Unit - 2
Operators in C++
An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations. C++ is rich in built-in operators and provide the following types of operators −
- Arithmetic Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment Operators
- Misc Operators
This chapter will examine the arithmetic, relational, logical, bitwise, assignment and other operators one by one.
Arithmetic Operators
There are following arithmetic operators supported by C++ language −
Assume variable A holds 10 and variable B holds 20, then −
Show Examples
Operator | Description | Example |
+ | Adds two operands | A + B will give 30 |
- | Subtracts second operand from the first | A - B will give -10 |
* | Multiplies both operands | A * B will give 200 |
/ | Divides numerator by de-numerator | B / A will give 2 |
% | Modulus Operator and remainder of after an integer division | B % A will give 0 |
++ | Increment operator, increases integer value by one | A++ will give 11 |
-- | Decrement operator, decreases integer value by one | A-- will give 9 |
Relational Operators
There are following relational operators supported by C++ language
Assume variable A holds 10 and variable B holds 20, then −
Show Examples
Operator | Description | Example |
== | Checks if the values of two operands are equal or not, if yes then condition becomes true. | (A == B) is not true. |
!= | Checks if the values of two operands are equal or not, if values are not equal then condition becomes true. | (A != B) is true. |
> | Checks if the value of left operand is greater than the value of right operand, if yes then condition becomes true. | (A > B) is not true. |
< | Checks if the value of left operand is less than the value of right operand, if yes then condition becomes true. | (A < B) is true. |
>= | Checks if the value of left operand is greater than or equal to the value of right operand, if yes then condition becomes true. | (A >= B) is not true. |
<= | Checks if the value of left operand is less than or equal to the value of right operand, if yes then condition becomes true. | (A <= B) is true. |
Logical Operators
There are following logical operators supported by C++ language.
Assume variable A holds 1 and variable B holds 0, then −
Show Examples
Operator | Description | Example |
&& | Called Logical AND operator. If both the operands are non-zero, then condition becomes true. | (A && B) is false. |
|| | Called Logical OR Operator. If any of the two operands is non-zero, then condition becomes true. | (A || B) is true. |
! | Called Logical NOT Operator. Use to reverses the logical state of its operand. If a condition is true, then Logical NOT operator will make false. | !(A && B) is true. |
Bitwise Operators
Bitwise operator works on bits and perform bit-by-bit operation. The truth tables for &, |, and ^ are as follows −
p | q | p & q | p | q | p ^ q |
0 | 0 | 0 | 0 | 0 |
0 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 |
Assume if A = 60; and B = 13; now in binary format they will be as follows −
A = 0011 1100
B = 0000 1101
-----------------
A&B = 0000 1100
A|B = 0011 1101
A^B = 0011 0001
~A = 1100 0011
The Bitwise operators supported by C++ language are listed in the following table. Assume variable A holds 60 and variable B holds 13, then −
Show Examples
Operator | Description | Example |
& | Binary AND Operator copies a bit to the result if it exists in both operands. | (A & B) will give 12 which is 0000 1100 |
| | Binary OR Operator copies a bit if it exists in either operand. | (A | B) will give 61 which is 0011 1101 |
^ | Binary XOR Operator copies the bit if it is set in one operand but not both. | (A ^ B) will give 49 which is 0011 0001 |
~ | Binary Ones Complement Operator is unary and has the effect of 'flipping' bits. | (~A ) will give -61 which is 1100 0011 in 2's complement form due to a signed binary number. |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | A << 2 will give 240 which is 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | A >> 2 will give 15 which is 0000 1111 |
Assignment Operators
There are following assignment operators supported by C++ language −
Show Examples
Operator | Description | Example |
= | Simple assignment operator, Assigns values from right side operands to left side operand. | C = A + B will assign value of A + B into C |
+= | Add AND assignment operator, It adds right operand to the left operand and assign the result to left operand. | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator, It subtracts right operand from the left operand and assign the result to left operand. | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator, It multiplies right operand with the left operand and assign the result to left operand. | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator, It divides left operand with the right operand and assign the result to left operand. | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator, It takes modulus using two operands and assign the result to left operand. | C %= A is equivalent to C = C % A |
<<= | Left shift AND assignment operator. | C <<= 2 is same as C = C << 2 |
>>= | Right shift AND assignment operator. | C >>= 2 is same as C = C >> 2 |
&= | Bitwise AND assignment operator. | C &= 2 is same as C = C & 2 |
^= | Bitwise exclusive OR and assignment operator. | C ^= 2 is same as C = C ^ 2 |
|= | Bitwise inclusive OR and assignment operator. | C |= 2 is same as C = C | 2 |
Misc Operators
The following table lists some other operators that C++ supports.
Sr.No | Operator & Description |
1 | Sizeof Sizeof operator returns the size of a variable. For example, sizeof(a), where ‘a’ is integer, and will return 4. |
2 | Condition ? X : Y Conditional operator (?). If Condition is true then it returns value of X otherwise returns value of Y. |
3 | , Comma operator causes a sequence of operations to be performed. The value of the entire comma expression is the value of the last expression of the comma-separated list. |
4 | . (dot) and -> (arrow) Member operators are used to reference individual members of classes, structures, and unions. |
5 | Cast Casting operators convert one data type to another. For example, int(2.2000) would return 2. |
6 | & Pointer operator & returns the address of a variable. For example &a; will give actual address of the variable. |
7 | * Pointer operator * is pointer to a variable. For example *var; will pointer to a variable var. |
Operators Precedence in C++
Operator precedence determines the grouping of terms in an expression. This affects how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has higher precedence than the addition operator −
For example x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Show Examples
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | << >> | Left to right |
Relational | < <= > >= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
A good understanding of how dynamic memory really works in C++ is essential to becoming a good C++ programmer. Memory in your C++ program is divided into two parts −
- The stack − All variables declared inside the function will take up memory from the stack.
- The heap − This is unused memory of the program and can be used to allocate the memory dynamically when program runs.
Many times, you are not aware in advance how much memory you will need to store particular information in a defined variable and the size of required memory can be determined at run time.
You can allocate memory at run time within the heap for the variable of a given type using a special operator in C++ which returns the address of the space allocated. This operator is called new operator.
If you are not in need of dynamically allocated memory anymore, you can use delete operator, which de-allocates memory that was previously allocated by new operator.
New and delete Operators
There is following generic syntax to use new operator to allocate memory dynamically for any data-type.
New data-type;
Here, data-type could be any built-in data type including an array or any user defined data types include class or structure. Let us start with built-in data types. For example we can define a pointer to type double and then request that the memory be allocated at execution time. We can do this using the new operator with the following statements −
Double* pvalue = NULL; // Pointer initialized with null
Pvalue = new double; // Request memory for the variable
The memory may not have been allocated successfully, if the free store had been used up. So it is good practice to check if new operator is returning NULL pointer and take appropriate action as below −
Double* pvalue = NULL;
If( !(pvalue = new double )) {
cout << "Error: out of memory." <<endl;
exit(1);
}
The malloc() function from C, still exists in C++, but it is recommended to avoid using malloc() function. The main advantage of new over malloc() is that new doesn't just allocate memory, it constructs objects which is prime purpose of C++.
At any point, when you feel a variable that has been dynamically allocated is not anymore required, you can free up the memory that it occupies in the free store with the ‘delete’ operator as follows −
Delete pvalue; // Release memory pointed to by pvalue
Let us put above concepts and form the following example to show how ‘new’ and ‘delete’ work −
#include <iostream>
Using namespace std;
Int main () {
double* pvalue = NULL; // Pointer initialized with null
pvalue = new double; // Request memory for the variable
*pvalue = 29494.99; // Store value at allocated address
cout << "Value of pvalue : " << *pvalue << endl;
delete pvalue; // free up the memory.
return 0;
}
If we compile and run above code, this would produce the following result −
Value of pvalue : 29495
Dynamic Memory Allocation for Arrays
Consider you want to allocate memory for an array of characters, i.e., string of 20 characters. Using the same syntax what we have used above we can allocate memory dynamically as shown below.
Char* pvalue = NULL; // Pointer initialized with null
Pvalue = new char[20]; // Request memory for the variable
To remove the array that we have just created the statement would look like this −
Delete [] pvalue; // Delete array pointed to by pvalue
Following the similar generic syntax of new operator, you can allocate for a multi-dimensional array as follows −
Double** pvalue = NULL; // Pointer initialized with null
Pvalue = new double [3][4]; // Allocate memory for a 3x4 array
However, the syntax to release the memory for multi-dimensional array will still remain same as above −
Delete [] pvalue; // Delete array pointed to by pvalue
Dynamic Memory Allocation for Objects
Objects are no different from simple data types. For example, consider the following code where we are going to use an array of objects to clarify the concept−
#include <iostream>
Using namespace std;
Class Box {
public:
Box() {
cout << "Constructor called!" <<endl;
}
~Box() {
cout << "Destructor called!" <<endl;
}
};
Int main() {
Box* myBoxArray = new Box[4];
delete [] myBoxArray; // Delete array
return 0;
}
If you were to allocate an array of four Box objects, the Simple constructor would be called four times and similarly while deleting these objects, destructor will also be called same number of times.
If we compile and run above code, this would produce the following result −
Constructor called!
Constructor called!
Constructor called!
Constructor called!
Destructor called!
Destructor called!
Destructor called!
Destructor called!
Key Takeaway
A good understanding of how dynamic memory really works in C++ is essential to becoming a good C++ programmer. Memory in your C++ program is divided into two parts −
- The stack − All variables declared inside the function will take up memory from the stack.
- The heap − This is unused memory of the program and can be used to allocate the memory dynamically when program runs.
Every object in C++ has access to its own address through an important pointer called this pointer. The this pointer is an implicit parameter to all member functions. Therefore, inside a member function, this may be used to refer to the invoking object.
Friend functions do not have a this pointer, because friends are not members of a class. Only member functions have a this pointer.
Let us try the following example to understand the concept of this pointer −
#include <iostream>
Using namespace std;
Class Box {
public:
// Constructor definition
Box(double l = 2.0, double b = 2.0, double h = 2.0) {
cout <<"Constructor called." << endl;
length = l;
breadth = b;
height = h;
}
double Volume() {
return length * breadth * height;
}
int compare(Box box) {
return this->Volume() > box.Volume();
}
private:
double length; // Length of a box
double breadth; // Breadth of a box
double height; // Height of a box
};
Int main(void) {
Box Box1(3.3, 1.2, 1.5); // Declare box1
Box Box2(8.5, 6.0, 2.0); // Declare box2
if(Box1.compare(Box2)) {
cout << "Box2 is smaller than Box1" <<endl;
} else {
cout << "Box2 is equal to or larger than Box1" <<endl;
}
return 0;
}
When the above code is compiled and executed, it produces the following result −
Constructor called.
Constructor called.
Box2 is equal to or larger than Box1
Key takeaway
Every object in C++ has access to its own address through an important pointer called this pointer. The this pointer is an implicit parameter to all member functions. Therefore, inside a member function, this may be used to refer to the invoking object.
Friend functions do not have a this pointer, because friends are not members of a class. Only member functions have a this pointer.
A program is consists of set of instructions. These instructions are not always written in linear sequence. Program is designed to make decisions and to execute set of instructions number of times. Control Structure satisfies these requirements.
Decision Making Statements
Decision making statements consists of two or more conditions specified to execute statement so that any condition becomes true or false another condition executes.
The if-else statement
The if else statement consists of if followed by else. Condition specified in if becomes true then statement in if block executes. When condition mentioned in if becomes false then statements in else execute.
Structure of the if – else
If(Condition){
}
Else{
}
Example
#include<iostream>
Using namespace std;
Int main(){
Int number=7;
If(number<5){
Cout<<”number is less than 5”;
}
Else{
Cout<<”number is greater than 5”;
}
Return 0;
}
Nested if-else
The Nested if- else consist of multiple if-else statements in another if – else. In this specified conditions are executed from top. While executing nested if else, when condition becomes true statement related with it gets execute and rest conditions are bypassed. When all the conditions become false last else statement gets execute.
Structure of the nested if – else:
If(Condition){
}
Else if{
}
Else if{
}
Else{
}
}
Example
#include<iostream>
Using namespace std;
Int main ()
{
Int i=5
If(i==10)
Cout<<”i is 10”;
Else if(i==7)
Cout<<”i is 7”;
Else if(i==5)
Cout<<”i is 5”;
Else
Cout<<i is not present;
Return 0;
}
}
Here output is 5. When i=5 condition will match it will give is 5 output if not then last output will be i is not present.
Goto
The goto
The goto is an unconditional jump type of statement. The goto is used to jump between programs from one point to another. The goto can be used to jump out of the nested loop.
Structure of the goto:
Goto label;
.
.
Label;
While executing program goto provides the information to goto instruction defined as a label. This label is initialized with goto statement before.
Example
#include<iostream>
Using namespace std;
Int main()
{
Number();
Return 0;
}
Void numbers()
{
Int a=1;
Label;
Cout<<a<<” “;
a++;
If(a<=5)
Goto label;
}
When the compiler will come to goto label then control will goto label defined before the loop and output will be 1 2 3 4 5.
Break
The break statement is used to terminate the execution where number of iterations is not unknown. It is used in loop to terminate the loop and then control goes to the first statement of the loop. The break statement is also in switch case.
Structure of the break:
Break;
Example
#include<iostream>
Using namespace std;
Int main(){
Int x;
For(x=10;x>=2;x--){
Cout<<”x:”<<x<<endl;
If(x==6)
{
Break;
}
}
Cout<<”break”;
Return 0;
}
When the compiler will reach to break it will come out and print the output. Output will be x=1,x=9,x=8,x=7,x=6,break.
Continue
The continue statement work like break statement but it is opposite of break. The Continue statement continues with execution of program by jumping from current code instruction to the next.
Structure of the break:
Continue;
Example
#include<iostream>
Using namespace std;
Int main()
{
For(int i=1;int<=5;i++){
If(i==3)
Continue;
Else
Cout<<i<<” “;
}
Return 0;
}
Output is 1 2 3 4 5.
Switch case
The switch statement is a branch selection statement. The switch statement consists of multiple cases and each case has number of statement. When the condition gets satisfied the statements associated with that case execute. The break statement is used inside switch loop to terminate the execution.
Structure of the switch statement:
Switch(n)
{
Case 1:
Break;
Case 2:
Break;
Case 3:
Break;
Default;
}
Example
Int main(){
Int a=2;
Switch(a){
Case 1: cout<<”Hello”;
Break;
Case 2:cout<<”World”;
Break;
Default:cout<<”Hello World”;
Break;
}
}
Return 0;
}
Output is “world”.
Loop statement like for loop
Loop Statements
The for loop
The for loop executes repeatedly until the condition specified in for loop is satisfied. When the condition becomes false it resumes with statement written in for loop.
Structure of the for loop:
For(initialization; condition
;increment/decrement){
Statements;
Initialization is the assignment to the variable which controls the loop. Condition is an expression, specifies the execution of loop. Increment/ decrement operator changes the variable as the loop executes.
Example:
#include<iostream>
Using namespace std;
Int main(){
For(i=1;i<=5;i++)
{
Cout<<I;
}
Return 0;
}
Output is 1 2 3 4 5.
Nested for loop
Nested for loop is for within for loop.
Structure of the nested for loop:
For(initialization; condition
;increment/decrement){
Statements;
For(initialization; condition
;increment/decrement){
Statements;
While Loop
The while loop consists of a condition with set of statements. The Execution of the while loop is done until the condition mentioned in while is true. When the condition becomes false the control comes out of loop.
Structure of the while loop:
While(Condition)
{
Statements;
}
Example:
Int main(){
Int x=1;
While(x<=10){
Cout<<I;
i++;
}
}
X is initialized to 1. The loop will execute till x<=6.
Output will be 1 2 3 4 5 6 7 8 9 10.
Do – while loop
The do – while loop consist of consist of condition mentioned at the bottom with while. The do – while executes till condition is true. As the condition is mentioned at bottom do – while loop is executed at least once.
Structure of the do – while:
Do{
Statements
}
While(Condition);
Example:
#include<iostream>
Using namespace std;
Int main(){
Int x=1;
Do{
Cout<<x;
x++;
}while(x<=10);
Return 0;
}
Output is: 1 2 3 4 5 6 7 8 9 10.
Main features of OOPs are:
Class
- Class is used to create user defined data type.
- C++ provides various built in types like float, int and char.
- Similarly, if user wants to create his own data type then he can create it with the help of class.
Class Book
- Here user defined data type “Book” is created with the help of class keyword class.
- Class consists of data members (variables) and associated member functions.
Inheritance
- It is a mechanism of extending functionality from one class to another.
- Class can reuse the code of another class if they are tied together by inheritance.
- The original class is called as base/super/parent class and inherited class is called derived/sub/child class.
- Sub class inherits some or all the features of base class.
- Sub class adds its own features to base class so that it has combined features of both the classes.
- In this way it supports the concept of reusability.
- It can very well model hierarchical classification as shown below.
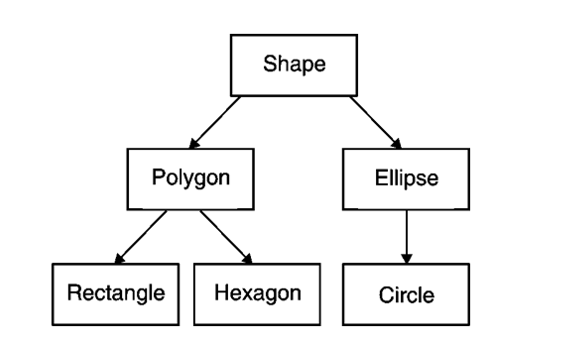
Fig: Inheritance
- Here Shape is considered as base class.
- Polygon and Ellipse are subclasses of class Shape.
- Rectangle and Hexagon are subclasses of Polygon so they inherit features of Polygon class.
- Similarly, Circle inherits features of Ellipse class.
Polymorphism
- It is ability to take more than one form depending upon the data types used in program.
- Consider the operation of finding area of any geometrical figure shown in fig.
- The operation “Area” is same but will take different forms depending upon type and number of arguments passed to “Area”.
- “Area” of square calculates area of square whereas “Area” of rectangle calculates area of rectangle.
- Though operation “Area” is same but it takes different forms depending upon type of geometrical figure.
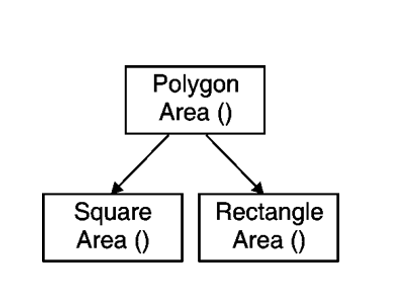
Fig: Polymorphism
Encapsulation
All C++ programs are composed of the following two fundamental elements −
Program statements (code) − This is the part of a program that performs actions and they are called functions.
Program data − The data is the information of the program which gets affected by the program functions.
Encapsulation is an Object Oriented Programming concept that binds together the data and functions that manipulate the data, and that keeps both safe from outside interference and misuse. Data encapsulation led to the important OOP concept of data hiding.
Data encapsulation is a mechanism of bundling the data, and the functions that use them and data abstraction is a mechanism of exposing only the interfaces and hiding the implementation details from the user.
C++ supports the properties of encapsulation and data hiding through the creation of user-defined types, called classes. We already have studied that a class can contain private, protected and public members. By default, all items defined in a class are private
Data Abstraction
- Data Abstraction is a process of providing only the essential details to the outside world and hiding the internal details, i.e., representing only the essential details in the program.
- Data Abstraction is a programming technique that depends on the seperation of the interface and implementation details of the program.
- Let's take a real life example of AC, which can be turned ON or OFF, change the temperature, change the mode, and other external components such as fan, swing. But, we don't know the internal details of the AC, i.e., how it works internally. Thus, we can say that AC seperates the implementation details from the external interface.
- C++ provides a great level of abstraction. For example, pow() function is used to calculate the power of a number without knowing the algorithm the function follows.
In C++ program if we implement class with private and public members then it is an example of data abstraction.
Introduction to Class
- Class is used to create user defined data type.
- C++ provides various built in types like float, int and char.
- Similarly, if user wants to create his own data type then he can create it with the help of class.
Class Book
- Here user defined data type “Book” is created with the help of class keyword class.
- Class consists of data members (variables) and associated member functions.
Structure of a class
Class |
Data Members |
Member functions |
- Data members (variables) represent attributes and member functions represent behaviour of class.
Structure of a “Book” class
Book |
Data Members Name Pages Price |
Member functions Accept() Display() |
- Class only provides template for object and does not create any memory space for object. Hence class is logical abstract entity.
Structure of class declaration
Class class_name
{
Access specifier:
Data Members;
Access specifier:
Member Functions;
};
Class
- Classes are created using keyword “class”.
- The body of class is enclosed within curly braces and terminated by semicolon.
- Class contains data members and Member functions. They are called as class members.
The main purpose of C++ programming is to add object orientation to the C programming language and classes are the central feature of C++ that supports object-oriented programming and are often called user-defined types.
A class is used to specify the form of an object and it combines data representation and methods for manipulating that data into one neat package. The data and functions within a class are called members of the class.
Define C++ Objects
A class provides the blueprints for objects, so basically an object is created from a class. We declare objects of a class with exactly the same sort of declaration that we declare variables of basic types. Following statements declare two objects of class Box −
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
Both of the objects Box1 and Box2 will have their own copy of data members.
Accessing the Data Members
The public data members of objects of a class can be accessed using the direct member access operator (.). Let us try the following example to make the things clear −
#include <iostream>
Using namespace std;
Class Box {
public:
double length; // Length of a box
double breadth; // Breadth of a box
double height; // Height of a box
};
Int main() {
Box Box1; // Declare Box1 of type Box
Box Box2; // Declare Box2 of type Box
double volume = 0.0; // Store the volume of a box here
// box 1 specification
Box1.height = 5.0;
Box1.length = 6.0;
Box1.breadth = 7.0;
// box 2 specification
Box2.height = 10.0;
Box2.length = 12.0;
Box2.breadth = 13.0;
// volume of box 1
volume = Box1.height * Box1.length * Box1.breadth;
cout << "Volume of Box1 : " << volume <<endl;
// volume of box 2
volume = Box2.height * Box2.length * Box2.breadth;
cout << "Volume of Box2 : " << volume <<endl;
return 0;
}
When the above code is compiled and executed, it produces the following result −
Volume of Box1 : 210
Volume of Box2 : 1560
It is important to note that private and protected members can not be accessed directly using direct member access operator (.). We will learn how private and protected members can be accessed.
Classes and Objects in Detail
So far, you have got very basic idea about C++ Classes and Objects. There are further interesting concepts related to C++ Classes and Objects which we will discuss in various sub-sections listed below −
Sr.No | Concept & Description |
1 | Class Member Functions A member function of a class is a function that has its definition or its prototype within the class definition like any other variable. |
2 | Class Access Modifiers A class member can be defined as public, private or protected. By default members would be assumed as private. |
3 | Constructor & Destructor A class constructor is a special function in a class that is called when a new object of the class is created. A destructor is also a special function which is called when created object is deleted. |
4 | Copy Constructor The copy constructor is a constructor which creates an object by initializing it with an object of the same class, which has been created previously. |
5 | Friend Functions A friend function is permitted full access to private and protected members of a class. |
6 | Inline Functions With an inline function, the compiler tries to expand the code in the body of the function in place of a call to the function. |
7 | This Pointer Every object has a special pointer this which points to the object itself. |
8 | Pointer to C++ Classes A pointer to a class is done exactly the same way a pointer to a structure is. In fact a class is really just a structure with functions in it. |
9 | Static Members of a Class Both data members and function members of a class can be declared as static. |
Access modifiers are also called access specifiers
C++ supports three access specifiers as follows:
1. Private
- It is default access specifier.
- Members declared under private section can be accessed only from within the class and not from outside the class.
Class Example
{
Int x;// x is private.
Public:
Void accept()
{
Cin>>x;
Cout<<x;
}
};
Variable x is accessible to accept() function as it is member function of the same class.
However if we try to access ‘x’ from outside the class it will throw error.
Int main()
{
Example Obj;
Obj.x=10; // error as x is private
:
:
}
2. public
Members declared under public section can be accessed from within the class and from outside the class.
Class Example
{
Int x;
Public:
Int y;//y is public
Void accept() // accept() is public
{
:
:
}
};
Int main()
{
Example obj;
Obj.y=10; // OK as obj is public
:
:
}
Variable y is public so it can be accessed from outside the class.
3. Protected
Members declared under protected section can be accessed from within the class and from derived class. It is used only in inheritance.
- Functions declared or defined in a class are called as Member functions or methods.
- They can be declared under private, public or protected section.
- They can access private data members of a class.
- One member function can call other member function directly without dot operator.
- Member functions are declared inside class but may be defined within or outside the class.
- Member function defined within a class is written as follows:
Returntype functionname(argument list)
{
Function Definition
}
Eg. Void display()
{
cout<<name<<pages<<price;
}
- Member function defined outside the class is written as follows:
Returntype classname :: functionname(argument list)
{
Function Definition
}
Example
Void Book :: display()
{
Cout<<name<<pages<<price;
}
Program
Write a C++ program to find if a number is even or odd using scope resolution operator.
Solution:
#include<iostream> Using namespace std ; Class Example { Int number; Public: Void accept(); Void EvenOdd(); }; Void Example::accept() { Cout<<“Enter number”; Cin>>number; } Void Example:: EvenOdd() { If(number%2= =0) Cout<<number<<“is even number”; Else Cout<<number<<“is odd number”; } Void main() { Example O; O.accept(); O.EvenOdd(); } | //Header file
//Declares class Example //Declares data member number. It is private data member. //Starts public section of class Example //Declares functions “accept” and EvenOdd
//End of class definition //Defining accept function outside class using scope resolution operator.
//Accepting value for number from user //End of accept function definition //Defining EvenOdd function outside class using scope resolution operator.
//Checks if number is even or odd
//End of EvenOddfunction //Start of main() function //Creates object O //Calls accept function //Calls EvenOdd function //End of main |
When a variable is declared as static, space for it gets allocated for the lifetime of the program. Even if the function is called multiple times, space for the static variable is allocated only once and the value of variable in the previous call gets carried through the next function call. This is useful for implementing in C/C++ or any other application where previous state of function needs to be stored.
// C++ program to demonstrate
// the use of static Static
// variables in a Function
#include <iostream>
#include <string>
Using namespace std;
Void demo()
{
// static variable
Static int count = 0;
Cout << count << " ";
// value is updated and
// will be carried to next
// function calls
Count++;
}
Int main()
{
For (int i=0; i<5; i++)
Demo();
Return 0;
}
Output:
0 1 2 3 4
You can see in the above program that the variable count is declared as static. So, its value is carried through the function calls. The variable count is not getting initialized for every time the function is called.
Static variables in a class: As the variables declared as static are initialized only once as they are allocated space in separate static storage so, the static variables in a class are shared by the objects. There can not be multiple copies of same static variables for different objects. Also because of this reason static variables can not be initialized using constructors.
// C++ program to demonstrate static
// variables inside a class
#include<iostream>
Using namespace std;
Class GfG
{
Public:
Static int i;
GfG()
{
// Do nothing
};
};
Int main()
{
GfG obj1;
GfG obj2;
Obj1.i =2;
Obj2.i = 3;
// prints value of i
Cout << obj1.i<<" "<<obj2.i;
}
You can see in the above program that we have tried to create multiple copies of the static variable i for multiple objects. But this didn’t happen. So, a static variable inside a class should be initialized explicitly by the user using the class name and scope resolution operator outside the class as shown below:
// C++ program to demonstrate static
// variables inside a class
#include<iostream>
Using namespace std;
Class GfG
{
Public:
Static int i;
GfG()
{
// Do nothing
};
};
Int GfG::i = 1;
Int main()
{
GfG obj;
// prints value of i
Cout << obj.i;
}
Output:
1
- Like array of other user-defined data types, an array of type class can also be created.
- The array of type class contains the objects of the class as its individual elements.
- Thus, an array of a class type is also known as an array of objects.
- An array of objects is declared in the same way as an array of any built-in data type.
Syntax:
Class_name array_name [size];
Example:
#include <iostream>
Class MyClass {
Int x;
Public:
Void setX(int i) { x = i; }
Int getX() { return x; }
};
Void main()
{
MyClass obs[4];
Int i;
For(i=0; i < 4; i++)
Obs[i].setX(i);
For(i=0; i < 4; i++)
Cout << "obs[" << i << "].getX(): " << obs[i].getX() << "\n";
Getch();
}
Output:
Obs[0].getX(): 0
Obs[1].getX(): 1
Obs[2].getX(): 2
Obs[3].getX(): 3
Key takeaway
- Like array of other user-defined data types, an array of type class can also be created.
- The array of type class contains the objects of the class as its individual elements.
- Thus, an array of a class type is also known as an array of objects.
- An array of objects is declared in the same way as an array of any built-in data type.
- Inline functions are functions which are replaced by corresponding function code for a function call.
- Usually function call takes control to corresponding function definition.
- This involves executing series of instructions, pushing elements in stack, saving register and coming back to function call.
- This process consumes execution time.
- So if function definition is very small(one or two statements)then instead of passing control to function definition function call is replaced by its corresponding code. Such functions are called as inline functions.
- Inline functions are defined using keyword inline as follows:
Inline return-type function-name(argument list)
{
Function body
}
Example
Inline int add(int x,int y, int z)
{
Return(x*y*z);
}
Function call
Add(10,20,25);
Will be expanded by its function definition.
Though we define function as inline compiler may ignore the request in following cases
- Function definition is large.
- Function is recursive
- Function return values
Program
Write a C++ program to illustrate inline functions.
Solution:
# include<iostream>
Using namespace std ;
Inline void data(int x) // Step 1 Defines function data as inline
{
Return (x%2==0)?cout<<“Even\n”:cout<<“Odd”;// Step 2
}
Void main()
{
Data(10);// Step 3 Calling inline function
Data(5);// Step 4
}
Explanation
Step 1:Function data is declared as inline with one argument.
It finds if a number is even or odd.
Inline void data(int x) //Defines function data as inline
Step 2:Conditional operator is used to check if number is even or odd.
Return (x%2==0)?cout<<“Even\n”:cout<<“Odd”;
Step 3:Pass parameter 10 to data function. Here data function is expanded to its corresponding code instead of passing control to function definition. It gives output as even number.
Data(10);
Step 4:Pass parameter 5 to data function. It gives output as odd number.
Data(5);
Output
Even
Odd
If a function is defined as a friend function in C++, then the protected and private data of a class can be accessed using the function.
By using the keyword friend compiler knows the given function is a friend function.
For accessing the data, the declaration of a friend function should be done inside the body of a class starting with the keyword friend.
Declaration of friend function in C++
- Class class_name
- {
- Friend data_type function_name(argument/s); // syntax of friend function.
- };
In the above declaration, the friend function is preceded by the keyword friend. The function can be defined anywhere in the program like a normal C++ function. The function definition does not use either the keyword friend or scope resolution operator.
Characteristics of a Friend function:
- The function is not in the scope of the class to which it has been declared as a friend.
- It cannot be called using the object as it is not in the scope of that class.
- It can be invoked like a normal function without using the object.
- It cannot access the member names directly and has to use an object name and dot membership operator with the member name.
- It can be declared either in the private or the public part.
C++ friend function Example
Let's see the simple example of C++ friend function used to print the length of a box.
- #include <iostream>
- Using namespace std;
- Class Box
- {
- Private:
- Int length;
- Public:
- Box(): length(0) { }
- Friend int printLength(Box); //friend function
- };
- Int printLength(Box b)
- {
- b.length += 10;
- Return b.length;
- }
- Int main()
- {
- Box b;
- Cout<<"Length of box: "<< printLength(b)<<endl;
- Return 0;
- }
Output:
Length of box: 10
Let's see a simple example when the function is friendly to two classes.
- #include <iostream>
- Using namespace std;
- Class B; // forward declarartion.
- Class A
- {
- Int x;
- Public:
- Void setdata(int i)
- {
- x=i;
- }
- Friend void min(A,B); // friend function.
- };
- Class B
- {
- Int y;
- Public:
- Void setdata(int i)
- {
- y=i;
- }
- Friend void min(A,B); // friend function
- };
- Void min(A a,B b)
- {
- If(a.x<=b.y)
- Std::cout << a.x << std::endl;
- Else
- Std::cout << b.y << std::endl;
- }
- Int main()
- {
- A a;
- B b;
- a.setdata(10);
- b.setdata(20);
- Min(a,b);
- Return 0;
- }
Output:
10
In the above example, min() function is friendly to two classes, i.e., the min() function can access the private members of both the classes A and B.
C++ Friend class
A friend class can access both private and protected members of the class in which it has been declared as friend.
Let's see a simple example of a friend class.
- #include <iostream>
- Using namespace std;
- Class A
- {
- Int x =5;
- Friend class B; // friend class.
- };
- Class B
- {
- Public:
- Void display(A &a)
- {
- Cout<<"value of x is : "<<a.x;
- }
- };
- Int main()
- {
- A a;
- B b;
- b.display(a);
- Return 0;
- }
Output:
Value of x is : 5
In the above example, class B is declared as a friend inside the class A. Therefore, B is a friend of class A. Class B can access the private members of class A.
Key takeaway
If a function is defined as a friend function in C++, then the protected and private data of a class can be accessed using the function.
By using the keyword friend compiler knows the given function is a friend function.
For accessing the data, the declaration of a friend function should be done inside the body of a class starting with the keyword friend.
References:
1. Object oriented programming By E. Balagurusamy.
2. C++ Programming –By D. Ravichandran
3. Let Us C++ By YashawantKanetkar.
4. Object Oriented Programming in C++ - Dr. G. T. Thampi, Dr. S. S. Mantha
5. Mastering C++ -By Venugopal.