Unit - 2
Introducing classes and objects
Classes
Class can be defined as the core part of Java. It is the basic construct in Java upon which the entire language is created since it defines the shape and nature of an object.
A class consist of group of objects having similar properties. It is a logical entity which can be more complex in nature.
Class Declaration
To declare a class, use the class keyword. A class can be defined as:
Class class_name{
Type obj_varaible1;
Type obj_variable2;
.....
Type obj_variableN;
Type method_name1(parameters){
//method body
}
....
Type method_nameN(parameters){
//method body
}
}
All the data or variables declare inside a class are known as instance variables. The actual logical resides within the methods. And the collection of methods and variables in class are known as members.
Example of Class
Consider an example of class student that has two instances name and grade. There is no method declaration.
Class Student{
String name;
Int grade;
}
Whenever a class is declare that means a template is created, it is not an actual object. Therefore, object creation and declaration we will see in further topics.
Similarly till now there is no method created in above example.
Methods
Java provides methods so much power and flexibility. Methods are collection of statements that are combined together to perform an specific operation.
Methods can be declare as
Type method_name(parameters){
//method body
}
In the above syntax type represents the type of data returned by the method. If a method doesn’t return a value, in that case the data type of method will be void.
Parameters are those variables which are used to receive the value of arguments passed to the method. In case of no parameter, parenthesis will be blank.
Those methods which have a return types, a value is return by the method using the following syntax:
Return value;
Adding a Method to class
Class Student{
String name;
Int grade;
Void info() // display infor of students
System.out.print("Name is " + name + “ “ + “Grade is” + grade);
}
Class Example{
Public static void main(String args[]}
Student mystud1 = new Student();
Student mystud2 = new Student();
//assigning values to Student’s object
Mystud1.name = “Rahul”;
Mystud1.grade = 5;
Mystud2.name = “Sita”;
Mystud2.grade = 6;
Mystud1.info();
Mystud2.info();
}
}
Output is
Name is Rahul Grade is 5
Name is Sita Grade is 6
Returning a Value
When a method is called it either returns a value or returns nothing. When a program calls a method, the control of program gets transferred to the method.
After executing the method the control goes back to the program in two cases either returns statement is encountered or it reaches the method closing braces.
In case method doesn’t return a value, then it must be declared as void.
There are two points which need to take care while retiring values:
- Data type of the value return by the method must be same as that of return type specified by the method.
- The variable where the return value will be stored must of same data type as the data type of returning variable.
Passing Parameters by Value
Parameters make a method more generalized. Such methods can operate on different types of data or can be used in various situations.
An example of parameterized method is
Int cube(int i)
{
Return i*i*i;
}
Objects
An object is an instance of class. In Java object can be defined as the physical as well as logical entity.
Objects can be described using three parameters:
- State is used to define the data or value of an object.
- Behavior represents the functionality of an object.
- Identity is used to assign a unique id to every object,
Creating an object
To create an object in Java a class is used.
Let’s take above example of class student. To create an object of student class, specify the class name followed by the object name and use the new keyboard.
Syntax to create an object is
Classname object_name = new classname();
See the objects mystud1 and mystud2 in the above example of Student class.
Accessing Instance variables and methods
User created objects can access the instance variables and methods. Following are the syntax to access instance variables and methods by objects:
/* object creation */
Classname object_name = new classname();
/* call a variable */
Object_name.variableName;
/* call a class method */
Object_name.MethodName();
See the complete program above.
Data hiding is one of the important features of Object Oriented Programming which allows preventing the functions of a program to access directly the internal representation of a class type. The access restriction to the class members is specified by the labeled public, private, and protected sections within the class body. The keywords public, private, and protected are called access specifiers.
A class can have multiple public, protected, or private labeled sections. Each section remains in effect until either another section label or the closing right brace of the class body is seen. The default access for members and classes is private.
Class Base {
Public:
// public members go here
Protected:
// protected members go here
Private:
// private members go here
};
The public Members
A public member is accessible from anywhere outside the class but within a program. You can set and get the value of public variables without any member function as shown in the following example −
#include <iostream>
Using namespace std;
Class Line {
Public:
Double length;
Void setLength( double len );
Double getLength( void );
};
// Member functions definitions
Double Line::getLength(void) {
Return length ;
}
Void Line::setLength( double len) {
Length = len;
}
// Main function for the program
Int main() {
Line line;
// set line length
Line.setLength(6.0);
Cout << "Length of line : " << line.getLength() <<endl;
// set line length without member function
Line.length = 10.0; // OK: because length is public
Cout << "Length of line : " << line.length <<endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result−
Length of line : 6
Length of line : 10
The private Members
A private member variable or function cannot be accessed, or even viewed from outside the class. Only the class and friend functions can access private members.
By default all the members of a class would be private, for example in the following class width is a private member, which means until you label a member, it will be assumed a private member −
Class Box {
Double width;
Public:
Double length;
Void setWidth( double wid );
Double getWidth( void );
};
Practically, we define data in private section and related functions in public section so that they can be called from outside of the class as shown in the following program.
#include <iostream>
Using namespace std;
Class Box {
Public:
Double length;
Void setWidth( double wid );
Double getWidth( void );
Private:
Double width;
};
// Member functions definitions
Double Box::getWidth(void) {
Return width ;
}
Void Box::setWidth( double wid ) {
Width = wid;
}
// Main function for the program
Int main() {
Box box;
// set box length without member function
Box.length = 10.0; // OK: because length is public
Cout << "Length of box : " << box.length <<endl;
// set box width without member function
// box.width = 10.0; // Error: because width is private
Box.setWidth(10.0); // Use member function to set it.
Cout << "Width of box : " << box.getWidth() <<endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result−
Length of box : 10
Width of box : 10
The protected Members
A protected member variable or function is very similar to a private member but it provided one additional benefit that they can be accessed in child classes which are called derived classes.
You will learn derived classes and inheritance in next chapter. For now you can check following example where I have derived one child class SmallBox from a parent class Box.
Following example is similar to above example and here width member will be accessible by any member function of its derived class SmallBox.
#include <iostream>
Using namespace std;
Class Box {
Protected:
Double width;
};
Class SmallBox:Box { // SmallBox is the derived class.
Public:
Void setSmallWidth( double wid );
Double getSmallWidth( void );
};
// Member functions of child class
Double SmallBox::getSmallWidth(void) {
Return width ;
}
Void SmallBox::setSmallWidth( double wid ) {
Width = wid;
}
// Main function for the program
Int main() {
SmallBox box;
// set box width using member function
Box.setSmallWidth(5.0);
Cout << "Width of box : "<< box.getSmallWidth() << endl;
Return 0;
}
When the above code is compiled and executed, it produces the following result−
Width of box : 5
KEY TAKEAWAY
Data hiding is one of the important features of Object Oriented Programming which allows preventing the functions of a program to access directly the internal representation of a class type. The access restriction to the class members is specified by the labelled public, private, and protected sections within the class body. The keywords public, private, and protected are called access specifiers.
A class can have multiple public, protected, or private labeled sections. Each section remains in effect until either another section label or the closing right brace of the class body is seen. The default access for members and classes is private.
They are used to control the visibility of members like classes, variables and methods.
- Public: Accessible to other classes defined in the same package as well as those defined in other packages.
- Default: Accessible to other classes in the same package but will be inaccessible to classes outside the package.
- Private: Access is restricted to class only.
- Protected: Accessed only by the subclasses in other package or any class within the package of the class of the protected member
Access specifiers are used for the following:
- Access specifiers for classes
- Access specifiers for instance variables
- Access specifiers for methods
- Access specifiers for constructors
1. Access specifiers for classes
A class can be declared public. Private access specifier cannot be applied to a class, and it’s the same for protected. If there is no access specifier mentioned, default access restrictions will be applied.
2. Access specifiers for Instance variables
All four specifiers- public, private, default and protected will be applicable for instance variables. Public variable can be modified from all classes, in or outside the package. Variables are accessible from classes in the same package only is access specifier is not stated. For private variables, access is restricted to class.
3. Access specifiers for methods
All four specifiers- public, private, default and protected will be applicable for methods as well.
4. Access specifiers for constructors
All four specifiers may be applied to the constructor as well. For a public declare constructor, an object can be created for any other class. If no access specifier mentioned, the object could be created from any class inside the same package. For private access specifiers, object maybe created in that class only.
In Java, the static keyword is mostly used for memory management. With variables, methods, blocks, and nested classes, we can use the static keyword. The static keyword refers to a class rather than a specific instance of that class.
The static can take the form of:
- Variable (also known as a class variable)
- Method (also known as a class method)
- Block
- Nested class
Static variable
A static variable is defined as a variable that has been declared as static.
The static variable can be used to refer to a property that is shared by all objects (but not unique to each object), such as an employee's firm name or a student's college name.
The static variable is only stored in memory once in the class area, when the class is loaded.
Example of static variable
//Java Program to demonstrate the use of static variable
Class Student{
Int rollno;//instance variable
String name;
Static String college ="ITS";//static variable
//constructor
Student(int r, String n){
Rollno = r;
Name = n;
}
//method to display the values
Void display (){System.out.println(rollno+" "+name+" "+college);}
}
//Test class to show the values of objects
Public class TestStaticVariable1{
Public static void main(String args[]){
Student s1 = new Student(111,"Karan");
Student s2 = new Student(222,"Aryan");
//we can change the college of all objects by the single line of code
//Student.college="BBDIT";
s1.display();
s2.display();
}
}
Output
111 Karan ITS
222 Aryan ITS
Static method
Any method that uses the static keyword is referred to as a static method.
- A class's static method, rather than the class's object, belongs to the class.
- A static method can be called without having to create a class instance.
- A static method can access and update the value of a static data member.
Example:
//Java Program to demonstrate the use of a static method.
Class Student{
Int rollno;
String name;
Static String college = "ITS";
//static method to change the value of static variable
Static void change(){
College = "BBDIT";
}
//constructor to initialize the variable
Student(int r, String n){
Rollno = r;
Name = n;
}
//method to display values
Void display(){System.out.println(rollno+" "+name+" "+college);}
}
//Test class to create and display the values of object
Public class TestStaticMethod{
Public static void main(String args[]){
Student.change();//calling change method
//creating objects
Student s1 = new Student(111,"Karan");
Student s2 = new Student(222,"Aryan");
Student s3 = new Student(333,"Sonoo");
//calling display method
s1.display();
s2.display();
s3.display();
}
}
Output
111 Karan BBDIT
222 Aryan BBDIT
333 Sonoo BBDIT
Key takeaway
In Java, the static keyword is mostly used for memory management.
With variables, methods, blocks, and nested classes, we can use the static keyword.
The static keyword refers to a class rather than a specific instance of that class.
Java allows us to create multiple methods in a class even with the same method name, until their parameters are different. In this case methods are said to be overloaded and this complete process is known as method overloading.
Method overloading is an implementation of polymorphism in a code which is “One interface, multiple methods” paradigm.
To invoke an overloaded method, it is important to pass the right number or arguments or parameters; since this will determine which version of method to actually call.
All the overloaded methods have different return type but we cant distinguish method based on the type of data they return.
Consider a simple example of method overloading:
Class Addition {
// Overloaded method takes two int parameters
public int add(int a, int b)
{
return (a + b);
}
// Overloaded method takes three int parameters
public int add(int a, int b, int c)
{
return (a + b + c);
}
// Overloaded method takes two double parameters
public double add(double a, double b)
{
return (a + b);
}
public static void main(String args[])
{
Addition a = new Addition();
System.out.println(a.add(100, 20));
System.out.println(a.add(11, 2, 3));
System.out.println(a.add(0.5, 2.5));
}
}
Output:
120
16
3.0
While creating a overloaded method it s not necessary that all the methods must relate to one another. For example one add() method can do addition, while other add() method can concatenate string; since each version of a method perform a separate activity you desire.
However, it is preferable to see a relationship between overloaded method as shown in the above example, where all add() method doing addition.
Benefits of Method Overloading
Technique over-burdening builds the coherence of the program.
This gives adaptability to developers with the goal that they can call similar strategy for various sorts of information.
This makes the code look spotless.
This lessens the execution time in light of the fact that the limiting is done in accumulation time itself.
Technique over-burdening limits the intricacy of the code.
With this, we can utilize the code once more, which saves memory.
Constructors perform the job of initializing an object. It resembles a method in Java, but a constructor is not a method and it doesn’t have any return type.
Properties of a constructor:
1. Name of the constructor is the same as its class name.
2. A default constructor is always present in a class implicitly.
3. There are three main types of constructors namely default, parameterized and copy constructors.
4. A constructor in Java can not be abstract, final, static and Synchronized.
Public class NewClass{
//This is the constructor
NewClass(){
}}
The new keyword here creates the object of class NewClass and invokes the constructor to initialize this newly created object.
Default constructor:
A default constructor is a constructor that is created implicitly by the JVM.
Program to demonstrate default constructors:
Class student
{
Int id;
String name;
Student()
{
System.out.println("Student id is 20 and name is Amruta");
}
Public static void main(String args[])
{
Student s = new student();
}
}
Output:

Parameterized constructor:
It is a constructor that has to be created explicitly by the programmer. This constructor has parameters that can be passed when a constructor is called at the time of object creation.
Program:
Class Student4
{
Int id;
String name;
Student4(int i,String n)
{
Id = i;
Name = n;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student4 s1 = new Student4(20,"Rema");
Student4 s2 = new Student4(23,"Sadhna");
s1.display();
s2.display();
}
}
Output:

Copy constructor:
A content of one constructor can be copied into another constructor using the objects created. Such a constructor is called as a copy constructor.
Program:
Class Student6
{
Int id;
String name;
Student6(int i,String n)
{
Id = i;
Name = n;
}
Student6(Student6 s)
{
Id = s.id;
Name =s.name;
}
Void display()
{
System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student6 s1 = new Student6(20,"AMRUTA");
Student6 s2 = new Student6(s1);
s1.display();
s2.display();
}
}
Output:

Constructor Overloading
Like method overloading we can also do constructor overloading. Overloaded constructors in Java can be implemented in the same way as method overloading. We can have more than one constructor with different parameter list with same name. Each constructor performs a different task.
Compiler differentiates between each constructor on the basis of their parameter list.
Example of constructor loading is given below:
Class Add {
int x;
int y;
// Overloaded constructor takes two int parameters
Add(int a, int b)
{
x = a;
y = b;
}
// Overloaded constructor single int parameters
Add(int a)
{
x = y = a;
}
// Overloaded constructor takes no parameters
Add()
{
x = 6;
y = 5;
}
int sum() {
return x + y;
}
}
Class OverloadAdd{
public static void main(String args[])
{
// create objects using the various constructors
Add cons1 = new Add(23, 1);
Add cons2 = new Add(8);
Add cons3 = new Add();
int s;
s = cons1.sum()
System.out.println(“Sum of constructor1 =” + s);
s = cons2.sum()
System.out.println(“Sum of constructor2 =” + s);
s = cons3.sum()
System.out.println(“Sum of constructor3 =” + s);
}
}
Output
Sum of constructor1 = 24
Sum of constructor2 = 16
Sum of constructor3 = 11
This keyword is a reference variable that refers to current object. It can be used in number of scenarios.
- Used to refer instance variable of the current class
- can be used in a constructor
- can be passed as an argument in the method call and constructor call
- can be used to invoke current class constructor
Program:
Class thisDemo
{
int i;
int j;
// Parameterized constructor
thisDemo(int i, int j)
{
this.i = i;
this.j = j;
}
void display()
{
System.out.println("i=" +i);
System.out.println("j=" +j);
}
public static void main(String[] args)
{
thisDemo object = new thisDemo(10, 20);
object.display();
}
}
Output:
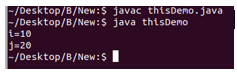
In java, garbage means unreferenced objects.
Garbage Collection is process of reclaiming the runtime unused memory automatically. In other words, it is a way to destroy the unused objects.
To do so, we were using free() function in C language and delete() in C++. But, in java it is performed automatically. So, java provides better memory management.
Advantage of Garbage Collection
- It makes java memory efficient because garbage collector removes the unreferenced objects from heap memory.
- It is automatically done by the garbage collector(a part of JVM) so we don't need to make extra efforts.
How can an object be unreferenced?
There are many ways:
- By nulling the reference
- By assigning a reference to another
- By anonymous object etc.
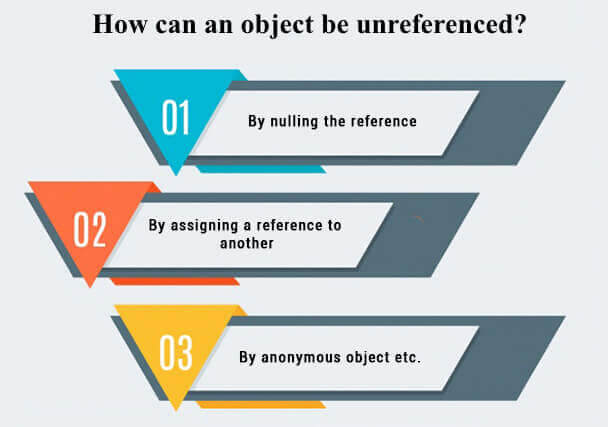
Fig: How can an object be unreferenced?
1) By nulling a reference:
- Employee e=new Employee();
- e=null;
2) By assigning a reference to another:
- Employee e1=new Employee();
- Employee e2=new Employee();
- e1=e2;//now the first object referred by e1 is available for garbage collection
3) By anonymous object:
- New Employee();
Finalize() method
The finalize() method is invoked each time before the object is garbage collected. This method can be used to perform cleanup processing. This method is defined in Object class as:
Protected void finalize(){}
Note: The Garbage collector of JVM collects only those objects that are created by new keyword. So if you have created any object without new, you can use finalize method to perform cleanup processing (destroying remaining objects).
Gc() method
The gc() method is used to invoke the garbage collector to perform cleanup processing. The gc() is found in System and Runtime classes.
- Public static void gc(){}
Note: Garbage collection is performed by a daemon thread called Garbage Collector(GC). This thread calls the finalize() method before object is garbage collected.
Simple Example of garbage collection in java
- Public class TestGarbage1{
- Public void finalize(){System.out.println("object is garbage collected");}
- Public static void main(String args[]){
- TestGarbage1 s1=new TestGarbage1();
- TestGarbage1 s2=new TestGarbage1();
- s1=null;
- s2=null;
- System.gc();
- }
- }
object is garbage collected
object is garbage collected
Note: Neither finalization nor garbage collection is guaranteed.
Key takeaway
Garbage Collection is process of reclaiming the runtime unused memory automatically. In other words, it is a way to destroy the unused objects.
To do so, we were using free() function in C language and delete() in C++. But, in java it is performed automatically. So, java provides better memory management.
Finalize () method
Finalize() is the method of Object class. This method is called just before an object is garbage collected. Finalize() method overrides to dispose system resources, perform clean-up activities and minimize memory leaks.
Syntax
Protected void finalize() throws Throwable
Throw
Throwable - the Exception is raised by this method
Example 1
- Public class JavafinalizeExample1 {
- Public static void main(String[] args)
- {
- JavafinalizeExample1 obj = new JavafinalizeExample1();
- System.out.println(obj.hashCode());
- Obj = null;
- // calling garbage collector
- System.gc();
- System.out.println("end of garbage collection");
- }
- @Override
- Protected void finalize()
- {
- System.out.println("finalize method called");
- }
- }
Output:
2018699554
End of garbage collection
Finalize method called
Key takeaway
Finalize() is the method of Object class. This method is called just before an object is garbage collected. Finalize() method overrides to dispose system resources, perform clean-up activities and minimize memory leaks.
The use of wrapper class in Java is to convert primitive types into object and object into primitive type. They are needed because Java is designed on the concept of object.
Need of Wrapper classes
- To convert the primitive data type into objects.
- Java provides the package name java.util.package which consists of all classes used to handle wrapper class.
- There are such data structures like ArrayList and Vector which doesn’t store primitive type, they only store Objects. Here we need wrapper class.
- It helps in synchronization of multithreading.
Different predefined wrapper classes
In Java, the java.lang package consist of total eight wrapper classes. These classes are:
Primitive Type | Wrapper class |
Boolean | Boolean |
Char | Character |
Byte | Byte |
Short | Short |
Int | Integer |
Long | Long |
Float | Float |
Double | Double |
Example of wrapper class
Public class Wrapper Demo {
public static void main(String[] args) {
Integer myInt = 7;
Double myDouble = 8.1;
Character myChar = 'D';
System.out.println(myInt.intValue());
System.out.println(myDouble.doubleValue());
System.out.println(myChar.charValue());
}
}
Output
7
8.1
D
Predefined Constructors for the wrapper classes
In Java, there are two types of constructor available for wrapper class:
- In first constructor, the primitive data type is passed as an argument.
- In second constructor, string is passed as an argument.
Example of both the constructor is:
Public class Demo
{
public static void main(String[] args)
{
Byte b1 = new Byte((byte) 20); //Constructor which takes byte value as an argument
Byte b2 = new Byte("20"); //Constructor which takes String as an argument
Short s1 = new Short((short) 10); //Constructor which takes short value as an argument
Short s2 = new Short("5"); //Constructor which takes String as an argument
Integer i1 = new Integer(10); //Constructor which takes int value as an argument
Integer i2 = new Integer("10"); //Constructor which takes String as an argument
Long l1 = new Long(20); //Constructor which takes long value as an argument
Long l2 = new Long("20"); //Constructor which takes String as an argument
Float f1 = new Float(14.2f); //Constructor which takes float value as an argument
Float f2 = new Float("8.6"); //Constructor which takes String as an argument
Float f3 = new Float(8.6d); //Constructor which takes double value as an argument
Double d1 = new Double(7.8d); //Constructor which takes double value as an argument
Double d2 = new Double("7.8"); //Constructor which takes String as an argument
Boolean bl1 = new Boolean(true); //Constructor which takes boolean value as an argument
Boolean bl2 = new Boolean("false"); //Constructor which takes String as an argument
Character c1 = new Character('E'); //Constructor which takes char value as an argument
Character c2 = new Character("xyz"); //Compile time error : String abc can not be converted to character
}
}
Normally, an array is a collection of similar type of elements which has contiguous memory location.
Java array is an object which contains elements of a similar data type. Additionally, The elements of an array are stored in a contiguous memory location. It is a data structure where we store similar elements. We can store only a fixed set of elements in a Java array.
Array in Java is index-based, the first element of the array is stored at the 0th index, 2nd element is stored on 1st index and so on.
Unlike C/C++, we can get the length of the array using the length member. In C/C++, we need to use the sizeof operator.
In Java, array is an object of a dynamically generated class. Java array inherits the Object class, and implements the Serializable as well as Cloneable interfaces. We can store primitive values or objects in an array in Java. Like C/C++, we can also create single dimensional or multidimensional arrays in Java.
Moreover, Java provides the feature of anonymous arrays which is not available in C/C++.
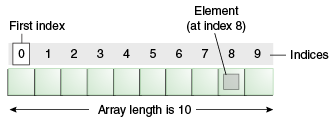
Fig: Example
Advantages
● Code Optimization: It makes the code optimized, we can retrieve or sort the data efficiently.
● Random access: We can get any data located at an index position.
Disadvantages
● Size Limit: We can store only the fixed size of elements in the array. It doesn't grow its size at runtime. To solve this problem, collection framework is used in Java which grows automatically.
Types of Array in java
There are two types of array.
● Single Dimensional Array
● Multidimensional Array
Single Dimensional Array in Java
Syntax to Declare an Array in Java
- DataType[] arr; (or)
- DataType []arr; (or)
- DataType arr[];
Instantiation of an Array in Java
ArrayRefVar=new datatype[size];
Example of Java Array
Let's see the simple example of java array, where we are going to declare, instantiate, initialize and traverse an array.
- //Java Program to illustrate how to declare, instantiate, initialize
- //and traverse the Java array.
- Class Testarray{
- Public static void main(String args[]){
- Int a[]=new int[5];//declaration and instantiation
- a[0]=10;//initialization
- a[1]=20;
- a[2]=70;
- a[3]=40;
- a[4]=50;
- //traversing array
- For(int i=0;i<a.length;i++)//length is the property of array
- System.out.println(a[i]);
- }}
Output:
10
20
70
40
50
Declaration, Instantiation and Initialization of Java Array
We can declare, instantiate and initialize the java array together by:
Int a[]={33,3,4,5};//declaration, instantiation and initialization
Let's see the simple example to print this array.
- //Java Program to illustrate the use of declaration, instantiation
- //and initialization of Java array in a single line
- Class Testarray1{
- Public static void main(String args[]){
- Int a[]={33,3,4,5};//declaration, instantiation and initialization
- //printing array
- For(int i=0;i<a.length;i++)//length is the property of array
- System.out.println(a[i]);
- }}
Output:
33
3
4
5
Passing Array to a Method in Java
We can pass the java array to method so that we can reuse the same logic on any array.
Let's see the simple example to get the minimum number of an array using a method.
- //Java Program to demonstrate the way of passing an array
- //to method.
- Class Testarray2{
- //creating a method which receives an array as a parameter
- Static void min(int arr[]){
- Int min=arr[0];
- For(int i=1;i<arr.length;i++)
- If(min>arr[i])
- Min=arr[i];
- System.out.println(min);
- }
- Public static void main(String args[]){
- Int a[]={33,3,4,5};//declaring and initializing an array
- Min(a);//passing array to method
- }}
Output:
3
Returning Array from the Method
We can also return an array from the method in Java.
- //Java Program to return an array from the method
- Class TestReturnArray{
- //creating method which returns an array
- Static int[] get(){
- Return new int[]{10,30,50,90,60};
- }
- Public static void main(String args[]){
- //calling method which returns an array
- Int arr[]=get();
- //printing the values of an array
- For(int i=0;i<arr.length;i++)
- System.out.println(arr[i]);
- }}
Output:
10
30
50
90
60
Multidimensional Array in Java
In such case, data is stored in row and column based index (also known as matrix form).
Syntax to Declare Multidimensional Array in Java
- DataType[][] arrayRefVar; (or)
- DataType [][]arrayRefVar; (or)
- DataType arrayRefVar[][]; (or)
- DataType []arrayRefVar[];
Example to instantiate Multidimensional Array in Java
Int[][] arr=new int[3][3];//3 row and 3 column
Example to initialize Multidimensional Array in Java
- Arr[0][0]=1;
- Arr[0][1]=2;
- Arr[0][2]=3;
- Arr[1][0]=4;
- Arr[1][1]=5;
- Arr[1][2]=6;
- Arr[2][0]=7;
- Arr[2][1]=8;
- Arr[2][2]=9;
Example of Multidimensional Java Array
Let's see the simple example to declare, instantiate, initialize and print the 2Dimensional array.
- //Java Program to illustrate the use of multidimensional array
- Class Testarray3{
- Public static void main(String args[]){
- //declaring and initializing 2D array
- Int arr[][]={{1,2,3},{2,4,5},{4,4,5}};
- //printing 2D array
- For(int i=0;i<3;i++){
- For(int j=0;j<3;j++){
- System.out.print(arr[i][j]+" ");
- }
- System.out.println();
- }
- }}
Output:
1 2 3
2 4 5
4 4 5
Multiplication of 2 Matrices in Java
In the case of matrix multiplication, a one-row element of the first matrix is multiplied by all the columns of the second matrix which can be understood by the image given below.
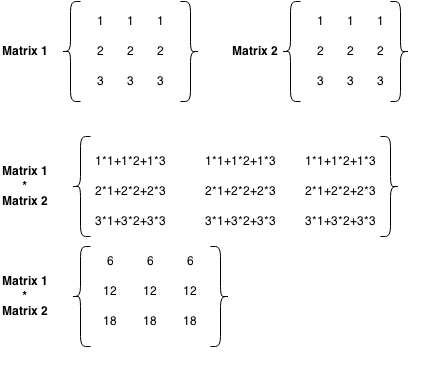
Fig: Matrix example
Let's see a simple example to multiply two matrices of 3 rows and 3 columns.
- //Java Program to multiply two matrices
- Public class MatrixMultiplicationExample{
- Public static void main(String args[]){
- //creating two matrices
- Int a[][]={{1,1,1},{2,2,2},{3,3,3}};
- Int b[][]={{1,1,1},{2,2,2},{3,3,3}};
- //creating another matrix to store the multiplication of two matrices
- Int c[][]=new int[3][3]; //3 rows and 3 columns
- //multiplying and printing multiplication of 2 matrices
- For(int i=0;i<3;i++){
- For(int j=0;j<3;j++){
- c[i][j]=0;
- For(int k=0;k<3;k++)
- {
- c[i][j]+=a[i][k]*b[k][j];
- }//end of k loop
- System.out.print(c[i][j]+" "); //printing matrix element
- }//end of j loop
- System.out.println();//new line
- }
- }}
Output:
6 6 6
12 12 12
18 18 18
Key takeaway
Java array is an object which contains elements of a similar data type. Additionally, the elements of an array are stored in a contiguous memory location. It is a data structure where we store similar elements. We can store only a fixed set of elements in a Java array.
Array in Java is index-based, the first element of the array is stored at the 0th index, 2nd element is stored on 1st index and so on.
Unlike C/C++, we can get the length of the array using the length member. In C/C++, we need to use the sizeof operator.
As the name suggests array of objects, Java provides us the flexibility to create an array of objects. This type of array store objects.
In an array, the element stores the reference variable of the object.
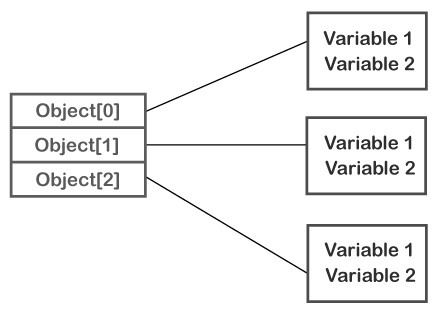
Fig: Array of Objects
Creating an Array of Objects
To create an array of Objects we use the Object class, which is the root class of all the classes.
Two syntax are there
Class_name[] objectArrayReference;
Or
Class_name objectArrayReference[];
Where objectArrayReference represents an array of objects.
Instantiating the Array of Objects
Syntax
Class_name obj[] = new Class_name[Array_length]
Consider a class name Person, and we want to instantiate that class with 5 objects or object reference then example will be
Person[] personObjects = new Person[2];
After instantiating an array of objects, we need to create the array of objects using the new keyboard. See the example below:
Class ExampleArray{
Public static void main(String args[])
{
//create an array of person object
Person[] obj = new Person[2] ;
//create & initialize actual person objects using constructor
Obj[0] = new Person("Rahul",24);
Obj[1] = new Person("Sita", 21);
//display the person object data
System.out.println("Person 1:");
Obj[0].display();
System.out.println("Person 2:");
Obj[1].display();
}
}
//Person class with person name and person age as attributes
Class Person
{
String per_name;
Int per_age;
//Person class constructor
Person(String n, int a)
{
Per_name = n;
Per_age = a;
}
Public void display()
{
System.out.print("Person Name = "+ per_name + " " + " Person age = "+per_age);
System.out.println();
}
}
Output
Person 1:
Person Name = RahulPerson age = 24
Person 2:
Person Name = SitaPerson age = 21
References:
1. Herbert Schildt, Java2: The Complete Reference, Tata McGraw-Hill
2. Object Oriented Programming with JAVA Essentilas and Applications, Mc Graw Hill
3. Core and Advanced Java, Black Book- dreamtech
4. Programming with JAVA- E Balagurusamy