Unit - 3
Inheritance, packages and interfaces
Inheritance is one of the main principles of OOP language. By using this principle one can inherit all the properties of other class. Inheritance allows us to manage information in a hierarchal manner.
Inheritance use two types of class: Superclass and subclass. Superclass also known as parent or base class is the class whose properties are inherited while subclass also known as derived or child class is the class who inherits the properties.
To inherit a class we simply use the extend keyword which allows to inherit the definition of class to another as shown below:
Class subclass-name extends superclass-name {
// body of class
}
Lets look at a simple example of inheritance:
Class First {// Superclass.
Int x, y;
Void showxy() {
System.out.println("x and y: " + x + " " + y);
}
}
Class Second extends First {// Subclass Second by extending class First.
Int z;
Void showz() {
System.out.println("z: " + z);
}
Void sum() {
System.out.println("x+y+z: " + (x+y+z));
}
}
Class Example {
Public static void main(String args[]) {
First superOb = new First();
Second subOb = new Second();
SuperOb.x = 100;
SuperOb.y = 200;
System.out.println("Data of superclass: ");
SuperOb.showxy();
System.out.println();
/* The subclass can access all public members of its superclass. */
SubOb.x = 45;
SubOb.y = 10;
SubOb.z = 5;
System.out.println("Contents of subOb: ");
SubOb.showxy();
SubOb.showz();
System.out.println();
System.out.println("Sum of i, j and k in subOb:");
SubOb.sum();
}
}
Output
Data of superclass:
x and y: 100 200
Contents of subOb:
x and y: 45 10
z: 5
Sum of x, y and z in subOb:
x+y+z: 60
Types of Inheritance
1. Single Inheritance
Also called as single level inheritance it is the mechanism of deriving a single sub class from a super class. The subclass will be defined by the keyword extends which signifies that the properties of the super class are extended to the sub class. The sub class will contain its own variables and methods as well as it can access methods and variables of the super class.
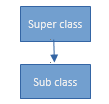
Syntax:
class Subclass-name extends Superclass-name
{
//methods and fields
}
Program:
Class x
{
Void one()
{
System.out.println("Printing one");
}
}
Class y extends x
{
Void two()
{
System.out.println("Printing two");
}
}
Public class test
{
Public static void main(String args[])
{
y t= new y();
t.two();
t.one();
}
}
Output:
Printing two
Printing one
2. Multi- level Inheritance
It is the mechanism of deriving a sub class from a super class and again a sub class is derived from this derived subclass which acts as a base class for the newly derived class. It includes a multi level base class which allows building a chain of classes where a derived class acts as super class.
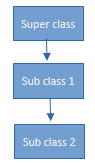
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}class three extends two
{
Void print_three()
{
System.out.println("Printing three");}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
t.print_three();
t.print_two(); t.print_one();
}
}
Output:
Printing three
Printing two
Printing one
3. Hierarchical Inheritance
In Hierarchical Inheritance, one class serves as a super class (base class) for more than one sub class.
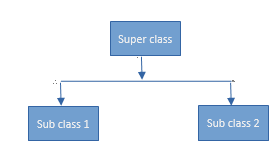
Program:
Class one
{
Void print_one()
{
System.out.println("Printing one");
}
}
Class two extends one
{
Void print_two()
{
System.out.println("Printing two");
}
}
Class three extends two
{
Void print_three()
{
System.out.println("Printing three");
}
}
Public class test1
{
Public static void main(String args[])
{
Three t= new three();
Two n= new two();t.print_three();
n.print_two();
t.print_one();
}}
Output:
Printing three
Printing two
Printing one
4. Hybrid Inheritance
It is the combination of Single inheritance and multi level inheritance.
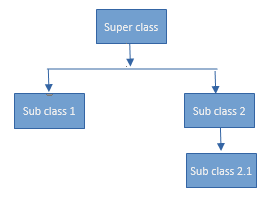
Significance of Inheritance
Inheritance is one of the most significant concept of object oriented programming which allow a class to inherit its properties to other class. This makes it easier to deploy and maintain an application.
Another benefit of using inheritance is code reusability. Code reusability means avoiding unnecessary code. In inheritance code can be used by other class and the subclass have to write its own unique properties only and rest of the properties will be inherited from the parent class or superclass.
Method overriding is also one benefit of using inheritance. Here a child class creates a method of same type as in parent class. In method overriding when we call the method, the child class method will be called. To call the parent class method we have to use super keyword.
In Java, method overriding is a technique through which a subclass or derived class can provide its own version of method whose implementation is given by one its super class.
By doing this a subclass is defining a method having same name, same parameter, and same return type similar to method in the superclass. Then the method in the sublass is said to override the method in the superclass.
The concept of runtime polymorphism in Java can be implemented using Method overriding.
The next important question is which class method will be executed. So the answer is
- When an object of a parent class makes a call to method, then the parent class method will be executed.
- When an object of a child class makes a call to method, then the child class method will be executed.
Hence, the type of object used for referring determines which version of the method will be executed in the case of overridden method.
A simple example showing how overridden method are created and called.
//Parent class declaration
Class Parent{
Void display()//method defined in parent class
{
System.out.println(Method of parent class”);
}
}
//Child class declaration
Class Child extends Parent{
Void display()//child class method overrides parent class method
{
System.out.println(Method of child class”);
}
}
Class Demo{
Public static void main(String[] args)
{
Parent p = new Parent(); // object of parent class
Parent o = new Child();// object of child class
p.display(); //reference made to parent class method by object p
o.display(); //reference made to child class method by object o
}
}
Output
Method of parent class
Method of child class
Rules for Method Overriding
- Overridden method must have same name and parameters.
- Method which is declared as final cannot be overridden.
- Static methods cannot be used to override.
- Overridden is not possible in private method.
- The return type of overriding method must be same.
- Constructors cannot be override.
- IS-A relationship must be fulfill in method overridden technique.
It refers to immediate parent class object. There are 3 ways to use the ‘super’ keyword:
- To refer immediate parent class instance variable.
2. To invoke immediate parent class method.
3. To invoke immediate parent class constructor.
Program to refer immediate parent class instance variable using super keyword
Class transport
{
Int maxspeed = 200;
}
Class vehicle extends transport
{
Int maxspeed =100;
}
Class car extends vehicle
{
Int maxspeed = 120;
Void disp()
{System.out.println("Max speed= "+super.maxspeed);
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.disp();
}
}
Output:
Max speed= 100
Program to invoke immediate parent class method
Class transport
{
Void message()
{
System.out.println("transport");
}
}
Class vehicle extends transport
{
Void message()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Int maxspeed = 120;
Void message()
{
System.out.println("car");
}
Void display()
{
Message();
Super.message();
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
c.display();}
}
Output:
Car
Vehicle
Program to invoke immediate parent class constructor
Class vehicle
{
Vehicle()
{
System.out.println("vehicle");
}
}
Class car extends vehicle
{
Car()
{
Super();
System.out.println("car");
}
}
Class veh
{
Public static void main(String arg[])
{
Car c = new car();
}
}
Output:
Vehicle
Car
Difference between Method Overloading and Method Overriding are:
S.NO | Method Overloading | Method Overriding |
1. | Method overloading is a compile-time polymorphism. | Method overriding is a run-time polymorphism. |
2. | It helps to increase the readability of the program. | It is used to grant the specific implementation of the method which is already provided by its parent class or superclass. |
3. | It occurs within the class. | It is performed in two classes with inheritance relationships. |
4. | Method overloading may or may not require inheritance. | Method overriding always needs inheritance. |
5. | In method overloading, methods must have the same name and different signatures. | In method overriding, methods must have the same name and same signature. |
6. | In method overloading, the return type can or can not be the same, but we just have to change the parameter. | In method overriding, the return type must be the same or co-variant. |
When a call to overridden method is made at run time instead of compile time, then this mechanism is known as Dynamic method dispatch. This is an example of run time polymorphism.
Like method overridden, here also the type of object decides which version of the method to be executed.
Advantages of using dynamic method dispatch are:
- It allows java to support runtime polymorphism.
- By using this, class methods can be shared by derived class methods with their own specific implementation.
- It allows subclass to create their own functionality in the derived method.
Abstract Classes
Abstract classes are the same as normal Java classes the difference is only that an abstract class uses abstract keyword while the normal Java class does not use.
We use the abstract keyword before the class name to declare the class as abstract.
An abstract class contains abstract methods as well as concrete methods.
If we want to use an abstract class, we have to inherit it from the base class.
If the class does not have the implementation of all the methods of the interface, we should declare the class as abstract. It provides complete abstraction. It means that fields are public static and final by default and methods are empty.
The syntax of abstract class is:
Public abstract class ClassName
{
Public abstract methodName();
}
Example of car:
Abstract class Car
{ //abstract method
Abstract void start();
//non-abstract method
Public void stop()
{
System.out.println("The car engine is not started.");
}
} //inherit abstract class
Public class Owner extends Car
{ //defining the body of the abstract method of the abstract class
Void start()
{
System.out.println("The car engine has been started.");
}
Public static void main(String[] args)
{ Owner obj = new Owner();
//calling abstract method
Obj.start();
//calling non-abstract method
Obj.stop();
}
}
OUTPUT:
The car has been started.
The car engine is not started.
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
When you want a variable to constantly store the same value, such as PI, the final keyword comes in handy (3.14159...).
The last keyword is referred to as a "modifier."
Example
Set a variable to final, to prevent it from being overridden/modified:
Public class Main {
Final int x = 10;
Public static void main(String[] args) {
Main myObj = new Main();
MyObj.x = 25; // will generate an error: cannot assign a value to a final variable
System.out.println(myObj.x);
}
}
Using Final with Inheritance
- The final keyword is final, which means we can't change anything.
- Variables, methods, and classes can all benefit from final keywords.
- If we use the final keyword for inheritance, that is, if we declare any method in the base class with the final keyword, the final method's implementation will be the same as in the derived class.
- If any other class extends this subclass, we can declare the final method in any subclass we wish.
Declare final variable with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final variable pa of string type i.e
The value of this variable is fixed throughout all
The derived classes or not overridden*/
Final String pa = "Hello , We are in parent class variable";
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of variable ch of string type i.e
The value of this variable is not fixed throughout all
The derived classes or overridden*/
String ch = "Hello , We are in child class variable";
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a variable pa by parent object
System.out.println(p.pa);
// Creation of Child class object
Child c = new Child();
// Calling a variable ch by Child object
System.out.println(c.ch);
// Calling a variable pa by Child object
System.out.println(c.pa);
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, We are in parent class variable
Hello, We are in child class variable
Hello, We are in parent class variable
Declare final methods with inheritance
// Declaring Parent class
Class Parent {
/* Creation of final method parent of void type i.e
The implementation of this method is fixed throughout
All the derived classes or not overridden*/
Final void parent() {
System.out.println("Hello , we are in parent method");
}
}
// Declaring Child class by extending Parent class
Class Child extends Parent {
/* Creation of final method child of void type i.e
The implementation of this method is not fixed throughout
All the derived classes or not overridden*/
Void child() {
System.out.println("Hello , we are in child method");
}
}
Class Test {
Public static void main(String[] args) {
// Creation of Parent class object
Parent p = new Parent();
// Calling a method parent() by parent object
p.parent();
// Creation of Child class object
Child c = new Child();
// Calling a method child() by Child class object
c.child();
// Calling a method parent() by child object
c.parent();
}
}
Output
D:\Programs>javac Test.java
D:\Programs>java Test
Hello, we are in parent method
Hello, we are in child method
Hello, we are in parent method
Key takeaway
The final keyword is a non-access modifier that prevents classes, attributes, and methods from being changed (impossible to inherit or override).
In Java, an interface is a reference type. It's comparable to a class. It consists of a set of abstract methods. When a class implements an interface, it inherits the interface's abstract methods.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods. Method bodies are only available for default and static methods.
The process of creating an interface is identical to that of creating a class. A class, on the other hand, describes an object's features and behaviors. An interface, on the other hand, contains the behaviors that a class implements.
Unless the interface's implementation class is abstract, all of the interface's methods must be declared in the class.
In the following ways, an interface is analogous to a class:
● Any number of methods can be included in an interface.
● An interface is written in a file with the extension.java, with the interface's name matching the file's name.
● A.class file contains the byte code for an interface.
● Packages have interfaces, and the bytecode file for each must be in the same directory structure as the package name.
Why do we use interfaces?
There are three primary reasons to utilize an interface. Below is a list of them.
● It's a technique for achieving abstraction.
● We can offer various inheritance functionality via an interface.
● It's useful for achieving loose coupling.
Declaration
The interface keyword is used to declare an interface. All methods in an interface are declared with an empty body, and all fields are public, static, and final by default. A class that implements an interface is required to implement all of the interface's functions.
Syntax
Interface <interface_name>{
// declare constant fields
// declare methods that abstract
// by default.
}
Key takeaway
In Java, an interface is a reference type.
An interface may also have constants, default methods, static methods, and nested types in addition to abstract methods.
Implement interfaces
When a class implements an interface, it's like the class signing a contract, agreeing to perform the interface's defined actions. If a class doesn't perform all of the interface's actions, it must declare itself abstract.
To implement an interface, a class utilizes the implements keyword. Following the extends component of the declaration, the implements keyword appears in the class declaration.
Example
/* File name : MammalInt.java */
Public class MammalInt implements Animal {
public void eat() {
System.out.println("Mammal eats");
}
public void travel() {
System.out.println("Mammal travels");
}
public int noOfLegs() {
return 0;
}
public static void main(String args[]) {
MammalInt m = new MammalInt();
m.eat();
m.travel();
}
}
Output
Mammal eats
Mammal travels
There are a few principles to follow when overriding methods specified in interfaces.
● Checked exceptions should not be declared on implementation methods other than those specified by the interface method or their subclasses.
● When overriding the methods, keep the signature of the interface method and the same return type or subtype.
● Interface methods do not need to be implemented if the implementation class is abstract.
When it comes to implementing interfaces, there are a few guidelines to follow.
● At any given time, a class can implement multiple interfaces.
● A class can only extend one other class while implementing several interfaces.
● In the same manner that a class can extend another class, an interface can extend another interface.
If a class implements multiple interfaces, or an interface extends multiple interfaces, it is known as multiple inheritance.
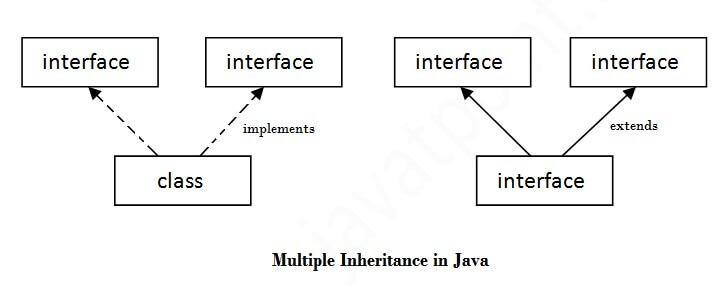
Interface Printable
{
Void print();
}
Interface Showable {
Void show();
}
Class A7 implements Printable,Showable
{ public void print(){System.out.println("Hello"); }
Public void show()
{ System.out.println("Welcome"); }
Public static void main(String args[])
{
A7 obj = new A7();
Obj.print();
Obj.show();
}
}
OUTPUT:
Hello
Welcome
Both abstract classes and interfaces are used to achieve abstraction so that abstract methods can be declared. Both the abstract class and the interface cannot be instantiated.
However, there are other distinctions between abstract class and interface, which are shown below.
Abstract class | Interface |
1) There are abstract and non-abstract methods in an abstract class. | Only abstract methods are allowed in an interface. It can also contain default and static methods with Java 8. |
2) Multiple inheritance is not supported by abstract classes. | Multiple inheritance is supported by the interface. |
3) Variables in an abstract class can be final, non-final, static, or non-static. | Only static and final variables are used in the interface. |
4) Interface implementation can be provided via an abstract class. | The implementation of an abstract class cannot be provided by an interface. |
5) To declare an abstract class, use the abstract keyword. | To declare an interface, use the interface keyword. |
6) An abstract class can implement numerous Java interfaces by extending another Java class. | Only another Java interface can be extended by an interface. |
7) The keyword "extends" can be used to extend an abstract class. | The keyword "implements" can be used to create an interface. |
8) Private, protected, and other class members can be found in a Java abstract class. | By default, members of a Java interface are public. |
9)Example: Public abstract class Shape{ Public abstract void draw(); } | Example: Public interface Drawable{ Void draw(); } |
A java package is a group of similar types of classes, interfaces and sub-packages.
Package in java can be categorized in two form, built-in package and user-defined package.
There are many built-in packages such as java, lang, awt, javax, swing, net, io, util, sql etc.
Advantage of Java Package
1) Java package is used to categorize the classes and interfaces so that they can be easily maintained.
2) Java package provides access protection.
3) Java package removes naming collision.
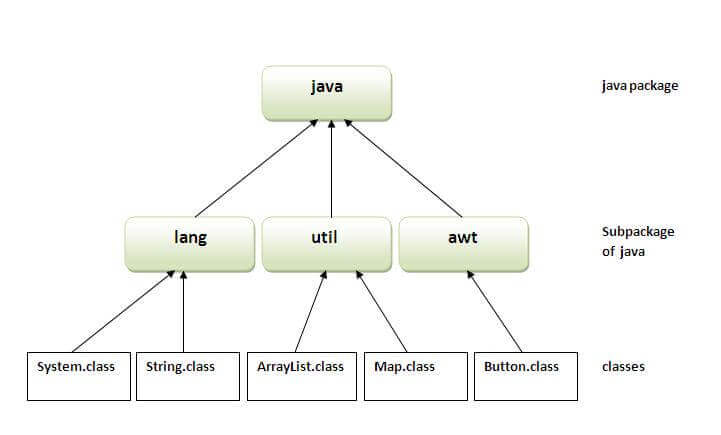
How to compile java package
If you are not using any IDE, you need to follow the syntax given below:
Javac -d directory javafilename
How to run java package program
You need to use fully qualified name e.g. Mypack.Simple etc to run the class.
Simple example of java package
The package keyword is used to create a package in java
//save as Simple.java
Package mypack;
Public class Simple
{
Public static void main(String args[]){
System.out.println("Welcome to package");
}
}
To Compile: javac -d. Simple.java
To Run: java mypack.Simple
OUTPUT: Welcome to package
Java API Package
- Java contains many predefined classes that are grouped into categories of related classes called packages.
- Together, these are known as the Java Application Programming Interface (Java API), or the Java class library.
- A great strength of Java is the Java API’s thousands of classes., which represents only a small portion of the reusable components in the Java API.
Java contains many predefined classes that are grouped into categories of related classes called packages. Together, these are known as the Java Application Programming Interface (Java API), or the Java class library. A great strength of Java is the Java API’s thousands of classes. Some key Java API packages that we use in Fig, which represents only a small portion of the reusable components in the Java API.
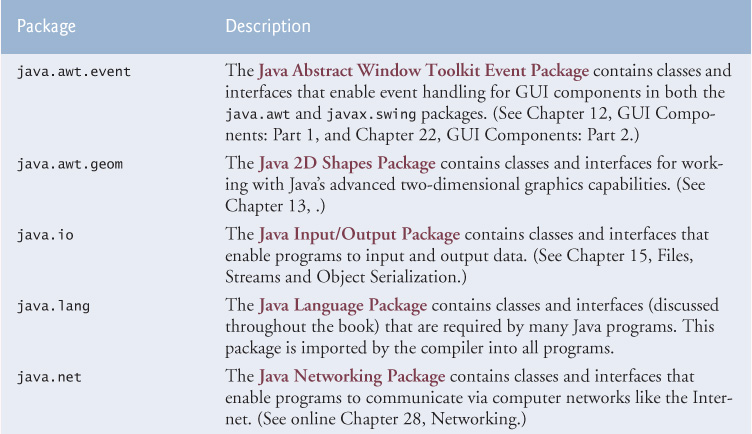
These are the packages that are defined by the user. First we create a directory myPackage (name should be same as the name of the package). Then create the MyClass inside the directory with the first statement being the package names.
Example:
// Name of the package must be same as the directory// under which this file is saved
Package myPackage;
Public class MyClass
{ public void getNames(String s)
{ System.out.println(s);
}
}
Now we can use the MyClass class in our program.
Public class PrintName
{
public static void main(String args[])
{ // Initializing the String variable with a value
String name = "GeeksforGeeks";
// Creating an instance of class MyClass in the package.
MyClass obj = new MyClass();
obj.getNames(name);
}
}
Note: MyClass.java must be saved inside the myPackage directory since it is a part of the package.
Accessing Packages
There are three ways to access the package from outside the package.
- Import package.*;
- Import package.classname;
- Fully qualified name.
1) Using packagename.*
- If you use package.* then all the classes and interfaces of this package will be accessible but not subpackages.
- The import keyword is used to make the classes and interface of another package accessible to the current package.
Example of package that import the packagename.*
//save by A.java
Package pack;
Public class A {
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.*;
Class B{
Public static void main(String args[])
{
A obj = new A();
Obj.msg();
}
}
OUTPUT: Hello
2) Using packagename.classname
If you import package.classname then only declared class of this package will be accessible.
Example of package by import package.classname
//save by A.java
Package pack;
Public class A
{
Public void msg(){System.out.println("Hello");}
}
//save by B.java
Package mypack;
Import pack.A;
Class B{
Public static void main(String args[]){
A obj = new A();
Obj.msg();
}
}
OUTPUT:
Hello
Using fully qualified name
- If we use fully qualified name then only declared class of this package will be accessible. Now there is no need to import.
- But we need to use fully qualified name every time when you are accessing the class or interface.
- It is generally used when two packages have same class name e.g. Java.util and java.sql packages contain Date class.
Example of package by import fully qualified name
//save by A.java
Package pack;
Public class A
{
Public void msg()
{ System.out.println("Hello"); }
}
//save by B.java
Package mypack;
Class B{
Public static void main(String args[]){
Pack.A obj = new pack.A();//using fully qualified name
Obj.msg();
}
}
OUTPUT:
Hello
Sequence of the program must be package then import then class.
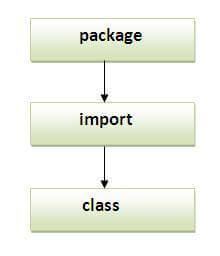
References:
1. Herbert Schildt, Java2: The Complete Reference, Tata McGraw-Hill
2. Object Oriented Programming with JAVA Essentilas and Applications, Mc Graw Hill
3. Core and Advanced Java, Black Book- dreamtech
4. Programming with JAVA- E Balagurusamy