Unit - 4
JSP and Web Services
● Java Server Pages (JSP) technology provides a simplified, fast way to create web pages that display dynamically-generated content. It is a technology used to create web applications.
● The JSP specification, developed through an industry-wide initiative led by Sun Microsystems in June, 1999, defines the interaction between the server and the JSP page, and describes the format and syntax of the page.
● JSP pages share the "Write Once, Run Anywhere" characteristics of Java technology. Java Server Pages are HTML pages embedded with snippets of Java code.
● It is an inverse of a Java Servlet. It provides additional features such as expression language, JSTL (JSP Standard Tag Library), custom tags etc.
● A JSP page is a page created by the web developer that includes JSP technology-specific and custom tags, in combination with other static (HTML or XML) tags.
● The JSP pages are easier to maintain than servlet because we can separate designing and development part.
● A JSP page has the extension .jsp or .jspx. This signals to the web server that the JSP engine will process elements on this page. Using the web.xml deployment descriptor, additional extensions can be associated with the JSP engine.
● Java Server Pages often provides the same purpose as that of Common Gateway Interface (CGI). But JSP offers several advantages in comparison with the CGL.
● JSP allows embedding Dynamic Elements in HTML Pages itself instead of having separate CGI files which increases the performance.
● They are always compiled before they are processed by the server.
● Java Server Pages are built on top of the Java Servlets API, so JSP has access to all the powerful Enterprise Java APIs, including JDBC, EJB etc.
● Java Server Pages (JSP) is a technology which is used to develop web pages by inserting JAVA code into the HTML pages by making special JSP tags.
● The JSP tags which allow java code to be included into it are <% —-java code-—%>. Java Server Pages (JSP) separates the dynamic part of your pages from the static HTML.
Example
HTML tags and text
<% Place JSP code here %>
HTML tags and text
i.e.
<U>
<%= request.getParameter("Welcome”) ⅝>
<∕U>
Key takeaway
Java Server Pages (JSP) technology provides a simplified, fast way to create web pages that display dynamically-generated content. It is a technology used to create web applications.
A servlet is a Java class that extends the capabilities of servers that host applications that are accessed using the request-response model. Servlets are mostly used to expand web server-hosted applications, but they can also respond to other types of requests. Java Servlet technology defines HTTP-specific servlet classes for such applications.
A JavaServer Pages (JSP) is a text document that contains two types of text: static data and dynamic data. Static data can be expressed in any text-based format (such as HTML, XML, SVG, and WML), whereas dynamic content is expressed using JSP elements.
Difference between jsp and servlet
JSP | Servlets |
JSP is an HTML-based programming language. | A java code is known as a servlet. |
Because JSP is java in HTML, it is simple to code. | Writing servlet code is more difficult than writing JSP code since servlet is html in Java. |
In the MVC architecture, the view for displaying output is JSP. | In the MVC paradigm, the servlet serves as a controller. |
Because the first step in the JSP lifecycle is to translate JSP to Java code and then compile, JSP is slower than Servlet. | Servlet is faster than JSP. |
Only http requests are accepted by JSP. | All protocol requests are accepted by Servlet. |
We can't override the service() method in JSP. | We can override the service() method in Servlet. |
Session management is enabled by default in JSP. | Session management is not enabled by default in Servlet; users must specifically enable it. |
JavaBeans are used to segregate business logic from presentation logic in JSP. | Everything, including business logic and display logic, must be implemented in a single servlet file in Servlet. |
JSP alteration is simple; all you have to do is click the refresh button. | Because Servlet modification necessitates reloading, recompiling, and restarting the server, it is a time-consuming process. |
Key takeaway
A servlet is a Java class that extends the capabilities of servers that host applications that are accessed using the request-response model. Servlets are mostly used to expand web server-hosted applications, but they can also respond to other types of requests. Java Servlet technology defines HTTP-specific servlet classes for such applications.
To create the first sample jsp page, write some htnιl code as given below, and save it by .jsp extension. We have save this file as hello.jsp. Put it in a folder and paste the folder in the web- apps directory in apache tomcat to run the jsp page.
Hello.jsp
<html>
<body>
<% out.print(4+5); %>
<∕body>
<∕html>
It will print 9 on the browser.
How to run a simple JSP Page?
Follow the following steps to execute this JSP page:
● Start the server
● Put the JSP file in a folder and deploy on the server
● Visit the browser by the URL http://localhost:portno/contextRoot/jspfile, for example, http://localhost:8888/myapplication/index.jsp
JSP is a programming language that is used to construct dynamic web applications. Servlets are more difficult to maintain than JSP pages. JSP pages are the polar opposite of Servlets in that Servlets embed HTML code within Java code, whereas JSP embeds Java code within HTML using JSP tags. A JSP page can accomplish anything that a Servlet can.
JSP allows us to create HTML pages with tags that we can use to include powerful Java programmes. A web designer can design and update JSP pages to provide the presentation layer, while a java developer can write server side complicated computational code without worrying about the web design. Furthermore, both layers can easily communicate via HTTP queries.
Advantages
● It's simple to code and maintain.
● Scalability and high performance.
● Because JSP is based on Java technology, it is platform agnostic.
Disadvantages
● Errors are difficult to track down.
● Time is wasted when access is granted for the first time.
● Its output is HTML, which is devoid of functionality.
Key takeaway
JSP is a programming language that is used to construct dynamic web applications. Servlets are more difficult to maintain than JSP pages. JSP pages are the polar opposite of Servlets in that Servlets embed HTML code within Java code, whereas JSP embeds Java code within HTML using JSP tags. A JSP page can accomplish anything that a Servlet can.
A JavaBean is a custom Java class developed in Java and coded to the JavaBeans API requirements.
The following are the properties that set a JavaBean apart from other Java classes:
● It has a no-argument default function Object() { [native code] }.
● Serializable and capable of implementing the Serializable interface are required.
● It may have a variety of read-only or write-only characteristics.
● The properties may have a number of "getter" and "setter" methods.
JavaBeans property
A JavaBean property is a named attribute that the object's user can access. Any Java data type, including the classes you define, can be used for the attribute.
A property on a JavaBean can be read, write, read only, or write only. Two methods in the JavaBean's implementation class are used to access JavaBean properties.
● getPropertyName() - To read a property named firstName, for example, your method would be called getFirstName(). Accessor is the name of this technique.
● setPropertyName() - For example, if the property name is firstName, your method name to write that value would be setFirstName(). Mutator is the name of this method.
A read-only property will have only one method, getPropertyName(), and a write-only attribute will have only one method, setPropertyName().
Why use JavaBean?
It is a reusable programme component, according to the Java white paper. A bean wraps numerous things into a single object that may be accessed from multiple locations. Furthermore, it is simple to maintain.
Simple example of JavaBean class
//Employee.java
Package mypack;
Public class Employee implements java.io.Serializable{
Private int id;
Private String name;
Public Employee(){}
Public void setId(int id){this.id=id;}
Public int getId(){return id;}
Public void setName(String name){this.name=name;}
Public String getName(){return name;}
}
How to access the JavaBean class?
We should utilize getter and setter methods to access the JavaBean class.
Package mypack;
Public class Test{
Public static void main(String args[]){
Employee e=new Employee();//object is created
e.setName("Arjun");//setting value to the object
System.out.println(e.getName());
}}
Advantages of JavaBean
The following are some of JavaBean's benefits:/p>
● Another programme can access the JavaBean attributes and methods.
● It makes reusing software components more easier.
Disadvantages of JavaBean
The following are some of JavaBean's drawbacks:
● JavaBeans can be changed. As a result, it is unable to benefit from immutable objects.
● The boilerplate code may result from creating the setter and getter methods for each property independently.
Key takeaway
A JavaBean is a custom Java class developed in Java and coded to the JavaBeans API requirements.
The model, view, and controller (MVC) paradigm splits a web application's logic into three discrete regions, or concerns. Each concern, in practise, has numerous components and files. Model classes, view templates, and controller classes are all present in a typical MVC application.
Here's how the various concerns operate:
Model - Logic is applied to data. Essentially, the model is responsible for the application's business logic as well as any other logic that isn't present in the display or controller. Data is represented and manipulated by model objects. The model in the CMS environment is based on XML data.
View - Data from the model is displayed. The view layer creates the application's user interface. A view template generates an HTML page to be served to a client using markup and JavaScript.
Controller - Handles the application's input logic. In response to user input, the controller transmits queries from the user to the model, and controller methods call views.
MVC is popular because it separates the application logic and user interface layer, allowing for separation of concerns. The Controller receives all application requests and then works with the Model to prepare any data that the View requires. The View then generates a final presentable response using the data prepared by the Controller. The MVC abstraction can be represented graphically as follows.
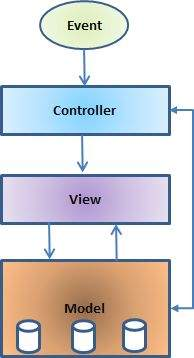
Fig 1: MVC
Features of MVC
● Testability is simple and painless. Framework that is highly tested, extensible, and pluggable.
● The MVC pattern gives you complete control over your HTML and URLs when creating a web application architecture.
● Utilize existing features like as ASP.NET, JSP, Django, and others.
● Model, View, and Controller are clearly separated in terms of logic. Application activities, including as business logic, Ul logic, and input logic, are separated.
● Routing URLs for SEO-Friendly URLs URL mapping that is both comprehensible and searchable.
● Test-Driven Development (TDD) is aided by the following resources (TDD).
Advantages
The following are some of the primary advantages of employing MVC architecture:
● Easy-to-maintain code that can easily be extended and expanded.
● Separately from the user, the MVC Model component can be tested.
● Support for new sorts of clients is made easier.
● Parallel development of the various components is possible.
● By splitting an application into three components, it aids in the avoidance of complexity. There's a model, a view, and a controller.
● It solely employs the Front Controller design, which uses a single controller to execute web application requests.
● Provides the best test-driven development support.
● It works effectively for online apps that have huge teams of web designers and engineers supporting them.
● Allows for a clear separation of concerns (SoC).
● Friendly to Search Engine Optimization (SEO).
● All classes and objects are self-contained, allowing you to test them independently.
● The MVC design pattern allows for the logical grouping of related controller actions.
Disadvantages
● This model is difficult to read, alter, unit test, and reuse.
● The navigation of the framework can be complicated at times since it introduces additional layers of abstraction, requiring users to adjust to the MVC decomposition requirements.
● There is no official validation support.
● Data has become more complex and inefficient.
● The challenge of using MVC with today's user interface.
● Parallel programming necessitates the use of numerous programmers.
● It is necessary to have a broad understanding of a variety of technologies.
● Keeping track of a large number of codes in the controller.
Key takeaway
The model, view, and controller (MVC) paradigm splits a web application's logic into three discrete regions, or concerns. Each concern, in practise, has numerous components and files. Model classes, view templates, and controller classes are all present in a typical MVC application.
JSP stands for JavaServer Pages, and it's a technique for creating dynamic and data-driven pages for your Java online applications. The Java Servlet specification governs JSP. Servlet and JSP technologies are used jointly in older Java projects. When writing servlets, you create Java code and include client-side markup (such as HTML) in it. You write the client-side script or markup and include JSP tags to connect your page to the Java backend while writing JSP.
HTML, XML, SOAP, and other document types are used to create JavaServer Pages.
In a word, JSP technology is a Servlet-based extension. We can use all of the Servlet's functionalities there. In addition, implicit objects, predefined tags, expression language, and Custom tags are all available in JSP, making JSP programming simple.
JSP, like Servlets, is hosted on a web server. Apache Tomcat, Jetty, Apache TomEE, Oracle WebLogic, WebSphere, Apache Geronimo, and others are examples of web servers. JSP is a Java servlet abstraction. JSP is a Servlet since it is transformed into Servlets at runtime.
The JavaServer Pages can be utilised on their own. It can also be used as the view component in a model–view–controller paradigm. It's typically used as a controller with the JavaBeans paradigm and Java servlets (or a framework like Apache Struts).
The JavaServer Pages technology allows you to create online content that includes both static and dynamic elements. Although JSP technology offers dynamic capabilities, it is a more natural way to create static information.
The following are the primary characteristics of JSP technology:
● JSP pages are text-based documents that outline how to process and generate a response to a request.
● You can use an expression language in JSP to access server-side objects.
● The JSP language has facilities for creating extensions.
The following are some of the benefits of JavaServer Pages over Servlets:
In JSP, we can use all of the Servlet's functionalities. In addition, implicit objects, predefined tags, expression language, and Custom tags are all available in JSP, making JSP programming simple.
JSP is less difficult to maintain. It's simple to manage because the business logic and presentation layers are readily separated. We combine our business and presentation logic with Servlet technology.
JSP development is faster than Servlet development because you don't have to recompile and redeploy them. We don't need to recompile and redeploy the project if the JSP page is changed.
Servlet technology has more code than JavaServer Pages technology. Many tags, such as action tags, JSTL, custom tags, and so on, can be used there. All of these things help to cut down on code. We can also employ expression language, implicit objects, and a variety of other features.
Because JavaServer Pages are based on the Java Servlets API, they have access to all of Enterprise Java's advanced technologies, such as JDBC, JNDI, EJB, JAXP, and so on.
JSP is part of the Java EE platform, which is a full platform for enterprise-class applications. This means that JSP may be used in the simplest as well as the most complicated and demanding applications.
Key takeaway
JSP stands for JavaServer Pages, and it's a technique for creating dynamic and data-driven pages for your Java online applications. The Java Servlet specification governs JSP. Servlet and JSP technologies are used jointly in older Java projects.
The Internet is the worldwide interconnection of hundreds of thousands of different types of computers that are connected to many networks. A web service is a defined technique for propagating messages between client and server applications on the World Wide Web. A web service is a software module that is designed to perform a set of specialised tasks. In cloud computing, web services can be found and invoked over the internet.
The client that invoked the web service would be able to receive functionality from the web service.
A web service is a collection of open protocols and standards that allow data to flow across different applications or systems. Web services can be used by software programmes written in a number of programming languages and running on a range of platforms to exchange data via computer networks like the Internet, much like inter-process communication on a single machine.
A web service is any software, application, or cloud technology that connects, interoperates, and exchanges data messages – often XML (Extensible Markup Language) – across the internet using standardised web protocols (HTTP or HTTPS).
Web services have the benefit of allowing programmes written in various languages to communicate with one another by sending data between clients and servers via a web service. A client makes an XML request to a web service, and the service responds with an XML response.
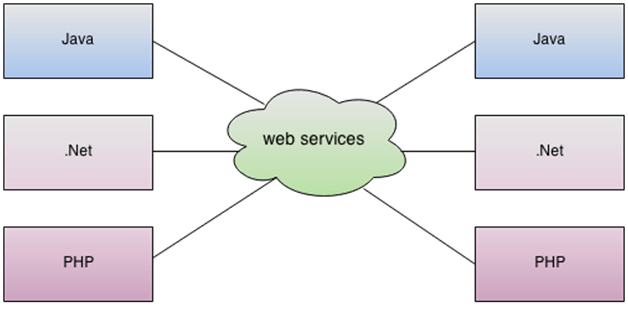
Fig 2: Web service
As seen in the diagram, Java,.net, and PHP programmes can connect with each other across the network via web services. The Java application, for example, can communicate with Java,.Net, and PHP applications. As a result, a web service is a language-independent communication method.
Features
● It can be accessed through the internet or intranet networks.
● A standardised XML communicating protocol.
● Independent of the operating system or programming language.
● It is self-descriptive when using the XML standard.
● It can be found using a simple locating method.
Components
XML + HTTP is the most fundamental web services platform. The following components are used by all typical web services:
● SOAP (Simple Object Access Protocol)
● UDDI (Universal Description, Discovery and Integration)
● WSDL (Web Services Description Language)
Working of web service
A web service uses open standards like HTML, XML, WSDL, and SOAP to allow communication between different applications. A web service relies on the assistance of
● XML to tag the data
● SOAP to transfer a message
● WSDL to describe the availability of service.
On Solaris, you can create a Java-based web service that can be accessed from your Windows Visual Basic programme.
You may also use C# to create new web services on Windows that can be called from your JavaServer Pages (JSP)-based web application running on Linux.
Key takeaway
A web service is a collection of open protocols and standards that allow data to flow across different applications or systems. Web services can be used by software programmes written in a number of programming languages and running on a range of platforms to exchange data via computer networks like the Internet, much like inter-process communication on a single machine.
This section demonstrates how to create and deploy a simple web service as well as two client applications: an application client and a web client. The service's source code can be found in tut-install/examples/jaxws/helloservice-war/, and the clients can be found in tut-install/examples/jaxws/hello-appclient/ and tut-install/examples/jaxws/hello-webclient/.
The diagram shows how JAX-WS handles communication between a web service and a client.

A Java class annotated with the javax.jws.WebService annotation is the starting point for constructing a JAX-WS web service. The @WebService annotation designates a web service endpoint for the class.
A service endpoint interface (SEI) or service endpoint implementation (SEI) is a Java interface or class that specifies the methods that a client can use to interact with the service. When creating a JAX-WS endpoint, no interface is necessary. The SEI is defined implicitly by the web service implementation class.
By adding the endpointInterface element to the @WebService annotation in the implementation class, you can define an explicit interface. The public methods made available in the endpoint implementation class must then be defined via an interface.
Basic Steps for Creating a Web Service and Client
The following are the fundamental steps for building a web service and client.
● Create a class for the implementation.
● Complete the implementation class by compiling it.
● Make a WAR file out of the files.
● Install the WAR file. GlassFish Server generates the web service artefacts that are needed to communicate with clients during deployment.
● The client class should be written.
● To connect to the service, use the wsimport Maven objective to produce and compile the web service artefacts.
● Create a client class and compile it.
● Run the client.
When you construct a service and client with NetBeans IDE, the IDE handles the wsimport operation for you.
Requirements of a JAX-WS Endpoint
Either the javax.jws.WebService or the javax.jws.WebServiceProvider annotation must be present in the implementing class.
● The implementing class may, but is not necessary to, use the endpointInterface element of the @WebService annotation to explicitly reference an SEI. An SEI is defined for the implementing class if no endpointInterface is given in @WebService.
● The implementing class's business methods must be public and not declared static or final.
● Web service clients must be able to access business methods that are annotated with javax.jws.WebMethod.
● JAXB-compatible arguments and return types are required for business methods exposed to web service clients. Types Supported by JAX-WS contains a list of JAXB default data type bindings.
● The implementing class should not be marked final or abstract.
● A default public function Object() { [native code] } must be present in the implementing class.
● The implementing class must not define the finalize method.
● The implementing class may use the javax.annotation.PostConstruct or the javax.annotation.PreDestroy annotations on its methods for lifecycle event callbacks.
The container calls the @PostConstruct method before the implementing class starts responding to web service clients.
Before the endpoint is removed from service, the container calls the @PreDestroy function.
Web Services Description Language (WSDL) is an acronym for Web Services Description Language. It's the industry standard for characterising web services. Microsoft and IBM collaborated to create WSDL.
The Web Services Description Language (WSDL) is an XML-based file that explains what the web service does to the client application. The WSDL file is used to describe the web service in a nutshell and provides the client with all of the information needed to connect to the web service and use all of its capability.
The WSDL file is used to describe the web service in a nutshell and provides the client with all of the information needed to connect to the web service and use all of its capability.
History
In March 2001, Ariba, IBM, and Microsoft submitted WSDL 1.1 as a W3C Note for describing services for the W3C XML Activity on XML Protocols.
The World Wide Web Consortium (W3C) has not endorsed WSDL 1.1, but it has just released a draught for version 2.0, which will be a recommendation (an official standard) and thus endorsed by the W3C.
Features
The Web Services Description Language (WSDL) is an XML-based framework for exchanging data in decentralised and distributed contexts.
The WSDL defines how to use a web service and what actions it will do.
The Web Services Description Language (WSDL) is a language for specifying how to interact with XML-based services.
Universal Description, Discovery, and Integration (UDDI), an XML-based global business registry, includes WSDL.
UDDI is written in the WSDL language.
WSDL is spelt W-S-D-L and is pronounced 'wiz-dull.'
Usage
To provide web services via the Internet, WSDL is frequently used with SOAP and XML Schema. The WSDL can be read by a client software connecting to a web service to determine what functions are available on the server. XML Schema is used to encapsulate any particular datatypes used in the WSDL file. After that, the client can use SOAP to call one of the functions provided in the WSDL.
Key takeaway
Web Services Description Language (WSDL) is an acronym for Web Services Description Language. It's the industry standard for characterising web services. Microsoft and IBM collaborated to create WSDL.
The acronym SOAP refers to the Simple Object Access Protocol. It's a web service access protocol based on XML.
SOAP (Simple Object Access Protocol) is a W3C recommendation for communication between two applications.
SOAP is a protocol that is based on XML. It works on any platform and in any language. You will be able to interface with other programming language applications by using SOAP.
In today's world, there are a plethora of applications created using several programming languages. There could be a web application written in Java, another in.Net, and yet another in PHP, for example.
In today's networked world, data exchange between applications is critical. However, data transmission between these disparate applications would be difficult. The complexity of the programming required to carry out this data interchange will also be high.
The use of XML (Extensible Markup Language) as an intermediate language for sharing data between programmes is one of the approaches tried to fight this complexity.
The XML markup language can be understood by any programming language. As a result, XML was chosen as the data interchange medium.
However, there are no common specifications for using XML for data sharing across all computer languages. SOAP software can help with this.
SOAP was created to deal with XML over HTTP and to establish a standard that could be applied to any applications.
Uses
What is the purpose of a standard like SOAP? Two applications can transmit rich, structured information by exchanging XML documents over HTTP without the need for an extra standard, such as SOAP, to explicitly provide a message envelope format and a mechanism to encode structured content.
SOAP provides a standard that eliminates the need for developers to create their own XML message format for each service they want to make available. The SOAP specification specifies an unambiguous XML message format based on the signature of the service method to be invoked. By getting the following service data, any developer familiar with the SOAP specification, working in any programming language, can make a correct SOAP XML request for a specific service and comprehend the answer from the service.
● Service name
● Method names implemented by the service
● Method signature of each method
● Address of the service implementation (expressed as a URI)
Because the method signature of the service indicates the XML document structure used for both the request and the response, using SOAP simplifies the process of exposing an existing software component as a Web service.
Advantages
● SOAP defines its own security, referred to as WS Security.
● SOAP web services are language and platform agnostic. They can be built in any programming language and run on any platform.
Disadvantages
● SOAP is slow because it employs an XML format that must be digested before it can be read. It establishes a number of guidelines that must be followed while designing SOAP applications. As a result, it is slow and uses a lot of bandwidth and resources.
● SOAP is WSDL-reliant, as it lacks any other mechanism for discovering services.
Key takeaway
SOAP is a protocol that is based on XML. It works on any platform and in any language. You will be able to interface with other programming language applications by using SOAP.
● The struts framework is an open source framework for creating well-structured web based applications.
● The struts framework was initially created by Craig McClanahan and donated to Apache Foundation in May, 2000 and Struts 1.0 was released in June 2001.
● The current stable release of Struts is Struts 2.3.16.1 in March 2, 2014.
● The Struts 2 framework is used to develop MVC (Model View Controller) based web applications. Struts 2 is the combination of webwork framework and struts
● The struts framework is based on the Model View Controller (MVC) standard which distinctly separates all the three layers - Model which represents the state of the application, View represents presentation and Controller shows controlling the application flow.
● The struts framework is a complete web framework as it provides complete web form components, validators, error handling, internationalization, tiles and more.
● Struts framework provides its own Controller component. It integrates with other technologies for both Model and View components.
● Struts can integrate well with Java Server Pages (JSP), Java Server Faces (JSF), JSTL, Velocity templates and many other presentation technologies for View. For Model, Struts works great with data access technologies like JDBC, Hibernate, EJB and many more.
Why struts! so popular
● A strut supports extensive validations where other frame works doesn’t
● Having inbuilt support for Il 8N
● Struts 2 actions classes are spring friendly so we can easily integrate
● In build AJAX themes to make the applications more dynamic
● It is good frame work for front end based applications.
How Struts! Works?
When the framework’s controller receives a request, it uses the configuration file, Struts- config.xml to find out the correct routing information. Based on the information configured in the configuration file, it internally invokes an Action class. The Action class interacts with the Model (also called the business layer) to access or update the underlying data in database/ file. The framework includes ActionForm classes to transfer data between Model and View.
Struts2 framework features
The main struts features are:
● Configurable MVC Components: In struts 2 framework, we provide all the components (view components and action) information in struts.xml file. If we need to change any information, we can simply change it in the xml file.
● POJO Based Actions: In struts 2, action class is POJO (Plain Old Java Object) i.e. a simple java class. Here, you are not forced to implement any interface or inherit any class.
● AJAX Support: Struts 2 provides support to ajax technology. It is used to make asynchronous request i.e. it doesn’t block the user. It sends only required field data to the server side not all. So it makes the performance fast.
● Integration Support: We can simply integrate the struts 2 application with hibernate, spring, tiles etc. frameworks.
● Various Result Types: We can use JSP, freemarker, velocity etc. technologies as the result in struts 2.
● Various Tag Support: Struts 2 provides various types of tags such as UI tags, Data tags, control tags etc to ease the development of struts 2 application.
● Theme and Template support: Struts 2 provides three types of theme support: xhtml, simple and css_xhtml. The xhtml is default theme of struts 2. Themes and templates can be used for common look and feel.
Struts 2 Disadvantages
● Bigger learning curve: To use MVC with Struts, you have to be comfortable with the standard JSP, Servlet APIs and a large & elaborate framework.
● Poor documentation: Compared to the standard servlet and JSP APIs, Struts has fewer online resources, and many first-time users find the online Apache documentation confusing and poorly organized.
● Less transparent: With Struts applications, there is a lot more going on behind the scenes than with normal Java-based Web applications which makes it difficult to understand the framework.
● In Struts architecture, there is only one controller servlet for the entire web application. This controller servlet is called ActionServlet and resides in the package org.apache.struts.action.
● It intercepts every client request and populates an ActionForm from the HTTP request parameters.
● ActionForm is a normal JavaBeans class. It has several attributes corresponding to the HTTP request parameters and getter, setter methods for those attributes. You have to create your own ActionForm for every HTTP request handled through the Struts framework by extending the org.apache.struts.action.ActionForm class as shown in the below architecture.
● Consider the following HTTP request for FirstApp web application:
Http://localhost:8080/FirstApp/create.do?firstName=AnandfelastName=Mustary
● The ActionForm class for this HTTP request is shown in the below example. The class MyForm extends the org.apache.struts.action.
● ActionForm class and contains two attributes: fιrstName and IastName. It also has getter and setter methods for these attributes. Use classes such as ActionForm for View Data Transfer Object. View Data Transfer Object is an object that holds the data from html page and transfers it around in the web tier framework and application classes.
∕∕ ActionForm
Public class MyForm extends ActionForm {
Private String firStName;
Private String IastName;
Public MyForm() {
fιrstName = IastName =
}
Public String getFirstName() {
Return firstName;
}
Public void setFirstName(String s) {
This, fir StName = s;
}
Public String getLastName() {
Return IastName;
}
Public void setLastName(String s) {
This.IastName = s;
}
}
● The Action Servlet then instantiates a Handler. The Handler class name is obtained from an XML file based on the URL path information. This XML file is referred to as Struts configuration file and by default named as struts-config.xml.
● The Handler is called Action in the Struts terminology. And you guessed it right! This class is created by extending the Action class in org.apache.struts.action package.
● The Action class is abstract and defines a single method called execute(). You override this method in your own Actions and invoke the business logic in this method. The execute() method returns the name of next view (JSP) to be shown to the user. The Action Servlet forwards to the selected view.
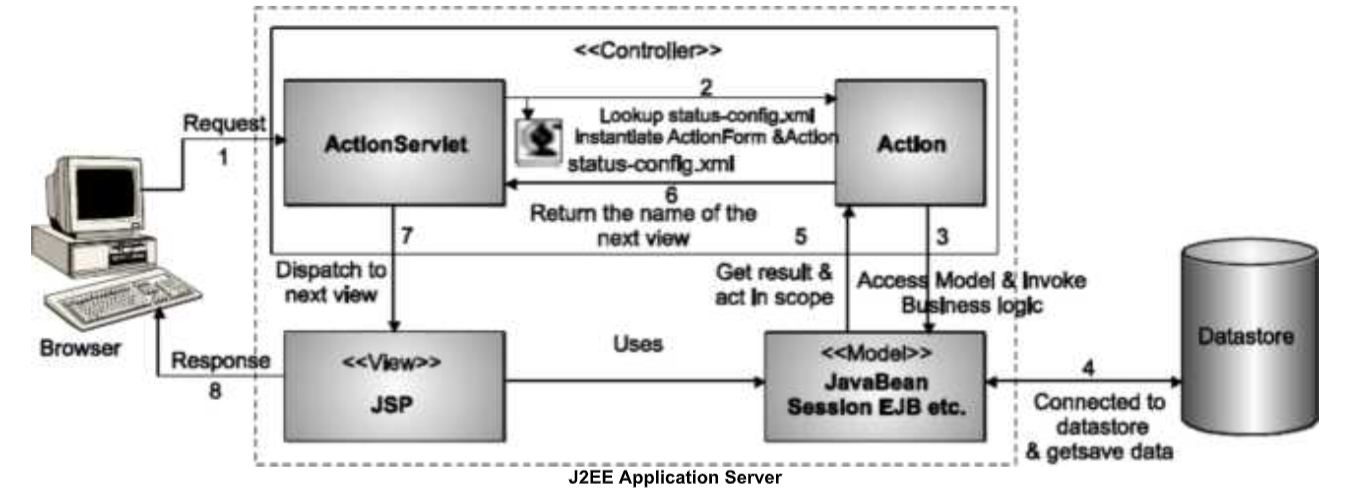
Fig 3: Struts architecture
The struts required few important configuration files: web.xml, struts.xml, struts- config.xml and struts.properties.
1. The web.xml file
● The web.xml configuration file is a J2EE configuration file that determines how elements of the HTTP request are processed by the servlet container. It is not strictly a Struts2 configuration file, but it is a file that needs to be configured for Struts2 to work.
● As discussed earlier, this file provides an entry point for any web application. The entry point of Struts2 application will be a filter defined in deployment descriptor (web.xml). Hence we will define an entry OfFilterDispatcher class in web.xml. The web.xml file needs to be created under the folder WebContent/WEB-INF.
● This is the first configuration file you will need to configure if you are starting without the aid of a template or tool that generates it. Following is the content of the web.xml file.
<7xml version=” 1.0” encoding-TJTF-8 n 7>
<display-name>Struts 2<∕display-name>
<welcome-file-list>
<welcome-file>index.jsp<∕welcome-file>
<∕welcome-file-list>
<filter>
<filter-name>struts2<∕filter-name>
<filter-class>
Org.apache.struts2.dispatcher.FilterDispatcher
<∕filter-class>
<∕filter>
<filter-mapping>
<filter-name>struts2<∕filter-name>
<url-pattern>∕*<∕url-pattern>
<∕filter-mapping>
<∕web-app>
2. The struts.xml file
● The struts.xml file contains the configuration information that you will be modifying as actions are developed. This file can be used to override default settings for an application, for example struts.devMode = false and other settings which are defined in the property file. This file can be created under the folder WEB-INFZclasses.
<7xml version=”!.0” encoding=”UTF-8”?>
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
”http:ZZstruts.apache.orgZdtdsZstruts-2.0.dtd”>
<struts>
<constant name-’struts.devMode” value-’true” Z>
<package name= ,, helloworld” extends=”struts-default”>
<action name=”hello”
Class-<struts2.HelloWorldAction”
Method=”execute”>
<result name=”success”>/HelloWorld.jsp</result>
<∕action>
<- more actions can be listed here ->
<∕package>
<— more packages can be listed here ->
<∕struts>
● All struts configuration file need to have the correct doctype as shown in the above example, <struts> is the root tag element, under which we declare different packages using <package>tags. Here <package> allows separation and modularization of the configuration. This is very useful when you have a large project and project is divided into different modules.
● Package Element: We can easily divide our struts application into sub modules. The package element specifies a module. You can have one or more packages in the struts.xml file, action element: The action is the sub element of package and represents an action. Result element: It is the sub element of action that specifies where to forward the request for this action.
3. The struts-config.xml file
The struts-config.xml configuration file is a link between the View and Model components in the Web Client.
Following is the sample struts-config.xml file:
<7xml version=”!.0” encoding-’ISO-8859-1” ?>
<! DOCTYPE StrutS-Config PUBLIC ’’-//Apache Software FoundationADTD Struts Configuration 1.0//EN” ’’ http://jakarta.apache.org/struts/dtds/struts-config_l_0.dtd” >
<struts-config>
<!— ========== Form Bean Definitions ============ —>
<form-beans>
<form-beanname-’login” type=”test.struts.LoginForm” ∕>
<∕form-beans>
<!-- ========== Global Forward Definitions ========= ->
<global-forwards>
<∕global-forwards>
<!— ========== Action Mapping Definitions ======== —>
<action-mappings>
<action
Path-’/login”
Type=”test.struts.LoginAction” >
<forwardname=”valid” path-’/jsp/MainMenu.jsp” ∕>
<forward name=”invalid” path=”/jsp/LoginView.jsp” ∕>
<∕action>
<∕action-mappings>
<∣ Conliollei Definitions
<controller
ContentType=YextZhtmhcharset=UTF-S ”
Debug=”3”
MaxFileSize=”1.618M”
Iocale=True” nocache=True”Z>
<Zstruts-config>
In the above example we used following commands:
● struts-config: This is the root node of the configuration file.
● form-beans: This is where you map your ActionForm subclass to a name. You use this name as an alias for your ActionForm throughout the rest of the struts-confιg.xml file, and even on your JSP pages.
● global forwards: This section maps a page on your webapp to a name. You can use this name to refer to the actual page. This avoids hardcoding URLs on your web pages.
● action-mappings: This is where you declare form handlers and they are also known as action mappings.
● Controller: This section configures Struts internals and rarely used in practical situations.
● plug-in: This section tells Struts where to find your properties files, which contain prompts and error messages
4. The struts.properties file
This configuration file provides a mechanism to change the default behavior of the framework. Actually all of the properties contained within the struts.properties configuration file can also be configured in the web.xml using the init-param, as well using the constant tag in the struts.xmlconfιguration file. But if you like to keep the things separate and more struts specific then you can create this file under the folder WEB-INFZclasses.
The values configured in this file will override the default values configured in default.properties which is contained in the struts2-core-a.b.c.jar distribution. There are a couple of properties that you might consider changing using struts.properties file:
Example
# ## When set to true, Struts will act much more friendly for developers struts.devMode = true
# ## Enables reloading of internationalization files
Struts.! 18n.reload = true
# ## Enables reloading of XML configuration files
Struts.confιguration.xml.reload = true
# ## Sets the port that the server is run on struts.url. Http.port = 8080
The # is used as a comment in struts framework.
● In struts2, the action class is POJO (Plain Old Java Object). The POJO means you are not forced to implement any interface or extend any class.
● Actions do three things. First, an action’s most important role, from the perspective of the framework’s architecture, is encapsulating the actual work to be done for a given request.
● The second major role is to serve as a data carrier in the framework’s automatic transfer of data from the request to the view.
● Finally, the action must assist the framework in determining which result should render the view that’ll be returned in the request response.
1. Actions Encapsulate the Actual Work
One of the central responsibilities of this role is the containment Ofbusiness logic; actions use the execute() method for this purpose. The code inside this method should concern itself only with the logic of the work associated with the request. Generally, the execution method should be specified that represents the business logic. The simple action class may look like: Sample Java
Package Commiralipublication;
Public class Sample {
Public String execute(){
Return ’’success”;
} }
2. Actions Provide Locus for Data Transfer
Being the model component of the framework also means that the action is expected to carry the data around. Since the data is held local to the action, it’s always conveniently available during the execution of the business logic. There might be a bunch of JavaBeans properties adding lines of code to the action, but when the execute() method references the data in those properties, the proximity of
The data makes that code all the more succinct.
Following example shows the code that allows Transferring request data request data to the action’s JavaBeans properties,
Example
Private String name;
Public String getName() { return name;
}
Public void setName(String name) { this.name = name;
}
Private String CustomGreeting;
Public String getCustomGreeting()
{
Return CustomGreeting;
}
Public void setCustomGreeting( String CustomGreeting ){
This. CustomGreeting = CustomGreeting;
}
The action merely implements JavaBeans properties for each piece of data that it wishes to carry. We saw this in action with the Sample application. Request parameters from the form are moved to properties that have matching names. As we saw, the framework does this automatically. In this case, the name parameter from the name collection form will be set on the name property. In addition to receiving the incoming data from the request, these JavaBeans properties on the action will also
Expose the data to the result.
3. Actions Return Control String for Result
The final duty of an action component is to return a control string that selects the result that should be rendered. The value of the return string must match the name of the desired result as configured in the declarative architecture. For instance, the SampleAction returns the string ’’SUCCESS”. As you can see from our XML declaration, SUCCESS is the name of one of the result components.
Example:
<action name=”Sample” class=”Name.First.Sample n >
<result name=”SUCCESS”>First/Sample.jsp</result>
<result name-ΕRROR π >∕Second∕Error.jsp<∕result>
<∕action>
The Sample application has a simple logic for determining which result it will choose. In fact, it’ll always choose the ’’SUCCESS” result. Most real-world actions will have a more complex determination process, and the result choices will almost always include some sort of error result to handle problems that might occur during the action ς s interaction with the model.
Create Action
The only requirement for actions in Struts2 is that there must be one no-argument method that returns either a String or Result object and must be a POJO. If the no-argument method is not specified, the default behavior is to use the execute() method. Optionally you can extend the ActionSupport class which implements six interfaces including Action interface. The Action interface is as follows:
Public interface Action {
Public static final String SUCCESS = ’’success”;
Public static final String NONE = ’’none”;
Public static final String ERROR = ’’error”;
Public static final String INPUT = ’’input”;
Public static final String LOGIN = ’’login”;
Public String execute() throws Exception;
}
Let write a code using action method in the Hello World example:
Example
Package Commiralipublication. Struts2;
Public class HelloWorldActionf
Private String name;
Public String execute() throws Exception {
Return ’’success”;
}
Public String getName() {
Return name;
}
Public void setName(String name) { this.name = name;
}
}
The above example represents that the action method controls the view, let us make the following change to the execute method and extend the class ActionSupport as follows: package com.niralipublication.struts2;
Create a View
Let us create the below jsp file HelloWorldJsp in the WebContent folder in your IDE. To do this, right click on the WebContent folder in the project explorer and select New <JSP File. This file will be called in case return result is SUCCESS which is a String constant ’’success” as defined in Action interface:
Example:
<%@page contentType-’textZhtml; Charset=UTF-8” %>
<%@ taglib prefix=”s” uri=”Zstruts-tags” %>
<html>
<head>
<title>Hello World<Ztitle>
<Zhead>
<body>
Hello World, <s:property value=”name”Z>
<Zbody>
<Zhtml>
Following is the file which will be invoked by the framework in case action result is ERROR which is equal to String constant ’’error”. Following is the content of AccessDeniedjsp
<%@page contentType-’textZhtml; charset=UTF-8 n %>
<%@ taglib prefix=”s” uri=”Zstruts-tags” %>
<html>
<head>
<title>Access Denied<Ztitle>
<∕head>
<body>
You are not authorized to view this page. Login First
<∕body>
<∕html>
We also need to create index.jsp in the WebContent folder. This file will serve as the initial action URL where the user can click to tell the Struts 2 framework to call the execute method of the HelloWorldAction class and render the HelloWorldjsp view.
Example
<%@ page language-java” contentType=”text∕html; charset=ISO-8859-l” pageEncoding=”ISO-8859-l”%>
<%@ taglib prefιx=”s” uri=’7struts-tags”%>
<!D0CTYPE html PUBLIC “√∕W3C∕∕DTD HTML 4.01 Transitional∕∕EN”
“http://www.w3.org/TR/html4/loose.dtd “>
<html>
<head>
<title>Hello World<∕title>
<∕head>
<body>
<hl>Hello World From Struts2<∕hl>
<form action- , hello”>
<label for=”name”>Please enter your name<∕label><br∕>
<input type=”text” name=”name”∕>
<input type=”submit” value=”Say Hello7>
<∕form>
<∕body>
<∕html>
Now let us create the we.xml file:
<7xml version=”1.0” encoding=”UTF-8”7>
<web-app xmlns:xsi=” http://www.w3 .org∕2001/XMLSchema-instance”
Xmlns=” http://java.sun.com/xml/ns/javaee “
Xmlns: web=”http ://j ava. Sun. Com/xml/ns/j avaee/web-app_2_5 .xsd” xsi: schemaLocation=”http://j ava. Sun. Com/xml/ns/j avaee http ://java. Sun. Com/xml/ns/j avaee/web- app_3_0 .xsd”;
Id=”WebAppJD”; version=”3.0”>
<display-name>Struts 2<∕display-name>
<welcome-file-list>
<welcome-file>indexjsp>∕welcome-file>
<∕welcome-file-list>
<fιlter>
<filter-name>struts2<∕filter-name>
<filter-class>
Org.apache.struts2. Dispatcher.FilterDispatcher
<∕fιlter-class>
<∕filter>
<fιlter-mapping>
<filter-name>struts2<;∕filter-name>
<url-pattern>∕*<∕url-pattern>
<∕filter-mapping>
<∕web-app>
Output:
Execute the Application
Http://localhost:8080/HelloWorldStruts2/index.jsp . This will give you following screen:
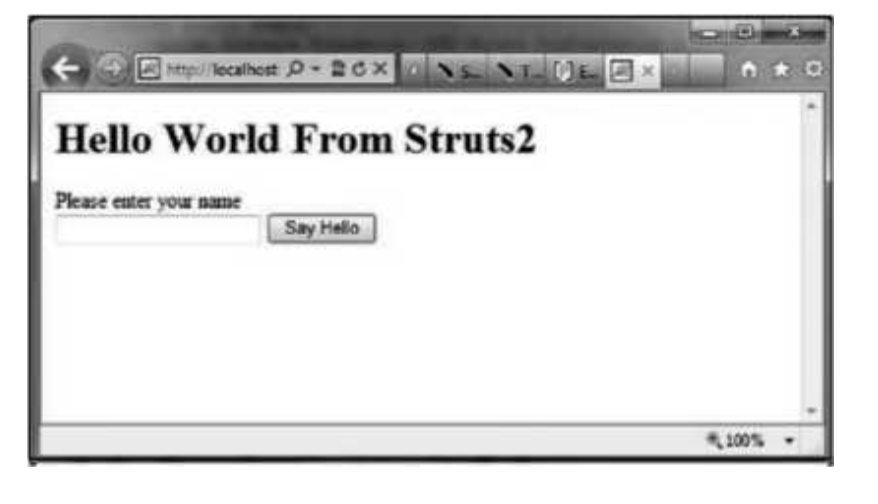
Interceptor is an object that is invoked at the preprocessing and postprocessing of a request. In Struts 2, interceptor is used to perform operations such as validation, exception handling, internationalization, displaying intermediate result etc.
There are many interceptors provided by struts2 framework. The struts2 default interceptors are as follows:
● alias It converts similar parameters that have different names between requests.
● createSession It creates and HttpSession object if it doesn’t exists.
● exception It maps exception to a result.
● cookie It adds a cookie to the current action.
● il8n It provides support to internationalization and localization.
● validation It provides support to input validation.
● token It prevents duplication submission of request.
● timer It outputs the time needed to execute an action.
● StaticParams It maps static properties to action properties.
● IileUpload It provides support to file upload in struts 2.
● exec And Wait It sends an intermediate waiting page for the result.
● ClearSession It unbinds the HttpSession object.
● checkbox It is used to handle the check boxes in the form. By this, we can detect the unchecked checkboxes.
● debugging It provides support of debugging.
Consider the example by using timer and param interceptors, the timer interceptor whose purpose is to measure how long it took to execute an action method. Same time Γm using params interceptor whose purpose is to send the request parameters to the action.
To define the interceptor, we need to declare an interceptor first. The interceptor’s element of package is used to specify interceptors. The interceptor element of interceptors is used to define the custom interceptor. The interceptor-ref subelement of action specifies the interceptor that will be applied for this action. Here, we are specifying the defaultstack interceptors.
Example: Design following pages to display HelloWorld using Interceptor
1. MyInterceptorjava
2. HelloWorldActionjava
3. HelloWorldjsp
4. Indexjsp
5. Struts.xml
6. Web.xml
Package com.niralipublication.struts2;
Import java.util.*;
Import com.xyz.abc.Actioninvocation;
Import com.xyz.abc.interceptor.AbstractInterceptor;
Example (a):
Public class MyInterceptor extends AbstractInterceptor {
Public String intercept(Actioninvocation invocation)throws Exception {
∕* let us do some pre-processing */
String output = ’’Pre-Processing”;
Systenioutprintln(Output);
∕* let us call action or next interceptor */ String result = invocation.invoke();
∕* let us do some post-processing */ output = ’’Post-Processing”;
Systenioutprintln(Output);
Return result;
}
}
Example (b): HelloWorldActionjava
Package com.niralipublication.struts2;
Import com.xyz.abc.ActionSupport;
Public class HelloWorldAction extends ActionSupport { private String name;
Public String execute() throws Exception {
System.outprintln( , Ιnside action.
Return ’’success”;
}
Public String getName() { return name;
}
Public void setName(String name) { this.name = name;
}
Example (c) : HelloWorldjsp
<%@page ContentType- , text∕html; charset=UTF-8 n %>
<%@ taglib prefix=”s” uri=”/struts-tags” %>
<html>
<head>
<title>Hello World<∕title>
<∕head>
<body>
Hello World, <s:property value=”name7>
<∕body>
<∕html>
Example (d) : index.jsp
<%@ page language=”]’ava” contentType=”text∕html; charset=ISO-8859-l&” pageEncoding=”ISO-8859-l”%>
<%@ taglib prefix-’s” uri=7struts-tags”%>
<!DOCTYPE html PUBLIC “-//W3C//DTD HTML 4.01 TransitionaVZEN”
“ http://www.w3.org/TR/html4/loose.dtd “>
<html>
<head>
<title>Hello World<∕title>
<∕head>
<body>
<hl>Hello World From Struts2<∕hl>
<form action-’hello”>
<label for=”name”>Please enter your name<∕label><br∕>
<input type=”text” name=”name”∕>
<input type=”submit” value=”Say Hello7>
<∕form>
<∕body>
<∕html>
Example 5.49 (e): web.xml
<7xml version-Ί.O” encoding=”UTF-8”7>
<web-app xmlns:xsi=” http://www.w3 .org∕2001/XMLSchema-instance”xmlns="http ://java. Sun.com/xmVns/javaee”
Xmlns: web=”http ://java. Sun. Com/xml/ns/j avaee/web-app_2_5 .xsd”
Xsi: schemaLocation=”http ://java. Sun. Com/xml/ns/j avaee http ://java. Sun. Com/xml/ns/javaee/web- app_3_0 .xsd” id=”WebAppJD” version=”3.0 n >
<display-name>Struts 2<∕display-name>
<welcome-file-list>
<welcome-file>index.jsp<∕welcome-file>
<∕welcome-file-list>
<fιlter>
<filter-name>struts2<∕filter-name>
<filter-class>
Org.apache.struts2.dispatcher.FilterDispatcher
<∕fιlter-class>
<∕filter>
<fιlter-mapping>
<filter-name>struts2<;∕filter-name>
<url-pattern>∕*<∕url-pattern>
<∕filter-mapping>
<∕web-app>
Example (f): struts.xml
<7xml version=” 1.0” encoding-’UTF-8’’?>
<!DOCTYPE struts PUBLIC
’’-//Apache Software Foundation∕∕DTD Struts Configuration 2.0//EN”
’’ http://struts.apache.Org/dtds/struts-2.0.dtd” >
<struts>
<constant name=”struts.devMode” value-’true” ∕>
<package name=”helloworld” extends=”struts-default”>
<interceptors>
<interceptor name=”myinterceptor”
Class- , com.niralipublication.struts2.MyInterceptor n ∕>
<∕interceptors>
<action name=”hello”
Class=”com.niralipublication.struts2.HelloWorldAction”
Method- , execute π >
<interceptor-ref name- , params7>
<interceptor-ref name=”myinterceptor” ∕>
<result name= ,, success’ , >∕HelloWorld.jsp<∕result>
<∕action>
<∕package>
<∕struts>
Finally, start Tomcat server and try to access URL
Http://localhost:8080/HelloWorldStruts2/index.jsp . This will give you following screen:
Output
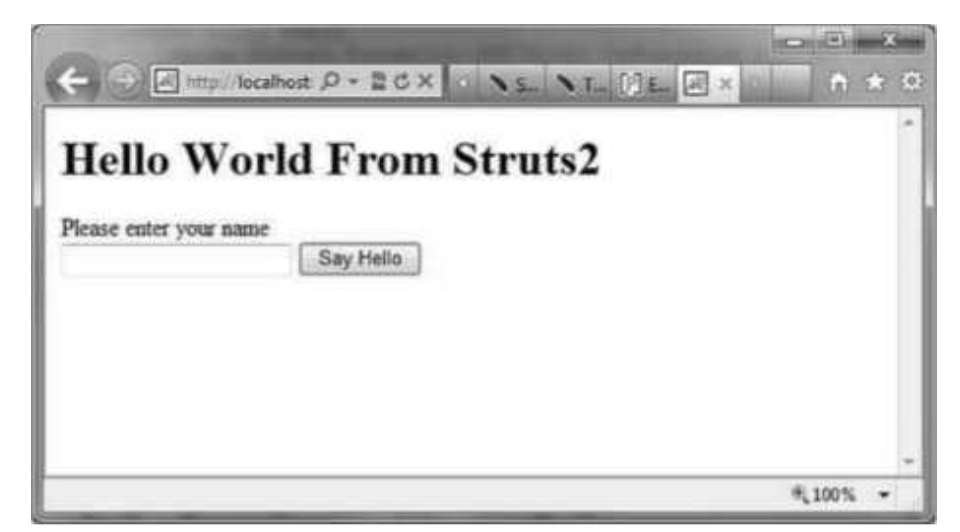
Now, enter any word in the text box and click Say Hello button to execute the defined action. Now if you will check the log generated, you will find following text at the bottom: Pre-Processing
Inside action....
Post-Processing
● The result tag is responsible for redirecting to the destination page which can be specified between the <result> elements together with the name of result (that is, type of result).
● The structure of struts.xml for all the web application of struts 2 is:
<!DOCTYPE>
<struts>
<package name = “name of package” extends = “super package name ,, >
<action name = “name of click action” class = “action class to be executed ,, >
<result name = “name of result’’> path of page to be redirected <∕result>
<∕action>
<∕package>
<∕struts>
● As we seen in the above syntax, after executing the action class, the target view page will be executed based on the configuration in xml file. That is, after the execution of business logic, the result tag is executed.
● For example, if the action method is to authenticate a user, there are three possible situations such as: successftιl login or unsuccessftιl login attempt or if the user tries to enter invalid login credentials for more number of times, the account gets locked.
● The result name actually refers to the type of result which can be categorized in nine types which can are described in figure below.
● There are some predefined result types also which are returned by the action class and compared here as string data type.
String SUCCESS = “success”
String NONE = “none”
String ERROR = “failure”
String INPUT = “input”
String LOGIN = “login”
Also, multiple results can be configured with in a single action element wherein, we can compare the returned string values from action class.
For example, multiple results can be given as:
Example:
<!DOCTYPE>
<struts>
<package name = “name of package” extends = “super package name ,, >
<action name = “name of click action” class = “action class to be executed ,, >
<result name = “failure”> Failure .jsp<;∕result>
<result name = “none”> Index Jsp<;∕result>
<result name = “input’’> Registration.]’sp<∕result>
<result name= “*”> Other Page Jsp<;∕result>
<∕action>
<∕package>
<∕struts>
Struts provide a number of predefined result types. The dispatcher is the default result type. The <results> tag is used to specify a result type in sturts.xml. The dispatcher, FreeMaker and redirect are the commonly used result types.
The user defined result types can be listed as follows:
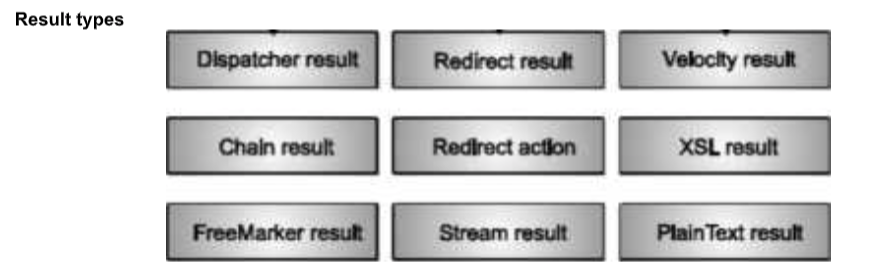
Fig: Result types
1. Dispatcher Result:
The dispatcher result is used to include or forward to a target view page (JSP). It is default result type in Struts2 framework. It uses RequestDispatcher interface to achieve it, where the target Server or JSP receive the same request and response either using forward() or include() method.
The example OfDispatcher result is as follows:
Example : H firstjsp
<%@page ContentType = ’’text/html” pageEncoding = ,, UTF-8”;%>
<%@taglib uri = , 7struts-tags” prefix = ”s” %>
<!DOCTYPE html>
<html>
<head>
<META HTTP-EQUIV = ’’refresh” CONTENT =”2;URL=DispatcherAction.action”>
<title>Struts2 Dispatcher Result<∕title>
<∕head>
<body>
<br∕>
<hl>
As the meta tag is set to 2 seconds, you will be redirected to the next page in 2 seconds..
<∕hl>
<∕body>
<∕html>
H req~disp.java
Package jsp_action
Import javax.servlet. Http.HttpServletRequest ;
Import org.apache.struts2.interceptor.ServletRequestAware;
Import com.opensymphony.xwork2.ActionSupport;
Public class req_disp extends ActionSupport implements ServletRequestAware { HttpServletRequest
Request;
String message;
Public void setServletRequest(HttpServletRequest request) { this.request = request;
}
∕∕ getter and setter method of message
Public void setMessage(String message) { this.message = message;
}
Public String getMessage(){
Return message;
}
Public String execute() throws Exception {
SetMessage(‘Εxample OfDispatcherResult in Struts2”);
Request.setAttribute(“name”, “ABC”);
Request.setAttribute( n address n , ’’Address of ABC”);
Return SUCCESS;
}
}
H next.jsp
<%@page ContentType = “textZhtml” pageEncoding = ”UTF-8”%>
<%@taglib prefix = ”s” uri = &”Zstruts-tags” %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv = ’’Content-Type” content = “textZhtml; charset = UTF-8 π >
<title>JSP Page<∕title>
<∕head>
<body>
<hl>
<hr∕>
Name :<sproperty value = ”#request.name” default = ’’Not Set’7><br>
Message :<s:property value = ’’message” default = ’’Not Set”Z><br>
Address: <s:property value = “#request.address” default = ’’Not Set7><br>
<Zhl>
<∕body>
<∕html>
∕∕ struts.xml
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
“http :ZZstruts. Apache. OrgZdtdsZstruts-2.0. Dtd π >
<struts>
<constant name = ”devMode” value = “true” Z>
<package name = “default” extends = “struts-defaulf’>
<action name =”DispatcherAction” class = “jsp_action.req_disp”>
<result name = ’’success” type = ”dispatcher”> jsp∕next.jsp<∕result>
<∕action>
<∕package>
<∕struts>
2. Chain Action Result:
It is used to achieve action chaining. In the action chaining the multiple actions can be executed in defined sequence. And the target view page will be displayed after last action. In the chain result type, the target resource will be any action which is already configured in struts.xml file.
The example is shown below:
Example
H firstjsp
<%@page ContentType = ’’text/html” pageEncoding = ”UTF-8”%>
<%@taglib uri = , 7struts-tags” prefix = ”s” %>
<!D0CTYPE html>
<html>
<head>
<META HTTP-EQUIV = ’’refresh” CONTENT = ”2;URL=ChainAction.action”>
<title>Struts2 Chain Result<∕title>
<∕head>
<body>
<hl>
After 2 seconds the page will automatically get refreshed and redirected to the next page..
<∕hl>
<∕body>
<∕html>
H next.jsp
<%@page ContentType = ’’text/html” pageEncoding = ”UTF-8”%>
<%@taglib prefix = ”s” uri = ’’/struts-tags” %>
<! DOCTYPE html>
<html>
<head>
<meta http-equiv = ’’Content-Type” content = ’’text/html; charset = UTF-8 ,, >
<title>JSP Page<∕title>
<∕head>
<body>
<ħl>
<hr∕>
Message: <s:property value = ’’message” default = ’’Not Set’7><br>
Name: <s:property value = ”#request.name” default = ’’Not Set7><br>Address : <s:property
Value = “#request. Address” default = ’’Not Set7><br> Company : <s:property value =
“#request.company” default = ’’Not Set7><br> Department: <s:property value = ”#request.dept”
Default = ’’Not Set7><br><∕hl><hr>
<a href = π first.jsp’ , <Try Again<∕a>
<∕body>
<∕html>
H req~disp.java
Package jsp_action;
Import javax. Servlethttp-HttpServletRequest;
Import org. Apache, strut s2. Interceptor-ServletRequestA ware;
Import com.opensymphony.xwork2.ActionSupport;
Public class req_disp extends ActionSupport implements ServletRequestAwaref HttpServletRequest
Request;
String message;
Public void setServletRequest(HttpServletRequest request) {
This.request = request;
}
Public String getMessage(){ return message;
}
Public void setMessage(String message)
{
This.message = message;
}
Public String execute() throws Exception {
SetMessage("Chain Action Example in Struts2 π );
Request.setAttribute(”name”, ’’ABC”);
Request.setAttπbute( n address n , n AddressLines n );
Return SUCCESS;
}
}
H ChainActionjava
Package jsp_action;
Import javax. Servlethttp-HttpServletRequest;
Import org. Apache, strut s2. Interceptor-ServletRequestA ware;
Import com.opensymphony.xwork2.ActionSupport;
Public class ChainAction extends ActionSupport implements ServletRequestAware {
HttpServletRequest request;
Public void setServletRequest(HttpServletRequest request) { this.request = request;
}
Public String execute() throws Exception {
Request.setAttribute( n company n , n My Company”);
Request.setAttribute( n dept n , ’’Information Technology”);
Return SUCCESS;
}
}
H struts.xml
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
“http:ZZstruts.apache.orgZdtdsZstruts-2-0.dtd”>
<struts>
<constant name = ’’devMode” value = ’’true” Z>
<package name = ’’default” extends = ”struts-default”>
<action name = ’’click” class = ”jsp_action.emirp_check”>
<result name = ’’success” type = n dispatcher n >jspZnext.jsp<Zresult>
<Zaction>
<action name = n ChainAction n class = ”jsp_action.ChainAction n >
<result name = ’’success” type = ”chain”>click</result>
<∕action>
<∕package>
<∕struts>
3. FreeMarker Result:
It is used to integrate the Freemarker templates in the view page. Freemaker is a popular templating engine that is used to generate output using predefined templates. Let us create a Freemaker template file called hello.fm with the following contents:
Hello WorldS{name}
Here above file is a template where name is a parameter which will be passed from outside using the defined action and keep this file in your CLASSPATH. Next, let us modify the struts.xml to specify the result as follows:
Example
Struts.xml
<7xml version=”!.0” encoding=”UTF-8”?>
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
” http: ZZstrut s. Apac he. OrgZdtdsZs trut s-2.0. Dtd”>
<struts>
<constant name=”struts.devMode” value-’true” Z>
<package name=”helloworld” extends=”struts-default”>
<action name=”hello”
Class=”com.niralipublication.struts2.HelloWorldAction”
Method- , execute π >
<result name=”success” type=”freemarker”>
<param name=”location’ , >Zhello.fm<Zparam>
<Zresult>
<Zaction>
<Zpackage>
<Zstruts>
4. Redirect Result:
The redirect result is used to redirect the browser request to the new resource. It works based on HttpServletResponse.sendRedirect () method. It is redirected by browser and the target resource can be part of the same application or cannot be part of the same application. That is, the resource should be apart from the application resources.
Example Of Redirect Result Application:
Example
ZZfirstjsp
<%@page ContentType = ’’textZhtml” pageEncoding = ”UTF-8”%>
<%(g)taglib uri = ”Zstruts-tags” prefix = ”s” %>
<!DOCTYPE html>
<html>
<head>
<META HTTP-EQUIV = ’’refresh” CONTENT = ”2;URL = Redirect.action”>
<title> Struts2 Redirect Result Example <∕title>
<∕head>
<body>
<hl>
The content will be refreshed after 2 seconds.
<∕hl>
<∕body>
<∕html>
//jsp_action.java
Package jsp_action;
Import javax. Servlethttp-HttpServletRequest;
Import org.apache.struts2.interceptor.ServletRequestAware;
Import com.opensymphony.xwork2.ActionSupport;
Public class Redirect extends ActionSupport implements ServletRequestAware { HttpServletRequest
Request;
String message;
Public void setServletRequest(HttpServletRequest request) {
This.request = request;
}
Public String getMessage()
{
Return message;
}
Public void SetMessage (String message)
{
This.message = message;
}
Public String execute() throws Exception {
SetMessage( π Redirect Result example in Struts2 n );
Request.setAttribute(”name”, “ABC”);
Request.setAttribute(”address”, ’’AddressLines”);
Return SUCCESS;
}
}
H next.jsp
<%@page ContentType = “textZhtml” pageEncoding = “UTF-8”%>
<%@taglib prefix = “s” uri = “Zstruts-tags” %>
<! DOCTYPE html>
<html>
<body>
<h2>
Struts2 redirect result example<br>
<hr>
Message : <s:property value = ’’message” default = ’’Not Sef7><br>
Name :<sproperty value = ”#request.name” default = ’’Not Sef 7><br>
Address : <s:property value = ”#request.address” default = ’’Not Set’7><br>
<Zh2>
<hr>
<a href = “fιrst.jsp”>Try Again<∕a>
<∕body>
<∕html>
H struts.xml
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
“http 7Zstruts. Apache. OrgZdtdsZstruts-2.0. Dtd π >
<struts>
<constant name = “devMode” value = “true” Z>
<package name = “default” extends = “struts-default”>
<action name = “Redirect” class = “jsp_action.Redirect’’>
<result name = “success” type = “redirect”> next.jsp <Zresult>
<Zaction>
<Zpackage>
<Zstruts>
Validation is a process of checking something against a standard. Struts 2 validation framework provides many generic built-in validation methods or validators to perform various validations. It enables the web container to perform validation rules before executing the actions. We can also create custom validators.
Syntax of Validator:
<validators>
<field name=”fieldName”>
<fιeld-validator type- , emaiΓ>
<message>message string<∕message>
<∕fιeld-validator>
<∕field>
<∕validators>
Ways of performing validations in struts 2.
1. By using built-in validators: Built-in validators in Struts 2 validation framework.
(a) required validator,
b. Requiredstring validator,
c. Validator,
d. Date validator,
e. Int validator,
F. Double validator,
g. Email validator,
h. Regex validator,
i. Url validator.
2. By using custom validators Struts 2 validations by built-in validators
Struts 2 validation framework provides many generic built-in validators to perform various validations like email validation, required validation, stringlength validation etc. These validations are also known as XML based validation. Built-in validators are placed in an xml file. The name of the xml file should be like actionClassName-validation.xml. No need to specify any validate() method in the action class.
Types of validators in struts 2.
1. FieldValidators.
2. Non-fιeld validators.
1. Field validators:
These validators act or perform validations on the single field i.e. field validators are not generic they are specific to the single field. More than one validator can be applied to one field.
Syntax:
<validators>
<field name=”fieldName”>
<fιeld-validator type=”validatorName”>
<message>message string<∕message>
<∕fιeld-validator>
<∕fιeld>
<∕validators>
2. Non-field validators:
These validators are the action level validators. Single validator can be applied to multiple fields.
Note: In this approach only one validator can be applied to a single field.
Syntax:
<validators>
<validator type=” ValidatorName ”>
<param name= π fιeldName’’>username<;∕param>
<message> message string <∕message>
<∕validator>
<∕validators>
A. Struts 2 required validator
The required validator is used to check that the specified field can’t be null.
Syntax:
<validators>
<fieldname-’fieldName ”>
<fιeld-validator type= n required n >
<message>message string<∕message>
<∕fιeld-validator>
<∕fιeld>
<∕validators>
Here we will take an example of required validator whose username and password would be captured using a simple page and we will put two validation to make sure that use always enters a password. The password field should not be null.
(b) Struts 2 stringlength validator
The stringlength validator is used check that the length of the string field is within a specified range. Field validator syntax of stringlength validator.
Example:
<validators>
<field name=”fieldName ”>
<fιeld-validator type-’stringlength ”>
<param name=”minLength”>minLength<∕param>
<param name=”maxLength”> maxLength<∕param>
<param name-’trim”>true<∕param>
<message>message string<∕message>
<∕fιeld-validator>
<∕fιeld>
<∕validators>
Struts 2 stringlength validator example:
//loginjsp
<%@ taglib uri-7struts-tags” prefix=”s”%>
<html>
<head>
<title>Struts 2 stringlength validator example<∕title>
<∕head>
<body>
<h3>This is a stringlength validator example .<Zh3>
<s:form action=”Login”>
<s:textfieldname-’userName” label=&”UserName”Z>
<s:passwordname=”password”label- , Password”Z>
<s:submit value-’login” align=”center , 7>
</s:form>
<∕body>
<∕html>
//web.xml
<7xml version=”l.0” encoding=”UTF-8”?>
<web-app version=”2.5”
Xmlns= n http ://java. Sun. ComZxmlZnsZj avaee ”
Xmlns:xsi=”http://www.w3.org/2001/XMLSchema-instance”
Xsi:schemaLocation=”http://java.sun.com/xml/ns/javaee http ://java. Sun. Com/xml/ns/javaee/web-app_2_5 .xsd’ , > <filter>
<filter-name>struts2<∕filter-name>
<filter-class>
Org.apache.struts2.dispatcher.ng.
Filter. StrutsPrepareAndExecuteF ilter
<∕fιlter-class>
<∕filter>
<fιlter-mapping>
<filter-name>struts2<∕filter-name>
<url-pattern>*<∕url-pattern>
<∕fιlter-mapping>
<welcome-file-list>
<welcome-file>login.jsp<∕welcome-fιle>
<∕welcome-file-list>
<∕web-app>
//struts.xml
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.OZZEN”
“ http://struts.apache.Org/dtds/struts-2.0.dtd” >
<struts>
<package name=”user” extends- , struts-default”>
<action name=”Login”
Class= n Comjavawithease.action.Login”>
<result name= n success n >∕welcome.jsp<∕result>
<result name= n input n >∕loginjsp<∕result>
<∕action>
<∕package>
<∕struts>
//Ioginjava
Import com.opensymphony.xwork2.ActionSupport;
Public class Login extends ActionSupport {
//data members
Private String userName;
Private String password;
//business logic
Public String execute(){ return SUCCESS;
}
//getter setters
Public String getUserName() { return userName;
}
Public void setUserName(String userName) { this.userName = userName;
}
Public String getPassword() { return password;
}
Public void setPassword(String password) { this.password = password;
}
}
//Login-Validatiomxml
<!DOCTYPE validators PUBLIC
n -∕∕OpenSymphony Group∕∕XWork Validator 1.0.2//EN”
“ http://www.opensymphony.com/xwork/xwork-validator-1.0.2.dtd’ r
<validators>
<fιeld name=”userName”>
<field-validator type= π stringlength π >
<param name= π minLength π >8<∕param>
<param name= π maxLength ,, > 16<∕param>
<param name= π trim n >true<∕param>
<message>
Username should be of 8 to 16 characters.
<∕message>
<∕field-validator>
<∕fιeld>
<fιeld name- , password”>
<fιeld-validator type- , string length’ ,>
<param name-ΙninLength”>8<∕param>
<param name-’maxLength”>l 6<∕param>
<param name-⅛hn n>true<∕param>
<message>
Password should be of 8 to 16 characters.
<∕message>
<∕field-validator>
<∕fιeld>
<∕validators>
//welcome.jsp
<%@ tagliburi=7struts-tags” prefιx=”s”%>
<html>
<head>
<title>Stmts 2 stringlength validator example<∕title>
<∕head>
<body>
<h3>This is a stringlength validator example.<∕h3>
Hello <s:property value- , userName” ∕>
<∕body>
<∕html>
Struts 2 email validator
The email validator is used check that the String field is a valid email address and it should not be empty.
Syntax: email validator
<validators>
<field name=”fieldName”>
<field-validator type- , emaiΓ>
<message>message string<∕message>
<∕fιeld-validator>
<∕field>
<∕validators>
(D) Struts 2 url validator
The url validator is used check that the specified url is valid or not.
Syntax of url Validator
<validators>
<field name=”fieldName”>
<fιeld-validator type=”url “>
<param name=”fιeldName”> url<∕param>
<message>message string<∕message>
<∕fιeld-validator>
<∕fιeld>
<∕validators>
● The Struts2 provides feature t for web application can created for global use. The web content will be changed according to the language selection by the user at run time.
● To implement the above concept, Struts2 Internationalization and localization is used. Internationalization is known H8n and localization is known IlOn.
● The main purpose of localization is to translate web content region specific information in the application into corresponding language and country code which is unique.
● A locale is an identifier and represents the local details of the user to the application. The local can be created by java.util.Locale class.
● When a user session begins, the Locale object is sent in the HTTP request as parameter.
● Internationalization is abbreviated i!8n because the word starts with an i and ends with an n, and there are 18 characters between the first i and the last n.
● Below is the list of some country code and their language code:
Country Country Language Language
Name Code Code
Itely IT Italian It
China CN Chinese zh
France FR French fr
Germany DE German de
India IN Hindi hi
Japan JP Japanese Ja
Spain ES Spanish Es
United US English EN
States
● Internationalization is abbreviated il8n because the word starts with an i and ends with an n, and there are 18 characters between the first i and the last n.
● Struts2 provides localization ie. Internationalization (i!8n) support through resource bundles, interceptors and tag libraries in the following places:
● The UI Tags
● Messages and Errors.
● Within action classes.
Resource Bundles
● Struts2 uses resource bundles to provide multiple language and locale options to the users of the web application. You don’t need to worry about writing pages in different languages. All you have to do is to create a resource bundle for each language that you want. The resource bundles will contain titles, messages, and other text in the language of your user. Resource bundles are the file that contains the key/value pairs for the default language of your application.
● The simplest naming format for a resource file is: bundlename_language_country.properties
● Here bundlename could be ActionClass, Interface, Superclass, Model, Package, Global resource properties. Next part language_country represents the country locale for example Spanish (Spain) locale is represented by es_ES and English (United States) locale is represented by en_US etc.
Here you can skip country part which is optional.
When you reference a message element by its key, Struts framework searches for a corresponding message bundle in the following order:
● ActionClass .properties
● Interface.properties
● SuperClass.properties
● IiiodeLproperties
● Package.properties
● Struts.properties
● global.properties
● To develop your application in multiple languages, you would have to maintain multiple property files corresponding to that languages/locale and define all the content in terms of key/value pairs.
● For example if you are going to develop your application for US English (Default), Spanish, and Franch the you would have to create three properties files. Here I will use global.propertiesfιle only; you can make use of different property files to segregate different type of messages.
Global.properties: By default English (United States) will be applied
Global_fr.properties: This will be used for French locale.
Global_es.properties: This will be used for Spanish locale.
Access the messages
● There are several ways to access the message resources, including getText, the text tag, key attribute of UI tags, and the H8n tag. Let us see them in brief:
● To display i!8n text, use a call to getText in the property tag, or any other tag, such as the UI tags as follows:
<s:property value-’getText(‘some.key’)” ∕>
The text tag retrieves a message from the default resource bundle ie. Struts.properties
<s:text name-’some.key” ∕>
The H8n tag pushes an arbitrary resource bundle on to the value stack. Other tags within the scope of the H8n tag can display messages from that resource bundle:
<s:i 18n name-’;some.package.bundle”>
<s:textname-’some.key” ∕>
The key attribute of most UI tags can be used to retrieve a message from a resource bundle:
<s:textfieldkey=”some.key” name=”textfιeldName”∕>
The exception handling is the common task in any Java based application. The Struts 2 framework provides the simple way to handle any uncaught exceptions generated by a web application and also provides robust exception handling, including the ability to automatically log any uncaught exceptions and redirect the user to a error web page. It works on exception interceptor which is part of default-stack in struts-default.xml file in any application.
There are two ways to handle exception:
1. Exception Handling Per Action:
If you need to handle an exception in a specific way for a certain action you can use the exception - mapping node within the action node.
Example
<action name- t ComplexMath”class-’Iiiralipublicatiomactions.ComplexMath’’>
<exception-mapping result-’error”
Exception-java.lang.NumberFormatException” ∕>
<result>∕WEB-INF∕pages∕Success.jsp<∕result>
<result name= π error ,,<∕WEB-INF∕pages∕Error .jsp<∕result>
<∕action>
In the above example, if NumberFormatException exception is occured then custom error page Errorjsp will be executed else framework searches in the global exception list and execute the respective page using global-result type.
Let us see a simple Hello World example with some modification in HelloWorldActionjava file. Here we purposely introduced a NullPointer Exception in our HelloWorldAction action code.
//HelloW OrldActionj ava
Package Commiralipublication. Struts2;
Import com.opensymphony.xwork2.ActionSupport;
Public class HelloWorldAction extends ActionSupport {
Private String name;
Public String execute(){
String x = null;
x = x.substring(O);
Return SUCCESS;
}
Public String getName() {
Return name;
}
Public void setName(String name) {
This.name = name;
}
}
//HelloWorldjsp
<%@ page contentType= π text∕html; charset=UTF-8” %>
<%@ taglibprefix- , s” uri=7struts-tags” %>
<html>
<head>
<title>Hello World<∕title>
<∕head>
<body>
Hello World, <s:property value- , name7>
<∕body>
<∕html>
∕∕ index.jsp
<%@ page language=”]ava” contentType= π text∕html; charset=ISO-8859-l” PageEncoding-ΙSO-
8859-Γ , %>
<%@ taglib prefix=”s” uri=7struts-tags”%>
<!D0CTYPE html PUBLIC ‘ , -ZZW3CZZDTD HTML 4.01 TransitionalZZEN u
“ http://www.w3.org/TR/html4/loose.dtd” >
<html>
<head>
<title>Hello World<∕title>
<∕head>
<body>
<hl>Hello World From Struts2<∕hl>
<form action-”hello”;>
<label for=”name”>Please enter your name<∕label><br∕>
<input type-’text” name-’name7>
<inputtype-’submit” value-’Say Hello7>
<∕form>
<∕body>
<∕html>
∕∕ struts.xml
<7xml version=”!.0” encoding=”UTF-8”?>
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
’’http: ZZstruts. Apache. OrgZdtdsZstrut s-2.0. Dtd ”>
<struts>
<constant name=”struts.devMode” value-’true” ∕>
<package name= ,, helloworld” extends=”struts-default”>
<action name=”hello”
Class=”com.niralipublication.struts2.HelloWorldAction”
Method=”execute”>
<result name=”success”>ZHelloWorld.jsp<Zresult>
<Zaction>
<Zpackage>
<Zstruts>
2. Global Exception Handling:
To enable global exception handling you need to add two nodes to struts.xml: global- exception- mapping and global-results. Using <global-exception-mappings> you can map global exception type for application and <global-results>is used for global result. If any exception occured in action and not configured the exception catching configuration with action configuration in struts.xml then framework searches in the global exception type and map with a result. This result type have to map using global result type. The global results mapping node relates the result value to a specific view page.
Example
<global-results>
<result name= n nulΓ>ZWEB-INFZpagesZError 1 .jsp<∕result>
<result name= n excep n >ZWEB-INFZpagesZError2.jsp<Result>
<∕global-results>
<global-exception-mappings>
<exception-mappingresult-’null” exception- , java.lang.NullPointerException n ∕>
<exception-mappingresult-’excep” exception- , java.lang.Exception”∕> <∕global-exception-mappings>
In the above code, IfNullPointer exception is not mapped in the respective Action class then framework will search in the global exception. And if any other exception is not mapped in the Action class then framework will search in the global exception list. In the above code Errorljsp will be executed if n NullPointerException n occurred and Error2.jsp will be executed if Any other exception will occured.
The struts.xml can be rewritten as:
<7xml version-’l.O” encoding=”UTF-8”?>
<!DOCTYPE struts PUBLIC
’’-//Apache Software FoundationZZDTD Struts Configuration 2.0ZZEN”
n http√Zstruts.apache.orgZdtdsZstruts-2.0.dtd n >
<struts>
<constant name-’struts.devMode” value-’true” ∕>
<package name= n helloworld” extends= n struts-default n >
<global-exception-mappings>
<exception-mapping exception-’java.lang.NullPointerException” result-’error” ∕>
<Zglobal-exception-mappings>
<action name-’hello”
Class= n com.niralipublication.struts2.HelloWorldAction n
Method- , execute n >
<result name= n success n >ZHelloWorld.jsp<Zresult>
<result name= n error n >ZError.jsp<Zresult>
<Zaction>
<Zpackage>
<Zstruts>
Struts framework also provides a way to create a struts application without using struts.xml file. Struts framework provides the annotation to achieve this. A struts application without using struts.xml file is also known as application with Zero Configuration.
The regular useful annotations are:
1. @Action: It is used for action mapping for execute method or marking a class as action class.
2. @Result: It is used to specify the view screen based on the outcome of execute method.
3. @Results: It is used to define multiple results for an action. It is a collection of results.
4. @RequiredFieldValidator: It is used to perform required validation on the field.
Example: Without struts.xml using annotation approach
//Ioginjsp
<%@ tagliburi=7struts-tags n prefιx= n s n %>
<html>
<head>
<title>Struts 2 zero configuration by annotation approach example<∕title>
<∕head>
<body>
<h3>This is a zero configuration by annotation approach example.<∕h3>
<s:property value-’message” ∕> <br∕>
<s:form action’login”>
<s:textfieldname-’userName” Iabel= n UserName n ∕>
<s:password name= n password n Iabel= n Password n ∕>
<s:submit value= n login n align=”center’7>
</s:form>
<∕body>
<∕html>
/∕web.xml
<7xml version= n 1.0 n encoding= ,, UTF-8 n 7>
<web-app version= n 2.5 n
Xmlns= n http ://java. Sun. Com/xml/ns/javaee n
Xmlns:xsi=”http://www.w3.org/2001/XMLScheina-instance”
Xsi:schemaLocation=”http://java.sun.com/xml/ns/javaee
Http ://java. Sun. Com/xml/ns/j avaee/web-app_2_5 .xsd’ , >
<filter>
<filter-name>struts2<∕filter-name>
<fιlter-class>
Org.apache.struts2.dispatcher.ng.
Filter. StrutsPrepareAndExecuteF ilter
<∕fιlter-class>
<∕filter>
<fιlter-mapping>
<fiher-name>struts2<∕filter-name>
<url-pattern>∕*<∕url-pattern>
<∕fιlter-mapping>
<welcome-file-list>
<welcome-file&>login.jsp<∕welcome-fιle>
<∕welcome-file-list>
<∕web-app>
//Loginjava
Import org. Apache, struts2. Conventiomannotatiom Aetion;
Import org. Apache, struts2. ConventiomannotatiomResult;
Import com.opensymphony.xwork2.ActionSupport;
@Action(value=”login”, results={
@Result(name=”success”,Iocation= n Zwelcomejsp”),
@Resuh(name=”error”,Iocation-Vlogimjsp n )
})
Public class Login extends ActionSupport {
//data members
Private String userName;
Private String password;
Private String message;
//business logic
Public String execute(){
If(userName.equals( n jai n ) && password.equals( n 1234 n )){ setMessage( n Hello ” +userName + ”,
You are Successfiilly logged in.”);
Return SUCCESS;
}else{
SetMessage(“Invalid username or password.”); return ERROR;
}
}
//getter setters
Public String getUserName() {
Return userName;
}
Public void setUserName(String userName) { this.userName = userName;
}
Public String getPassword() { return password;
}
Public void setPassword(String password) { this.password = password;
}
Public String getMessage() { return message;
}
Public void setMessage(String message) { this.message = message;
}
}
//login-error .jsp
<jsp:include page=”/login, jsp”><∕jsp: include>
//login-error .jsp
<%@ taglib uri=7struts-tags” prefιx=”s”%>
<html>
<head>
<title>Struts 2 zero configuration by annotation approach example<∕title>
<∕head>
<body>
<h3>This is a zero configuration by annotation approach example <∕h3>
<s:property value-’message” ∕>
<∕body>
<∕html>
Web applications have become an inextricable aspect of our life. They are simple to use, but difficult to create. Web application development is made easier with WYSIWYG editors, form builders, mashup editors, and markup authoring tools. Advanced Web Applications, on the other hand, necessitate server-side programming, which is beyond the capabilities of end-user developers. In this paper, we look at how declarative languages can help end-users become Web developers by making Web application development easier.
We begin by identifying nine different stages of end-user Web application development, ranging from basic visual customization to expert three-tier programming. The presentation tier would then be expanded to handle all aspects of Web application development. We offer XFormsDB, a unified XForms-based framework that can be used to construct both client-side and server-side Web applications.
Furthermore, we propose the XFormsRTC language extension for introducing actual real-time communication capabilities to XForms. We also show how to use the XFormsDB Integrated Development Environment (XIDE), which helps end-users create highly interactive data-driven Web applications. XIDE encourages the transition from markup authoring and snippet programming to single and unified language programming at all levels of Web application development.
Highly interactive data-driven Web Applications—hereafter referred to as Web Applications—are typically built using a three-tier design [2]. The presentation tier (i.e., user interface) is defined in HTML and CSS, with client-side application logic provided through multiple JavaScript embeddings. The logic tier (i.e., server-side application logic) is written in an object-oriented (e.g., Java or Ruby) or scripting (e.g., PHP) language and communicates with the client using HTML, XML, or JSON formats. Finally, for data management, the data tier (i.e., application data) employs either an Object-Relational Mapping (ORM) library or SQL statements.
Web applications typically include both imperative (Java and JavaScript) and declarative (HTML, CSS, and SQL) components. As a result, Web developers must be familiar with a variety of programming languages as well as the conceptual distinctions between them. Each layer is often developed by tier-specific professionals (e.g., Web designers, software engineers, and database experts).
End-users, on the other hand, are also involved in Web development. They design and edit Web Applications that aid them in their regular tasks without the assistance of professionals. End-users post in forums and wikis, build mashups and polls, edit media-rich blog pages, and create basic HTML pages, among other things. Non-professionals who conduct some Web programming to support their professional or recreational interests are referred to as end-user developers in the following.
End-user developers frequently use dedicated visual tools to create Web Applications, such as survey builders, mashup editors, or component-based tools. Unfortunately, these visual tools have their limitations. Professional tools are more expressive, but they necessitate a deeper understanding of several programming languages, technologies, and paradigms. As a result, end-user developers are confronted with the above-mentioned complications of professional Web application development.
We want to close the gap between end-user Web application development and professional Web application development. End-users who need to progress beyond markup authoring and snippet programming are our emphasis. First, we look at how moving from end-user to professional Web development hampers Web application development. At the same time, we identify the most significant learning obstacles. Then we'll talk about how end users might use their existing Web development skills to get around these learning obstacles.
Declarative Web Application Development is the topic of this study. The following are our unique contributions to the framework, IDE, and minor/other contributions:
● End-user Web Application development activities are classified into nine levels, with an emphasis on sophisticated Web development (minor).
● Unifying Web Application development with a presentation-centric architectural approach based on W3C-standardized declarative languages (framework).
● Set of requirements for adding standard server-side and database-related functionality to a presentation-centric declarative language, including real-time communication features (framework).
● XFormsDB is a language extension that turns XForms into a complete Web application development language (framework).
● XFormsRTC is a language extension that adds real-time communication capabilities to XForms (framework).
● Web framework (XFormsDB) for declarative Web application development that uses XForms and the XFormsDB language extension (framework).
● XIDE is a web-based visual authoring tool for the XFormsDB platform that helps lower-level end-user developers construct Web applications (IDE).
● The XFormsDB framework and the XIDE authoring tool are being evaluated (framework and IDE).
References:
- Robert W. Sebesta, “Programming the World Wide Web”, 4th Edition, Pearson education, 2008
- Chris Bates, “Web Programming Building Internet Applications”, 3rd Edition, Wiley India, 2006.
- Xue Bai et al, “The web Warrior Guide to Web Programming”, Thomson, 2003.
- Marty Hall, Larry Brown, “Core Web Programming", Second Edition, Pearson Education, 2001, ISBN 978-0130897930.