Unit 1
C Language Fundamentals, Arrays And Strings
Array is defined as a collection of elements of same data type. Hence it is also called as homogeneous variable. Total number of elements in array defines size of array. Position of element in an array defines index or subscript of particular element. Array index always starts with zero.
Bound Checking Arrays (1-D, 2-D)
- One Dimensional Array:
The array which is used to represent and store data in a linear form is called as 'single or one dimensional array.'
Syntax:
Data-type array_name[size];
Example 2:
Int a[3] = {2, 3, 5};
Char ch[10] = "Data" ;
Float x[3] = {2.5, 3.50,489.98} ;
Total Size of an array(in Bytes) can be calculated as follows:
Total size(in bytes) = length of array * size of data type
In above example, a is an array of type integer which has storage size of 3 elements. One integer variable occupies 2 bytes. Hence the total size would be 3 * 2 = 6 bytes.
Memory Allocation :
5 | 10 | 15 | 2 | 4 |
Index/Subscript 0 1 2 3 4
Address 1000 1002 1004 1006 1008
Fig 1 : Memory allocation for one dimensional array
P1: Program to display all elements of 1-D array.
#include<stdio.h>
#include<conio.h>
void main()
{
int a[20];
int n,i;
printf("\n Enter the size of array :");
scanf("%d",&n);
printf("size of array is %d",n);
printf("\n Enter Values to store in array one by one by pressing ENTER :");
for(i=0;i<n;i++)
{
scanf("%d",&a[i]);
}
printf("\n The Values in array are..");
for(i=0;i<n;i++)
{
printf("\n %d",a[i]);
}
getch();
}
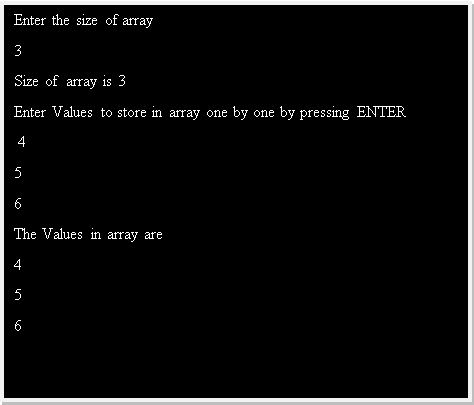
P2: Program to copy 1-D array to another 1-D array
#include <stdio.h>
#include<conio.h>
Void main()
{
Int n, i, j, a[100], b[100];// a is original array and b is copied array
Printf("Enter the number of elements in array a\n");
Scanf("%d", &n);
Printf("Enter the array elements in a\n");
For (i= 0; i < n ; i++)
Scanf("%d", &a[i]);
/*
Copying elements from array a into array b */
For (i =0;i<n;i++)
b[i] = a[i];
/*
Printing copied array b
.
*/
Printf("Elements of array b are\n");
For (i = 0; i < n; i++)
Printf("%d\n", b[i]);
Getch();
}
Output:
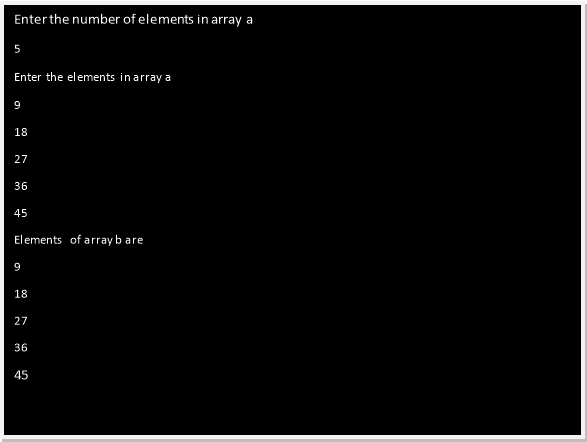
P3:Program to find reverse of 1-D array
#include <stdio.h>
#include<conio.h>
Void main()
{
Int n, i, j, a[100], b[100];// a is original array and b is reversed array
Printf("Enter the number of elements in array\n");
Scanf("%d", &n);
Printf("Enter the array elements\n");
For (i= 0; i < n ; i++)
Scanf("%d", &a[i]);
/*
* Copying elements into array b starting from end of array a
*/
For (i = n - 1, j = 0; i >= 0; i--, j++)
b[j] = a[i];
/*
Printing reversed array b
.
*/
Printf("Reverse array is\n");
For (i = 0; i < n; i++)
Printf("%d\n", a[i]);
Getch();
}
Output:
2. Multi Dimensional Array
1-D array has 1 row and multiple columns but multidimensional array has of multiple rows and multiple columns.
Two Dimensional array:
It is the simplest multidimensional array. They are also called as matrix.
Declaration:
Data-type array_name[no.of rows][no. Of columns];
Total no of elements= No.of rows * No. Of Columns
Example 3: int a[2][3]
This example illustrates two dimensional array a of 2 rows and 3 columns.
Col 0 Col 1 Col 2
Row 0
a[0][0]
| a[0][1]
| a[0][2]
|
a[1][0]
| a[1][1]
| a[1][2]
|
Row 1
Fig.2: Index positions of elements of a[2][3]
Total no of elements: 2*3=6
Total no of bytes occupied: 6( total no.of elements )*2(Size of one element)=12
Initialization and Storage Representation:
In C, two dimensional arrays can be initialized in different number of ways. Specification of no. Of columns in 2 dimensional array is mandatory while no of rows is optional.
Int a[2][3]={{1,3,0}, // Elements of 1st row
{-1,5,9}}; // Elements of 2nd row
OR
Int a[][3]={{1,3,0}, // Elements of 1st row
{-1,5,9}}; // Elements of 2nd row
OR
Int a[2][3]={1,3,0,-1,5,9};
Fig. 3 gives general storage representation of 2 dimensional array
Col 0 Col 1 Col n
Row 0
a[0][0]
| a[0][1]
|
--- | a[0][n]
|
a[1][0]
| a[1][1]
|
--- | a[1][n]
|
- | -
| - | - |
a[m][0] |
a[m][1] |
|
a[m][n] |
Row 1
-
-
Row m
Fig.3 General Storage Representation of 2 dimensional array.
Eg 4. Int a[2][3]={1,3,0,-1,5,9}
1
| 3 | 0 |
-1
| 5 | 9 |
Row 0
Row 1
Col 0 col1 col2
Accessing Two-Dimensional Array Elements:
An element in 2-dimensional array is accessed by using the subscripts i.e. row index and column index of the array. For example:
Int val = A[1][2];
The above statement will access element from the 2nd row and third column of the array A i.e. element 9.
Strings are also called as the array of character.
- A special character usually known as null character (\0) is used to indicate the end of the string.
- To read and write the string in C program %s access specifier is required.
- C language provides several built in functions for the manipulation of the string
- To use the built in functions for string, user need to include string.h header file.
- Syntax for declaring string is
Char string name[size of string];
Char is the data type, user can give any name to the string by following all the rules of defining name of variable. Size of the string is the number of alphabet user wants to store in string.
Example:
Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | Void main() | Execution of program begins |
4. | { | String is declare with name str and size of storing 10 alphbates |
5. | Char str[10]; | |
6. | Clrscr(); | Clear the output of previous screen |
7. | Printf("enter a string"); | Print “enter a string” |
8. | Scanf("%s",&str); | Entered string is stored at address of str |
9. | Printf("\n user entered string is %s",str); | Print: user entered string is (string entered by user) |
10. | Getch(); | Used to hold the output screen |
11. | } | Indicates end of scope of main function |
Following are the list of string manipulation function
Sr. No. | String Function | Purpose |
Strcat | Use to concatenate (append) one string to another | |
2. | Strcmp | Use to compare one string with another. (Note: The comparison is case sensitive) |
3. | Strchr
| Use to locate the first occurrence of a particular character in a given string |
4. | Strcpy | Use to copy one string to another. |
5. | Strlen | Use to find the length of a string in bytes, |
1.7 C++ language have 32 keywords.
Variable are declared along with data types according to which data can be stored.
Compiler in C++ allocates memory to variable based on the data type is used to declare variable.
It consists of three types of data types – Primitive data type, Derived data types and user defined data types.
Primitive data type.
- Integer
Int keyword is used for integer data types. Memory space required by integer is 4 bytes and range is from -2147483648 to 2147483647.
- Character
Char keyword is used for character data types. It is used to store character data. . Memory space required by integer is 1 byte and range is from -128 to 127 or 0 to 255.
- Boolean
Bool keyword is used for character data types. It is used to store Boolean values. It stores only true or false.
- Floating point
Float keyword is used for character data types. It is used to store decimal values. Memory space required by integer is 4 byte.
- Double Floating Point
Double keyword is used for character data types. It is used to store double precision decimal values. Memory space required by integer is 8 byte.
Derived Data types
Derived data types are the user defined data types , defined by the user.
It consists of struct, enumerations and classes.
Structure in which different data type variables can be defined.
Class is the basis of object oriented programming.
Enumeration is the set of integer constants that are assigned to variables.
Void Data type
Void data type is used for value les entity. It is used for function which do not return any value.
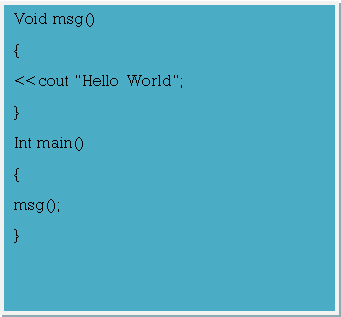
Constant are same as variables but once initialized, cannot change by program.
Constants are fixed values and also called as literals.
Example –
- Integer constants – 2,7,0,454,1204.
- Real constants – 6.7, 4556.78,3432.988
- Character constants – ‘a’,’G’.
- String Constants – “Hello World”.
Every expression in C language contains set of operators and operands. Operators are the special symbols which are used to indicate the type of operation whereas operands are the data member or variables operating as per operation specified by operator. One expression contains one or more set of operators and operands. So to avoid the ambiguity in execution of expression, C compiler fixed the precedence of operator. Depending on the operation, operators are classified into following category.
- Arithmetic Operator: These are the operators which are useful in performing mathematical calculations. Following are the list of arithmetic operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
+ | Used to add two operands | Result of A+B is 15 | |
2. | - | Used to subtract two operands | Result of A-B is 5 |
3. | * | Used to multiply two operands | Result of A*B is 50 |
4. | / | Used to divide two operands | Result of A/B is 2 |
5. | % | Find reminder of the division of two operand | Result of A%B is 0 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of addition of %d and %d is %d",a,b,a+b);
Printf("\n Result of subtraction of %d and %d is %d",a,b,a-b);
Printf("\n Result of multiplication %d and %d is %d",a,b,a*b);
Printf("\n Result of division of %d and %d is %d",a,b,a/b);
Printf("\n Result of modulus of %d and %d is %d",a,b,a%b);
Getch();
}
Output:
Enter two numbers
5
3
Result of addition of 5 and 3 is 8
Result of subtraction of 5 and 3 is 2
Result of multiplication of 5 and 3 is 15
Result of division of 5 and 3 is 1
Result of modulus of 5 and 3 is 2
2. Relational Operators: Relational operator used to compare two operands. Relational operator produce result in terms of binary values. It returns 1 when the result is true and 0 when result is false. Following are the list of relational operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | < | This is less than operator which is used to check whether the value of left operand is less than the value of right operand or not | Result of A<B is false
|
2. | > | This is greater than operator which is used to check whether the value of left operand is greater than the value of right operand or not | Result of A>B is true |
3. | <= | This is less than or equal to operator | Result of A<=B is false |
4. | >= | This is greater than or equal to operator | Result of A>=B is true |
5. | == | This is equal to operator which is used to check value of both operands are equal or not | Result of A==B is false |
6. | != | This is not equal to operator which is used to check value of both operands are equal or not | Result of A!=B is true |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of less than operator of %d and %d is %d",a,b,a<b);
Printf("\n Result of greater than operator of %d and %d is %d",a,b,a>b);
Printf("\n Result of leass than or equal to operator %d and %d is %d",a,b,a<=b);
Printf("\n Result of greater than or equal to operator of %d and %d is %d", a,b,a>=b);
Printf("\n Result of double equal to operator of %d and %d is %d",a,b,a==b);
Printf("\n Result of not equal to operator of %d and %d is %d",a,b,a!=b);
Getch();
}
Output:
Enter two numbers
5
3
Result of less than operator of 5 and 3 is 0
Result of greater than operator of 5 and 3 is 1
Result of less than or equal to operator of 5 and 3 is 0
Result of greater than or equal to operator of 5 and 3 is 1
Result of double equal to operator of 5 and 3 is 0
Result of not equal to operator of 5 and 3 is 1
3. Logical Operators: Logical operators are used to compare logical values of two operands. Following are the list of logical operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | && | This is logical AND, it returns true when both the values are non zero | Result of (A&&B) is true |
2. | || | This is logical OR, it returns true when any of two value is non zero | Result of (A||B) is true |
3. | ! | This is logical NOT operator, it is used to reverse the logical state of operand | Result of !(A&&B) is false |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of logical and operator of %d and %d is %d",a,b,a&&b);
Printf("\n Result of logical or operator of %d and %d is %d",a,b,a||b);
Printf("\n Result of logical not operator of %d and and %d is %d",a,b,!(a&&b));
Getch();
}
Output:
Enter two numbers
5
3
Result of logical and operator of 5 and 3 is 1
Result of logical or operator of 5 and 3 is 1
Result of logical not operator of 5 and 3 is 0
4. Assignment Operators: Assignment operator is used to assign the value to variable. To understand the operation assume variable A contains value 10 and variable contains value 5.
Example: A=B;
In the above example value of variable B is assign to variable A. Result of this expression is value of A is 5 and value of B is 5.
5. Increments and Decrement Operators: These operator are used to increase or decrease the value of variable by one. Following are the list of logical operator in C language. To understand the operation assume variable A contains value 10.
Sr. No. | Operator | Description | Example |
1. | ++ | This is incremental operator which is used to increase the value of variable by 1 | Result of (A++) is value of A is 11 |
2. | -- | This is decremental operator which is used to decrease the value of variable by 1 | Result of (A--) is value of A is 9 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a=5,b=5,c=5,d=5;
Clrscr();
Printf("Original value of a, b, c, d is %d \t %d \t %d \t %d",a,b,c,d);
a++;
Printf("\n New value of a is %d",a);
++b;
Printf("\n New value of b is %d",b);
Printf("\n New value of c is %d",c++);
Printf("\n New value of d is %d",++d);
Getch();
}
Output:
Original value of a, b, c, d is 5 5 5 5
New value of a is 6
New value of b is 6
New value of c is 5
New value of d is 6
6. Bitwise Operators: These operators are used to work on the each bits of data. These operators can work on the binary data. If the data is non binary then computer first of all convert it into binary form and then perform the operation. Following are the list of logical operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5. So the binary values of
A = 0000 1010 and
B = 0000 0101
Sr. No. | Operator | Description | Example |
1. | & | This is bitwise AND | Result of (A&B) is 0000 0000 |
2. | | | This is bitwise OR | Result of (A|B) is 0000 1111 |
3. | ^ | This is bitwise Exclusive | Result of (A^B) is 0000 1111 |
4. | << | This is used to shift bit on left side | Result of (A<<B) is |
5. | >> | This is used to shift bit on right side | Result of (A>>B) is |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of bitwise and operator of %d and %d is %d",a,b,a&b);
Printf("\n Result of bitwise or operator of %d and %d is %d",a,b,a|b);
Printf("\n Result of bitwise exclusive operator of %d and %d is %d",a,b,a^b);
Printf("\n Result of bitwise left shift operator of %d and %d is %d",a,b,a<<b);
Printf("\n Result of bitwise right shift operator of %d and %d is %d",a,b,a>>b);
Getch();
}
Output:
Enter two numbers
5
3
Result of bitwise and operator of 5 and 3 is 1
Result of bitwise or operator of 5 and 3 is 7
Result of bitwise exclusive operator of 5 and 3 is 6
Result of bitwise left shift operator of 5 and 3 is 40
Result of bitwise right shift operator of 5 and 3 is 0
It is a symbol performs an action applied to variables ST.
Operands are the symbol on which operation is performed.
Operators are classified as unary and binary operators.
Binary operators are called as Arithmetic, Binary, Logical, Assignment, and Conditional and relational.
Expression may have one or more operators in it. If expression has multiple operators then these operators are solved according to hierarchy of operators. Hierarchy determines the order in which these operators are executed.
Table : Hierarchy of operators
Functions | Top Priority |
Power | |
Mod |
|
*,/ |
|
+,- |
|
=,<,>,<=,>= |
|
NOT |
|
AND |
|
OR | Least Priority |
Ex. 1
Find the result of the following operations.
Sr. No. | Operation |
1. | 10/2 |
2. | 15 MOD 2 |
3. | NOT TRUE |
4. | 40 < =35 |
5. | FALSE AND TRUE |
6. | 60 – 15 |
7. | 55>10 |
8. | TRUE OR FALSE |
9. | – 10> 5 |
10. | 10* 0.3 |
Soln. :
Sr. No. | Operation | Solution |
1. | 10/2 | 5 |
2. | 15 MOD 2 | 1 |
3. | NOT TRUE | FALSE |
4. | 40 < =35 | FALSE |
5. | FALSE AND TRUE | FALSE |
6. | 60 – 15 | 45 |
7. | 55>10 | TRUE |
8. | TRUE OR FALSE | TRUE |
9. | – 10> 5 | FALSE |
10. | 10* 0.3 | 3 |
Ex. 2
Using Hierarchy chart, list the order in which following operations would be processed?
1. +,-,* 2. OR,*,<
3. NOT, AND,* 4. AND,OR,NOT
5. NOT,+,\ 6. MOD,\,<
7. <,AND,>,+
Soln. :
Sr. No. | Given Expression | Solution |
1 | +,-,* | *, +, - |
2 | OR,*,< | *, <, OR |
3 | NOT, AND,* | *, NOT, AND |
4 | AND,OR,NOT | NOT, AND, OR |
5 | NOT,+,\ | \, +, NOT |
6 | MOD,\,< | MOD, \, < |
7 | <,AND,>,+ | +, <,>,AND |
Control structure is the important concept in high level programming languages. Control structures are the instruction or the group of instructions in the programming language which are responsible to determine the sequence of other instructions in the program. Control structure is used for the reusability of the code. Control structure is useful when the program demands to repeatedly execute block of code for specific number of times or till certain condition is satisfied. Control structures can control the flow of execution of program based on some conditions. Following are the advantages of using control structures in C language.
- Increases the reusability of the code
- Reduces complexity, improves clarity, and facilitates debugging and modifying
- Easy to define in flowcharts
- Increases programmer productivity.
- Control statements are also useful in the indentation of the program.
There are two types of control statements
Conditional Branching: In conditional branching decision point is based on the run time logic. Conditional branching makes use of both selection logic and iteration logic. In conditional branching flow of program is transfer when the specified condition is satisfied.
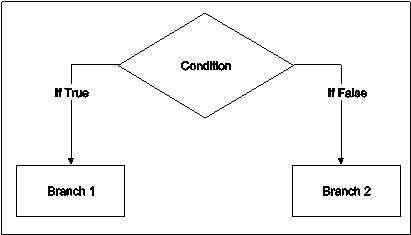
Fig. Flowchart for conditional branching
Unconditional Branching: In Unconditional branching flow of program is transfer to a particular location without concerning the condition. Unconditional branching makes use of sequential logic. In unconditional branching flow of program is transfer as per the instruction.
Conditional Branching
In conditional branching change in sequence of statement execution is depends upon the specified condition. Conditional statements allow programmer to check a condition and execute certain parts of code depending on the result of conditional expression. Conditional expression must be resulted into Boolean value. In C language, there are two forms of conditional statements:
1. If-----else statement: It is used to select one option between two alternatives
2. Switch statement: It is used to select one option between multiple alternative
- If Statements
This statement permits the programmer to allocate condition on the execution of a statement. If the evaluated condition found to be true, the single statement following the "if" is execute. If the condition is found to be false, the following statement is skipped. Syntax of the if statement is as follows
if (condition)
statement1;
statement2;
In the above syntax, “if” is the keyword and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and then statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
if-----else statement
This statement permits the programmer to execute a statement out of the two statements. If the evaluated condition is found to be true, the single statement following the "if" is executed and statement following else is skipped. If the condition is found to be false, statement following the "if" is skipped and statement following else is executed.
In this statement “if” part is compulsory whereas “else” is the optional part. For every “if” statement there may be or may not be “else” statement but for every “else” statement there must be “if” part otherwise compiler will gives “Misplaced else” error.
Syntax of the “if----else” statement is as follows
If (condition)
Statement1;
Else
Statement2;
In the above syntax, “if” and “else” are the keywords and condition in parentheses must evaluate to true or false. If the condition is satisfied (true) then compiler will execute statement1 and skip statement2. If the condition is not satisfied (false) then compiler will skip statement1 and directly execute statement2.
Nested if-----else statements :
Nested “if-----else” statements are used when programmer wants to check multiple conditions. Nested “if---else” contains several “if---else” a statement out of which only one statement is executed. Number of “if----else” statements is equal to the number of conditions to be checked. Following is the syntax for nested “if---else” statements for three conditions
If (condition1)
Statement1;
Else if (condition2)
Statement2;
Else if (condition3)
Statement3;
Else
Statement4;
In the above syntax, compiler first check condition1, if it trues then it will execute statement1 and skip all the remaining statements. If condition1 is false then compiler directly checks condition2, if it is true then compiler execute statement2 and skip all the remaining statements. If condition2 is also false then compiler directly checks condition3, if it is true then compiler execute statement3 otherwise it will execute statement 4.
Note:
If the test expression is evaluated to true,
• Statements inside the body of if are executed.
• Statements inside the body of else are skipped from execution.
If the test expression is evaluated to false,
• Statements inside the body of else are executed
• Statements inside the body of if are skipped from execution.
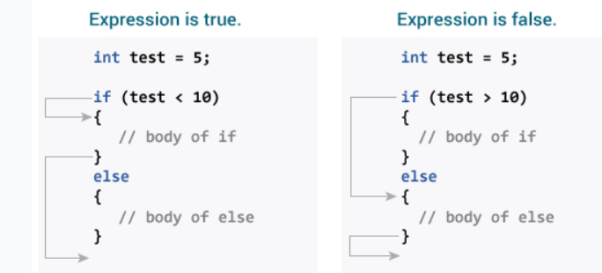
// Check whether an integer is odd or even
#include <stdio.h>
Int main() {
Int number;
Printf("Enter an integer: ");
Scanf("%d", &number);
// True if the remainder is 0
If (number%2 == 0) {
Printf("%d is an even integer.",number);
}
Else {
Printf("%d is an odd integer.",number);
}
Return 0;
}
Program to relate two integers using =, > or < symbol
#include <stdio.h>
Int main() {
Int number1, number2;
Printf("Enter two integers: ");
Scanf("%d %d", &number1, &number2);
//checks if the two integers are equal.
If(number1 == number2) {
Printf("Result: %d = %d",number1,number2);
}
//checks if number1 is greater than number2.
Else if (number1 > number2) {
Printf("Result: %d > %d", number1, number2);
}
//checks if both test expressions are false
Else {
Printf("Result: %d < %d",number1, number2);
}
Return 0;
}
- Switch Statements
This statement permits the programmer to choose one option out of several options depending on one condition. When the “switch” statement is executed, the expression in the switch statement is evaluated and the control is transferred directly to the group of statements whose “case” label value matches with the value of the expression. Syntax for switch statement is as follows:
Switch(expression)
{
Case constant1:
Statements 1;
Break;
Case constant2:
Statements 2;
Break;
…………………..
Default:
Statements n;
Break;
}
In the above, “switch”, “case”, “break” and “default” are keywords. Out of which “switch” and “case” are the compulsory keywords whereas “break” and “default” is optional keywords.
- “switch” keyword is used to start switch statement with conditional expression.
- “case” is the compulsory keyword which labeled with a constant value. If the value of expression matches with the case value then statement followed by “case” statement is executed.
- “break” is the optional keyword in switch statement. The execution of “break” statement causes the transfer of flow of execution outside the “switch” statements scope. Absence of “break” statement causes the execution of all the following “case” statements without concerning value of the expression.
- “default” is the optional keyword in “switch” statement. When the value of expression is not match with the any of the “case” statement then the statement following “default” keyword is executed.
Example: Program to spell user entered single digit number in English is as follows
#include<stdio.h>
#include<conio.h>
Void main()
{
Int n;
Clrscr();
Printf("Enter a number");
Scanf("%d",&n);
Switch(n)
{
Case 0: printf("\n Zero");
Break;
Case 1: printf("\n One");
Break;
Case 2: printf("\n Two");
Break;
Case 3: printf("\n Three");
Break;
Case 4: printf("\n Four");
Break;
Case 5: printf("\n Five");
Break;
Case 6: printf("\n Six");
Break;
Case 7: printf("\n Seven");
Break;
Case 8: printf("\n Eight");
Break;
Case 9: printf("\n Nine");
Break;
Default: printf("Given number is not single digit number");
}
Getch();
}
Output
Enter a number
5
Five
Unconditional Branching
In unconditional branching statements are executed without concerning the condition. Unconditional statements allow programmer to transfer the flow of execution to any part of the program. In C language, there are four types of unconditional statements:
- Break statement: It is the unconditional jump instruction. It is used mainly with “switch” and loop statements. The execution of “break” instruction, transfer the flow of program outside the loop or “switch” statement Syntax of “break” statement is as follows
break;
When “break” statement used inside loops then execution of “break” statement causes the immediate termination of loop and compiler will execute next statement after the loop statement.
2. Goto statement: “goto” statement is used to transfer the flow of program to any part of the program. The syntax of “go to” statement is as follows
goto label name;
In the above syntax “goto” is keyword which is used to transfer the flow of execution to the label specified after it. Label is an identifier that is used to mark the target statement to which the control is transferred. The target statement must be labeled and the syntax is as follows
Label name statement;
In the above syntax label name can be anything but it should be same as that of name of the label specified in “goto” statement, a colon must follow the label. Each labeled statement within the function must have a unique label, i.e., no two statements can have the same label.
3. Continue statement: It is used mainly with “switch” and “loop” statements. The execution of “continue” instruction, skip the remaining iteration of the loop and transfer he flow of program to the loop control instruction. Syntax of “continue” statement is as follows
Continue;
4. Return statement: The “return” statement terminates the execution of the current function and return control to the calling function. The “return” statement immediately ends the function, ignoring everything else in the function after it is called. It immediately returns to the calling function and continue from the point that it is called. Return also able to "return" a value which can be use in the calling function.
. Decision Making Statements.
Decision making statements consists of two or more conditions specified to execute statement so that any condition becomes true or false another condition executes.
The if-else statement.
The if else statement consists of if followed by else. Condition specified in if becomes true then statement in if block executes. When condition mentioned in if becomes false then statements in else execute.
Structure of the if – else.
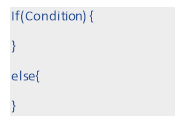
e.g.
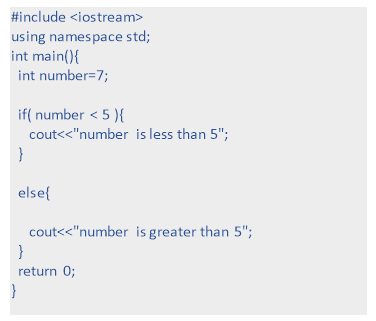
Nested if – else
The Nested if- else consist of multiple if-else statements in another if – else. In this specified conditions are executed from top. While executing nested if else, when condition becomes true statement related with it gets execute and rest conditions are bypassed. When all the conditions become false last else statement gets execute.
Structure of the nested if – else:
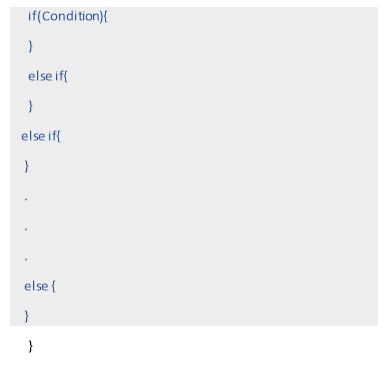
e.g.
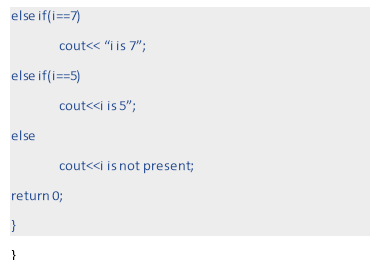
Here output is 5. When i=5 condition will match it will give is 5 output if not then last output will be i is not present.
The goto
The goto is an unconditional jump type of statement. The goto is used to jump between program from one point to another. The goto can be used to jump out of the nested loop.
Structure of the goto:
While executing program goto provides the information to goto instruction defined as a label. This label is initialized with goto statement before.
e.g.
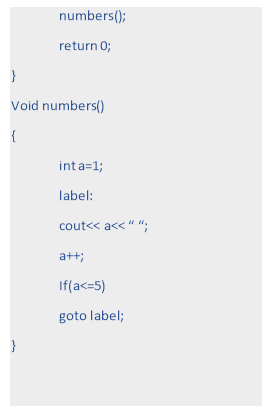
When the compiler will come to goto label then control will goto label defined before the loop and output will be 1 2 3 4 5.
The break
The break statement is used to terminate the execution where number of iterations is not unknown. It is used in loop to terminate the loop and then control goes to the first statement of the loop. The break statement is also in switch case.
Structure of the break:
e.g.
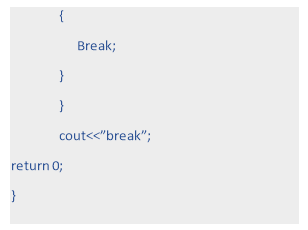
When the compiler will reach to break it will come out and print the output. Output will be x=1,x=9,x=8,x=7,x=6,break.
Similar to other languages C language also provides the facility of function. Function is the block of code which is used to perform a specific task. In c language the complete program is composed of function.
Functions are useful to divide c programs into smaller modules. Programmer can invoked these modules anywhere inside c program for any number of times.
Functions are used to increase readability of the code. Size of program can be reduce by using functions. By using function, programmer can divide complex tasks into smaller manageable tasks and test them independently before using them together.
Functions of C language are defined with the type of function. The type of functions indicates the data type of value which will return by function. In order to use function in the program, initially programmer have to inform compiler about the function. This is also called as defining a function.
In C programme all the function definition present outside the main function. All function need to be declared and defined before use. Function declaration requires function name, argument list, and return type.
Return Type Function name (Argument list)
{
Statement 1;
Statement 2;
…………...
Statement n;
}
Parameter Passing by Value
This is the default way of passing the parameters to the function. This is achieved by passing the copy of data to the function. This mechanism is also called as call by value. In case of parameter passing by value, the changes made to the formal arguments in the called function have no effect on the values of actual arguments in the calling function.
This mechanism is used when programmer don't want to change the value of passed parameters. When parameters are passed by value then functions in C create copies of the passed in variables and do required processing on these copied variables.
Pass-by-value is implemented by actual data transfer so additional storage is required to maintain the copies of passed parameters.
Example:
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
Void swap( int p1, int p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( a, b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
Getch();
}
Void swap( int p1, int p2 )
{
Int t;
t = p2;
p2 = p1;
p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output :
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b (p2) = 10
After: Value of a = 10 and value of b = 20
Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.
Parameter Passing by Reference
This mechanism is used when programmer want a function to do the changes in passed parameters and reflect those changes back to the calling function. This mechanism is also called as call by reference. This is achieved by passing the address of variable to the function and function body can directly work over the addresses. Advantage of pass by reference is efficiency in both time and space. Whereas disadvantages are access to formal parameters is slow and inadvertent and erroneous changes may be made to the actual parameter.
Example:
#include <stdio.h>
#include<conio.h>
Void swap( int *p1, int *p2 );
Int main()
{
Int a = 10;
Int b = 20;
Printf("Before: Value of a = %d and value of b = %d\n", a, b );
Swap( &a, &b );
Printf("After: Value of a = %d and value of b = %d\n", a, b );
}
Void swap( int *p1, int *p2 )
{
Int t;
t = *p2;
*p2 = *p1;
*p1 = t;
Printf("Value of a (p1) = %d and value of b(p2) = %d\n", *p1, *p2 );
}
Output :
Before: Value of a = 10 and value of b = 20
Value of a (p1) = 20 and value of b(p2) = 10
After: Value of a = 20 and value of b = 10
Note: In the above example the values of “a” and “b” are changes after calling swap function.
In programming languages, if a program allows you to call a function inside the same function, then it is called a recursive call of the function.
Void recursion() {
Recursion(); /* function calls itself */
}
Int main() {
Recursion();
}
The C programming language supports recursion, i.e., a function to call itself. But while using recursion, programmers has to be careful to define an exit condition from the function, otherwise it will go into an infinite loop.
Recursive functions are useful to solve many mathematical problems, such as calculating the factorial of a number, generating Fibonacci series, etc.
The following example calculates the factorial of a given number using a recursive function −
#include <stdio.h>
Unsigned long long int factorial(unsigned int i) {
If(i <= 1) {
Return 1;
}
Return i * factorial(i - 1);
}
Int main() {
Int i = 12;
Printf("Factorial of %d is %d\n", i, factorial(i));
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Factorial of 12 is 479001600
The following example generates the Fibonacci series for a given number using a recursive function −
#include <stdio.h>
Int fibonacci(int i) {
If(i == 0) {
Return 0;
}
If(i == 1) {
Return 1;
}
Return fibonacci(i-1) + fibonacci(i-2);
}
Int main() {
Int i;
For (i = 0; i < 10; i++) {
Printf("%d\t\n", fibonacci(i));
}
Return 0;
}
When the above code is compiled and executed, it produces the following result −
0
1
1
2
3
5
8
13
21
34
A storage class is used to describe the following things:
- The variable scope.
- The location where the variable will be stored.
- The initialized value of a variable.
- A lifetime of a variable.
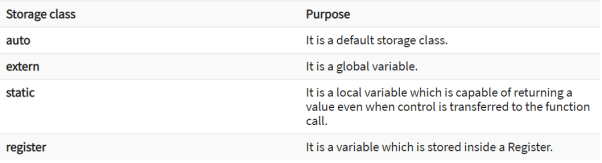