Unit - 2
Structures, Union and File Handling
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
● Ordinary variables can hold one piece of information
● Arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
● Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
● Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
● Struct keyword is used to declare structure.
● Members of structure are enclosed within opening and closing braces.
● Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
● Declaration of Structure reserves no space.
● It is nothing but the “ Template / Map / Shape ” of the structure .
● Memory is created , very first time when the variable is created / Instance is created.
Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1, e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Structure Initialization
1. When we declare a structure, memory is not allocated for uninitialized variable.
2. Let us discuss very familiar example of structure student, we can initialize structure variable in different ways –
Way 1: Declare and Initialize
Struct student
{
Char name[20];
Int roll;
Float marks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2: Declaring and Initializing Multiple Variables
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3: Initializing Single member
Struct student
{
Int mark1;
Int mark2;
Int mark3;
} sub1={67};
Though there are three members of structure, only one is initialized , Then remaining two members are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4: Initializing inside main
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
Void main()
{
Struct student s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below declaration statement will never allocate memory
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
We need to initialize structure variable to allocate some memory to the structure. Struct student s1 = {89,54,65};
Accessing Structure Elements
● Array elements are accessed using the Subscript variable, Similarly Structure members are accessed using dot [.] operator.
● (.) is called as “Structure member Operator”.
● Use this Operator in between “Structure variable” & “member name”
Struct employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Struct employee e= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
Printf(“%f”, e. Salary);
}
Key takeaway
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit.
The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address. Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Array of structure
In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
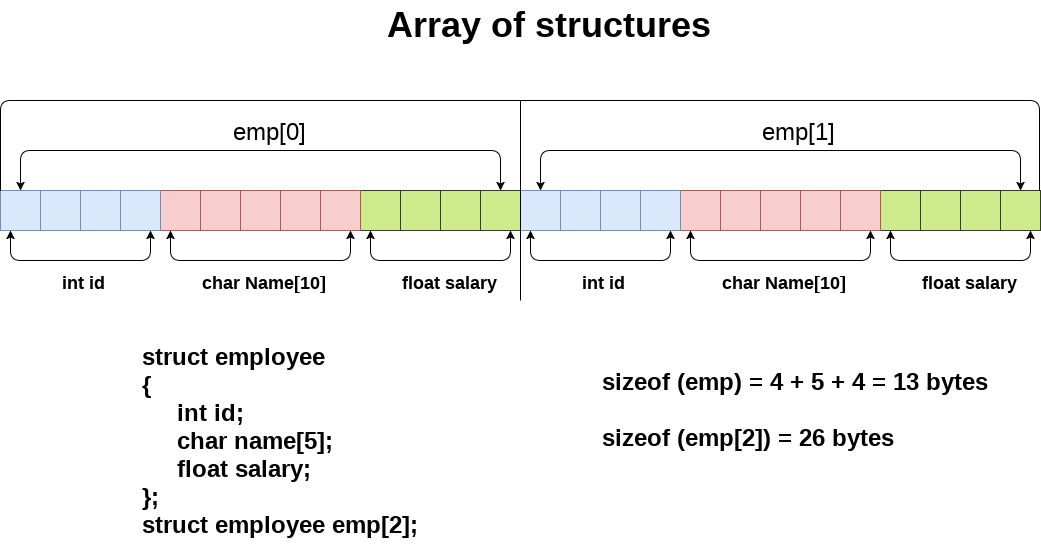
Fig: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Structure pointer
The structure pointer points to the memory block address where the Structure is stored. Like a pointer that points to another variable in memory of any data type (int, char, float). We utilise a structure pointer to tell the address of a structure in memory by referring the pointer variable ptr to the structure variable.
Declare a Structure Pointer
A structure pointer's declaration is comparable to that of a structure variable's declaration. As a result, the structure pointer and variable can be declared both inside and outside of the main() method. In C, we use the asterisk (*) symbol before the variable name to declare a pointer variable.
Struct structure_name *ptr;
Following the definition of the structure pointer, we must initialise it, as seen in the code:
Initialization of the Structure Pointer
Ptr = &structure_variable;
We can also immediately initialise a Structure Pointer when declaring a pointer.
Struct structure_name *ptr = &structure_variable;
As can be seen, a pointer ptr points to the Structure's structure variable location.
Access Structure member using pointer:
The Structure pointer can be used in two ways to access the structure's members:
● Using ( * ) asterisk or indirection operator and dot ( . ) operator.
● Using arrow ( -> ) operator or membership operator.
Program to access the structure member using structure pointer and the dot operator
Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
We constructed the Subject structure in the preceding programme, which has data components such as sub name (char), sub id (int), sub duration (char), and sub type (char) (char). The structure variable is sub, and the structure pointer variable is ptr, which points to the address of the sub variable, as in ptr = &sub. Each *ptr now has access to the address of a member of the Subject structure.
Program to access the structure member using structure pointer and arrow (->) operator
Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
In the preceding programme, we constructed an Employee structure with the pointer variables *ptr1 and *ptr2 and two structure variables emp1 and emp2. The members of the structure Employee are name, id, age, gender, and city. The pointer variable and arrow operator, which determine their memory space, are used by all Employee structure members to take their respective values from the user one by one.
Key takeaway
The feature of nesting one structure within another structure in C allows us to design complicated data types.
An array of structures is a collection of many structure variables, each of which contains information on a different entity.
The structure pointer points to the memory block address where the Structure is stored.
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function. Consider the following example, which uses the structural variable employee to call the function display(), which displays an employee's data.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void display(struct employee);
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
display(emp);
}
Void display(struct employee emp)
{
printf("Printing the details....\n");
printf("%s %s %d %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Self- referential structure
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
The structures pointing to the same type of structures are self-referential in nature.
Struct node {
intdata1;
chardata2;
struct node* link;
};
Int main()
{
struct node ob;
return0;
}
In the above example ‘link’ is a pointer to a structure of type ‘node’. Hence, the structure ‘node’ is a self-referential structure with ‘link’ as the referencing pointer.
An important point to consider is that the pointer should be initialized properly before accessing, as by default it contains garbage value.
Key takeaway
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function.
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
When operand is a data type:
#include <stdio.h>
Int main()
{
int x=89; // variable declaration.
printf("size of the variable x is %d", sizeof(x)); // Displaying the size of ?x? variable.
printf("\nsize of the integer data type is %d",sizeof(int)); //Displaying the size of integer data type.
printf("\nsize of the character data type is %d",sizeof(char)); //Displaying the size of character data type.
printf("\nsize of the floating data type is %d",sizeof(float)); //Displaying the size of floating data type.
Return 0;
}
The sizeof() operator is used in the preceding code to print the size of various data types such as int, char, and float.
Output
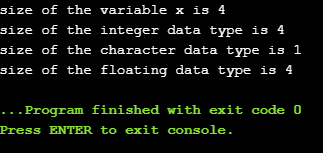
When operand is an expression
#include <stdio.h>
Int main()
{
double i=78.0; //variable initialization.
float j=6.78; //variable initialization.
printf("size of (i+j) expression is : %d",sizeof(i+j)); //Displaying the size of the expression (i+j).
return 0;
}
We created two variables, I and 'j,' of type double and float, respectively, in the following code, and then we used the sizeof(i+j) operator to print the size of the statement.
Output
Size of (i+j) expression is : 8
Typedef keyword
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Key takeaway
The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis.
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme.
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Accessing members of a union
- Array elements are accessed using the Subscript variable, Similarly Union members are accessed using dot [.] operator.
- (.) is called as “union member Operator”.
- Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P-
Garbage value, ABC
Key takeaway
Unions are quite similar to the structures in C. Union is also a derived type as structure.
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Most of the programs are written to store the information fetched from the program. One such way is to store the fetched information in a file. Different operations that can be performed on a file are:
- Creation of a new file (fopen with attributes as “a” or “a+” or “w” or “w++”)
- Opening an existing file (fopen)
- Reading from file (fscanf or fgets)
- Writing to a file (fprintf or fputs)
- Moving to a specific location in a file (fseek, rewind)
- Closing a file (fclose)
The text in the brackets denotes the functions used for performing those operations.
Functions in File Operations:
File opening modes in C:
“r” – Searches file. If the file is opened successfully, fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file cannot be opened, fopen( ) returns NULL.
“w” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“a” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer that points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“r+” – Searches file. If is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. Returns NULL, if unable to open the file.
“w+” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist a new file is created. Returns NULL, if unable to open file.
“a+” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
As given above, if you want to perform operations on a binary file, then you have to append ‘b’ at the last. For example, instead of “w”, you have to use “wb”, instead of “a+” you have to use “a+b”. For performing the operations on the file, a special pointer called File pointer is used which is declared as
FILE *filePointer;
So, the file can be opened as
FilePointer = fopen(“fileName.txt”, “w”)
The second parameter can be changed to contain all the attributes listed in the above table.
Reading from a file –
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Writing a file –:
The file write operations can be perfomed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Closing a file:
After every successful fie operations, you must always close a file. For closing a file, you have to use fclose function. The snippet for closing a file is given as :
FILE *filePointer;
FilePointer= fopen(“fileName.txt”, “w”);
---------- Some file Operations -------
Fclose(filePointer)
Example 1: Program to Open a File, Write in it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Write in it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Get the data to be written in file
Char dataToBeWritten[50]
= "GeeksforGeeks-A Computer Science Portal for Geeks";
// Open the existing file GfgTest.c using fopen()
// in write mode using "w" attribute
FilePointer = fopen("GfgTest.c", "w") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Write the dataToBeWritten into the file
If ( strlen ( dataToBeWritten ) > 0 )
{
// writing in the file using fputs()
Fputs(dataToBeWritten, filePointer) ;
Fputs("\n", filePointer) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully written in file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
Example 2: Program to Open a File, Read from it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Read from it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Declare the variable for the data to be read from file
Char dataToBeRead[50];
// Open the existing file GfgTest.c using fopen()
// in read mode using "r" attribute
FilePointer = fopen("GfgTest.c", "r") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Read the dataToBeRead from the file
// using fgets() method
While( fgets ( dataToBeRead, 50, filePointer ) != NULL )
{
// Print the dataToBeRead
Printf( "%s" , dataToBeRead ) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully read from file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
File I/O functions
Functions used in File Handling are as follows:
1. Open
● Before opening a file it should be linked to appropriate stream i.e. input, output or input/output.
● If we want to create input stream we will write
Ifstream infile;
For output stream we will write
Ofstream outfile;
For Input/Output stream we will write
Fstream iofile;
● Assume we want to write data to file named “Example” so we will use output stream. Then file can be opened as follows,
Ofstream outfile;
Outfile.open(“Example”);
● Now if we want to read data from file, so we will use input stream. Then file can be opened as follows,
Ifstream infile;
Infile.open(“Example”);
2. Close()
We use member function close() to close a file. The close function neither takes parameters nor returns any value.
e.g. Outfile.close();
Program to illustrate working with files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Char str1[50];
Ofstream fout; // create out stream
Fout.open(“Sample”); //opening file named “Sample” with open function
Cout<<”Enter string”;
Cin>>str;//accepting string from user
Fout<<str<<\n”; //writing string to “Sample”file
Fout.close(); //closing “Sample” file
Ifstream fin; //create in stream
Fin.open(“Sample”); // open “Sample” file for reading”
Fin>>str; reading string from “Sample” file
Cout<<”String is”<<str;// display string to user
Fin.close();
Return 0 ;
}
Output
Enter String
C++
String is C++
3. Read()
● It is used to read binary file.
● It is written as follows.
In.read((char *)&S, sizeof(S));
Where “in” is input stream.
‘S’ is variable.
● It takes two arguments. First argument is the address of variable S and second is length of variable S in bytes.
4. Write()
● It is used to write binary file.
● It is written as follows
Out.write((char *)&S, sizeof(S));
Where “out” is output streams and ‘S’ is variable.
Program to illustrate I/O operations (read and write) on binary files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int marks[5],i;
Cout<<”Enter marks”;
For(i=0;i<=5;i++)
Cin>>marks[i];
Ofstream fout;
Fout.open(“Example”);// open file for writing
Fout.write((char*)& marks, sizeof(marks));// write data to file
Fout.close();
Ifstream fin;
Fin.open(“Example”); // open file for reading
Fin.read((char*)& marks, sizeof (marks));// reading data from file
Cout<<”Marks are”;
For(i=0;i<=5;i++)
Cout<<marks[i]; //displaying data to screen
Fin.close();
Return 0 ;
}
5. Detecting end of file
● If there is no more data to read in a file, this condition is called as end of file.
● End of file in C++ can be detected by using function eof().
● It is a member function of ios class.
● It returns a non zero value (true) if end of file is encountered, otherwise returns zero.
Program to illustrate eof() function.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int x;
Ifstream fin; // declares stream
Fin.open(“myfile”, ios::in);// open file in read mode
Fin>>x;//get first number
While(!fin.eof())//if not at end of file, continue
{ reading numbers
Cout<<x<<endl;//print numbers
Fin>>x;//get another number
}
Fin.close();//close file
Return 0 ;
}
Binary files
Executables, sound files, image files, object files, and other types of binary files are all examples of binary files. The fact that each byte of a binary file can be one of 256-bit patterns is what makes them binary. Binary encoding, also known as BCP (Binary Communications Protocol), consists of values ranging from 0 to 255.
Binary files are made up of a long string of the characters "1" and "0" arranged in complex patterns. In contrast to ASCII, these characters can be used to construct any type of data, including text and images. In binary encoding, most language elements, such as integers, real numbers, and operator names, are expressed by fewer characters than in ASCII encoding.
Reading data from text files:
We can use the following methods to read text from text files:
Read() - to read total data from file
Read(n)- to read n char from a file
Read line()- to read only one line
Read line()- to read at lines into a list
Eg:
Code: Read all data
F = open(‘abc:txt’,’r’)
Data = f.read()
Print(data)
f.close()
Output: Contents of ‘abc.txt’ will be printed
Eg:
Code read lines:
F = open(‘abc.txt’,’r’)
Lines =f.read lines()
For line in lines:
Print (line,end = “ “)
F.close ()
Writing Data to text files:
We can write character data to text files by using the following 2 methods.
1)Write (str)
2)Write lines (list of lines)
Eg: f=open(‘xyz.txt’,’w’)
f.write(‘SAE In’)
f.write(‘Kondhwa In’)
f.write(‘Pune’)
Print(‘data are written successfully’)
f.close()
Note: In the given code, data present in the file will be overridden every time if w run the program. Instead of overriding if we want append operation then we should open the file as follows.
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Write
The file write operations can be performed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Append
Create a C application that reads data from the user and writes it to a file. In C programming, how to add data to the end of a file. In this piece, I'll go through how to handle files in append mode. I'll show you how to use C's append file mode to append data to a file.
Example
Source file content
I love programming.
Programming with files is fun.
String to append
Learning C programming at Codeforwin is simple and easy.
Output file content after append
I love programming.
Programming with files is fun.
Learning C programming at Codeforwin is simple and easy.
Getc()
To read a character from a file, use the getc() function.
The getc() method has the following syntax:
Char getc (FILE *fp);
Example
FILE *fp;
Char ch;
Ch = getc(fp);
Putc()
To write a character into a file, use the putc() function.
The putc() method has the following syntax:
Putc (char ch, FILE *fp);
Example,
FILE *fp;
Char ch;
Putc(ch, fp);
Example for putc() and getc() functions
#include<stdio.h>
Int main(){
char ch;
FILE *fp;
fp=fopen("std1.txt","w"); //opening file in write mode
printf("enter the text.press cntrl Z:\n");
while((ch = getchar())!=EOF){
putc(ch,fp); // writing each character into the file
}
fclose(fp);
fp=fopen("std1.txt","r");
printf("text on the file:\n");
while ((ch=getc(fp))!=EOF){ // reading each character from file
putchar(ch); // displaying each character on to the screen
}
fclose(fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
Enter the text.press cntrl Z:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
^Z
Text on the file:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
Getw()
When reading a number from a file, the getw() function is utilized.
The getw() method has the following syntax:
Syntax
Int getw (FILE *fp);
Example
FILE *fp;
Int num;
Num = getw(fp);
Putw()
The putw() function is used to save a number to a file.
The putw() method has the following syntax:
Syntax
Putw (int num, FILE *fp);
Example
FILE *fp;
Int num;
Putw(num, fp);
Example for using putw() and getw()
#include<stdio.h>
Int main( ){
FILE *fp;
int i;
fp = fopen ("num.txt", "w");
for (i =1; i<= 10; i++){
putw (i, fp);
}
fclose (fp);
fp =fopen ("num.txt", "r");
printf ("file content is\n");
for (i =1; i<= 10; i++){
i= getw(fp);
printf ("%d",i);
printf("\n");
}
fclose (fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
File content is
1
2
3
4
5
6
7
8
9
10
Gets()
The function gets () reads a line from the keyboard.
Declaration: char *gets (char *string)
The gets function is used to read a string (a series of characters) from the keyboard. We can read the string from standard input/keyboard in a C programme as shown below.
Gets (string);
Puts()
The puts () function displays a line on the o/p screen.
Declaration: int fputs (const char *string, FILE *fp)
The fputs method saves a string to a file named fp. As shown below, in a C programme, we write a string to a file.
Fputs (fp, “some data”);
Scanf()
We utilise the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Printf()
For generating formatted or standard outputs in compliance with a format definition, we use the printf() function. The printf function's parameters are the output data and the format specification string ().
Syntax for printf()
Printf (format_specifiers, info_a, info_b,…….. );
Example of printf()
Printf(“%d %c”, info_a, info_b);
The conversion character is the character we define after the 'percent' character. It's because 'percent' permits any data type to be converted into another data type for further printing.
Conversion Character | Description and Meaning of Character |
c | The data is taken in the form of characters by the application. |
d | The data is converted into integers using this software (decimals). |
f | The application generates data in the form of a double or a float with a Precision 6 by default. |
s | Unless and until the programme encounters a NULL character, the programme recognises the data as a string and prints a single character from it. |
Sprintf()
"String print" is what sprintf stands for. It is a file handling function in the C programming language that is used to send formatted output to a string. Instead of publishing to the terminal, the sprintf() function saves the output to the sprintf char buffer.
Syntax
Int sprintf(char *str, const char *format, ...)
Parameter values
The sprintf() method accepts the following parameter values as input:
● str: This is a pointer to an array of char elements that holds the resultant string. It's where you'll place the data.
● format: This is a C string that describes the output and includes placeholders for the integer arguments that will be added into the formatted string. It refers to the string containing the text that will be written to the buffer. It is made up of characters and optional format specifiers beginning with %.
Example
In this example, the variable num is of the type float. The sprintf() function converts the values in the num variable into a string, which is then put in buffer.
#include<stdio.h>
Int main() {
float num = 9.9;
printf("Before using sprintf(), data is float type: %f\n", num);
char buffer[50]; //for storing the converted string
sprintf(buffer, "%f", num);
printf("After using sprintf() data is string type: %s", buffer);
}
Output:
Before using sprintf(), data is float type: 9.900000
After using sprintf() data is string type: 9.900000
Fprint()
To write a set of characters into a file, use the fprintf() function. It generates formatted output and transmits it to a stream.
Syntax:
Int fprintf(FILE *stream, const char *format [, argument, ...])
Example
#include <stdio.h>
Main(){
FILE *fp;
fp = fopen("file.txt", "w");//opening file
fprintf(fp, "Hello file by fprintf...\n");//writing data into file
fclose(fp);//closing file
}
Fwrite()
Syntax:
Size_t fwrite(const void *ptr, size_t size, size_t n, FILE *fp);
The fwrite() function writes data to the file that is specified by the void pointer ptr.
● ptr: it refers to the memory block that holds the data to be written.
● size: This determines the number of bytes to be written for each item.
● n: The number of things to be written is denoted by the letter n.
● fp: This is a reference to the file that will be used to store data items.
It returns the number of items successfully written to the file if it succeeds. It returns a number smaller than n if there is an error. The two inputs (size and n) and return value of fwrite() are of type size t, which is an unsigned int on most systems.
Example:
Writing structure
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student student_1 = {"Tina", 12, 88.123};
Fwrite(&student_1, sizeof(student_1), 1, fp);
The contents of variable student 1 are written to the file.
Fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Fflush()
The fflush function in the C programming language sends any unwritten data to the stream's buffer. The fflush function will flush any streams with unwritten data in the buffer if stream is a null pointer.
Syntax
The fflush function in C has the following syntax:
Int fflush(FILE *stream);
Stream
The stream should be flushed.
Returns
If the fflush function is successful, it returns zero; otherwise, it returns EOF.
Fclose()
To close a file, use the fclose() method. After you've completed all of the procedures on the file, you must close it. The following is the syntax for the fclose() function:
Int fclose( FILE *fp );
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
References:
- The C- Programming Language - By Brian W Kernighan and Dennis Ritchie
- C- Programming in an open source paradigm: By R.K. Kamat, K .S. Oza, S.R. Patil
- The GNU C Programming Tutorial -By Mark Burgess
- Let us C- By Yashwant Kanetkar
Unit - 2
Structures, Union and File Handling
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
● Ordinary variables can hold one piece of information
● Arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
● Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
● Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
● Struct keyword is used to declare structure.
● Members of structure are enclosed within opening and closing braces.
● Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
● Declaration of Structure reserves no space.
● It is nothing but the “ Template / Map / Shape ” of the structure .
● Memory is created , very first time when the variable is created / Instance is created.
Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1, e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Structure Initialization
1. When we declare a structure, memory is not allocated for uninitialized variable.
2. Let us discuss very familiar example of structure student, we can initialize structure variable in different ways –
Way 1: Declare and Initialize
Struct student
{
Char name[20];
Int roll;
Float marks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2: Declaring and Initializing Multiple Variables
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3: Initializing Single member
Struct student
{
Int mark1;
Int mark2;
Int mark3;
} sub1={67};
Though there are three members of structure, only one is initialized , Then remaining two members are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4: Initializing inside main
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
Void main()
{
Struct student s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below declaration statement will never allocate memory
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
We need to initialize structure variable to allocate some memory to the structure. Struct student s1 = {89,54,65};
Accessing Structure Elements
● Array elements are accessed using the Subscript variable, Similarly Structure members are accessed using dot [.] operator.
● (.) is called as “Structure member Operator”.
● Use this Operator in between “Structure variable” & “member name”
Struct employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Struct employee e= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
Printf(“%f”, e. Salary);
}
Key takeaway
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit.
The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address. Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Array of structure
In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
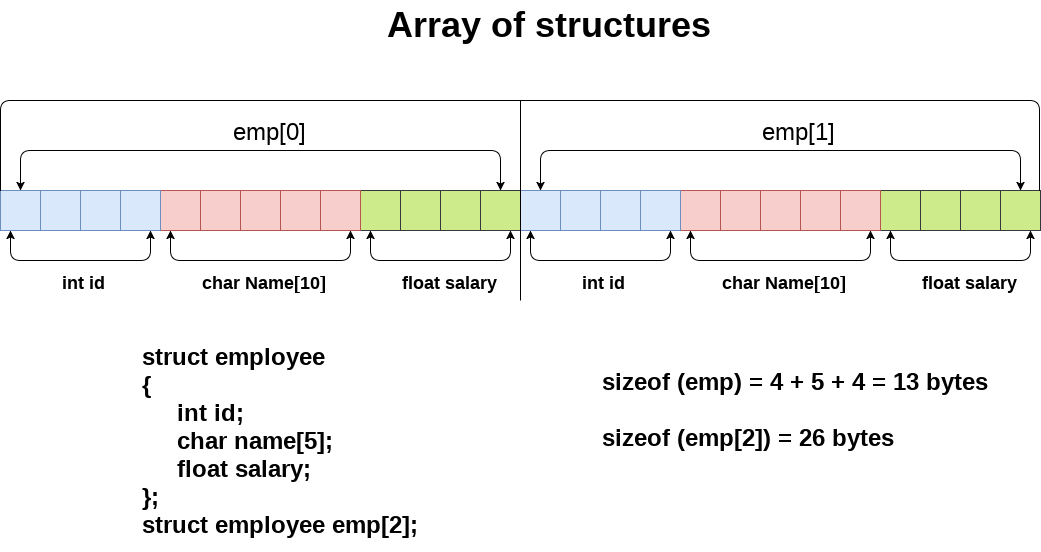
Fig: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Structure pointer
The structure pointer points to the memory block address where the Structure is stored. Like a pointer that points to another variable in memory of any data type (int, char, float). We utilise a structure pointer to tell the address of a structure in memory by referring the pointer variable ptr to the structure variable.
Declare a Structure Pointer
A structure pointer's declaration is comparable to that of a structure variable's declaration. As a result, the structure pointer and variable can be declared both inside and outside of the main() method. In C, we use the asterisk (*) symbol before the variable name to declare a pointer variable.
Struct structure_name *ptr;
Following the definition of the structure pointer, we must initialise it, as seen in the code:
Initialization of the Structure Pointer
Ptr = &structure_variable;
We can also immediately initialise a Structure Pointer when declaring a pointer.
Struct structure_name *ptr = &structure_variable;
As can be seen, a pointer ptr points to the Structure's structure variable location.
Access Structure member using pointer:
The Structure pointer can be used in two ways to access the structure's members:
● Using ( * ) asterisk or indirection operator and dot ( . ) operator.
● Using arrow ( -> ) operator or membership operator.
Program to access the structure member using structure pointer and the dot operator
Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
We constructed the Subject structure in the preceding programme, which has data components such as sub name (char), sub id (int), sub duration (char), and sub type (char) (char). The structure variable is sub, and the structure pointer variable is ptr, which points to the address of the sub variable, as in ptr = &sub. Each *ptr now has access to the address of a member of the Subject structure.
Program to access the structure member using structure pointer and arrow (->) operator
Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
In the preceding programme, we constructed an Employee structure with the pointer variables *ptr1 and *ptr2 and two structure variables emp1 and emp2. The members of the structure Employee are name, id, age, gender, and city. The pointer variable and arrow operator, which determine their memory space, are used by all Employee structure members to take their respective values from the user one by one.
Key takeaway
The feature of nesting one structure within another structure in C allows us to design complicated data types.
An array of structures is a collection of many structure variables, each of which contains information on a different entity.
The structure pointer points to the memory block address where the Structure is stored.
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function. Consider the following example, which uses the structural variable employee to call the function display(), which displays an employee's data.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void display(struct employee);
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
display(emp);
}
Void display(struct employee emp)
{
printf("Printing the details....\n");
printf("%s %s %d %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Self- referential structure
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
The structures pointing to the same type of structures are self-referential in nature.
Struct node {
intdata1;
chardata2;
struct node* link;
};
Int main()
{
struct node ob;
return0;
}
In the above example ‘link’ is a pointer to a structure of type ‘node’. Hence, the structure ‘node’ is a self-referential structure with ‘link’ as the referencing pointer.
An important point to consider is that the pointer should be initialized properly before accessing, as by default it contains garbage value.
Key takeaway
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function.
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
When operand is a data type:
#include <stdio.h>
Int main()
{
int x=89; // variable declaration.
printf("size of the variable x is %d", sizeof(x)); // Displaying the size of ?x? variable.
printf("\nsize of the integer data type is %d",sizeof(int)); //Displaying the size of integer data type.
printf("\nsize of the character data type is %d",sizeof(char)); //Displaying the size of character data type.
printf("\nsize of the floating data type is %d",sizeof(float)); //Displaying the size of floating data type.
Return 0;
}
The sizeof() operator is used in the preceding code to print the size of various data types such as int, char, and float.
Output
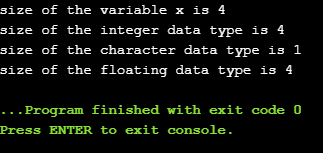
When operand is an expression
#include <stdio.h>
Int main()
{
double i=78.0; //variable initialization.
float j=6.78; //variable initialization.
printf("size of (i+j) expression is : %d",sizeof(i+j)); //Displaying the size of the expression (i+j).
return 0;
}
We created two variables, I and 'j,' of type double and float, respectively, in the following code, and then we used the sizeof(i+j) operator to print the size of the statement.
Output
Size of (i+j) expression is : 8
Typedef keyword
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Key takeaway
The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis.
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme.
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Accessing members of a union
- Array elements are accessed using the Subscript variable, Similarly Union members are accessed using dot [.] operator.
- (.) is called as “union member Operator”.
- Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P-
Garbage value, ABC
Key takeaway
Unions are quite similar to the structures in C. Union is also a derived type as structure.
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Most of the programs are written to store the information fetched from the program. One such way is to store the fetched information in a file. Different operations that can be performed on a file are:
- Creation of a new file (fopen with attributes as “a” or “a+” or “w” or “w++”)
- Opening an existing file (fopen)
- Reading from file (fscanf or fgets)
- Writing to a file (fprintf or fputs)
- Moving to a specific location in a file (fseek, rewind)
- Closing a file (fclose)
The text in the brackets denotes the functions used for performing those operations.
Functions in File Operations:
File opening modes in C:
“r” – Searches file. If the file is opened successfully, fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file cannot be opened, fopen( ) returns NULL.
“w” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“a” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer that points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“r+” – Searches file. If is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. Returns NULL, if unable to open the file.
“w+” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist a new file is created. Returns NULL, if unable to open file.
“a+” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
As given above, if you want to perform operations on a binary file, then you have to append ‘b’ at the last. For example, instead of “w”, you have to use “wb”, instead of “a+” you have to use “a+b”. For performing the operations on the file, a special pointer called File pointer is used which is declared as
FILE *filePointer;
So, the file can be opened as
FilePointer = fopen(“fileName.txt”, “w”)
The second parameter can be changed to contain all the attributes listed in the above table.
Reading from a file –
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Writing a file –:
The file write operations can be perfomed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Closing a file:
After every successful fie operations, you must always close a file. For closing a file, you have to use fclose function. The snippet for closing a file is given as :
FILE *filePointer;
FilePointer= fopen(“fileName.txt”, “w”);
---------- Some file Operations -------
Fclose(filePointer)
Example 1: Program to Open a File, Write in it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Write in it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Get the data to be written in file
Char dataToBeWritten[50]
= "GeeksforGeeks-A Computer Science Portal for Geeks";
// Open the existing file GfgTest.c using fopen()
// in write mode using "w" attribute
FilePointer = fopen("GfgTest.c", "w") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Write the dataToBeWritten into the file
If ( strlen ( dataToBeWritten ) > 0 )
{
// writing in the file using fputs()
Fputs(dataToBeWritten, filePointer) ;
Fputs("\n", filePointer) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully written in file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
Example 2: Program to Open a File, Read from it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Read from it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Declare the variable for the data to be read from file
Char dataToBeRead[50];
// Open the existing file GfgTest.c using fopen()
// in read mode using "r" attribute
FilePointer = fopen("GfgTest.c", "r") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Read the dataToBeRead from the file
// using fgets() method
While( fgets ( dataToBeRead, 50, filePointer ) != NULL )
{
// Print the dataToBeRead
Printf( "%s" , dataToBeRead ) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully read from file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
File I/O functions
Functions used in File Handling are as follows:
1. Open
● Before opening a file it should be linked to appropriate stream i.e. input, output or input/output.
● If we want to create input stream we will write
Ifstream infile;
For output stream we will write
Ofstream outfile;
For Input/Output stream we will write
Fstream iofile;
● Assume we want to write data to file named “Example” so we will use output stream. Then file can be opened as follows,
Ofstream outfile;
Outfile.open(“Example”);
● Now if we want to read data from file, so we will use input stream. Then file can be opened as follows,
Ifstream infile;
Infile.open(“Example”);
2. Close()
We use member function close() to close a file. The close function neither takes parameters nor returns any value.
e.g. Outfile.close();
Program to illustrate working with files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Char str1[50];
Ofstream fout; // create out stream
Fout.open(“Sample”); //opening file named “Sample” with open function
Cout<<”Enter string”;
Cin>>str;//accepting string from user
Fout<<str<<\n”; //writing string to “Sample”file
Fout.close(); //closing “Sample” file
Ifstream fin; //create in stream
Fin.open(“Sample”); // open “Sample” file for reading”
Fin>>str; reading string from “Sample” file
Cout<<”String is”<<str;// display string to user
Fin.close();
Return 0 ;
}
Output
Enter String
C++
String is C++
3. Read()
● It is used to read binary file.
● It is written as follows.
In.read((char *)&S, sizeof(S));
Where “in” is input stream.
‘S’ is variable.
● It takes two arguments. First argument is the address of variable S and second is length of variable S in bytes.
4. Write()
● It is used to write binary file.
● It is written as follows
Out.write((char *)&S, sizeof(S));
Where “out” is output streams and ‘S’ is variable.
Program to illustrate I/O operations (read and write) on binary files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int marks[5],i;
Cout<<”Enter marks”;
For(i=0;i<=5;i++)
Cin>>marks[i];
Ofstream fout;
Fout.open(“Example”);// open file for writing
Fout.write((char*)& marks, sizeof(marks));// write data to file
Fout.close();
Ifstream fin;
Fin.open(“Example”); // open file for reading
Fin.read((char*)& marks, sizeof (marks));// reading data from file
Cout<<”Marks are”;
For(i=0;i<=5;i++)
Cout<<marks[i]; //displaying data to screen
Fin.close();
Return 0 ;
}
5. Detecting end of file
● If there is no more data to read in a file, this condition is called as end of file.
● End of file in C++ can be detected by using function eof().
● It is a member function of ios class.
● It returns a non zero value (true) if end of file is encountered, otherwise returns zero.
Program to illustrate eof() function.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int x;
Ifstream fin; // declares stream
Fin.open(“myfile”, ios::in);// open file in read mode
Fin>>x;//get first number
While(!fin.eof())//if not at end of file, continue
{ reading numbers
Cout<<x<<endl;//print numbers
Fin>>x;//get another number
}
Fin.close();//close file
Return 0 ;
}
Binary files
Executables, sound files, image files, object files, and other types of binary files are all examples of binary files. The fact that each byte of a binary file can be one of 256-bit patterns is what makes them binary. Binary encoding, also known as BCP (Binary Communications Protocol), consists of values ranging from 0 to 255.
Binary files are made up of a long string of the characters "1" and "0" arranged in complex patterns. In contrast to ASCII, these characters can be used to construct any type of data, including text and images. In binary encoding, most language elements, such as integers, real numbers, and operator names, are expressed by fewer characters than in ASCII encoding.
Reading data from text files:
We can use the following methods to read text from text files:
Read() - to read total data from file
Read(n)- to read n char from a file
Read line()- to read only one line
Read line()- to read at lines into a list
Eg:
Code: Read all data
F = open(‘abc:txt’,’r’)
Data = f.read()
Print(data)
f.close()
Output: Contents of ‘abc.txt’ will be printed
Eg:
Code read lines:
F = open(‘abc.txt’,’r’)
Lines =f.read lines()
For line in lines:
Print (line,end = “ “)
F.close ()
Writing Data to text files:
We can write character data to text files by using the following 2 methods.
1)Write (str)
2)Write lines (list of lines)
Eg: f=open(‘xyz.txt’,’w’)
f.write(‘SAE In’)
f.write(‘Kondhwa In’)
f.write(‘Pune’)
Print(‘data are written successfully’)
f.close()
Note: In the given code, data present in the file will be overridden every time if w run the program. Instead of overriding if we want append operation then we should open the file as follows.
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Write
The file write operations can be performed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Append
Create a C application that reads data from the user and writes it to a file. In C programming, how to add data to the end of a file. In this piece, I'll go through how to handle files in append mode. I'll show you how to use C's append file mode to append data to a file.
Example
Source file content
I love programming.
Programming with files is fun.
String to append
Learning C programming at Codeforwin is simple and easy.
Output file content after append
I love programming.
Programming with files is fun.
Learning C programming at Codeforwin is simple and easy.
Getc()
To read a character from a file, use the getc() function.
The getc() method has the following syntax:
Char getc (FILE *fp);
Example
FILE *fp;
Char ch;
Ch = getc(fp);
Putc()
To write a character into a file, use the putc() function.
The putc() method has the following syntax:
Putc (char ch, FILE *fp);
Example,
FILE *fp;
Char ch;
Putc(ch, fp);
Example for putc() and getc() functions
#include<stdio.h>
Int main(){
char ch;
FILE *fp;
fp=fopen("std1.txt","w"); //opening file in write mode
printf("enter the text.press cntrl Z:\n");
while((ch = getchar())!=EOF){
putc(ch,fp); // writing each character into the file
}
fclose(fp);
fp=fopen("std1.txt","r");
printf("text on the file:\n");
while ((ch=getc(fp))!=EOF){ // reading each character from file
putchar(ch); // displaying each character on to the screen
}
fclose(fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
Enter the text.press cntrl Z:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
^Z
Text on the file:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
Getw()
When reading a number from a file, the getw() function is utilized.
The getw() method has the following syntax:
Syntax
Int getw (FILE *fp);
Example
FILE *fp;
Int num;
Num = getw(fp);
Putw()
The putw() function is used to save a number to a file.
The putw() method has the following syntax:
Syntax
Putw (int num, FILE *fp);
Example
FILE *fp;
Int num;
Putw(num, fp);
Example for using putw() and getw()
#include<stdio.h>
Int main( ){
FILE *fp;
int i;
fp = fopen ("num.txt", "w");
for (i =1; i<= 10; i++){
putw (i, fp);
}
fclose (fp);
fp =fopen ("num.txt", "r");
printf ("file content is\n");
for (i =1; i<= 10; i++){
i= getw(fp);
printf ("%d",i);
printf("\n");
}
fclose (fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
File content is
1
2
3
4
5
6
7
8
9
10
Gets()
The function gets () reads a line from the keyboard.
Declaration: char *gets (char *string)
The gets function is used to read a string (a series of characters) from the keyboard. We can read the string from standard input/keyboard in a C programme as shown below.
Gets (string);
Puts()
The puts () function displays a line on the o/p screen.
Declaration: int fputs (const char *string, FILE *fp)
The fputs method saves a string to a file named fp. As shown below, in a C programme, we write a string to a file.
Fputs (fp, “some data”);
Scanf()
We utilise the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Printf()
For generating formatted or standard outputs in compliance with a format definition, we use the printf() function. The printf function's parameters are the output data and the format specification string ().
Syntax for printf()
Printf (format_specifiers, info_a, info_b,…….. );
Example of printf()
Printf(“%d %c”, info_a, info_b);
The conversion character is the character we define after the 'percent' character. It's because 'percent' permits any data type to be converted into another data type for further printing.
Conversion Character | Description and Meaning of Character |
c | The data is taken in the form of characters by the application. |
d | The data is converted into integers using this software (decimals). |
f | The application generates data in the form of a double or a float with a Precision 6 by default. |
s | Unless and until the programme encounters a NULL character, the programme recognises the data as a string and prints a single character from it. |
Sprintf()
"String print" is what sprintf stands for. It is a file handling function in the C programming language that is used to send formatted output to a string. Instead of publishing to the terminal, the sprintf() function saves the output to the sprintf char buffer.
Syntax
Int sprintf(char *str, const char *format, ...)
Parameter values
The sprintf() method accepts the following parameter values as input:
● str: This is a pointer to an array of char elements that holds the resultant string. It's where you'll place the data.
● format: This is a C string that describes the output and includes placeholders for the integer arguments that will be added into the formatted string. It refers to the string containing the text that will be written to the buffer. It is made up of characters and optional format specifiers beginning with %.
Example
In this example, the variable num is of the type float. The sprintf() function converts the values in the num variable into a string, which is then put in buffer.
#include<stdio.h>
Int main() {
float num = 9.9;
printf("Before using sprintf(), data is float type: %f\n", num);
char buffer[50]; //for storing the converted string
sprintf(buffer, "%f", num);
printf("After using sprintf() data is string type: %s", buffer);
}
Output:
Before using sprintf(), data is float type: 9.900000
After using sprintf() data is string type: 9.900000
Fprint()
To write a set of characters into a file, use the fprintf() function. It generates formatted output and transmits it to a stream.
Syntax:
Int fprintf(FILE *stream, const char *format [, argument, ...])
Example
#include <stdio.h>
Main(){
FILE *fp;
fp = fopen("file.txt", "w");//opening file
fprintf(fp, "Hello file by fprintf...\n");//writing data into file
fclose(fp);//closing file
}
Fwrite()
Syntax:
Size_t fwrite(const void *ptr, size_t size, size_t n, FILE *fp);
The fwrite() function writes data to the file that is specified by the void pointer ptr.
● ptr: it refers to the memory block that holds the data to be written.
● size: This determines the number of bytes to be written for each item.
● n: The number of things to be written is denoted by the letter n.
● fp: This is a reference to the file that will be used to store data items.
It returns the number of items successfully written to the file if it succeeds. It returns a number smaller than n if there is an error. The two inputs (size and n) and return value of fwrite() are of type size t, which is an unsigned int on most systems.
Example:
Writing structure
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student student_1 = {"Tina", 12, 88.123};
Fwrite(&student_1, sizeof(student_1), 1, fp);
The contents of variable student 1 are written to the file.
Fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Fflush()
The fflush function in the C programming language sends any unwritten data to the stream's buffer. The fflush function will flush any streams with unwritten data in the buffer if stream is a null pointer.
Syntax
The fflush function in C has the following syntax:
Int fflush(FILE *stream);
Stream
The stream should be flushed.
Returns
If the fflush function is successful, it returns zero; otherwise, it returns EOF.
Fclose()
To close a file, use the fclose() method. After you've completed all of the procedures on the file, you must close it. The following is the syntax for the fclose() function:
Int fclose( FILE *fp );
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
References:
- The C- Programming Language - By Brian W Kernighan and Dennis Ritchie
- C- Programming in an open source paradigm: By R.K. Kamat, K .S. Oza, S.R. Patil
- The GNU C Programming Tutorial -By Mark Burgess
- Let us C- By Yashwant Kanetkar
Unit - 2
Structures, Union and File Handling
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
● Ordinary variables can hold one piece of information
● Arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
● Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
● Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
● Struct keyword is used to declare structure.
● Members of structure are enclosed within opening and closing braces.
● Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
● Declaration of Structure reserves no space.
● It is nothing but the “ Template / Map / Shape ” of the structure .
● Memory is created , very first time when the variable is created / Instance is created.
Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1, e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Structure Initialization
1. When we declare a structure, memory is not allocated for uninitialized variable.
2. Let us discuss very familiar example of structure student, we can initialize structure variable in different ways –
Way 1: Declare and Initialize
Struct student
{
Char name[20];
Int roll;
Float marks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2: Declaring and Initializing Multiple Variables
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3: Initializing Single member
Struct student
{
Int mark1;
Int mark2;
Int mark3;
} sub1={67};
Though there are three members of structure, only one is initialized , Then remaining two members are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4: Initializing inside main
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
Void main()
{
Struct student s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below declaration statement will never allocate memory
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
We need to initialize structure variable to allocate some memory to the structure. Struct student s1 = {89,54,65};
Accessing Structure Elements
● Array elements are accessed using the Subscript variable, Similarly Structure members are accessed using dot [.] operator.
● (.) is called as “Structure member Operator”.
● Use this Operator in between “Structure variable” & “member name”
Struct employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Struct employee e= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
Printf(“%f”, e. Salary);
}
Key takeaway
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit.
The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address. Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Array of structure
In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
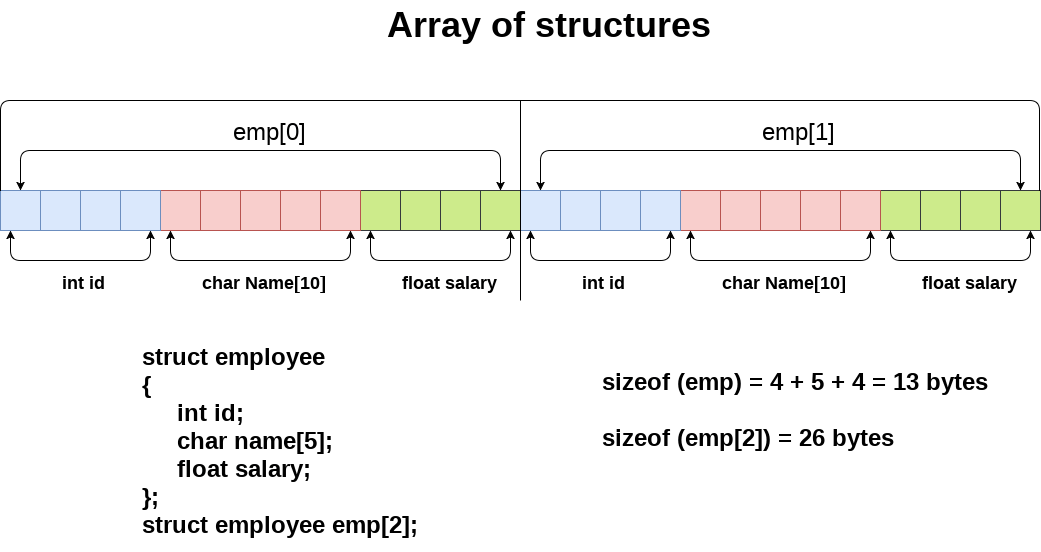
Fig: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Structure pointer
The structure pointer points to the memory block address where the Structure is stored. Like a pointer that points to another variable in memory of any data type (int, char, float). We utilise a structure pointer to tell the address of a structure in memory by referring the pointer variable ptr to the structure variable.
Declare a Structure Pointer
A structure pointer's declaration is comparable to that of a structure variable's declaration. As a result, the structure pointer and variable can be declared both inside and outside of the main() method. In C, we use the asterisk (*) symbol before the variable name to declare a pointer variable.
Struct structure_name *ptr;
Following the definition of the structure pointer, we must initialise it, as seen in the code:
Initialization of the Structure Pointer
Ptr = &structure_variable;
We can also immediately initialise a Structure Pointer when declaring a pointer.
Struct structure_name *ptr = &structure_variable;
As can be seen, a pointer ptr points to the Structure's structure variable location.
Access Structure member using pointer:
The Structure pointer can be used in two ways to access the structure's members:
● Using ( * ) asterisk or indirection operator and dot ( . ) operator.
● Using arrow ( -> ) operator or membership operator.
Program to access the structure member using structure pointer and the dot operator
Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
We constructed the Subject structure in the preceding programme, which has data components such as sub name (char), sub id (int), sub duration (char), and sub type (char) (char). The structure variable is sub, and the structure pointer variable is ptr, which points to the address of the sub variable, as in ptr = &sub. Each *ptr now has access to the address of a member of the Subject structure.
Program to access the structure member using structure pointer and arrow (->) operator
Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
In the preceding programme, we constructed an Employee structure with the pointer variables *ptr1 and *ptr2 and two structure variables emp1 and emp2. The members of the structure Employee are name, id, age, gender, and city. The pointer variable and arrow operator, which determine their memory space, are used by all Employee structure members to take their respective values from the user one by one.
Key takeaway
The feature of nesting one structure within another structure in C allows us to design complicated data types.
An array of structures is a collection of many structure variables, each of which contains information on a different entity.
The structure pointer points to the memory block address where the Structure is stored.
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function. Consider the following example, which uses the structural variable employee to call the function display(), which displays an employee's data.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void display(struct employee);
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
display(emp);
}
Void display(struct employee emp)
{
printf("Printing the details....\n");
printf("%s %s %d %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Self- referential structure
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
The structures pointing to the same type of structures are self-referential in nature.
Struct node {
intdata1;
chardata2;
struct node* link;
};
Int main()
{
struct node ob;
return0;
}
In the above example ‘link’ is a pointer to a structure of type ‘node’. Hence, the structure ‘node’ is a self-referential structure with ‘link’ as the referencing pointer.
An important point to consider is that the pointer should be initialized properly before accessing, as by default it contains garbage value.
Key takeaway
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function.
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
When operand is a data type:
#include <stdio.h>
Int main()
{
int x=89; // variable declaration.
printf("size of the variable x is %d", sizeof(x)); // Displaying the size of ?x? variable.
printf("\nsize of the integer data type is %d",sizeof(int)); //Displaying the size of integer data type.
printf("\nsize of the character data type is %d",sizeof(char)); //Displaying the size of character data type.
printf("\nsize of the floating data type is %d",sizeof(float)); //Displaying the size of floating data type.
Return 0;
}
The sizeof() operator is used in the preceding code to print the size of various data types such as int, char, and float.
Output
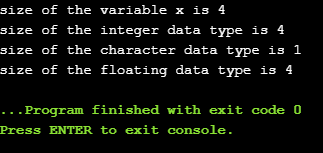
When operand is an expression
#include <stdio.h>
Int main()
{
double i=78.0; //variable initialization.
float j=6.78; //variable initialization.
printf("size of (i+j) expression is : %d",sizeof(i+j)); //Displaying the size of the expression (i+j).
return 0;
}
We created two variables, I and 'j,' of type double and float, respectively, in the following code, and then we used the sizeof(i+j) operator to print the size of the statement.
Output
Size of (i+j) expression is : 8
Typedef keyword
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Key takeaway
The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis.
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme.
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Accessing members of a union
- Array elements are accessed using the Subscript variable, Similarly Union members are accessed using dot [.] operator.
- (.) is called as “union member Operator”.
- Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P-
Garbage value, ABC
Key takeaway
Unions are quite similar to the structures in C. Union is also a derived type as structure.
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Most of the programs are written to store the information fetched from the program. One such way is to store the fetched information in a file. Different operations that can be performed on a file are:
- Creation of a new file (fopen with attributes as “a” or “a+” or “w” or “w++”)
- Opening an existing file (fopen)
- Reading from file (fscanf or fgets)
- Writing to a file (fprintf or fputs)
- Moving to a specific location in a file (fseek, rewind)
- Closing a file (fclose)
The text in the brackets denotes the functions used for performing those operations.
Functions in File Operations:
File opening modes in C:
“r” – Searches file. If the file is opened successfully, fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file cannot be opened, fopen( ) returns NULL.
“w” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“a” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer that points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“r+” – Searches file. If is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. Returns NULL, if unable to open the file.
“w+” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist a new file is created. Returns NULL, if unable to open file.
“a+” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
As given above, if you want to perform operations on a binary file, then you have to append ‘b’ at the last. For example, instead of “w”, you have to use “wb”, instead of “a+” you have to use “a+b”. For performing the operations on the file, a special pointer called File pointer is used which is declared as
FILE *filePointer;
So, the file can be opened as
FilePointer = fopen(“fileName.txt”, “w”)
The second parameter can be changed to contain all the attributes listed in the above table.
Reading from a file –
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Writing a file –:
The file write operations can be perfomed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Closing a file:
After every successful fie operations, you must always close a file. For closing a file, you have to use fclose function. The snippet for closing a file is given as :
FILE *filePointer;
FilePointer= fopen(“fileName.txt”, “w”);
---------- Some file Operations -------
Fclose(filePointer)
Example 1: Program to Open a File, Write in it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Write in it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Get the data to be written in file
Char dataToBeWritten[50]
= "GeeksforGeeks-A Computer Science Portal for Geeks";
// Open the existing file GfgTest.c using fopen()
// in write mode using "w" attribute
FilePointer = fopen("GfgTest.c", "w") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Write the dataToBeWritten into the file
If ( strlen ( dataToBeWritten ) > 0 )
{
// writing in the file using fputs()
Fputs(dataToBeWritten, filePointer) ;
Fputs("\n", filePointer) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully written in file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
Example 2: Program to Open a File, Read from it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Read from it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Declare the variable for the data to be read from file
Char dataToBeRead[50];
// Open the existing file GfgTest.c using fopen()
// in read mode using "r" attribute
FilePointer = fopen("GfgTest.c", "r") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Read the dataToBeRead from the file
// using fgets() method
While( fgets ( dataToBeRead, 50, filePointer ) != NULL )
{
// Print the dataToBeRead
Printf( "%s" , dataToBeRead ) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully read from file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
File I/O functions
Functions used in File Handling are as follows:
1. Open
● Before opening a file it should be linked to appropriate stream i.e. input, output or input/output.
● If we want to create input stream we will write
Ifstream infile;
For output stream we will write
Ofstream outfile;
For Input/Output stream we will write
Fstream iofile;
● Assume we want to write data to file named “Example” so we will use output stream. Then file can be opened as follows,
Ofstream outfile;
Outfile.open(“Example”);
● Now if we want to read data from file, so we will use input stream. Then file can be opened as follows,
Ifstream infile;
Infile.open(“Example”);
2. Close()
We use member function close() to close a file. The close function neither takes parameters nor returns any value.
e.g. Outfile.close();
Program to illustrate working with files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Char str1[50];
Ofstream fout; // create out stream
Fout.open(“Sample”); //opening file named “Sample” with open function
Cout<<”Enter string”;
Cin>>str;//accepting string from user
Fout<<str<<\n”; //writing string to “Sample”file
Fout.close(); //closing “Sample” file
Ifstream fin; //create in stream
Fin.open(“Sample”); // open “Sample” file for reading”
Fin>>str; reading string from “Sample” file
Cout<<”String is”<<str;// display string to user
Fin.close();
Return 0 ;
}
Output
Enter String
C++
String is C++
3. Read()
● It is used to read binary file.
● It is written as follows.
In.read((char *)&S, sizeof(S));
Where “in” is input stream.
‘S’ is variable.
● It takes two arguments. First argument is the address of variable S and second is length of variable S in bytes.
4. Write()
● It is used to write binary file.
● It is written as follows
Out.write((char *)&S, sizeof(S));
Where “out” is output streams and ‘S’ is variable.
Program to illustrate I/O operations (read and write) on binary files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int marks[5],i;
Cout<<”Enter marks”;
For(i=0;i<=5;i++)
Cin>>marks[i];
Ofstream fout;
Fout.open(“Example”);// open file for writing
Fout.write((char*)& marks, sizeof(marks));// write data to file
Fout.close();
Ifstream fin;
Fin.open(“Example”); // open file for reading
Fin.read((char*)& marks, sizeof (marks));// reading data from file
Cout<<”Marks are”;
For(i=0;i<=5;i++)
Cout<<marks[i]; //displaying data to screen
Fin.close();
Return 0 ;
}
5. Detecting end of file
● If there is no more data to read in a file, this condition is called as end of file.
● End of file in C++ can be detected by using function eof().
● It is a member function of ios class.
● It returns a non zero value (true) if end of file is encountered, otherwise returns zero.
Program to illustrate eof() function.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int x;
Ifstream fin; // declares stream
Fin.open(“myfile”, ios::in);// open file in read mode
Fin>>x;//get first number
While(!fin.eof())//if not at end of file, continue
{ reading numbers
Cout<<x<<endl;//print numbers
Fin>>x;//get another number
}
Fin.close();//close file
Return 0 ;
}
Binary files
Executables, sound files, image files, object files, and other types of binary files are all examples of binary files. The fact that each byte of a binary file can be one of 256-bit patterns is what makes them binary. Binary encoding, also known as BCP (Binary Communications Protocol), consists of values ranging from 0 to 255.
Binary files are made up of a long string of the characters "1" and "0" arranged in complex patterns. In contrast to ASCII, these characters can be used to construct any type of data, including text and images. In binary encoding, most language elements, such as integers, real numbers, and operator names, are expressed by fewer characters than in ASCII encoding.
Reading data from text files:
We can use the following methods to read text from text files:
Read() - to read total data from file
Read(n)- to read n char from a file
Read line()- to read only one line
Read line()- to read at lines into a list
Eg:
Code: Read all data
F = open(‘abc:txt’,’r’)
Data = f.read()
Print(data)
f.close()
Output: Contents of ‘abc.txt’ will be printed
Eg:
Code read lines:
F = open(‘abc.txt’,’r’)
Lines =f.read lines()
For line in lines:
Print (line,end = “ “)
F.close ()
Writing Data to text files:
We can write character data to text files by using the following 2 methods.
1)Write (str)
2)Write lines (list of lines)
Eg: f=open(‘xyz.txt’,’w’)
f.write(‘SAE In’)
f.write(‘Kondhwa In’)
f.write(‘Pune’)
Print(‘data are written successfully’)
f.close()
Note: In the given code, data present in the file will be overridden every time if w run the program. Instead of overriding if we want append operation then we should open the file as follows.
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Write
The file write operations can be performed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Append
Create a C application that reads data from the user and writes it to a file. In C programming, how to add data to the end of a file. In this piece, I'll go through how to handle files in append mode. I'll show you how to use C's append file mode to append data to a file.
Example
Source file content
I love programming.
Programming with files is fun.
String to append
Learning C programming at Codeforwin is simple and easy.
Output file content after append
I love programming.
Programming with files is fun.
Learning C programming at Codeforwin is simple and easy.
Getc()
To read a character from a file, use the getc() function.
The getc() method has the following syntax:
Char getc (FILE *fp);
Example
FILE *fp;
Char ch;
Ch = getc(fp);
Putc()
To write a character into a file, use the putc() function.
The putc() method has the following syntax:
Putc (char ch, FILE *fp);
Example,
FILE *fp;
Char ch;
Putc(ch, fp);
Example for putc() and getc() functions
#include<stdio.h>
Int main(){
char ch;
FILE *fp;
fp=fopen("std1.txt","w"); //opening file in write mode
printf("enter the text.press cntrl Z:\n");
while((ch = getchar())!=EOF){
putc(ch,fp); // writing each character into the file
}
fclose(fp);
fp=fopen("std1.txt","r");
printf("text on the file:\n");
while ((ch=getc(fp))!=EOF){ // reading each character from file
putchar(ch); // displaying each character on to the screen
}
fclose(fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
Enter the text.press cntrl Z:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
^Z
Text on the file:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
Getw()
When reading a number from a file, the getw() function is utilized.
The getw() method has the following syntax:
Syntax
Int getw (FILE *fp);
Example
FILE *fp;
Int num;
Num = getw(fp);
Putw()
The putw() function is used to save a number to a file.
The putw() method has the following syntax:
Syntax
Putw (int num, FILE *fp);
Example
FILE *fp;
Int num;
Putw(num, fp);
Example for using putw() and getw()
#include<stdio.h>
Int main( ){
FILE *fp;
int i;
fp = fopen ("num.txt", "w");
for (i =1; i<= 10; i++){
putw (i, fp);
}
fclose (fp);
fp =fopen ("num.txt", "r");
printf ("file content is\n");
for (i =1; i<= 10; i++){
i= getw(fp);
printf ("%d",i);
printf("\n");
}
fclose (fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
File content is
1
2
3
4
5
6
7
8
9
10
Gets()
The function gets () reads a line from the keyboard.
Declaration: char *gets (char *string)
The gets function is used to read a string (a series of characters) from the keyboard. We can read the string from standard input/keyboard in a C programme as shown below.
Gets (string);
Puts()
The puts () function displays a line on the o/p screen.
Declaration: int fputs (const char *string, FILE *fp)
The fputs method saves a string to a file named fp. As shown below, in a C programme, we write a string to a file.
Fputs (fp, “some data”);
Scanf()
We utilise the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Printf()
For generating formatted or standard outputs in compliance with a format definition, we use the printf() function. The printf function's parameters are the output data and the format specification string ().
Syntax for printf()
Printf (format_specifiers, info_a, info_b,…….. );
Example of printf()
Printf(“%d %c”, info_a, info_b);
The conversion character is the character we define after the 'percent' character. It's because 'percent' permits any data type to be converted into another data type for further printing.
Conversion Character | Description and Meaning of Character |
c | The data is taken in the form of characters by the application. |
d | The data is converted into integers using this software (decimals). |
f | The application generates data in the form of a double or a float with a Precision 6 by default. |
s | Unless and until the programme encounters a NULL character, the programme recognises the data as a string and prints a single character from it. |
Sprintf()
"String print" is what sprintf stands for. It is a file handling function in the C programming language that is used to send formatted output to a string. Instead of publishing to the terminal, the sprintf() function saves the output to the sprintf char buffer.
Syntax
Int sprintf(char *str, const char *format, ...)
Parameter values
The sprintf() method accepts the following parameter values as input:
● str: This is a pointer to an array of char elements that holds the resultant string. It's where you'll place the data.
● format: This is a C string that describes the output and includes placeholders for the integer arguments that will be added into the formatted string. It refers to the string containing the text that will be written to the buffer. It is made up of characters and optional format specifiers beginning with %.
Example
In this example, the variable num is of the type float. The sprintf() function converts the values in the num variable into a string, which is then put in buffer.
#include<stdio.h>
Int main() {
float num = 9.9;
printf("Before using sprintf(), data is float type: %f\n", num);
char buffer[50]; //for storing the converted string
sprintf(buffer, "%f", num);
printf("After using sprintf() data is string type: %s", buffer);
}
Output:
Before using sprintf(), data is float type: 9.900000
After using sprintf() data is string type: 9.900000
Fprint()
To write a set of characters into a file, use the fprintf() function. It generates formatted output and transmits it to a stream.
Syntax:
Int fprintf(FILE *stream, const char *format [, argument, ...])
Example
#include <stdio.h>
Main(){
FILE *fp;
fp = fopen("file.txt", "w");//opening file
fprintf(fp, "Hello file by fprintf...\n");//writing data into file
fclose(fp);//closing file
}
Fwrite()
Syntax:
Size_t fwrite(const void *ptr, size_t size, size_t n, FILE *fp);
The fwrite() function writes data to the file that is specified by the void pointer ptr.
● ptr: it refers to the memory block that holds the data to be written.
● size: This determines the number of bytes to be written for each item.
● n: The number of things to be written is denoted by the letter n.
● fp: This is a reference to the file that will be used to store data items.
It returns the number of items successfully written to the file if it succeeds. It returns a number smaller than n if there is an error. The two inputs (size and n) and return value of fwrite() are of type size t, which is an unsigned int on most systems.
Example:
Writing structure
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student student_1 = {"Tina", 12, 88.123};
Fwrite(&student_1, sizeof(student_1), 1, fp);
The contents of variable student 1 are written to the file.
Fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Fflush()
The fflush function in the C programming language sends any unwritten data to the stream's buffer. The fflush function will flush any streams with unwritten data in the buffer if stream is a null pointer.
Syntax
The fflush function in C has the following syntax:
Int fflush(FILE *stream);
Stream
The stream should be flushed.
Returns
If the fflush function is successful, it returns zero; otherwise, it returns EOF.
Fclose()
To close a file, use the fclose() method. After you've completed all of the procedures on the file, you must close it. The following is the syntax for the fclose() function:
Int fclose( FILE *fp );
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
References:
- The C- Programming Language - By Brian W Kernighan and Dennis Ritchie
- C- Programming in an open source paradigm: By R.K. Kamat, K .S. Oza, S.R. Patil
- The GNU C Programming Tutorial -By Mark Burgess
- Let us C- By Yashwant Kanetkar
Unit - 2
Structures, Union and File Handling
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
● Ordinary variables can hold one piece of information
● Arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
● Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
● Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
● Struct keyword is used to declare structure.
● Members of structure are enclosed within opening and closing braces.
● Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
● Declaration of Structure reserves no space.
● It is nothing but the “ Template / Map / Shape ” of the structure .
● Memory is created , very first time when the variable is created / Instance is created.
Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1, e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Structure Initialization
1. When we declare a structure, memory is not allocated for uninitialized variable.
2. Let us discuss very familiar example of structure student, we can initialize structure variable in different ways –
Way 1: Declare and Initialize
Struct student
{
Char name[20];
Int roll;
Float marks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2: Declaring and Initializing Multiple Variables
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3: Initializing Single member
Struct student
{
Int mark1;
Int mark2;
Int mark3;
} sub1={67};
Though there are three members of structure, only one is initialized , Then remaining two members are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4: Initializing inside main
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
Void main()
{
Struct student s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below declaration statement will never allocate memory
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
We need to initialize structure variable to allocate some memory to the structure. Struct student s1 = {89,54,65};
Accessing Structure Elements
● Array elements are accessed using the Subscript variable, Similarly Structure members are accessed using dot [.] operator.
● (.) is called as “Structure member Operator”.
● Use this Operator in between “Structure variable” & “member name”
Struct employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Struct employee e= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
Printf(“%f”, e. Salary);
}
Key takeaway
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit.
Unit - 2
Structures, Union and File Handling
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit (Unlike arrays which permit a programmer to group only elements of same data type).
Why Use Structures
● Ordinary variables can hold one piece of information
● Arrays can hold a number of pieces of information of the same data type.
For example, suppose you want to store data about a book. You might want to store its name (a string), its price (a float) and number of pages in it (an int).
If data about say 3 such books is to be stored, then we can follow two approaches:
● Construct individual arrays, one for storing names, another for storing prices and still another for storing number of pages.
● Use a structure variable.
Suppose we want to create a employee database. Then, we can define a structure called employee with three elements id, name and salary. The syntax of this structure is as follows:
Struct employee
{ int id;
Char name[50];
Float salary;
};
Note:
● Struct keyword is used to declare structure.
● Members of structure are enclosed within opening and closing braces.
● Usually structure type declaration appears at the top of the source code file, before any variables or functions are defined or maintained in separate header file.
● Declaration of Structure reserves no space.
● It is nothing but the “ Template / Map / Shape ” of the structure .
● Memory is created , very first time when the variable is created / Instance is created.
Structure variable declaration:
We can declare the variable of structure in two ways
- Declare the structure inside main function
- Declare the structure outside the main function.
1. Declare the structure inside main function
Following example show you, how structure variable is declared inside main function
Struct employee
{
Int id;
Char name[50];
Float salary;
};
Int main()
{
Struct employee e1, e2;
Return 0;
}
In this example the variable of structure employee is created inside main function that e1, e2.
2. Declare the structure outside main function
Following example show you, how structure variable is declared outside the main function
Struct employee
{
Int id;
Char name[50];
Float salary;
}e1,e2;
Structure Initialization
1. When we declare a structure, memory is not allocated for uninitialized variable.
2. Let us discuss very familiar example of structure student, we can initialize structure variable in different ways –
Way 1: Declare and Initialize
Struct student
{
Char name[20];
Int roll;
Float marks;
}std1 = { "Poonam",89,78.3 };
In the above code snippet, we have seen that structure is declared and as soon as after declaration we
Have initialized the structure variable.
Std1 = { "Poonam",89,78.3 }
This is the code for initializing structure variable in C programming
Way 2: Declaring and Initializing Multiple Variables
Struct student
{
Char name[20];
Int roll;
Float marks;
}
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
In this example, we have declared two structure variables in above code. After declaration of variable we have initialized two variable.
Std1 = {"Poonam" ,67, 78.3};
Std2 = {"Vishal",62, 71.3};
Way 3: Initializing Single member
Struct student
{
Int mark1;
Int mark2;
Int mark3;
} sub1={67};
Though there are three members of structure, only one is initialized , Then remaining two members are initialized with Zero. If there are variables of other data type then their initial values will be –
Data Type Default value if not initialized
Integer 0
Float 0.00
Char NULL
Way 4: Initializing inside main
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
Void main()
{
Struct student s1 = {89,54,65};
- - - - --
- - - - --
- - - - --
};
When we declare a structure then memory won’t be allocated for the structure. i.e only writing below declaration statement will never allocate memory
Struct student
{
Int mark1;
Int mark2;
Int mark3;
};
We need to initialize structure variable to allocate some memory to the structure. Struct student s1 = {89,54,65};
Accessing Structure Elements
● Array elements are accessed using the Subscript variable, Similarly Structure members are accessed using dot [.] operator.
● (.) is called as “Structure member Operator”.
● Use this Operator in between “Structure variable” & “member name”
Struct employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Struct employee e= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
Printf(“%f”, e. Salary);
}
Key takeaway
A structure can be considered as a template used for defining a collection of variables under a single name. Structures help programmers to group elements of different data types into a single logical unit.
The feature of nesting one structure within another structure in C allows us to design complicated data types. For example, we could need to keep an entity employee's address in a structure. Street number, city, state, and pin code are all possible subparts of the attribute address. As a result, we must store the employee's address in a different structure and nest the structure address into the structure employee to store the employee's address. Consider the programme below.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
printf("Printing the employee information....\n");
printf("name: %s\nCity: %s\nPincode: %d\nPhone: %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Output
Enter employee information?
Arun
Delhi
110001
1234567890
Printing the employee information....
Name: Arun
City: Delhi
Pincode: 110001
Phone: 1234567890
In the following methods, the structure can be nested.
● By separate structure
● By Embedded structure
1) Separate structure
We've created two structures here, but the dependent structure should be utilised as a member inside the primary structure. Consider the following illustration.
Struct Date
{
int dd;
int mm;
int yyyy;
};
Struct Employee
{
int id;
char name[20];
struct Date doj;
}emp1;
Doj (date of joining) is a Date variable, as you can see. In this case, doj is a member of the Employee structure. We may utilise the Date structure in a variety of structures this way.
2) Embedded structure
We can declare the structure inside the structure using the embedded structure. As a result, it uses fewer lines of code, but it cannot be applied to numerous data structures. Consider the following illustration.
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}emp1;
Accessing Nested Structure
We can access the member of the nested structure by Outer_Structure.Nested_Structure.member as given below:
e1.doj.dd
e1.doj.mm
e1.doj.yyyy
Example of Nested Structure
#include <stdio.h>
#include <string.h>
Struct Employee
{
int id;
char name[20];
struct Date
{
int dd;
int mm;
int yyyy;
}doj;
}e1;
Int main( )
{
//storing employee information
e1.id=101;
strcpy(e1.name, "Sonoo Jaiswal");//copying string into char array
e1.doj.dd=10;
e1.doj.mm=11;
e1.doj.yyyy=2014;
//printing first employee information
printf( "employee id : %d\n", e1.id);
printf( "employee name : %s\n", e1.name);
printf( "employee date of joining (dd/mm/yyyy) : %d/%d/%d\n", e1.doj.dd,e1.doj.mm,e1.doj.yyyy);
return 0;
}
Output:
Employee id : 101
Employee name : Sonoo Jaiswal
Employee date of joining (dd/mm/yyyy) : 10/11/2014
Array of structure
In C, an array of structures is a collection of many structure variables, each of which contains information on a different entity. In C, an array of structures is used to store data about many entities of various data kinds. The collection of structures is another name for the array of structures.
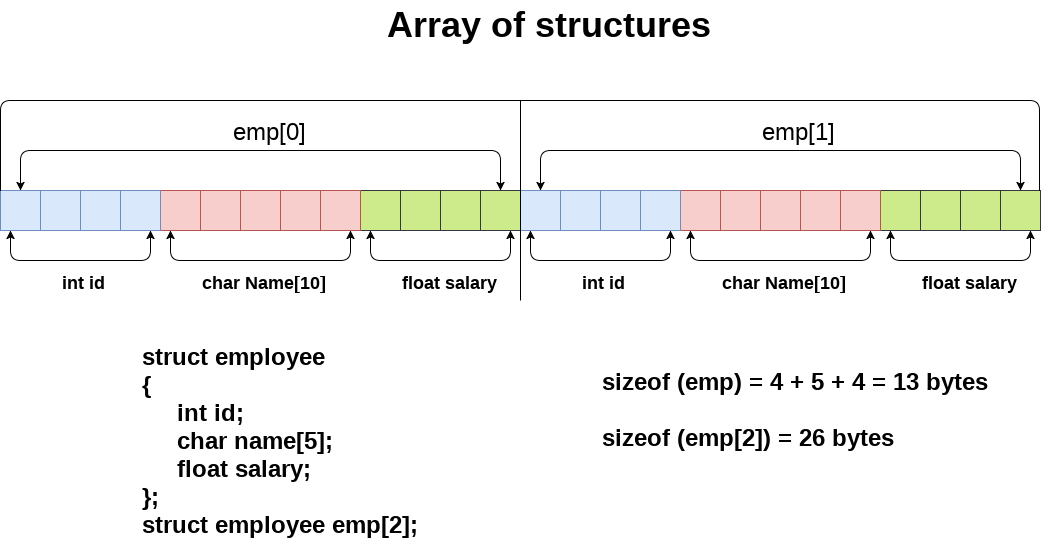
Fig: Array of structure
Let's look at an array of structures that stores and outputs information for five students.
#include<stdio.h>
#include <string.h>
Struct student{
Int rollno;
Char name[10];
};
Int main(){
Int i;
Struct student st[5];
Printf("Enter Records of 5 students");
For(i=0;i<5;i++){
Printf("\nEnter Rollno:");
Scanf("%d",&st[i].rollno);
Printf("\nEnter Name:");
Scanf("%s",&st[i].name);
}
Printf("\nStudent Information List:");
For(i=0;i<5;i++){
Printf("\nRollno:%d, Name:%s",st[i].rollno,st[i].name);
}
return 0;
}
Output:
Enter Records of 5 students
Enter Rollno:10
Enter Name:Sonu
Enter Rollno:20
Enter Name:Ratana
Enter Rollno:30
Enter Name:Vomika
Enter Rollno:40
Enter Name:James
Enter Rollno:50
Enter Name:Sadaf
Student Information List:
Rollno:10, Name:Sonu
Rollno:20, Name:Ratanaa
Rollno:30, Name:Vomika
Rollno:40, Name:James
Rollno:50, Name:Sadaf
Structure pointer
The structure pointer points to the memory block address where the Structure is stored. Like a pointer that points to another variable in memory of any data type (int, char, float). We utilise a structure pointer to tell the address of a structure in memory by referring the pointer variable ptr to the structure variable.
Declare a Structure Pointer
A structure pointer's declaration is comparable to that of a structure variable's declaration. As a result, the structure pointer and variable can be declared both inside and outside of the main() method. In C, we use the asterisk (*) symbol before the variable name to declare a pointer variable.
Struct structure_name *ptr;
Following the definition of the structure pointer, we must initialise it, as seen in the code:
Initialization of the Structure Pointer
Ptr = &structure_variable;
We can also immediately initialise a Structure Pointer when declaring a pointer.
Struct structure_name *ptr = &structure_variable;
As can be seen, a pointer ptr points to the Structure's structure variable location.
Access Structure member using pointer:
The Structure pointer can be used in two ways to access the structure's members:
● Using ( * ) asterisk or indirection operator and dot ( . ) operator.
● Using arrow ( -> ) operator or membership operator.
Program to access the structure member using structure pointer and the dot operator
Consider the following example of creating a Subject structure and accessing its components with a structure pointer that points to the Subject variable's address in C.
#include <stdio.h>
// create a structure Subject using the struct keyword
Struct Subject
{
// declare the member of the Course structure
char sub_name[30];
int sub_id;
char sub_duration[50];
char sub_type[50];
};
Int main()
{
struct Subject sub; // declare the Subject variable
struct Subject *ptr; // create a pointer variable (*ptr)
ptr = ⊂ /* ptr variable pointing to the address of the structure variable sub */
strcpy (sub.sub_name, " Computer Science");
sub.sub_id = 1201;
strcpy (sub.sub_duration, "6 Months");
strcpy (sub.sub_type, " Multiple Choice Question");
// print the details of the Subject;
printf (" Subject Name: %s\t ", (*ptr).sub_name);
printf (" \n Subject Id: %d\t ", (*ptr).sub_id);
printf (" \n Duration of the Subject: %s\t ", (*ptr).sub_duration);
printf (" \n Type of the Subject: %s\t ", (*ptr).sub_type);
return 0;
}
Output:
Subject Name: Computer Science
Subject Id: 1201
Duration of the Subject: 6 Months
Type of the Subject: Multiple Choice Question
We constructed the Subject structure in the preceding programme, which has data components such as sub name (char), sub id (int), sub duration (char), and sub type (char) (char). The structure variable is sub, and the structure pointer variable is ptr, which points to the address of the sub variable, as in ptr = &sub. Each *ptr now has access to the address of a member of the Subject structure.
Program to access the structure member using structure pointer and arrow (->) operator
Consider a C programme that uses the pointer and the arrow (->) operator to access structure members.
#include
// create Employee structure
Struct Employee
{
// define the member of the structure
char name[30];
int id;
int age;
char gender[30];
char city[40];
};
// define the variables of the Structure with pointers
Struct Employee emp1, emp2, *ptr1, *ptr2;
Int main()
{
// store the address of the emp1 and emp2 structure variable
ptr1 = &emp1;
ptr2 = &emp2;
printf (" Enter the name of the Employee (emp1): ");
scanf (" %s", &ptr1->name);
printf (" Enter the id of the Employee (emp1): ");
scanf (" %d", &ptr1->id);
printf (" Enter the age of the Employee (emp1): ");
scanf (" %d", &ptr1->age);
printf (" Enter the gender of the Employee (emp1): ");
scanf (" %s", &ptr1->gender);
printf (" Enter the city of the Employee (emp1): ");
scanf (" %s", &ptr1->city);
printf (" \n Second Employee: \n");
printf (" Enter the name of the Employee (emp2): ");
scanf (" %s", &ptr2->name);
printf (" Enter the id of the Employee (emp2): ");
scanf (" %d", &ptr2->id);
printf (" Enter the age of the Employee (emp2): ");
scanf (" %d", &ptr2->age);
printf (" Enter the gender of the Employee (emp2): ");
scanf (" %s", &ptr2->gender);
printf (" Enter the city of the Employee (emp2): ");
scanf (" %s", &ptr2->city);
printf ("\n Display the Details of the Employee using Structure Pointer");
printf ("\n Details of the Employee (emp1) \n");
printf(" Name: %s\n", ptr1->name);
printf(" Id: %d\n", ptr1->id);
printf(" Age: %d\n", ptr1->age);
printf(" Gender: %s\n", ptr1->gender);
printf(" City: %s\n", ptr1->city);
printf ("\n Details of the Employee (emp2) \n");
printf(" Name: %s\n", ptr2->name);
printf(" Id: %d\n", ptr2->id);
printf(" Age: %d\n", ptr2->age);
printf(" Gender: %s\n", ptr2->gender);
printf(" City: %s\n", ptr2->city);
return 0;
}
Output:
Enter the name of the Employee (emp1): John
Enter the id of the Employee (emp1): 1099
Enter the age of the Employee (emp1): 28
Enter the gender of the Employee (emp1): Male
Enter the city of the Employee (emp1): California
Second Employee:
Enter the name of the Employee (emp2): Maria
Enter the id of the Employee (emp2): 1109
Enter the age of the Employee (emp2): 23
Enter the gender of the Employee (emp2): Female
Enter the city of the Employee (emp2): Los Angeles
Display the Details of the Employee using Structure Pointer
Details of the Employee (emp1)
Name: John
Id: 1099
Age: 28
Gender: Male
City: California
Details of the Employee (emp2) Name: Maria
Id: 1109
Age: 23
Gender: Female
City: Los Angeles
In the preceding programme, we constructed an Employee structure with the pointer variables *ptr1 and *ptr2 and two structure variables emp1 and emp2. The members of the structure Employee are name, id, age, gender, and city. The pointer variable and arrow operator, which determine their memory space, are used by all Employee structure members to take their respective values from the user one by one.
Key takeaway
The feature of nesting one structure within another structure in C allows us to design complicated data types.
An array of structures is a collection of many structure variables, each of which contains information on a different entity.
The structure pointer points to the memory block address where the Structure is stored.
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function. Consider the following example, which uses the structural variable employee to call the function display(), which displays an employee's data.
#include<stdio.h>
Struct address
{
char city[20];
int pin;
char phone[14];
};
Struct employee
{
char name[20];
struct address add;
};
Void display(struct employee);
Void main ()
{
struct employee emp;
printf("Enter employee information?\n");
scanf("%s %s %d %s",emp.name,emp.add.city, &emp.add.pin, emp.add.phone);
display(emp);
}
Void display(struct employee emp)
{
printf("Printing the details....\n");
printf("%s %s %d %s",emp.name,emp.add.city,emp.add.pin,emp.add.phone);
}
Self- referential structure
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
The structures pointing to the same type of structures are self-referential in nature.
Struct node {
intdata1;
chardata2;
struct node* link;
};
Int main()
{
struct node ob;
return0;
}
In the above example ‘link’ is a pointer to a structure of type ‘node’. Hence, the structure ‘node’ is a self-referential structure with ‘link’ as the referencing pointer.
An important point to consider is that the pointer should be initialized properly before accessing, as by default it contains garbage value.
Key takeaway
A structure, like other variables, can be provided to a function. We can either pass the structure members or the structure variable all at once into the function.
Self -Referential structures are those structures that have one or more pointers which point to the same type of structure as their member.
In C, the sizeof() operator is frequently used. It determines the number of char-sized storage units used to store the expression or data type. The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis. The data type can be pointer data types or complex data types like unions and structs, in addition to primitive data types like integer and floating data types.
Need of sizeof() operator
The storage size of basic data types is mostly known by programmes. The data type's storage size is constant, but it differs when implemented on different platforms. For example, we dynamically allocate the array space by using sizeof() operator:
Int *ptr=malloc(10*sizeof(int));
The sizeof() operation is applied to the cast of type int in the preceding example. The malloc() method is used to allocate memory and returns a reference to the memory that has been allocated. The memory space is calculated by multiplying the amount of bytes occupied by the int data type by ten.
Depending on the kind of operand, the sizeof() operator operates differently.
● Operand is a data type
● Operand is an expression
When operand is a data type:
#include <stdio.h>
Int main()
{
int x=89; // variable declaration.
printf("size of the variable x is %d", sizeof(x)); // Displaying the size of ?x? variable.
printf("\nsize of the integer data type is %d",sizeof(int)); //Displaying the size of integer data type.
printf("\nsize of the character data type is %d",sizeof(char)); //Displaying the size of character data type.
printf("\nsize of the floating data type is %d",sizeof(float)); //Displaying the size of floating data type.
Return 0;
}
The sizeof() operator is used in the preceding code to print the size of various data types such as int, char, and float.
Output
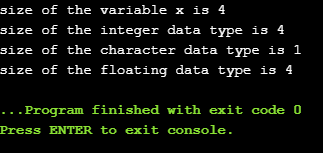
When operand is an expression
#include <stdio.h>
Int main()
{
double i=78.0; //variable initialization.
float j=6.78; //variable initialization.
printf("size of (i+j) expression is : %d",sizeof(i+j)); //Displaying the size of the expression (i+j).
return 0;
}
We created two variables, I and 'j,' of type double and float, respectively, in the following code, and then we used the sizeof(i+j) operator to print the size of the statement.
Output
Size of (i+j) expression is : 8
Typedef keyword
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme. It works similarly to how we construct aliases for commands. In a nutshell, this keyword is used to change the name of a variable that already exists.
Syntax of typedef
Typedef <existing_name> <alias_name>
'existing name' is the name of an already existing variable in the aforementioned syntax, while 'alias name' is another name for the same variable.
If we wish to create a variable of type unsigned int, for example, it will be a time-consuming operation if we want to declare several variables of this kind. We utilise the typedef keyword to solve the problem.
Typedef unsigned int unit;
We used the typedef keyword to declare the unsigned int unit variable in the above statements.
By writing the following statement, we can now create variables of type unsigned int:
Unit a, b;
Rather than writing:
Unsigned int a, b;
So far, we've seen how the typedef keyword provides a convenient shortcut by offering an alternate name for a variable that already exists. This keyword comes in handy when working with lengthy data types, particularly structure declarations.
Example
#include <stdio.h>
Int main()
{
Typedef unsigned int unit;
Unit i,j;
i=10;
j=20;
Printf("Value of i is :%d",i);
Printf("\nValue of j is :%d",j);
Return 0;
}
Output
Value of i is :10
Value of j is :20
Key takeaway
The sizeof() operator has only one operand, which can either be an expression or a data typecast with the cast encapsulated in parenthesis.
The typedef keyword is used in C programming to give meaningful names to variables that already exist in the programme.
Unions are quite similar to the structures in C. Union is also a derived type as structure. Union can be defined in same manner as structures just the keyword used in defining union in union where keyword used in defining structure was struct.
Union car
{
Char name[50];
Int price;
};
Union variables can be created in similar manner as structure variable.
Union car
{
Char name[50];
Int price;
}c1, c2, *c3;
OR;
Union car
{
Char name[50];
Int price;
};
-------Inside Function-----------
Union car c1, c2, *c3;
In both cases, union variables c1, c2 and union pointer variable c3 of type union car is created.
Accessing members of a union
- Array elements are accessed using the Subscript variable, Similarly Union members are accessed using dot [.] operator.
- (.) is called as “union member Operator”.
- Use this Operator in between “Union variable”&“member name”
Union employee
{
Int id;
Char name[50];
Float salary;
} ;
Void main()
{
Union employee e1= { 1, “ABC”, 50000 };
Printf(“%d”, e. Id);
Printf(“%s”, e. Name);
}
O/P-
Garbage value, ABC
Key takeaway
Unions are quite similar to the structures in C. Union is also a derived type as structure.
Structure | Union |
A structure can be described with the struct keyword. | A union can be described using the union keyword. |
Every member of the structure has their own memory spot. | A memory location is shared by all data participants of a union. |
Changing the importance of one data member in the structure has no effect on the other data members. | When the value of one data member is changed, the value of the other data members in the union is also changed. |
It allows you to initialize several members at the same time. | It allows you to only initialize the first union member. |
The structure's total size is equal to the amount of each data member's size. | The largest data member determines the union's total size. |
It is primarily used to store different types of data. | It is primarily used to store one of the numerous data types available. |
It takes up space for every member mentioned in the inner parameters. | It takes up space for the member with the largest size in the inner parameters. |
It allows you to build a versatile collection. | A versatile array is not supported. |
Any member can be retrieved at any time. | In the union, you can only access one member at a time. |
Most of the programs are written to store the information fetched from the program. One such way is to store the fetched information in a file. Different operations that can be performed on a file are:
- Creation of a new file (fopen with attributes as “a” or “a+” or “w” or “w++”)
- Opening an existing file (fopen)
- Reading from file (fscanf or fgets)
- Writing to a file (fprintf or fputs)
- Moving to a specific location in a file (fseek, rewind)
- Closing a file (fclose)
The text in the brackets denotes the functions used for performing those operations.
Functions in File Operations:
File opening modes in C:
“r” – Searches file. If the file is opened successfully, fopen( ) loads it into memory and sets up a pointer which points to the first character in it. If the file cannot be opened, fopen( ) returns NULL.
“w” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“a” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer that points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
“r+” – Searches file. If is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the first character in it. Returns NULL, if unable to open the file.
“w+” – Searches file. If the file exists, its contents are overwritten. If the file doesn’t exist a new file is created. Returns NULL, if unable to open file.
“a+” – Searches file. If the file is opened successfully fopen( ) loads it into memory and sets up a pointer which points to the last character in it. If the file doesn’t exist, a new file is created. Returns NULL, if unable to open file.
As given above, if you want to perform operations on a binary file, then you have to append ‘b’ at the last. For example, instead of “w”, you have to use “wb”, instead of “a+” you have to use “a+b”. For performing the operations on the file, a special pointer called File pointer is used which is declared as
FILE *filePointer;
So, the file can be opened as
FilePointer = fopen(“fileName.txt”, “w”)
The second parameter can be changed to contain all the attributes listed in the above table.
Reading from a file –
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Writing a file –:
The file write operations can be perfomed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Closing a file:
After every successful fie operations, you must always close a file. For closing a file, you have to use fclose function. The snippet for closing a file is given as :
FILE *filePointer;
FilePointer= fopen(“fileName.txt”, “w”);
---------- Some file Operations -------
Fclose(filePointer)
Example 1: Program to Open a File, Write in it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Write in it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Get the data to be written in file
Char dataToBeWritten[50]
= "GeeksforGeeks-A Computer Science Portal for Geeks";
// Open the existing file GfgTest.c using fopen()
// in write mode using "w" attribute
FilePointer = fopen("GfgTest.c", "w") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Write the dataToBeWritten into the file
If ( strlen ( dataToBeWritten ) > 0 )
{
// writing in the file using fputs()
Fputs(dataToBeWritten, filePointer) ;
Fputs("\n", filePointer) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully written in file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
Example 2: Program to Open a File, Read from it, And Close the File
Filter_none
Brightness_4
// C program to Open a File,
// Read from it, And Close the File
# include <stdio.h>
# include <string.h>
Int main( )
{
// Declare the file pointer
FILE *filePointer ;
// Declare the variable for the data to be read from file
Char dataToBeRead[50];
// Open the existing file GfgTest.c using fopen()
// in read mode using "r" attribute
FilePointer = fopen("GfgTest.c", "r") ;
// Check if this filePointer is null
// which maybe if the file does not exist
If ( filePointer == NULL )
{
Printf( "GfgTest.c file failed to open." ) ;
}
Else
{
Printf("The file is now opened.\n") ;
// Read the dataToBeRead from the file
// using fgets() method
While( fgets ( dataToBeRead, 50, filePointer ) != NULL )
{
// Print the dataToBeRead
Printf( "%s" , dataToBeRead ) ;
}
// Closing the file using fclose()
Fclose(filePointer) ;
Printf("Data successfully read from file GfgTest.c\n");
Printf("The file is now closed.") ;
}
Return 0;
}
File I/O functions
Functions used in File Handling are as follows:
1. Open
● Before opening a file it should be linked to appropriate stream i.e. input, output or input/output.
● If we want to create input stream we will write
Ifstream infile;
For output stream we will write
Ofstream outfile;
For Input/Output stream we will write
Fstream iofile;
● Assume we want to write data to file named “Example” so we will use output stream. Then file can be opened as follows,
Ofstream outfile;
Outfile.open(“Example”);
● Now if we want to read data from file, so we will use input stream. Then file can be opened as follows,
Ifstream infile;
Infile.open(“Example”);
2. Close()
We use member function close() to close a file. The close function neither takes parameters nor returns any value.
e.g. Outfile.close();
Program to illustrate working with files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Char str1[50];
Ofstream fout; // create out stream
Fout.open(“Sample”); //opening file named “Sample” with open function
Cout<<”Enter string”;
Cin>>str;//accepting string from user
Fout<<str<<\n”; //writing string to “Sample”file
Fout.close(); //closing “Sample” file
Ifstream fin; //create in stream
Fin.open(“Sample”); // open “Sample” file for reading”
Fin>>str; reading string from “Sample” file
Cout<<”String is”<<str;// display string to user
Fin.close();
Return 0 ;
}
Output
Enter String
C++
String is C++
3. Read()
● It is used to read binary file.
● It is written as follows.
In.read((char *)&S, sizeof(S));
Where “in” is input stream.
‘S’ is variable.
● It takes two arguments. First argument is the address of variable S and second is length of variable S in bytes.
4. Write()
● It is used to write binary file.
● It is written as follows
Out.write((char *)&S, sizeof(S));
Where “out” is output streams and ‘S’ is variable.
Program to illustrate I/O operations (read and write) on binary files.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int marks[5],i;
Cout<<”Enter marks”;
For(i=0;i<=5;i++)
Cin>>marks[i];
Ofstream fout;
Fout.open(“Example”);// open file for writing
Fout.write((char*)& marks, sizeof(marks));// write data to file
Fout.close();
Ifstream fin;
Fin.open(“Example”); // open file for reading
Fin.read((char*)& marks, sizeof (marks));// reading data from file
Cout<<”Marks are”;
For(i=0;i<=5;i++)
Cout<<marks[i]; //displaying data to screen
Fin.close();
Return 0 ;
}
5. Detecting end of file
● If there is no more data to read in a file, this condition is called as end of file.
● End of file in C++ can be detected by using function eof().
● It is a member function of ios class.
● It returns a non zero value (true) if end of file is encountered, otherwise returns zero.
Program to illustrate eof() function.
#include<iostream>
Using namespace std;
#include<fstream>
Int main()
{
Int x;
Ifstream fin; // declares stream
Fin.open(“myfile”, ios::in);// open file in read mode
Fin>>x;//get first number
While(!fin.eof())//if not at end of file, continue
{ reading numbers
Cout<<x<<endl;//print numbers
Fin>>x;//get another number
}
Fin.close();//close file
Return 0 ;
}
Binary files
Executables, sound files, image files, object files, and other types of binary files are all examples of binary files. The fact that each byte of a binary file can be one of 256-bit patterns is what makes them binary. Binary encoding, also known as BCP (Binary Communications Protocol), consists of values ranging from 0 to 255.
Binary files are made up of a long string of the characters "1" and "0" arranged in complex patterns. In contrast to ASCII, these characters can be used to construct any type of data, including text and images. In binary encoding, most language elements, such as integers, real numbers, and operator names, are expressed by fewer characters than in ASCII encoding.
Reading data from text files:
We can use the following methods to read text from text files:
Read() - to read total data from file
Read(n)- to read n char from a file
Read line()- to read only one line
Read line()- to read at lines into a list
Eg:
Code: Read all data
F = open(‘abc:txt’,’r’)
Data = f.read()
Print(data)
f.close()
Output: Contents of ‘abc.txt’ will be printed
Eg:
Code read lines:
F = open(‘abc.txt’,’r’)
Lines =f.read lines()
For line in lines:
Print (line,end = “ “)
F.close ()
Writing Data to text files:
We can write character data to text files by using the following 2 methods.
1)Write (str)
2)Write lines (list of lines)
Eg: f=open(‘xyz.txt’,’w’)
f.write(‘SAE In’)
f.write(‘Kondhwa In’)
f.write(‘Pune’)
Print(‘data are written successfully’)
f.close()
Note: In the given code, data present in the file will be overridden every time if w run the program. Instead of overriding if we want append operation then we should open the file as follows.
The file read operations can be performed using functions fscanf or fgets. Both the functions performed the same operations as that of scanf and gets but with an additional parameter, the file pointer. So, it depends on you if you want to read the file line by line or character by character.
And the code snippet for reading a file is as:
FILE * filePointer;
FilePointer = fopen(“fileName.txt”, “r”);
Fscanf(filePointer, "%s %s %s %d", str1, str2, str3, &year);
Write
The file write operations can be performed by the functions fprintf and fputs with similarities to read operations. The snippet for writing to a file is as:
FILE *filePointer ;
FilePointer = fopen(“fileName.txt”, “w”);
Fprintf(filePointer, "%s %s %s %d", "We", "are", "in", 2012);
Append
Create a C application that reads data from the user and writes it to a file. In C programming, how to add data to the end of a file. In this piece, I'll go through how to handle files in append mode. I'll show you how to use C's append file mode to append data to a file.
Example
Source file content
I love programming.
Programming with files is fun.
String to append
Learning C programming at Codeforwin is simple and easy.
Output file content after append
I love programming.
Programming with files is fun.
Learning C programming at Codeforwin is simple and easy.
Getc()
To read a character from a file, use the getc() function.
The getc() method has the following syntax:
Char getc (FILE *fp);
Example
FILE *fp;
Char ch;
Ch = getc(fp);
Putc()
To write a character into a file, use the putc() function.
The putc() method has the following syntax:
Putc (char ch, FILE *fp);
Example,
FILE *fp;
Char ch;
Putc(ch, fp);
Example for putc() and getc() functions
#include<stdio.h>
Int main(){
char ch;
FILE *fp;
fp=fopen("std1.txt","w"); //opening file in write mode
printf("enter the text.press cntrl Z:\n");
while((ch = getchar())!=EOF){
putc(ch,fp); // writing each character into the file
}
fclose(fp);
fp=fopen("std1.txt","r");
printf("text on the file:\n");
while ((ch=getc(fp))!=EOF){ // reading each character from file
putchar(ch); // displaying each character on to the screen
}
fclose(fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
Enter the text.press cntrl Z:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
^Z
Text on the file:
Hi Welcome to TutorialsPoint
Here I am Presenting Question and answers in C Programming Language
Getw()
When reading a number from a file, the getw() function is utilized.
The getw() method has the following syntax:
Syntax
Int getw (FILE *fp);
Example
FILE *fp;
Int num;
Num = getw(fp);
Putw()
The putw() function is used to save a number to a file.
The putw() method has the following syntax:
Syntax
Putw (int num, FILE *fp);
Example
FILE *fp;
Int num;
Putw(num, fp);
Example for using putw() and getw()
#include<stdio.h>
Int main( ){
FILE *fp;
int i;
fp = fopen ("num.txt", "w");
for (i =1; i<= 10; i++){
putw (i, fp);
}
fclose (fp);
fp =fopen ("num.txt", "r");
printf ("file content is\n");
for (i =1; i<= 10; i++){
i= getw(fp);
printf ("%d",i);
printf("\n");
}
fclose (fp);
return 0;
}
Output
When the above program is executed, it produces the following result −
File content is
1
2
3
4
5
6
7
8
9
10
Gets()
The function gets () reads a line from the keyboard.
Declaration: char *gets (char *string)
The gets function is used to read a string (a series of characters) from the keyboard. We can read the string from standard input/keyboard in a C programme as shown below.
Gets (string);
Puts()
The puts () function displays a line on the o/p screen.
Declaration: int fputs (const char *string, FILE *fp)
The fputs method saves a string to a file named fp. As shown below, in a C programme, we write a string to a file.
Fputs (fp, “some data”);
Scanf()
We utilise the scanf() function to get formatted or standard inputs so that the printf() function can give the programme a variety of conversion possibilities.
Syntax for scanf()
Scanf (format_specifier, &data_a, &data_b,……); // Here, & refers to the address operator
The scanf() function's goal is to read the characters from the standard input, convert them according to the format string, and then store the accessible inputs in the memory slots represented by the other arguments.
Example of scanf()
Scanf(“%d %c”, &info_a,&info_b);
The '&' character does not preface the data name when considering string data names in a programme.
Sscanf()
Int sscanf(const char *str, const char *format,...) is a C function that reads formatted input from a string.
Declaration
The sscanf() function is declared as follows.
Int sscanf(const char *str, const char *format, ...)
Parameters
● str - This is the C string that the function uses as its data source.
● format - is a C string that contains one or more of the items listed below: Format specifiers, whitespace characters, and non-whitespace characters
This prototype is followed by a format specifier: [=%[*] [width] [modifiers]type=]
Sr.No. | Argument & Description |
1 | * This is an optional initial asterisk that signals that the data from the stream will be read but not saved in the appropriate parameter. |
2 | Width The maximum amount of characters to be read in the current reading operation is specified here. |
3 | Modifiers For the data pointed by the corresponding additional argument, specifies a size other than int (in the case of d, I and n), unsigned int (in the case of o, u, and x), or float (in the case of e, f, and g): h: unsigned short int (for d, I and n) or short int (for d, I and n) (for o, u and x) l: long integer (for d, I and n), or unsigned long integer (for o, u, and x), or double int (for e, f and g) L stands for long double (for e, f and g). |
4 | Type A character that specifies the type of data to be read as well as how it should be read. |
Fscanf()
To read a collection of characters from a file, use the fscanf() method. It reads a single word from the file and returns EOF when the file reaches the end.
Syntax:
Int fscanf(FILE *stream, const char *format [, argument, ...])
Example:
#include <stdio.h>
Main(){
FILE *fp;
char buff[255];//creating char array to store data of file
fp = fopen("file.txt", "r");
while(fscanf(fp, "%s", buff)!=EOF){
printf("%s ", buff );
}
fclose(fp);
}
Output:
Hello file by fprintf...
Fread()
The fread() function is the fwrite() function's counterpart. To read binary data, the fread() function is widely used. It takes the same parameters as the fwrite() function. The fread() method has the following syntax:
Syntax:
Size_t fread(void *ptr, size_t size, size_t n, FILE *fp);
After reading from the file, the ptr is the starting address of the RAM block where data will be stored. The function reads n items from the file, each of which takes up the amount of bytes indicated in the second argument. It reads n items from the file and returns n if it succeeds. It returns a number smaller than n in the event of an error or the end of the file.
Example
Reading a structure array
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student arr_student[100];
Fread(&arr_student, sizeof(struct student), 10, fp);
This reads the first 10 struct student elements from the file and places them in the arr_studen variable.
Printf()
For generating formatted or standard outputs in compliance with a format definition, we use the printf() function. The printf function's parameters are the output data and the format specification string ().
Syntax for printf()
Printf (format_specifiers, info_a, info_b,…….. );
Example of printf()
Printf(“%d %c”, info_a, info_b);
The conversion character is the character we define after the 'percent' character. It's because 'percent' permits any data type to be converted into another data type for further printing.
Conversion Character | Description and Meaning of Character |
c | The data is taken in the form of characters by the application. |
d | The data is converted into integers using this software (decimals). |
f | The application generates data in the form of a double or a float with a Precision 6 by default. |
s | Unless and until the programme encounters a NULL character, the programme recognises the data as a string and prints a single character from it. |
Sprintf()
"String print" is what sprintf stands for. It is a file handling function in the C programming language that is used to send formatted output to a string. Instead of publishing to the terminal, the sprintf() function saves the output to the sprintf char buffer.
Syntax
Int sprintf(char *str, const char *format, ...)
Parameter values
The sprintf() method accepts the following parameter values as input:
● str: This is a pointer to an array of char elements that holds the resultant string. It's where you'll place the data.
● format: This is a C string that describes the output and includes placeholders for the integer arguments that will be added into the formatted string. It refers to the string containing the text that will be written to the buffer. It is made up of characters and optional format specifiers beginning with %.
Example
In this example, the variable num is of the type float. The sprintf() function converts the values in the num variable into a string, which is then put in buffer.
#include<stdio.h>
Int main() {
float num = 9.9;
printf("Before using sprintf(), data is float type: %f\n", num);
char buffer[50]; //for storing the converted string
sprintf(buffer, "%f", num);
printf("After using sprintf() data is string type: %s", buffer);
}
Output:
Before using sprintf(), data is float type: 9.900000
After using sprintf() data is string type: 9.900000
Fprint()
To write a set of characters into a file, use the fprintf() function. It generates formatted output and transmits it to a stream.
Syntax:
Int fprintf(FILE *stream, const char *format [, argument, ...])
Example
#include <stdio.h>
Main(){
FILE *fp;
fp = fopen("file.txt", "w");//opening file
fprintf(fp, "Hello file by fprintf...\n");//writing data into file
fclose(fp);//closing file
}
Fwrite()
Syntax:
Size_t fwrite(const void *ptr, size_t size, size_t n, FILE *fp);
The fwrite() function writes data to the file that is specified by the void pointer ptr.
● ptr: it refers to the memory block that holds the data to be written.
● size: This determines the number of bytes to be written for each item.
● n: The number of things to be written is denoted by the letter n.
● fp: This is a reference to the file that will be used to store data items.
It returns the number of items successfully written to the file if it succeeds. It returns a number smaller than n if there is an error. The two inputs (size and n) and return value of fwrite() are of type size t, which is an unsigned int on most systems.
Example:
Writing structure
Struct student
{
char name[10];
int roll;
float marks;
};
Struct student student_1 = {"Tina", 12, 88.123};
Fwrite(&student_1, sizeof(student_1), 1, fp);
The contents of variable student 1 are written to the file.
Fseek()
To set the file pointer to a certain offset, use the fseek() function. It's used to save data to a file at a certain location.
Syntax:
Int fseek(FILE *stream, long int offset, int whence)
Whence, the fseek() function uses three constants: SEEK SET, SEEK CUR, and SEEK END.
Example
#include <stdio.h>
Void main(){
FILE *fp;
fp = fopen("myfile.txt","w+");
fputs("This is javatpoint", fp);
fseek( fp, 7, SEEK_SET );
fputs("sonoo jaiswal", fp);
fclose(fp);
}
Output
This is sonam jaiswal
Ftell()
The ftell() function returns the supplied stream's current file position. After shifting the file pointer to the end of the file, we can use the ftell() function to retrieve the total size of the file. The SEEK END constant can be used to relocate the file pointer to the end of the file.
Syntax:
Long int ftell(FILE *stream)
Example
#include <stdio.h>
#include <conio.h>
Void main (){
FILE *fp;
int length;
clrscr();
fp = fopen("file.txt", "r");
fseek(fp, 0, SEEK_END);
length = ftell(fp);
fclose(fp);
printf("Size of file: %d bytes", length);
getch();
}
Output:
Size of file: 21 bytes
Fflush()
The fflush function in the C programming language sends any unwritten data to the stream's buffer. The fflush function will flush any streams with unwritten data in the buffer if stream is a null pointer.
Syntax
The fflush function in C has the following syntax:
Int fflush(FILE *stream);
Stream
The stream should be flushed.
Returns
If the fflush function is successful, it returns zero; otherwise, it returns EOF.
Fclose()
To close a file, use the fclose() method. After you've completed all of the procedures on the file, you must close it. The following is the syntax for the fclose() function:
Int fclose( FILE *fp );
Rewind()
The file pointer is set to the beginning of the stream using the rewind() function. It's useful if you'll be using stream frequently.
Syntax:
Void rewind(FILE *stream)
Example
#include<stdio.h>
#include<conio.h>
Void main(){
FILE *fp;
Char c;
Clrscr();
Fp=fopen("file.txt","r");
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Rewind(fp);//moves the file pointer at beginning of the file
While((c=fgetc(fp))!=EOF){
Printf("%c",c);
}
Fclose(fp);
Getch();
}
Output:
This is a simple textthis is a simple text
The rewind() function, as you can see, moves the file pointer to the beginning of the file, which is why "this is simple text" is printed twice. If the rewind() function is not used, "this is simple text" will only be printed once.
References:
- The C- Programming Language - By Brian W Kernighan and Dennis Ritchie
- C- Programming in an open source paradigm: By R.K. Kamat, K .S. Oza, S.R. Patil
- The GNU C Programming Tutorial -By Mark Burgess
- Let us C- By Yashwant Kanetkar