Unit - 4
Java Server Pages
● Java Server Pages (JSP) technology provides a simplified, fast way to create web pages that display dynamically-generated content. It is a technology used to create web applications.
● The JSP specification, developed through an industry-wide initiative led by Sun Microsystems in June, 1999, defines the interaction between the server and the JSP page, and describes the format and syntax of the page.
● JSP pages share the "Write Once, Run Anywhere" characteristics of Java technology. Java Server Pages are HTML pages embedded with snippets of Java code.
● It is an inverse of a Java Servlet. It provides additional features such as expression language, JSTL (JSP Standard Tag Library), custom tags etc.
● A JSP page is a page created by the web developer that includes JSP technology-specific and custom tags, in combination with other static (HTML or XML) tags.
● The JSP pages are easier to maintain than servlet because we can separate designing and development part.
● A JSP page has the extension .jsp or .jspx. This signals to the web server that the JSP engine will process elements on this page. Using the web.xml deployment descriptor, additional extensions can be associated with the JSP engine.
● Java Server Pages often provides the same purpose as that of Common Gateway Interface (CGI). But JSP offers several advantages in comparison with the CGL.
● JSP allows embedding Dynamic Elements in HTML Pages itself instead of having separate CGI files which increases the performance.
● They are always compiled before they are processed by the server.
● Java Server Pages are built on top of the Java Servlets API, so JSP has access to all the powerful Enterprise Java APIs, including JDBC, EJB etc.
● Java Server Pages (JSP) is a technology which is used to develop web pages by inserting JAVA code into the HTML pages by making special JSP tags.
● The JSP tags which allow java code to be included into it are <% —-java code-—%>. Java Server Pages (JSP) separates the dynamic part of your pages from the static HTML.
Example
HTML tags and text
<% Place JSP code here %>
HTML tags and text
i.e.
<U>
<%= request.getParameter("Welcome”) ⅝>
<∕U>
Why use JSP?
JavaServer Pages are often used in the same way that programs written with the Popular Gateway Interface are (CGI). However, as compared to CGI, JSP has some advantages.
● Since JSP allows Dynamic Elements to be embedded in HTML Pages rather than requiring separate CGI files, performance is greatly improved.
● Unlike CGI/Perl, which allows the server to load an interpreter and the target script each time the page is requested, JSPs are always compiled until they are processed by the server.
● JSPs, including Servlets, are designed on top of the Java Servlets API, so they have access to all of the powerful Enterprise Java APIs, such as JDBC, JNDI, EJB, JAXP, and so on.
● The model supported by Java servlet template engines allows JSP pages to be used in conjunction with servlets that manage business logic.
Finally, JSP is an essential component of Java Enterprise Edition (Java EE), a comprehensive framework for enterprise-class applications. As a result, JSP can be used in a wide range of applications, from the easiest to the most complex and challenging.
Advantages of Jsp
● It's easy to code and maintain.
● Scalability and high performance.
● Since JSP is based on Java technology, it is platform independent.
Key takeaway
Java Server Pages (JSP) technology provides a simplified, fast way to create web pages that display dynamically-generated content. It is a technology used to create web applications.
A servlet is a Java class that extends the capabilities of servers that host applications that are accessed using the request-response model. Servlets are mostly used to expand web server-hosted applications, but they can also respond to other types of requests. Java Servlet technology defines HTTP-specific servlet classes for such applications.
A JavaServer Pages (JSP) is a text document that contains two types of text: static data and dynamic data. Static data can be expressed in any text-based format (such as HTML, XML, SVG, and WML), whereas dynamic content is expressed using JSP elements.
Difference between jsp and servlet
JSP | Servlets |
JSP is an HTML-based programming language. | A java code is known as a servlet. |
Because JSP is java in HTML, it is simple to code. | Writing servlet code is more difficult than writing JSP code since servlet is html in Java. |
In the MVC architecture, the view for displaying output is JSP. | In the MVC paradigm, the servlet serves as a controller. |
Because the first step in the JSP lifecycle is to translate JSP to Java code and then compile, JSP is slower than Servlet. | Servlet is faster than JSP. |
Only http requests are accepted by JSP. | All protocol requests are accepted by Servlet. |
We can't override the service() method in JSP. | We can override the service() method in Servlet. |
Session management is enabled by default in JSP. | Session management is not enabled by default in Servlet; users must specifically enable it. |
JavaBeans are used to segregate business logic from presentation logic in JSP. | Everything, including business logic and display logic, must be implemented in a single servlet file in Servlet. |
JSP alteration is simple; all you have to do is click the refresh button. | Because Servlet modification necessitates reloading, recompiling, and restarting the server, it is a time-consuming process. |
Key takeaway
A servlet is a Java class that extends the capabilities of servers that host applications that are accessed using the request-response model. Servlets are mostly used to expand web server-hosted applications, but they can also respond to other types of requests. Java Servlet technology defines HTTP-specific servlet classes for such applications.
The life cycle of a Java Server Page is defined as the process that began with its construction and then evolved into a servlet, after which the servlet lifecycle took over. This is how the procedure continues until it is completed.
The JSP life cycle includes the following steps:
● Translation of JSP page to Servlet
● Compilation of JSP page (Compilation of JSP into test.java)
● Classloading (test.java to test.class)
● Instantiation (Object of the generated Servlet is created)
● Initialization (jspInit() method is invoked by the container)
● Request processing (_jspService()is invoked by the container)
● JSP Cleanup (jspDestroy() method is invoked by the container)
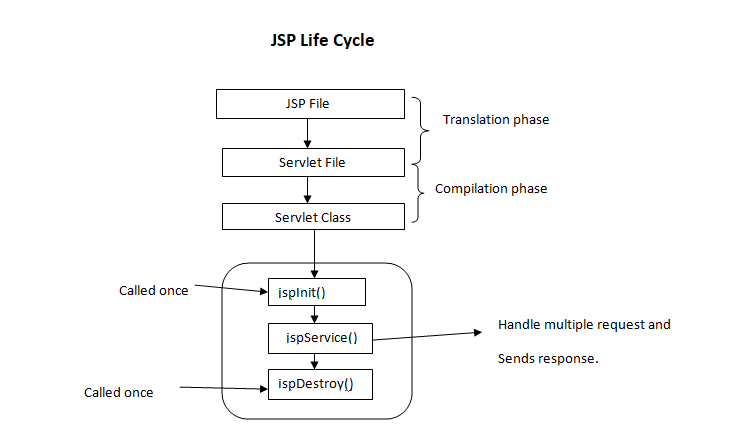
Fig: JSP life cycle
Translation of JSP page to Servlet:
The JSP life cycle begins with this phase. This part of the translation is concerned with JSP's syntactic accuracy. The test.jsp file is converted to test.java in this case.
Compilation of JSP page:
The created java servlet file (test.java) is compiled into a class file in this step (test.class).
Classloading:
The container now contains the Servlet class that was loaded from the JSP source.
Instantiation:
A new instance of the class is created here. By responding to queries, the container manages one or more instances.
Initialization:
The jspInit() method is only invoked once during the life cycle, just after the Servlet instance is created from JSP.
Request processing:
JSP's raised requests are served via the _jspService() function. As parameters, it accepts request and response objects. This procedure is not overridable.
JSP Cleanup:
The jspDestroy() method is used to remove the JSP from the container's use or to kill the method for servlets. If you need to do any cleanup tasks, such as removing open files or releasing database connections, you can override jspDestroy().
<% % > tags are used to write JSP scripting elements. The JSP engine processes the code within percent percent > tags when the JSP page is translated. The rest of the text on the JSP page is HTML code or plain text.
Different types of scripting elements
Scripting elements | Example |
Comment | <%-- comment --%> |
Directive | <%@ directive %> |
Declaration | <%! declarations %> |
Scriptlets | <% scriptlets %> |
Expression | <%= expression %> |
Declaration
We know that a JSP page is eventually converted into a Servlet class. When we declare a variable or method in JSP using the Declaration Tag, it means that the declaration is made within the Servlet class but outside of the service(or any other) method. Within the Declaration Tag, you can declare static members, instance variables, and methods.
The syntax for JSP Declarations is as follows:
<%! declaration; [ declaration; ]+ ... %>
The following is the XML equivalent of the above syntax:
<jsp:declaration>
code fragment
</jsp:declaration>
An example of a JSP Declaration is shown below.
<%! int i = 0; %>
<%! int a, b, c; %>
<%! Circle a = new Circle(2.0); %>
Example of method declarations
<%@ page language="java" contentType="text/html" %>
<html>
<body bgcolor="white"> <%!
String randomColor( ) {
java.util.Random random = new java.util.Random( );
int red = (int) (random.nextFloat( ) * 255);
int green = (int) (random.nextFloat( ) * 255);
int blue = (int) (random.nextFloat( ) * 255);
return "#" +
Integer.toString(red, 16) +
Integer.toString(green, 16) +
Integer.toString(blue, 16);
}
%>
Scriptlets
You may use the Scriptlet Tag to write Java code inside a JSP page. The Scriptlet tag uses script/java code to enforce the _jspService method features.
The Scriptlet Tag has the following syntax:
<% JAVA CODE %>
Example
<!DOCTYPE html>
<html>
<head>
<title>Scriptlet Tag</title>
</head>
<% int count = 2; %>
<body>
Calculated page count <% out.println(cnt); %>
</body>
</html>
Expression
The Expression Tag is used to print java language expressions that are placed within tags. Any java language expression that can be used as an argument to the out.print() method can be stored in an expression tag.
A programming language expression is evaluated, transformed to a String, and inserted where the expression appears in the JSP file in a JSP expression element.
Because an expression's value is converted to a String, you can use it in a JSP file within a line of text, whether or not it is tagged with HTML.
Any expression that is legitimate according to the Java Language Specification can be used in the expression element, although a semicolon cannot be used to finish an expression.
Expression Tag Syntax
<%= expression %>
Example
<!DOCTYPE html>
<html>
<body>
<%= "A JSP based string" %>
</body>
</html>
Key takeaway
- A declaration element, which is used to declare variables and methods in a JSP page, is another option.
- A declaration defines one or more variables or methods that can be used later in the JSP file in Java code.
- Any expression that is legitimate according to the Java Language Specification can be used in the expression element, although a semicolon cannot be used to finish an expression.
These Objects are Java objects that the JSP Container makes accessible to developers in each page, and the developer can call them without having to declare them explicitly. Pre-defined variables are another name for JSP Implicit Objects.
The nine Implicit Objects that JSP supports are mentioned in the table below.
No. | Object | Description |
1 | Request | This is the HttpServletRequest object that the request is associated with. |
2 | Response | This is the HttpServletResponse object that corresponds to the client's response. |
3 | Out | This is the PrintWriter object, which is responsible for sending output to the client. |
4 | Session | This is the HttpSession object that the request is connected with. |
5 | Application | The application context is associated with this ServletContext object. |
6 | Config | This is the page's associated ServletConfig item. |
7 | PageContext | This includes the use of server-specific features such as faster JspWriters. |
8 | Page | This is just another name for this, and it's used to call the methods specified by the translated servlet class. |
9 | Exception | The Exception object allows designated JSPs to access exception data. |
The request Object
The request object is a javax.servlet instance. Http.HttpServletRequest is an entity that represents a http.HttpServletRequest request. When a client requests a page, the JSP engine generates a new object to represent it.
The request object contains methods for retrieving HTTP header information such as form data, cookies, and HTTP methods, among other things.
The response Object
The answer object is a javax.servlet.http.HttpServletResponse object, which is an instance of javax.servlet.http.HttpServletResponse. The server creates an object to represent the answer to the client in the same way that it creates the request object.
The interfaces for creating new HTTP headers are also defined by the response object. The JSP programmer will use this object to add new cookies, date stamps, HTTP status codes, and so on.
The out Object
The out implicit object is a javax.servlet.jsp.JspWriter object that is used to submit content as part of a response.
Depending on whether the page is buffered or not, the initial JspWriter object is generated in a different way. The buffered = 'false' attribute of the page directive can be used to switch off buffering.
Many of the methods in the JspWriter object are the same as those in the java.io.PrintWriter class. JspWriter, on the other hand, has several additional buffering methods. JspWriter, unlike the PrintWriter object, throws IOExceptions.
The session Object
The session object is a javax.servlet.http.HttpSession instance that behaves in the same way that session objects in Java Servlets do. The session object is used to keep track of a client's session from one request to the next.
The application Object
The application object is a wrapper around the ServletContext object for the created Servlet, and is actually a javax.servlet. ServletContext kind.
This object represents a JSP page during its entire life cycle. This object is generated when the JSP page is first loaded and is removed when the jspDestroy() method is used to delete the JSP page.
You will ensure that all JSP files that make up your web application have access to it by adding an attribute to application.
The config Object
The config object is an instantiation of javax.servlet.ServletConfig and For the created servlet, is a direct wrapper around the ServletConfig item.
This object gives the JSP programmer access to Servlet or JSP engine initialization parameters including paths and file positions, among other things.
The pageContext Object
A javax.servlet.jsp.PageContext object is an instance of the pageContext object. The entire JSP page is represented by the pageContext object.
This object is designed to provide access to page information while removing most of the implementation specifics.
The page Object
This object is a real-time reference to the page's case. It's similar to an object that represents the entire JSP page.
The page object is essentially the same as this object.
The Exception Object
The exception object is a wrapper that contains the previous page's exception. It's usually used to come up with a suitable answer to an error state.
Key takeaway
- These Objects are Java objects that the JSP Container makes accessible to developers in each page, and the developer can call them without having to declare them explicitly.
- Pre-defined variables are another name for JSP Implicit Objects.
- The nine Implicit Objects that JSP supports.
These guidelines give the container guidance and instructions on how to manage certain aspects of the JSP processing.
The overall structure of the servlet class is affected by a JSP directive. It normally takes the form of the following.
<%@ directive attribute = "value" %>
A directive may have a number of attributes, which you can mention as key-value pairs separated by commas in a table.
The space between the @ symbol and the directive name, as well as the space between the last attribute and the closing percent >, is optional.
A directive tag may be one of three styles.
No. | Directives | Description |
1 | <%@ page ... %> | Scripting language, error page, and buffering specifications are all page-dependent attributes. |
2 | <%@ include ... %> | During the translation process, a file is included. |
3 | <%@ taglib ... %> | Declares a tag library of custom behavior that can be included throughout the page. |
Page Directives
The page directive is used to give the container instructions. These guidelines apply to the current JSP page. Page instructions can be coded anywhere in your JSP page. Page instructions are coded at the top of the JSP page by convention.
The page directive's basic syntax is as follows:
<%@ page attribute = "value" %>
Include Directives
During the translation process, the include directive is used to include a file. During the conversion process, this directive instructs the container to merge the content of other external files with the current JSP. Include directives can be placed anywhere in your JSP page.
This directive's general use form is as follows:
<%@ include file = "relative url" >
Taglib Directives
Custom JSP tags that look like HTML or XML tags can be specified using the JavaServer Pages API, and a tag library is a collection of user-defined tags that enforce custom actions.
The taglib directive declares that your JSP page uses a collection of custom tags, specifies the library's location, and specifies how to locate the custom tags in your JSP page.
The taglib directive has the following syntax.
<%@ taglib uri="uri" prefix = "prefixOfTag" >
Standard(Action) tags are provided by the JSP specification for use in JSP pages. Since scriptlet code is technically not recommended nowadays, these tags are used to delete or exclude scriptlet code from your JSP page. Putting java code directly within your JSP page is considered poor practice.
The jsp: prefix is used to start standard tags. There are a number of JSP Standard Action tags that are used to carry out particular tasks.
The following are some examples of JSP Standard Action Tags:
Action Tag | Description |
Jsp : forward | The request will be forwarded to a new tab. Usage : <jsp:forward page="Relative URL" /> |
Jsp : useBean | This method locates or creates a JavaBean. Usage : <jsp:useBean id="beanId" /> |
Jsp : getProperty | a property from a JavaBean instance is retrieved. Usage : <jsp:useBean id="beanId" ... /> ... <jsp:getProperty name="beanId" property="someProperty …../> The name of the pre-defined bean whose property we want to access is beanName. |
Jsp : setProperty | Data can be stored in any JavaBeans instance's property. Usage : <jsp:useBean id="beanId" ... /> ... <jsp:setProperty name="beanId" property="someProperty" Value="some value"/> The name of the pre-defined bean whose property we want to access is beanName. |
Jsp : include | Includes a JSP page's runtime answer in the current page
|
Jsp : plugin | Creates an OBJECT or EMBED tag for Java Applets using a client browser-specific build.
|
Jsp : fallback | If the java plugin isn't accessible on the client, it provides an alternative text. If the included jsp plugin is not loaded, you can use this to print a message. |
Jsp : element | Dynamically defines XML components. |
Jsp : attribute | The attribute of a dynamically defined XML entity is defined. |
Jsp : body | Used to include the tag body in regular or custom tags. |
Jsp : param | Parameters are added to the request object. |
Jsp : text | In JSP pages and papers, it's used to write template text. Usage : <jsp:text>Template data</jsp:text> |
Jsp : forward
The jsp:forward action element is used to forward the request to another resource, which could be jsp, html, or something else entirely.
Syntax of jsp:forward action tag without parameter
<jsp:forward page="relativeURL | <%= expression %>" />
Syntax of jsp:forward action tag with parameter
<jsp:forward page="relativeURL | <%= expression %>">
<jsp:param name="parametername" value="parametervalue | <%=expression%>" />
</jsp:forward>
Example
We are simply passing the request to the printdate.jsp file in this example.
Index.jsp
<html>
<body>
<h2>this is index page</h2>
<jsp:forward page="printdate.jsp" />
</body>
</html>
Printdate.jsp
<html>
<body>
<% out.print("Today is:"+java.util.Calendar.getInstance().getTime()); %>
</body>
</html>
Example of jsp:forward action tag with parameter
The request is forwarded to the printdate.jsp file with parameter in this example, and the printdate.jsp file outputs the parameter value together with the date and time.
Index.jsp
<html>
<body>
<h2>this is index page</h2>
<jsp:forward page="printdate.jsp" >
<jsp:param name="name" value="javatpoint.com" />
</jsp:forward>
</body>
</html>
Printdate.jsp
<html>
<body>
<% out.print("Today is:"+java.util.Calendar.getInstance().getTime()); %>
<%= request.getParameter("name") %>
</body>
</html>
Jsp: include
You can use the "include action" to include another resource in the page that is being created.
Syntax
<jsp:include page = "Page URL" flush = "Boolean Value" />
Example
<!DOCTYPE html>
<html>
<head>
<title>JSP include Action</title>
</head>
<body>
<h2>JSP page: with include</h2>
<jsp:include page="header.jsp" flush="false" />
</body>
</html>
Key takeaway
Standard(Action) tags are provided by the JSP specification for use in JSP pages. Since scriptlet code is technically not recommended nowadays, these tags are used to delete or exclude scriptlet code from your JSP page. Putting java code directly within your JSP page is considered poor practice.
Write some HTML code as shown below and save it with the.jsp extension to build the first JSP page. This file has been called index.jsp. To run the JSP website, put it in a folder and paste it in the web-apps directory of Apache Tomcat.
Index.jsp
Let's look at a simple JSP example in which we use the scriptlet tag to embed Java code in the JSP tab. The scriptlet tag will be covered later.
<html>
<body>
<% out.print(2*5); %>
</body>
</html>
On the browser, it will print 10.
Example
<html>
<head>
<title>My First JSP Page</title>
</head>
<%
int count = 0;
%>
<body>
Page Count is <% out.println(++count); %>
</body>
</html>
How to run a simple Jsp page
To run this JSP page, go through the steps below:
● Begin the server.
● Place the JSP file in a folder and publish it to the server.
● Use the URL to open the window.
References:
- Herbert Schildt, Java2: The Complete Reference, Tata McGraw-Hill
- Object Oriented Programming with JAVA Essentials and Applications , McGraw Hill
- Core and Advanced Java, Black Book- dreamtech
- Murach’s Java Servlets and JSP