Unit - 4
Interfaces and Packages
An interface is a reference type in Java. It is similar to class. It is a collection of abstract methods. A class implements an interface, thereby inheriting the abstract methods of the interface.
Along with abstract methods, an interface may also contain constants, default methods, static methods, and nested types. Method bodies exist only for default methods and static methods.
Writing an interface is similar to writing a class. But a class describes the attributes and behaviors of an object. And an interface contains behaviors that a class implements.
Unless the class that implements the interface is abstract, all the methods of the interface need to be defined in the class.
An interface is similar to a class in the following ways −
● An interface can contain any number of methods.
● An interface is written in a file with a .java extension, with the name of the interface matching the name of the file.
● The byte code of an interface appears in a .class file.
● Interfaces appear in packages, and their corresponding bytecode file must be in a directory structure that matches the package name.
However, an interface is different from a class in several ways, including −
● You cannot instantiate an interface.
● An interface does not contain any constructors.
● All of the methods in an interface are abstract.
● An interface cannot contain instance fields. The only fields that can appear in an interface must be declared both static and final.
● An interface is not extended by a class; it is implemented by a class.
● An interface can extend multiple interfaces.
Features of Interface:
- We can achieve total abstraction.
- We can use multiple interfaces in a class that leads to multiple inheritance.
- It also helps to achieve loose coupling.
Syntax
Public interface XYZ
{
Public void method();
}
To use an interface in a class, Java provides a keyword called implements. We provide the necessary implementation of the method that we have declared in the interface.
Both abstract classes and interfaces are used to achieve abstraction so that abstract methods can be declared. Both the abstract class and the interface cannot be instantiated.
However, there are other distinctions between abstract class and interface, which are shown below.
Abstract class | Interface |
1) There are abstract and non-abstract methods in an abstract class. | Only abstract methods are allowed in an interface. It can also contain default and static methods with Java 8. |
2) Multiple inheritance is not supported by abstract classes. | Multiple inheritance is supported by the interface. |
3) Variables in an abstract class can be final, non-final, static, or non-static. | Only static and final variables are used in the interface. |
4) Interface implementation can be provided via an abstract class. | The implementation of an abstract class cannot be provided by an interface. |
5) To declare an abstract class, use the abstract keyword. | To declare an interface, use the interface keyword. |
6) An abstract class can implement numerous Java interfaces by extending another Java class. | Only another Java interface can be extended by an interface. |
7) The keyword "extends" can be used to extend an abstract class. | The keyword "implements" can be used to create an interface. |
8) Private, protected, and other class members can be found in a Java abstract class. | By default, members of a Java interface are public. |
9)Example: Public abstract class Shape{ Public abstract void draw(); } | Example: Public interface Drawable{ Void draw(); } |
Declaring Interfaces
The interface keyword is used to declare an interface. Here is a simple example to declare an interface −
Example
Following is an example of an interface −
/* File name : NameOfInterface.java */
Import java.lang.*;
// Any number of import statements
Public interface NameOfInterface {
// Any number of final, static fields
// Any number of abstract method declarations\
}
Interfaces have the following properties −
● An interface is implicitly abstract. You do not need to use the abstract keyword while declaring an interface.
● Each method in an interface is also implicitly abstract, so the abstract keyword is not needed.
● Methods in an interface are implicitly public.
Example
/* File name : Animal.java */
Interface Animal {
Public void eat();
Public void travel();
}
Implementing Interfaces
When a class implements an interface, you can think of the class as signing a contract, agreeing to perform the specific behaviors of the interface. If a class does not perform all the behaviors of the interface, the class must declare itself as abstract.
A class uses the implements keyword to implement an interface. The implements keyword appears in the class declaration following the extends portion of the declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
Public void eat() {
System.out.println("Mammal eats");
}
Public void travel() {
System.out.println("Mammal travels");
}
Public int noOfLegs() {
Return 0;
}
Public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
This will produce the following result −
Output
Mammal eats
Mammal travels
When overriding methods defined in interfaces, there are several rules to be followed −
● Checked exceptions should not be declared on implementation methods other than the ones declared by the interface method or subclasses of those declared by the interface method.
● The signature of the interface method and the same return type or subtype should be maintained when overriding the methods.
● An implementation class itself can be abstract and if so, interface methods need not be implemented.
When implementation interfaces, there are several rules −
● A class can implement more than one interface at a time.
● A class can extend only one class, but implement many interfaces.
● An interface can extend another interface, in a similar way as a class can extend another class.
Extending Interfaces
An interface can extend another interface in the same way that a class can extend another class. The extends keyword is used to extend an interface, and the child interface inherits the methods of the parent interface.
The following Sports interface is extended by Hockey and Football interfaces.
Example
// Filename: Sports.java
Public interface Sports {
Public void setHomeTeam(String name);
Public void setVisitingTeam(String name);
}
// Filename: Football.java
Public interface Football extends Sports {
Public void homeTeamScored(int points);
Public void visitingTeamScored(int points);
Public void endOfQuarter(int quarter);
}
// Filename: Hockey.java
Public interface Hockey extends Sports {
Public void homeGoalScored();
Public void visitingGoalScored();
Public void endOfPeriod(int period);
Public void overtimePeriod(int ot);
}
The Hockey interface has four methods, but it inherits two from Sports; thus, a class that implements Hockey needs to implement all six methods. Similarly, a class that implements Football needs to define the three methods from Football and the two methods from Sports.
Extending Multiple Interfaces
A Java class can only extend one parent class. Multiple inheritance is not allowed. Interfaces are not classes, however, and an interface can extend more than one parent interface.
The extends keyword is used once, and the parent interfaces are declared in a comma-separated list.
For example, if the Hockey interface extended both Sports and Event, it would be declared as −
Example
Public interface Hockey extends Sports, Event
Key takeaway
An interface is a reference type in Java. It is similar to class. It is a collection of abstract methods. A class implements an interface, thereby inheriting the abstract methods of the interface.
Along with abstract methods, an interface may also contain constants, default methods, static methods, and nested types. Method bodies exist only for default methods and static methods.
Writing an interface is similar to writing a class. But a class describes the attributes and behaviors of an object. And an interface contains behaviors that a class implements.
Variables can be declared as object references that use an interface instead of a class.
Such a variable can refer to any instance that implements the stated interface.
When you use one of these references to invoke a method, the right version is used based on the actual instance of the interface being referred to.
This is one of the most important characteristics of interfaces. The method to be executed is dynamically looked up at runtime, allowing classes to be built after the code that calls methods on them.
This is analogous to accessing a subclass object using a superclass reference.
The callback() function is invoked in the following example using an interface reference variable:
Interface Callback {
Void callback(int param);
}
Class Client implements Callback {
// Implement Callback's interface
Public void callback(int p) {
System.out.println("callback called with " + p);
}
Void aa() {
System.out.println("hi.");
}
}
Public class Main{
Public static void main(String args[]) {
Callback c = new Client();
c.callback(42);
}
}
The following is the program's output:
Callback called with 42
Despite the fact that the variable c is of the interface type Callback, it has been assigned an instance of Client.
The callback() method can be used using c, but it cannot access any other members of the Client class.
Only the methods declared by the interface declaration are known to an interface reference variable.
As a result, because aa() is defined by Client but not Callback, c could not be used to access it.
Key takeaway
Variables can be declared as object references that use an interface instead of a class type.
● A java package is a group of similar types of classes, interfaces and sub-packages.
● Package in java can be categorized in two form, built-in package and user-defined package.
● There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
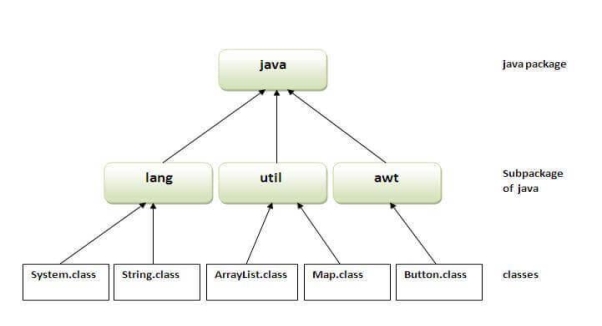
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
Javac -d directory javafilename
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
Simple example of java package
The package keyword is used to create a package in java
//save as Simple.java
Package mypack;
Public class Simple
{
Public static void main(String args[]){
System.out.println("Welcome to package");
}
}
To Compile: javac -d. Simple.java
To Run: java mypack.Simple
The -d is a switch that tells the compiler where to put the class file i.e. it represents destination. The . Represents the current folder.
OUTPUT: Welcome to package
Accessing Packages
There are three ways to access the package from outside the package.
- Import package.*;
- Import package.classname;
- Fully qualified name.
1) Using packagename.*
- If you use package.* then all the classes and interfaces of this package will be accessible but not subpackages.
- The import keyword is used to make the classes and interface of another package accessible to the current package.
Example of package that import the packagename.*
//save by A.java
Package pack;
Public class A {
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[])
{
A obj = new A();
Obj.msg();
}
}
OUTPUT: Hello
2) Using packagename.classname
If you import package.class \name then only declared class of this package will be accessible.
Example of package by import package.classname
//save by A.java
Package pack;
Public class A
{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.A;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
OUTPUT: Hello
Using fully qualified name
● If we use fully qualified name then only declared class of this package will be accessible. Now there is no need to import.
● But we need to use fully qualified name every time when you are accessing the class or interface.
● It is generally used when two packages have same class name e.g. Java.util and java.sql packages contain Date class.
Example of package by import fully qualified name
//save by A.java
Package pack;
Public class A
{
Public void msg()
{ System.out.println("Hello"); }
}
//save by B.java
Package mypack;
Class B{
Public static void main(String args[]){
Pack.A obj = new pack.A();//using fully qualified name
Obj.msg();
}
}
OUTPUT: Hello
Note: If you import a package, subpackages will not be imported.
If you import a package, all the classes and interface of that package will be imported excluding the classes and interfaces of the subpackages. Hence, you need to import the subpackage as well.
Sequence of the program must be package then import then class.
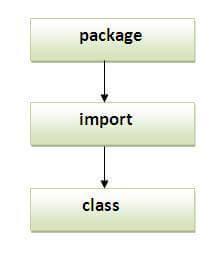
Key takeaway
A java package is a group of similar types of classes, interfaces and sub-packages. Package in java can be categorized in two form, built-in package and user-defined package. There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc. Here, we will have the detailed learning of creating and using user-defined packages.
CLASSPATH: CLASSPATH is an environment variable which is used by Application ClassLoader to locate and load the .class files. The CLASSPATH defines the path, to find third-party and user-defined classes that are not extensions or part of Java platform. Include all the directories which contain .class files and JAR files when setting the CLASSPATH.
You need to set the CLASSPATH if:
● You need to load a class that is not present in the current directory or any sub-directories.
● You need to load a class that is not in a location specified by the extensions mechanism.
The CLASSPATH depends on what you are setting the CLASSPATH. The CLASSPATH has a directory name or file name at the end. The following points describe what should be the end of the CLASSPATH.
● If a JAR or zip, the file contains class files, the CLASSPATH end with the name of the zip or JAR file.
● If class files placed in an unnamed package, the CLASSPATH ends with the directory that contains the class files.
● If class files placed in a named package, the CLASSPATH ends with the directory that contains the root package in the full package name, that is the first package in the full package name.
The default value of CLASSPATH is a dot (.). It means the only current directory searched. The default value of CLASSPATH overrides when you set the CLASSPATH variable or using the -classpath command (for short -cp). Put a dot (.) in the new setting if you want to include the current directory in the search path.
If CLASSPATH finds a class file which is present in the current directory, then it will load the class and use it, irrespective of the same name class presents in another directory which is also included in the CLASSPATH.
If you want to set multiple classpaths, then you need to separate each CLASSPATH by a semicolon (;).
The third-party applications (MySQL and Oracle) that use the JVM can modify the CLASSPATH environment variable to include the libraries they use. The classes can be stored in directories or archives files. The classes of the Java platform are stored in rt.jar.
There are two ways to ways to set CLASSPATH: through Command Prompt or by setting Environment Variable.
Let's see how to set CLASSPATH of MySQL database:
Step 1: Click on the Windows button and choose Control Panel. Select System.
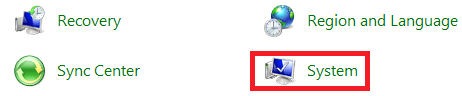
Step 2: Click on Advanced System Settings.
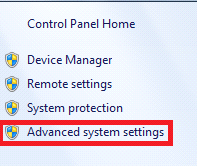
Step 3: A dialog box will open. Click on Environment Variables.
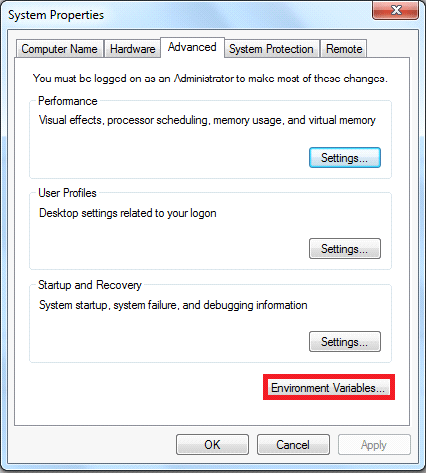
Step 4: If the CLASSPATH already exists in System Variables, click on the Edit button then put a semicolon (;) at the end. Paste the Path of MySQL-Connector Java.jar file.
If the CLASSPATH doesn't exist in System Variables, then click on the New button and type Variable name as CLASSPATH and Variable value as C:\Program Files\Java\jre1.8\MySQL-Connector Java.jar;.;
Remember: Put ;.; at the end of the CLASSPATH.
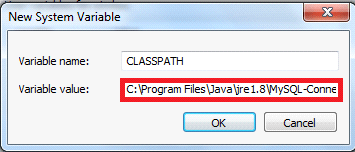
How to Set CLASSPATH in Windows Using Command Prompt
Type the following command in your Command Prompt and press enter.
Set CLASSPATH=%CLASSPATH%;C:\Program Files\Java\jre1.8\rt.jar;
In the above command, The set is an internal DOS command that allows the user to change the variable value. CLASSPATH is a variable name. The variable enclosed in percentage sign (%) is an existing environment variable. The semicolon is a separator, and after the (;) there is the PATH of rt.jar file.
How ext folder works in Java
The ext directory works a bit like the CLASSPATH. Ext directory is the part of the class loading mechanism. The classes which are available within JARs in the ext directory are available to Java applications.
Key takeaway
CLASSPATH is an environment variable which is used by Application ClassLoader to locate and load the .class files. The CLASSPATH defines the path, to find third-party and user-defined classes that are not extensions or part of Java platform. Include all the directories which contain .class files and JAR files when setting the CLASSPATH.
Java Packages & API
A package in Java is used to group related classes. Think of it as a folder in a file directory. We use packages to avoid name conflicts, and to write a better maintainable code. Packages are divided into two categories:
● Built-in Packages (packages from the Java API)
● User-defined Packages (create your own packages)
Built-in Packages
The Java API is a library of prewritten classes, that are free to use, included in the Java Development Environment.
The library contains components for managing input, database programming, and much more.
The library is divided into packages and classes. Meaning you can either import a single class (along with its methods and attributes), or a whole package that contain all the classes that belong to the specified package.
To use a class or a package from the library, you need to use the import keyword:
Syntax
Import package.name.Class; // Import a single class
Import package.name.*; // Import the whole package
Import a Class
If you find a class you want to use, for example, the Scanner class, which is used to get user input, write the following code:
Example
Import java.util.Scanner;
In the example above, java.util is a package, while Scanner is a class of the java.util package.
To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read a complete line:
Example
Using the Scanner class to get user input:
Import java.util.Scanner;
Class MyClass {
Public static void main(String[] args) {
Scanner myObj = new Scanner(System.in);
System.out.println("Enter username");
String userName = myObj.nextLine();
System.out.println("Username is: " + userName);
}
}
Import a Package
There are many packages to choose from. In the previous example, we used the Scanner class from the java.util package. This package also contains date and time facilities, random-number generator and other utility classes.
To import a whole package, end the sentence with an asterisk sign (*). The following example will import ALL the classes in the java.util package:
Example
Import java.util.*;
User-defined Packages
To create your own package, you need to understand that Java uses a file system directory to store them. Just like folders on your computer:
Example
└── root
└── mypack
└── MyPackageClass.java
To create a package, use the package keyword:
MyPackageClass.java
Package mypack;
Class MyPackageClass {
Public static void main(String[] args) {
System.out.println("This is my package!");
}
}
Save the file as MyPackageClass.java, and compile it:
C:\Users\Your Name>javac MyPackageClass.java
Then compile the package:
C:\Users\Your Name>javac -d . MyPackageClass.java
This forces the compiler to create the "mypack" package.
The -d keyword specifies the destination for where to save the class file. You can use any directory name, like c:/user (windows), or, if you want to keep the package within the same directory, you can use the dot sign ".", like in the example above.
Note: The package name should be written in lower case to avoid conflict with class names.
When we compiled the package in the example above, a new folder was created, called "mypack".
To run the MyPackageClass.java file, write the following:
C:\Users\Your Name>java mypack.MyPackageClass
The output will be:
This is my package!
Key takeaway
A package in Java is used to group related classes. Think of it as a folder in a file directory. We use packages to avoid name conflicts, and to write a better maintainable code. Packages are divided into two categories:
● Built-in Packages (packages from the Java API)
● User-defined Packages (create your own packages)
References:
- Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
- CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
- JAVA Complete Reference (9th Edition) Herbert Schelidt.
- T. Budd, “Understanding Object- Oriented Programming with Java”, Pearson Education, Updated Edition (New Java 2 Coverage), 1999.
Unit - 4
Interfaces and Packages
An interface is a reference type in Java. It is similar to class. It is a collection of abstract methods. A class implements an interface, thereby inheriting the abstract methods of the interface.
Along with abstract methods, an interface may also contain constants, default methods, static methods, and nested types. Method bodies exist only for default methods and static methods.
Writing an interface is similar to writing a class. But a class describes the attributes and behaviors of an object. And an interface contains behaviors that a class implements.
Unless the class that implements the interface is abstract, all the methods of the interface need to be defined in the class.
An interface is similar to a class in the following ways −
● An interface can contain any number of methods.
● An interface is written in a file with a .java extension, with the name of the interface matching the name of the file.
● The byte code of an interface appears in a .class file.
● Interfaces appear in packages, and their corresponding bytecode file must be in a directory structure that matches the package name.
However, an interface is different from a class in several ways, including −
● You cannot instantiate an interface.
● An interface does not contain any constructors.
● All of the methods in an interface are abstract.
● An interface cannot contain instance fields. The only fields that can appear in an interface must be declared both static and final.
● An interface is not extended by a class; it is implemented by a class.
● An interface can extend multiple interfaces.
Features of Interface:
- We can achieve total abstraction.
- We can use multiple interfaces in a class that leads to multiple inheritance.
- It also helps to achieve loose coupling.
Syntax
Public interface XYZ
{
Public void method();
}
To use an interface in a class, Java provides a keyword called implements. We provide the necessary implementation of the method that we have declared in the interface.
Both abstract classes and interfaces are used to achieve abstraction so that abstract methods can be declared. Both the abstract class and the interface cannot be instantiated.
However, there are other distinctions between abstract class and interface, which are shown below.
Abstract class | Interface |
1) There are abstract and non-abstract methods in an abstract class. | Only abstract methods are allowed in an interface. It can also contain default and static methods with Java 8. |
2) Multiple inheritance is not supported by abstract classes. | Multiple inheritance is supported by the interface. |
3) Variables in an abstract class can be final, non-final, static, or non-static. | Only static and final variables are used in the interface. |
4) Interface implementation can be provided via an abstract class. | The implementation of an abstract class cannot be provided by an interface. |
5) To declare an abstract class, use the abstract keyword. | To declare an interface, use the interface keyword. |
6) An abstract class can implement numerous Java interfaces by extending another Java class. | Only another Java interface can be extended by an interface. |
7) The keyword "extends" can be used to extend an abstract class. | The keyword "implements" can be used to create an interface. |
8) Private, protected, and other class members can be found in a Java abstract class. | By default, members of a Java interface are public. |
9)Example: Public abstract class Shape{ Public abstract void draw(); } | Example: Public interface Drawable{ Void draw(); } |
Declaring Interfaces
The interface keyword is used to declare an interface. Here is a simple example to declare an interface −
Example
Following is an example of an interface −
/* File name : NameOfInterface.java */
Import java.lang.*;
// Any number of import statements
Public interface NameOfInterface {
// Any number of final, static fields
// Any number of abstract method declarations\
}
Interfaces have the following properties −
● An interface is implicitly abstract. You do not need to use the abstract keyword while declaring an interface.
● Each method in an interface is also implicitly abstract, so the abstract keyword is not needed.
● Methods in an interface are implicitly public.
Example
/* File name : Animal.java */
Interface Animal {
Public void eat();
Public void travel();
}
Implementing Interfaces
When a class implements an interface, you can think of the class as signing a contract, agreeing to perform the specific behaviors of the interface. If a class does not perform all the behaviors of the interface, the class must declare itself as abstract.
A class uses the implements keyword to implement an interface. The implements keyword appears in the class declaration following the extends portion of the declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
Public void eat() {
System.out.println("Mammal eats");
}
Public void travel() {
System.out.println("Mammal travels");
}
Public int noOfLegs() {
Return 0;
}
Public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
This will produce the following result −
Output
Mammal eats
Mammal travels
When overriding methods defined in interfaces, there are several rules to be followed −
● Checked exceptions should not be declared on implementation methods other than the ones declared by the interface method or subclasses of those declared by the interface method.
● The signature of the interface method and the same return type or subtype should be maintained when overriding the methods.
● An implementation class itself can be abstract and if so, interface methods need not be implemented.
When implementation interfaces, there are several rules −
● A class can implement more than one interface at a time.
● A class can extend only one class, but implement many interfaces.
● An interface can extend another interface, in a similar way as a class can extend another class.
Extending Interfaces
An interface can extend another interface in the same way that a class can extend another class. The extends keyword is used to extend an interface, and the child interface inherits the methods of the parent interface.
The following Sports interface is extended by Hockey and Football interfaces.
Example
// Filename: Sports.java
Public interface Sports {
Public void setHomeTeam(String name);
Public void setVisitingTeam(String name);
}
// Filename: Football.java
Public interface Football extends Sports {
Public void homeTeamScored(int points);
Public void visitingTeamScored(int points);
Public void endOfQuarter(int quarter);
}
// Filename: Hockey.java
Public interface Hockey extends Sports {
Public void homeGoalScored();
Public void visitingGoalScored();
Public void endOfPeriod(int period);
Public void overtimePeriod(int ot);
}
The Hockey interface has four methods, but it inherits two from Sports; thus, a class that implements Hockey needs to implement all six methods. Similarly, a class that implements Football needs to define the three methods from Football and the two methods from Sports.
Extending Multiple Interfaces
A Java class can only extend one parent class. Multiple inheritance is not allowed. Interfaces are not classes, however, and an interface can extend more than one parent interface.
The extends keyword is used once, and the parent interfaces are declared in a comma-separated list.
For example, if the Hockey interface extended both Sports and Event, it would be declared as −
Example
Public interface Hockey extends Sports, Event
Key takeaway
An interface is a reference type in Java. It is similar to class. It is a collection of abstract methods. A class implements an interface, thereby inheriting the abstract methods of the interface.
Along with abstract methods, an interface may also contain constants, default methods, static methods, and nested types. Method bodies exist only for default methods and static methods.
Writing an interface is similar to writing a class. But a class describes the attributes and behaviors of an object. And an interface contains behaviors that a class implements.
Variables can be declared as object references that use an interface instead of a class.
Such a variable can refer to any instance that implements the stated interface.
When you use one of these references to invoke a method, the right version is used based on the actual instance of the interface being referred to.
This is one of the most important characteristics of interfaces. The method to be executed is dynamically looked up at runtime, allowing classes to be built after the code that calls methods on them.
This is analogous to accessing a subclass object using a superclass reference.
The callback() function is invoked in the following example using an interface reference variable:
Interface Callback {
Void callback(int param);
}
Class Client implements Callback {
// Implement Callback's interface
Public void callback(int p) {
System.out.println("callback called with " + p);
}
Void aa() {
System.out.println("hi.");
}
}
Public class Main{
Public static void main(String args[]) {
Callback c = new Client();
c.callback(42);
}
}
The following is the program's output:
Callback called with 42
Despite the fact that the variable c is of the interface type Callback, it has been assigned an instance of Client.
The callback() method can be used using c, but it cannot access any other members of the Client class.
Only the methods declared by the interface declaration are known to an interface reference variable.
As a result, because aa() is defined by Client but not Callback, c could not be used to access it.
Key takeaway
Variables can be declared as object references that use an interface instead of a class type.
● A java package is a group of similar types of classes, interfaces and sub-packages.
● Package in java can be categorized in two form, built-in package and user-defined package.
● There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
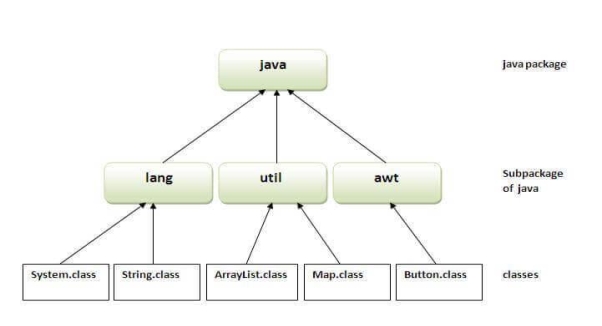
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
Javac -d directory javafilename
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
Simple example of java package
The package keyword is used to create a package in java
//save as Simple.java
Package mypack;
Public class Simple
{
Public static void main(String args[]){
System.out.println("Welcome to package");
}
}
To Compile: javac -d. Simple.java
To Run: java mypack.Simple
The -d is a switch that tells the compiler where to put the class file i.e. it represents destination. The . Represents the current folder.
OUTPUT: Welcome to package
Accessing Packages
There are three ways to access the package from outside the package.
- Import package.*;
- Import package.classname;
- Fully qualified name.
1) Using packagename.*
- If you use package.* then all the classes and interfaces of this package will be accessible but not subpackages.
- The import keyword is used to make the classes and interface of another package accessible to the current package.
Example of package that import the packagename.*
//save by A.java
Package pack;
Public class A {
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[])
{
A obj = new A();
Obj.msg();
}
}
OUTPUT: Hello
2) Using packagename.classname
If you import package.class \name then only declared class of this package will be accessible.
Example of package by import package.classname
//save by A.java
Package pack;
Public class A
{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.A;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
OUTPUT: Hello
Using fully qualified name
● If we use fully qualified name then only declared class of this package will be accessible. Now there is no need to import.
● But we need to use fully qualified name every time when you are accessing the class or interface.
● It is generally used when two packages have same class name e.g. Java.util and java.sql packages contain Date class.
Example of package by import fully qualified name
//save by A.java
Package pack;
Public class A
{
Public void msg()
{ System.out.println("Hello"); }
}
//save by B.java
Package mypack;
Class B{
Public static void main(String args[]){
Pack.A obj = new pack.A();//using fully qualified name
Obj.msg();
}
}
OUTPUT: Hello
Note: If you import a package, subpackages will not be imported.
If you import a package, all the classes and interface of that package will be imported excluding the classes and interfaces of the subpackages. Hence, you need to import the subpackage as well.
Sequence of the program must be package then import then class.
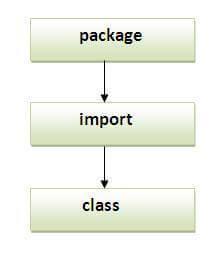
Key takeaway
A java package is a group of similar types of classes, interfaces and sub-packages. Package in java can be categorized in two form, built-in package and user-defined package. There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc. Here, we will have the detailed learning of creating and using user-defined packages.
CLASSPATH: CLASSPATH is an environment variable which is used by Application ClassLoader to locate and load the .class files. The CLASSPATH defines the path, to find third-party and user-defined classes that are not extensions or part of Java platform. Include all the directories which contain .class files and JAR files when setting the CLASSPATH.
You need to set the CLASSPATH if:
● You need to load a class that is not present in the current directory or any sub-directories.
● You need to load a class that is not in a location specified by the extensions mechanism.
The CLASSPATH depends on what you are setting the CLASSPATH. The CLASSPATH has a directory name or file name at the end. The following points describe what should be the end of the CLASSPATH.
● If a JAR or zip, the file contains class files, the CLASSPATH end with the name of the zip or JAR file.
● If class files placed in an unnamed package, the CLASSPATH ends with the directory that contains the class files.
● If class files placed in a named package, the CLASSPATH ends with the directory that contains the root package in the full package name, that is the first package in the full package name.
The default value of CLASSPATH is a dot (.). It means the only current directory searched. The default value of CLASSPATH overrides when you set the CLASSPATH variable or using the -classpath command (for short -cp). Put a dot (.) in the new setting if you want to include the current directory in the search path.
If CLASSPATH finds a class file which is present in the current directory, then it will load the class and use it, irrespective of the same name class presents in another directory which is also included in the CLASSPATH.
If you want to set multiple classpaths, then you need to separate each CLASSPATH by a semicolon (;).
The third-party applications (MySQL and Oracle) that use the JVM can modify the CLASSPATH environment variable to include the libraries they use. The classes can be stored in directories or archives files. The classes of the Java platform are stored in rt.jar.
There are two ways to ways to set CLASSPATH: through Command Prompt or by setting Environment Variable.
Let's see how to set CLASSPATH of MySQL database:
Step 1: Click on the Windows button and choose Control Panel. Select System.
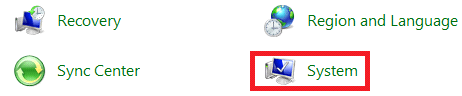
Step 2: Click on Advanced System Settings.
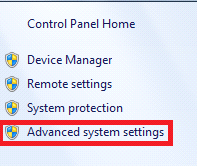
Step 3: A dialog box will open. Click on Environment Variables.
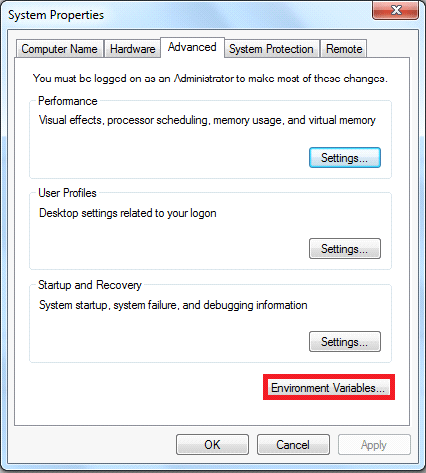
Step 4: If the CLASSPATH already exists in System Variables, click on the Edit button then put a semicolon (;) at the end. Paste the Path of MySQL-Connector Java.jar file.
If the CLASSPATH doesn't exist in System Variables, then click on the New button and type Variable name as CLASSPATH and Variable value as C:\Program Files\Java\jre1.8\MySQL-Connector Java.jar;.;
Remember: Put ;.; at the end of the CLASSPATH.
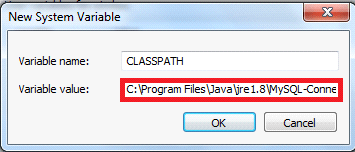
How to Set CLASSPATH in Windows Using Command Prompt
Type the following command in your Command Prompt and press enter.
Set CLASSPATH=%CLASSPATH%;C:\Program Files\Java\jre1.8\rt.jar;
In the above command, The set is an internal DOS command that allows the user to change the variable value. CLASSPATH is a variable name. The variable enclosed in percentage sign (%) is an existing environment variable. The semicolon is a separator, and after the (;) there is the PATH of rt.jar file.
How ext folder works in Java
The ext directory works a bit like the CLASSPATH. Ext directory is the part of the class loading mechanism. The classes which are available within JARs in the ext directory are available to Java applications.
Key takeaway
CLASSPATH is an environment variable which is used by Application ClassLoader to locate and load the .class files. The CLASSPATH defines the path, to find third-party and user-defined classes that are not extensions or part of Java platform. Include all the directories which contain .class files and JAR files when setting the CLASSPATH.
Java Packages & API
A package in Java is used to group related classes. Think of it as a folder in a file directory. We use packages to avoid name conflicts, and to write a better maintainable code. Packages are divided into two categories:
● Built-in Packages (packages from the Java API)
● User-defined Packages (create your own packages)
Built-in Packages
The Java API is a library of prewritten classes, that are free to use, included in the Java Development Environment.
The library contains components for managing input, database programming, and much more.
The library is divided into packages and classes. Meaning you can either import a single class (along with its methods and attributes), or a whole package that contain all the classes that belong to the specified package.
To use a class or a package from the library, you need to use the import keyword:
Syntax
Import package.name.Class; // Import a single class
Import package.name.*; // Import the whole package
Import a Class
If you find a class you want to use, for example, the Scanner class, which is used to get user input, write the following code:
Example
Import java.util.Scanner;
In the example above, java.util is a package, while Scanner is a class of the java.util package.
To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine() method, which is used to read a complete line:
Example
Using the Scanner class to get user input:
Import java.util.Scanner;
Class MyClass {
Public static void main(String[] args) {
Scanner myObj = new Scanner(System.in);
System.out.println("Enter username");
String userName = myObj.nextLine();
System.out.println("Username is: " + userName);
}
}
Import a Package
There are many packages to choose from. In the previous example, we used the Scanner class from the java.util package. This package also contains date and time facilities, random-number generator and other utility classes.
To import a whole package, end the sentence with an asterisk sign (*). The following example will import ALL the classes in the java.util package:
Example
Import java.util.*;
User-defined Packages
To create your own package, you need to understand that Java uses a file system directory to store them. Just like folders on your computer:
Example
└── root
└── mypack
└── MyPackageClass.java
To create a package, use the package keyword:
MyPackageClass.java
Package mypack;
Class MyPackageClass {
Public static void main(String[] args) {
System.out.println("This is my package!");
}
}
Save the file as MyPackageClass.java, and compile it:
C:\Users\Your Name>javac MyPackageClass.java
Then compile the package:
C:\Users\Your Name>javac -d . MyPackageClass.java
This forces the compiler to create the "mypack" package.
The -d keyword specifies the destination for where to save the class file. You can use any directory name, like c:/user (windows), or, if you want to keep the package within the same directory, you can use the dot sign ".", like in the example above.
Note: The package name should be written in lower case to avoid conflict with class names.
When we compiled the package in the example above, a new folder was created, called "mypack".
To run the MyPackageClass.java file, write the following:
C:\Users\Your Name>java mypack.MyPackageClass
The output will be:
This is my package!
Key takeaway
A package in Java is used to group related classes. Think of it as a folder in a file directory. We use packages to avoid name conflicts, and to write a better maintainable code. Packages are divided into two categories:
● Built-in Packages (packages from the Java API)
● User-defined Packages (create your own packages)
References:
- Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
- CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
- JAVA Complete Reference (9th Edition) Herbert Schelidt.
- T. Budd, “Understanding Object- Oriented Programming with Java”, Pearson Education, Updated Edition (New Java 2 Coverage), 1999.