Unit - 1
Object Oriented Concepts
Object Oriented Programming
- Program is divided into number of objects.
- Objects are considered as real life entities that contain data and functions.
- Data is given more importance than functions.
- Data is not accessible to functions of other classes and hence data security is ensured.
- It employs bottom up approach.
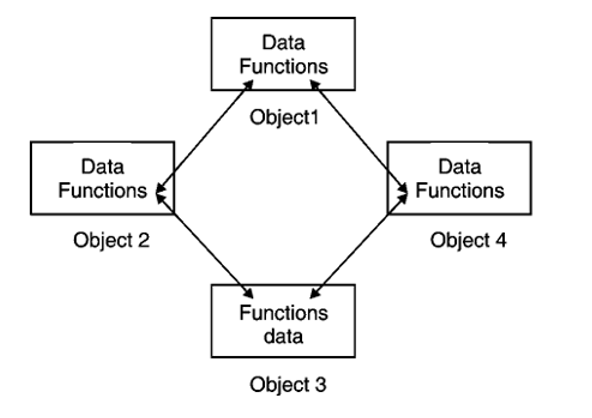
Fig: Structure of Object Oriented Programming
Fig. Shows how objects can communicate with other objects with the help of functions. Here data is not accessible to external functions which ensure data security.
Need of object-oriented programming
- Real Time systems
- Object Oriented Databases
- Simulation and Modelling
- Neural networks, AI and Expert systems
OOP Paradigms
- The major motivating factor in the invention of object-oriented approach is to remove some of the flaws encountered in the procedural approach.
- OOP treats data as a critical element in the program development and does not allow it to flow freely around the systems.
- It ties data more closely to the functions that operate on it, and protects it from accidental modification from outside functions.
- OOP allows decomposition of a problem into a number of entities called objects and then builds data and functions around these objects.
- The data of an object can be accessed only by the function associated with that object.
- However, functions of one object can access the the functions of other objects.
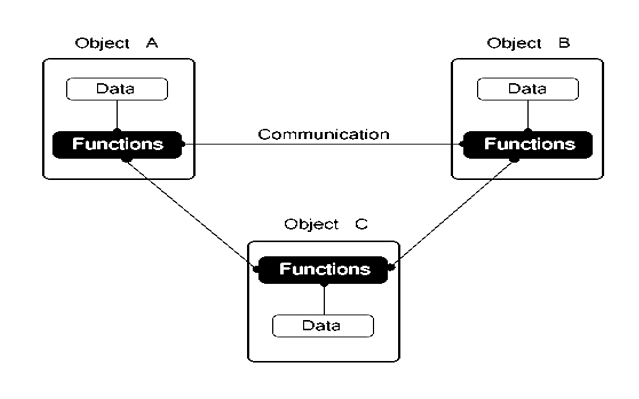
Some of the striking features of object-oriented programming are
- Emphasis is on data rather than procedure.
- Programs are divided into what are known as objects.
- Data structures are designed such that they characterize the objects.
- Data is hidden and cannot be accessed by external functions.
- Objects may communicate with each other through functions.
- New data and functions can be easily added whenever necessary.
- Follows bottom-up approach in program design.
Advantages of OOP
- Real world complex problems can be modelled very well using OOP as OOP consists of object that represents real life entity.
- Using Inheritance class can reuse code of another class. This leads to faster software development and saves coding efforts and time.
- OOP ensures data security by making members of class private. Private members are accessible to same class hence data is not invaded by external functions.
- We can perform variety of tasks with the help of single function or operator by function or operator overloading.
- OOP provides better software development productivity than traditional programming languages due to data hiding, inheritance, polymorphism and templates.
Difference between POP and OOP
Sr. No. | Key | OOP | POP |
1. | Definition | Stands for Object Oriented Programming | Stands for Procedural Oriented Programming |
2. | Division | A program is divided to objects and their interactions. | A program is divided into functions and they interacts. |
3. | Approach | Follows bottom up approach | Follows top down approach |
4. | Data Hiding | Encapsulation is used to hide data. | Data is globally accessible. No data hiding present. |
5. | Inheritance supported | Supported | Not supported |
6. | Access control | Access control is supported via access modifiers. | No access modifiers are supported |
7. | Example | C++ | C |
Inheritance
- It is a mechanism of extending functionality from one class to another.
- Class can reuse the code of another class if they are tied together by inheritance.
- The original class is called as base/super/parent class and inherited class is called derived/sub/child class.
- Sub class inherits some or all the features of base class.
- Sub class adds its own features to base class so that it has combined features of both the classes.
- In this way it supports the concept of reusability.
- It can very well model hierarchical classification as shown below.
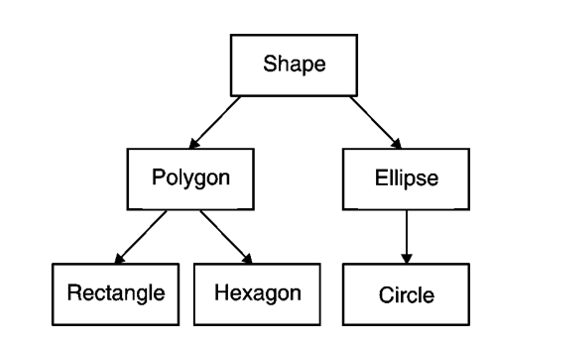
Fig: Inheritance
- Here Shape is considered as base class.
- Polygon and Ellipse are subclasses of class Shape.
- Rectangle and Hexagon are subclasses of Polygon so they inherit features of Polygon class.
- Similarly, Circle inherits features of Ellipse class.
Polymorphism
- It is ability to take more than one form depending upon the data types used in program.
- Consider the operation of finding area of any geometrical figure shown in fig.
- The operation “Area” is same but will take different forms depending upon type and number of arguments passed to “Area”.
- “Area” of square calculates area of square whereas “Area” of rectangle calculates area of rectangle.
- Though operation “Area” is same but it takes different forms depending upon type of geometrical figure.
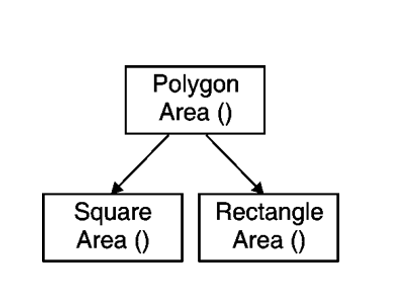
Fig: Polymorphism
Encapsulation
All C++ programs are composed of the following two fundamental elements −
Program statements (code) − This is the part of a program that performs actions and they are called functions.
Program data − The data is the information of the program which gets affected by the program functions.
Encapsulation is an Object Oriented Programming concept that binds together the data and functions that manipulate the data, and that keeps both safe from outside interference and misuse. Data encapsulation led to the important OOP concept of data hiding.
Data encapsulation is a mechanism of bundling the data, and the functions that use them and data abstraction is a mechanism of exposing only the interfaces and hiding the implementation details from the user.
C++ supports the properties of encapsulation and data hiding through the creation of user-defined types, called classes. We already have studied that a class can contain private, protected and public members. By default, all items defined in a class are private. For example −
Class Box {
public:
double getVolume(void) {
return length * breadth * height;
}
private:
double length; // Length of a box
double breadth; // Breadth of a box
double height; // Height of a box
};
The variables length, breadth, and height are private. This means that they can be accessed only by other members of the Box class, and not by any other part of your program. This is one way encapsulation is achieved.
To make parts of a class public (i.e., accessible to other parts of your program), you must declare them after the public keyword. All variables or functions defined after the public specifier are accessible by all other functions in your program.
Making one class a friend of another exposes the implementation details and reduces encapsulation. The ideal is to keep as many of the details of each class hidden from all other classes as possible.
Data Encapsulation Example
Any C++ program where you implement a class with public and private members is an example of data encapsulation and data abstraction. Consider the following example −
#include <iostream>
Using namespace std;
Class Adder {
public:
// constructor
Adder(int i = 0) {
total = i;
}
// interface to outside world
void addNum(int number) {
total += number;
}
// interface to outside world
int getTotal() {
return total;
};
private:
// hidden data from outside world
int total;
};
Int main() {
Adder a;
a.addNum(10);
a.addNum(20);
a.addNum(30);
cout << "Total " << a.getTotal() <<endl;
return 0;
}
When the above code is compiled and executed, it produces the following result −
Total 60
Above class adds numbers together, and returns the sum. The public members addNum and getTotal are the interfaces to the outside world and a user needs to know them to use the class. The private member total is something that is hidden from the outside world, but is needed for the class to operate properly.
Designing Strategy
Most of us have learnt to make class members private by default unless we really need to expose them. That's just good encapsulation.
This is applied most frequently to data members, but it applies equally to all members, including virtual functions.
Key takeaway
Encapsulation is an Object Oriented Programming concept that binds together the data and functions that manipulate the data, and that keeps both safe from outside interference and misuse. Data encapsulation led to the important OOP concept of data hiding.
Data encapsulation is a mechanism of bundling the data, and the functions that use them and data abstraction is a mechanism of exposing only the interfaces and hiding the implementation details from the user.
Data Abstraction
- Data Abstraction is a process of providing only the essential details to the outside world and hiding the internal details, i.e., representing only the essential details in the program.
- Data Abstraction is a programming technique that depends on the seperation of the interface and implementation details of the program.
- Let's take a real life example of AC, which can be turned ON or OFF, change the temperature, change the mode, and other external components such as fan, swing. But, we don't know the internal details of the AC, i.e., how it works internally. Thus, we can say that AC seperates the implementation details from the external interface.
- C++ provides a great level of abstraction. For example, pow() function is used to calculate the power of a number without knowing the algorithm the function follows.
In C++ program if we implement class with private and public members then it is an example of data abstraction.
Data Abstraction can be achieved in two ways:
- Abstraction using classes
- Abstraction in header files.
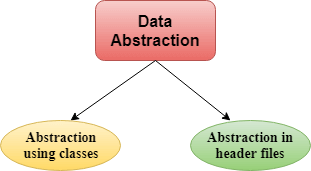
Fig: Data Abstraction
Abstraction using classes: An abstraction can be achieved using classes. A class is used to group all the data members and member functions into a single unit by using the access specifiers. A class has the responsibility to determine which data member is to be visible outside and which is not.
Abstraction in header files: An another type of abstraction is header file. For example, pow() function available is used to calculate the power of a number without actually knowing which algorithm function uses to calculate the power. Thus, we can say that header files hides all the implementation details from the user.
Access Specifiers Implement Abstraction:
- Public specifier: When the members are declared as public, members can be accessed anywhere from the program.
- Private specifier: When the members are declared as private, members can only be accessed only by the member functions of the class.
Let's see a simple example of abstraction in header files.
// program to calculate the power of a number.
- #include <iostream>
- #include<math.h>
- Using namespace std;
- Int main()
- {
- Int n = 4;
- Int power = 3;
- Int result = pow(n,power); // pow(n,power) is the power function
- Std::cout << "Cube of n is : " <<result<< std::endl;
- Return 0;
- }
Output:
Cube of n is : 64
In the above example, pow() function is used to calculate 4 raised to the power 3. The pow() function is present in the math.h header file in which all the implementation details of the pow() function is hidden.
Let's see a simple example of data abstraction using classes.
- #include <iostream>
- Using namespace std;
- Class Sum
- {
- Private: int x, y, z; // private variables
- Public:
- Void add()
- {
- Cout<<"Enter two numbers: ";
- Cin>>x>>y;
- z= x+y;
- Cout<<"Sum of two number is: "<<z<<endl;
- }
- };
- Int main()
- {
- Sum sm;
- Sm.add();
- Return 0;
- }
Output:
Enter two numbers:
3
6
Sum of two number is: 9
In the above example, abstraction is achieved using classes. A class 'Sum' contains the private members x, y and z are only accessible by the member functions of the class.
Advantages of Abstraction:
- Implementation details of the class are protected from the inadvertent user level errors.
- A programmer does not need to write the low level code.
- Data Abstraction avoids the code duplication, i.e., programmer does not have to undergo the same tasks every time to perform the similar operation.
- The main aim of the data abstraction is to reuse the code and the proper partitioning of the code across the classes.
- Internal implementation can be changed without affecting the user level code.
Key takeaway
- Data Abstraction is a process of providing only the essential details to the outside world and hiding the internal details, i.e., representing only the essential details in the program.
- Data Abstraction is a programming technique that depends on the seperation of the interface and implementation details of the program.
- Let's take a real life example of AC, which can be turned ON or OFF, change the temperature, change the mode, and other external components such as fan, swing. But, we don't know the internal details of the AC, i.e., how it works internally. Thus, we can say that AC seperates the implementation details from the external interface.
The major purpose of C++ programming is to introduce the concept of object orientation to the C programming language.
Object Oriented Programming is a paradigm that provides many concepts such as inheritance, data binding, polymorphism etc.
The programming paradigm where everything is represented as an object is known as truly object-oriented programming language. Smalltalk is considered as the first truly object-oriented programming language.
OOPs (Object Oriented Programming System)
Object means a real word entity such as pen, chair, table etc. Object-Oriented Programming is a methodology or paradigm to design a program using classes and objects. It simplifies the software development and maintenance by providing some concepts:
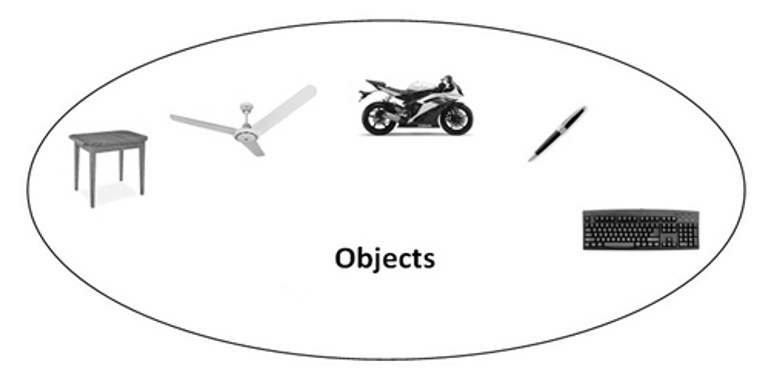
- Object
- Class
- Inheritance
- Polymorphism
- Abstraction
- Encapsulation
Object
Any entity that has state and behaviour is known as an object. For example: chair, pen, table, keyboard, bike etc. It can be physical and logical.
Class
Collection of objects is called class. It is a logical entity.
Inheritance
When one object acquires all the properties and behaviours of parent object i.e. known as inheritance. It provides code reusability. It is used to achieve runtime polymorphism.
Polymorphism
When one task is performed by different ways i.e. known as polymorphism. For example: to convince the customer differently, to draw something e.g. shape or rectangle etc.
In C++, we use Function overloading and Function overriding to achieve polymorphism.
Abstraction
Hiding internal details and showing functionality is known as abstraction. For example: phone call, we don't know the internal processing.
In C++, we use abstract class and interface to achieve abstraction.
Encapsulation
Binding (or wrapping) code and data together into a single unit is known as encapsulation. For example: capsule, it is wrapped with different medicines.
Advantage of OOPs over Procedure-oriented programming language
- OOPs makes development and maintenance easier where as in Procedure-oriented programming language it is not easy to manage if code grows as project size grows.
- OOPs provide data hiding whereas in Procedure-oriented programming language a global data can be accessed from anywhere.
- OOPs provide ability to simulate real-world event much more effectively. We can provide the solution of real word problem if we are using the Object-Oriented Programming language.
Tokens
- Tokens are the smallest unit of the program meaningful to the compiler.
- Tokens are classified as – Keywords, Identifiers, Contestants, Strings, Operators.
Keywords
- In programming language keywords are reserved word and performs specific task.
- The keyword cannot used as variable because these are directly referred by compiler and assigning new value to keyword is not allowed.
- C++ language have 32 keywords.
Auto double int struct
Break else long switch
Case enum register typedef
Char extern return union
Const float short unsigned
Continue for signed void
Default goto sizeof volatile
Do if static while
Identifiers
- Identifiers are used to name the variables, arrays and functions.
- These are the user defined.
- To constitute the name digits, letters and underscore is used. Underscore is used as first character.
- Identifiers should not be same as keyword.
- After declaration identifiers are allowed to use anywhere in the program.
- It should not consist of white space.
- Example - _ab5.
Constant
- Constant are same as variables but once initialized, cannot change by program.
- Constants are fixed values and also called as literals.
- Example –
- Integer constants – 2,7,0,454,1204.
- Real constants – 6.7, 4556.78,3432.988
- Character constants – ‘a’,’G’.
- String Constants – “Hello World”.
In C++, data types are declarations for variables. This determines the type and size of data associated with variables.
For example,
Int age = 13;
Here, age is a variable of type int. Meaning, the variable can only store integers of either 2 or 4 bytes.
C++ Fundamental Data Types
The table below shows the fundamental data types, their meaning, and their sizes (in bytes):
Data TypeMeaningSize (in Bytes)
IntInteger2 or 4
FloatFloating-point4
DoubleDouble Floating-point8
CharCharacter1
Wchar_tWide Character2
BoolBoolean1
VoidEmpty0
1. Int
The int keyword is used to indicate integers.
Its size is usually 4 bytes. Meaning, it can store values from -2147483648 to 214748647.
For example,
Int salary = 85000;
2. float and double
Float and double are used to store floating-point numbers (decimals and exponentials).
The size of float is 4 bytes and the size of double is 8 bytes. Hence, double has two times the precision of float. To learn more, visit C++ float and double.
For example,
Float area = 64.74;
Double volume = 134.64534;
As mentioned above, these two data types are also used for exponentials. For example,
Double distance = 45E12 // 45E12 is equal to 45*10^12
3. Char
Keyword char is used for characters.
Its size is 1 byte.
Characters in C++ are enclosed inside single quotes ' '.
For example,
Char test = 'h';
Note: In C++, an integer value is stored in a char variable rather than the character itself. To learn more, visit C++ characters.
4. wchar_t
Wide character wchar_t is similar to the char data type, except its size is 2 bytes instead of 1.
It is used to represent characters that require more memory to represent them than a single char.
For example,
Wchar_t test = L'ם' // storing Hebrew character;
When we consider a C++ program, it can be defined as a collection of objects that communicate via invoking each other's methods. Let us now briefly look into what a class, object, methods, and instant variables mean.
Object − Objects have states and behaviors. Example: A dog has states - color, name, breed as well as behaviors - wagging, barking, eating. An object is an instance of a class.
Class − A class can be defined as a template/blueprint that describes the behaviors/states that object of its type support.
Methods − A method is basically a behavior. A class can contain many methods. It is in methods where the logics are written, data is manipulated and all the actions are executed.
Instance Variables − Each object has its unique set of instance variables. An object's state is created by the values assigned to these instance variables.
C++ Program Structure
Let us look at a simple code that would print the words Hello World.
#include <iostream>
Using namespace std;
// main() is where program execution begins.
Int main() {
cout << "Hello World"; // prints Hello World
return 0;
}
Let us look at the various parts of the above program −
The C++ language defines several headers, which contain information that is either necessary or useful to your program. For this program, the header <iostream> is needed.
The line using namespace std; tells the compiler to use the std namespace. Namespaces are a relatively recent addition to C++.
The next line '// main() is where program execution begins.' is a single-line comment available in C++. Single-line comments begin with // and stop at the end of the line.
The line int main() is the main function where program execution begins.
The next line cout << "Hello World"; causes the message "Hello World" to be displayed on the screen.
The next line return 0; terminates main( )function and causes it to return the value 0 to the calling process.
Compile and Execute C++ Program
Let's look at how to save the file, compile and run the program. Please follow the steps given below −
Open a text editor and add the code as above.
Save the file as: hello.cpp
Open a command prompt and go to the directory where you saved the file.
Type 'g++ hello.cpp' and press enter to compile your code. If there are no errors in your code the command prompt will take you to the next line and would generate a.out executable file.
Now, type 'a.out' to run your program.
You will be able to see ' Hello World ' printed on the window.
$ g++ hello.cpp
$ ./a.out
Hello World
Make sure that g++ is in your path and that you are running it in the directory containing file hello.cpp.
You can compile C/C++ programs using makefile.
The C++ standard libraries provide an extensive set of input/output capabilities which we will see in subsequent chapters. This chapter will discuss very basic and most common I/O operations required for C++ programming.
C++ I/O occurs in streams, which are sequences of bytes. If bytes flow from a device like a keyboard, a disk drive, or a network connection etc. to main memory, this is called input operation and if bytes flow from main memory to a device like a display screen, a printer, a disk drive, or a network connection, etc., this is called output operation.
I/O Library Header Files
There are following header files important to C++ programs −
Sr.No | Header File & Function and Description |
1 | <iostream> This file defines the cin, cout, cerr and clog objects, which correspond to the standard input stream, the standard output stream, the un-buffered standard error stream and the buffered standard error stream, respectively. |
2 | <iomanip> This file declares services useful for performing formatted I/O with so-called parameterized stream manipulators, such as setw and setprecision. |
3 | <fstream> This file declares services for user-controlled file processing. We will discuss about it in detail in File and Stream related chapter. |
The Standard Output Stream (cout)
The predefined object cout is an instance of ostream class. The cout object is said to be "connected to" the standard output device, which usually is the display screen. The cout is used in conjunction with the stream insertion operator, which is written as << which are two less than signs as shown in the following example.
#include <iostream>
Using namespace std;
Int main() {
char str[] = "Hello C++";
cout << "Value of str is : " << str << endl;
}
When the above code is compiled and executed, it produces the following result−
Value of str is : Hello C++
The C++ compiler also determines the data type of variable to be output and selects the appropriate stream insertion operator to display the value. The << operator is overloaded to output data items of built-in types integer, float, double, strings and pointer values.
The insertion operator << may be used more than once in a single statement as shown above and endl is used to add a new-line at the end of the line.
The Standard Input Stream (cin)
The predefined object cin is an instance of istream class. The cin object is said to be attached to the standard input device, which usually is the keyboard. The cin is used in conjunction with the stream extraction operator, which is written as >> which are two greater than signs as shown in the following example.
#include <iostream>
Using namespace std;
Int main() {
char name[50];
cout << "Please enter your name: ";
cin >> name;
cout << "Your name is: " << name << endl;
}
When the above code is compiled and executed, it will prompt you to enter a name. You enter a value and then hit enter to see the following result −
Please enter your name: cplusplus
Your name is: cplusplus
The C++ compiler also determines the data type of the entered value and selects the appropriate stream extraction operator to extract the value and store it in the given variables.
The stream extraction operator >> may be used more than once in a single statement. To request more than one datum you can use the following −
Cin >> name >> age;
This will be equivalent to the following two statements −
Cin >> name;
Cin >> age;
The Standard Error Stream (cerr)
The predefined object cerr is an instance of ostream class. The cerr object is said to be attached to the standard error device, which is also a display screen but the object cerr is un-buffered and each stream insertion to cerr causes its output to appear immediately.
The cerr is also used in conjunction with the stream insertion operator as shown in the following example.
#include <iostream>
Using namespace std;
Int main() {
char str[] = "Unable to read....";
cerr << "Error message : " << str << endl;
}
When the above code is compiled and executed, it produces the following result−
Error message : Unable to read....
The Standard Log Stream (clog)
The predefined object clog is an instance of ostream class. The clog object is said to be attached to the standard error device, which is also a display screen but the object clog is buffered. This means that each insertion to clog could cause its output to be held in a buffer until the buffer is filled or until the buffer is flushed.
The clog is also used in conjunction with the stream insertion operator as shown in the following example.
#include <iostream>
Using namespace std;
Int main() {
char str[] = "Unable to read....";
clog << "Error message : " << str << endl;
}
When the above code is compiled and executed, it produces the following result−
Error message : Unable to read....
You would not be able to see any difference in cout, cerr and clog with these small examples, but while writing and executing big programs the difference becomes obvious. So it is good practice to display error messages using cerr stream and while displaying other log messages then clog should be used.
References:
1. Object oriented programming By E. Balagurusamy.
2. C++ Programming –By D. Ravichandran
3. Let Us C++ By YashawantKanetkar.
4. Object Oriented Programming in C++ - Dr. G. T. Thampi, Dr. S. S. Mantha
5. Mastering C++ -By Venugopal.