Unit - 3
Inheritance
Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
The idea behind inheritance in Java is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields of the parent class. Moreover, you can add new methods and fields in your current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
Why use inheritance in java
● For Method overriding (so runtime polymorphism can be achieved).
● For Code Reusability.
Terms used in Inheritance
● Class: A class is a group of objects which have common properties. It is a template or blueprint from which objects are created.
● Sub Class/Child Class: Subclass is a class which inherits the other class. It is also called a derived class, extended class, or child class.
● Super Class/Parent Class: Superclass is the class from where a subclass inherits the features. It is also called a base class or a parent class.
● Reusability: As the name specifies, reusability is a mechanism which facilitates you to reuse the fields and methods of the existing class when you create a new class. You can use the same fields and methods already defined in the previous class.
The syntax of Java Inheritance
- Class Subclass-name extends Superclass-name
- {
- //methods and fields
- }
The extends keyword indicates that you are making a new class that derives from an existing class. The meaning of "extends" is to increase the functionality.
In the terminology of Java, a class which is inherited is called a parent or superclass, and the new class is called child or subclass.
Java Inheritance Example
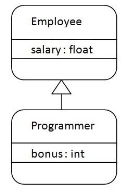
As displayed in the above figure, Programmer is the subclass and Employee is the superclass. The relationship between the two classes is Programmer IS-A Employee. It means that Programmer is a type of Employee.
- Class Employee{
- Float salary=40000;
- }
- Class Programmer extends Employee{
- Int bonus=10000;
- Public static void main(String args[]){
- Programmer p=new Programmer();
- System.out.println("Programmer salary is:"+p.salary);
- System.out.println("Bonus of Programmer is:"+p.bonus);
- }
- }
Programmer salary is:40000.0
Bonus of programmer is:10000
In the above example, Programmer object can access the field of own class as well as of Employee class i.e. code reusability.
Types of Inheritance
1. Single Inheritance
Also called as single level inheritance it is the mechanism of deriving a single sub class from a super class. The subclass will be defined by the keyword extends which signifies that the properties of the super class are extended to the sub class. The sub class will contain its own variables and methods as well as it can access methods and variables of the super class.
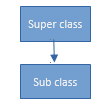
Syntax:
class Subclass-name extends Superclass-name
{
//methods and fields
}
Program:
Class x
{
Void one()
{
System.out.println("Printing one");
}
}
Class y extends x
{
Void two()
{
System.out.println("Printing two");
}
}
Public class test
{
Public static void main(String args[])
{
y t= new y();
t.two();
t.one();
}
}
Output:
Printing two
Printing one
2. Multi- level Inheritance
It is the mechanism of deriving a sub class from a super class and again a sub class is derived from this derived subclass which acts as a base class for the newly derived class. It includes a multi level base class which allows building a chain of classes where a derived class acts as super class.
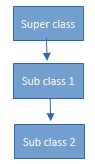
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}class three extends two
{
Void print_three()
{
System.out.println("Printing three");}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
t.print_three();
t.print_two(); t.print_one();
}
}
Output:
Printing three
Printing two
Printing one
3. Hierarchical Inheritance
In Hierarchical Inheritance, one class serves as a super class (base class) for more than one sub class.
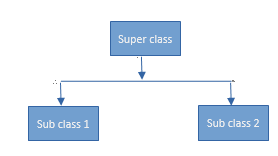
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}
Class three extends two
{
Void print_three()
{
System.out.println("Printing three");
}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
Two n= new two();t.print_three();
n.print_two();
t.print_one();
}}
Output:
Printing three
Printing two
Printing one
4. Hybrid Inheritance
It is the combination of Single inheritance and multi level inheritance.
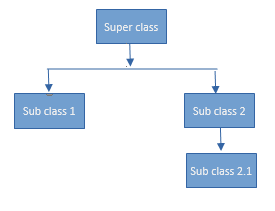
Key takeaway
- Inheritance in Java is a mechanism in which one object acquires all the properties and behaviors of a parent object. It is an important part of OOPs (Object Oriented programming system).
- In Java, there are three types of inheritance based on class: single, multilayer, and hierarchical inheritance.
- Multiple and hybrid inheritance are only enabled through interfaces in Java programming.
● Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
● It can only be inherited by the concrete classes (descendent classes), where a concrete class can be instantiated.
● Abstract class act as a generic class having attributes and methods which can be commonly shared and accessed by the concrete classes.
● The attributes and methods in the abstract class are non-static, accessible by inheriting classes.
● Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class
● It can be represented as below
Abstract public class RentalCar {
// your code goes here
}
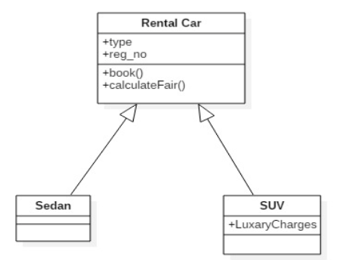
Fig: Example of abstract class
Key takeaway
- Abstract class is a class that has no direct instances but whose descendant classes have direct instances.
- Abstract can be differentiated by a normal class by writing a abstract keyword in the declaration of the class.
In Java, method overriding occurs when a subclass (child class) has the same method as the parent class.
In other words, method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
Usage of Java Method Overriding
● Method overriding is a technique for providing a particular implementation of a method that has already been implemented by its superclass.
● For runtime polymorphism, method overriding is employed.
Rules for method overriding
● The argument list should match that of the overridden method exactly.
● The return type must be the same as or a subtype of the return type stated in the superclass's original overridden method.
● The access level must be lower than the access level of the overridden method. For instance, if the superclass method is marked public, the subclass overriding method cannot be either private or protected.
● Instance methods can only be overridden if the subclass inherits them.
● It is not possible to override a method that has been declared final.
● A static method cannot be overridden, but it can be re-declared.
● A method can't be overridden if it can't be inherited.
● Any superclass function that is not declared private or final can be overridden by a subclass in the same package as the instance's superclass.
● Only non-final methods labeled public or protected can be overridden by a subclass from a different package.
● Regardless of whether the overridden method throws exceptions or not, an overriding method can throw any unchecked exceptions. The overriding method, on the other hand, should not throw any checked exceptions that aren't specified by the overridden method. In comparison to the overridden method, the overriding method can throw fewer or narrower exceptions.
● Overriding constructors is not possible.
Example
//Java Program to illustrate the use of Java Method Overriding
//Creating a parent class.
Class Vehicle{
//defining a method
void run(){System.out.println("Vehicle is running");}
}
//Creating a child class
Class Bike2 extends Vehicle{
//defining the same method as in the parent class
void run(){System.out.println("Bike is running safely");}
public static void main(String args[]){
Bike2 obj = new Bike2();//creating object
obj.run();//calling method
}
}
Output
Bike is running safely
Key takeaway
Method overriding occurs when a subclass provides a customized implementation of a method specified by one of its parent classes.
It refers to an immediate parent class object. There are 3 ways to use the ‘super’ keyword:
- To refer immediate parent class instance variable.
- To invoke immediate parent class method.
- To invoke immediate parent class constructor.
Program to refer immediate parent class instance variable using super keyword
Class transport
{
Int maxspeed = 200;
}
Class vehicle extends transport
{
Int maxspeed =100;
}
Class car extends vehicle
{
Int maxspeed = 120;
Void disp()
{System.out.println("Max speed= "+super.maxspeed);
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.disp();
}
}
Output:
Max speed= 100
Program to invoke immediate parent class method
Class transport
{
Void message()
{
System.out.println("transport");
}
}
Class vehicle extends transport
{
Void message()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Int maxspeed = 120;
Void message()
{
System.out.println("car");
}
Void display()
{
Message();
Super.message();
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.display();}
}
Output:
Car
Vehicle
Program to invoke immediate parent class constructor
Class vehicle
{
Vehicle()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Car()
{
Super();
System.out.println("car");
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
}
}
Output:
Vehicle
Car
Key takeaway
In Java, the super keyword is a reference variable that refers to an immediate parent class object.
When you create a subclass instance, you're also creating an instance of the parent class, which is referenced to by the super reference variable.
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
When you want a variable to constantly store the same value, such as PI, the final keyword comes in handy (3.14159...).
The last keyword is referred to as a "modifier."
Example
Set a variable to final, to prevent it from being overridden/modified:
Public class Main {
final int x = 10;
public static void main(String[] args) {
Main myObj = new Main();
myObj.x = 25; // will generate an error: cannot assign a value to a final variable
System.out.println(myObj.x);
}
}
Using Final with Inheritance
● The final keyword is final, which means we can't change anything.
● Variables, methods, and classes can all benefit from final keywords.
● If we use the final keyword for inheritance, that is, if we declare any method in the base class with the final keyword, the final method's implementation will be the same as in the derived class.
● If any other class extends this subclass, we can declare the final method in any subclass we wish.
Declare final variable with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final variable pa of string type i.e
the value of this variable is fixed throughout all
the derived classes or not overridden*/
final String pa = "Hello , We are in parent class variable";
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of variable ch of string type i.e
the value of this variable is not fixed throughout all
the derived classes or overridden*/
String ch = "Hello , We are in child class variable";
}
Class Test {
public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a variable pa by parent object
System.out.println(p.pa);
// Creation of Child class object
Child c = new Child();
// Calling a variable ch by Child object
System.out.println(c.ch);
// Calling a variable pa by Child object
System.out.println(c.pa);
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, We are in parent class variable
Hello, We are in child class variable
Hello, We are in parent class variable
Declare final methods with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final method parent of void type i.e
the implementation of this method is fixed throughout
all the derived classes or not overridden*/
final void parent() {
System.out.println("Hello , we are in parent method");
}
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of final method child of void type i.e
the implementation of this method is not fixed throughout
all the derived classes or not overridden*/
void child() {
System.out.println("Hello , we are in child method");
}
}
Class Test {
public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a method parent() by parent object
p.parent();
// Creation of Child class object
Child c = new Child();
// Calling a method child() by Child class object
c.child();
// Calling a method parent() by child object
c.parent();
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, we are in parent method
Hello, we are in child method
Hello, we are in parent method
Key takeaway
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
A java package is a group of similar types of classes, interfaces and sub-packages.
Package in java can be categorized in two form, built-in package and user-defined package.
There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
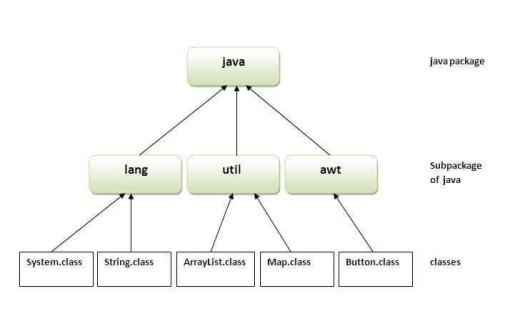
Fig: Package
Simple example of java package
The package keyword is used to create a package in java.
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
Javac -d directory javafilename
For example
Javac -d . Simple.java
The -d switch specifies the destination where to put the generated class file. You can use any directory name like /home (in case of Linux), d:/abc (in case of windows) etc. If you want to keep the package within the same directory, you can use . (dot).
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
To Compile: javac -d . Simple.java
To Run: java mypack.Simple
Output: Welcome to package
The -d is a switch that tells the compiler where to put the class file i.e. it represents destination. The . Represents the current folder.
How to access package from another package?
There are three ways to access the package from outside the package.
- Import package.*;
- Import package.classname;
- Fully qualified name.
1) Using packagename.*
If you use package.* then all the classes and interfaces of this package will be accessible but not subpackages.
The import keyword is used to make the classes and interface of another package accessible to the current package.
Example of package that import the packagename.*
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Import pack.*;
- Class B{
- Public static void main(String args[]){
- A obj = new A();
- Obj.msg();
- }
- }
Output:
Hello
2) Using packagename.classname
If you import package.classname then only declared class of this package will be accessible.
Example of package by import package.classname
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Import pack.A;
- Class B{
- Public static void main(String args[]){
- A obj = new A();
- Obj.msg();
- }
- }
Output:
Hello
3) Using fully qualified name
If you use fully qualified name then only declared class of this package will be accessible. Now there is no need to import. But you need to use fully qualified name every time when you are accessing the class or interface.
It is generally used when two packages have same class name e.g. Java.util and java.sql packages contain Date class.
Example of package by import fully qualified name
- //save by A.java
- Package pack;
- Public class A{
- Public void msg(){System.out.println("Hello");}
- }
- //save by B.java
- Package mypack;
- Class B{
- Public static void main(String args[]){
- Pack.A obj = new pack.A();//using fully qualified name
- Obj.msg();
- }
- }
Output:
Hello
Note: If you import a package, subpackages will not be imported.
If you import a package, all the classes and interface of that package will be imported excluding the classes and interfaces of the subpackages. Hence, you need to import the subpackage as well.
Note: Sequence of the program must be package then import then class.
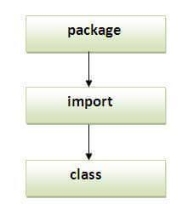
Fig: Sequence
Sub package in java
Package inside the package is called the sub package. It should be created to categorize the package further.
Let's take an example, Sun Microsystem has defined a package named java that contains many classes like System, String, Reader, Writer, Socket etc. These classes represent a particular group e.g. Reader and Writer classes are for Input/Output operation, Socket and Server Socket classes are for networking etc and so on. So, Sun has subcategorized the java package into sub packages such as lang, net, io etc. and put the Input/Output related classes in io package, Server and Server Socket classes in net packages and so on.
The standard of defining a package is domain.company.package e.g. Com.javatpoint.bean or org.sssit.dao.
Example of Sub package
- Package com.javatpoint.core;
- Class Simple{
- Public static void main(String args[]){
- System.out.println("Hello subpackage");
- }
- }
To Compile: javac -d . Simple.java
To Run: java com.javatpoint.core.Simple
Output: Hello subpackage
How to send the class file to another directory or drive?
There is a scenario, I want to put the class file of A.java source file in classes folder of c: drive. For example:
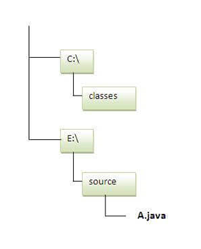
- //save as Simple.java
- Package mypack;
- Public class Simple{
- Public static void main(String args[]){
- System.out.println("Welcome to package");
- }
- }
To Compile:
e:\sources> javac -d c:\classes Simple.java
To Run:
To run this program from e:\source directory, you need to set classpath of the directory where the class file resides.
e:\sources> set classpath=c:\classes;.;
e:\sources> java mypack.Simple
Another way to run this program by -classpath switch of java:
The -classpath switch can be used with javac and java tool.
To run this program from e:\source directory, you can use -classpath switch of java that tells where to look for class file. For example:
e:\sources> java -classpath c:\classes mypack.Simple
Output:Welcome to package
Ways to load the class files or jar files
There are two ways to load the class files temporary and permanent.
● Temporary
- By setting the classpath in the command prompt
- By -classpath switch
● Permanent
- By setting the classpath in the environment variables
- By creating the jar file, that contains all the class files, and copying the jar file in the jre/lib/ext folder.
Rule: There can be only one public class in a java source file and it must be saved by the public class name.
- //save as C.java otherwise Compile Time Error
- Class A{}
- Class B{}
- Public class C{}
How to put two public classes in a package?
If you want to put two public classes in a package, have two java source files containing one public class, but keep the package name same. For example:
- //save as A.java
- Package javatpoint;
- Public class A{}
- //save as B.java
- Package javatpoint;
- Public class B{}
Advantages
1) The Java package is used to organize the classes and interfaces in order to make them easier to maintain.
2) Access control is provided via the Java package.
3) The Java package eliminates naming conflicts.
Access protection
The scope of the class and its members is defined by access modifiers (data and methods). Private members, for example, can be accessed by other members of the same class (methods). Java has several degrees of security that allow members (variables and methods) within classes, subclasses, and packages to be visible.
Packages are used to encapsulate information and act as containers for classes and subpackages. Data and methods are contained in classes. When it comes to the visibility of class members between classes and packages, Java offers four options:
● Subclasses in the same package
● Non-subclasses in the same package
● Subclasses in different packages
● Classes that are neither in the same package nor subclasses
These categories require a variety of access methods, which are provided by the three main access modifiers private, public, and protected.
Simply remember that private can only be seen within its class, public can be seen from anyone, and protected can only be accessed in subclasses within the hierarchy.
There are just two access modifiers that a class can have: default and public. If a class has default access, it can only be accessed by other code inside the same package. However, if the class is public, it can be accessed from anywhere by any code.
Key takeaway
A java package is a group of similar types of classes, interfaces and sub-packages.
Package in java can be categorized in two form, built-in package and user-defined package.
There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
Java Packages & API
A package in Java is used to group related classes. Think of it as a folder in a file directory. We use packages to avoid name conflicts, and to write a better maintainable code. Packages are divided into two categories:
● Built-in Packages (packages from the Java API)
● User-defined Packages (create your own packages)
Built-in Packages
The Java API is a library of prewritten classes, that are free to use, included in the Java Development Environment.
The library contains components for managing input, database programming, and much more.
The library is divided into packages and classes. Meaning you can either import a single class (along with its methods and attributes), or a whole package that contain all the classes that belong to the specified package.
To use a class or a package from the library, you need to use the import keyword:
Syntax
Import package.name.Class; // Import a single class
Import package.name.*; // Import the whole package
Import a Class
If you find a class you want to use, for example, the Scanner class, which is used to get user input, write the following code:
Example
Import java.util.Scanner;
In the example above, java.util is a package, while Scanner is a class of the java.util package.
To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read a complete line:
Example
Using the Scanner class to get user input:
Import java.util.Scanner;
Class MyClass {
public static void main(String[] args) {
Scanner myObj = new Scanner(System.in);
System.out.println("Enter username");
String userName = myObj.nextLine();
System.out.println("Username is: " + userName);
}
}
Import a Package
There are many packages to choose from. In the previous example, we used the Scanner class from the java.util package. This package also contains date and time facilities, random-number generator and other utility classes.
To import a whole package, end the sentence with an asterisk sign (*). The following example will import ALL the classes in the java.util package:
Example
Import java.util.*;
User-defined Packages
To create your own package, you need to understand that Java uses a file system directory to store them. Just like folders on your computer:
Example
└── root
└── mypack
└── MyPackageClass.java
To create a package, use the package keyword:
MyPackageClass.java
Package mypack;
Class MyPackageClass {
public static void main(String[] args) {
System.out.println("This is my package!");
}
}
Save the file as MyPackageClass.java, and compile it:
C:\Users\Your Name>javac MyPackageClass.java
Then compile the package:
C:\Users\Your Name>javac -d . MyPackageClass.java
This forces the compiler to create the "mypack" package.
The -d keyword specifies the destination for where to save the class file. You can use any directory name, like c:/user (windows), or, if you want to keep the package within the same directory, you can use the dot sign ".", like in the example above.
Note: The package name should be written in lower case to avoid conflict with class names.
When we compiled the package in the example above, a new folder was created, called "mypack".
To run the MyPackageClass.java file, write the following:
C:\Users\Your Name>java mypack.MyPackageClass
The output will be:
This is my package!
Key takeaway
A package in Java is used to group related classes. Think of it as a folder in a file directory. We use packages to avoid name conflicts, and to write a better maintainable code. Packages are divided into two categories:
● Built-in Packages (packages from the Java API)
● User-defined Packages (create your own packages)
In Java, an interface is a reference type. It's comparable to a class. It consists of a set of abstract methods. When a class implements an interface, it inherits the interface's abstract methods.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods. Method bodies are only available for default and static methods.
The process of creating an interface is identical to that of creating a class. A class, on the other hand, describes an object's features and behaviors. An interface, on the other hand, contains the behaviors that a class implements.
Unless the interface's implementation class is abstract, all of the interface's methods must be declared in the class.
In the following ways, an interface is analogous to a class:
● Any number of methods can be included in an interface.
● An interface is written in a file with the extension.java, with the interface's name matching the file's name.
● A.class file contains the byte code for an interface.
● Packages have interfaces, and the bytecode file for each must be in the same directory structure as the package name.
Why do we use interfaces?
There are three primary reasons to utilize an interface. Below is a list of them.
● It's a technique for achieving abstraction.
● We can offer various inheritance functionality via an interface.
● It's useful for achieving loose coupling.
Declaration
The interface keyword is used to declare an interface. All methods in an interface are declared with an empty body, and all fields are public, static, and final by default. A class that implements an interface is required to implement all of the interface's functions.
Syntax
Interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Key takeaway
In Java, an interface is a reference type.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods.
Implement interfaces
When a class implements an interface, it's like the class signing a contract, agreeing to perform the interface's defined actions. If a class doesn't perform all of the interface's actions, it must declare itself abstract.
To implement an interface, a class utilizes the implements keyword. Following the extends component of the declaration, the implements keyword appears in the class declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
public void eat() {
System.out.println("Mammal eats");
}
public void travel() {
System.out.println("Mammal travels");
}
public int noOfLegs() {
return 0;
}
public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Output
Mammal eats
Mammal travels
There are a few principles to follow when overriding methods specified in interfaces.
● Checked exceptions should not be declared on implementation methods other than those specified by the interface method or their subclasses.
● When overriding the methods, keep the signature of the interface method and the same return type or subtype.
● Interface methods do not need to be implemented if the implementation class is abstract.
When it comes to implementing interfaces, there are a few guidelines to follow.
● At any given time, a class can implement multiple interfaces.
● A class can only extend one other class while implementing several interfaces.
● In the same manner that a class can extend another class, an interface can extend another interface.
● The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that the normal flow of the application can be maintained.
● In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
What is Exception in Java
Dictionary Meaning: Exception is an abnormal condition.
In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
What is Exception Handling?
Exception Handling is a mechanism to handle runtime errors such as ClassNotFoundException, IOException, SQLException, RemoteException, etc.
The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application that is why we use exception handling. Let's take a scenario:
- Statement 1;
- Statement 2;
- Statement 3;
- Statement 4;
- Statement 5;//exception occurs
- Statement 6;
- Statement 7;
- Statement 8;
- Statement 9;
- Statement 10;
Suppose there are 10 statements in your program and there occurs an exception at statement 5, the rest of the code will not be executed i.e. statement 6 to 10 will not be executed. If we perform exception handling, the rest of the statement will be executed. That is why we use exception handling in Java.
Benefits of exception handling
● When an exception occurs, exception handling guarantees that the program's flow is preserved.
● This allows us to identify the different types of faults.
● This allows us to develop error-handling code separately from the rest of the code.
When an exception occurs, exception handling ensures that the program's flow does not break. For example, if a programme has a large number of statements and an exception occurs in the middle of executing some of them, the statements after the exception will not be executed, and the programme will end abruptly.
By handling, we ensure that all of the statements are executed and that the program's flow is not disrupted.
Key takeaway
The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that the normal flow of the application can be maintained.
There are mainly two types of exceptions: checked and unchecked. An error is considered as the unchecked exception. However, according to Oracle, there are three types of exceptions namely:
1) Checked Exception:
❖ The classes that directly inherit the Throwable class except RuntimeException and Error are known as checked exceptions.
❖ For example, IOException, SQLException, etc. Checked exceptions are checked at compile-time.
1. ClassNotFoundException: The ClassNotFoundException is a kind of checked exception that is thrown when we attempt to use a class that does not exist.
Checked exceptions are those exceptions that are checked by the Java compiler itself.
2. FileNotFoundException: The FileNotFoundException is a checked exception that is thrown when we attempt to access a non-existing file.
3. InterruptedException: InterruptedException is a checked exception that is thrown when a thread is in sleeping or waiting state and another thread attempt to interrupt it.
4. InstantiationException: This exception is also a checked exception that is thrown when we try to create an object of abstract class or interface. That is, an InstantiationException exception occurs when an abstract class or interface is instantiated.
5. IllegalAccessException: The IllegalAccessException is a checked exception and it is thrown when a method is called in another method or class but the calling method or class does not have permission to access that method.
6. CloneNotSupportedException: This checked exception is thrown when we try to clone an object without implementing the cloneable interface.
7. NoSuchFieldException: This is a checked exception that is thrown when an unknown variable is used in a program.
8. NoSuchMethodException: This checked exception is thrown when the undefined method is used in a program.
2) Unchecked Exception
❖ The classes that inherit the RuntimeException are known as unchecked exceptions.
❖ For example, ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException, etc
❖ Unchecked exceptions are not checked at compile-time, but they are checked at runtime.
Let’s see a brief description of them.
1. ArithmeticException: This exception is thrown when arithmetic problems, such as a number is divided by zero, is occurred. That is, it is caused by maths error.
2. ClassCastException: The ClassCastException is a runtime exception that is thrown by JVM when we attempt to invalid typecasting in the program. That is, it is thrown when we cast an object to a subclass of which an object is not an instance.
3. IllegalArgumentException: This runtime exception is thrown by programmatically when an illegal or appropriate argument is passed to call a method. This exception class has further two subclasses:
● Number Format Exception
● Illegal Thread State Exception
Numeric Format Exception: Number Format Exception is thrown by programmatically when we try to convert a string into the numeric type and the process of illegal conversion fails. That is, it occurs due to the illegal conversion of a string to a numeric format.
Illegal Thread State Exception: Illegal Thread State Exception is a runtime exception that is thrown by programmatically when we attempt to perform any operation on a thread but it is incompatible with the current thread state.
4. IndexOutOfBoundsException: This exception class is thrown by JVM when an array or string is going out of the specified index. It has two further subclasses:
● ArrayIndexOutOfBoundsException
● StringIndexOutOfBoundsException
ArrayIndexOutOfBoundsException: ArrayIndexOutOfBoundsException exception is thrown when an array element is accessed out of the index.
StringIndexOutOfBoundsException: StringIndexOutOfBoundsException exception is thrown when a String or StringBuffer element is accessed out of the index.
5. Null Pointer Exception: Null Pointer Exception is a runtime exception that is thrown by JVM when we attempt to use null instead of an object. That is, it is thrown when the reference is null.
6. Array Store Exception: This exception occurs when we attempt to store any value in an array which is not of array type. For example, suppose, an array is of integer type but we are trying to store a value of an element of another type.
7. IllegalStateException: The IllegalStateException exception is thrown by programmatically when the runtime environment is not in an appropriate state for calling any method.
8. IllegalMonitorStateException: This exception is thrown when a thread does not have the right to monitor an object and tries to access wait(), notify(), and notifyAll() methods of the object.
9. NegativeArraySizeException: The NegativeArraySizeException exception is thrown when an array is created with a negative size.
3) Error
Error is irrecoverable. Some examples of errors are Out Of Memory Error, Virtual Machine Error, Assertion Error etc.
Difference between Checked and Unchecked Exceptions
Checked Exception | Unchecked Exception |
These exceptions are checked at compile time. These exceptions are handled at compile time too. | These exceptions are just opposite to the checked exceptions. These exceptions are not checked and handled at compile time. |
These exceptions are direct subclasses of exception but not extended from RuntimeException class. | They are the direct subclasses of the RuntimeException class. |
The code gives a compilation error in the case when a method throws a checked exception. The compiler is not able to handle the exception on its own. | The code compiles without any error because the exceptions escape the notice of the compiler. These exceptions are the results of user-created errors in programming logic. |
These exceptions mostly occur when the probability of failure is too high. | These exceptions occur mostly due to programming mistakes. |
Common checked exceptions include IOException, DataAccessException, InterruptedException, etc. | Common unchecked exceptions include ArithmeticException, InvalidClassException, NullPointerException, etc. |
These exceptions are propagated using the throws keyword. | These are automatically propagated. |
It is required to provide the try-catch and try-finally block to handle the checked exception. | In the case of unchecked exception it is not mandatory. |
If a method does not handle a checked exception, the throws keyword must be used to declare it. The keyword throws is found at the end of a method's signature.
The throw keyword can be used to throw an exception, either a newly instantiated one or one that you have just caught.
The exception object that will be thrown is specified. The Exception has a message attached to it that describes the error. These errors could be caused by user inputs, the server, or something else entirely.
The throw keyword in Java can be used to throw either checked or unchecked exceptions. Its primary function is to throw a custom exception.
We may also use the throw keyword to establish our own set of circumstances and explicitly throw an exception. If we divide a number by another number, for example, we can throw an Arithmetic Exception. All we have to do now is set the condition and use the throw keyword to throw an exception.
Syntax
throw new exception_class("error message");
Example
Public class TestThrow1 {
//function to check if person is eligible to vote or not
public static void validate(int age) {
if(age<18) {
//throw Arithmetic exception if not eligible to vote
throw new ArithmeticException("Person is not eligible to vote");
}
else {
System.out.println("Person is eligible to vote!!");
}
}
//main method
public static void main(String args[]){
//calling the function
validate(13);
System.out.println("rest of the code...");
}
}
Output

Throws
To define an exception in Java, use the throws keyword. It informs the programmer that an exception may occur. As a result, it is preferable for the programmer to provide exception handling code so that the program's usual flow can be maintained.
Exception Handling is mostly used to deal with exceptions that have been checked. If an unchecked exception arises, such as a Null Pointer Exception, it is the programmers' fault for not inspecting the code before using it.
Syntax
return_type method_name() throws exception_class_name{
//method code
}
Example
Import java.io.IOException;
Class Testthrows1{
void m()throws IOException{
throw new IOException("device error");//checked exception
}
void n()throws IOException{
m();
}
void p(){
try{
n();
}catch(Exception e){System.out.println("exception handled");}
}
public static void main(String args[]){
Testthrows1 obj=new Testthrows1();
obj.p();
System.out.println("normal flow...");
}
}
Output
Exception handled
Normal flow...
Key takeaway
The throw keyword can be used to throw an exception, either a newly instantiated one or one that you have just caught.
If a method does not handle a checked exception, the throws keyword must be used to declare it. The keyword throws is found at the end of a method's signature.
Difference between throw and throws in Java
There are many differences between throw and throws keywords. A list of differences between throw and throws are given below:
No. | Throw | Throws |
1) | Java throw keyword is used to explicitly throw an exception. | Java throws keyword is used to declare an exception. |
2) | Checked exception cannot be propagated using throw only. | Checked exception can be propagated with throws. |
3) | Throw is followed by an instance. | Throws is followed by class. |
4) | Throw is used within the method. | Throws is used with the method signature. |
5) | You cannot throw multiple exceptions. | You can declare multiple exceptions e.g. |
In Java, you can construct your own exceptions. When writing your own exception classes, keep the following factors in mind:
● Throwable must be the parent of all exceptions.
● You must extend the Exception class if you want to build a checked exception that is enforced automatically by the Handle or Declare Rule.
● You must extend the RuntimeNException class if you want to write a runtime exception.
As shown below, we may create our own Exception class.
Class MyException extends Exception
{
}
To construct your own Exception, simply extend the default Exception class. These are regarded as exceptions that have been checked. The Insufficient Funds Exception class is a user-defined exception that extends the Exception class and is therefore examined. An exception class is similar to any other class in that it has properties and methods that are helpful.
Example
// File Name InsufficientFundsException.java
Import java.io.*;
Public class InsufficientFundsException extends Exception {
private double amount;
public InsufficientFundsException(double amount) {
this.amount = amount;
}
public double getAmount() {
return amount;
}
}
The following Checking Account class has a withdraw() method that throws an Insufficient Funds Exception to demonstrate how to use our user-defined exception.
// File Name CheckingAccount.java
Import java.io.*;
Public class CheckingAccount {
private double balance;
private int number;
public CheckingAccount(int number) {
this.number = number;
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) throws InsufficientFundsException {
if(amount <= balance) {
balance -= amount;
}else {
double needs = amount - balance;
throw new InsufficientFundsException(needs);
}
}
public double getBalance() {
return balance;
}
public int getNumber() {
return number;
}
}
The following BankDemo program shows how to use CheckingAccount's deposit() and withdraw() methods.
// File Name BankDemo.java
Public class BankDemo {
public static void main(String [] args) {
CheckingAccount c = new CheckingAccount(101);
System.out.println("Depositing $500...");
c.deposit(500.00);
try {
System.out.println("\nWithdrawing $100...");
c.withdraw(100.00);
System.out.println("\nWithdrawing $600...");
c.withdraw(600.00);
} catch (InsufficientFundsException e) {
System.out.println("Sorry, but you are short $" + e.getAmount());
e.printStackTrace();
}
}
}
Output
Depositing $500...
Withdrawing $100...
Withdrawing $600...
Sorry, but you are short $200.0
InsufficientFundsException
at CheckingAccount.withdraw(CheckingAccount.java:25)
at BankDemo.main(BankDemo.java:13)
Built in exceptions
Exceptions that are present in Java libraries are known as built-in exceptions. These exceptions are appropriate for explaining specific mistake scenarios. The following is a list of noteworthy Java built-in exceptions.
Arithmetic Exception
When an unusual circumstance in an arithmetic operation occurs, it is thrown.
Array Index out of Bounds Exception
It's thrown when an array has been accessed with an incorrect index. The index can be negative, or it can be more than or equal to the array's size.
Class Not Found Exception
When we try to access a class whose definition is missing, this Exception is thrown.
File Not Found Exception
When a file is not accessible or does not open, this Exception is thrown.
IO Exception
When an input-output operation fails or is interrupted, this exception is issued.
Interrupted Exception
When a thread is interrupted while waiting, sleeping, or processing, this exception is issued.
No Such Field Exception
It's thrown when a class doesn't have the requested field (or variable).
NoSuchMethodException
It's thrown when you try to call a method that doesn't exist.
NullPointerException
When referring to the members of a null object, this exception is thrown. Nothing is represented by null.
NumberFormatException
When a method fails to convert a string to a numeric representation, this exception is thrown.
RuntimeException
Any exception that happens during runtime is represented by this variable.
StringIndexOutOfBoundsException
It's thrown by String class methods when an index is either negative or larger than the string's length.
Example
// Java program to demonstrate ArithmeticException
Class ArithmeticException_Demo
{
public static void main(String args[])
{
try {
int a = 30, b = 0;
int c = a/b; // cannot divide by zero
System.out.println ("Result = " + c);
}
catch(ArithmeticException e) {
System.out.println ("Can't divide a number by 0");
}
}
}
Output
Can't divide a number by 0
References:
1. Sun Certified Java Programmer for Java 6 by Kathy Sierra.
2. The Java TM Programming Language (3rd Edition) by Arnold, Holmes, Gosling, Goteti.
3. Core Java for Beginners by Rashmi Kanta Das(III Edition) Vikas Publication.
4. Java A Beginner's Guide, Fifth Edition, Tata McGra.