Unit - 5
Exception Handling and I/O Streams
● The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that the normal flow of the application can be maintained.
● In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
What is Exception in Java
Dictionary Meaning: Exception is an abnormal condition.
In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
What is Exception Handling?
Exception Handling is a mechanism to handle runtime errors such as ClassNotFoundException, IOException, SQLException, RemoteException, etc.
The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application that is why we use exception handling. Let's take a scenario:
- Statement 1;
- Statement 2;
- Statement 3;
- Statement 4;
- Statement 5;//exception occurs
- Statement 6;
- Statement 7;
- Statement 8;
- Statement 9;
- Statement 10;
Suppose there are 10 statements in your program and there occurs an exception at statement 5, the rest of the code will not be executed i.e. statement 6 to 10 will not be executed. If we perform exception handling, the rest of the statement will be executed. That is why we use exception handling in Java.
Benefits of exception handling
● When an exception occurs, exception handling guarantees that the program's flow is preserved.
● This allows us to identify the different types of faults.
● This allows us to develop error-handling code separately from the rest of the code.
When an exception occurs, exception handling ensures that the program's flow does not break. For example, if a programme has a large number of statements and an exception occurs in the middle of executing some of them, the statements after the exception will not be executed, and the programme will end abruptly.
By handling, we ensure that all of the statements are executed and that the program's flow is not disrupted.
Key takeaway
The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that the normal flow of the application can be maintained.
Hierarchy of Java Exception classes
The java.lang.Throwable class is the root class of Java Exception hierarchy which is inherited by two subclasses: Exception and Error. A hierarchy of Java Exception classes are given below:
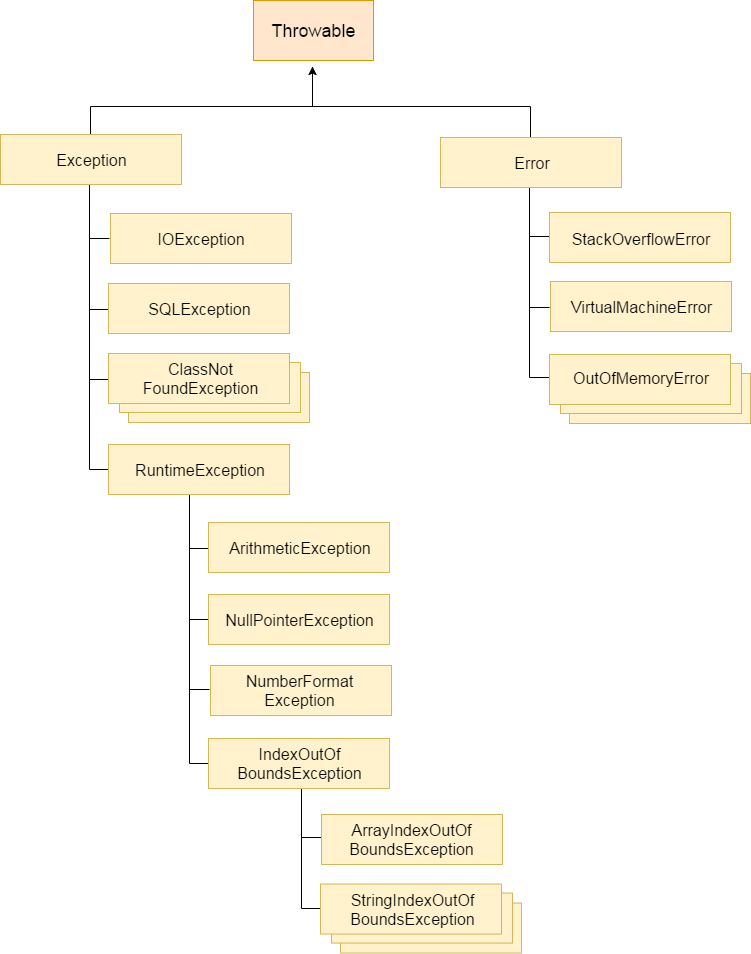
Fig 1 - A hierarchy of Java Exception classes
Types of Java Exceptions
There are mainly two types of exceptions: checked and unchecked. Here, an error is considered as the unchecked exception. According to Oracle, there are three types of exceptions:
- Checked Exception
- Unchecked Exception
- Error
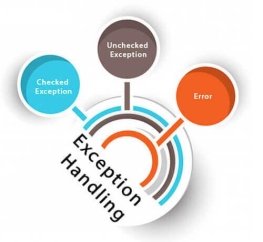
Fig 2 – Exception handling
Difference between Checked and Unchecked Exceptions
1) Checked Exception
The classes which directly inherit Throwable class except Runtime Exception and Error are known as checked exceptions e.g. IO Exception, SQL Exception etc. Checked exceptions are checked at compile-time.
2) Unchecked Exception
The classes which inherit Runtime Exception are known as unchecked exceptions e.g. Arithmetic Exception, Null Pointer Exception, Array Index Out Of Bounds Exception etc. Unchecked exceptions are not checked at compile-time, but they are checked at runtime.
3) Error
Error is irrecoverable e.g. Out Of Memory Error, Virtual Machine Error, Assertion Error etc.
Java Exception Keywords
There are 5 keywords which are used in handling exceptions in Java.
Keyword | Description |
Try | The "try" keyword is used to specify a block where we should place exception code. The try block must be followed by either catch or finally. It means, we can't use try block alone. |
Catch | The "catch" block is used to handle the exception. It must be preceded by try block which means we can't use catch block alone. It can be followed by finally block later. |
Finally | The "finally" block is used to execute the important code of the program. It is executed whether an exception is handled or not. |
Throw | The "throw" keyword is used to throw an exception. |
Throws | The "throws" keyword is used to declare exceptions. It doesn't throw an exception. It specifies that there may occur an exception in the method. It is always used with method signature. |
Java Exception Handling Example
Let's see an example of Java Exception Handling where we using a try-catch statement to handle the exception.
- Public class JavaExceptionExample{
- Public static void main(String args[]){
- Try{
- //code that may raise exception
- Int data=100/0;
- }catch(ArithmeticException e){System.out.println(e);}
- //rest code of the program
- System.out.println("rest of the code...");
- }
- }
Output:
Exception in thread main java.lang.ArithmeticException:/ by zero
Rest of the code…
In the above example, 100/0 raises an Arithmetic Exception which is handled by a try-catch block.
Common Scenarios of Java Exceptions
There are given some scenarios where unchecked exceptions may occur. They are as follows:
1) A scenario where Arithmetic Exception occurs
If we divide any number by zero, there occurs an Arithmetic Exception.
- Int a=50/0;//Arithmetic Exception
2) A scenario where Null Pointer Exception occurs
If we have a null value in any variable, performing any operation on the variable throws a Null Pointer Exception.
- String s=null;
- System.out.println(s.length());//NullPointerException
3) A scenario where Number Format Exception occurs
The wrong formatting of any value may occur Number Format Exception. Suppose I have a string variable that has characters, converting this variable into digit will occur Number Format Exception.
- String s="abc";
- Int i=Integer.parseInt(s);//Number Format Exception
4) A scenario where ArrayIndexOutOfBoundsException occurs
If you are inserting any value in the wrong index, it would result in ArrayIndexOutOfBoundsException as shown below:
- Int a[]=new int[5];
- a[10]=50; //ArrayIndexOutOfBoundsException
Key takeaway
The java.lang.Throwable class is the root class of Java Exception hierarchy which is inherited by two subclasses: Exception and Error.
There are mainly two types of exceptions: checked and unchecked. An error is considered as the unchecked exception. However, according to Oracle, there are three types of exceptions namely:
1) Checked Exception:
❖ The classes that directly inherit the Throwable class except RuntimeException and Error are known as checked exceptions.
❖ For example, IOException, SQLException, etc. Checked exceptions are checked at compile-time.
1. ClassNotFoundException: The ClassNotFoundException is a kind of checked exception that is thrown when we attempt to use a class that does not exist.
Checked exceptions are those exceptions that are checked by the Java compiler itself.
2. FileNotFoundException: The FileNotFoundException is a checked exception that is thrown when we attempt to access a non-existing file.
3. InterruptedException: InterruptedException is a checked exception that is thrown when a thread is in sleeping or waiting state and another thread attempt to interrupt it.
4. InstantiationException: This exception is also a checked exception that is thrown when we try to create an object of abstract class or interface. That is, InstantiationException exception occurs when an abstract class or interface is instantiated.
5. IllegalAccessException: The IllegalAccessException is a checked exception and it is thrown when a method is called in another method or class but the calling method or class does not have permission to access that method.
6. CloneNotSupportedException: This checked exception is thrown when we try to clone an object without implementing the cloneable interface.
7. NoSuchFieldException: This is a checked exception that is thrown when an unknown variable is used in a program.
8. NoSuchMethodException: This checked exception is thrown when the undefined method is used in a program.
2) Unchecked Exception
❖ The classes that inherit the RuntimeException are known as unchecked exceptions.
❖ For example, ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException, etc
❖ Unchecked exceptions are not checked at compile-time, but they are checked at runtime.
Let’s see a brief description of them.
1. ArithmeticException: This exception is thrown when arithmetic problems, such as a number is divided by zero, is occurred. That is, it is caused by maths error.
2. ClassCastException: The ClassCastException is a runtime exception that is thrown by JVM when we attempt to invalid typecasting in the program. That is, it is thrown when we cast an object to a subclass of which an object is not an instance.
3. IllegalArgumentException: This runtime exception is thrown by programmatically when an illegal or appropriate argument is passed to call a method. This exception class has further two subclasses:
● Number Format Exception
● Illegal Thread State Exception
Numeric Format Exception: Number Format Exception is thrown by programmatically when we try to convert a string into the numeric type and the process of illegal conversion fails. That is, it occurs due to the illegal conversion of a string to a numeric format.
Illegal Thread State Exception: Illegal Thread State Exception is a runtime exception that is thrown by programmatically when we attempt to perform any operation on a thread but it is incompatible with the current thread state.
4. IndexOutOfBoundsException: This exception class is thrown by JVM when an array or string is going out of the specified index. It has two further subclasses:
● ArrayIndexOutOfBoundsException
● StringIndexOutOfBoundsException
ArrayIndexOutOfBoundsException: ArrayIndexOutOfBoundsException exception is thrown when an array element is accessed out of the index.
StringIndexOutOfBoundsException: StringIndexOutOfBoundsException exception is thrown when a String or StringBuffer element is accessed out of the index.
5. NullPointerException: NullPointerException is a runtime exception that is thrown by JVM when we attempt to use null instead of an object. That is, it is thrown when the reference is null.
6. ArrayStoreException: This exception occurs when we attempt to store any value in an array which is not of array type. For example, suppose, an array is of integer type but we are trying to store a value of an element of another type.
7. IllegalStateException: The IllegalStateException exception is thrown by programmatically when the runtime environment is not in an appropriate state for calling any method.
8. IllegalMonitorStateException: This exception is thrown when a thread does not have the right to monitor an object and tries to access wait(), notify(), and notifyAll() methods of the object.
9. NegativeArraySizeException: The NegativeArraySizeException exception is thrown when an array is created with a negative size.
3) Error
Error is irrecoverable. Some examples of errors are Out Of Memory Error, Virtual Machine Error, Assertion Error etc.
Difference between Checked and Unchecked Exceptions
Checked Exception | Unchecked Exception |
These exceptions are checked at compile time. These exceptions are handled at compile time too. | These exceptions are just opposite to the checked exceptions. These exceptions are not checked and handled at compile time. |
These exceptions are direct subclasses of exception but not extended from RuntimeException class. | They are the direct subclasses of the RuntimeException class. |
The code gives a compilation error in the case when a method throws a checked exception. The compiler is not able to handle the exception on its own. | The code compiles without any error because the exceptions escape the notice of the compiler. These exceptions are the results of user-created errors in programming logic. |
These exceptions mostly occur when the probability of failure is too high. | These exceptions occur mostly due to programming mistakes. |
Common checked exceptions include IOException, DataAccessException, InterruptedException, etc. | Common unchecked exceptions include ArithmeticException, InvalidClassException, NullPointerException, etc. |
These exceptions are propagated using the throws keyword. | These are automatically propagated. |
It is required to provide the try-catch and try-finally block to handle the checked exception. | In the case of unchecked exception it is not mandatory. |
Key takeaway
The classes that directly inherit the Throwable class except RuntimeException and Error are known as checked exceptions.
The classes that inherit the RuntimeException are known as unchecked exceptions.
Java provides five keywords that are used to handle the exception. The following table describes each.
Keyword | Description |
Try | The "try" keyword is used to specify a block where we should place an exception code. It means we can't use try block alone. The try block must be followed by either catch or finally. |
Catch | The "catch" block is used to handle the exception. It must be preceded by try block which means we can't use catch block alone. It can be followed by finally block later. |
Finally | The "finally" block is used to execute the necessary code of the program. It is executed whether an exception is handled or not. |
Throw | The "throw" keyword is used to throw an exception. |
Throws | The "throws" keyword is used to declare exceptions. It specifies that there may occur an exception in the method. It doesn't throw an exception. It is always used with method signature. |
A) try block:
a) Syntax of try block with catch block:
Try {
//Block of statement}
Catch(Exception Handler class)
{
}
b) Syntax of try block with finally block:
Try {
//Block of statement}
Finally {
}
c) Syntax of try block with catch and finally block:
Try {
//Block of statement}
Catch(Exception Handler class)
{
} finally{
}
B) Catch block:
a) Syntax of try block with catch block:
Try {
//Block of statement}
Catch(Exception Handler class)
{
}
b) Multiple catch block:
Syntax of try block with catch block:
Try {
//Block of statement}
Catch(Exception Handler subclass)
{
}catch(Exception Handler super class){
}
C) Nested try block:
Syntax of nested try block
Try{ // block of statement
Try{
// block of statement
}
Catch(Exception Handler class)
{
}
Catch(Exception Handler class)
{
}
D) Finally:
a) Syntax of finally without catch
Try{
//block of statement
}
Finally {
}
b) Syntax of finally with catch
Try {
//Block of statement}
Catch(Exception Handler class)
{
} finally{
}
E) throw:
Syntax:
Throw exception_object;
F) throws:
Syntax:
MethodName () throws comma separated list of exceptionClassNames{}
Difference between throw and throws
Throw keyword | Throws keyword |
|
|
Difference between final, finally and finalize
● The final, finally, and finalize are keywords in Java that are used in exception handling. Each of these keywords has a different functionality.
● The basic difference between final, finally and finalize is that the final is an access modifier, finally is the block in Exception Handling and finalize is the method of object class.
Key | Final | Finally | Finalize |
Definition | Final is the keyword and access modifier which is used to apply restrictions on a class, method or variable. | Finally is the block in Java Exception Handling to execute the important code whether the exception occurs or not. | Finalize is the method in Java which is used to perform clean up processing just before object is garbage collected. |
Applicable to | Final keyword is used with the classes, methods and variables. | Finally block is always related to the try and catch block in exception handling. | Finalize() method is used with the objects. |
Functionality | (1) Once declared, final variable becomes constant and cannot be modified. | (1) finally block runs the important code even if exception occurs or not. | Finalize method performs the cleaning activities with respect to the object before its destruction. |
Execution | Final method is executed only when we call it. | Finally block is executed as soon as the try-catch block is executed. It's execution is not dependant on the exception. | Finalize method is executed just before the object is destroyed. |
Java try block
Java try block is used to enclose the code that might throw an exception. It must be used within the method.
If an exception occurs at the particular statement of try block, the rest of the block code will not execute. So, it is recommended not to keeping the code in try block that will not throw an exception.
Java try block must be followed by either catch or finally block.
Syntax of Java try-catch
- Try{
- //code that may throw an exception
- }catch(Exception_class_Name ref){}
Syntax of try-finally block
- Try{
- //code that may throw an exception
- }finally{}
Java catch block
Java catch block is used to handle the Exception by declaring the type of exception within the parameter. The declared exception must be the parent class exception ( i.e., Exception) or the generated exception type. However, the good approach is to declare the generated type of exception.
The catch block must be used after the try block only. You can use multiple catch block with a single try block.
Problem without exception handling
Let's try to understand the problem if we don't use a try-catch block.
Example 1
- Public class TryCatchExample1 {
- Public static void main(String[] args) {
- Int data=50/0; //may throw exception
- System.out.println("rest of the code");
- }
- }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
As displayed in the above example, the rest of the code is not executed (in such case, the rest of the code statement is not printed).
There can be 100 lines of code after exception. So all the code after exception will not be executed.
Solution by exception handling
Let's see the solution of the above problem by a java try-catch block.
Example 2
- Public class TryCatchExample2 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- //handling the exception
- Catch(ArithmeticException e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Java.lang.ArithmeticException: / by zero
Rest of the code
Now, as displayed in the above example, the rest of the code is executed, i.e., the rest of the code statement is printed.
Example 3
In this example, we also kept the code in a try block that will not throw an exception.
- Public class TryCatchExample3 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- // if exception occurs, the remaining statement will not exceute
- System.out.println("rest of the code");
- }
- // handling the exception
- Catch(ArithmeticException e)
- {
- System.out.println(e);
- }
- }
- }
Output:
Java.lang.ArithmeticException: / by zero
Here, we can see that if an exception occurs in the try block, the rest of the block code will not execute.
Example 4
Here, we handle the exception using the parent class exception.
- Public class TryCatchExample4 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- // handling the exception by using Exception class
- Catch(Exception e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Java.lang.ArithmeticException: / by zero
Rest of the code
Example 5
Let's see an example to print a custom message on exception.
- Public class TryCatchExample5 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- // handling the exception
- Catch(Exception e)
- {
- // displaying the custom message
- System.out.println("Can't divided by zero");
- }
- }
- }
Output:
Can't divided by zero
Example 6
Let's see an example to resolve the exception in a catch block.
- Public class TryCatchExample6 {
- Public static void main(String[] args) {
- Int i=50;
- Int j=0;
- Int data;
- Try
- {
- Data=i/j; //may throw exception
- }
- // handling the exception
- Catch(Exception e)
- {
- // resolving the exception in catch block
- System.out.println(i/(j+2));
- }
- }
- }
Output:
25
Example 7
In this example, along with try block, we also enclose exception code in a catch block.
- Public class TryCatchExample7 {
- Public static void main(String[] args) {
- Try
- {
- Int data1=50/0; //may throw exception
- }
- // handling the exception
- Catch(Exception e)
- {
- // generating the exception in catch block
- Int data2=50/0; //may throw exception
- }
- System.out.println("rest of the code");
- }
- }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
Here, we can see that the catch block didn't contain the exception code. So, enclose exception code within a try block and use catch block only to handle the exceptions.
Example 8
In this example, we handle the generated exception (Arithmetic Exception) with a different type of exception class (ArrayIndexOutOfBoundsException).
- Public class TryCatchExample8 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- // try to handle the ArithmeticException using ArrayIndexOutOfBoundsException
- Catch(ArrayIndexOutOfBoundsException e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
Example 9
Let's see an example to handle another unchecked exception.
- Public class TryCatchExample9 {
- Public static void main(String[] args) {
- Try
- {
- Int arr[]= {1,3,5,7};
- System.out.println(arr[10]); //may throw exception
- }
- // handling the array exception
- Catch(ArrayIndexOutOfBoundsException e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Java.lang.ArrayIndexOutOfBoundsException: 10
Rest of the code
Example 10
Let's see an example to handle checked exception.
- Import java.io.FileNotFoundException;
- Import java.io.PrintWriter;
- Public class TryCatchExample10 {
- Public static void main(String[] args) {
- PrintWriter pw;
- Try {
- Pw = new PrintWriter("jtp.txt"); //may throw exception
- Pw.println("saved");
- }
- // providing the checked exception handler
- Catch (FileNotFoundException e) {
- System.out.println(e);
- }
- System.out.println("File saved successfully");
- }
- }
Output:
File saved successfully
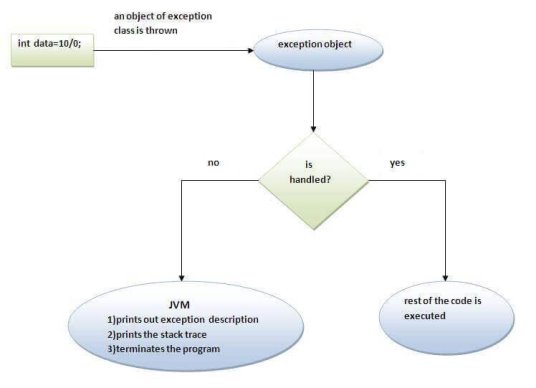
Fig 3: Internal working of java try-catch block
The JVM firstly checks whether the exception is handled or not. If exception is not handled, JVM provides a default exception handler that performs the following tasks:
● Prints out exception description.
● Prints the stack trace (Hierarchy of methods where the exception occurred).
● Causes the program to terminate.
But if exception is handled by the application programmer, normal flow of the application is maintained i.e. rest of the code is executed.
Key takeaway
Java try block is used to enclose the code that might throw an exception. It must be used within the method.
Java catch block is used to handle the Exception by declaring the type of exception within the parameter.
You can use the throw keyword to re-throw an exception that has been cached in a catch block (which is used to throw the exception objects).
You can re-throw the same exception without altering it while re-throwing exceptions.
Try {
Int result = (arr[a])/(arr[b]);
System.out.println("Result of "+arr[a]+"/"+arr[b]+": "+result);
}
Catch(ArithmeticException e) {
Throw e;
}
Alternatively, you can wrap it in a new exception and throw it. Exception chaining or exception wrapping is when you wrap a cached exception within another exception and throw it. This allows you to change your exception by throwing a higher level of exception while retaining the abstraction.
Try {
Int result = (arr[a])/(arr[b]);
System.out.println("Result of "+arr[a]+"/"+arr[b]+": "+result);
}
Catch(ArrayIndexOutOfBoundsException e) {
Throw new IndexOutOfBoundsException();
}
Example
In the Java example below, our demoMethod() code may throw an ArrayIndexOutOfBoundsException and an ArithmeticException. These two exceptions are caught in two different catch blocks.
We re-throw both exceptions in the catch blocks, one by wrapping it within the higher exceptions and the other directly.
Import java.util.Arrays;
Import java.util.Scanner;
Public class RethrowExample {
Public void demoMethod() {
Scanner sc = new Scanner(System.in);
Int[] arr = {10, 20, 30, 2, 0, 8};
System.out.println("Array: "+Arrays.toString(arr));
System.out.println("Choose numerator and denominator(not 0) from this array (enter positions 0 to 5)");
Int a = sc.nextInt();
Int b = sc.nextInt();
Try {
Int result = (arr[a])/(arr[b]);
System.out.println("Result of "+arr[a]+"/"+arr[b]+": "+result);
}
Catch(ArrayIndexOutOfBoundsException e) {
Throw new IndexOutOfBoundsException();
}
Catch(ArithmeticException e) {
Throw e;
}
}
Public static void main(String [] args) {
New RethrowExample().demoMethod();
}
}
Output1
Array: [10, 20, 30, 2, 0, 8]
Choose numerator and denominator(not 0) from this array (enter positions 0 to 5)
0
4
Exception in thread "main" java.lang.ArithmeticException: / by zero
At myPackage.RethrowExample.demoMethod(RethrowExample.java:16)
At myPackage.RethrowExample.main(RethrowExample.java:25)
Output2
Array: [10, 20, 30, 2, 0, 8]
Choose numerator and denominator(not 0) from this array (enter positions 0 to 5)
124
5
Exception in thread "main" java.lang.IndexOutOfBoundsException
At myPackage.RethrowExample.demoMethod(RethrowExample.java:17)
At myPackage.RethrowExample.main(RethrowExample.java:23)
The graphical user interface (GUI) is a key visible display of objects that the user sees and interacts with on the screen in the world of computer programming (GUI). You've always known what a GUI is, even if you didn't realize it was called that. It's what you're engaging with right now as you navigate the web using whatever browser and operating system you're using.
There are numerous programming languages available, and Java is simply one of them. For each language, there are special words linked to the GUI code. Because each language has its unique set of codes, it's important to master the right programming syntax (rules) for each language to maximize efficiency. Learning the syntax for the GUI in Java so that you may master these programming skills and utilize them in your future projects is reason enough to understand the syntax for the GUI in Java.
GUI Components
Components are add-ons or extensions to containers that help fill in the gaps in the content and make up the rest of the user interface. A textbox, a button, a checkbox, or even a simple label are all examples of controls. When you mix both containers and components, you begin to see the bigger picture of what the GUI is and why it is at the heart of every programme or application's user experience.
● A graphical user interface component (GUI component) is an object that represents a screen element like a button or a text field.
● The java.awt and javax.swing packages are primarily responsible for GUI-related classes.
● The Abstract Windowing Toolkit (AWT) was the first Java graphical user interface library.
● The Swing package adds new components and makes them more versatile.
● To make a Java GUI-based programme, you'll need both packages.
Labels, text fields, text areas, buttons, and other graphical user interface components are all available in Java. Containers in the Abstract Windowing Toolkit (AWT) can also contain these components. Frames (windows), canvases (for drawing), and panels are examples of containers (which are used to group components). Frames (windows) hold panels and canvases, whereas buttons and other components can be placed directly on the frames or in panels within the frames.
When the window in which these GUI components are located is drawn, they are automatically drawn. As a result, we won't have to type commands to draw them.
The event model in Java is used to manage these GUI components.
When a user interacts with a component (clicks a button, types in a field, selects from a pop-up menu, etc. ), the component that you connect with generates an event. The programmer must specify one or more objects for each component of your application to "listen" for events from that component. If your software contains a "start" button, you must designate one or more objects to be informed when the user presses the button.
Buttons
Button is a class in the java.awt package that represents screen buttons. The constructor is:
Public Button(String label);
When this code is run, it produces a button with the word "label" printed on it. In most cases, the button is large enough to see the label. a parameterless constructor is also available for creating an unlabeled button.
Buttons react to a wide range of signals. You may, for example, include a
Public void addActionListener(ActionListener listener);
Adding buttons to a Frame or Panel
By delivering the message: "Add a button to a frame or panel," we can add a button to a frame or panel.
MyFrame.add(startButton);
Normally we include such code in the constructor for the frame or panel. Hence usually we just write this. Add(startButton) or simply add(startButton).
Here's some code for ButtonDemo, a class that represents a Frame specialization with two buttons. The class extends Frame from the java.awt package. The constructor for Frame takes a String parameter and creates a window with that string in the title bar. The constructor for ButtonDemo calls the superclass constructor, and then sets the size of the new Frame to be 400 x 200. The setLayout command instructs the new Frame to add new components across the panel from left to right.
If there isn't enough room, new components will be put in new rows, starting from the left. The button-making code should be self-explanatory. The buttons are added to the frame using the add commands. The ButtonListener class is explained further down. It builds objects that respond to button clicks by doing actions. As a result, we instruct the two buttons to notify myButtonListener when the user clicks on them (using the addActionListener method). Finally, because Frame, Button, and FlowLayout are all classes in the java.awt package, we had to import java.awt.*.
Import java.awt.*;
Public class ButtonDemo extends Frame
{
Protected Button startButton, stopButton;
// Constructor sets features of the frame, creates buttons,
// adds them to the frame, and assigns an object to listen
// to them
Public ButtonDemo()
{
Super("Button demo"); // calls Frame constructor
// which adds title to window
SetSize(400,200); // sets the size of the window
// sets layout so objects added go from left to right
// until fill up row and then go to next row.
// There are other layout options, including GridLayout.
SetLayout(new FlowLayout());
// create two new buttons w/labels start and stop
StartButton = new Button("Start");
StopButton = new Button("Stop");
Add(startButton); // add buttons to frame
Add(stopButton);
// create an object to listen to both buttons:
ButtonListener myButtonListener = new ButtonListener();
// tell buttons that myButtonListener should be notified
StartButton.addActionListener(myButtonListener);
StopButton.addActionListener(myButtonListener);
SetVisible(true); // Show the window on the screen.
// Leave this out and you won't see it!
}
// Trivial main program associated with ButtonDemo
// Simply creates it and shows it.
Public static void main(String args[]){
// Create an instance of Buttons
ButtonDemo app = new ButtonDemo();
}
}
The class's main programme just produces an object from the class, which then displays itself on the screen. This minimum amount of work is nearly always done by main programmes associated with frames.
ActionListeners for Buttons
Objects that implement the ActionListener interface must implement the actionPerformed method, which accepts an ActionEvent parameter. When a button is pressed, any objects added as ActionListeners for that button are notified by executing their actionPerformed function with an argument containing information about the specific event that occurred. As a result, the system constructs the ActionEvent object and delivers it to the listener automatically.
The most useful methods of ActionEvent are
Public String getActionCommand();
And
Public Object getSource();
If you send getActionCommand to an ActionEvent that represents a button click, it will return a string that corresponds to the button's label. GetSource returns the object where the event occurred as a reference. Because the class ButtonListener implements ActionListener, an object of this class can be used as a listener on a button. The actionPerformed method is straightforward. It utilises getActionCommand to get the label of the button that was clicked, and then prints a different message depending on what the button was. (Recall that each of the two buttons in ButtonDemo has the same ActionListener, so getActionCommand needs to find out which one was clicked.)
This class has no explicit function Object() { [native code] } because it is so simple (it doesn't even have instance variables). Instead, it contains a default function Object() { [native code] } (with no parameters) that simply allocates storage for newly built instances, exactly like all other classes. Because ActionEvent and the ActionListener interface are both in the java.awt.event package, we must import it.
Import java.awt.event.*;
Public class ButtonListener implements ActionListener{
Public void actionPerformed(ActionEvent evt)
{
// Get label of the button clicked in event passed in
String arg = evt.getActionCommand();
If (arg.equals("Start"))
System.out.println("Start button");
Else if (arg.equals("Stop"))
System.out.println("Stop button");
}
}
Labels
A Label is a straightforward component that holds a string. The assemblers are
Public Label() // creates label with no text
Public Label(String text) //create label with text
The methods offered are as follows:
Public String getText() // return label text
Public void setText(String s) // sets the label text
The wording in Labels, on the other hand, is rarely changed.
Text Fields
A TextField is a single-line input field where the user can type. It is an effective method of obtaining text input from the user. The assemblers are
Public TextField () // creates text field with no text
Public TextField (int columns)
// create text field with appropriate # of columns
Public TextField (String s) // create text field with s displayed
Public TextField (String s, int columns)
// create text field with s displayed & approp. Width
Methods
Public void setEditable(boolean s)
// if false the TextField is not user editable
Public String getText() // return label text
Public void setText(String s) // sets the label text
For this component, there are a slew of different options.
When a user fills into a text field and then presses the return or enter key, an event is generated that may be handled by the same ActionListener that is used with Buttons. However, getActionCommand does not return any relevant String in this scenario. The method getSource is used in this case. As a result, we can write: to reply to the user hitting the return key while typing in a TextField myField:
Public void actionPerformed(ActionEvent evt)
{
If(evt.getSource() == myField){
String contents = myField.getText();
System.out.println("The field contained: "+contents);
} else ...
}
It's worth noting that using myField in actionPerformed only makes sense if we have either an inner class (and hence access to all of the outer class's instance variables) or if the Frame or Panel where myField is specified is the related ActionListener.
You can link a TextListener to the TextField if you want to be alerted whenever any modification is made to it (whether or not the user hits the return key). Details can be found in the online documentation.
Text Areas
TextArea is a class that allows you to create a text area that can accommodate many lines of text. It's similar to TextField with the exception that no special event is triggered when the return key is pressed.
The assemblers are
Public TextArea(int rows, int columns)
// create text area with appropriate # rows and columns
Public TextArea(String s, int rows, in columns)
// create text area with rows, columns, and displaying s
Methods
Public void setEditable(boolean s)
// if false the TextArea is not user editable
Public String getText() // return text in TextArea
Public void setText(String s) // sets the text
Key takeaway
Components are add-ons or extensions to containers that help fill in the gaps in the content and make up the rest of the user interface. A textbox, a button, a checkbox, or even a simple label are all examples of controls.
Java Database Connectivity (JDBC) is a Java application programming interface (API) that specifies how a client can interact with a database. It is a Java-based data access technology that is used to link Java databases. Oracle Corporation's Java Standard Edition platform includes it. It is geared toward relational databases and provides techniques for querying and updating data in a database. In the Java virtual machine (JVM) host environment, a JDBC-to-ODBC bridge allows connections to any ODBC-accessible data source.
Java Database Connectivity (JDBC) is a Sun Microsystems specification that provides a common abstraction (API or Protocol) for Java programmes to interface with multiple databases. It implements the Java database connectivity standard in the language. It's used to create programmes that connect to databases. Databases and spreadsheets can be accessed via JDBC and the database driver. JDBC APIs can be used to access enterprise data stored in a relational database (RDB).
JDBC is an API (application programming interface) for interacting with databases in Java programming.
JDBC classes and interfaces enable applications to communicate user requests to a specific database.
JDBC's Purpose
Enterprise applications built with the JAVA EE technology must connect with databases in order to store application-specific data. As a result, communicating with a database necessitates effective database connectivity, which can be accomplished with the ODBC (Open database connectivity) driver. This driver is used in conjunction with JDBC to connect or communicate with a variety of databases, including Oracle, MS Access, Mysql, and SQL Server.
Components
JDBC is made up of four basic components that allow it to interact with a database. The following is a list of them:
● JDBC API - It offers a variety of methods and interfaces for interacting with databases.
It includes the following two packages, which contain the Java SE and Java EE platforms to demonstrate WORA (write once run anywhere) capabilities.
1. Java.sql.*;
2. Javax.sql.*;
It also establishes a standard for connecting a client application to a database.
● JDBC Driver Manager - The JDBC Driver Manager loads a database-specific driver into an application in order to connect to a database. It's used to process the user's request by making a database-specific call to the database.
● JDBC Test Suite - It is used to test JDBC Drivers' operations (such as insertion, deletion, and updating).
● JDBC-ODBC Bridge Drivers - Bridge Drivers for JDBC and ODBC: It establishes a connection between database drivers and the database. This bridge converts ODBC function calls to JDBC method calls. It makes use of the
Sun.jdbc.odbc
This package contains a native library for accessing ODBC properties.
Key takeaway
Java Database Connectivity (JDBC) is a Java application programming interface (API) that specifies how a client can interact with a database. It is a Java-based data access technology that is used to link Java databases.
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Stream:
● A stream is a method to sequentially access a file.
● I/O Stream means an input source or output destination representing different types of sources
● e.g., disk files. The java.io package provides classes that allow you to convert between Unicode character streams and byte streams of non-Unicode text.
Stream – A sequence of data.
Input Stream: reads data from source.
Output Stream: writes data to destination.

Fig 4 - Stream
Java provides strong but flexible support for I/O related to files and networks but this tutorial covers very basic functionality related to streams and I/O
Byte Stream Classes:
● Byte Stream classes are used to read bytes from the input stream and write bytes to the output stream.
● In other word, Byte Stream classes read/write the data of 8-bits. We can store video, audio, characters, etc., by using Byte Stream classes.
● These classes are part of the java.io package.
● The Byte Stream classes are divided into two types of classes, i.e., Input Stream and Output Stream. These classes are abstract and the super classes of all the Input/Output stream classes.
Input Stream Class:
The Input Stream class provides methods to read bytes from a file, console or memory. It is an abstract class
SN | Class | Description | ||
1 | Buffered Input Stream | This class provides methods to read bytes from the buffer. | ||
2 | Byte Array Input Stream | This class provides methods to read bytes from the byte array. | ||
3 | Data Input Stream | This class provides methods to read Java primitive data types. | ||
4 | File Input Stream | This class provides methods to read bytes from a file. | ||
5 | Filter Input Stream | This class contains methods to read bytes from the other input streams, which are used as the primary source of data. | ||
6 | Object Input Stream | This class provides methods to read objects. | ||
7 | Piped Input Stream | This class provides methods to read from a piped output stream to which the piped input stream must be connected. | ||
8 | Sequence Input Stream | This class provides methods to connect multiple Input Stream and read data from them. | ||
The Input Stream class contains various methods to read the data from an input stream. These methods are overridden by the classes that inherit the Input Stream class.
However, the methods are given in the following table.
SN | Method | Description |
1 | Int read() | This method returns an integer, an integral representation of the next available byte of the input. The integer -1 is returned once the end of the input is encountered. |
2 | Int read (byte buffer []) | This method is used to read the specified buffer length bytes from the input and returns the total number of bytes successfully read. It returns -1 once the end of the input is encountered. |
3 | Int read (byte buffer [], int loc, int nBytes) | This method is used to read the 'nBytes' bytes from the buffer starting at a specified location, 'loc'. It returns the total number of bytes successfully read from the input. It returns -1 once the end of the input is encountered. |
4 | Int available () | This method returns the number of bytes that are available to read. |
5 | Void mark(int nBytes) | This method is used to mark the current position in the input stream until the specified nBytes are read. |
6 | Void reset () | This method is used to reset the input pointer to the previously set mark. |
7 | Long skip (long nBytes) | This method is used to skip the nBytes of the input stream and returns the total number of bytes that are skipped. |
8 | Void close () | This method is used to close the input source. If an attempt is made to read even after the closing, IO Exception is thrown by the method. |
Output Stream Class
● The Output Stream is an abstract class that is used to write 8-bit bytes to the stream. It is the superclass of all the output stream classes.
● It is inherited by various subclasses that are given in the following table.
SN | Class | Description |
1 | Buffered Output Stream | This class provides methods to write the bytes to the buffer. |
2 | Byte Array Output Stream | This class provides methods to write bytes to the byte array. |
3 | Data Output Stream | This class provides methods to write the java primitive data types. |
4 | File Output Stream | This class provides methods to write bytes to a file. |
5 | Filter Output Stream | This class provides methods to write to other output streams. |
6 | Object Output Stream | This class provides methods to write objects. |
7 | Piped Output Stream | It provides methods to write bytes to a piped output stream. |
8 | Print Stream | It provides methods to print Java primitive data types. |
The Output Stream class provides various methods to write bytes to the output streams. The methods are given in the following table.
SN | Method | Description |
1 | Void write (int i) | This method is used to write the specified single byte to the output stream. |
2 | Void write (byte buffer []) | It is used to write a byte array to the output stream. |
3 | Void write (bytes buffer [], int loc, int nBytes) | It is used to write nByte bytes to the output stream from the buffer starting at the specified location. |
4 | Void flush () | It is used to flush the output stream and writes the pending buffered bytes. |
5 | Void close () | It is used to close the output stream. However, if we try to close the already closed output stream, the IO Exception will be thrown by this method. |
Character Stream
● Character Stream classes to overcome the limitations of Byte Stream classes, which can only handle the 8-bit bytes and is not compatible to work directly with the Unicode characters.
● Character Stream classes are used to work with 16-bit Unicode characters. They can perform operations on characters, char arrays and Strings.
● Character Stream classes are mainly used to read characters from the source and write them to the destination.
● These classes are divided into two types of classes, I.e., Reader class and Writer class.
Reader Class
◆ Reader class is used to read the 16-bit characters from the input stream.
◆ All methods of the Reader class throw an IO Exception. The subclasses of the Reader class are given in the following table
SN | Class | Description |
1. | Buffered Reader | It provides methods to read characters from the buffer. |
2. | Char Array Reader | It provides methods to read characters from the char array. |
3. | File Reader | It provides methods to read characters from the file. |
4. | Filter Reader | It provides methods to read characters from the underlying character input stream. |
5 | Input Stream Reader | It provides methods to convert bytes to characters. |
6 | Piped Reader | It provides methods to read characters from the connected piped output stream. |
7 | String Reader | It provides methods to read characters from a string. |
The Reader class methods are given in the following table.
SN | Method | Description |
1 | Int read () | It returns the integral representation of the next character present in the input. It returns -1 if the end of the input is encountered. |
2 | Int read (char buffer []) | It is used to read from the specified buffer. It returns the total number of characters successfully read. It returns -1 if the end of the input is encountered. |
3 | Int read (char buffer [], int loc, int nChars) | It is used to read the specified nChars from the buffer at the specified location. It returns the total number of characters successfully read. |
4 | Void mark (int nchars) | It is used to mark the current position in the input stream until nChars characters are read. |
5 | Void reset () | It is used to reset the input pointer to the previous set mark. |
6 | Long skip (long nChars) | It is used to skip the specified nChars characters from the input stream and returns the number of characters skipped. |
7 | Boolean ready () | It returns a boolean value true if the next request of input is ready. Otherwise, it returns false. |
8 | Void close () | It is used to close the input stream. However, if the program attempts to access the input, it generates IO Exception. |
Writer Class
Writer class is used to write 16-bit Unicode characters to the output stream.
SN | Class | Description |
1 | Buffered Writer | It provides methods to write characters to the buffer. |
2 | File Writer | It provides methods to write characters to the file. |
3 | Char Array Writer | It provides methods to write the characters to the character array. |
4 | Output Stream Writer | It provides methods to convert from bytes to characters. |
5 | Piped Writer | It provides methods to write the characters to the piped output stream. |
6 | String Writer | It provides methods to write the characters to the string. |
The subclasses of the Writer class are used to write the characters onto the output stream. The subclasses of the Writer class are given in the below table.
To write the characters to the output stream, the Write class provides various methods given in the following table.
SN | Method | Description |
1 | Void write () | It is used to write the data to the output stream. |
2 | Void write (int i) | It is used to write a single character to the output stream. |
3 | Void write (char buffer []) | It is used to write the array of characters to the output stream. |
4 | Void write (char buffer [], int loc, int nChars) | It is used to write the nChars characters to the character array from the specified location. |
5 | Void close () | It is used to close the output stream. However, this generates the IO Exception if an attempt is made to write to the output stream after closing the stream. |
6 | Void flush () | It is used to flush the output stream and writes the waiting buffered characters. |
Key takeaway
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Java Console Class
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
Let's see a simple example to read text from console.
- String text=System.console().readLine();
- System.out.println("Text is: "+text);
Java Console class declaration
Let's see the declaration for Java.io.Console class:
- Public final class Console extends Object implements Flushable
Java Console class methods
Method | Description |
Reader reader() | It is used to retrieve the reader object associated with the console |
String readLine() | It is used to read a single line of text from the console. |
String readLine(String fmt, Object... Args) | It provides a formatted prompt then reads the single line of text from the console. |
Char[] readPassword() | It is used to read password that is not being displayed on the console. |
Char[] readPassword(String fmt, Object... Args) | It provides a formatted prompt then reads the password that is not being displayed on the console. |
Console format(String fmt, Object... Args) | It is used to write a formatted string to the console output stream. |
Console printf(String format, Object... Args) | It is used to write a string to the console output stream. |
PrintWriter writer() | It is used to retrieve the PrintWriter object associated with the console. |
Void flush() | It is used to flushes the console. |
How to get the object of Console
System class provides a static method console() that returns the singleton instance of Console class.
- Public static Console console(){}
Let's see the code to get the instance of Console class.
- Console c=System.console();
Java Console Example
- Import java.io.Console;
- Class ReadStringTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter your name: ");
- String n=c.readLine();
- System.out.println("Welcome "+n);
- }
- }
Output
Enter your name: Nakul Jain
Welcome Nakul Jain
Java Console Example to read password
- Import java.io.Console;
- Class ReadPasswordTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter password: ");
- Char[] ch=c.readPassword();
- String pass=String.valueOf(ch);//converting char array into string
- System.out.println("Password is: "+pass);
- }
- }
Output
Enter password:
Password is: 123
Key takeaway
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
We can work with files in Java using the File Class. The java.io package contains this File Class. To use the File class, first create an object of the class and then specify the file's name.
Why is File Handling Necessary?
File handling is an essential aspect of any programming language since it allows us to save the output of any programme in a file and execute operations on it.
To put it another way, file handling is the process of reading and writing data to a file.
Example
// Import the File class
Import java.io.File
// Specify the filename
File obj = new File("filename.txt");
To perform I/O operations on a file, Java employs the concept of a stream. So, let's look at what a Stream is in Java.
What is a Stream?
A stream in Java is a collection of data that can be of two types.
Byte Stream
This primarily applies to byte data. The file handling process with a byte stream is when an input is provided and executed using byte data.
Character Stream
A character stream is a stream in which characters are incorporated. The file handling process with a character stream is the processing of incoming data using characters.
Now that you understand what a stream is, continue reading this article on File Handling in Java to learn about the many methods that may be used to perform actions on files such as generating, reading, and writing.
Java File Methods
The methods for conducting operations on Java files are shown in the table below.
Method | Type | Description |
CanRead() | Boolean | It tests whether the file is readable or not |
CanWrite() | Boolean | It tests whether the file is writable or not |
CreateNewFile() | Boolean | This method creates an empty file |
Delete() | Boolean | Deletes a file |
Exists() | Boolean | It tests whether the file exists |
GetName() | String | Returns the name of the file |
GetAbsolutePath() | String | Returns the absolute pathname of the file |
Length() | Long | Returns the size of the file in bytes |
List() | String[] | Returns an array of the files in the directory |
Mkdir() | Boolean | Creates a directory |
Key takeaway
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
References:
- Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
- CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
- JAVA Complete Reference (9th Edition) Herbait Schelidt.
- T. Budd, “Understanding Object- Oriented Programming with Java”, Pearson Education, Updated Edition (New Java 2 Coverage), 1999.
Unit - 5
Exception Handling and I/O Streams
● The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that the normal flow of the application can be maintained.
● In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
What is Exception in Java
Dictionary Meaning: Exception is an abnormal condition.
In Java, an exception is an event that disrupts the normal flow of the program. It is an object which is thrown at runtime.
What is Exception Handling?
Exception Handling is a mechanism to handle runtime errors such as ClassNotFoundException, IOException, SQLException, RemoteException, etc.
The core advantage of exception handling is to maintain the normal flow of the application. An exception normally disrupts the normal flow of the application that is why we use exception handling. Let's take a scenario:
- Statement 1;
- Statement 2;
- Statement 3;
- Statement 4;
- Statement 5;//exception occurs
- Statement 6;
- Statement 7;
- Statement 8;
- Statement 9;
- Statement 10;
Suppose there are 10 statements in your program and there occurs an exception at statement 5, the rest of the code will not be executed i.e. statement 6 to 10 will not be executed. If we perform exception handling, the rest of the statement will be executed. That is why we use exception handling in Java.
Benefits of exception handling
● When an exception occurs, exception handling guarantees that the program's flow is preserved.
● This allows us to identify the different types of faults.
● This allows us to develop error-handling code separately from the rest of the code.
When an exception occurs, exception handling ensures that the program's flow does not break. For example, if a programme has a large number of statements and an exception occurs in the middle of executing some of them, the statements after the exception will not be executed, and the programme will end abruptly.
By handling, we ensure that all of the statements are executed and that the program's flow is not disrupted.
Key takeaway
The Exception Handling in Java is one of the powerful mechanisms to handle the runtime errors so that the normal flow of the application can be maintained.
Hierarchy of Java Exception classes
The java.lang.Throwable class is the root class of Java Exception hierarchy which is inherited by two subclasses: Exception and Error. A hierarchy of Java Exception classes are given below:
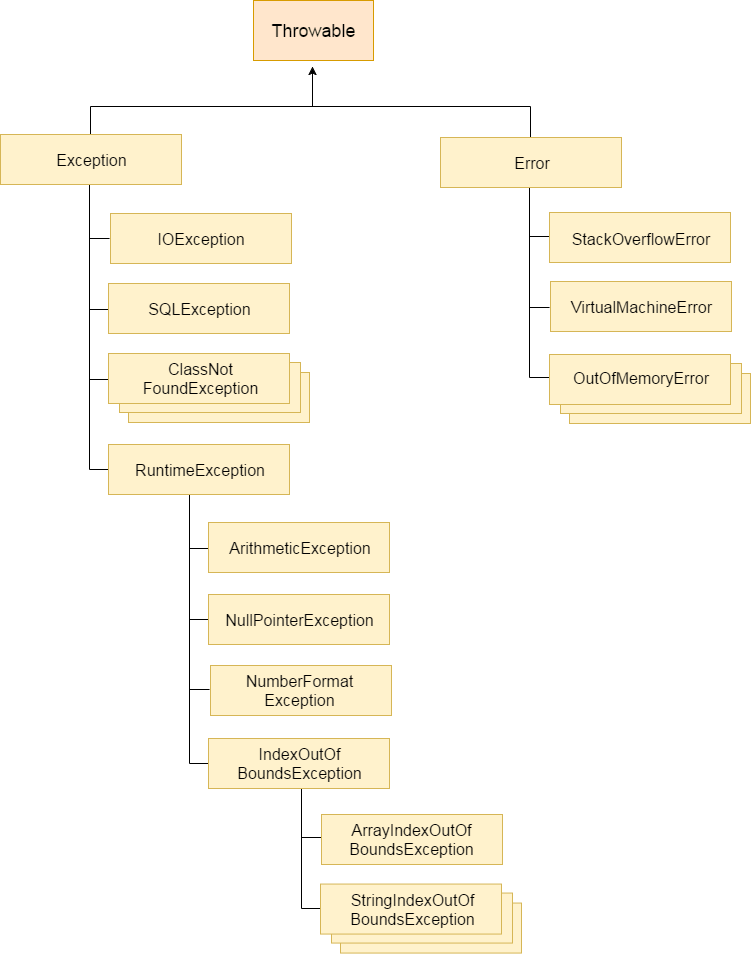
Fig 1 - A hierarchy of Java Exception classes
Types of Java Exceptions
There are mainly two types of exceptions: checked and unchecked. Here, an error is considered as the unchecked exception. According to Oracle, there are three types of exceptions:
- Checked Exception
- Unchecked Exception
- Error
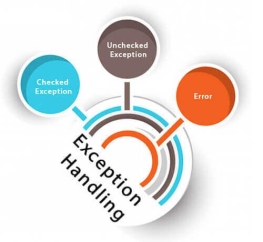
Fig 2 – Exception handling
Difference between Checked and Unchecked Exceptions
1) Checked Exception
The classes which directly inherit Throwable class except Runtime Exception and Error are known as checked exceptions e.g. IO Exception, SQL Exception etc. Checked exceptions are checked at compile-time.
2) Unchecked Exception
The classes which inherit Runtime Exception are known as unchecked exceptions e.g. Arithmetic Exception, Null Pointer Exception, Array Index Out Of Bounds Exception etc. Unchecked exceptions are not checked at compile-time, but they are checked at runtime.
3) Error
Error is irrecoverable e.g. Out Of Memory Error, Virtual Machine Error, Assertion Error etc.
Java Exception Keywords
There are 5 keywords which are used in handling exceptions in Java.
Keyword | Description |
Try | The "try" keyword is used to specify a block where we should place exception code. The try block must be followed by either catch or finally. It means, we can't use try block alone. |
Catch | The "catch" block is used to handle the exception. It must be preceded by try block which means we can't use catch block alone. It can be followed by finally block later. |
Finally | The "finally" block is used to execute the important code of the program. It is executed whether an exception is handled or not. |
Throw | The "throw" keyword is used to throw an exception. |
Throws | The "throws" keyword is used to declare exceptions. It doesn't throw an exception. It specifies that there may occur an exception in the method. It is always used with method signature. |
Java Exception Handling Example
Let's see an example of Java Exception Handling where we using a try-catch statement to handle the exception.
- Public class JavaExceptionExample{
- Public static void main(String args[]){
- Try{
- //code that may raise exception
- Int data=100/0;
- }catch(ArithmeticException e){System.out.println(e);}
- //rest code of the program
- System.out.println("rest of the code...");
- }
- }
Output:
Exception in thread main java.lang.ArithmeticException:/ by zero
Rest of the code…
In the above example, 100/0 raises an Arithmetic Exception which is handled by a try-catch block.
Common Scenarios of Java Exceptions
There are given some scenarios where unchecked exceptions may occur. They are as follows:
1) A scenario where Arithmetic Exception occurs
If we divide any number by zero, there occurs an Arithmetic Exception.
- Int a=50/0;//Arithmetic Exception
2) A scenario where Null Pointer Exception occurs
If we have a null value in any variable, performing any operation on the variable throws a Null Pointer Exception.
- String s=null;
- System.out.println(s.length());//NullPointerException
3) A scenario where Number Format Exception occurs
The wrong formatting of any value may occur Number Format Exception. Suppose I have a string variable that has characters, converting this variable into digit will occur Number Format Exception.
- String s="abc";
- Int i=Integer.parseInt(s);//Number Format Exception
4) A scenario where ArrayIndexOutOfBoundsException occurs
If you are inserting any value in the wrong index, it would result in ArrayIndexOutOfBoundsException as shown below:
- Int a[]=new int[5];
- a[10]=50; //ArrayIndexOutOfBoundsException
Key takeaway
The java.lang.Throwable class is the root class of Java Exception hierarchy which is inherited by two subclasses: Exception and Error.
There are mainly two types of exceptions: checked and unchecked. An error is considered as the unchecked exception. However, according to Oracle, there are three types of exceptions namely:
1) Checked Exception:
❖ The classes that directly inherit the Throwable class except RuntimeException and Error are known as checked exceptions.
❖ For example, IOException, SQLException, etc. Checked exceptions are checked at compile-time.
1. ClassNotFoundException: The ClassNotFoundException is a kind of checked exception that is thrown when we attempt to use a class that does not exist.
Checked exceptions are those exceptions that are checked by the Java compiler itself.
2. FileNotFoundException: The FileNotFoundException is a checked exception that is thrown when we attempt to access a non-existing file.
3. InterruptedException: InterruptedException is a checked exception that is thrown when a thread is in sleeping or waiting state and another thread attempt to interrupt it.
4. InstantiationException: This exception is also a checked exception that is thrown when we try to create an object of abstract class or interface. That is, InstantiationException exception occurs when an abstract class or interface is instantiated.
5. IllegalAccessException: The IllegalAccessException is a checked exception and it is thrown when a method is called in another method or class but the calling method or class does not have permission to access that method.
6. CloneNotSupportedException: This checked exception is thrown when we try to clone an object without implementing the cloneable interface.
7. NoSuchFieldException: This is a checked exception that is thrown when an unknown variable is used in a program.
8. NoSuchMethodException: This checked exception is thrown when the undefined method is used in a program.
2) Unchecked Exception
❖ The classes that inherit the RuntimeException are known as unchecked exceptions.
❖ For example, ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException, etc
❖ Unchecked exceptions are not checked at compile-time, but they are checked at runtime.
Let’s see a brief description of them.
1. ArithmeticException: This exception is thrown when arithmetic problems, such as a number is divided by zero, is occurred. That is, it is caused by maths error.
2. ClassCastException: The ClassCastException is a runtime exception that is thrown by JVM when we attempt to invalid typecasting in the program. That is, it is thrown when we cast an object to a subclass of which an object is not an instance.
3. IllegalArgumentException: This runtime exception is thrown by programmatically when an illegal or appropriate argument is passed to call a method. This exception class has further two subclasses:
● Number Format Exception
● Illegal Thread State Exception
Numeric Format Exception: Number Format Exception is thrown by programmatically when we try to convert a string into the numeric type and the process of illegal conversion fails. That is, it occurs due to the illegal conversion of a string to a numeric format.
Illegal Thread State Exception: Illegal Thread State Exception is a runtime exception that is thrown by programmatically when we attempt to perform any operation on a thread but it is incompatible with the current thread state.
4. IndexOutOfBoundsException: This exception class is thrown by JVM when an array or string is going out of the specified index. It has two further subclasses:
● ArrayIndexOutOfBoundsException
● StringIndexOutOfBoundsException
ArrayIndexOutOfBoundsException: ArrayIndexOutOfBoundsException exception is thrown when an array element is accessed out of the index.
StringIndexOutOfBoundsException: StringIndexOutOfBoundsException exception is thrown when a String or StringBuffer element is accessed out of the index.
5. NullPointerException: NullPointerException is a runtime exception that is thrown by JVM when we attempt to use null instead of an object. That is, it is thrown when the reference is null.
6. ArrayStoreException: This exception occurs when we attempt to store any value in an array which is not of array type. For example, suppose, an array is of integer type but we are trying to store a value of an element of another type.
7. IllegalStateException: The IllegalStateException exception is thrown by programmatically when the runtime environment is not in an appropriate state for calling any method.
8. IllegalMonitorStateException: This exception is thrown when a thread does not have the right to monitor an object and tries to access wait(), notify(), and notifyAll() methods of the object.
9. NegativeArraySizeException: The NegativeArraySizeException exception is thrown when an array is created with a negative size.
3) Error
Error is irrecoverable. Some examples of errors are Out Of Memory Error, Virtual Machine Error, Assertion Error etc.
Difference between Checked and Unchecked Exceptions
Checked Exception | Unchecked Exception |
These exceptions are checked at compile time. These exceptions are handled at compile time too. | These exceptions are just opposite to the checked exceptions. These exceptions are not checked and handled at compile time. |
These exceptions are direct subclasses of exception but not extended from RuntimeException class. | They are the direct subclasses of the RuntimeException class. |
The code gives a compilation error in the case when a method throws a checked exception. The compiler is not able to handle the exception on its own. | The code compiles without any error because the exceptions escape the notice of the compiler. These exceptions are the results of user-created errors in programming logic. |
These exceptions mostly occur when the probability of failure is too high. | These exceptions occur mostly due to programming mistakes. |
Common checked exceptions include IOException, DataAccessException, InterruptedException, etc. | Common unchecked exceptions include ArithmeticException, InvalidClassException, NullPointerException, etc. |
These exceptions are propagated using the throws keyword. | These are automatically propagated. |
It is required to provide the try-catch and try-finally block to handle the checked exception. | In the case of unchecked exception it is not mandatory. |
Key takeaway
The classes that directly inherit the Throwable class except RuntimeException and Error are known as checked exceptions.
The classes that inherit the RuntimeException are known as unchecked exceptions.
Java provides five keywords that are used to handle the exception. The following table describes each.
Keyword | Description |
Try | The "try" keyword is used to specify a block where we should place an exception code. It means we can't use try block alone. The try block must be followed by either catch or finally. |
Catch | The "catch" block is used to handle the exception. It must be preceded by try block which means we can't use catch block alone. It can be followed by finally block later. |
Finally | The "finally" block is used to execute the necessary code of the program. It is executed whether an exception is handled or not. |
Throw | The "throw" keyword is used to throw an exception. |
Throws | The "throws" keyword is used to declare exceptions. It specifies that there may occur an exception in the method. It doesn't throw an exception. It is always used with method signature. |
A) try block:
a) Syntax of try block with catch block:
Try {
//Block of statement}
Catch(Exception Handler class)
{
}
b) Syntax of try block with finally block:
Try {
//Block of statement}
Finally {
}
c) Syntax of try block with catch and finally block:
Try {
//Block of statement}
Catch(Exception Handler class)
{
} finally{
}
B) Catch block:
a) Syntax of try block with catch block:
Try {
//Block of statement}
Catch(Exception Handler class)
{
}
b) Multiple catch block:
Syntax of try block with catch block:
Try {
//Block of statement}
Catch(Exception Handler subclass)
{
}catch(Exception Handler super class){
}
C) Nested try block:
Syntax of nested try block
Try{ // block of statement
Try{
// block of statement
}
Catch(Exception Handler class)
{
}
Catch(Exception Handler class)
{
}
D) Finally:
a) Syntax of finally without catch
Try{
//block of statement
}
Finally {
}
b) Syntax of finally with catch
Try {
//Block of statement}
Catch(Exception Handler class)
{
} finally{
}
E) throw:
Syntax:
Throw exception_object;
F) throws:
Syntax:
MethodName () throws comma separated list of exceptionClassNames{}
Difference between throw and throws
Throw keyword | Throws keyword |
|
|
Difference between final, finally and finalize
● The final, finally, and finalize are keywords in Java that are used in exception handling. Each of these keywords has a different functionality.
● The basic difference between final, finally and finalize is that the final is an access modifier, finally is the block in Exception Handling and finalize is the method of object class.
Key | Final | Finally | Finalize |
Definition | Final is the keyword and access modifier which is used to apply restrictions on a class, method or variable. | Finally is the block in Java Exception Handling to execute the important code whether the exception occurs or not. | Finalize is the method in Java which is used to perform clean up processing just before object is garbage collected. |
Applicable to | Final keyword is used with the classes, methods and variables. | Finally block is always related to the try and catch block in exception handling. | Finalize() method is used with the objects. |
Functionality | (1) Once declared, final variable becomes constant and cannot be modified. | (1) finally block runs the important code even if exception occurs or not. | Finalize method performs the cleaning activities with respect to the object before its destruction. |
Execution | Final method is executed only when we call it. | Finally block is executed as soon as the try-catch block is executed. It's execution is not dependant on the exception. | Finalize method is executed just before the object is destroyed. |
Java try block
Java try block is used to enclose the code that might throw an exception. It must be used within the method.
If an exception occurs at the particular statement of try block, the rest of the block code will not execute. So, it is recommended not to keeping the code in try block that will not throw an exception.
Java try block must be followed by either catch or finally block.
Syntax of Java try-catch
- Try{
- //code that may throw an exception
- }catch(Exception_class_Name ref){}
Syntax of try-finally block
- Try{
- //code that may throw an exception
- }finally{}
Java catch block
Java catch block is used to handle the Exception by declaring the type of exception within the parameter. The declared exception must be the parent class exception ( i.e., Exception) or the generated exception type. However, the good approach is to declare the generated type of exception.
The catch block must be used after the try block only. You can use multiple catch block with a single try block.
Problem without exception handling
Let's try to understand the problem if we don't use a try-catch block.
Example 1
- Public class TryCatchExample1 {
- Public static void main(String[] args) {
- Int data=50/0; //may throw exception
- System.out.println("rest of the code");
- }
- }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
As displayed in the above example, the rest of the code is not executed (in such case, the rest of the code statement is not printed).
There can be 100 lines of code after exception. So all the code after exception will not be executed.
Solution by exception handling
Let's see the solution of the above problem by a java try-catch block.
Example 2
- Public class TryCatchExample2 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- //handling the exception
- Catch(ArithmeticException e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Java.lang.ArithmeticException: / by zero
Rest of the code
Now, as displayed in the above example, the rest of the code is executed, i.e., the rest of the code statement is printed.
Example 3
In this example, we also kept the code in a try block that will not throw an exception.
- Public class TryCatchExample3 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- // if exception occurs, the remaining statement will not exceute
- System.out.println("rest of the code");
- }
- // handling the exception
- Catch(ArithmeticException e)
- {
- System.out.println(e);
- }
- }
- }
Output:
Java.lang.ArithmeticException: / by zero
Here, we can see that if an exception occurs in the try block, the rest of the block code will not execute.
Example 4
Here, we handle the exception using the parent class exception.
- Public class TryCatchExample4 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- // handling the exception by using Exception class
- Catch(Exception e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Java.lang.ArithmeticException: / by zero
Rest of the code
Example 5
Let's see an example to print a custom message on exception.
- Public class TryCatchExample5 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- // handling the exception
- Catch(Exception e)
- {
- // displaying the custom message
- System.out.println("Can't divided by zero");
- }
- }
- }
Output:
Can't divided by zero
Example 6
Let's see an example to resolve the exception in a catch block.
- Public class TryCatchExample6 {
- Public static void main(String[] args) {
- Int i=50;
- Int j=0;
- Int data;
- Try
- {
- Data=i/j; //may throw exception
- }
- // handling the exception
- Catch(Exception e)
- {
- // resolving the exception in catch block
- System.out.println(i/(j+2));
- }
- }
- }
Output:
25
Example 7
In this example, along with try block, we also enclose exception code in a catch block.
- Public class TryCatchExample7 {
- Public static void main(String[] args) {
- Try
- {
- Int data1=50/0; //may throw exception
- }
- // handling the exception
- Catch(Exception e)
- {
- // generating the exception in catch block
- Int data2=50/0; //may throw exception
- }
- System.out.println("rest of the code");
- }
- }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
Here, we can see that the catch block didn't contain the exception code. So, enclose exception code within a try block and use catch block only to handle the exceptions.
Example 8
In this example, we handle the generated exception (Arithmetic Exception) with a different type of exception class (ArrayIndexOutOfBoundsException).
- Public class TryCatchExample8 {
- Public static void main(String[] args) {
- Try
- {
- Int data=50/0; //may throw exception
- }
- // try to handle the ArithmeticException using ArrayIndexOutOfBoundsException
- Catch(ArrayIndexOutOfBoundsException e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Exception in thread "main" java.lang.ArithmeticException: / by zero
Example 9
Let's see an example to handle another unchecked exception.
- Public class TryCatchExample9 {
- Public static void main(String[] args) {
- Try
- {
- Int arr[]= {1,3,5,7};
- System.out.println(arr[10]); //may throw exception
- }
- // handling the array exception
- Catch(ArrayIndexOutOfBoundsException e)
- {
- System.out.println(e);
- }
- System.out.println("rest of the code");
- }
- }
Output:
Java.lang.ArrayIndexOutOfBoundsException: 10
Rest of the code
Example 10
Let's see an example to handle checked exception.
- Import java.io.FileNotFoundException;
- Import java.io.PrintWriter;
- Public class TryCatchExample10 {
- Public static void main(String[] args) {
- PrintWriter pw;
- Try {
- Pw = new PrintWriter("jtp.txt"); //may throw exception
- Pw.println("saved");
- }
- // providing the checked exception handler
- Catch (FileNotFoundException e) {
- System.out.println(e);
- }
- System.out.println("File saved successfully");
- }
- }
Output:
File saved successfully
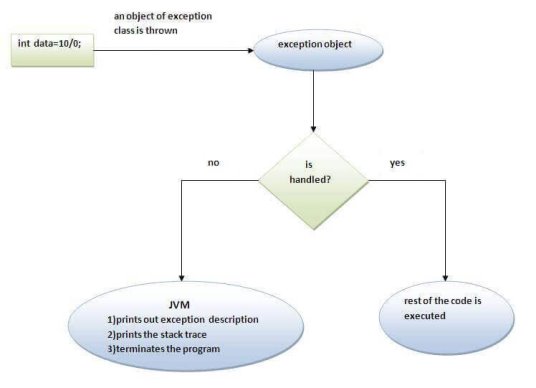
Fig 3: Internal working of java try-catch block
The JVM firstly checks whether the exception is handled or not. If exception is not handled, JVM provides a default exception handler that performs the following tasks:
● Prints out exception description.
● Prints the stack trace (Hierarchy of methods where the exception occurred).
● Causes the program to terminate.
But if exception is handled by the application programmer, normal flow of the application is maintained i.e. rest of the code is executed.
Key takeaway
Java try block is used to enclose the code that might throw an exception. It must be used within the method.
Java catch block is used to handle the Exception by declaring the type of exception within the parameter.
You can use the throw keyword to re-throw an exception that has been cached in a catch block (which is used to throw the exception objects).
You can re-throw the same exception without altering it while re-throwing exceptions.
Try {
Int result = (arr[a])/(arr[b]);
System.out.println("Result of "+arr[a]+"/"+arr[b]+": "+result);
}
Catch(ArithmeticException e) {
Throw e;
}
Alternatively, you can wrap it in a new exception and throw it. Exception chaining or exception wrapping is when you wrap a cached exception within another exception and throw it. This allows you to change your exception by throwing a higher level of exception while retaining the abstraction.
Try {
Int result = (arr[a])/(arr[b]);
System.out.println("Result of "+arr[a]+"/"+arr[b]+": "+result);
}
Catch(ArrayIndexOutOfBoundsException e) {
Throw new IndexOutOfBoundsException();
}
Example
In the Java example below, our demoMethod() code may throw an ArrayIndexOutOfBoundsException and an ArithmeticException. These two exceptions are caught in two different catch blocks.
We re-throw both exceptions in the catch blocks, one by wrapping it within the higher exceptions and the other directly.
Import java.util.Arrays;
Import java.util.Scanner;
Public class RethrowExample {
Public void demoMethod() {
Scanner sc = new Scanner(System.in);
Int[] arr = {10, 20, 30, 2, 0, 8};
System.out.println("Array: "+Arrays.toString(arr));
System.out.println("Choose numerator and denominator(not 0) from this array (enter positions 0 to 5)");
Int a = sc.nextInt();
Int b = sc.nextInt();
Try {
Int result = (arr[a])/(arr[b]);
System.out.println("Result of "+arr[a]+"/"+arr[b]+": "+result);
}
Catch(ArrayIndexOutOfBoundsException e) {
Throw new IndexOutOfBoundsException();
}
Catch(ArithmeticException e) {
Throw e;
}
}
Public static void main(String [] args) {
New RethrowExample().demoMethod();
}
}
Output1
Array: [10, 20, 30, 2, 0, 8]
Choose numerator and denominator(not 0) from this array (enter positions 0 to 5)
0
4
Exception in thread "main" java.lang.ArithmeticException: / by zero
At myPackage.RethrowExample.demoMethod(RethrowExample.java:16)
At myPackage.RethrowExample.main(RethrowExample.java:25)
Output2
Array: [10, 20, 30, 2, 0, 8]
Choose numerator and denominator(not 0) from this array (enter positions 0 to 5)
124
5
Exception in thread "main" java.lang.IndexOutOfBoundsException
At myPackage.RethrowExample.demoMethod(RethrowExample.java:17)
At myPackage.RethrowExample.main(RethrowExample.java:23)
The graphical user interface (GUI) is a key visible display of objects that the user sees and interacts with on the screen in the world of computer programming (GUI). You've always known what a GUI is, even if you didn't realize it was called that. It's what you're engaging with right now as you navigate the web using whatever browser and operating system you're using.
There are numerous programming languages available, and Java is simply one of them. For each language, there are special words linked to the GUI code. Because each language has its unique set of codes, it's important to master the right programming syntax (rules) for each language to maximize efficiency. Learning the syntax for the GUI in Java so that you may master these programming skills and utilize them in your future projects is reason enough to understand the syntax for the GUI in Java.
GUI Components
Components are add-ons or extensions to containers that help fill in the gaps in the content and make up the rest of the user interface. A textbox, a button, a checkbox, or even a simple label are all examples of controls. When you mix both containers and components, you begin to see the bigger picture of what the GUI is and why it is at the heart of every programme or application's user experience.
● A graphical user interface component (GUI component) is an object that represents a screen element like a button or a text field.
● The java.awt and javax.swing packages are primarily responsible for GUI-related classes.
● The Abstract Windowing Toolkit (AWT) was the first Java graphical user interface library.
● The Swing package adds new components and makes them more versatile.
● To make a Java GUI-based programme, you'll need both packages.
Labels, text fields, text areas, buttons, and other graphical user interface components are all available in Java. Containers in the Abstract Windowing Toolkit (AWT) can also contain these components. Frames (windows), canvases (for drawing), and panels are examples of containers (which are used to group components). Frames (windows) hold panels and canvases, whereas buttons and other components can be placed directly on the frames or in panels within the frames.
When the window in which these GUI components are located is drawn, they are automatically drawn. As a result, we won't have to type commands to draw them.
The event model in Java is used to manage these GUI components.
When a user interacts with a component (clicks a button, types in a field, selects from a pop-up menu, etc. ), the component that you connect with generates an event. The programmer must specify one or more objects for each component of your application to "listen" for events from that component. If your software contains a "start" button, you must designate one or more objects to be informed when the user presses the button.
Buttons
Button is a class in the java.awt package that represents screen buttons. The constructor is:
Public Button(String label);
When this code is run, it produces a button with the word "label" printed on it. In most cases, the button is large enough to see the label. a parameterless constructor is also available for creating an unlabeled button.
Buttons react to a wide range of signals. You may, for example, include a
Public void addActionListener(ActionListener listener);
Adding buttons to a Frame or Panel
By delivering the message: "Add a button to a frame or panel," we can add a button to a frame or panel.
MyFrame.add(startButton);
Normally we include such code in the constructor for the frame or panel. Hence usually we just write this. Add(startButton) or simply add(startButton).
Here's some code for ButtonDemo, a class that represents a Frame specialization with two buttons. The class extends Frame from the java.awt package. The constructor for Frame takes a String parameter and creates a window with that string in the title bar. The constructor for ButtonDemo calls the superclass constructor, and then sets the size of the new Frame to be 400 x 200. The setLayout command instructs the new Frame to add new components across the panel from left to right.
If there isn't enough room, new components will be put in new rows, starting from the left. The button-making code should be self-explanatory. The buttons are added to the frame using the add commands. The ButtonListener class is explained further down. It builds objects that respond to button clicks by doing actions. As a result, we instruct the two buttons to notify myButtonListener when the user clicks on them (using the addActionListener method). Finally, because Frame, Button, and FlowLayout are all classes in the java.awt package, we had to import java.awt.*.
Import java.awt.*;
Public class ButtonDemo extends Frame
{
Protected Button startButton, stopButton;
// Constructor sets features of the frame, creates buttons,
// adds them to the frame, and assigns an object to listen
// to them
Public ButtonDemo()
{
Super("Button demo"); // calls Frame constructor
// which adds title to window
SetSize(400,200); // sets the size of the window
// sets layout so objects added go from left to right
// until fill up row and then go to next row.
// There are other layout options, including GridLayout.
SetLayout(new FlowLayout());
// create two new buttons w/labels start and stop
StartButton = new Button("Start");
StopButton = new Button("Stop");
Add(startButton); // add buttons to frame
Add(stopButton);
// create an object to listen to both buttons:
ButtonListener myButtonListener = new ButtonListener();
// tell buttons that myButtonListener should be notified
StartButton.addActionListener(myButtonListener);
StopButton.addActionListener(myButtonListener);
SetVisible(true); // Show the window on the screen.
// Leave this out and you won't see it!
}
// Trivial main program associated with ButtonDemo
// Simply creates it and shows it.
Public static void main(String args[]){
// Create an instance of Buttons
ButtonDemo app = new ButtonDemo();
}
}
The class's main programme just produces an object from the class, which then displays itself on the screen. This minimum amount of work is nearly always done by main programmes associated with frames.
ActionListeners for Buttons
Objects that implement the ActionListener interface must implement the actionPerformed method, which accepts an ActionEvent parameter. When a button is pressed, any objects added as ActionListeners for that button are notified by executing their actionPerformed function with an argument containing information about the specific event that occurred. As a result, the system constructs the ActionEvent object and delivers it to the listener automatically.
The most useful methods of ActionEvent are
Public String getActionCommand();
And
Public Object getSource();
If you send getActionCommand to an ActionEvent that represents a button click, it will return a string that corresponds to the button's label. GetSource returns the object where the event occurred as a reference. Because the class ButtonListener implements ActionListener, an object of this class can be used as a listener on a button. The actionPerformed method is straightforward. It utilises getActionCommand to get the label of the button that was clicked, and then prints a different message depending on what the button was. (Recall that each of the two buttons in ButtonDemo has the same ActionListener, so getActionCommand needs to find out which one was clicked.)
This class has no explicit function Object() { [native code] } because it is so simple (it doesn't even have instance variables). Instead, it contains a default function Object() { [native code] } (with no parameters) that simply allocates storage for newly built instances, exactly like all other classes. Because ActionEvent and the ActionListener interface are both in the java.awt.event package, we must import it.
Import java.awt.event.*;
Public class ButtonListener implements ActionListener{
Public void actionPerformed(ActionEvent evt)
{
// Get label of the button clicked in event passed in
String arg = evt.getActionCommand();
If (arg.equals("Start"))
System.out.println("Start button");
Else if (arg.equals("Stop"))
System.out.println("Stop button");
}
}
Labels
A Label is a straightforward component that holds a string. The assemblers are
Public Label() // creates label with no text
Public Label(String text) //create label with text
The methods offered are as follows:
Public String getText() // return label text
Public void setText(String s) // sets the label text
The wording in Labels, on the other hand, is rarely changed.
Text Fields
A TextField is a single-line input field where the user can type. It is an effective method of obtaining text input from the user. The assemblers are
Public TextField () // creates text field with no text
Public TextField (int columns)
// create text field with appropriate # of columns
Public TextField (String s) // create text field with s displayed
Public TextField (String s, int columns)
// create text field with s displayed & approp. Width
Methods
Public void setEditable(boolean s)
// if false the TextField is not user editable
Public String getText() // return label text
Public void setText(String s) // sets the label text
For this component, there are a slew of different options.
When a user fills into a text field and then presses the return or enter key, an event is generated that may be handled by the same ActionListener that is used with Buttons. However, getActionCommand does not return any relevant String in this scenario. The method getSource is used in this case. As a result, we can write: to reply to the user hitting the return key while typing in a TextField myField:
Public void actionPerformed(ActionEvent evt)
{
If(evt.getSource() == myField){
String contents = myField.getText();
System.out.println("The field contained: "+contents);
} else ...
}
It's worth noting that using myField in actionPerformed only makes sense if we have either an inner class (and hence access to all of the outer class's instance variables) or if the Frame or Panel where myField is specified is the related ActionListener.
You can link a TextListener to the TextField if you want to be alerted whenever any modification is made to it (whether or not the user hits the return key). Details can be found in the online documentation.
Text Areas
TextArea is a class that allows you to create a text area that can accommodate many lines of text. It's similar to TextField with the exception that no special event is triggered when the return key is pressed.
The assemblers are
Public TextArea(int rows, int columns)
// create text area with appropriate # rows and columns
Public TextArea(String s, int rows, in columns)
// create text area with rows, columns, and displaying s
Methods
Public void setEditable(boolean s)
// if false the TextArea is not user editable
Public String getText() // return text in TextArea
Public void setText(String s) // sets the text
Key takeaway
Components are add-ons or extensions to containers that help fill in the gaps in the content and make up the rest of the user interface. A textbox, a button, a checkbox, or even a simple label are all examples of controls.
Java Database Connectivity (JDBC) is a Java application programming interface (API) that specifies how a client can interact with a database. It is a Java-based data access technology that is used to link Java databases. Oracle Corporation's Java Standard Edition platform includes it. It is geared toward relational databases and provides techniques for querying and updating data in a database. In the Java virtual machine (JVM) host environment, a JDBC-to-ODBC bridge allows connections to any ODBC-accessible data source.
Java Database Connectivity (JDBC) is a Sun Microsystems specification that provides a common abstraction (API or Protocol) for Java programmes to interface with multiple databases. It implements the Java database connectivity standard in the language. It's used to create programmes that connect to databases. Databases and spreadsheets can be accessed via JDBC and the database driver. JDBC APIs can be used to access enterprise data stored in a relational database (RDB).
JDBC is an API (application programming interface) for interacting with databases in Java programming.
JDBC classes and interfaces enable applications to communicate user requests to a specific database.
JDBC's Purpose
Enterprise applications built with the JAVA EE technology must connect with databases in order to store application-specific data. As a result, communicating with a database necessitates effective database connectivity, which can be accomplished with the ODBC (Open database connectivity) driver. This driver is used in conjunction with JDBC to connect or communicate with a variety of databases, including Oracle, MS Access, Mysql, and SQL Server.
Components
JDBC is made up of four basic components that allow it to interact with a database. The following is a list of them:
● JDBC API - It offers a variety of methods and interfaces for interacting with databases.
It includes the following two packages, which contain the Java SE and Java EE platforms to demonstrate WORA (write once run anywhere) capabilities.
1. Java.sql.*;
2. Javax.sql.*;
It also establishes a standard for connecting a client application to a database.
● JDBC Driver Manager - The JDBC Driver Manager loads a database-specific driver into an application in order to connect to a database. It's used to process the user's request by making a database-specific call to the database.
● JDBC Test Suite - It is used to test JDBC Drivers' operations (such as insertion, deletion, and updating).
● JDBC-ODBC Bridge Drivers - Bridge Drivers for JDBC and ODBC: It establishes a connection between database drivers and the database. This bridge converts ODBC function calls to JDBC method calls. It makes use of the
Sun.jdbc.odbc
This package contains a native library for accessing ODBC properties.
Key takeaway
Java Database Connectivity (JDBC) is a Java application programming interface (API) that specifies how a client can interact with a database. It is a Java-based data access technology that is used to link Java databases.
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Stream:
● A stream is a method to sequentially access a file.
● I/O Stream means an input source or output destination representing different types of sources
● e.g., disk files. The java.io package provides classes that allow you to convert between Unicode character streams and byte streams of non-Unicode text.
Stream – A sequence of data.
Input Stream: reads data from source.
Output Stream: writes data to destination.

Fig 4 - Stream
Java provides strong but flexible support for I/O related to files and networks but this tutorial covers very basic functionality related to streams and I/O
Byte Stream Classes:
● Byte Stream classes are used to read bytes from the input stream and write bytes to the output stream.
● In other word, Byte Stream classes read/write the data of 8-bits. We can store video, audio, characters, etc., by using Byte Stream classes.
● These classes are part of the java.io package.
● The Byte Stream classes are divided into two types of classes, i.e., Input Stream and Output Stream. These classes are abstract and the super classes of all the Input/Output stream classes.
Input Stream Class:
The Input Stream class provides methods to read bytes from a file, console or memory. It is an abstract class
SN | Class | Description | ||
1 | Buffered Input Stream | This class provides methods to read bytes from the buffer. | ||
2 | Byte Array Input Stream | This class provides methods to read bytes from the byte array. | ||
3 | Data Input Stream | This class provides methods to read Java primitive data types. | ||
4 | File Input Stream | This class provides methods to read bytes from a file. | ||
5 | Filter Input Stream | This class contains methods to read bytes from the other input streams, which are used as the primary source of data. | ||
6 | Object Input Stream | This class provides methods to read objects. | ||
7 | Piped Input Stream | This class provides methods to read from a piped output stream to which the piped input stream must be connected. | ||
8 | Sequence Input Stream | This class provides methods to connect multiple Input Stream and read data from them. | ||
The Input Stream class contains various methods to read the data from an input stream. These methods are overridden by the classes that inherit the Input Stream class.
However, the methods are given in the following table.
SN | Method | Description |
1 | Int read() | This method returns an integer, an integral representation of the next available byte of the input. The integer -1 is returned once the end of the input is encountered. |
2 | Int read (byte buffer []) | This method is used to read the specified buffer length bytes from the input and returns the total number of bytes successfully read. It returns -1 once the end of the input is encountered. |
3 | Int read (byte buffer [], int loc, int nBytes) | This method is used to read the 'nBytes' bytes from the buffer starting at a specified location, 'loc'. It returns the total number of bytes successfully read from the input. It returns -1 once the end of the input is encountered. |
4 | Int available () | This method returns the number of bytes that are available to read. |
5 | Void mark(int nBytes) | This method is used to mark the current position in the input stream until the specified nBytes are read. |
6 | Void reset () | This method is used to reset the input pointer to the previously set mark. |
7 | Long skip (long nBytes) | This method is used to skip the nBytes of the input stream and returns the total number of bytes that are skipped. |
8 | Void close () | This method is used to close the input source. If an attempt is made to read even after the closing, IO Exception is thrown by the method. |
Output Stream Class
● The Output Stream is an abstract class that is used to write 8-bit bytes to the stream. It is the superclass of all the output stream classes.
● It is inherited by various subclasses that are given in the following table.
SN | Class | Description |
1 | Buffered Output Stream | This class provides methods to write the bytes to the buffer. |
2 | Byte Array Output Stream | This class provides methods to write bytes to the byte array. |
3 | Data Output Stream | This class provides methods to write the java primitive data types. |
4 | File Output Stream | This class provides methods to write bytes to a file. |
5 | Filter Output Stream | This class provides methods to write to other output streams. |
6 | Object Output Stream | This class provides methods to write objects. |
7 | Piped Output Stream | It provides methods to write bytes to a piped output stream. |
8 | Print Stream | It provides methods to print Java primitive data types. |
The Output Stream class provides various methods to write bytes to the output streams. The methods are given in the following table.
SN | Method | Description |
1 | Void write (int i) | This method is used to write the specified single byte to the output stream. |
2 | Void write (byte buffer []) | It is used to write a byte array to the output stream. |
3 | Void write (bytes buffer [], int loc, int nBytes) | It is used to write nByte bytes to the output stream from the buffer starting at the specified location. |
4 | Void flush () | It is used to flush the output stream and writes the pending buffered bytes. |
5 | Void close () | It is used to close the output stream. However, if we try to close the already closed output stream, the IO Exception will be thrown by this method. |
Character Stream
● Character Stream classes to overcome the limitations of Byte Stream classes, which can only handle the 8-bit bytes and is not compatible to work directly with the Unicode characters.
● Character Stream classes are used to work with 16-bit Unicode characters. They can perform operations on characters, char arrays and Strings.
● Character Stream classes are mainly used to read characters from the source and write them to the destination.
● These classes are divided into two types of classes, I.e., Reader class and Writer class.
Reader Class
◆ Reader class is used to read the 16-bit characters from the input stream.
◆ All methods of the Reader class throw an IO Exception. The subclasses of the Reader class are given in the following table
SN | Class | Description |
1. | Buffered Reader | It provides methods to read characters from the buffer. |
2. | Char Array Reader | It provides methods to read characters from the char array. |
3. | File Reader | It provides methods to read characters from the file. |
4. | Filter Reader | It provides methods to read characters from the underlying character input stream. |
5 | Input Stream Reader | It provides methods to convert bytes to characters. |
6 | Piped Reader | It provides methods to read characters from the connected piped output stream. |
7 | String Reader | It provides methods to read characters from a string. |
The Reader class methods are given in the following table.
SN | Method | Description |
1 | Int read () | It returns the integral representation of the next character present in the input. It returns -1 if the end of the input is encountered. |
2 | Int read (char buffer []) | It is used to read from the specified buffer. It returns the total number of characters successfully read. It returns -1 if the end of the input is encountered. |
3 | Int read (char buffer [], int loc, int nChars) | It is used to read the specified nChars from the buffer at the specified location. It returns the total number of characters successfully read. |
4 | Void mark (int nchars) | It is used to mark the current position in the input stream until nChars characters are read. |
5 | Void reset () | It is used to reset the input pointer to the previous set mark. |
6 | Long skip (long nChars) | It is used to skip the specified nChars characters from the input stream and returns the number of characters skipped. |
7 | Boolean ready () | It returns a boolean value true if the next request of input is ready. Otherwise, it returns false. |
8 | Void close () | It is used to close the input stream. However, if the program attempts to access the input, it generates IO Exception. |
Writer Class
Writer class is used to write 16-bit Unicode characters to the output stream.
SN | Class | Description |
1 | Buffered Writer | It provides methods to write characters to the buffer. |
2 | File Writer | It provides methods to write characters to the file. |
3 | Char Array Writer | It provides methods to write the characters to the character array. |
4 | Output Stream Writer | It provides methods to convert from bytes to characters. |
5 | Piped Writer | It provides methods to write the characters to the piped output stream. |
6 | String Writer | It provides methods to write the characters to the string. |
The subclasses of the Writer class are used to write the characters onto the output stream. The subclasses of the Writer class are given in the below table.
To write the characters to the output stream, the Write class provides various methods given in the following table.
SN | Method | Description |
1 | Void write () | It is used to write the data to the output stream. |
2 | Void write (int i) | It is used to write a single character to the output stream. |
3 | Void write (char buffer []) | It is used to write the array of characters to the output stream. |
4 | Void write (char buffer [], int loc, int nChars) | It is used to write the nChars characters to the character array from the specified location. |
5 | Void close () | It is used to close the output stream. However, this generates the IO Exception if an attempt is made to write to the output stream after closing the stream. |
6 | Void flush () | It is used to flush the output stream and writes the waiting buffered characters. |
Key takeaway
The java.io package contains nearly every class you might ever need to perform input and output (I/O) in Java. All these streams represent an input source and an output destination. The stream in the java.io package supports many data such as primitives, object, localized characters, etc.
Java Console Class
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
Let's see a simple example to read text from console.
- String text=System.console().readLine();
- System.out.println("Text is: "+text);
Java Console class declaration
Let's see the declaration for Java.io.Console class:
- Public final class Console extends Object implements Flushable
Java Console class methods
Method | Description |
Reader reader() | It is used to retrieve the reader object associated with the console |
String readLine() | It is used to read a single line of text from the console. |
String readLine(String fmt, Object... Args) | It provides a formatted prompt then reads the single line of text from the console. |
Char[] readPassword() | It is used to read password that is not being displayed on the console. |
Char[] readPassword(String fmt, Object... Args) | It provides a formatted prompt then reads the password that is not being displayed on the console. |
Console format(String fmt, Object... Args) | It is used to write a formatted string to the console output stream. |
Console printf(String format, Object... Args) | It is used to write a string to the console output stream. |
PrintWriter writer() | It is used to retrieve the PrintWriter object associated with the console. |
Void flush() | It is used to flushes the console. |
How to get the object of Console
System class provides a static method console() that returns the singleton instance of Console class.
- Public static Console console(){}
Let's see the code to get the instance of Console class.
- Console c=System.console();
Java Console Example
- Import java.io.Console;
- Class ReadStringTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter your name: ");
- String n=c.readLine();
- System.out.println("Welcome "+n);
- }
- }
Output
Enter your name: Nakul Jain
Welcome Nakul Jain
Java Console Example to read password
- Import java.io.Console;
- Class ReadPasswordTest{
- Public static void main(String args[]){
- Console c=System.console();
- System.out.println("Enter password: ");
- Char[] ch=c.readPassword();
- String pass=String.valueOf(ch);//converting char array into string
- System.out.println("Password is: "+pass);
- }
- }
Output
Enter password:
Password is: 123
Key takeaway
The Java Console class is be used to get input from console. It provides methods to read texts and passwords.
If you read password using Console class, it will not be displayed to the user.
The java.io.Console class is attached with system console internally. The Console class is introduced since 1.5.
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
We can work with files in Java using the File Class. The java.io package contains this File Class. To use the File class, first create an object of the class and then specify the file's name.
Why is File Handling Necessary?
File handling is an essential aspect of any programming language since it allows us to save the output of any programme in a file and execute operations on it.
To put it another way, file handling is the process of reading and writing data to a file.
Example
// Import the File class
Import java.io.File
// Specify the filename
File obj = new File("filename.txt");
To perform I/O operations on a file, Java employs the concept of a stream. So, let's look at what a Stream is in Java.
What is a Stream?
A stream in Java is a collection of data that can be of two types.
Byte Stream
This primarily applies to byte data. The file handling process with a byte stream is when an input is provided and executed using byte data.
Character Stream
A character stream is a stream in which characters are incorporated. The file handling process with a character stream is the processing of incoming data using characters.
Now that you understand what a stream is, continue reading this article on File Handling in Java to learn about the many methods that may be used to perform actions on files such as generating, reading, and writing.
Java File Methods
The methods for conducting operations on Java files are shown in the table below.
Method | Type | Description |
CanRead() | Boolean | It tests whether the file is readable or not |
CanWrite() | Boolean | It tests whether the file is writable or not |
CreateNewFile() | Boolean | This method creates an empty file |
Delete() | Boolean | Deletes a file |
Exists() | Boolean | It tests whether the file exists |
GetName() | String | Returns the name of the file |
GetAbsolutePath() | String | Returns the absolute pathname of the file |
Length() | Long | Returns the size of the file in bytes |
List() | String[] | Returns an array of the files in the directory |
Mkdir() | Boolean | Creates a directory |
Key takeaway
In Java, file handling entails reading and writing data to a file. The java.io package's File class allows us to work with a variety of file formats. You must create an object of the File class and supply the filename or directory name in order to utilize it.
References:
- Programming in Java. Second Edition. Oxford Higher Education. (Sachin Malhotra/Saura V Choudhary)
- CORE JAVA For Beginners. (Rashmi Kanta Das), Vikas Publication
- JAVA Complete Reference (9th Edition) Herbait Schelidt.
- T. Budd, “Understanding Object- Oriented Programming with Java”, Pearson Education, Updated Edition (New Java 2 Coverage), 1999.