Unit - 2
Classes and Objects
Class can be defined as the core part of Java. It is the basic construct in Java upon which the entire language is created since it defines the shape and nature of an object.
A class consist of group of objects having similar properties. It is a logical entity which can be more complex in nature.
Class Declaration
To declare a class, use the class keyword. A class can be defined as:
Class class_name{
Type obj_varaible1;
Type obj_variable2;
.....
Type obj_variableN;
Type method_name1(parameters){
//method body
}
....
Type method_nameN(parameters){
//method body
}
}
All the data or variables declare inside a class are known as instance variables. The actual logical resides within the methods. And the collection of methods and variables in class are known as members.
Example of Class
Consider an example of class student that has two instances name and grade. There is no method declaration.
Class Student{
String name;
Int grade;
}
Whenever a class is declare that means a template is created, it is not an actual object. Therefore, object creation and declaration we will see in further topics.
Similarly till now there is no method created in above example.
Key takeaway
Class can be defined as the core part of Java. It is the basic construct in Java upon which the entire language is created since it defines the shape and nature of an object.
An object is an instance of class. In Java object can be defined as the physical as well as logical entity.
Objects can be described using three parameters:
● State is used to define the data or value of an object.
● Behavior represents the functionality of an object.
● Identity is used to assign a unique id to every object,
Creating an object
To create an object in Java a class is used.
Let’s take above example of class student. To create an object of student class, specify the class name followed by the object name and use the new keyboard.
Syntax to create an object is
Classname object_name = new classname();
See the objects mystud1 and mystud2 in the above example of Student class.
Accessing Instance variables and methods
User created objects can access the instance variables and methods. Following are the syntax to access instance variables and methods by objects:
/* object creation */
Classname object_name = new classname();
/* call a variable */
Object_name.variableName;
/* call a class method */
Object_name.MethodName();
Key takeaway
An object is an instance of class. In Java object can be defined as the physical as well as logical entity.
Variables in Java can only contain pointers to objects, not objects themselves.
The objects are created and allocated in memory apart from the variable declarations. Specifically:
● All objects denoted by a literal (such as String literals, e.g., "foo", "ciao", etc.) are created in memory at compile time;
● all other objects must be generated and allocated explicitly.
A reference to a class object is stored in a variable with the class type (i.e., the address of the memory location where the object is allocated).
Example:
String s;
s = "xxx";
The first statement declares a String variable s. This variable has not yet been initialised. The second statement assigns a reference to the object specified by "xxx" to such a variable.
It's worth noting that two variables can both refer to the same object.
Example:
String s, t;
s = "xxx";
t = s;
Both t and s hold a reference to the object denoted by "xxx" after these two statements.
Object reference variables can also have a specific value, which is null. This value indicates that the variable isn't associated with any object. Variables with null values should not be confused with variables that have not been initialised. A variable that has not been initialised has no value, even if it is null.
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Properties of a constructor:
1. Name of the constructor is the same as its class name.
2. A default constructor is always present in a class implicitly.
3. There are three main types of constructors namely default, parameterized and copy constructors.
4. A constructor in Java can not be abstract, final, static and Synchronized.
Public class NewClass{
//This is the constructor
NewClass(){
}}
The new keyword here creates the object of class NewClass and invokes the constructor to initialize this newly created object.
Default constructor:
A default constructor is a constructor that is created implicitly by the JVM.
Program to demonstrate default constructors:
Class student
{
Int id;
String name;
Student()
{
System.out.println("Student id is 20 and name is Amruta");
}
Public static void main(String args[])
{
Student s = new student();
}
}
Output:

Parameterized constructor:
It is a constructor that has to be created explicitly by the programmer. This constructor has parameters that can be passed when a constructor is called at the time of object creation.
Program:
Class Student4
{
Int id;
String name;
Student4(int i,String n)
{
Id = i;
Name = n;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student4 s1 = new Student4(20,"Rema");
Student4 s2 = new Student4(23,"Sadhna");
s1.display();
s2.display();
}
}
Output:

Copy constructor:
A content of one constructor can be copied into another constructor using the objects created. Such a constructor is called as a copy constructor.
Program:
Class Student6
{
Int id;
String name;
Student6(int i,String n)
{
Id = i;
Name = n;
}
Student6(Student6 s)
{
Id = s.id;
Name =s.name;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student6 s1 = new Student6(20,"AMRUTA");
Student6 s2 = new Student6(s1);
s1.display();
s2.display();
}
}
Output:

Key takeaway
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
Compiler differentiates between each constructor on the basis of their parameter list.
Example of constructor loading is given below:
Class Add {
Int x;
Int y;
// Overloaded constructor takes two int parameters
Add(int a, int b)
{
x = a;
y = b;
}
// Overloaded constructor single int parameters
Add(int a)
{
x = y = a;
}
// Overloaded constructor takes no parameters
Add()
{
x = 6;
y = 5;
}
Int sum() {
Return x + y;
}
}
Class OverloadAdd{
Public static void main(String args[])
{
// create objects using the various constructors
Add cons1 = new Add(23, 1);
Add cons2 = new Add(8);
Add cons3 = new Add();
Int s;
s = cons1.sum()
System.out.println(“Sum of constructor1 =” + s);
s = cons2.sum()
System.out.println(“Sum of constructor2 =” + s);
s = cons3.sum()
System.out.println(“Sum of constructor3 =” + s);
}
}
Output
Sum of constructor1 = 24
Sum of constructor2 = 16
Sum of constructor3 = 11
Key takeaway
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
If a class has multiple methods having same name but different in parameters, it is known as Method Overloading.
If we have to perform only one operation, having same name of the methods increases the readability of the program.
Suppose you have to perform addition of the given numbers but there can be any number of arguments, if you write the method such as a(int,int) for two parameters, and b(int,int,int) for three parameters then it may be difficult for you as well as other programmers to understand the behavior of the method because its name differs.
So, we perform method overloading to figure out the program quickly.
Advantage of method overloading
Method overloading increases the readability of the program.
Different ways to overload the method
There are two ways to overload the method in java
- By changing number of arguments
- By changing the data type
In Java, Method Overloading is not possible by changing the return type of the method only.
1) Method Overloading: changing no. Of arguments
In this example, we have created two methods, first add() method performs addition of two numbers and second add method performs addition of three numbers.
In this example, we are creating static methods so that we don't need to create instance for calling methods.
- Class Adder{
- Static int add(int a,int b){return a+b;}
- Static int add(int a,int b,int c){return a+b+c;}
- }
- Class TestOverloading1{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));
- System.out.println(Adder.add(11,11,11));
- }}
Output:
22
33
2) Method Overloading: changing data type of arguments
In this example, we have created two methods that differs in data type. The first add method receives two integer arguments and second add method receives two double arguments.
- Class Adder{
- Static int add(int a, int b){return a+b;}
- Static double add(double a, double b){return a+b;}
- }
- Class TestOverloading2{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));
- System.out.println(Adder.add(12.3,12.6));
- }}
Output:
22
24.9
Q) Why Method Overloading is not possible by changing the return type of method only?
In java, method overloading is not possible by changing the return type of the method only because of ambiguity. Let's see how ambiguity may occur:
- Class Adder{
- Static int add(int a,int b){return a+b;}
- Static double add(int a,int b){return a+b;}
- }
- Class TestOverloading3{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));//ambiguity
- }}
Output:
Compile Time Error: method add(int,int) is already defined in class Adder
System.out.println(Adder.add(11,11)); //Here, how can java determine which sum() method should be called?
Note: Compile Time Error is better than Run Time Error. So, java compiler renders compiler time error if you declare the same method having same parameters.
Can we overload java main() method?
Yes, by method overloading. You can have any number of main methods in a class by method overloading. But JVM calls main() method which receives string array as arguments only. Let's see the simple example:
- Class TestOverloading4{
- Public static void main(String[] args){System.out.println("main with String[]");}
- Public static void main(String args){System.out.println("main with String");}
- Public static void main(){System.out.println("main without args");}
- }
Output:
Main with String[]
Key takeaway
If a class has multiple methods having same name but different in parameters, it is known as Method Overloading.
If we have to perform only one operation, having same name of the methods increases the readability of the program.
Recursion is the process of a function calling itself directly or indirectly, and the associated function is known as a recursive function. Certain problems can be solved quickly using the recursive algorithm. Towers of Hanoi (TOH), Inorder/Preorder/Postorder Tree Traversals, DFS of Graph, and others are examples of such issues.
In Java, recursion is the process of a method repeatedly calling itself. In Java, a recursive method is one that calls itself.
It makes the code small but difficult to comprehend.
Syntax:
Returntype methodname(){
//code to be executed
Methodname();//calling same method
}
Example
Public class RecursionExample2 {
Static int count=0;
Static void p(){
Count++;
If(count<=5){
System.out.println("hello "+count);
p();
}
}
Public static void main(String[] args) {
p();
}
}
Output
Hello 1
Hello 2
Hello 3
Hello 4
Hello 5
Example: Factorial number
Public class RecursionExample3 {
Static int factorial(int n){
If (n == 1)
Return 1;
Else
Return(n * factorial(n-1));
}
Public static void main(String[] args) {
System.out.println("Factorial of 5 is: "+factorial(5));
}
}
Output
Factorial of 5 is: 120
Working of above program:
Factorial(5)
Factorial(4)
Factorial(3)
Factorial(2)
Factorial(1)
Return 1
Return 2*1 = 2
Return 3*2 = 6
Return 4*6 = 24
Return 5*24 = 120
Advantages and disadvantages
New storage places for variables are allocated on the stack when a recursive call is initiated. The old variables and parameters are deleted from the stack as each recursive call returns. As a result, recursion typically consumes more memory and is slower.
A recursive solution, on the other hand, is significantly easier and requires less time to write, debug, and maintain.
Key takeaway
Recursion is the process of a function calling itself directly or indirectly, and the associated function is known as a recursive function. Certain problems can be solved quickly using the recursive algorithm.
As we all know, in Java, passing by value is always preferred over passing by reference. So, in this piece, we'll look at how this concept is validated when sending a primitive or a reference to a method.
When a primitive type is supplied as an argument to a method, the value assigned to that primitive is sent to the method, and that value becomes local to that method, meaning that any change to that value by the method will not affect the value of the primitive that you have passed to the method.
While passing a reference to a method, Java follows the same pass by value rule. Let's take a look at how this works.
In Java, a reference maintains the memory location of an object generated if it is assigned to that reference; otherwise, it is started as null. The important thing to know is that the value of a reference is the memory position of the assigned object, so anytime we send a reference as an argument to a method, we are actually passing the memory address of the object that is assigned to that reference. This technically means that the target method has access to the memory location of our constructed object.
As a result, if the target method accesses our object and modifies any of its properties, we will see the new value in our original object.
Example
Public class PassByValue {
Static int k =10;
Static void passPrimitive(int j) {
System.out.println("the value of passed primitive is " + j);
j = j + 1;
}
Static void passReference(EmployeeTest emp) {
EmployeeTest reference = emp;
System.out.println("the value of name property of our object is "+ emp.getName());
Reference.setName("Bond");
}
Public static void main(String[] args) {
EmployeeTest ref = new EmployeeTest();
Ref.setName("James");
PassPrimitive(k);
System.out.println("Value of primitive after get passed to method is "+ k);
PassReference(ref);
System.out.println("Value of property of object after reference get passed to method is "+ ref.getName());
}
}
Class EmployeeTest {
String name;
Public String getName() {
Return name;
}
Public void setName(String name) {
This.name = name;
}
}
Output
The value of passed primitive is 10
Value of primitive after get passed to method is 10
The value of name property of our object is James
Value of property of object after reference get passed to method is Bond
In Java, the new operator is used to create new objects. It's also possible to utilise it to make an array object.
Declaring a class in Java is essentially the same as defining a new data type. The blueprint for objects is provided by a class. A class can be used to make an object.
Let's have a look at the stages involved in constructing an object from a class.
Declaration - A variable declaration that includes a variable name and an object type.
Instantiation - The object is created with the help of the 'new' keyword.
Initialization - Initialization After the 'new' keyword, a call to a function Object() { [native code] } is made. The new object is initialised with this call.
Example
Public class Main {
Public static void main(String[] args) {
Double[] myList = new double[] {1.9, 2.9, 3.4, 3.5};
// Print all the array elements
For (int i = 0; i < myList.length; i++) {
System.out.println(myList[i] + " ");
}
// Summing all elements
Double total = 0;
For (int i = 0; i < myList.length; i++) {
Total += myList[i];
}
System.out.println("Total is " + total);
// Finding the largest element
Double max = myList[0];
For (int i = 1; i < myList.length; i++) {
If (myList[i] > max) max = myList[i];
}
System.out.println("Max is " + max);
}
}
Output
1.9
2.9
3.4
3.5
Total is 11.7
Max is 3.5
Key takeaway
In Java, the new operator is used to create new objects. It's also possible to utilise it to make an array object.
Static Keyword
Static keyword in Java is mainly used for memory management. We can use static keyword with variables, methods, blocks and nested classes.
The main purpose of using static keyword is in the case when we want to share the same variable or method of a given class without creating their instances.
To declare such variable or methods prefix the static keyword with the variable name or method name.
If a member is declared as static, that means it can be accessed before the creation of any object of that class and without need of any object reference.
The best example of static keyword is the main(). We declare it as static so that it must be called before the existence of any object.
One more advantage of using static is that if any variable is declared using static then no copy of static variable is made separately for the created objects. Instead all the objects of the class use the same static variable.
Besides this their few restrictions in case of static method:
● They can call only other static methods and not any other type of methods.
● Only static data can access them.
● They cannot use this or super keyword.
Example
Class DemoStatic {
Static int x = 4; //static variable
Static int y;
Static void abc(int i) { //static method
System.out.println("i = " + i);
System.out.println("x = " + x);
System.out.println("y = " + y);
}
Static { //static block
System.out.println(“Example of static block initialized”);
y = x * 4;
}
Public static void main(String args[]) {
Abc(8);
}
}
Output
Example of static block initialized.
i = 8
x = 4
y = 16
To use a static method outside a class, following syntax will be used:
Classname.methodname()
This Keyword
This keyword is a reference variable that refers to current object. It can be used in number of scenarios.
- Used to refer instance variable of the current class
- can be used in a constructor
- can be passed as an argument in the method call and constructor call
- can be used to invoke current class constructor
Program:
Class thisDemo
{
Int i;
Int j;
// Parameterized constructor
ThisDemo(int i, int j)
{
This.i = i;
This.j = j;
}
Void display()
{
System.out.println("i=" +i);
System.out.println("j=" +j);
}
Public static void main(String[] args)
{
ThisDemo object = new thisDemo(10, 20);
Object.display();
}
}
Output:
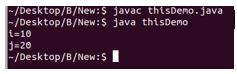
Key takeaway
This keyword is a reference variable that refers to current object. It can be used in number of scenarios.
Static keyword in Java is mainly used for memory management. We can use static keyword with variables, methods, blocks and nested classes.
Finalize() is the method of Object class. This method is called just before an object is garbage collected. Finalize() method overrides to dispose system resources, perform clean-up activities and minimize memory leaks.
Syntax
- Protected void finalize() throws Throwable
Throw
Throwable - the Exception is raised by this method
Example 1
- Public class JavafinalizeExample1 {
- Public static void main(String[] args)
- {
- JavafinalizeExample1 obj = new JavafinalizeExample1();
- System.out.println(obj.hashCode());
- Obj = null;
- // calling garbage collector
- System.gc();
- System.out.println("end of garbage collection");
- }
- @Override
- Protected void finalize()
- {
- System.out.println("finalize method called");
- }
- }
Output:
2018699554
End of garbage collection
Finalize method called
Key takeaway
Finalize() is the method of Object class. This method is called just before an object is garbage collected. Finalize() method overrides to dispose system resources, perform clean-up activities and minimize memory leaks.
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers. They are as follows:
● public - A public modifier's access level is visible everywhere. It can be accessible from within and outside the class, from within and outside the package, and from within and outside the package.
● protected - A protected modifier's access level is both within the package and outside the package via a child class. The child class cannot be accessed from outside the package unless it is created.
● default - A default modifier's access level is limited to the package. It's not possible to get to it from outside the package. If you don't indicate an access level, the default will be used.
● private - A private modifier's access level is limited to the class. It isn't accessible outside of the class.
The access modifier for that member (variable or method) will be default if it is not declared as public, protected, or private. Access modifiers can also be applied to classes.
Private is the most restrictive of the four access modifiers, whereas public is the least restrictive. The following is the syntax for declaring an access modifier:
Access-modifier data-type variable-name;
Java Access Modifiers
Let's look at a basic table to see how access modifiers work in Java.
Access Modifier | Within class | Within package | Outside package by subclass only | Outside package |
Private | Y | N | N | N |
Default | Y | Y | N | N |
Protected | Y | Y | Y | N |
Public | Y | Y | Y | Y |
Private
Only members of the class have access to the private access modifier.
An example of a private access modifier in action
We've built two classes in this example: A and Simple. Private data members and private methods are found in a class. There is a compile-time problem since we are accessing these secret members from outside the class.
Class A{
Private int data=40;
Private void msg(){System.out.println("Hello java");}
}
Public class Simple{
Public static void main(String args[]){
A obj=new A();
System.out.println(obj.data);//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Default
If you don't specify a modifier, it will be treated as default. The default modifier is only available within the package. It's not possible to get to it from outside the package. It allows for more accessibility than a private setting. It is, nevertheless, more limited than protected and open.
A default access modifier is one example.
We've produced two packages in this example: pack and mypack. We're accessing A from outside its package because A isn't public and so can't be accessed from outside the package.
//save by A.java
Package pack;
Class A{
Void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Protected
The protected access modifier can be used both inside and outside of the package, but only through inheritance.
The data member, method, and function Object() { [native code] } can all have the protected access modifier applied to them. It is not applicable to the class.
It is more accessible than the standard modifier.
A protected access modifier is an example.
We've produced two packages in this example: pack and mypack. The public A class of the pack package can be accessed from outside the package. However, because this package's msg function is protected, it can only be accessible from outside the class through inheritance.
//save by A.java
Package pack;
Public class A{
Protected void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B extends A{
Public static void main(String args[]){
B obj = new B();
Obj.msg();
}
}
Output:Hello
Public
The public access modifier can be used everywhere. Among all the modifiers, it has the broadest application.
A public access modifier is an example.
//save by A.java
Package pack;
Public class A{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
Output: Hello
Key takeaway
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers.
Basically, there are two types of modifiers in Java, access modifiers and non-access modifiers.
In Java there are four types of access modifiers according to the level of access required for classes, variable, methods and constructors. Access modifiers like public, private, protected and default.
Default Access Modifier
In this access modifier we do not specify any modifier for a class, variable, method and constructor.
Such types of variables or method can be used by any other class. There are no restrictions in this access modifier.
There is no syntax for default modifier as declare the variables and methods without any modifier keyword.
Example-
String name = “Disha”;
Boolean processName() {
Return true;
}
Private Access Modifier
This access modifier uses the private keyword. By using this access modifier the methods, variable and constructors become private and can only be accessed by the member of that same class.
It is the most restrict access specifier. Class and interface cannot be declared using the private keyword.
By using private keyword we can implement the concept of encapsulation, which hides data from the outside world.
Example of private access modifier
Public class ExampleAcces {
Private String ID;
Public String getID() {
Return this.ID;
}
Public void setID(String ID) {
This.ID = ID;
}
}
In the above example the ID variable of the ExampleAccess class is private, so no other class can retrieve or set its value easily.
So, to access this variable for use in the outside world, we defined two public methods: getID(), which returns the value of ID, and setID(String), which sets its value.
Public Access Modifier
As the name suggest a public access modifier can be easily accessed by any other class or methods.
Whenever we specify public keyword to a class, method, constructor etc, then they can be accessed from any other class in the same package. Outside package we have to import that class for access because of the concept of inheritance.
In the below example, function uses public access modifier:
Public static void main(String[] arguments) {
// ...
}
It is necessary to declare the main method as public, else Java interpreter will not be able to called it to run the class.
Protected Access Modifier
In this access modifier, in a superclass if variables, methods and constructors are declared as protected then only the subclass in other package or any class within the same package can access it.
It cannot be assigned to class and interfaces. In general method’s fields can be declared as protected: however methods and fields of interface cannot be defined as protected.
It cannot be defined on the class.
An example of protected access modifier is shown below:
Class Person {
Protected int ID(ID pid) {
// implementation details
}
}
Class Employee extends Person {
Int ID(ID pid) {
// implementation details
}
}
Tips for Access Modifier
- A public method declared in a superclass, must also be public in all subclasses.
- In a superclass if a method is declared as protected, then in subclass it should be either protected or public, they cannot be private.
- Private methods cannot be inherited.
Key takeaway
Basically, there are two types of modifiers in Java, access modifiers and non-access modifiers.
Inner Classes (Nested Classes)
● Java inner class or nested class is a class that is declared inside the class or interface.
● We use inner classes to logically group classes and interfaces in one place to be more readable and maintainable.
● it can access all the members of the outer class, including private data members and methods.
Syntax of Inner class
Class Java_Outer_class
{ //code
Class Java_Inner_class
{ //code }
}
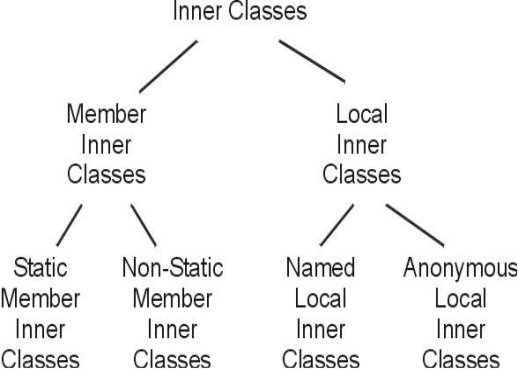
Advantage of Java inner classes
- Nested classes represent a particular type of relationship that is it can access all the members (data members and methods) of the outer class, including private.
- Nested classes are used to develop more readable and maintainable code because it logically group classes and interfaces in one place only.
- Code Optimization: It requires less code to write.
Need of Java Inner class
● Sometimes users need to program a class in such a way so that no other class can access it.
● Therefore, it would be better if you include it within other classes.
● If all the class objects are a part of the outer object then it is easier to nest that class inside the outer class.
● That way all the outer class can access all the objects of the inner class.
Types of Nested classes
There are two types of nested classes non-static and static nested classes. The non-static nested classes are also known as inner classes.
- Non-static nested class (inner class)
- Member inner class
- Anonymous inner class
- Local inner class
- Static nested class
Type | Description |
Member Inner Class | A class created within class and outside method. |
Anonymous Inner Class | A class created for implementing an interface or extending class. The java compiler decides its name. |
Local Inner Class | A class was created within the method. |
Static Nested Class | A static class was created within the class. |
Nested Interface | An interface created within class or interface. |
Java Inner Classes
Java inner class or nested class is a class which is declared inside the class or interface.
We use inner classes to logically group classes and interfaces in one place so that it can be more readable and maintainable.
Additionally, it can access all the members of outer class including private data members and methods.
Syntax of Inner class
- Class Java_Outer_class{
- //code
- Class Java_Inner_class{
- //code
- }
- }
Advantage of java inner classes
There are basically three advantages of inner classes in java. They are as follows:
1) Nested classes represent a special type of relationship that is it can access all the members (data members and methods) of outer class including private.
2) Nested classes are used to develop more readable and maintainable code because it logically group classes and interfaces in one place only.
3) Code Optimization: It requires less code to write.
Do You Know
● What is the internal code generated by the compiler for member inner class?
● What are the two ways to create anonymous inner class?
● Can we access the non-final local variable inside the local inner class?
● How to access the static nested class?
● Can we define an interface within the class?
● Can we define a class within the interface?
Difference between nested class and inner class in Java
Inner class is a part of nested class. Non-static nested classes are known as inner classes.
Types of Nested classes
There are two types of nested classes non-static and static nested classes.The non-static nested classes are also known as inner classes.
● Non-static nested class (inner class)
- Member inner class
- Anonymous inner class
- Local inner class
● Static nested class
Type | Description |
Member Inner Class | A class created within class and outside method. |
Anonymous Inner Class | A class created for implementing interface or extending class. Its name is decided by the java compiler. |
Local Inner Class | A class created within method. |
Static Nested Class | A static class created within class. |
Nested Interface | An interface created within class or interface. |
Java provides a number of access modifiers to set access levels for classes, variables, methods, and constructors. The four access levels are −
● Visible to the package, the default. No modifiers are needed.
● Visible to the class only (private).
● Visible to the world (public).
● Visible to the package and all subclasses (protected).
Key takeaway
Java inner class or nested class is a class that is declared inside the class or interface.
There are two types of nested classes non-static and static nested classes. The non-static nested classes are also known as inner classes.
- Java anonymous inner class is an inner class without a name and for which only a single object is created.
- An anonymous inner class can be useful when making an instance of an object with certain "extras" such as overloading methods of a class or interface, without having to actually subclass a class.
- In simple words, a class that has no name is known as an anonymous inner class in Java. It should be used if you have to override a method of class or interface. Java Anonymous inner class can be created in two ways:
- Class (may be abstract or concrete).
- Interface
Java anonymous inner class example using class
TestAnonymousInner.java
Abstract class Person
{
Abstract void eat();
}
Class TestAnonymousInner
{
Public static void main(String args[])
{
Person p=new Person(){
Void eat()
{ System.out.println("nice fruits"); }
};
p.eat();
}
}
OUTPUT:
Nice fruit
Key takeaway
Java anonymous inner class is an inner class without a name and for which only a single object is created.
● Abstract classes are the same as normal Java classes the difference is only that an abstract class uses abstract keyword while the normal Java class does not use.
● We use the abstract keyword before the class name to declare the class as abstract.
● An abstract class contains abstract methods as well as concrete methods.
● If we want to use an abstract class, we have to inherit it from the base class.
● If the class does not have the implementation of all the methods of the interface, we should declare the class as abstract. It provides complete abstraction. It means that fields are public static and final by default and methods are empty.
The syntax of abstract class is:
Public abstract class ClassName
{
Public abstract methodName();
}
Example of car:
Abstract class Car
{ //abstract method
Abstract void start();
//non-abstract method
Public void stop()
{
System.out.println("The car engine is not started.");
}
} //inherit abstract class
Public class Owner extends Car
{ //defining the body of the abstract method of the abstract class
Void start()
{
System.out.println("The car engine has been started.");
}
Public static void main(String[] args)
{ Owner obj = new Owner();
//calling abstract method
Obj.start();
//calling non-abstract method
Obj.stop();
}
}
OUTPUT:
The car has been started.
The car engine is not started.
Abstract Method
The method that does not has method body is known as abstract method. In other words, without an implementation is known as abstract method. It always declares in the abstract class. It means the class itself must be abstract if it has abstract method. To create an abstract method, we use the keyword abstract.
Syntax
- Abstract void method_name();
Example of abstract method
Demo.java
- Abstract class Demo //abstract class
- {
- //abstract method declaration
- Abstract void display();
- }
- Public class MyClass extends Demo
- {
- //method implementation
- Void display()
- {
- System.out.println("Abstract method?");
- }
- Public static void main(String args[])
- {
- //creating object of abstract class
- Demo obj = new MyClass();
- //invoking abstract method
- Obj.display();
- }
- }
Output:
Abstract method...
Key takeaway
Abstract classes are the same as normal Java classes the difference is only that an abstract class uses abstract keyword while the normal Java class does not use.
References:
- Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
- CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
- JAVA Complete Reference (9th Edition) Herbert Schelidt.
- T. Budd, “Understanding Object- Oriented Programming with Java”, Pearson Education, Updated Edition (New Java 2 Coverage), 1999.
Unit - 2
Classes and Objects
Class can be defined as the core part of Java. It is the basic construct in Java upon which the entire language is created since it defines the shape and nature of an object.
A class consist of group of objects having similar properties. It is a logical entity which can be more complex in nature.
Class Declaration
To declare a class, use the class keyword. A class can be defined as:
Class class_name{
Type obj_varaible1;
Type obj_variable2;
.....
Type obj_variableN;
Type method_name1(parameters){
//method body
}
....
Type method_nameN(parameters){
//method body
}
}
All the data or variables declare inside a class are known as instance variables. The actual logical resides within the methods. And the collection of methods and variables in class are known as members.
Example of Class
Consider an example of class student that has two instances name and grade. There is no method declaration.
Class Student{
String name;
Int grade;
}
Whenever a class is declare that means a template is created, it is not an actual object. Therefore, object creation and declaration we will see in further topics.
Similarly till now there is no method created in above example.
Key takeaway
Class can be defined as the core part of Java. It is the basic construct in Java upon which the entire language is created since it defines the shape and nature of an object.
An object is an instance of class. In Java object can be defined as the physical as well as logical entity.
Objects can be described using three parameters:
● State is used to define the data or value of an object.
● Behavior represents the functionality of an object.
● Identity is used to assign a unique id to every object,
Creating an object
To create an object in Java a class is used.
Let’s take above example of class student. To create an object of student class, specify the class name followed by the object name and use the new keyboard.
Syntax to create an object is
Classname object_name = new classname();
See the objects mystud1 and mystud2 in the above example of Student class.
Accessing Instance variables and methods
User created objects can access the instance variables and methods. Following are the syntax to access instance variables and methods by objects:
/* object creation */
Classname object_name = new classname();
/* call a variable */
Object_name.variableName;
/* call a class method */
Object_name.MethodName();
Key takeaway
An object is an instance of class. In Java object can be defined as the physical as well as logical entity.
Variables in Java can only contain pointers to objects, not objects themselves.
The objects are created and allocated in memory apart from the variable declarations. Specifically:
● All objects denoted by a literal (such as String literals, e.g., "foo", "ciao", etc.) are created in memory at compile time;
● all other objects must be generated and allocated explicitly.
A reference to a class object is stored in a variable with the class type (i.e., the address of the memory location where the object is allocated).
Example:
String s;
s = "xxx";
The first statement declares a String variable s. This variable has not yet been initialised. The second statement assigns a reference to the object specified by "xxx" to such a variable.
It's worth noting that two variables can both refer to the same object.
Example:
String s, t;
s = "xxx";
t = s;
Both t and s hold a reference to the object denoted by "xxx" after these two statements.
Object reference variables can also have a specific value, which is null. This value indicates that the variable isn't associated with any object. Variables with null values should not be confused with variables that have not been initialised. A variable that has not been initialised has no value, even if it is null.
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Properties of a constructor:
1. Name of the constructor is the same as its class name.
2. A default constructor is always present in a class implicitly.
3. There are three main types of constructors namely default, parameterized and copy constructors.
4. A constructor in Java can not be abstract, final, static and Synchronized.
Public class NewClass{
//This is the constructor
NewClass(){
}}
The new keyword here creates the object of class NewClass and invokes the constructor to initialize this newly created object.
Default constructor:
A default constructor is a constructor that is created implicitly by the JVM.
Program to demonstrate default constructors:
Class student
{
Int id;
String name;
Student()
{
System.out.println("Student id is 20 and name is Amruta");
}
Public static void main(String args[])
{
Student s = new student();
}
}
Output:

Parameterized constructor:
It is a constructor that has to be created explicitly by the programmer. This constructor has parameters that can be passed when a constructor is called at the time of object creation.
Program:
Class Student4
{
Int id;
String name;
Student4(int i,String n)
{
Id = i;
Name = n;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student4 s1 = new Student4(20,"Rema");
Student4 s2 = new Student4(23,"Sadhna");
s1.display();
s2.display();
}
}
Output:

Copy constructor:
A content of one constructor can be copied into another constructor using the objects created. Such a constructor is called as a copy constructor.
Program:
Class Student6
{
Int id;
String name;
Student6(int i,String n)
{
Id = i;
Name = n;
}
Student6(Student6 s)
{
Id = s.id;
Name =s.name;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student6 s1 = new Student6(20,"AMRUTA");
Student6 s2 = new Student6(s1);
s1.display();
s2.display();
}
}
Output:

Key takeaway
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
Compiler differentiates between each constructor on the basis of their parameter list.
Example of constructor loading is given below:
Class Add {
Int x;
Int y;
// Overloaded constructor takes two int parameters
Add(int a, int b)
{
x = a;
y = b;
}
// Overloaded constructor single int parameters
Add(int a)
{
x = y = a;
}
// Overloaded constructor takes no parameters
Add()
{
x = 6;
y = 5;
}
Int sum() {
Return x + y;
}
}
Class OverloadAdd{
Public static void main(String args[])
{
// create objects using the various constructors
Add cons1 = new Add(23, 1);
Add cons2 = new Add(8);
Add cons3 = new Add();
Int s;
s = cons1.sum()
System.out.println(“Sum of constructor1 =” + s);
s = cons2.sum()
System.out.println(“Sum of constructor2 =” + s);
s = cons3.sum()
System.out.println(“Sum of constructor3 =” + s);
}
}
Output
Sum of constructor1 = 24
Sum of constructor2 = 16
Sum of constructor3 = 11
Key takeaway
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
If a class has multiple methods having same name but different in parameters, it is known as Method Overloading.
If we have to perform only one operation, having same name of the methods increases the readability of the program.
Suppose you have to perform addition of the given numbers but there can be any number of arguments, if you write the method such as a(int,int) for two parameters, and b(int,int,int) for three parameters then it may be difficult for you as well as other programmers to understand the behavior of the method because its name differs.
So, we perform method overloading to figure out the program quickly.
Advantage of method overloading
Method overloading increases the readability of the program.
Different ways to overload the method
There are two ways to overload the method in java
- By changing number of arguments
- By changing the data type
In Java, Method Overloading is not possible by changing the return type of the method only.
1) Method Overloading: changing no. Of arguments
In this example, we have created two methods, first add() method performs addition of two numbers and second add method performs addition of three numbers.
In this example, we are creating static methods so that we don't need to create instance for calling methods.
- Class Adder{
- Static int add(int a,int b){return a+b;}
- Static int add(int a,int b,int c){return a+b+c;}
- }
- Class TestOverloading1{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));
- System.out.println(Adder.add(11,11,11));
- }}
Output:
22
33
2) Method Overloading: changing data type of arguments
In this example, we have created two methods that differs in data type. The first add method receives two integer arguments and second add method receives two double arguments.
- Class Adder{
- Static int add(int a, int b){return a+b;}
- Static double add(double a, double b){return a+b;}
- }
- Class TestOverloading2{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));
- System.out.println(Adder.add(12.3,12.6));
- }}
Output:
22
24.9
Q) Why Method Overloading is not possible by changing the return type of method only?
In java, method overloading is not possible by changing the return type of the method only because of ambiguity. Let's see how ambiguity may occur:
- Class Adder{
- Static int add(int a,int b){return a+b;}
- Static double add(int a,int b){return a+b;}
- }
- Class TestOverloading3{
- Public static void main(String[] args){
- System.out.println(Adder.add(11,11));//ambiguity
- }}
Output:
Compile Time Error: method add(int,int) is already defined in class Adder
System.out.println(Adder.add(11,11)); //Here, how can java determine which sum() method should be called?
Note: Compile Time Error is better than Run Time Error. So, java compiler renders compiler time error if you declare the same method having same parameters.
Can we overload java main() method?
Yes, by method overloading. You can have any number of main methods in a class by method overloading. But JVM calls main() method which receives string array as arguments only. Let's see the simple example:
- Class TestOverloading4{
- Public static void main(String[] args){System.out.println("main with String[]");}
- Public static void main(String args){System.out.println("main with String");}
- Public static void main(){System.out.println("main without args");}
- }
Output:
Main with String[]
Key takeaway
If a class has multiple methods having same name but different in parameters, it is known as Method Overloading.
If we have to perform only one operation, having same name of the methods increases the readability of the program.
Recursion is the process of a function calling itself directly or indirectly, and the associated function is known as a recursive function. Certain problems can be solved quickly using the recursive algorithm. Towers of Hanoi (TOH), Inorder/Preorder/Postorder Tree Traversals, DFS of Graph, and others are examples of such issues.
In Java, recursion is the process of a method repeatedly calling itself. In Java, a recursive method is one that calls itself.
It makes the code small but difficult to comprehend.
Syntax:
Returntype methodname(){
//code to be executed
Methodname();//calling same method
}
Example
Public class RecursionExample2 {
Static int count=0;
Static void p(){
Count++;
If(count<=5){
System.out.println("hello "+count);
p();
}
}
Public static void main(String[] args) {
p();
}
}
Output
Hello 1
Hello 2
Hello 3
Hello 4
Hello 5
Example: Factorial number
Public class RecursionExample3 {
Static int factorial(int n){
If (n == 1)
Return 1;
Else
Return(n * factorial(n-1));
}
Public static void main(String[] args) {
System.out.println("Factorial of 5 is: "+factorial(5));
}
}
Output
Factorial of 5 is: 120
Working of above program:
Factorial(5)
Factorial(4)
Factorial(3)
Factorial(2)
Factorial(1)
Return 1
Return 2*1 = 2
Return 3*2 = 6
Return 4*6 = 24
Return 5*24 = 120
Advantages and disadvantages
New storage places for variables are allocated on the stack when a recursive call is initiated. The old variables and parameters are deleted from the stack as each recursive call returns. As a result, recursion typically consumes more memory and is slower.
A recursive solution, on the other hand, is significantly easier and requires less time to write, debug, and maintain.
Key takeaway
Recursion is the process of a function calling itself directly or indirectly, and the associated function is known as a recursive function. Certain problems can be solved quickly using the recursive algorithm.
As we all know, in Java, passing by value is always preferred over passing by reference. So, in this piece, we'll look at how this concept is validated when sending a primitive or a reference to a method.
When a primitive type is supplied as an argument to a method, the value assigned to that primitive is sent to the method, and that value becomes local to that method, meaning that any change to that value by the method will not affect the value of the primitive that you have passed to the method.
While passing a reference to a method, Java follows the same pass by value rule. Let's take a look at how this works.
In Java, a reference maintains the memory location of an object generated if it is assigned to that reference; otherwise, it is started as null. The important thing to know is that the value of a reference is the memory position of the assigned object, so anytime we send a reference as an argument to a method, we are actually passing the memory address of the object that is assigned to that reference. This technically means that the target method has access to the memory location of our constructed object.
As a result, if the target method accesses our object and modifies any of its properties, we will see the new value in our original object.
Example
Public class PassByValue {
Static int k =10;
Static void passPrimitive(int j) {
System.out.println("the value of passed primitive is " + j);
j = j + 1;
}
Static void passReference(EmployeeTest emp) {
EmployeeTest reference = emp;
System.out.println("the value of name property of our object is "+ emp.getName());
Reference.setName("Bond");
}
Public static void main(String[] args) {
EmployeeTest ref = new EmployeeTest();
Ref.setName("James");
PassPrimitive(k);
System.out.println("Value of primitive after get passed to method is "+ k);
PassReference(ref);
System.out.println("Value of property of object after reference get passed to method is "+ ref.getName());
}
}
Class EmployeeTest {
String name;
Public String getName() {
Return name;
}
Public void setName(String name) {
This.name = name;
}
}
Output
The value of passed primitive is 10
Value of primitive after get passed to method is 10
The value of name property of our object is James
Value of property of object after reference get passed to method is Bond
In Java, the new operator is used to create new objects. It's also possible to utilise it to make an array object.
Declaring a class in Java is essentially the same as defining a new data type. The blueprint for objects is provided by a class. A class can be used to make an object.
Let's have a look at the stages involved in constructing an object from a class.
Declaration - A variable declaration that includes a variable name and an object type.
Instantiation - The object is created with the help of the 'new' keyword.
Initialization - Initialization After the 'new' keyword, a call to a function Object() { [native code] } is made. The new object is initialised with this call.
Example
Public class Main {
Public static void main(String[] args) {
Double[] myList = new double[] {1.9, 2.9, 3.4, 3.5};
// Print all the array elements
For (int i = 0; i < myList.length; i++) {
System.out.println(myList[i] + " ");
}
// Summing all elements
Double total = 0;
For (int i = 0; i < myList.length; i++) {
Total += myList[i];
}
System.out.println("Total is " + total);
// Finding the largest element
Double max = myList[0];
For (int i = 1; i < myList.length; i++) {
If (myList[i] > max) max = myList[i];
}
System.out.println("Max is " + max);
}
}
Output
1.9
2.9
3.4
3.5
Total is 11.7
Max is 3.5
Key takeaway
In Java, the new operator is used to create new objects. It's also possible to utilise it to make an array object.
Static Keyword
Static keyword in Java is mainly used for memory management. We can use static keyword with variables, methods, blocks and nested classes.
The main purpose of using static keyword is in the case when we want to share the same variable or method of a given class without creating their instances.
To declare such variable or methods prefix the static keyword with the variable name or method name.
If a member is declared as static, that means it can be accessed before the creation of any object of that class and without need of any object reference.
The best example of static keyword is the main(). We declare it as static so that it must be called before the existence of any object.
One more advantage of using static is that if any variable is declared using static then no copy of static variable is made separately for the created objects. Instead all the objects of the class use the same static variable.
Besides this their few restrictions in case of static method:
● They can call only other static methods and not any other type of methods.
● Only static data can access them.
● They cannot use this or super keyword.
Example
Class DemoStatic {
Static int x = 4; //static variable
Static int y;
Static void abc(int i) { //static method
System.out.println("i = " + i);
System.out.println("x = " + x);
System.out.println("y = " + y);
}
Static { //static block
System.out.println(“Example of static block initialized”);
y = x * 4;
}
Public static void main(String args[]) {
Abc(8);
}
}
Output
Example of static block initialized.
i = 8
x = 4
y = 16
To use a static method outside a class, following syntax will be used:
Classname.methodname()
This Keyword
This keyword is a reference variable that refers to current object. It can be used in number of scenarios.
- Used to refer instance variable of the current class
- can be used in a constructor
- can be passed as an argument in the method call and constructor call
- can be used to invoke current class constructor
Program:
Class thisDemo
{
Int i;
Int j;
// Parameterized constructor
ThisDemo(int i, int j)
{
This.i = i;
This.j = j;
}
Void display()
{
System.out.println("i=" +i);
System.out.println("j=" +j);
}
Public static void main(String[] args)
{
ThisDemo object = new thisDemo(10, 20);
Object.display();
}
}
Output:
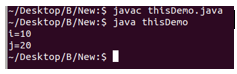
Key takeaway
This keyword is a reference variable that refers to current object. It can be used in number of scenarios.
Static keyword in Java is mainly used for memory management. We can use static keyword with variables, methods, blocks and nested classes.
Finalize() is the method of Object class. This method is called just before an object is garbage collected. Finalize() method overrides to dispose system resources, perform clean-up activities and minimize memory leaks.
Syntax
- Protected void finalize() throws Throwable
Throw
Throwable - the Exception is raised by this method
Example 1
- Public class JavafinalizeExample1 {
- Public static void main(String[] args)
- {
- JavafinalizeExample1 obj = new JavafinalizeExample1();
- System.out.println(obj.hashCode());
- Obj = null;
- // calling garbage collector
- System.gc();
- System.out.println("end of garbage collection");
- }
- @Override
- Protected void finalize()
- {
- System.out.println("finalize method called");
- }
- }
Output:
2018699554
End of garbage collection
Finalize method called
Key takeaway
Finalize() is the method of Object class. This method is called just before an object is garbage collected. Finalize() method overrides to dispose system resources, perform clean-up activities and minimize memory leaks.
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers. They are as follows:
● public - A public modifier's access level is visible everywhere. It can be accessible from within and outside the class, from within and outside the package, and from within and outside the package.
● protected - A protected modifier's access level is both within the package and outside the package via a child class. The child class cannot be accessed from outside the package unless it is created.
● default - A default modifier's access level is limited to the package. It's not possible to get to it from outside the package. If you don't indicate an access level, the default will be used.
● private - A private modifier's access level is limited to the class. It isn't accessible outside of the class.
The access modifier for that member (variable or method) will be default if it is not declared as public, protected, or private. Access modifiers can also be applied to classes.
Private is the most restrictive of the four access modifiers, whereas public is the least restrictive. The following is the syntax for declaring an access modifier:
Access-modifier data-type variable-name;
Java Access Modifiers
Let's look at a basic table to see how access modifiers work in Java.
Access Modifier | Within class | Within package | Outside package by subclass only | Outside package |
Private | Y | N | N | N |
Default | Y | Y | N | N |
Protected | Y | Y | Y | N |
Public | Y | Y | Y | Y |
Private
Only members of the class have access to the private access modifier.
An example of a private access modifier in action
We've built two classes in this example: A and Simple. Private data members and private methods are found in a class. There is a compile-time problem since we are accessing these secret members from outside the class.
Class A{
Private int data=40;
Private void msg(){System.out.println("Hello java");}
}
Public class Simple{
Public static void main(String args[]){
A obj=new A();
System.out.println(obj.data);//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Default
If you don't specify a modifier, it will be treated as default. The default modifier is only available within the package. It's not possible to get to it from outside the package. It allows for more accessibility than a private setting. It is, nevertheless, more limited than protected and open.
A default access modifier is one example.
We've produced two packages in this example: pack and mypack. We're accessing A from outside its package because A isn't public and so can't be accessed from outside the package.
//save by A.java
Package pack;
Class A{
Void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();//Compile Time Error
Obj.msg();//Compile Time Error
}
}
Protected
The protected access modifier can be used both inside and outside of the package, but only through inheritance.
The data member, method, and function Object() { [native code] } can all have the protected access modifier applied to them. It is not applicable to the class.
It is more accessible than the standard modifier.
A protected access modifier is an example.
We've produced two packages in this example: pack and mypack. The public A class of the pack package can be accessed from outside the package. However, because this package's msg function is protected, it can only be accessible from outside the class through inheritance.
//save by A.java
Package pack;
Public class A{
Protected void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B extends A{
Public static void main(String args[]){
B obj = new B();
Obj.msg();
}
}
Output:Hello
Public
The public access modifier can be used everywhere. Among all the modifiers, it has the broadest application.
A public access modifier is an example.
//save by A.java
Package pack;
Public class A{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
Output: Hello
Key takeaway
Access control is a method, or encapsulation attribute, that limits the access of particular members of a class to specific areas of a programme. The access modifiers can be used to restrict access to members of a class. In Java, there are four access modifiers.
Basically, there are two types of modifiers in Java, access modifiers and non-access modifiers.
In Java there are four types of access modifiers according to the level of access required for classes, variable, methods and constructors. Access modifiers like public, private, protected and default.
Default Access Modifier
In this access modifier we do not specify any modifier for a class, variable, method and constructor.
Such types of variables or method can be used by any other class. There are no restrictions in this access modifier.
There is no syntax for default modifier as declare the variables and methods without any modifier keyword.
Example-
String name = “Disha”;
Boolean processName() {
Return true;
}
Private Access Modifier
This access modifier uses the private keyword. By using this access modifier the methods, variable and constructors become private and can only be accessed by the member of that same class.
It is the most restrict access specifier. Class and interface cannot be declared using the private keyword.
By using private keyword we can implement the concept of encapsulation, which hides data from the outside world.
Example of private access modifier
Public class ExampleAcces {
Private String ID;
Public String getID() {
Return this.ID;
}
Public void setID(String ID) {
This.ID = ID;
}
}
In the above example the ID variable of the ExampleAccess class is private, so no other class can retrieve or set its value easily.
So, to access this variable for use in the outside world, we defined two public methods: getID(), which returns the value of ID, and setID(String), which sets its value.
Public Access Modifier
As the name suggest a public access modifier can be easily accessed by any other class or methods.
Whenever we specify public keyword to a class, method, constructor etc, then they can be accessed from any other class in the same package. Outside package we have to import that class for access because of the concept of inheritance.
In the below example, function uses public access modifier:
Public static void main(String[] arguments) {
// ...
}
It is necessary to declare the main method as public, else Java interpreter will not be able to called it to run the class.
Protected Access Modifier
In this access modifier, in a superclass if variables, methods and constructors are declared as protected then only the subclass in other package or any class within the same package can access it.
It cannot be assigned to class and interfaces. In general method’s fields can be declared as protected: however methods and fields of interface cannot be defined as protected.
It cannot be defined on the class.
An example of protected access modifier is shown below:
Class Person {
Protected int ID(ID pid) {
// implementation details
}
}
Class Employee extends Person {
Int ID(ID pid) {
// implementation details
}
}
Tips for Access Modifier
- A public method declared in a superclass, must also be public in all subclasses.
- In a superclass if a method is declared as protected, then in subclass it should be either protected or public, they cannot be private.
- Private methods cannot be inherited.
Key takeaway
Basically, there are two types of modifiers in Java, access modifiers and non-access modifiers.
Inner Classes (Nested Classes)
● Java inner class or nested class is a class that is declared inside the class or interface.
● We use inner classes to logically group classes and interfaces in one place to be more readable and maintainable.
● it can access all the members of the outer class, including private data members and methods.
Syntax of Inner class
Class Java_Outer_class
{ //code
Class Java_Inner_class
{ //code }
}
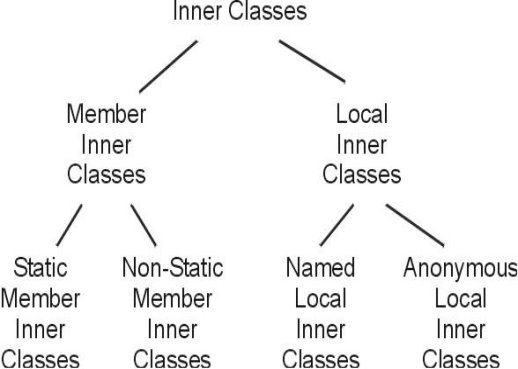
Advantage of Java inner classes
- Nested classes represent a particular type of relationship that is it can access all the members (data members and methods) of the outer class, including private.
- Nested classes are used to develop more readable and maintainable code because it logically group classes and interfaces in one place only.
- Code Optimization: It requires less code to write.
Need of Java Inner class
● Sometimes users need to program a class in such a way so that no other class can access it.
● Therefore, it would be better if you include it within other classes.
● If all the class objects are a part of the outer object then it is easier to nest that class inside the outer class.
● That way all the outer class can access all the objects of the inner class.
Types of Nested classes
There are two types of nested classes non-static and static nested classes. The non-static nested classes are also known as inner classes.
- Non-static nested class (inner class)
- Member inner class
- Anonymous inner class
- Local inner class
- Static nested class
Type | Description |
Member Inner Class | A class created within class and outside method. |
Anonymous Inner Class | A class created for implementing an interface or extending class. The java compiler decides its name. |
Local Inner Class | A class was created within the method. |
Static Nested Class | A static class was created within the class. |
Nested Interface | An interface created within class or interface. |
Java Inner Classes
Java inner class or nested class is a class which is declared inside the class or interface.
We use inner classes to logically group classes and interfaces in one place so that it can be more readable and maintainable.
Additionally, it can access all the members of outer class including private data members and methods.
Syntax of Inner class
- Class Java_Outer_class{
- //code
- Class Java_Inner_class{
- //code
- }
- }
Advantage of java inner classes
There are basically three advantages of inner classes in java. They are as follows:
1) Nested classes represent a special type of relationship that is it can access all the members (data members and methods) of outer class including private.
2) Nested classes are used to develop more readable and maintainable code because it logically group classes and interfaces in one place only.
3) Code Optimization: It requires less code to write.
Do You Know
● What is the internal code generated by the compiler for member inner class?
● What are the two ways to create anonymous inner class?
● Can we access the non-final local variable inside the local inner class?
● How to access the static nested class?
● Can we define an interface within the class?
● Can we define a class within the interface?
Difference between nested class and inner class in Java
Inner class is a part of nested class. Non-static nested classes are known as inner classes.
Types of Nested classes
There are two types of nested classes non-static and static nested classes.The non-static nested classes are also known as inner classes.
● Non-static nested class (inner class)
- Member inner class
- Anonymous inner class
- Local inner class
● Static nested class
Type | Description |
Member Inner Class | A class created within class and outside method. |
Anonymous Inner Class | A class created for implementing interface or extending class. Its name is decided by the java compiler. |
Local Inner Class | A class created within method. |
Static Nested Class | A static class created within class. |
Nested Interface | An interface created within class or interface. |
Java provides a number of access modifiers to set access levels for classes, variables, methods, and constructors. The four access levels are −
● Visible to the package, the default. No modifiers are needed.
● Visible to the class only (private).
● Visible to the world (public).
● Visible to the package and all subclasses (protected).
Key takeaway
Java inner class or nested class is a class that is declared inside the class or interface.
There are two types of nested classes non-static and static nested classes. The non-static nested classes are also known as inner classes.
- Java anonymous inner class is an inner class without a name and for which only a single object is created.
- An anonymous inner class can be useful when making an instance of an object with certain "extras" such as overloading methods of a class or interface, without having to actually subclass a class.
- In simple words, a class that has no name is known as an anonymous inner class in Java. It should be used if you have to override a method of class or interface. Java Anonymous inner class can be created in two ways:
- Class (may be abstract or concrete).
- Interface
Java anonymous inner class example using class
TestAnonymousInner.java
Abstract class Person
{
Abstract void eat();
}
Class TestAnonymousInner
{
Public static void main(String args[])
{
Person p=new Person(){
Void eat()
{ System.out.println("nice fruits"); }
};
p.eat();
}
}
OUTPUT:
Nice fruit
Key takeaway
Java anonymous inner class is an inner class without a name and for which only a single object is created.
● Abstract classes are the same as normal Java classes the difference is only that an abstract class uses abstract keyword while the normal Java class does not use.
● We use the abstract keyword before the class name to declare the class as abstract.
● An abstract class contains abstract methods as well as concrete methods.
● If we want to use an abstract class, we have to inherit it from the base class.
● If the class does not have the implementation of all the methods of the interface, we should declare the class as abstract. It provides complete abstraction. It means that fields are public static and final by default and methods are empty.
The syntax of abstract class is:
Public abstract class ClassName
{
Public abstract methodName();
}
Example of car:
Abstract class Car
{ //abstract method
Abstract void start();
//non-abstract method
Public void stop()
{
System.out.println("The car engine is not started.");
}
} //inherit abstract class
Public class Owner extends Car
{ //defining the body of the abstract method of the abstract class
Void start()
{
System.out.println("The car engine has been started.");
}
Public static void main(String[] args)
{ Owner obj = new Owner();
//calling abstract method
Obj.start();
//calling non-abstract method
Obj.stop();
}
}
OUTPUT:
The car has been started.
The car engine is not started.
Abstract Method
The method that does not has method body is known as abstract method. In other words, without an implementation is known as abstract method. It always declares in the abstract class. It means the class itself must be abstract if it has abstract method. To create an abstract method, we use the keyword abstract.
Syntax
- Abstract void method_name();
Example of abstract method
Demo.java
- Abstract class Demo //abstract class
- {
- //abstract method declaration
- Abstract void display();
- }
- Public class MyClass extends Demo
- {
- //method implementation
- Void display()
- {
- System.out.println("Abstract method?");
- }
- Public static void main(String args[])
- {
- //creating object of abstract class
- Demo obj = new MyClass();
- //invoking abstract method
- Obj.display();
- }
- }
Output:
Abstract method...
Key takeaway
Abstract classes are the same as normal Java classes the difference is only that an abstract class uses abstract keyword while the normal Java class does not use.
References:
- Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
- CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
- JAVA Complete Reference (9th Edition) Herbert Schelidt.
- T. Budd, “Understanding Object- Oriented Programming with Java”, Pearson Education, Updated Edition (New Java 2 Coverage), 1999.