Unit 2
Classes & Objects
Introduction to Class
- Class is used to create user defined data type.
- C++ provides various built in types like float, int and char.
- Similarly, if user wants to create his own data type then he can create it with the help of class.
Class Book
- Here user defined data type “Book” is created with the help of class keyword class.
- Class consists of data members (variables) and associated member functions.
Structure of a class
Class |
Data Members |
Member functions |
- Data members (variables) represent attributes and member functions represent behaviour of class.
Structure of a “Book” class
Book |
Data Members Name Pages Price |
Member functions Accept() Display() |
- Class only provides template for object and does not create any memory space for object. Hence class is logical abstract entity.
☞ Structure of class declaration
Class class_name
{
Access specifier:
Data Members;
Access specifier:
Member Functions;
};
We will discuss new terms in above declaration.
☞ Class
- Classes are created using keyword “class”.
- The body of class is enclosed within curly braces and terminated by semicolon.
- Class contains data members and Member functions. They are called as class members.
☞
☞ Access Specifier
It specifies if class members are accessible to external functions (functions of other classes).
C++ provides three access specifiers :
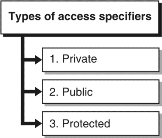
Fig. C3.2 : Types of access specifiers
1. private
- Private members are accessible to functions of same class.
- They are not accessible to functions of other classes.
- By default members of class are private.
- Private members are specified by keyword “private”.
2. public
- Public members are accessible to functions of other classes.
- Public members are specified by keyword “public”.
3. protected
- It is used only when inheritance is involved.
- Protected members of super class are accessible to its immediate sub class.
- Protected members are specified by keyword “protected”.
- If all member functions of a class are “friend” to another class then such class is known as friend class.
Class A
{
Friend class B;
};
- Here class B is declared as friend of class A. So B can access all private and protected members of class A, but A does not have access to private and protected members of class B.
Write a program to calculate area of cube and triangle using friend class
Solution :
#include <iostream>
Using namespace std;
Class cube; // Step 1 forward declaration
Class Triangle {
Intx,y;
Public:
Triangle(inta,int b) // constructor
{
x=a;
y=b;
}
Void display()
{
Cout<< "Area of triangle is " << ½*x*y <<endl;
}
Void area(cube &); // Step 2
};
Class cube{
Int s;
Public:
Cube(int p )
{
s=p;
}
Void show()
{
Cout<< "Volume of cube is: " << s*s*s <<endl;
}
Friend class triangle; //Step 3 triangle is friend of class cube
};
Void triangle::area(cube &p)
{
x = p.s; //Step 4
y = p.s;
}
Int main () {
Triangle T(10,5);
Cube C(10);
T.display();
C.show();
T.area(C);
T.display();
C.show();
Return 0;
}
- Class cube is referred in class triangle before defining it. So it is declare beforehand below header file. See step 1, this is called as forward declaration.
- As triangle is declared as friend of class cube it can access private and protected members of class cube. See step 3.
- It can access member s of class cube. See step 4.
Output
Area of triangle is 25
Volume of cube is 1000
Area of triangle is 50
Volume of cube is 1000
KEY TAKEAWAY
Introduction to Class
- Class is used to create user defined data type.
- C++ provides various built in types like float, int and char.
- Similarly, if user wants to create his own data type then he can create it with the help of class.
A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. It operates on any object of the class of which it is a member, and has access to all the members of a class for that object.
Let us take previously defined class to access the members of the class using a member function instead of directly accessing them −
Class Box {
Public:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
Double getVolume(void);// Returns box volume
};
Member functions can be defined within the class definition or separately using scope resolution operator, : −. Defining a member function within the class definition declares the function inline, even if you do not use the inline specifier. So either you can define Volume() function as below −
Class Box {
Public:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
Double getVolume(void) {
Return length * breadth * height;
}
};
If you like, you can define the same function outside the class using the scope resolution operator (::) as follows −
Double Box::getVolume(void) {
Return length * breadth * height;
}
Here, only important point is that you would have to use class name just before :: operator. A member function will be called using a dot operator (.) on a object where it will manipulate data related to that object only as follows −
Box myBox; // Create an object
MyBox.getVolume(); // Call member function for the object
Let us put above concepts to set and get the value of different class members in a class −
#include <iostream>
Using namespace std;
Class Box {
Public:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
// Member functions declaration
Double getVolume(void);
Void setLength( double len );
Void setBreadth( double bre );
Void setHeight( double hei );
};
// Member functions definitions
Double Box::getVolume(void) {
Return length * breadth * height;
}
Void Box::setLength( double len ) {
Length = len;
}
Void Box::setBreadth( double bre ) {
Breadth = bre;
}
Void Box::setHeight( double hei ) {
Height = hei;
}
// Main function for the program
Int main() {
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
Double volume = 0.0; // Store the volume of a box here
// box 1 specification
Box1.setLength(6.0);
Box1.setBreadth(7.0);
Box1.setHeight(5.0);
// box 2 specification
Box2.setLength(12.0);
Box2.setBreadth(13.0);
Box2.setHeight(10.0);
// volume of box 1
Volume = Box1.getVolume();
Cout << "Volume of Box1 : " << volume <<endl;
// volume of box 2
Volume = Box2.getVolume();
Cout << "Volume of Box2 : " << volume <<endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Volume of Box1 : 210
Volume of Box2 : 1560
KEY TAKEAWAY
A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. It operates on any object of the class of which it is a member, and has access to all the members of a class for that object.
Static data members are class members that are declared using the static keyword. There is only one copy of the static data member in the class, even if there are many class objects. This is because all the objects share the static data member. The static data member is always initialized to zero when the first class object is created.
The syntax of the static data members is given as follows −
Static data_type data_member_name;
In the above syntax, static keyword is used. The data_type is the C++ data type such as int, float etc. The data_member_name is the name provided to the data member.
A program that demonstrates the static data members in C++ is given as follows −
Example
#include <iostream>
#include<string.h>
Using namespace std;
Class Student {
Private:
Int rollNo;
Char name[10];
Int marks;
Public:
Static int objectCount;
Student() {
ObjectCount++;
}
Void getdata() {
Cout << "Enter roll number: "<<endl;
Cin >> rollNo;
Cout << "Enter name: "<<endl;
Cin >> name;
Cout << "Enter marks: "<<endl;
Cin >> marks;
}
Void putdata() {
Cout<<"Roll Number = "<< rollNo <<endl;
Cout<<"Name = "<< name <<endl;
Cout<<"Marks = "<< marks <<endl;
Cout<<endl;
}
};
Int Student::objectCount = 0;
Int main(void) {
Student s1;
s1.getdata();
s1.putdata();
Student s2;
s2.getdata();
s2.putdata();
Student s3;
s3.getdata();
s3.putdata();
Cout << "Total objects created = " << Student::objectCount << endl;
Return 0;
}
Output
The output of the above program is as follows −
Enter roll number: 1
Enter name: Mark
Enter marks: 78
Roll Number = 1
Name = Mark
Marks = 78
Enter roll number: 2
Enter name: Nancy
Enter marks: 55
Roll Number = 2
Name = Nancy
Marks = 55
Enter roll number: 3
Enter name: Susan
Enter marks: 90
Roll Number = 3
Name = Susan
Marks = 90
Total objects created = 3
In the above program, the class student has three data members denoting the student roll number, name and marks. The objectCount data member is a static data member that contains the number of objects created of class Student. Student() is a constructor that increments objectCount each time a new class object is created.
There are 2 member functions in class. The function getdata() obtains the data from the user and putdata() displays the data. The code snippet for this is as follows −
Class Student {
Private:
Int rollNo;
Char name[10];
Int marks;
Public:
Static int objectCount;
Student() {
ObjectCount++;
}
Void getdata() {
Cout << "Enter roll number: "<<endl;
Cin >> rollNo;
Cout << "Enter name: "<<endl;
Cin >> name;
Cout << "Enter marks: "<<endl;
Cin >> marks;
}
Void putdata() {
Cout<<"Roll Number = "<< rollNo <<endl;
Cout<<"Name = "<< name <<endl;
Cout<<"Marks = "<< marks <<endl;
Cout<<endl;
}
};
In the function main(), there are three objects of class Student i.e. s1, s2 and s3. For each of these objects getdata() and putdata() are called. At the end, the value of objectCount is displayed. This is given below −
Int main(void) {
Student s1;
s1.getdata();
s1.putdata();
Student s2;
s2.getdata();
s2.putdata();
Student s3;
s3.getdata();
s3.putdata();
Cout << "Total objects created = " << Student::objectCount << endl;
Return 0;
}
KEY TAKEAWAY
Static data members are class members that are declared using the static keyword. There is only one copy of the static data member in the class, even if there are many class objects. This is because all the objects share the static data member. The static data member is always initialized to zero when the first class object is created.
We can define class members static using static keyword. When we declare a member of a class as static it means no matter how many objects of the class are created, there is only one copy of the static member.
A static member is shared by all objects of the class. All static data is initialized to zero when the first object is created, if no other initialization is present. We can't put it in the class definition but it can be initialized outside the class as done in the following example by redeclaring the static variable, using the scope resolution operator :: to identify which class it belongs to.
Let us try the following example to understand the concept of static data members −
#include <iostream>
Using namespace std;
Class Box {
Public:
Static int objectCount;
// Constructor definition
Box(double l = 2.0, double b = 2.0, double h = 2.0) {
Cout <<"Constructor called." << endl;
Length = l;
Breadth = b;
Height = h;
// Increase every time object is created
ObjectCount++;
}
Double Volume() {
Return length * breadth * height;
}
Private:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
};
// Initialize static member of class Box
Int Box::objectCount = 0;
Int main(void) {
Box Box1(3.3, 1.2, 1.5); // Declare box1
Box Box2(8.5, 6.0, 2.0); // Declare box2
// Print total number of objects.
Cout << "Total objects: " << Box::objectCount << endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Constructor called.
Constructor called.
Total objects: 2
Static Function Members
By declaring a function member as static, you make it independent of any particular object of the class. A static member function can be called even if no objects of the class exist and the static functions are accessed using only the class name and the scope resolution operator ::.
A static member function can only access static data member, other static member functions and any other functions from outside the class.
Static member functions have a class scope and they do not have access to the this pointer of the class. You could use a static member function to determine whether some objects of the class have been created or not.
Let us try the following example to understand the concept of static function members −
#include <iostream>
Using namespace std;
Class Box {
Public:
Static int objectCount;
// Constructor definition
Box(double l = 2.0, double b = 2.0, double h = 2.0) {
Cout <<"Constructor called." << endl;
Length = l;
Breadth = b;
Height = h;
// Increase every time object is created
ObjectCount++;
}
Double Volume() {
Return length * breadth * height;
}
Static int getCount() {
Return objectCount;
}
Private:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
};
// Initialize static member of class Box
Int Box::objectCount = 0;
Int main(void) {
// Print total number of objects before creating object.
Cout << "Inital Stage Count: " << Box::getCount() << endl;
Box Box1(3.3, 1.2, 1.5); // Declare box1
Box Box2(8.5, 6.0, 2.0); // Declare box2
// Print total number of objects after creating object.
Cout << "Final Stage Count: " << Box::getCount() << endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Inital Stage Count: 0
Constructor called.
Constructor called.
Final Stage Count: 2
KEY TAKEAWAY
A static member function can only access static data member, other static member functions and any other functions from outside the class.
Static member functions have a class scope and they do not have access to the this pointer of the class. You could use a static member function to determine whether some objects of the class have been created or not.
Data hiding is one of the important features of Object Oriented Programming which allows preventing the functions of a program to access directly the internal representation of a class type. The access restriction to the class members is specified by the labeled public, private, and protected sections within the class body. The keywords public, private, and protected are called access specifiers.
A class can have multiple public, protected, or private labeled sections. Each section remains in effect until either another section label or the closing right brace of the class body is seen. The default access for members and classes is private.
Class Base {
Public:
// public members go here
Protected:
// protected members go here
Private:
// private members go here
};
The public Members
A public member is accessible from anywhere outside the class but within a program. You can set and get the value of public variables without any member function as shown in the following example −
#include <iostream>
Using namespace std;
Class Line {
Public:
Double length;
Void setLength( double len );
Double getLength( void );
};
// Member functions definitions
Double Line::getLength(void) {
Return length ;
}
Void Line::setLength( double len) {
Length = len;
}
// Main function for the program
Int main() {
Line line;
// set line length
Line.setLength(6.0);
Cout << "Length of line : " << line.getLength() <<endl;
// set line length without member function
Line.length = 10.0; // OK: because length is public
Cout << "Length of line : " << line.length <<endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Length of line : 6
Length of line : 10
The private Members
A private member variable or function cannot be accessed, or even viewed from outside the class. Only the class and friend functions can access private members.
By default all the members of a class would be private, for example in the following class width is a private member, which means until you label a member, it will be assumed a private member −
Class Box {
Double width;
Public:
Double length;
Void setWidth( double wid );
Double getWidth( void );
};
Practically, we define data in private section and related functions in public section so that they can be called from outside of the class as shown in the following program.
#include <iostream>
Using namespace std;
Class Box {
Public:
Double length;
Void setWidth( double wid );
Double getWidth( void );
Private:
Double width;
};
// Member functions definitions
Double Box::getWidth(void) {
Return width ;
}
Void Box::setWidth( double wid ) {
Width = wid;
}
// Main function for the program
Int main() {
Box box;
// set box length without member function
Box.length = 10.0; // OK: because length is public
Cout << "Length of box : " << box.length <<endl;
// set box width without member function
// box.width = 10.0; // Error: because width is private
Box.setWidth(10.0); // Use member function to set it.
Cout << "Width of box : " << box.getWidth() <<endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Length of box : 10
Width of box : 10
The protected Members
A protected member variable or function is very similar to a private member but it provided one additional benefit that they can be accessed in child classes which are called derived classes.
You will learn derived classes and inheritance in next chapter. For now you can check following example where I have derived one child class SmallBox from a parent class Box.
Following example is similar to above example and here width member will be accessible by any member function of its derived class SmallBox.
#include <iostream>
Using namespace std;
Class Box {
Protected:
Double width;
};
Class SmallBox:Box { // SmallBox is the derived class.
Public:
Void setSmallWidth( double wid );
Double getSmallWidth( void );
};
// Member functions of child class
Double SmallBox::getSmallWidth(void) {
Return width ;
}
Void SmallBox::setSmallWidth( double wid ) {
Width = wid;
}
// Main function for the program
Int main() {
SmallBox box;
// set box width using member function
Box.setSmallWidth(5.0);
Cout << "Width of box : "<< box.getSmallWidth() << endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Width of box : 5
KEY TAKEAWAY
Data hiding is one of the important features of Object Oriented Programming which allows preventing the functions of a program to access directly the internal representation of a class type. The access restriction to the class members is specified by the labelled public, private, and protected sections within the class body. The keywords public, private, and protected are called access specifiers.
A class can have multiple public, protected, or private labeled sections. Each section remains in effect until either another section label or the closing right brace of the class body is seen. The default access for members and classes is private.
A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. It operates on any object of the class of which it is a member, and has access to all the members of a class for that object.
Let us take previously defined class to access the members of the class using a member function instead of directly accessing them −
Class Box {
Public:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
Double getVolume(void);// Returns box volume
};
Member functions can be defined within the class definition or separately using scope resolution operator: −. Defining a member function within the class definition declares the function inline, even if you do not use the inline specifier. So either you can define Volume() function as below −
Class Box {
Public:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
Double getVolume(void) {
Return length * breadth * height;
}
};
If you like, you can define the same function outside the class using the scope resolution operator (::) as follows −
Double Box::getVolume(void) {
Return length * breadth * height;
}
Here, only important point is that you would have to use class name just before :: operator. A member function will be called using a dot operator (.) on a object where it will manipulate data related to that object only as follows −
Box myBox; // Create an object
MyBox.getVolume(); // Call member function for the object
Let us put above concepts to set and get the value of different class members in a class −
#include <iostream>
Using namespace std;
Class Box {
Public:
Double length; // Length of a box
Double breadth; // Breadth of a box
Double height; // Height of a box
// Member functions declaration
Double getVolume(void);
Void setLength( double len );
Void setBreadth( double bre );
Void setHeight( double hei );
};
// Member functions definitions
Double Box::getVolume(void) {
Return length * breadth * height;
}
Void Box::setLength( double len ) {
Length = len;
}
Void Box::setBreadth( double bre ) {
Breadth = bre;
}
Void Box::setHeight( double hei ) {
Height = hei;
}
// Main function for the program
Int main() {
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
Double volume = 0.0; // Store the volume of a box here
// box 1 specification
Box1.setLength(6.0);
Box1.setBreadth(7.0);
Box1.setHeight(5.0);
// box 2 specification
Box2.setLength(12.0);
Box2.setBreadth(13.0);
Box2.setHeight(10.0);
// volume of box 1
Volume = Box1.getVolume();
Cout << "Volume of Box1 : " << volume <<endl;
// volume of box 2
Volume = Box2.getVolume();
Cout << "Volume of Box2 : " << volume <<endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Volume of Box1 : 210
Volume of Box2 : 1560
KEY TAKEAWAY
A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. It operates on any object of the class of which it is a member, and has access to all the members of a class for that object.
An object of class represents a single record in memory, if we want more than one record of class type, we have to create an array of class or object. As we know, an array is a collection of similar type, therefore an array can be a collection of class type.
Syntax for Array of object
Class class-name
{
Datatype var1;
Datatype var2;
- - - - - - - - - -
Datatype varN;
Method1();
Method2();
- - - - - - - - - -
MethodN();
};
Class-name obj[ size ];
Example for Array of object
#include<iostream.h>
#include<conio.h>
Class Employee
{
Int Id;
Char Name[25];
Int Age;
Long Salary;
Public:
Void GetData() //Statement 1 : Defining GetData()
{
Cout<<"\n\tEnter Employee Id : ";
Cin>>Id;
Cout<<"\n\tEnter Employee Name : ";
Cin>>Name;
Cout<<"\n\tEnter Employee Age : ";
Cin>>Age;
Cout<<"\n\tEnter Employee Salary : ";
Cin>>Salary;
}
Void PutData() //Statement 2 : Defining PutData()
{
Cout<<"\n"<<Id<<"\t"<<Name<<"\t"<<Age<<"\t"<<Salary;
}
};
Void main()
{
Int i;
Employee E[3]; //Statement 3 : Creating Array of 3 Employees
For(i=0;i<3;i++)
{
Cout<<"\nEnter details of "<<i+1<<" Employee";
E[i].GetData();
}
Cout<<"\nDetails of Employees";
For(i=0;i<3;i++)
E[i].PutData();
}
Output :
Enter details of 1 Employee
Enter Employee Id : 101
Enter Employee Name : Suresh
Enter Employee Age : 29
Enter Employee Salary : 45000
Enter details of 2 Employee
Enter Employee Id : 102
Enter Employee Name : Mukesh
Enter Employee Age : 31
Enter Employee Salary : 51000
Enter details of 3 Employee
Enter Employee Id : 103
Enter Employee Name : Ramesh
Enter Employee Age : 28
Enter Employee Salary : 47000
Details of Employees
101 Suresh 29 45000
102 Mukesh 31 51000
103 Ramesh 28 47000
In the above example, we are getting and displaying the data of 3 employee using array of object. Statement 1 is creating an array of Employee Emp to store the records of 3 employees.
KEY TAKEAWAY
An object of class represents a single record in memory, if we want more than one record of class type, we have to create an array of class or object. As we know, an array is a collection of similar type, therefore an array can be a collection of class type.
In C++ we can pass class’s objects as arguments and also return them from a function the same way we pass and return other variables. No special keyword or header file is required to do so.
Passing an Object as argument
To pass an object as an argument we write the object name as the argument while calling the function the same way we do it for other variables.
Syntax:
Function_name(object_name);
Example: In this Example there is a class which has an integer variable ‘a’ and a function ‘add’ which takes an object as argument. The function is called by one object and takes another as an argument. Inside the function, the integer value of the argument object is added to that on which the ‘add’ function is called. In this method, we can pass objects as an argument and alter them.
// C++ program to show passing // of objects to a function
#include <bits/stdc++.h> Using namespace std;
Class Example { Public: Int a;
// This function will take // an object as an argument Void add(Example E) { a = a + E.a; } };
// Driver Code Int main() {
// Create objects Example E1, E2;
// Values are initialized for both objects E1.a = 50; E2.a = 100;
Cout << "Initial Values \n"; Cout << "Value of object 1: " << E1.a << "\n& object 2: " << E2.a << "\n\n";
// Passing object as an argument // to function add() E2.add(E1);
// Changed values after passing // object as argument Cout << "New values \n"; Cout << "Value of object 1: " << E1.a << "\n& object 2: " << E2.a << "\n\n";
Return 0; } |
Output:
Initial Values
Value of object 1: 50
& object 2: 100
New values
Value of object 1: 50
& object 2: 150
Returning Object as argument
Syntax:
Object = return object_name;
Example: In the above example we can see that the add function does not return any value since its return-type is void. In the following program the add function returns an object of type ‘Example'(i.e., class name) whose value is stored in E3.
In this example, we can see both the things that are how we can pass the objects as well as return them. When the object E3 calls the add function it passes the other two objects namely E1 & E2 as arguments. Inside the function, another object is declared which calculates the sum of all the three variables and returns it to E3.
This code and the above code is almost the same, the only difference is that this time the add function returns an object whose value is stored in another object of the same class ‘Example’ E3. Here the value of E1 is displayed by object1, the value of E2 by object2 and value of E3 by object3.
// C++ program to show passing // of objects to a function
#include <bits/stdc++.h> Using namespace std;
Class Example { Public: Int a;
// This function will take // object as arguments and // return object Example add(Example Ea, Example Eb) { Example Ec; Ec.a = 50; Ec.a = Ec.a + Ea.a + Eb.a;
// returning the object Return Ec; } }; Int main() { Example E1, E2, E3;
// Values are initialized // for both objects E1.a = 50; E2.a = 100; E3.a = 0;
Cout << "Initial Values \n"; Cout << "Value of object 1: " << E1.a << ", \nobject 2: " << E2.a << ", \nobject 3: " << E3.a << "\n";
// Passing object as an argument // to function add() E3 = E3.add(E1, E2); // Changed values after // passing object as an argument Cout << "New values \n"; Cout << "Value of object 1: " << E1.a << ", \nobject 2: " << E2.a << ", \nobject 3: " << E3.a << "\n";
Return 0; } |
Output:
Initial Values
Value of object 1: 50,
Object 2: 100,
Object 3: 0
New values
Value of object 1: 50,
Object 2: 100,
Object 3: 200
KEY TAKEAWAY
In C++ we can pass class’s objects as arguments and also return them from a function the same way we pass and return other variables. No special keyword or header file is required to do so.
C++ Constructor
In C++, constructor is a special method which is invoked automatically at the time of object creation. It is used to initialize the data members of new object generally. The constructor in C++ has the same name as class or structure.
There can be two types of constructors in C++.
- Default constructor
- Parameterized constructor
C++ Default Constructor
A constructor which has no argument is known as default constructor. It is invoked at the time of creating object.
Let's see the simple example of C++ default Constructor.
- #include <iostream>
- Using namespace std;
- Class Employee
- {
- Public:
- Employee()
- {
- Cout<<"Default Constructor Invoked"<<endl;
- }
- };
- Int main(void)
- {
- Employee e1; //creating an object of Employee
- Employee e2;
- Return 0;
- }
Output:
Default Constructor Invoked
Default Constructor Invoked
C++ Parameterized Constructor
A constructor which has parameters is called parameterized constructor. It is used to provide different values to distinct objects.
Let's see the simple example of C++ Parameterized Constructor.
#include <iostream>
Using namespace std;
Class Employee {
Public:
Int id;//data member (also instance variable)
String name;//data member(also instance variable)
Float salary;
Employee(int i, string n, float s)
{
Id = i;
Name = n;
Salary = s;
}
Void display()
{
Cout<<id<<" "<<name<<" "<<salary<<endl;
}
};
Int main(void) {
Employee e1 =Employee(101, "Sonoo", 890000); //creating an object of Employee
Employee e2=Employee(102, "Nakul", 59000);
e1.display();
e2.display();
Return 0;
}
Output:
101 Sonoo 890000
102 Nakul 59000
KEY TAKEAWAY
In C++, constructor is a special method which is invoked automatically at the time of object creation. It is used to initialize the data members of new object generally. The constructor in C++ has the same name as class or structure.
There can be two types of constructors in C++.
- Default constructor
- Parameterized constructor
In C++, We can have more than one constructor in a class with same name, as long as each has a different list of arguments. This concept is known as Constructor Overloading and is quite similar to function overloading.
- Overloaded constructors essentially have the same name (name of the class) and different number of arguments.
- A constructor is called depending upon the number and type of arguments passed.
- While creating the object, arguments must be passed to let compiler know, which constructor needs to be called.
// C++ program to illustrate // Constructor overloading #include <iostream> Using namespace std;
Class construct {
Public: Float area;
// Constructor with no parameters Construct() { Area = 0; }
// Constructor with two parameters Construct(int a, int b) { Area = a * b; }
Void disp() { Cout<< area<< endl; } };
Int main() { // Constructor Overloading // with two different constructors // of class name Construct o; Construct o2( 10, 20);
o.disp(); o2.disp(); Return 1; } |
Output:
0
200
KEY TAKEAWAY
In C++, We can have more than one constructor in a class with same name, as long as each has a different list of arguments. This concept is known as Constructor Overloading and is quite similar to function overloading.
- Overloaded constructors essentially have the same name (name of the class) and different number of arguments.
- A constructor is called depending upon the number and type of arguments passed.
- While creating the object, arguments must be passed to let compiler know, which constructor needs to be called.
Destructors in C++ are members functions in a class that delete an object. They are called when the class object goes out of scope such as when the function ends, the program ends, a delete variable is called etc.
Destructors are different from normal member functions as they don’t take any argument and don’t return anything. Also, destructors have the same name as their class and their name is preceded by a title(~).
A program that demonstrates destructors in C++ is given as follows.
Example
#include<iostream>
Using namespace std;
Class Demo {
Private:
Int num1, num2;
Public:
Demo(int n1, int n2) {
Cout<<"Inside Constructor"<<endl;
Num1 = n1;
Num2 = n2;
}
Void display() {
Cout<<"num1 = "<< num1 <<endl;
Cout<<"num2 = "<< num2 <<endl;
}
~Demo() {
Cout<<"Inside Destructor";
}
};
Int main() {
Demo obj1(10, 20);
Obj1.display();
Return 0;
}
Output
Inside Constructor
Num1 = 10
Num2 = 20
Inside Destructor
In the above program, the class Demo contains a parameterized constructor that initializes num1 and num2 with the values provided by n1 and n2. It also contains a function display() that prints the value of num1 and num2. There is also a destructor in Demo that is called when the scope of the class object is ended. The code snippet for this is given as follows.
Class Demo {
Private:
Int num1, num2;
Public:
Demo(int n1, int n2) {
Cout<<"Inside Constructor"<<endl;
Num1 = n1;
Num2 = n2;
}
Void display() {
Cout<<"num1 = "<< num1 <<endl;
Cout<<"num2 = "<< num2 <<endl;
}
~Demo() {
Cout<<"Inside Destructor";
}
};
The function main() contains the object definition for an object of class type Demo. Then the function display() is called. This is shown below.
Demo obj1(10, 20);
Obj1.display();
KEY TAKEAWAY
Destructors in C++ are members functions in a class that delete an object. They are called when the class object goes out of scope such as when the function ends, the program ends, a delete variable is called etc.
Destructors are different from normal member functions as they don’t take any argument and don’t return anything. Also, destructors have the same name as their class and their name is preceded by a tilde(~).
References:
1. E Balagurusamy, “Programming with C++”, Tata McGraw Hill, 3rd Edition.
2. Herbert Schildt, “The Complete Reference C++”, 4th Edition.
3. Robert Lafore, “Object Oriented Programming in C++”, Sams Publishing, 4th Edition.
4. Matt Weisfeld, “The Object-Oriented Thought Process”, Pearson Education.