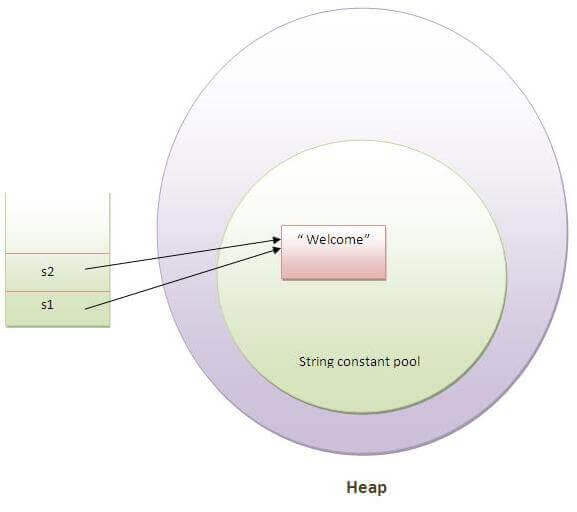
Fig – Example
In the above example, only one object will be created. Firstly, JVM will not find any string object with the value "Welcome" in string constant pool, that is why it will create a new object. After that it will find the string with the value "Welcome" in the pool, it will not create a new object but will return the reference to the same instance.
Note: String objects are stored in a special memory area known as the "string constant pool".
Why Java uses the concept of String literal?
To make Java more memory efficient (because no new objects are created if it exists already in the string constant pool).
2) By new keyword
String s=new String("Welcome");//creates two objects and one reference variable
In such case, JVM will create a new string object in normal (non-pool) heap memory, and the literal "Welcome" will be placed in the string constant pool. The variable s will refer to the object in a heap (non-pool).
Java String Example
- Public class StringExample{
- Public static void main(String args[]){
- String s1="java";//creating string by java string literal
- Char ch[]={'s','t','r','i','n','g','s'};
- String s2=new String(ch);//converting char array to string
- String s3=new String("example");//creating java string by new keyword
- System.out.println(s1);
- System.out.println(s2);
- System.out.println(s3);
- }}
Java
Strings
Example
Java String class methods
The java.lang.String class provides many useful methods to perform operations on sequence of char values.
No. | Method | Description |
1 | Char charAt(int index) | Returns char value for the particular index |
2 | Int length() | Returns string length |
3 | Static String format(String format, Object... Args) | Returns a formatted string. |
4 | Static String format(Locale l, String format, Object... Args) | Returns formatted string with given locale. |
5 | String substring(int beginIndex) | Returns substring for given begin index. |
6 | String substring(int beginIndex, int endIndex) | Returns substring for given begin index and end index. |
7 | Boolean contains(CharSequence s) | Returns true or false after matching the sequence of char value. |
8 | Static String join(CharSequence delimiter, CharSequence... Elements) | Returns a joined string. |
9 | Static String join(CharSequence delimiter, Iterable<? extends CharSequence> elements) | Returns a joined string. |
10 | Boolean equals(Object another) | Checks the equality of string with the given object. |
11 | Boolean isEmpty() | Checks if string is empty. |
12 | String concat(String str) | Concatenates the specified string. |
13 | String replace(char old, char new) | Replaces all occurrences of the specified char value. |
14 | String replace(CharSequence old, CharSequence new) | Replaces all occurrences of the specified CharSequence. |
15 | Static String equalsIgnoreCase(String another) | Compares another string. It doesn't check case. |
16 | String[] split(String regex) | Returns a split string matching regex. |
17 | String[] split(String regex, int limit) | Returns a split string matching regex and limit. |
18 | String intern() | Returns an interned string. |
19 | Int indexOf(int ch) | Returns the specified char value index. |
20 | Int indexOf(int ch, int fromIndex) | Returns the specified char value index starting with given index. |
21 | Int indexOf(String substring) | Returns the specified substring index. |
22 | Int indexOf(String substring, int fromIndex) | Returns the specified substring index starting with given index. |
23 | String toLowerCase() | Returns a string in lowercase. |
24 | String toLowerCase(Locale l) | Returns a string in lowercase using specified locale. |
25 | String toUpperCase() | Returns a string in uppercase. |
26 | String toUpperCase(Locale l) | Returns a string in uppercase using specified locale. |
27 | String trim() | Removes beginning and ending spaces of this string. |
28 | Static String valueOf(int value) | Converts given type into string. It is an overloaded method. |
Key takeaway
In Java, string is basically an object that represents sequence of char values. An array of characters works same as Java string. For example:
3. char[] ch={'j','a','v','a','t','p','o','i','n','t'};
4. String s=new String(ch);
Is same as:
2. String s="javatpoint";
Java String class provides a lot of methods to perform operations on strings such as compare(), concat(), equals(), split(), length(), replace(), compareTo(), intern(), substring() etc.
String Buffer
String Buffer class creates strings that can be modified in terms of length and content. This class can be used to insert characters and substrings in middle of string or append the string to the end. The objects of String Buffer class can be created by instantiating the String Buffer class.
Syntax:
StringBuffer stringName=new StringBuffer(“String”);
StringBuffer str=new StringBuffer(“Welcome students”);
Methods of String Buffer class
Method call | Task Performed |
StringBuffer.delete(int start, int end) | Removes the characters in a substring of this sequence |
StringBuffer.deleteCharAt(int index) | Removes the char at the specified position in this sequence |
StringBuffer.append(String str) | Appends the specified string to this character sequence |
IndexOf(String str) | Returns the index within this string of the first occurrence of the specified substring |
StringBuffer.reverse() | Reverses the string |
StringBuffer.replace(int start, int end, String str) | Replaces the string with the given string at the given start and end position |
Program to demonstrate use of String Buffer class
Class stringBuffer
{
Public static void main(String args[])
{
StringBuffer str = new StringBuffer("Welcome");
System.out.println("Original String is: "+str);
System.out.println(str.append(" JAVA"));
System.out.println(str.insert(8,"HI "));
System.out.println(str.delete(8,11));
System.out.println(str.replace(8,12,"USER"));
System.out.println(str.charAt(8));
System.out.println("The length of the string is: "+ str.length());
System.out.println(str.substring(8));
System.out.println(str.indexOf("S"));
System.out.println(str.reverse());
}
}
String Builder
In Java, String class are immutable i.e. no modification will be done in the succession of character. Therefore, StringBuilder came in use which creates a mutable or modifiable succession of characters.
The functionality of String Builder and String Buffer is almost same. Both of them helps in making mutable sequence of characters.
The StringBuilder class does not ensure synchronization while the String Buffer class does. Still StringBuilder class is the best option for working on a single thread, as it is much faster than String Buffer.
Class Declaration of StringBuilder
The class declaration is done using the java.lang.StringBuilder class which is a part of java.lang package.
Syntax is:
Public final class StringBuilder
Extends Object
Implements Serializable, CharSequence
Constructors in JavaStringBuilder
- StringBuilder(): Create a default string builder with no character in it having capacity of 16 characters.
- StringBuilder(int capacity): By using this we can create a string builder without characters in it and the capacity will be decided by the capacity argument.
- StringBuilder(CharSequence seq): It create a string builder having same characters as specified in CharSequence.
- StringBuilder(String str): It creates a string builder which initialized to the contents of the specified string.
Example of StringBuilder
Import java.util.*;
Import java.util.concurrent.LinkedBlockingQueue;
Public class Example {
public static void main(String[] args)
throws Exception
{
// StringBuilder object using StringBuilder constructor()
StringBuilder obj1 = new StringBuilder();
obj1.append("My First Program");
System.out.println("String 1 = " + obj1.toString());
// StringBuilder object using StringBuilder(CharSequence) constructor
StringBuilder obj2 = new StringBuilder("Hello");
System.out.println("String 2 = " + obj2.toString());
// StringBuilder object using StringBuilder(capacity) constructor
StringBuilder obj3 = new StringBuilder(12);
System.out.println("String 3 = " + obj3.capacity());
// StringBuilder object using StringBuilder(String) constructor
StringBuilder obj4 = new StringBuilder(obj2.toString());
System.out.println("String 4 = " + obj4.toString());
}
}
Output
String 1 = My First Program
String 2 = Hello
String 3 = 12
String 4 = Hello
Different StringBuilder Methods
- StringBuilder append(String a): Use to append the given string with the already present string.
- StringBuilder insert(int i, String a): Use to insert the string into existing string on a particular character position.
- StringBuilder replace(int start, int end, String a): Use to replace the existing string with the new string only from the start to end index provided.
- StringBuilder delete(int start, int end): Use to delete a particular length string from the existing string based on the start and end index.
- StringBuilder reverse(): Use to reverse the string.
- Int capacity(): Reflect the capacity of current StringBuilder.
- Void ensureCapacity(int min): Use to set a minimum value for the StringBuilder capacity.
- Char charAt(int index): Use to return the specified index character.
- Int length(): Return the total length of the string.
- String substring(int start): Return the substring staring from the given index till end.
- String substring(int start, int end): Return the substring between the start and the end index.
- Int indexOf(String a): Return the index of first instance of string.
- Int lastIndexOf(String a): Return the index where the string ends.
- Void trimToSize(): Reduce the size of the StringBuilder.
Example based on some of the methods given above
Public class Example{
Public static void main(String[] args){
StringBuilder s = new StringBuilder(“Hello ”);
s.append(“World”);
System.out.println(s);
s.insert(2,”World”);
System.out.println(s);
s.replace(2,4,”World”);
System.out.println(s);
s.delete(2,4);
System.out.println(s);
s.reverse();
System.out.println(s);
}
}
Output
Hello World
HeWorldllo
HeWorldo
Heo
OlleH
In Java APIs, the String class provides a variety of constructors.
The constructors of the String class that are most typically used are as follows:
● String()
● String(String str)
● String(char chars[ ])
● String(char chars[ ], int startIndex, int count)
● String(byte byteArr[ ])
● String(byte byteArr[ ], int startIndex, int count)
String() - We'll use the default constructor to make an empty String. The following is the usual syntax for creating an empty string in a Java program:
String s = new String();
It will generate a string object with no value in the memory region.
String(String str) - In the heap region, it will build a string object and store the specified value in it. The following is the standard syntax for creating a string object with a provided string str:
String st = new String(String str);
For example:
String s2 = new String("Hello Java");
Hello Java is included in the object str.
String(char chars[ ]) - The array of characters is stored in a string object created by this constructor. The following is the basic syntax for creating a string object with a provided array of characters:
String str = new String(char char[])
For example:
char chars[ ] = { 'a', 'b', 'c', 'd' };
String s3 = new String(chars);
The address of the value stored in the heap area is kept in the object reference variable s3.
String(char chars[ ], int startIndex, int count) - With a subrange of a character array, this constructor builds and initializes a string object.
The startIndex and count arguments specify the index at which the subrange begins and the number of characters to be copied, respectively.
The following is the standard syntax for creating a string object with the specified subrange of a character array:
String str = new String(char chars[ ], int startIndex, int count);
For example:
Char chars[ ] = { 'w', 'i', 'n', 'd', 'o', 'w', 's' };
String str = new String(chars, 2, 3);
Because the starting index is 2 and the total number of characters to be copied is 3, the object str contains the address of the value "ndo" stored in the heap region.
String(byte byteArr[ ]) - This constructor creates a new string object by decoding the given array of bytes (i.e., ASCII values into characters) using the system's default character set.
Example
Package stringPrograms;
Public class ByteArray
{
Public static void main(String[] args)
{
Byte b[] = { 97, 98, 99, 100 }; // Range of bytes: -128 to 127. These byte values will be converted into corresponding characters.
String s = new String(b);
System.out.println(s);
}
}
Output:
Abcd
String(byte byteArr[ ], int startIndex, int count) - The ASCII values are decoded using the system's default character set, and a new string object is created.
Example
Package stringPrograms;
Public class ByteArray
{
Public static void main(String[] args)
{
byte b[] = { 65, 66, 67, 68, 69, 70 }; // Range of bytes: -128 to 127.
String s = new String(b, 2, 4); // CDEF
System.out.println(s);
}
}
Output
CDEF
In Java, we can compare Strings based on their content and references.
It's utilized for things like authentication (using the equals() method), sorting (using the compareTo() method), and reference matching (using the == operator).
In Java, there are three techniques to compare strings:
● By Using equals() Method
● By Using == Operator
● By compareTo() Method
By using equals () methods
The equals() function of the String class compares the string's original content. It compares string values for equality. Two methods are available in the String class:
● public boolean equals(Object another) compares this string to the specified object.
● public boolean equalsIgnoreCase(String another) compares this string to another string, ignoring case.
Example
Class Teststringcomparison2{
Public static void main(String args[]){
String s1="Sachin";
String s2="SACHIN";
System.out.println(s1.equals(s2));//false
System.out.println(s1.equalsIgnoreCase(s2));//true
}
}
Output
False
True
The methods of the String class are used in the given program. If two String objects are equal and have the same case, the equals() function returns true. Regardless of the case of strings, equalsIgnoreCase() returns true.
By using == operators
The == operator compares references to each other rather than values.
Example
Class Teststringcomparison3{
Public static void main(String args[]){
String s1="Sachin";
String s2="Sachin";
String s3=new String("Sachin");
System.out.println(s1==s2);//true (because both refer to same instance)
System.out.println(s1==s3);//false(because s3 refers to instance created in nonpool)
}
}
Output
True
False
By Using compareTo() method
The compareTo() method of the String class compares two strings lexicographically and returns an integer value indicating whether the first string is less than, equal to, or greater than the second string.
Assume that s1 and s2 are two different String objects. If:
● s1 == s2: The method returns 0.
● s1 > s2: The method returns a positive value.
● s1 < s2: The method returns a negative value.
Example
Class Teststringcomparison4{
Public static void main(String args[]){
String s1="Sachin";
String s2="Sachin";
String s3="Ratan";
System.out.println(s1.compareTo(s2));//0
System.out.println(s1.compareTo(s3));//1(because s1>s3)
System.out.println(s3.compareTo(s1));//-1(because s3 < s1 )
}
}
Output
0
1
-1
Because String objects are immutable, modifying a String requires either copying it into a String Buffer or StringBuilder, or calling a String method that creates a new copy of the string with your changes applied.
Substring()
Substring can be used to extract a substring ( ). It comes in two varieties. The first is this:
String substring(int startIndex)
The index at which the substring will begin is specified by startIndex. This form returns a duplicate of the substring that starts at startIndex and ends at the invoking string's end.
The second form of substring( ) lets you to specify the substring's start and end indexes:
String substring(int startIndex, int endIndex)
The initial index is specified by startIndex, while the ending index is specified by endIndex. The string returned contains all of the characters from the start index to the finish index, but not including them.
Substring() is used in the following software to replace all instances of one substring with another within a string:
// Substring replacement.
Class StringReplace {
Public static void main(String args[]) {
String org = "This is a test. This is, too.";
String search = "is";
String sub = "was"; String result = ""; int i;
Do { // replace all matching substrings
System.out.println(org);
i = org.indexOf(search); if(i != -1) {
Result = org.substring(0, i); result = result + sub;
Result = result + org.substring(i + search.length()); org = result;
}
} while(i != -1);
}
}
Output
This is a test. This is, too.
Thwas is a test. This is, too.
Thwas was a test. This is, too.
Thwas was a test. Thwas is, too.
Thwas was a test. Thwas was, too.
Concat()
Concat() is used to join two strings together, as shown here: string concatenation (String str)
This method returns a new object with the invoking string appended to the end and the contents of str appended to the beginning. The function concat() performs is the same as +.
Example
String s1 = "one";
String s2 = s1.concat("two");
s2 is filled with the string "onetwo." It yields the same result as the sequence below:
String s1 = "one";
String s2 = s1 + "two";
Replace()
There are two versions of the replace() method. The first swaps out all instances of one character in the invoking string for another. Its general shape is as follows:
String replace(char original, char replacement)
The character to be replaced by the character given by replacement is specified by original. The returned string is the result of the operation. As an example,
String s = "Hello".replace('l', 'w');
s is filled with the string "Hewwo."
Replace(second )'s variant swaps out one character sequence for another. It takes the following general form:
String replace(CharSequence original, CharSequence replacement)
Trim()
The trim() method returns a copy of the invoking string that has been stripped of any leading and following whitespace. It takes the following general form:
Trim the strings ( ) Here's an illustration:
String s = " Hello World ".trim();
The string "Hello World" is now in s.
When processing user commands, the trim() technique comes in handy. For instance, the following application asks the user for the name of a state before displaying the capital of that state. Trim( ) is used to eliminate any preceding or trailing whitespace that the user may have entered inadvertently.
// Using trim() to process commands.
Import java.io.*;
Class UseTrim {
Public static void main(String args[]) throws IOException
{
// create a BufferedReader using System.
In BufferedReader br = new
BufferedReader(new InputStreamReader(System.in)); String str;
System.out.println("Enter 'stop' to quit.");
System.out.println("Enter State: ");
Do {
Str = br.readLine();
Str = str.trim(); // remove whitespace
If(str.equals("Illinois")) System.out.println("Capital is Springfield.");
Else if(str.equals("Missouri")) System.out.println("Capital is Jefferson City.");
Else if(str.equals("California")) System.out.println("Capital is Sacramento.");
Else if(str.equals("Washington")) System.out.println("Capital is Olympia.");
// ...
} while(!str.equals("stop"));
}
}
Key takeaway
Because String objects are immutable, modifying a String requires either copying it into a StringBuffer or StringBuilder, or calling a String method that creates a new copy of the string with your changes applied.
References:
1. Sun Certified Java Programmer for Java 6 by Kathy Sierra.
2. The Java TM Programming Language (3rd Edition) by Arnold, Holmes, Gosling, Goteti.
3. Core Java for Beginners by Rashmi Kanta Das(III Edition) Vikas Publication.
4. Java A Beginner's Guide, Fifth Edition, Tata McGra.