Unit 6
Queue
Queue
Basic features of Queue
Applications of Queue
Implementation of Queue arrangement
1. Queue
A queue is an object or more specifically as abstract data structure that permits the subsequent operations
Working of Queue
Queue operations work as follows:
Enqueue Operation
Dequeue Operation
Complexity Analysis of Queue Operations
Representation of queue using arrays
H | E | L | L | O |
|
|
|
|
|
|
|
|
|
0 1 2 3 4 5 6
Front=0 rear=4
Algorithm to insert any element in a queue
Step 1: IF REAR = MAX - 1
Write OVERFLOW
Go to step
[END OF IF]
Step 2: IF FRONT = -1 and REAR = -1
SET FRONT = REAR = 0
ELSE
SET REAR = REAR + 1
[END OF IF]
Step 3: Set QUEUE[REAR] = NUM
Step 4: EXIT
H | E | L | L | O | G |
|
0 1 2 3 4 5 6
Front=0 rear=5
Algorithm to delete an element from the queue
Algorithm
Step 1: IF FRONT = -1 or FRONT > REAR
Write UNDERFLOW
ELSE
SET VAL = QUEUE[FRONT]
SET FRONT = FRONT + 1
[END OF IF]
Step 2: EXIT
| E | L | L | O | G |
|
0 1 2 3 4 5 6
Front=1 rear=5
Queue using link list
To implement queue using linked list,
1. To insert a new node into the queue...
enQueue(value) - Inserting an element into the Queue
2. To delete a node from the queue
deQueue() - Deleting an Element from Queue
3. To display the elements (nodes) of a queue...
display() - Displaying the elements of Queue
1. Enqueue
Queues maintain two data pointers, front and rear. Therefore, its operations are comparatively difficult to implement than that of stacks.
The following steps should be taken to enqueue (insert) data into a queue –
Algorithm for enqueue operation
procedure enqueue(data)
{ if queue is full
return overflow
end if
rear ← rear + 1
queue[rear] ← data
return true
} end procedure
2. Dequeue
Accessing data from the queue is a process of two tasks − access the data where front is pointing and remove the data after access. The following steps are taken to perform dequeue operation −
Algorithm for dequeue operataion
procedure dequeue
{ if queue is empty
return underflow
end if
data = queue[front]
front ← front + 1
return true
} end procedure
3. Peek
peek()This function helps to see the data at the front of the queue.
Algorithm
begin procedure peek
{
return queue[front]
} end procedure
Example:- int peek()
{
return queue[front];
}
4. Isfull
isfull() if we are using single dimension array for implementation of queue, to check for the rear pointer to reach at MAXSIZE to determine that the queue is full. To maintain the queue in a circular linked-list, the algorithm will differ.
Algorithm
begin procedure isfull
{ if rear equals to MAXSIZE
return true
else
return false
endif
} end procedure
Example :- bool isfull()
{
if(rear == MAXSIZE - 1)
return true;
else
return false;
}
5. Isempty
isempty() If the value of front is less than MIN or 0, it tells that the queue is not yet initialized, hence empty.
Algorithm
begin procedure isempty
if front is less than MIN OR front is greater than rear
return true
else
return false
endif
end procedure
Example:- bool isempty()
{
if(front < 0 || front > rear)
return true:
else
return false;
}
Figure: -Circular queue
Basic features of Circular Queue
1) In case of a circular queue, head pointer will always point to the front of the queue, and tail pointer will always point to the top of the queue.
2) Initially, the top and therefore the tail pointers are going to be pointing to an equivalent location, this is able to mean that the queue is empty.
3) New data is usually added to the situation pointed by the tail pointer, and once the info is added, tail pointer is incremented to point to subsequent available location.
4) In a circular queue, data isn't actually far away from the queue. Only the top pointer is incremented by one position when dequeue is executed. Because the queue data is merely the info between head and tail, hence the info left outside isn't a neighborhood of the queue anymore, hence removed.
5) The head and therefore the tail pointer will get reinitialized to 0 whenever they reach the top of the queue.
6) Also, the top and therefore the tail pointers can cross one another. In other words, head pointers are often greater than the tail. This may happen once we dequeue the queue a few of times and therefore the tail pointer gets reinitialized upon reaching the top of the queue.
Implementation of Circular Queue
Steps for implementing circular queue
1) Initialize the queue, with size of the queue defined (maxSize), and head and tail pointers.
2) Enqueue: Check if the amount of elements is adequate to max’Size - 1:
3) Dequeue: Check if the amount of elements within the queue is zero:
4) Finding the size:
Applications of Circular queue
Advantages of Circular queue
Disadvantages of Circular queue
Single line queue
Multiple line queue
Implementation of k queues
Method 1 (Divide the array in slots of size n/k)
Method 2 (A space efficient implementation)
Algorithms for basic primitive operations on queue
1. Insertion at rear
Function: QINSERT_REAR (Q, F, R, N,Y)
Given F and R tips that could the front and rear elements of a queue respectively. Queue Q consisting of N element. This procedure inserts Y at rear end of Queue.
IF R >= N
2. Then write (‘OVERFLOW’)
Return
[Increment REAR pointer]
R ←R + 1
3. [Insert element ]
Q[R] ←Y
4. [Is front pointer properly set
IF F=0
Then F ←1
Return
2. Deletion from front
Function: QDELETE_FRONT (Q, F, R)
•Given F and R tips that could the front and rear elements of a queue respectively. Queue Q consisting of N element. This function deleted and element from front of the Queue.
IF F= 0
Then write (‘UNDERFLOW’)
Return (0) (0 denotes an empty Queue)
2. [Decrement element]
Y ←Q[F]
3. [Queue empty?]
IF F=R
Then F←R←0
Else F←F+1 (increment front pointer)
4. [Return element]
Return (Y)
Algorithms for basic primitive operations in Circular Queue
1. Insertion in circular queue
Function: CQINSERT (F, R, Q, N, Y)
Given F and R tips that could the front and rear elements of a circular queue respectively. Circular queue Q consisting of N elements. This procedure inserts Y at buttocks of Circular queue.
If R = N
Then R← 1
Else R ← R + 1
2. [Overflow]
If F = R
Then Write (‘Overflow’)
Return
3. [Insert element]
Q[R] ← Y
4. [Is front pointer properly set?]
If F = 0
Then F ← 1
Return
2. Deletion in circular queue
Function CQDELETE (F, R, Q, N)
Given F and R tips that could the front and rear elements of a Circular queue respectively. Circular Queue Q consisting of N elements. This function deleted and element from front of the Circular Queue. Y is temporary pointer variable.
If F = 0
Then Write (‘UNDERFLOW’)
Return (0)
2. [Delete Element]
Y ← Q[F]
3. [Queue Empty?]
If F = R
Then F ← R ← 0
Return (Y)
4. [Increment front pointer]
If F = N
Then F ← 1
Else F ← F + 1
Return (Y)
Basic primitive operations for DeQueue
1. Insertion in dequeue
Function: -DQINSERT_FRONT (Q, F, R, N,Y)
Given F and R tips that could the front and rear elements of a queue, a queue consisting of N elements and a component Y, this procedure inserts Y at the front of the queue.
IF F = 0
Then write (‘EMPTY’)
Return
IF F=1
Then write (‘OVERFLOW’)
Return
2. [Decrement front pointer]
F ←F-1
3. [Insert element ]
Q[F] ←Y
Return
2. Deletion in dequeue
Function: DQDELETE_REAR (Q, F, R)
• Given F and R tips that could the front and rear elements of a queue. And a queue Q to which they correspond, this function deletes and returns the last element from the front of a queue. And Y is temporary variable.
IF R= 0
Then write (‘UNDERFLOW’)
Return (0)
2. [Delete element]
Y ←Q[R]
3. [Queue empty?]
IF R=F
Then R←F←0
Else R←R-1 (decrement front pointer)
4. [Return element]
Return (Y)
Display function in dequeue
Function: - DQUEUE_DISPLAY (F,R,Q)
•Given F and Rare tips that could the front and rear elements of a queue, a queue contains N element. This procedure display Queue contents
IF F >= R
Then write (‘QUEUE IS EMPTY’)
Return
2. [Display content]
FOR (I=FRONT; I=REAR;I++)
Write (Q[I])
3. [Return statement]
Return
- Input restricted dequeue- allows insertion at just one end
- Output restricted dequeue- allows deletion from just one end
Double Ended Queue
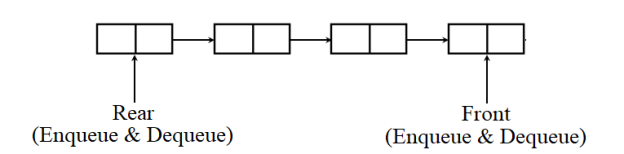
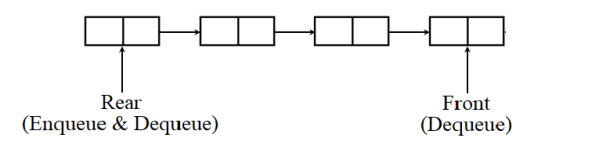
Implementation of Double ended Queue
Here we'll implement a double ended queue employing a circular array. It’ll have the subsequent methods:
1. Insert Elements at Front
When one element is added- 10
Now insert 12 at front
Now insert 14 at front
2. Insert Elements at back
Insert 21 at rear
3. Delete First Element
Delete from front
4. Delete Last Element
Figure:-Priority Queue viewed as a single queue with insertion allowed at any position
Figure: - Priority Queue viewed as a Viewed as a set of queue
Priority Queues
Operations
A max-priority queue also can provide these additional operations:
Implementations
1) Unordered array: A simple, yet inefficient implementation, as retrieving the max element would require searching the whole array.
2) Sorted array: This is not a really efficient implementation either. Inserting a replacement element requires linearly searching the array for the right position. Removing similarly requires a linear time: the remainder of the weather got to be adjusted (shifted) into correct positions.
3) Hash table: Although inserting into a hash table takes constant time (given an honest hash function), finding the max element takes linear time. Therefore, this is able to be a poor choice for the underlying arrangement.
4) Heap: It seems that that a heap makes an efficient priority queue.
The complexities of the common operations on a priority queue:
Operations | Unordered array | Sorted array | Hash table
| Binary heap |
Insert | Θ(1) | Θ(N) | Θ(1) | Θ(log(N)) |
Max element | Θ(N) | Θ(1) | Θ(N) | Θ(1) |
removeMaxElement | Θ(N) | Θ(1) | Θ(N) | Θ(log(N)) |
ASCENDING-->
Items are entered arbitrarily & only the smallest item may be removed. If apq is an ascending priority queue, the operation pqinsert (apq, x) inserts element x into apq and pqmindelete(apq) removes the minimum element from apq and returns its value.
DESCENDING-->
Items are entered arbitrarily & only the largest item may be removed. A descending priority queue is similar but allows deletion of only the largest item. The operations applicable to a descending priority queue, dpq, are pqinsert(dpq,x) and pqmaxdelete(dpq). pqinsert(dpq,x) inserts an element x into dpq and is logically identical to pqinsert for an ascending priority queue. pqmaxdelete(dpq) removes the maximum element from dpq and returns its value.
Using Heaps-
Heap is generally preferred for priority queue implementation because heaps provide better performance compared arrays or linked list. In a Binary Heap, getHighestPriority() can be implemented in O(1) time, insert() can be implemented in O(Logn) time and deleteHighestPriority() can also be implemented in O(Logn) time.
With Fibonacci heap, insert() and getHighestPriority() can be implemented in O(1) amortized time and deleteHighestPriority() can be implemented in O(Logn) amortized time.
Applications of Priority Queue:
1) CPU Scheduling
2) Graph algorithms like Dijkstra’s shortest path algorithm, Prim’s Minimum Spanning Tree, etc
3) All queue applications where priority is involved