Unit - 3
Macro and Macro Processors
Macros are single-line abbreviations for a certain group of instructions. Once the macro is defined, these groups of instructions can be used anywhere in a program. It is sometimes necessary for an assembly language programmer to repeat some blocks of code in the course of a program. The programmer needs to define a single machine instruction to represent a block of code for employing a macro in the program.
The macro proves to be useful when instead of writing the entire block again and again, you can simply write the macro that you have already defined. An assembly language macro is an instruction that represents several other machine language instructions at once.
Macros are one-line acronyms for a specific set of commands. These collections of instructions can be used anywhere in a program once the macro has been defined.
During the course of a program, an assembly language programmer may need to repeat some blocks of code. To use a macro in a program, the programmer must define a single machine instruction to represent a block of code. Instead of writing the complete block over and over, the macro comes in handy when you can simply write the macro you've already defined. An assembly language macro is a single instruction that acts as a proxy for several machine language commands.
The macro facility allows you to provide a name to a sequence that appears multiple times in a program, and then use that name whenever that sequence is encountered. All you have to do now is use the macro instruction definition to give a sequence a name.
The structure shown below demonstrates how to define a macro in a program:
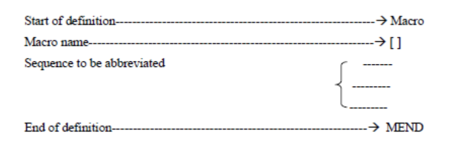
The first line of the macro definition is the MACRO pseudo-op, which is described by this structure. The name line for macro is the next line, which identifies the macro instruction name. Following the macro name is a line that contains the abbreviated sequence of instructions. The actual macro instruction is contained in each instruction. MEND pseudo-op is the last statement in the macro definition. This pseudo-op marks the end of the macro definition and the end of the macro instruction definition.
Key takeaway
Macros are single-line abbreviations for a certain group of instructions. Once the macro is defined, these groups of instructions can be used anywhere in a program.
Macros are one-line acronyms for a specific set of commands.
Macro is a specification unit for programme generation via expansion. It's a one-word acronym for a set of instructions. MACRO is often defined at the beginning or end of a programme.
Syntax of Macro Definitions:
1) Macro header: It contains the keyword ‘MACRO’.
2) Macro prototype statement syntax:
< Macro Name > [ & < Formal Parameters > ]
3) Model Statements: It contains 1 or more simple assembly statements, which will replace MACRO CALL while macro expansion.
4) MACRO END MARKER: It contains keyword ‘MEND’.
Macros call
< MACRO NAME > [<ACTUAL Parameters > ]
When actual parameters are supplied, the formal parameters are replaced with actual parameters. We only have genuine parameters in macro expansion.
Example of MACRO:
Start of definition - MACRO
Macro name ( Prototype ) – My MACRO
Macro body (Model Statements ) – ADD AREG, X
ADD BREG, X
End of Macro Definition - MEND.
Example
The following macro will conduct a memory increment operation, that is, it will take contents from memory, increment them, and then store them back into memory.
MACRO Definition Statement Type
MACRO Macro Header
INCR & MEM, & VAL, & R Macro prototype
MOVER & R, & MEM Model statements or
ADD & R, & VAL macro body
MOVEM & R, & MEM
MEND Macro End.
START 300
INCR A, B, BREAG Macro call.
STOP
A DS 1
B DS 1
END
The macro expand function is the standard way to expand a macro call. During the expansion process, a hook is provided for a user function to obtain control.
[Function]
Macroexpand form &optional env
Macroexpand-1 form &optional env
If form is a macro call, macroexpand-1 will expand it once and return two values: the expansion and the time. The two values form and nil are returned if form is not a macro call.
Only if the cons' car is a symbol that names a macro is a form regarded as a macro call. The environment env is similar to the one used by the evaluator (see evalhook); it is set to null by default. Any local macro definitions created by macrolet within env will be taken into account. If only form is given as an argument, then the environment is effectively null, and only global macro definitions (as established by defmacro) will be considered.
When you call a macro, it expands. The macro statement is replaced by a sequence of assembly statements during macro expansion.
A macro call is indicated in the middle of the graphic in the programme. INITZ, to be precise. During the execution of a programme, which is called? Every macro starts with the MACRO keyword and concludes with the ENDM keyword (end macro). When a macro is invoked, the full code of the macro is substituted in the programme in which it is invoked. As a result, the outcome of the macro code is shown on the figure's right side. In high-level programming languages, macros are called.
(C programming)
#define max(a,b) a>b?a:b
Main () {
Int x , y;
x=4; y=6;
z = max(x, y); }
C programming statements were used to create the above software. Takes two inputs, a and b, to define the macro max. This macro can be called just like any other C function, with the same syntax. As a result, following preprocessing,
Becomes z = x>y ? x: y;
After macro expansion, the whole code would appear like this.
#define max(a,b) a>b?a:b
Main()
{ int x , y;
x=4; y=6;z = x>y?x:y; }
Basic functions
● Macro Definition
● Macro Invocation
● Macro Expansion
● One-Pass Algorithm
● Data Structure
Macro Definition
In macro definition, two new assembler instructions are used:
● MACRO: identify the beginning of a macro definition
● MEND: identify the end of a macro definition
Label op operands
Name MACRO parameters
:
Body
:
MEND
Parameters: The parameters of the macro instruction are identified by the entries in the operand field.
● We require each parameter begins with ‘&’
Body: the statements that will be created as a result of the macro's expansion
Prototype for the macro: The macro name and parameters create a pattern or prototype for the programmer's macro instructions.
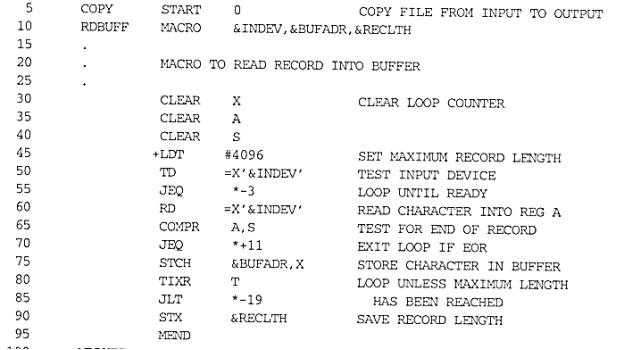
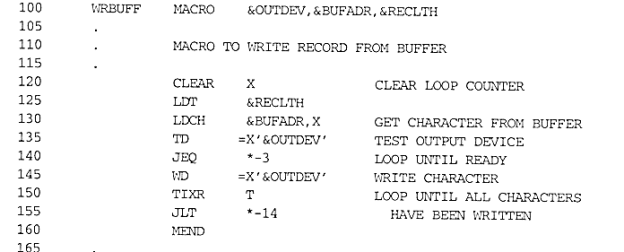
Fig 1: Example of macro definition
Macro Invocation
The name of the macro instruction being executed, as well as the arguments used to expand the macro, are given in a macro invocation statement (a macro call).
Subroutine Call vs. Macro Invocation
● The macro body's statements are extended each time the macro is called.
● Regardless matter how many times the subroutine is invoked, the subroutine's statements only appear once.
● Although macro invocation is faster than calling a procedure, the code size is bigger.
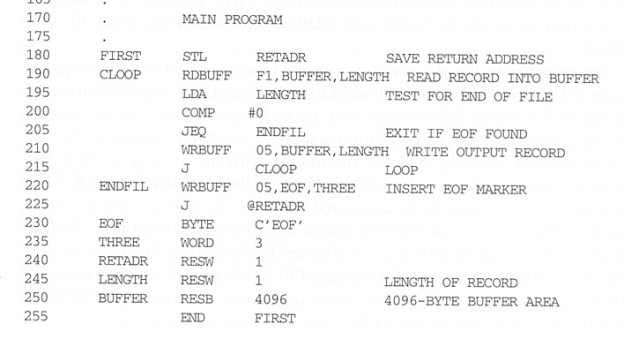
Fig 2: Example of macro invocation
Macro Expansion
The statements that make up the macro's body will be expanded from each macro invocation statement.
The parameters in the macro prototype are replaced with arguments from the macro invocation.
● The arguments and parameters are linked together based on their relative placements.
○ The first parameter in the macro prototype corresponds to the first argument in the macro invocation, and so on.
The macro body's comment lines have been removed, but individual statement comments have been kept.
A remark line has been added to the macro invocation statement.
The label from the macro invocation statement CLOOP has been applied to the first statement created by the macro expansion.
This enables a programmer to use a macro instruction in the same manner that an assembler language mnemonic would be used.
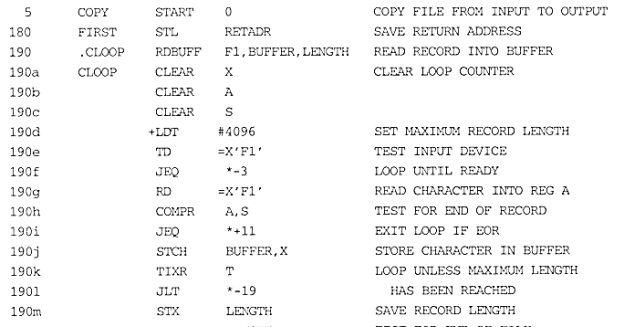
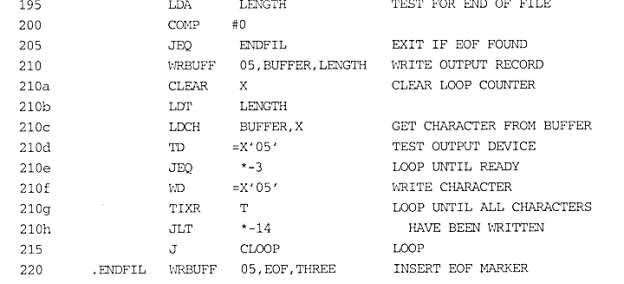
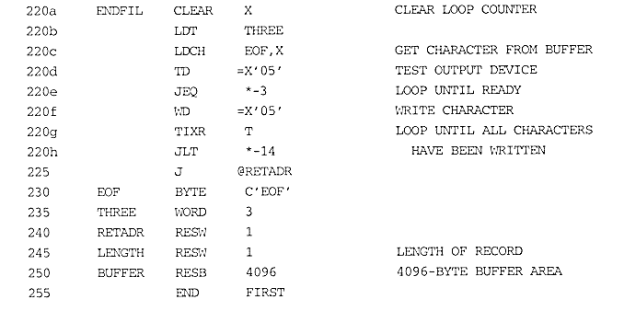
Fig 3: Example of macro expansion
Macro Processors Algorithm and Data Structures
● Two-pass macro processor
● One-pass macro processor
Two-pass macro processor
● Pass1: process all macro definitions
● Pass2: expand all macro invocation statements
Problem
● Does not allow nested macro definitions
● Nested macro definitions
○ The body of a macro contains definitions of other macros
● Because all macros would have to be defined before any macro invocations could be extended during the first pass
Solution
One-pass macro processor
Nested Macros Definition
● MACROS (for SIC)
Provides the RDBUFF and WRBUFF definitions provided in SIC instructions.
● MACROX (for SIC/XE)
Contains the SIC/XE instructions for the RDBUFF and WRBUFF definitions.
A programme that will run on a SIC system will use MACROS, but a programme that will run on a SIC/XE system will use MACROX. RDBUFF and WRBUFF are not defined by MACROS or MACROX. Only when an invocation of MACROS or MACROX is expanded are these definitions evaluated.
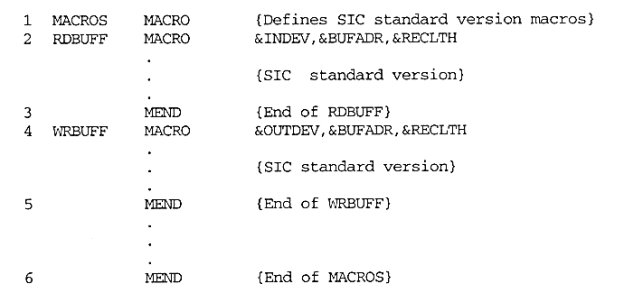
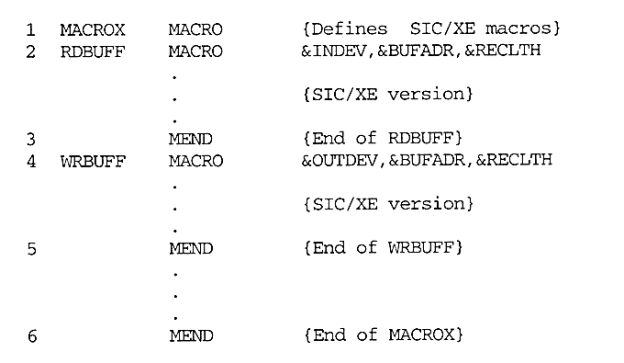
Fig 4: Example of recursive macro definition
One-pass macro processor
● Recursive macro definition can be handled using a one-pass macro processor that alternates between macro definition and macro expansion in a recursive manner.
● The definition of a macro must exist in the source programme before any statements that call that macro because of the one-pass structure.
Three Main Data Structures
DEFTAB
● A table used to store macro definitions, which includes:
○ macro prototype
○ macro body
● The comment lines have been eliminated.
● For the parameters, positional notation was employed to save time while substituting arguments.
● For example, the first argument & INDEV has been transformed to?1 (representing the prototype's first parameter).
NAMTAB
● The macro names are stored in a name table.
● It acts as a DEFTAB index.
● The beginning and conclusion of the macro definition are indicated by pointers.
ARGTAB
● The arguments used in the expansion of macro invocation are stored in an argument table.
● Arguments are swapped for the respective parameters in the macro body as the macro is extended.
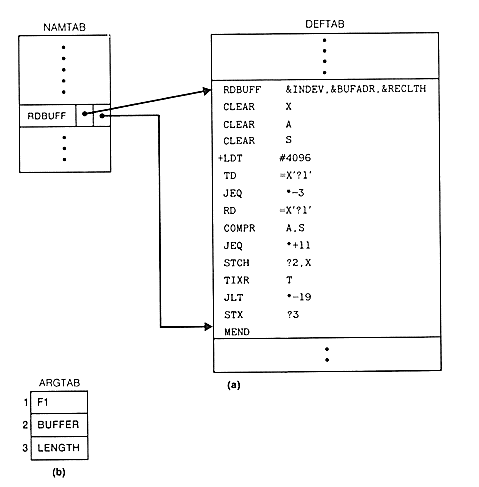
Fig 5: Data structure
● In the source programming language, a macro is a collection of frequently used statements.
● Each macro instruction is replaced by a series of source language statements by Macro Processor. This is referred to as macro expansion.
● The programmer can leave the mechanical specifics to the macro processor when using macro instructions.
● The design of a macro processor is unrelated to the computer architecture on which it runs.
● Definition, invocation, and expansion are all part of the Macro Processor.
● Databases and data structures that are adaptable.
● Name of macro arguments/parameter attributes.
● At macro definition time, the default arguments/formal parameter value.
● At the time of the macro call, the argument values are numeric, and the actual parameter value is numeric.
● Macros with comments.
The macro preprocessor takes an assembly programme with macro definitions and calls and converts it to an assembly programme without macro definitions and calls. A macro preprocessor is depicted schematically in Figure. The software generated by the macro preprocessor can now be passed to an assembler for conversion to the target language.
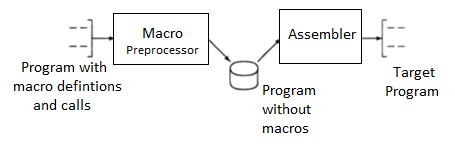
Fig 6: A schematic of A macro preprocessor
As a result, the macro preprocessor separates macro expansion from the programme assembly process. It's cost-effective because it can make use of an existing assembler. It is not, however, as efficient as a macro assembler, which handles both macro expansion and assembly.
Design Overview
We start by making a list of all the tasks required in macro expansion.
● Identify the program's macro calls.
● Determine the formal parameter values.
● Maintain the values of macro-declared expansion time variables.
● Organize the flow of expansion time control.
● Determine the sequence symbols' values.
● Extend the scope of a model statement.
To arrive at a design specification for each activity, apply the four-step approach below:
● Determine the information required to complete a task.
● Create a suitable data structure for storing the data.
● Determine the processing that will be required to retrieve the data.
● Determine the amount of processing required to complete the task.
The following is a description of how to apply this approach to each of the preprocessing tasks:
Identify macro calls
The Macro name table (MNT) is a table that contains the names of all macros defined in a programme. When a macro definition is processed, a macro name is entered in this table. The preprocessor compares the string found in its mnemonic field with the Macro names in MNT while processing a statement in the source programme. If there is a match, it means the current statement is a macro call.
Determine value of formal parameters
During the expansion of a macro call, a table called the actual parameter table (APT) is used to store the values of formal parameters. The table's entries are all pairs.
(<formal parameter name>, <value>)
This table requires two pieces of information: the names of formal parameters and the default values of Keyword parameters. Each macro uses a table called the parameter default table (PDT) for this purpose. This table, which would be available from a macro's MNT entry, would contain pairs of the form (formal parameter name>, default value>). If a macro call statement does not specify a value for a parameter, the default value from PDT to APT is duplicated.
Maintain expansion time variables
For this reason, a table of expansion time variables is kept. A couple of forms are included in the table
(<EV name>, <value>)
Except when a preprocessor statement or a model statement under expansion refers to an EV, the value field of a pair is.
Organize expansion time control flow
The body of a macro, which consists of preprocessor and model statements, is kept in a table called the Macro definition table (MDT) and is used during macro expansion. When a model statement is visited for expansion, the flow of control during macro expansion dictates when it is visited. Algorithms can be used to do this. The first statement of the Macro body in the MDT is used to initialize MEC. It is updated when a model statement is expanded or when a macro preprocessor statement is processed.
Determines values of sequencing symbols
This information is stored in a sequence symbol table (SST). There are pairs of the form in the table.
(<sequencing symbol name>,<MDT entry #>)
Where <MDT entry #> is the number of the MDT entry which contains the model statement defining the Sequencing symbol. When a statement with the Sequencing symbol in its label field is encountered, or when a reference is encountered before its definition, this entry is made.
Perform expansion of a model statement
Given the following, this is a simple task:
● The MDT element holding the model statement is pointed to by MEC.
● Formal parameter and EV values can be found in APT and EVT, respectively.
● SST can be used to find the model statement that defines a Sequencing symbol.
The parameter is expanded in a model statement by conducting a lexical substitution, and EV is utilized in the model statement.
References:
- System Programming J.J. Donovan Tata McGraw-Hill Education
- System Programming D M Dhamdhere McGraw-Hill Publication
- System Programming Srimanta pal OXFORD publication
Unit - 3
Macro and Macro Processors
Macros are single-line abbreviations for a certain group of instructions. Once the macro is defined, these groups of instructions can be used anywhere in a program. It is sometimes necessary for an assembly language programmer to repeat some blocks of code in the course of a program. The programmer needs to define a single machine instruction to represent a block of code for employing a macro in the program.
The macro proves to be useful when instead of writing the entire block again and again, you can simply write the macro that you have already defined. An assembly language macro is an instruction that represents several other machine language instructions at once.
Macros are one-line acronyms for a specific set of commands. These collections of instructions can be used anywhere in a program once the macro has been defined.
During the course of a program, an assembly language programmer may need to repeat some blocks of code. To use a macro in a program, the programmer must define a single machine instruction to represent a block of code. Instead of writing the complete block over and over, the macro comes in handy when you can simply write the macro you've already defined. An assembly language macro is a single instruction that acts as a proxy for several machine language commands.
The macro facility allows you to provide a name to a sequence that appears multiple times in a program, and then use that name whenever that sequence is encountered. All you have to do now is use the macro instruction definition to give a sequence a name.
The structure shown below demonstrates how to define a macro in a program:
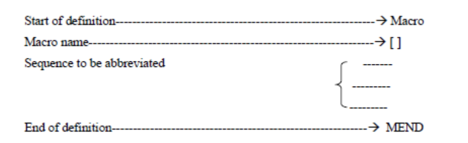
The first line of the macro definition is the MACRO pseudo-op, which is described by this structure. The name line for macro is the next line, which identifies the macro instruction name. Following the macro name is a line that contains the abbreviated sequence of instructions. The actual macro instruction is contained in each instruction. MEND pseudo-op is the last statement in the macro definition. This pseudo-op marks the end of the macro definition and the end of the macro instruction definition.
Key takeaway
Macros are single-line abbreviations for a certain group of instructions. Once the macro is defined, these groups of instructions can be used anywhere in a program.
Macros are one-line acronyms for a specific set of commands.
Macro is a specification unit for programme generation via expansion. It's a one-word acronym for a set of instructions. MACRO is often defined at the beginning or end of a programme.
Syntax of Macro Definitions:
1) Macro header: It contains the keyword ‘MACRO’.
2) Macro prototype statement syntax:
< Macro Name > [ & < Formal Parameters > ]
3) Model Statements: It contains 1 or more simple assembly statements, which will replace MACRO CALL while macro expansion.
4) MACRO END MARKER: It contains keyword ‘MEND’.
Macros call
< MACRO NAME > [<ACTUAL Parameters > ]
When actual parameters are supplied, the formal parameters are replaced with actual parameters. We only have genuine parameters in macro expansion.
Example of MACRO:
Start of definition - MACRO
Macro name ( Prototype ) – My MACRO
Macro body (Model Statements ) – ADD AREG, X
ADD BREG, X
End of Macro Definition - MEND.
Example
The following macro will conduct a memory increment operation, that is, it will take contents from memory, increment them, and then store them back into memory.
MACRO Definition Statement Type
MACRO Macro Header
INCR & MEM, & VAL, & R Macro prototype
MOVER & R, & MEM Model statements or
ADD & R, & VAL macro body
MOVEM & R, & MEM
MEND Macro End.
START 300
INCR A, B, BREAG Macro call.
STOP
A DS 1
B DS 1
END
The macro expand function is the standard way to expand a macro call. During the expansion process, a hook is provided for a user function to obtain control.
[Function]
Macroexpand form &optional env
Macroexpand-1 form &optional env
If form is a macro call, macroexpand-1 will expand it once and return two values: the expansion and the time. The two values form and nil are returned if form is not a macro call.
Only if the cons' car is a symbol that names a macro is a form regarded as a macro call. The environment env is similar to the one used by the evaluator (see evalhook); it is set to null by default. Any local macro definitions created by macrolet within env will be taken into account. If only form is given as an argument, then the environment is effectively null, and only global macro definitions (as established by defmacro) will be considered.
When you call a macro, it expands. The macro statement is replaced by a sequence of assembly statements during macro expansion.
A macro call is indicated in the middle of the graphic in the programme. INITZ, to be precise. During the execution of a programme, which is called? Every macro starts with the MACRO keyword and concludes with the ENDM keyword (end macro). When a macro is invoked, the full code of the macro is substituted in the programme in which it is invoked. As a result, the outcome of the macro code is shown on the figure's right side. In high-level programming languages, macros are called.
(C programming)
#define max(a,b) a>b?a:b
Main () {
Int x , y;
x=4; y=6;
z = max(x, y); }
C programming statements were used to create the above software. Takes two inputs, a and b, to define the macro max. This macro can be called just like any other C function, with the same syntax. As a result, following preprocessing,
Becomes z = x>y ? x: y;
After macro expansion, the whole code would appear like this.
#define max(a,b) a>b?a:b
Main()
{ int x , y;
x=4; y=6;z = x>y?x:y; }
Basic functions
● Macro Definition
● Macro Invocation
● Macro Expansion
● One-Pass Algorithm
● Data Structure
Macro Definition
In macro definition, two new assembler instructions are used:
● MACRO: identify the beginning of a macro definition
● MEND: identify the end of a macro definition
Label op operands
Name MACRO parameters
:
Body
:
MEND
Parameters: The parameters of the macro instruction are identified by the entries in the operand field.
● We require each parameter begins with ‘&’
Body: the statements that will be created as a result of the macro's expansion
Prototype for the macro: The macro name and parameters create a pattern or prototype for the programmer's macro instructions.
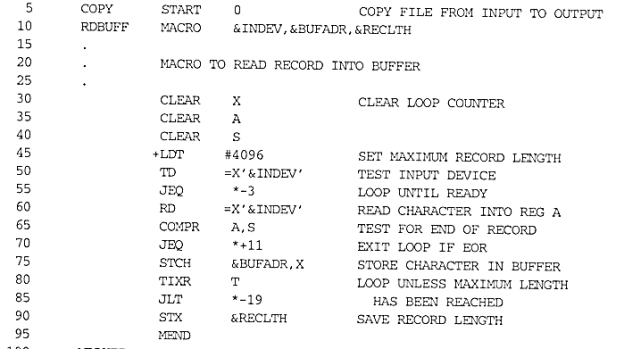
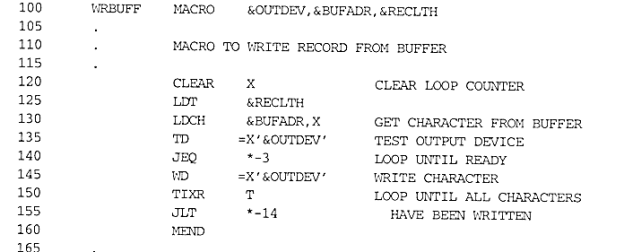
Fig 1: Example of macro definition
Macro Invocation
The name of the macro instruction being executed, as well as the arguments used to expand the macro, are given in a macro invocation statement (a macro call).
Subroutine Call vs. Macro Invocation
● The macro body's statements are extended each time the macro is called.
● Regardless matter how many times the subroutine is invoked, the subroutine's statements only appear once.
● Although macro invocation is faster than calling a procedure, the code size is bigger.
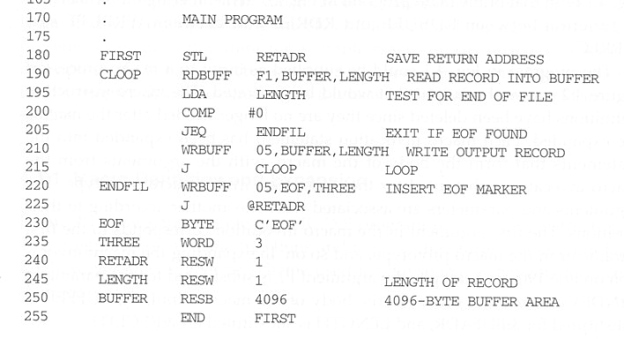
Fig 2: Example of macro invocation
Macro Expansion
The statements that make up the macro's body will be expanded from each macro invocation statement.
The parameters in the macro prototype are replaced with arguments from the macro invocation.
● The arguments and parameters are linked together based on their relative placements.
○ The first parameter in the macro prototype corresponds to the first argument in the macro invocation, and so on.
The macro body's comment lines have been removed, but individual statement comments have been kept.
A remark line has been added to the macro invocation statement.
The label from the macro invocation statement CLOOP has been applied to the first statement created by the macro expansion.
This enables a programmer to use a macro instruction in the same manner that an assembler language mnemonic would be used.
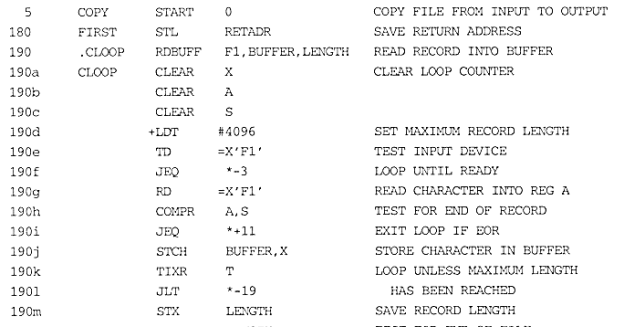
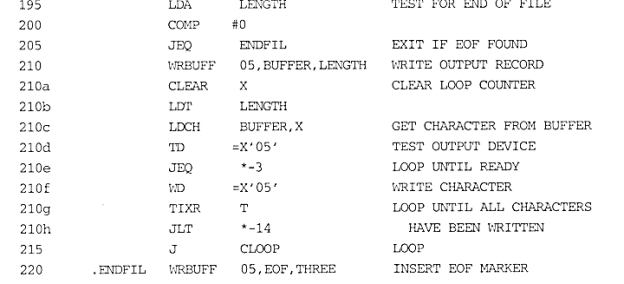
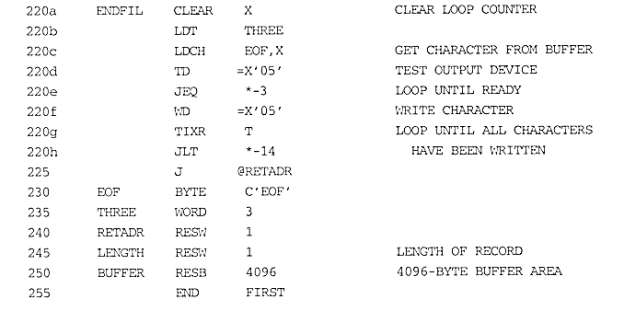
Fig 3: Example of macro expansion
Macro Processors Algorithm and Data Structures
● Two-pass macro processor
● One-pass macro processor
Two-pass macro processor
● Pass1: process all macro definitions
● Pass2: expand all macro invocation statements
Problem
● Does not allow nested macro definitions
● Nested macro definitions
○ The body of a macro contains definitions of other macros
● Because all macros would have to be defined before any macro invocations could be extended during the first pass
Solution
One-pass macro processor
Nested Macros Definition
● MACROS (for SIC)
Provides the RDBUFF and WRBUFF definitions provided in SIC instructions.
● MACROX (for SIC/XE)
Contains the SIC/XE instructions for the RDBUFF and WRBUFF definitions.
A programme that will run on a SIC system will use MACROS, but a programme that will run on a SIC/XE system will use MACROX. RDBUFF and WRBUFF are not defined by MACROS or MACROX. Only when an invocation of MACROS or MACROX is expanded are these definitions evaluated.
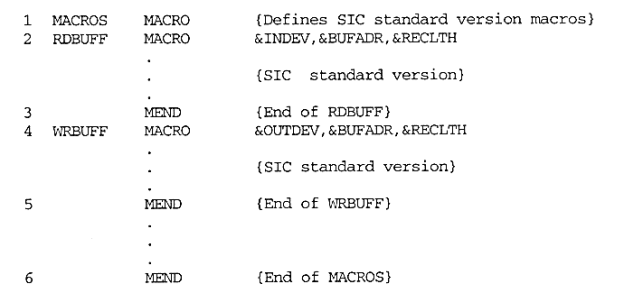
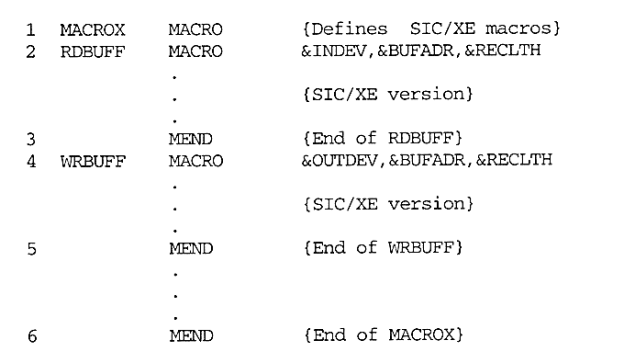
Fig 4: Example of recursive macro definition
One-pass macro processor
● Recursive macro definition can be handled using a one-pass macro processor that alternates between macro definition and macro expansion in a recursive manner.
● The definition of a macro must exist in the source programme before any statements that call that macro because of the one-pass structure.
Three Main Data Structures
DEFTAB
● A table used to store macro definitions, which includes:
○ macro prototype
○ macro body
● The comment lines have been eliminated.
● For the parameters, positional notation was employed to save time while substituting arguments.
● For example, the first argument & INDEV has been transformed to?1 (representing the prototype's first parameter).
NAMTAB
● The macro names are stored in a name table.
● It acts as a DEFTAB index.
● The beginning and conclusion of the macro definition are indicated by pointers.
ARGTAB
● The arguments used in the expansion of macro invocation are stored in an argument table.
● Arguments are swapped for the respective parameters in the macro body as the macro is extended.
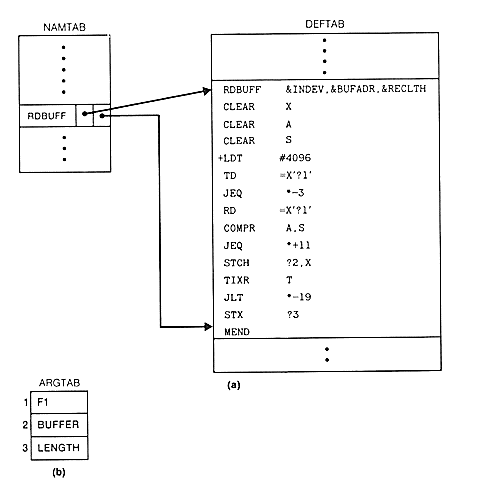
Fig 5: Data structure
● In the source programming language, a macro is a collection of frequently used statements.
● Each macro instruction is replaced by a series of source language statements by Macro Processor. This is referred to as macro expansion.
● The programmer can leave the mechanical specifics to the macro processor when using macro instructions.
● The design of a macro processor is unrelated to the computer architecture on which it runs.
● Definition, invocation, and expansion are all part of the Macro Processor.
● Databases and data structures that are adaptable.
● Name of macro arguments/parameter attributes.
● At macro definition time, the default arguments/formal parameter value.
● At the time of the macro call, the argument values are numeric, and the actual parameter value is numeric.
● Macros with comments.
The macro preprocessor takes an assembly programme with macro definitions and calls and converts it to an assembly programme without macro definitions and calls. A macro preprocessor is depicted schematically in Figure. The software generated by the macro preprocessor can now be passed to an assembler for conversion to the target language.
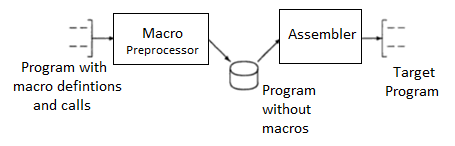
Fig 6: A schematic of A macro preprocessor
As a result, the macro preprocessor separates macro expansion from the programme assembly process. It's cost-effective because it can make use of an existing assembler. It is not, however, as efficient as a macro assembler, which handles both macro expansion and assembly.
Design Overview
We start by making a list of all the tasks required in macro expansion.
● Identify the program's macro calls.
● Determine the formal parameter values.
● Maintain the values of macro-declared expansion time variables.
● Organize the flow of expansion time control.
● Determine the sequence symbols' values.
● Extend the scope of a model statement.
To arrive at a design specification for each activity, apply the four-step approach below:
● Determine the information required to complete a task.
● Create a suitable data structure for storing the data.
● Determine the processing that will be required to retrieve the data.
● Determine the amount of processing required to complete the task.
The following is a description of how to apply this approach to each of the preprocessing tasks:
Identify macro calls
The Macro name table (MNT) is a table that contains the names of all macros defined in a programme. When a macro definition is processed, a macro name is entered in this table. The preprocessor compares the string found in its mnemonic field with the Macro names in MNT while processing a statement in the source programme. If there is a match, it means the current statement is a macro call.
Determine value of formal parameters
During the expansion of a macro call, a table called the actual parameter table (APT) is used to store the values of formal parameters. The table's entries are all pairs.
(<formal parameter name>, <value>)
This table requires two pieces of information: the names of formal parameters and the default values of Keyword parameters. Each macro uses a table called the parameter default table (PDT) for this purpose. This table, which would be available from a macro's MNT entry, would contain pairs of the form (formal parameter name>, default value>). If a macro call statement does not specify a value for a parameter, the default value from PDT to APT is duplicated.
Maintain expansion time variables
For this reason, a table of expansion time variables is kept. A couple of forms are included in the table
(<EV name>, <value>)
Except when a preprocessor statement or a model statement under expansion refers to an EV, the value field of a pair is.
Organize expansion time control flow
The body of a macro, which consists of preprocessor and model statements, is kept in a table called the Macro definition table (MDT) and is used during macro expansion. When a model statement is visited for expansion, the flow of control during macro expansion dictates when it is visited. Algorithms can be used to do this. The first statement of the Macro body in the MDT is used to initialize MEC. It is updated when a model statement is expanded or when a macro preprocessor statement is processed.
Determines values of sequencing symbols
This information is stored in a sequence symbol table (SST). There are pairs of the form in the table.
(<sequencing symbol name>,<MDT entry #>)
Where <MDT entry #> is the number of the MDT entry which contains the model statement defining the Sequencing symbol. When a statement with the Sequencing symbol in its label field is encountered, or when a reference is encountered before its definition, this entry is made.
Perform expansion of a model statement
Given the following, this is a simple task:
● The MDT element holding the model statement is pointed to by MEC.
● Formal parameter and EV values can be found in APT and EVT, respectively.
● SST can be used to find the model statement that defines a Sequencing symbol.
The parameter is expanded in a model statement by conducting a lexical substitution, and EV is utilized in the model statement.
References:
- System Programming J.J. Donovan Tata McGraw-Hill Education
- System Programming D M Dhamdhere McGraw-Hill Publication
- System Programming Srimanta pal OXFORD publication