UNIT 1
Computer Basics
1. Explain implicit type casting in detail
Implicit type casting
Implicit type casting means conversion of data types without losing its original meaning. This type of typecasting is essential when you want to change data types without changing the significance of the values stored inside the variable.
Implicit type conversion happens automatically when a value is copied to its compatible data type. During conversion, strict rules for type conversion are applied. If the operands are of two different data types, then an operand having lower data type is automatically converted into a higher data type. This type of type conversion can be seen in the following example.
#include<stdio.h>
Int main(){
Short a=10; //initializing variable of short data type
Int b; //declaring int variable
b=a; //implicit type casting
Printf("%d\n",a);
Printf("%d\n",b);
}
Output
10
10
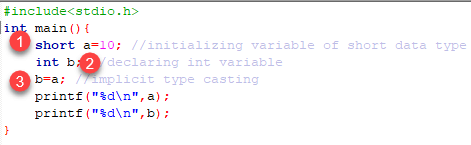
- In the given example, we have declared a variable of short data type with value initialized as 10.
- On the second line, we have declared a variable of an int data type.
- On the third line, we have assigned the value of variable s to the variable a. On third line implicit type conversion is performed as the value from variable s which is of short data type is copied into the variable a which is of an int data type.
Converting Character to Int
Consider the example of adding a character decoded in ASCII with an integer:
#include <stdio.h>
Main() {
Int number = 1;
Char character = 'k'; /*ASCII value is 107 */
Int sum;
Sum = number + character;
Printf("Value of sum : %d\n", sum );
}
Output:
Value of sum : 108
Here, compiler has done an integer promotion by converting the value of 'k' to ASCII before performing the actual addition operation.
Arithmetic Conversion Hierarchy
The compiler first proceeds with promoting a character to an integer. If the operands still have different data types, then they are converted to the highest data type that appears in the following hierarchy chart:
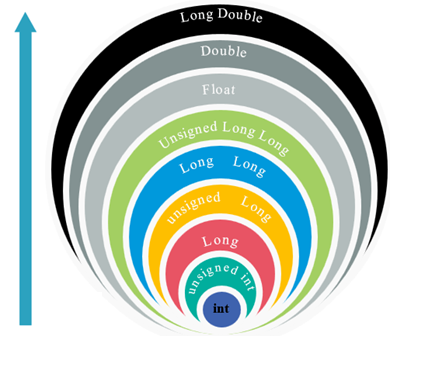
Consider the following example to understand the concept:
#include <stdio.h>
Main() {
Int num = 13;
Char c = 'k'; /* ASCII value is 107 */
Float sum;
Sum = num + c;
Printf("sum = %f\n", sum );}
Output:
Sum = 120.000000
First of all, the c variable gets converted to integer, but the compiler converts num and c into "float" and adds them to produce a 'float' result.
Important Points about Implicit Conversions
- Implicit type of type conversion is also called as standard type conversion. We do not require any keyword or special statements in implicit type casting.
- Converting from smaller data type into larger data type is also called as type promotion. In the above example, we can also say that the value of s is promoted to type integer.
- The implicit type conversion always happens with the compatible data types.
We cannot perform implicit type casting on the data types which are not compatible with each other such as:
- Converting float to an int will truncate the fraction part hence losing the meaning of the value.
- Converting double to float will round up the digits.
- Converting long int to int will cause dropping of excess high order bits.
In all the above cases, when we convert the data types, the value will lose its meaning. Generally, the loss of meaning of the value is warned by the compiler.
'C' programming provides another way of typecasting which is explicit type casting.
2. Explain explicit type casting in detail
Explicit type casting
In implicit type conversion, the data type is converted automatically. There are some scenarios in which we may have to force type conversion. Suppose we have a variable div that stores the division of two operands which are declared as an int data type.
Int result, var1=10, var2=3;
Result=var1/var2;
In this case, after the division performed on variables var1 and var2 the result stored in the variable "result" will be in an integer format. Whenever this happens, the value stored in the variable "result" loses its meaning because it does not consider the fraction part which is normally obtained in the division of two numbers.
To force the type conversion in such situations, we use explicit type casting.
It requires a type casting operator. The general syntax for type casting operations is as follows:
(type-name) expression
Here,
- The type name is the standard 'C' language data type.
- An expression can be a constant, a variable or an actual expression.
Let us write a program to demonstrate implementation of explicit type-casting in 'C'.
#include<stdio.h>
Int main()
{
Float a = 1.2;
//int b = a; //Compiler will throw an error for this
Int b = (int)a + 1;
Printf("Value of a is %f\n", a);
Printf("Value of b is %d\n",b);
Return 0;
}
Output:
Value of a is 1.200000
Value of b is 2
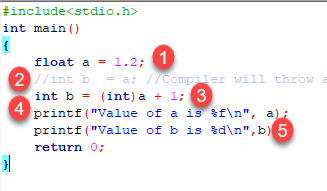
- We have initialized a variable 'a' of type float.
- Next, we have another variable 'b' of integer data type. Since the variable 'a' and 'b' are of different data types, 'C' won't allow the use of such expression and it will raise an error. In some versions of 'C,' the expression will be evaluated but the result will not be desired.
- To avoid such situations, we have typecast the variable 'a' of type float. By using explicit type casting methods, we have successfully converted float into data type integer.
- We have printed value of 'a' which is still a float
- After typecasting, the result will always be an integer 'b.'
In this way, we can implement explicit type casting in 'C' programming.
3. Write an example of arithmetic operators
Arithmetic Operators
// Working of arithmetic operators
Int main()
{
Int a = 9,b = 4, c;
c = a+b;
Printf("a+b = %d \n",c);
c = a-b;
Printf("a-b = %d \n",c);
c = a*b;
Printf("a*b = %d \n",c);
c = a/b;
Printf("a/b = %d \n",c);
c = a%b;
Printf("Remainder when a divided by b = %d \n",c);
Return 0;
}
Output
a+b = 13
a-b = 5
a*b = 36
a/b = 2
Remainder when a divided by b=1
The operators +, - and * computes addition, subtraction, and multiplication respectively as you might have expected.
In normal calculation, 9/4 = 2.25. However, the output is 2 in the program.
It is because both the variables a and b are integers. Hence, the output is also an integer. The compiler neglects the term after the decimal point and shows answer 2 instead of 2.25.
The modulo operator % computes the remainder. When a=9 is divided by b=4, the remainder is 1. The % operator can only be used with integers.
Suppose a = 5.0, b = 2.0, c = 5 and d = 2. Then in C programming,
// Either one of the operands is a floating-point number
a/b = 2.5
a/d = 2.5
c/b = 2.5
// Both operands are integers
c/d = 2
4. Write an example of relational operators
Relational Operators
// Working of relational operators
Int main()
{
Int a = 5, b = 5, c = 10;
Printf("%d == %d is %d \n", a, b, a == b);
Printf("%d == %d is %d \n", a, c, a == c);
Printf("%d > %d is %d \n", a, b, a > b);
Printf("%d > %d is %d \n", a, c, a > c);
Printf("%d < %d is %d \n", a, b, a < b);
Printf("%d < %d is %d \n", a, c, a < c);
Printf("%d != %d is %d \n", a, b, a != b);
Printf("%d != %d is %d \n", a, c, a != c);
Printf("%d >= %d is %d \n", a, b, a >= b);
Printf("%d >= %d is %d \n", a, c, a >= c);
Printf("%d <= %d is %d \n", a, b, a <= b);
Printf("%d <= %d is %d \n", a, c, a <= c);
Return 0;
}
Output
5 == 5 is 1
5 == 10 is 0
5 > 5 is 0
5 > 10 is 0
5 < 5 is 0
5 < 10 is 1
5 != 5 is 0
5 != 10 is 1
5 >= 5 is 1
5 >= 10 is 0
5 <= 5 is 1
5 <= 10 is 1
5. Explain Operator Precedence and Associativity
Operator precedence determines the grouping of terms in an expression and decides how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has a higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has a higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | << >> | Left to right |
Relational | < <= > >= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
Example Code
#include <stdio.h>
Main() {
Int a = 20;
Int b = 10;
Int c = 15;
Int d = 5;
Int e;
e = (a + b) * c / d; // ( 30 * 15 ) / 5
Printf("Value of (a + b) * c / d is : %d\n", e );
e = ((a + b) * c) / d; // (30 * 15 ) / 5
Printf("Value of ((a + b) * c) / d is : %d\n" , e );
e = (a + b) * (c / d); // (30) * (15/5)
Printf("Value of (a + b) * (c / d) is : %d\n", e );
e = a + (b * c) / d; // 20 + (150/5)
Printf("Value of a + (b * c) / d is : %d\n" , e );
Return 0;
}
Output
Value of (a + b) * c / d is : 90
Value of ((a + b) * c) / d is : 90
Value of (a + b) * (c / d) is : 90
Value of a + (b * c) / d is : 50
6. What is Structure of C program?
Before we study the basic building blocks of the C programming language, let us look at a bare minimum C program structure so that we can take it as a reference in the upcoming chapters.
Hello World Example
A C program basically consists of the following parts −
- Preprocessor Commands
- Functions
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" −
#include <stdio.h>
Int main() {
/* my first program in C */
Printf("Hello, World! \n");
Return 0;
}
Let us take a look at the various parts of the above program −
- The first line of the program #include <stdio.h> is a preprocessor command, which tells a C compiler to include stdio.h file before going to actual compilation.
- The next line int main() is the main function where the program execution begins.
- The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. So such lines are called comments in the program.
- The next line printf(...) is another function available in C which causes the message "Hello, World!" to be displayed on the screen.
- The next line return 0; terminates the main() function and returns the value 0.
Compile and Execute C Program
Let us see how to save the source code in a file, and how to compile and run it. Following are the simple steps −
- Open a text editor and add the above-mentioned code.
- Save the file as hello.c
- Open a command prompt and go to the directory where you have saved the file.
- Type gcc hello.c and press enter to compile your code.
- If there are no errors in your code, the command prompt will take you to the next line and would generate a.out executable file.
- Now, type a.out to execute your program.
- You will see the output "Hello World" printed on the screen.
$ gcc hello.c
$ ./a.out
Hello, World!
Make sure the gcc compiler is in your path and that you are running it in the directory containing the source file hello.c.
7. Define Character set, Data types, Constants
Character Set
The character set are set of words, digits, symbols and operators that are valid in C.
There are four types of Character Set:-
Character Set | ||
1. | Letters | Uppercase A-Z |
2. | Digits | All digits 0-9 |
3. | Special Characters | All Symbols: , . : ; ? ' " ! | \ / ~ _$ % # & ^ * - + < > ( ) { } [ ] |
4. | White Spaces | Blank space, Horizintal tab, Carriage return, New line, Form feed |
Tokens
Tokens are the smallest unit of the program meaningful to the compiler.
Tokens are classified as – Keywords, Identifiers, Contestants, Strings, Operators.
Data Types
A data type specifies the type of data that a variable can store such as integer, floating, character, etc.
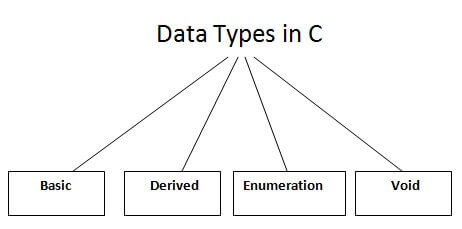
There are the following data types in C language.
Types | Data Types |
Basic Data Type | Int, char, float, double |
Derived Data Type | Array, pointer, structure, union |
Enumeration Data Type | Enum |
Void Data Type | Void |
Basic Data Types
The basic data types are integer-based and floating-point based. C language supports both signed and unsigned literals.
The memory size of the basic data types may change according to 32 or 64-bit operating system.
Let's see the basic data types. Its size is given according to 32-bit architecture.
Data Types | Memory Size | Range |
Char | 1 byte | −128 to 127 |
Signed char | 1 byte | −128 to 127 |
Unsigned char | 1 byte | 0 to 255 |
Short | 2 byte | −32,768 to 32,767 |
Signed short | 2 byte | −32,768 to 32,767 |
Unsigned short | 2 byte | 0 to 65,535 |
Int | 2 byte | −32,768 to 32,767 |
Signed int | 2 byte | −32,768 to 32,767 |
Unsigned int | 2 byte | 0 to 65,535 |
Short int | 2 byte | −32,768 to 32,767 |
Signed short int | 2 byte | −32,768 to 32,767 |
Unsigned short int | 2 byte | 0 to 65,535 |
Long int | 4 byte | -2,147,483,648 to 2,147,483,647 |
Signed long int | 4 byte | -2,147,483,648 to 2,147,483,647 |
Unsigned long int | 4 byte | 0 to 4,294,967,295 |
Float | 4 byte |
|
Double | 8 byte |
|
Long double | 10 byte |
|
Constants
A constant is a value or variable that can't be changed in the program, for example: 10, 20, 'a', 3.4, "c programming" etc.
There are different types of constants in C programming.
List of Constants in C
Constant | Example |
Decimal Constant | 10, 20, 450 etc. |
Real or Floating-point Constant | 10.3, 20.2, 450.6 etc. |
Octal Constant | 021, 033, 046 etc. |
Hexadecimal Constant | 0x2a, 0x7b, 0xaa etc. |
Character Constant | 'a', 'b', 'x' etc. |
String Constant | "c", "c program", "c in javatpoint" etc. |
2 ways to define constant in C
There are two ways to define constant
Const keyword
- #define preprocessor
1) C const keyword
The const keyword is used to define constant in C programming.
- Const float PI=3.14;
Now, the value of PI variable can't be changed.
- #include<stdio.h>
- Int main(){
- Const float PI=3.14;
- Printf("The value of PI is: %f",PI);
- Return 0;
- }
Output:
The value of PI is: 3.140000
If you try to change the the value of PI, it will render compile time error.
- #include<stdio.h>
- Int main(){
- Const float PI=3.14;
- PI=4.5;
- Printf("The value of PI is: %f",PI);
- Return 0;
- }
Output:
Compile Time Error: Cannot modify a const object
2) C #define preprocessor
The #define preprocessor is also used to define constant.
8. What is flowchart and explain flow chart symbols?
Flowchart is a diagrammatic representation of sequence of logical steps of a program. Flowcharts use simple geometric shapes to depict processes and arrows to show relationships and process/data flow.
Flowchart Symbols
Here is a chart for some of the common symbols used in drawing flowcharts.
Symbol | Symbol Name | Purpose |
![]() | Start/Stop | Used at the beginning and end of the algorithm to show start and end of the program. |
![]() | Process | Indicates processes like mathematical operations. |
![]() | Input/ Output | Used for denoting program inputs and outputs. |
![]() | Decision | Stands for decision statements in a program, where answer is usually Yes or No. |
![]() | Arrow | Shows relationships between different shapes. |
![]() | On-page Connector | Connects two or more parts of a flowchart, which are on the same page. |
![]() | Off-page Connector | Connects two parts of a flowchart which are spread over different pages. |
Guidelines for Developing Flowcharts
These are some points to keep in mind while developing a flowchart −
- Flowchart can have only one start and one stop symbol
- On-page connectors are referenced using numbers
- Off-page connectors are referenced using alphabets
- General flow of processes is top to bottom or left to right
- Arrows should not cross each other
Example Flowcharts
Here is the flowchart for going to the market to purchase a pen.
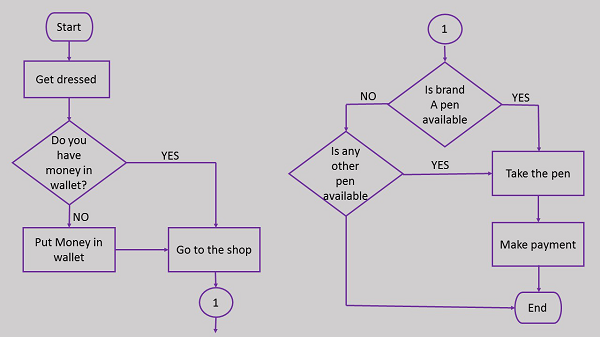
Here is a flowchart to calculate the average of two numbers.
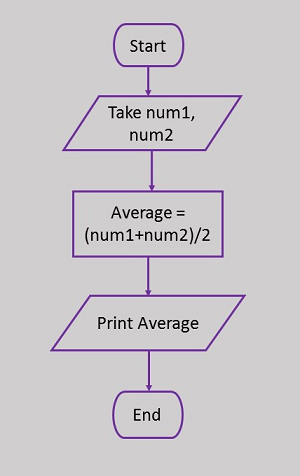
9. What is an Algorithm?
An algorithm is a process or a set of rules required to perform calculations or some other problem-solving operations especially by a computer. The formal definition of an algorithm is that it contains the finite set of instructions which are being carried in a specific order to perform the specific task. It is not the complete program or code; it is just a solution (logic) of a problem, which can be represented either as an informal description using a Flowchart or Pseudocode.
Characteristics of an Algorithm
The following are the characteristics of an algorithm:
- Input: An algorithm has some input values. We can pass 0 or some input value to an algorithm.
- Output: We will get 1 or more output at the end of an algorithm.
- Unambiguity: An algorithm should be unambiguous which means that the instructions in an algorithm should be clear and simple.
- Finiteness: An algorithm should have finiteness. Here, finiteness means that the algorithm should contain a limited number of instructions, i.e., the instructions should be countable.
- Effectiveness: An algorithm should be effective as each instruction in an algorithm affects the overall process.
- Language independent: An algorithm must be language-independent so that the instructions in an algorithm can be implemented in any of the languages with the same output.
Dataflow of an Algorithm
- Problem: A problem can be a real-world problem or any instance from the real-world problem for which we need to create a program or the set of instructions. The set of instructions is known as an algorithm.
- Algorithm: An algorithm will be designed for a problem which is a step by step procedure.
- Input: After designing an algorithm, the required and the desired inputs are provided to the algorithm.
- Processing unit: The input will be given to the processing unit, and the processing unit will produce the desired output.
- Output: The output is the outcome or the result of the program.
10. Explain external ports in computer system
A port is a physical docking point using which an external device can be connected to the computer. It can also be programmatic docking point through which information flows from a program to the computer or over the Internet.
Characteristics of Ports
A port has the following characteristics −
- External devices are connected to a computer using cables and ports.
- Ports are slots on the motherboard into which a cable of external device is plugged in.
- Examples of external devices attached via ports are the mouse, keyboard, monitor, microphone, speakers, etc.
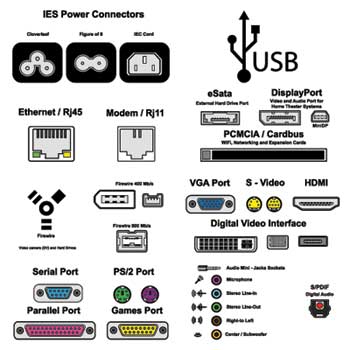
Let us now discuss a few important types of ports −
Serial Port
- Used for external modems and older computer mouse
- Two versions: 9 pin, 25 pin model
- Data travels at 115 kilobits per second
Parallel Port
- Used for scanners and printers
- Also called printer port
- 25 pin model
- IEEE 1284-compliant Centronics port
PS/2 Port
- Used for old computer keyboard and mouse
- Also called mouse port
- Most of the old computers provide two PS/2 port, each for the mouse and keyboard
- IEEE 1284-compliant Centronics port
Universal Serial Bus (or USB) Port
- It can connect all kinds of external USB devices such as external hard disk, printer, scanner, mouse, keyboard, etc.
- It was introduced in 1997.
- Most of the computers provide two USB ports as minimum.
- Data travels at 12 megabits per seconds.
- USB compliant devices can get power from a USB port.
VGA Port
- Connects monitor to a computer's video card.
- It has 15 holes.
- Similar to the serial port connector. However, serial port connector has pins, VGA port has holes.
Power Connector
- Three-pronged plug.
- Connects to the computer's power cable that plugs into a power bar or wall socket.
Firewire Port
- Transfers large amount of data at very fast speed.
- Connects camcorders and video equipment to the computer.
- Data travels at 400 to 800 megabits per seconds.
- Invented by Apple.
- It has three variants: 4-Pin FireWire 400 connector, 6-Pin FireWire 400 connector, and 9-Pin FireWire 800 connector.
Modem Port
- Connects a PC's modem to the telephone network.
Ethernet Port
- Connects to a network and high speed Internet.
- Connects the network cable to a computer.
- This port resides on an Ethernet Card.
- Data travels at 10 megabits to 1000 megabits per seconds depending upon the network bandwidth.
Game Port
- Connect a joystick to a PC
- Now replaced by USB
Digital Video Interface, DVI port
- Connects Flat panel LCD monitor to the computer's high-end video graphic cards.
- Very popular among video card manufacturers.
Sockets
- Sockets connect the microphone and speakers to the sound card of the computer.