Unit - 2
Looping Statements and Array
The looping can be defined as repeating the same process multiple times until a specific condition satisfies. There are three types of loops used in the C language.
Why use loops in C language?
The looping simplifies the complex problems into the easy ones. It enables us to alter the flow of the program so that instead of writing the same code again and again, we can repeat the same code for a finite number of times. For example, if we need to print the first 10 natural numbers then, instead of using the printf statement 10 times, we can print inside a loop which runs up to 10 iterations.
Advantage of loops in C
1) It provides code reusability.
2) Using loops, we do not need to write the same code again and again.
3) Using loops, we can traverse over the elements of data structures (array or linked lists).
Types of C Loops
There are three types of loops is given below:
- Do while
- While
- For
Key takeaway
The looping can be defined as repeating the same process multiple times until a specific condition satisfies. There are three types of loops used in the C language.
For loop
The for loop in C language is used to iterate the statements or a part of the program several times. It is frequently used to traverse the data structures like the array and linked list.
Syntax of for loop in C
The syntax of for loop in c language is given below:
- For(Expression 1; Expression 2; Expression 3){
- //code to be executed
- }
Flowchart of for loop in C
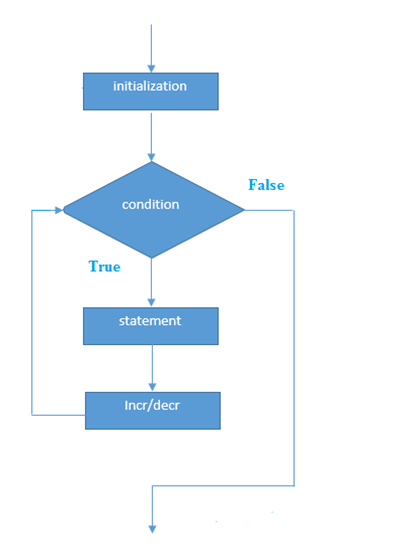
C for loop Examples
Let's see the simple program of for loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=0;
- For(i=1;i<=10;i++){
- Printf("%d \n",i);
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
C Program: Print table for the given number using C for loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- For(i=1;i<=10;i++){
- Printf("%d \n",(number*i));
- }
- Return 0;
- }
Output
Enter a number: 2
2
4
6
8
10
12
14
16
18
20
Enter a number: 1000
1000
2000
3000
4000
5000
6000
7000
8000
9000
10000
Properties of Expression 1
● The expression represents the initialization of the loop variable.
● We can initialize more than one variable in Expression 1.
● Expression 1 is optional.
● In C, we can not declare the variables in Expression 1. However, It can be an exception in some compilers.
Example 1
- #include <stdio.h>
- Int main()
- {
- Int a,b,c;
- For(a=0,b=12,c=23;a<2;a++)
- {
- Printf("%d ",a+b+c);
- }
- }
Output
35 36
Example 2
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- For(;i<5;i++)
- {
- Printf("%d ",i);
- }
- }
Output
1 2 3 4
Properties of Expression 2
● Expression 2 is a conditional expression. It checks for a specific condition to be satisfied. If it is not, the loop is terminated.
● Expression 2 can have more than one condition. However, the loop will iterate until the last condition becomes false. Other conditions will be treated as statements.
● Expression 2 is optional.
● Expression 2 can perform the task of expression 1 and expression 3. That is, we can initialize the variable as well as update the loop variable in expression 2 itself.
● We can pass zero or non-zero value in expression 2. However, in C, any non-zero value is true, and zero is false by default.
Example
- #include <stdio.h>
- Int main()
- {
- Int i;
- For(i=0;i<=4;i++)
- {
- Printf("%d ",i);
- }
- }
Output
0 1 2 3 4
Loop body
The braces {} are used to define the scope of the loop. However, if the loop contains only one statement, then we don't need to use braces. A loop without a body is possible. The braces work as a block separator, i.e., the value variable declared inside for loop is valid only for that block and not outside. Consider the following example.
- #include<stdio.h>
- Void main ()
- {
- Int i;
- For(i=0;i<10;i++)
- {
- Int i = 20;
- Printf("%d ",i);
- }
- }
Output
20 20 20 20 20 20 20 20 20 20
Infinitive for loop in C
To make a for loop infinite, we need not give any expression in the syntax. Instead of that, we need to provide two semicolons to validate the syntax of the for loop. This will work as an infinite for loop.
- #include<stdio.h>
- Void main ()
- {
- For(;;)
- {
- Printf("welcome to javatpoint");
- }
- }
While loop
While loop is also known as a pre-tested loop. In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition. It can be viewed as a repeating if statement. The while loop is mostly used in the case where the number of iterations is not known in advance.
Syntax of while loop in C language
The syntax of while loop in c language is given below:
- While(condition){
- //code to be executed
- }
Flowchart of while loop in C
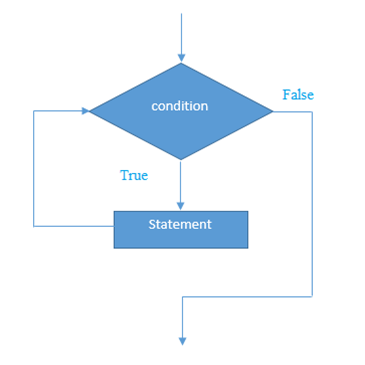
Example of the while loop in C language
Let's see the simple program of while loop that prints table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- While(i<=10){
- Printf("%d \n",i);
- i++;
- }
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using while loop in C
- #include<stdio.h>
- Int main(){
- Int i=1,number=0,b=9;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- While(i<=10){
- Printf("%d \n",(number*i));
- i++;
- }
- Return 0;
- }
Output
Enter a number: 50
50
100
150
200
250
300
350
400
450
500
Enter a number: 100
100
200
300
400
500
600
700
800
900
1000
Properties of while loop
● A conditional expression is used to check the condition. The statements defined inside the while loop will repeatedly execute until the given condition fails.
● The condition will be true if it returns 0. The condition will be false if it returns any non-zero number.
● In while loop, the condition expression is compulsory.
● Running a while loop without a body is possible.
● We can have more than one conditional expression in while loop.
● If the loop body contains only one statement, then the braces are optional.
Example 1
- #include<stdio.h>
- Void main ()
- {
- Int j = 1;
- While(j+=2,j<=10)
- {
- Printf("%d ",j);
- }
- Printf("%d",j);
- }
Output
3 5 7 9 11
Example 2
- #include<stdio.h>
- Void main ()
- {
- Int x = 10, y = 2;
- While(x+y-1)
- {
- Printf("%d %d",x--,y--);
- }
- }
Output
Infinite loop
Infinitive while loop in C
If the expression passed in while loop results in any non-zero value then the loop will run the infinite number of times.
- While(1){
- //statement
- }
Do while
The do while loop is a post tested loop. Using the do-while loop, we can repeat the execution of several parts of the statements. The do-while loop is mainly used in the case where we need to execute the loop at least once. The do-while loop is mostly used in menu-driven programs where the termination condition depends upon the end user.
Do while loop syntax
The syntax of the C language do-while loop is given below:
- Do{
- //code to be executed
- }while(condition);
Example 1
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Char c;
- Int choice,dummy;
- Do{
- Printf("\n1. Print Hello\n2. Print Javatpoint\n3. Exit\n");
- Scanf("%d",&choice);
- Switch(choice)
- {
- Case 1 :
- Printf("Hello");
- Break;
- Case 2:
- Printf("Javatpoint");
- Break;
- Case 3:
- Exit(0);
- Break;
- Default:
- Printf("please enter valid choice");
- }
- Printf("do you want to enter more?");
- Scanf("%d",&dummy);
- Scanf("%c",&c);
- }while(c=='y');
- }
Output
1. Print Hello
2. Print Javatpoint
3. Exit
1
Hello
Do you want to enter more?
y
1. Print Hello
2. Print Javatpoint
3. Exit
2
Javatpoint
Do you want to enter more?
n
Flowchart of do while loop
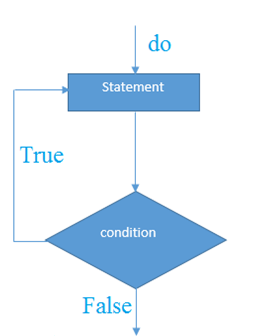
Do while example
There is given the simple program of c language do while loop where we are printing the table of 1.
- #include<stdio.h>
- Int main(){
- Int i=1;
- Do{
- Printf("%d \n",i);
- i++;
- }while(i<=10);
- Return 0;
- }
Output
1
2
3
4
5
6
7
8
9
10
Program to print table for the given number using do while loop
- #include<stdio.h>
- Int main(){
- Int i=1,number=0;
- Printf("Enter a number: ");
- Scanf("%d",&number);
- Do{
- Printf("%d \n",(number*i));
- i++;
- }while(i<=10);
- Return 0;
- }
Output
Enter a number: 5
5
10
15
20
25
30
35
40
45
50
Enter a number: 10
10
20
30
40
50
60
70
80
90
100
Infinitive do while loop
The do-while loop will run infinite times if we pass any non-zero value as the conditional expression.
- Do{
- //statement
- }while(1);
Nested Loops in C
C supports nesting of loops in C. Nesting of loops is the feature in C that allows the looping of statements inside another loop. Let's observe an example of nesting loops in C.
Any number of loops can be defined inside another loop, i.e., there is no restriction for defining any number of loops. The nesting level can be defined at n times. You can define any type of loop inside another loop; for example, you can define 'while' loop inside a 'for' loop.
Syntax of Nested loop
- Outer_loop
- {
- Inner_loop
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Outer_loop and Inner_loop are the valid loops that can be a 'for' loop, 'while' loop or 'do-while' loop.
Nested for loop
The nested for loop means any type of loop which is defined inside the 'for' loop.
- For (initialization; condition; update)
- {
- For(initialization; condition; update)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested for loop
- #include <stdio.h>
- Int main()
- {
- Int n;// variable declaration
- Printf("Enter the value of n :");
- // Displaying the n tables.
- For(int i=1;i<=n;i++) // outer loop
- {
- For(int j=1;j<=10;j++) // inner loop
- {
- Printf("%d\t",(i*j)); // printing the value.
- }
- Printf("\n");
- }
Output:
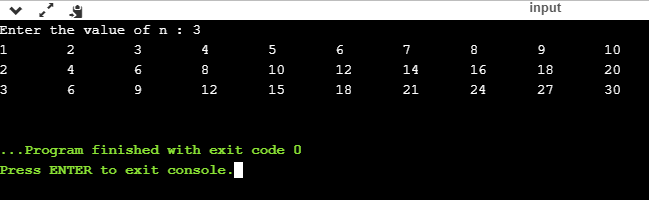
Nested while loop
The nested while loop means any type of loop which is defined inside the 'while' loop.
- While(condition)
- {
- While(condition)
- {
- // inner loop statements.
- }
- // outer loop statements.
- }
Example of nested while loop
- #include <stdio.h>
- Int main()
- {
- Int rows; // variable declaration
- Int columns; // variable declaration
- Int k=1; // variable initialization
- Printf("Enter the number of rows :"); // input the number of rows.
- Scanf("%d",&rows);
- Printf("\nEnter the number of columns :"); // input the number of columns.
- Scanf("%d",&columns);
- Int a[rows][columns]; //2d array declaration
- Int i=1;
- While(i<=rows) // outer loop
- {
- Int j=1;
- While(j<=columns) // inner loop
- {
- Printf("%d\t",k); // printing the value of k.
- k++; // increment counter
- j++;
- }
- i++;
- Printf("\n");
- }
- }
Output:
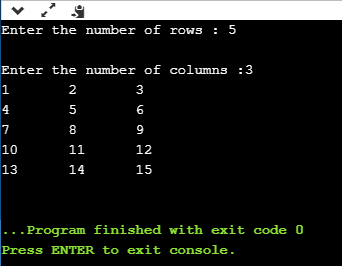
Nested do..while loop
The nested do..while loop means any type of loop which is defined inside the 'do..while' loop.
- Do
- {
- Do
- {
- // inner loop statements.
- }while(condition);
- // outer loop statements.
- }while(condition);
Example of nested do..while loop.
- #include <stdio.h>
- Int main()
- {
- /*printing the pattern
- ********
- ********
- ********
- ******** */
- Int i=1;
- Do // outer loop
- {
- Int j=1;
- Do // inner loop
- {
- Printf("*");
- j++;
- }while(j<=8);
- Printf("\n");
- i++;
- }while(i<=4);
- }
Output:
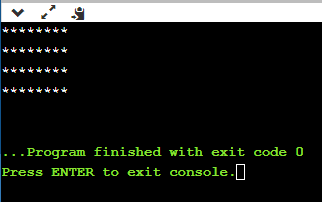
Key takeaway
The for loop in C language is used to iterate the statements or a part of the program several times.
In general, a while loop allows a part of the code to be executed multiple times depending upon a given boolean condition.
The do-while loop is mainly used in the case where we need to execute the loop at least once.
Difference between while and do while loop
While | Do While |
It initially checks the condition before executing the statement. | This loop will run the statement(s) at least once before checking the condition. |
Before beginning the body of a loop, the while loop allows for the initialization of counter variables. | The do while loop allows you to set counter variables before and after the loop starts. |
It's a loop with an entry control. | It's a loop with a controlled exit. |
A semicolon is not required at the end of a while condition. | At the end of the while condition, we need to add a semicolon. |
In the case of a single statement, brackets are required. | Brackets are required at all times. |
The condition is given at the start of the loop in this loop. | After the block has been executed, the loop condition is defined. |
If the condition is false, the statement can be executed zero times. | The statement is run at least once. |
Syntax: While (condition) { Statements; // loop body } | Syntax: Do{ Statements; //loop body } while (condition); |
Break statement
The break is a keyword in C which is used to bring the program control out of the loop. The break statement is used inside loops or switch statement. The break statement breaks the loop one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops. The break statement in C can be used in the following two scenarios:
- With switch case
- With loop
Syntax:
- //loop or switch case
- Break;
Flowchart of break in c
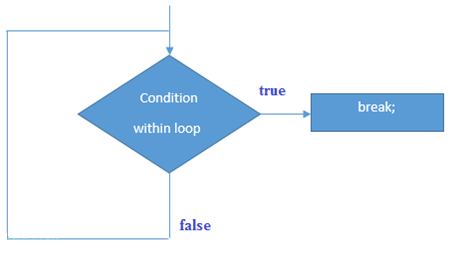
Example
- #include<stdio.h>
- #include<stdlib.h>
- Void main ()
- {
- Int i;
- For(i = 0; i<10; i++)
- {
- Printf("%d ",i);
- If(i == 5)
- Break;
- }
- Printf("came outside of loop i = %d",i);
- }
Output
0 1 2 3 4 5 came outside of loop i = 5
Example of C break statement with switch case
C break statement with the nested loop
In such case, it breaks only the inner loop, but not outer loop.
- #include<stdio.h>
- Int main(){
- Int i=1,j=1;//initializing a local variable
- For(i=1;i<=3;i++){
- For(j=1;j<=3;j++){
- Printf("%d &d\n",i,j);
- If(i==2 && j==2){
- Break;//will break loop of j only
- }
- }//end of for loop
- Return 0;
- }
Output
1 1
1 2
1 3
2 1
2 2
3 1
3 2
3 3
As you can see the output on the console, 2 3 is not printed because there is a break statement after printing i==2 and j==2. But 3 1, 3 2 and 3 3 are printed because the break statement is used to break the inner loop only.
Break statement with while loop
Consider the following example to use break statement inside while loop.
- #include<stdio.h>
- Void main ()
- {
- Int i = 0;
- While(1)
- {
- Printf("%d ",i);
- i++;
- If(i == 10)
- Break;
- }
- Printf("came out of while loop");
- }
Output
0 1 2 3 4 5 6 7 8 9 came out of while loop
Break statement with do-while loop
Consider the following example to use the break statement with a do-while loop.
- #include<stdio.h>
- Void main ()
- {
- Int n=2,i,choice;
- Do
- {
- i=1;
- While(i<=10)
- {
- Printf("%d X %d = %d\n",n,i,n*i);
- i++;
- }
- Printf("do you want to continue with the table of %d , enter any non-zero value to continue.",n+1);
- Scanf("%d",&choice);
- If(choice == 0)
- {
- Break;
- }
- n++;
- }while(1);
- }
Output
2 X 1 = 2
2 X 2 = 4
2 X 3 = 6
2 X 4 = 8
2 X 5 = 10
2 X 6 = 12
2 X 7 = 14
2 X 8 = 16
2 X 9 = 18
2 X 10 = 20
Do you want to continue with the table of 3 , enter any non-zero value to continue.1
3 X 1 = 3
3 X 2 = 6
3 X 3 = 9
3 X 4 = 12
3 X 5 = 15
3 X 6 = 18
3 X 7 = 21
3 X 8 = 24
3 X 9 = 27
3 X 10 = 30
Do you want to continue with the table of 4 , enter any non-zero value to continue.0
Continue statement
The continue statement in C language is used to bring the program control to the beginning of the loop. The continue statement skips some lines of code inside the loop and continues with the next iteration. It is mainly used for a condition so that we can skip some code for a particular condition.
Syntax:
- //loop statements
- Continue;
- //some lines of the code which is to be skipped
Continue statement example 1
- #include<stdio.h>
- Void main ()
- {
- Int i = 0;
- While(i!=10)
- {
- Printf("%d", i);
- Continue;
- i++;
- }
- }
Output
Infinite loop
Key takeaway
The break statement breaks the loop one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops.
The continue statement skips some lines of code inside the loop and continues with the next iteration.
An infinite loop is a looping construct that does not terminate the loop and executes the loop forever. It is also called an indefinite loop or an endless loop. It either produces a continuous output or no output.
When to use an infinite loop
An infinite loop is useful for those applications that accept the user input and generate the output continuously until the user exits from the application manually. In the following situations, this type of loop can be used:
● All the operating systems run in an infinite loop as it does not exist after performing some task. It comes out of an infinite loop only when the user manually shuts down the system.
● All the servers run in an infinite loop as the server responds to all the client requests. It comes out of an indefinite loop only when the administrator shuts down the server manually.
● All the games also run in an infinite loop. The game will accept the user requests until the user exits from the game.
We can create an infinite loop through various loop structures. The following are the loop structures through which we will define the infinite loop:
● For loop
● While loop
● Do-while loop
● Go to statement
● C macros
For loop
Let's see the infinite 'for' loop. The following is the definition for the infinite for loop:
- For(; ;)
- {
- // body of the for loop.
- }
As we know that all the parts of the 'for' loop are optional, and in the above for loop, we have not mentioned any condition; so, this loop will execute infinite times.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- For(;;)
- {
- Printf("Hello javatpoint");
- }
- Return 0;
- }
In the above code, we run the 'for' loop infinite times, so "Hello javatpoint" will be displayed infinitely.
Output
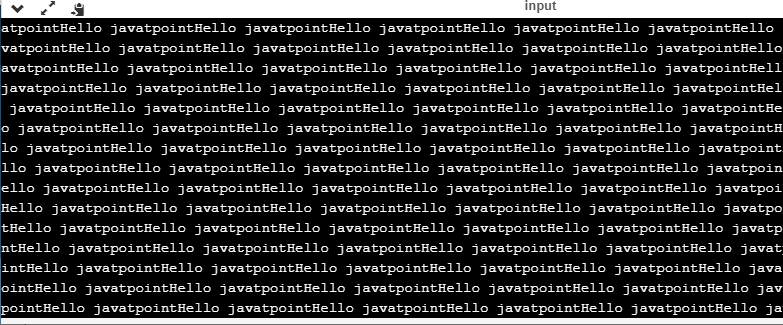
While loop
Now, we will see how to create an infinite loop using a while loop. The following is the definition for the infinite while loop:
- While(1)
- {
- // body of the loop..
- }
In the above while loop, we put '1' inside the loop condition. As we know that any non-zero integer represents the true condition while '0' represents the false condition.
Let's look at a simple example.
- #include <stdio.h>
- Int main()
- {
- Int i=0;
- While(1)
- {
- i++;
- Printf("i is :%d",i);
- }
- Return 0;
- }
In the above code, we have defined a while loop, which runs infinite times as it does not contain any condition. The value of 'i' will be updated an infinite number of times.
Output
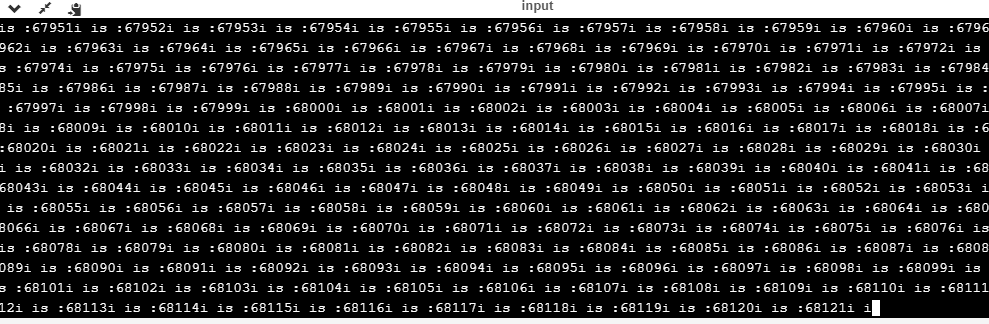
Do..while loop
The do..while loop can also be used to create the infinite loop. The following is the syntax to create the infinite do..while loop.
- Do
- {
- // body of the loop..
- }while(1);
The above do..while loop represents the infinite condition as we provide the '1' value inside the loop condition. As we already know that non-zero integer represents the true condition, so this loop will run infinite times.
Goto statement
We can also use the goto statement to define the infinite loop.
- Infinite_loop;
- // body statements.
- Goto infinite_loop;
In the above code, the goto statement transfers the control to the infinite loop.
Macros
We can also create the infinite loop with the help of a macro constant. Let's understand through an example.
- #include <stdio.h>
- #define infinite for(;;)
- Int main()
- {
- Infinite
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined a macro named as 'infinite', and its value is 'for(;;)'. Whenever the word 'infinite' comes in a program then it will be replaced with a 'for(;;)'.
Output
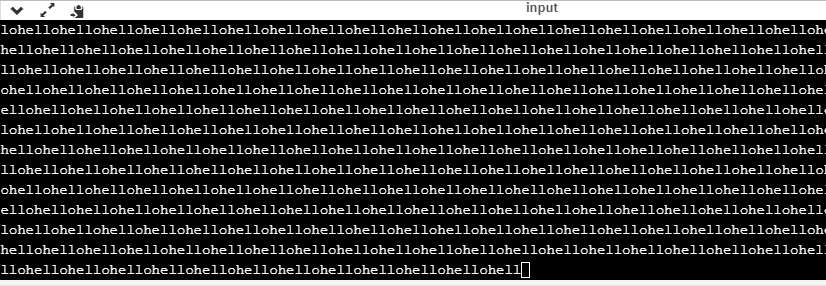
Till now, we have seen various ways to define an infinite loop. However, we need some approach to come out of the infinite loop. In order to come out of the infinite loop, we can use the break statement.
Let's understand through an example.
- #include <stdio.h>
- Int main()
- {
- Char ch;
- While(1)
- {
- Ch=getchar();
- If(ch=='n')
- {
- Break;
- }
- Printf("hello");
- }
- Return 0;
- }
In the above code, we have defined the while loop, which will execute an infinite number of times until we press the key 'n'. We have added the 'if' statement inside the while loop. The 'if' statement contains the break keyword, and the break keyword brings control out of the loop.
Unintentional infinite loops
Sometimes the situation arises where unintentional infinite loops occur due to the bug in the code. If we are the beginners, then it becomes very difficult to trace them. Below are some measures to trace an unintentional infinite loop:
● We should examine the semicolons carefully. Sometimes we put the semicolon at the wrong place, which leads to the infinite loop.
- #include <stdio.h>
- Int main()
- {
- Int i=1;
- While(i<=10);
- {
- Printf("%d", i);
- i++;
- }
- Return 0;
- }
In the above code, we put the semicolon after the condition of the while loop which leads to the infinite loop. Due to this semicolon, the internal body of the while loop will not execute.
● We should check the logical conditions carefully. Sometimes by mistake, we place the assignment operator (=) instead of a relational operator (= =).
- #include <stdio.h>
- Int main()
- {
- Char ch='n';
- While(ch='y')
- {
- Printf("hello");
- }
- Return 0;
- }
In the above code, we use the assignment operator (ch='y') which leads to the execution of loop infinite number of times.
● We use the wrong loop condition which causes the loop to be executed indefinitely.
- #include <stdio.h>
- Int main()
- {
- For(int i=1;i>=1;i++)
- {
- Printf("hello");
- }
- Return 0;
- }
The above code will execute the 'for loop' infinite number of times. As we put the condition (i>=1), which will always be true for every condition, it means that "hello" will be printed infinitely.
● We should be careful when we are using the break keyword in the nested loop because it will terminate the execution of the nearest loop, not the entire loop.
- #include <stdio.h>
- Int main()
- {
- While(1)
- {
- For(int i=1;i<=10;i++)
- {
- If(i%2==0)
- {
- Break;
- }
- }
- }
- Return 0;
- }
In the above code, the while loop will be executed an infinite number of times as we use the break keyword in an inner loop. This break keyword will bring the control out of the inner loop, not from the outer loop.
● We should be very careful when we are using the floating-point value inside the loop as we cannot underestimate the floating-point errors.
- #include <stdio.h>
- Int main()
- {
- Float x = 3.0;
- While (x != 4.0) {
- Printf("x = %f\n", x);
- x += 0.1;
- }
- Return 0;
- }
In the above code, the loop will run infinite times as the computer represents a floating-point value as a real value. The computer will represent the value of 4.0 as 3.999999 or 4.000001, so the condition (x !=4.0) will never be false. The solution to this problem is to write the condition as (k<=4.0).
Key takeaway
An infinite loop is a looping construct that does not terminate the loop and executes the loop forever. It is also called an indefinite loop or an endless loop. It either produces a continuous output or no output.
An array is defined as the collection of similar type of data items stored at contiguous memory locations. Arrays are the derived data type in C programming language which can store the primitive type of data such as int, char, double, float, etc. It also has the capability to store the collection of derived data types, such as pointers, structure, etc. The array is the simplest data structure where each data element can be randomly accessed by using its index number.
C array is beneficial if you have to store similar elements. For example, if we want to store the marks of a student in 6 subjects, then we don't need to define different variables for the marks in the different subject. Instead of that, we can define an array which can store the marks in each subject at the contiguous memory locations.
By using the array, we can access the elements easily. Only a few lines of code are required to access the elements of the array.
Properties of Array
The array contains the following properties.
● Each element of an array is of same data type and carries the same size, i.e., int = 4 bytes.
● Elements of the array are stored at contiguous memory locations where the first element is stored at the smallest memory location.
● Elements of the array can be randomly accessed since we can calculate the address of each element of the array with the given base address and the size of the data element.
Advantage of C Array
1) Code Optimization: Less code to the access the data.
2) Ease of traversing: By using the for loop, we can retrieve the elements of an array easily.
3) Ease of sorting: To sort the elements of the array, we need a few lines of code only.
4) Random Access: We can access any element randomly using the array.
Disadvantage of C Array
1) Fixed Size: Whatever size, we define at the time of declaration of the array, we can't exceed the limit. So, it doesn't grow the size dynamically like LinkedList which we will learn later.
Declaration of C Array
We can declare an array in the c language in the following way.
Data_type array_name[array_size];
Now, let us see the example to declare the array.
Int marks[5];
Here, int is the data_type, marks are the array_name, and 5 is the array_size.
Key takeaway
An array is defined as the collection of similar type of data items stored at contiguous memory locations. Arrays are the derived data type in C programming language which can store the primitive type of data such as int, char, double, float, etc.
● A variable that can store a fixed-size collection of elements of the same data type is called an array.
● You can randomly access the elements of an array. You can also determine the address of each array element.
● The array's elements are stored in contiguous memory regions.
● The pointer points to the first memory block in the array name's memory block.
● An array can be an integer, character, or float data type that is only initialised once during the definition process.
● An array's individual elements can be changed without affecting the rest of the array.
● The index number can be used to distinguish all elements in an array.
The simplest way to initialize an array is by using the index of each element. We can initialize each element of the array by using the index. Consider the following example.
- Marks[0]=80;//initialization of array
- Marks[1]=60;
- Marks[2]=70;
- Marks[3]=85;
- Marks[4]=75;
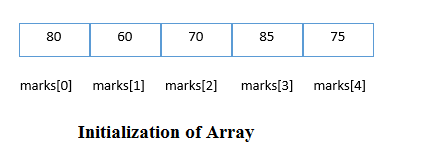
C Array: Declaration with Initialization
We can initialize the c array at the time of declaration. Let's see the code.
- Int marks[5]={20,30,40,50,60};
In such case, there is no requirement to define the size. So it may also be written as the following code.
- Int marks[]={20,30,40,50,60};
Let's see the C program to declare and initialize the array in C.
- #include<stdio.h>
- Int main(){
- Int i=0;
- Int marks[5]={20,30,40,50,60};//declaration and initialization of array
- //traversal of array
- For(i=0;i<5;i++){
- Printf("%d \n",marks[i]);
- }
- Return 0;
- }
Output
20
30
40
50
60
C Array Example: Sorting an array
In the following program, we are using bubble sort method to sort the array in ascending order.
- #include<stdio.h>
- Void main ()
- {
- Int i, j,temp;
- Int a[10] = { 10, 9, 7, 101, 23, 44, 12, 78, 34, 23};
- For(i = 0; i<10; i++)
- {
- For(j = i+1; j<10; j++)
- {
- If(a[j] > a[i])
- {
- Temp = a[i];
- a[i] = a[j];
- a[j] = temp;
- }
- }
- }
- Printf("Printing Sorted Element List ...\n");
- For(i = 0; i<10; i++)
- {
- Printf("%d\n",a[i]);
- }
- }
Program to print the largest and second largest element of the array.
- #include<stdio.h>
- Void main ()
- {
- Int arr[100],i,n,largest,sec_largest;
- Printf("Enter the size of the array?");
- Scanf("%d",&n);
- Printf("Enter the elements of the array?");
- For(i = 0; i<n; i++)
- {
- Scanf("%d",&arr[i]);
- }
- Largest = arr[0];
- Sec_largest = arr[1];
- For(i=0;i<n;i++)
- {
- If(arr[i]>largest)
- {
- Sec_largest = largest;
- Largest = arr[i];
- }
- Else if (arr[i]>sec_largest && arr[i]!=largest)
- {
- Sec_largest=arr[i];
- }
- }
- Printf("largest = %d, second largest = %d",largest,sec_largest);
- }
Arrays are represented on the computer by diagrams that show how much memory they consume. The square boxes that represent every bit of the memory symbolise this. The value of the element can be written inside the box. The memory representation in an array is depicted in the diagram below. Each box reflects the amount of RAM required to store one element array. The memory addresses of the other data are stored in the pointers. A black disc with an arrow pointing to the referring data represents them.
Let's write some code and represent it in memory.
Int a [5];
. . . . .
a[0] =1;
For (int i = 1; i< 5; i++)
{
a[i] = a[i-1]* 2;
}
'a' is the memory pointer for all of the elements in the example above. There is now a data bit allocation in the computer memory that looks like this:
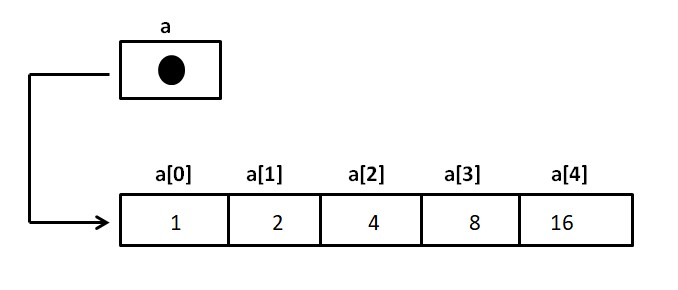
A one-dimensional array is a group of elements having the same datatype and same name. Individual elements are referred to using common name and unique index of the elements.
The simplest form of an array is one-dimensional-array. The array itself is given name and its elements are referred to by their subscripts. In , an array is denoted as follows:
Array_name[array_size]
Where size specifies the number of elements in the array and the subscript (also called index) value ranges from 0 through size-1.
Declare One Dimensional Array
Here is the general form to declare one dimensional array in
Data_typearray_name[array_size];
Here, data_type is any valid data type, array_name is the name of the array, and array_size is the size of array. Here is an example, declaring an array named arr of int type, having maximum element size of 10 elements
Intarr[10];
Initialize One Dimensional Array
Here is the general form to initialize values to one dimensional array
Data_typearray_name[array_size] = {comma_separated_element_list};
Here is an example, declaring and initializing values to the array name arr of type int, containing 10 elements
Intarr[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
One Dimensional Array Example
Here are some example program, demonstrating one dimensional array
/*One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[5] = {1, 2, 3, 4, 5};
Inti;
For(i=0; i<5; i++)
{
Cout<<"arr["<<i<<"] = "<<arr[i]<<"\n";
}
Getch();
}
Here is the sample output of this program:
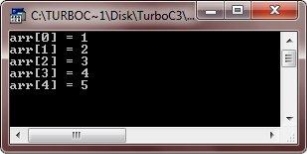
Here is another example, also demonstrating one dimension array in
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[10];
Inti;
Int sum=0, avg=0;
Cout<<"Enter 10 array elements: ";
For(i=0; i<10; i++)
{
Cin>>arr[i];
Sum = sum + arr[i];
}
Cout<<"\nThe array elements are: \n";
For(i=0; i<10; i++)
{
Cout<<arr[i]<<" ";
}
Cout<<"\n\nSum of all elements is: "<<sum;
Avg = sum/10;
Cout<<"\nAnd average is: "<<avg;
Getch();
}
Here is the sample run of the above program:
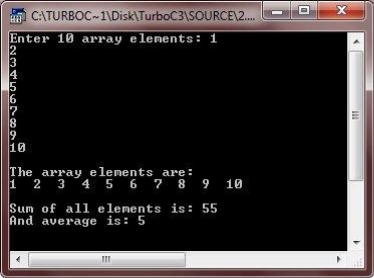
Below is another program on one-dimensional array in
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[5];
Inti, position, index;
Cout<<"Enter 5 array elements: ";
For(i=0; i<5; i++)
{
Cin>>arr[i];
}
Cout<<"\nIndex\t\tPosition";
For(i=0; i<5; i++)
{
Cout<<"\n";
Cout<<i<<" = "<<arr[i]<<"\t\t"<<i+1<<" = "<<arr[i+1];
}
Cout<<" (Garbage value/not of array)";
Cout<<"\n\nImportant - Array element can only be accessed by indexing the array\n";
Cout<<"Note - Array index always starts from 0";
Getch();
}
Below is the sample run of this program:
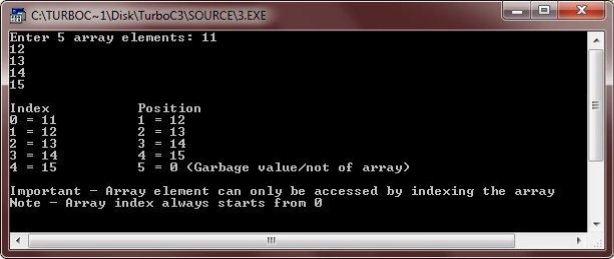
Let's take one more program, on single or one dimensional array
/* One Dimensional Array */
#include<iostream.h>
#include<conio.h>
Void main()
{
Clrscr();
Intarr[10];
Inti, position, index;
Cout<<"Enter 10 array elements: ";
For(i=0; i<10; i++)
{
Cin>>arr[i];
}
Cout<<"Accessing element at position...Enter position...";
Cin>>position;
Cout<<"\nElement present at position "<<position<<" is "<<arr[position+1];
Cout<<"\n\nAccessing element at index..Enter index..";
Cin>>index;
Cout<<"\nElement present at index "<<index<<" is "<<arr[index];
Getch();
}
Here is the sample run of the above program:
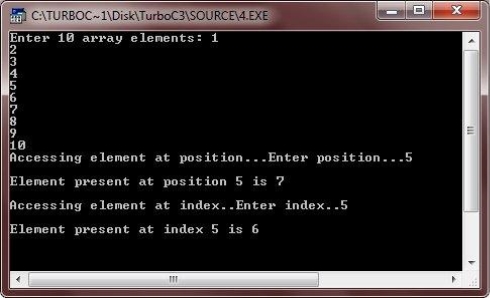
Key takeaway
A one-dimensional array is a group of elements having the same datatype and same name.
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns. However, 2D arrays are created to implement a relational database lookalike data structure. It provides ease of holding the bulk of data at once which can be passed to any number of functions wherever required.
Declaration of two dimensional Array in C
The syntax to declare the 2D array is given below.
Data_type array_name[rows][columns];
Consider the following example.
Int twodimen[4][3];
Here, 4 is the number of rows, and 3 is the number of columns.
Initialization of 2D Array in C
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array. The two-dimensional array can be declared and defined in the following way.
Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
Two-dimensional array example in C
- #include<stdio.h>
- Int main(){
- Int i=0,j=0;
- Int arr[4][3]={{1,2,3},{2,3,4},{3,4,5},{4,5,6}};
- //traversing 2D array
- For(i=0;i<4;i++){
- For(j=0;j<3;j++){
- Printf("arr[%d] [%d] = %d \n",i,j,arr[i][j]);
- }//end of j
- }//end of i
- Return 0;
- }
Output
Arr[0][0] = 1
Arr[0][1] = 2
Arr[0][2] = 3
Arr[1][0] = 2
Arr[1][1] = 3
Arr[1][2] = 4
Arr[2][0] = 3
Arr[2][1] = 4
Arr[2][2] = 5
Arr[3][0] = 4
Arr[3][1] = 5
Arr[3][2] = 6
C 2D array example: Storing elements in a matrix and printing it.
- #include <stdio.h>
- Void main ()
- {
- Int arr[3][3],i,j;
- For (i=0;i<3;i++)
- {
- For (j=0;j<3;j++)
- {
- Printf("Enter a[%d][%d]: ",i,j);
- Scanf("%d",&arr[i][j]);
- }
- }
- Printf("\n printing the elements ....\n");
- For(i=0;i<3;i++)
- {
- Printf("\n");
- For (j=0;j<3;j++)
- {
- Printf("%d\t",arr[i][j]);
- }
- }
- }
Output
Enter a[0][0]: 56
Enter a[0][1]: 10
Enter a[0][2]: 30
Enter a[1][0]: 34
Enter a[1][1]: 21
Enter a[1][2]: 34
Enter a[2][0]: 45
Enter a[2][1]: 56
Enter a[2][2]: 78
Printing the elements ....
56 10 30
34 21 34
45 56 78
Key takeaway
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns.
Strings are actually a one-dimensional array of characters terminated by a null character '\0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word "Hello". To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word "Hello."
Char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
If you follow the rule of array initialization then you can write the above statement as follows −
Char greeting[] = "Hello";
Following is the memory presentation of the above defined string in C/C++ −
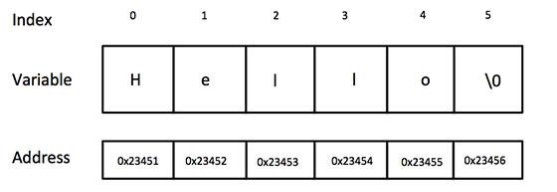
Actually, you do not place the null character at the end of a string constant. The C compiler automatically places the '\0' at the end of the string when it initializes the array. Let us try to print the above mentioned string −
#include <stdio.h>
Int main () {
Char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
Printf("Greeting message: %s\n", greeting );
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Greeting message: Hello
C supports a wide range of functions that manipulate null-terminated strings −
Sr.No. | Function & Purpose |
1 | Strcpy(s1, s2); Copies string s2 into string s1. |
2 | Strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | Strlen(s1); Returns the length of string s1. |
4 | Strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | Strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | Strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
The following example uses some of the above-mentioned functions −
#include <stdio.h>
#include <string.h>
Int main () {
Char str1[12] = "Hello";
Char str2[12] = "World";
Char str3[12];
Int len;
/* copy str1 into str3 */
Strcpy(str3, str1);
Printf("strcpy( str3, str1) : %s\n", str3 );
/* concatenates str1 and str2 */
Strcat( str1, str2);
Printf("strcat( str1, str2): %s\n", str1 );
/* total lenghth of str1 after concatenation */
Len = strlen(str1);
Printf("strlen(str1) : %d\n", len );
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Strcpy( str3, str1) : Hello
Strcat( str1, str2): HelloWorld
Strlen(str1) : 10
The string.h header defines one variable type, one macro, and various functions for manipulating arrays of characters.
Library Variables
Following is the variable type defined in the header string.h −
Sr.No. | Variable & Description |
1 | Size_t This is the unsigned integral type and is the result of the sizeof keyword. |
Library Macros
Following is the macro defined in the header string.h −
Sr.No. | Macro & Description |
1 | NULL This macro is the value of a null pointer constant. |
Library Functions
Following are the functions defined in the header string.h −
Sr.No. | Function & Description |
1 | Void *memchr(const void *str, int c, size_t n) Searches for the first occurrence of the character c (an unsigned char) in the first n bytes of the string pointed to, by the argument str. |
2 | Int memcmp(const void *str1, const void *str2, size_t n) Compares the first n bytes of str1 and str2. |
3 | Void *memcpy(void *dest, const void *src, size_t n) Copies n characters from src to dest. |
4 | Void *memmove(void *dest, const void *src, size_t n) Another function to copy n characters from str2 to str1. |
5 | Void *memset(void *str, int c, size_t n) Copies the character c (an unsigned char) to the first n characters of the string pointed to, by the argument str. |
6 | Char *strcat(char *dest, const char *src) Appends the string pointed to, by src to the end of the string pointed to by dest. |
7 | Char *strncat(char *dest, const char *src, size_t n) Appends the string pointed to, by src to the end of the string pointed to, by dest up to n characters long. |
8 | Char *strchr(const char *str, int c) Searches for the first occurrence of the character c (an unsigned char) in the string pointed to, by the argument str. |
9 | Int strcmp(const char *str1, const char *str2) Compares the string pointed to, by str1 to the string pointed to by str2. |
10 | Int strncmp(const char *str1, const char *str2, size_t n) Compares at most the first n bytes of str1 and str2. |
11 | Int strcoll(const char *str1, const char *str2) Compares string str1 to str2. The result is dependent on the LC_COLLATE setting of the location. |
12 | Char *strcpy(char *dest, const char *src) Copies the string pointed to, by src to dest. |
13 | Char *strncpy(char *dest, const char *src, size_t n) Copies up to n characters from the string pointed to, by src to dest. |
14 | Size_t strcspn(const char *str1, const char *str2) Calculates the length of the initial segment of str1 which consists entirely of characters not in str2. |
15 | Char *strerror(int errnum) Searches an internal array for the error number errnum and returns a pointer to an error message string. |
16 | Size_t strlen(const char *str) Computes the length of the string str up to but not including the terminating null character. |
17 | Char *strpbrk(const char *str1, const char *str2) Finds the first character in the string str1 that matches any character specified in str2. |
18 | Char *strrchr(const char *str, int c) Searches for the last occurrence of the character c (an unsigned char) in the string pointed to by the argument str. |
19 | Size_t strspn(const char *str1, const char *str2) Calculates the length of the initial segment of str1 which consists entirely of characters in str2. |
20 | Char *strstr(const char *haystack, const char *needle) Finds the first occurrence of the entire string needle (not including the terminating null character) which appears in the string haystack. |
21 | Char *strtok(char *str, const char *delim) Breaks string str into a series of tokens separated by delim. |
22 | Size_t strxfrm(char *dest, const char *src, size_t n) Transforms the first n characters of the string src into current locale and places them in the string dest. |
Key takeaway
Strings are actually a one-dimensional array of characters terminated by a null character '\0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
References:
- The C Programming Language - By Brian W Kernighan and Dennis Ritchie
- C programming in an open source paradigm:– By R. K. Kamat, K . S. Oza, S.R. Patil
- The GNU C Programming Tutorial ‐By Mark Burgess
- Let us C- By Yashwant Kanetkar