UNIT 2
Control Structures
- What is control structures in C and explain its types?
Control Structures are just a way to specify flow of control in programs. Any algorithm or program can be clearer and more understood if they use self-contained modules called as logic or control structures. It basically analyses and chooses in which direction a program flows based on certain parameters or conditions. There are three basic types of logic, or flow of control, known as:
- Sequence logic, or sequential flow
- Selection logic, or conditional flow
- Iteration logic, or repetitive flow
Let us see them in detail:
- Sequential Logic (Sequential Flow)
Sequential logic as the name suggests follows a serial or sequential flow in which the flow depends on the series of instructions given to the computer. Unless new instructions are given, the modules are executed in the obvious sequence. The sequences may be given, by means of numbered steps explicitly. Also, implicitly follows the order in which modules are written. Most of the processing, even some complex problems, will generally follow this elementary flow pattern.
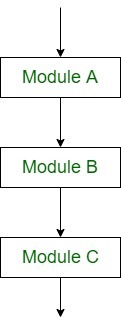
Sequential Control flow
2. Selection Logic (Conditional Flow)
Selection Logic simply involves a number of conditions or parameters which decides one out of several written modules. The structures which use these type of logic are known as Conditional Structures. These structures can be of three types:
- Single Alternative This structure has the form:
- If (condition) then:
- [Module A]
[End of If structure]
- Double Alternative This structure has the form:
- If (Condition), then:
- [Module A]
- Else:
- [Module B]
- [End if structure]
- Multiple Alternatives This structure has the form:
- If (condition A), then:
- [Module A]
- Else if (condition B), then:
- [Module B]
- ..
- ..
- Else if (condition N), then:
- [Module N]
- [End If structure]
In this way, the flow of the program depends on the set of conditions that are written. This can be more understood by the following flow charts:
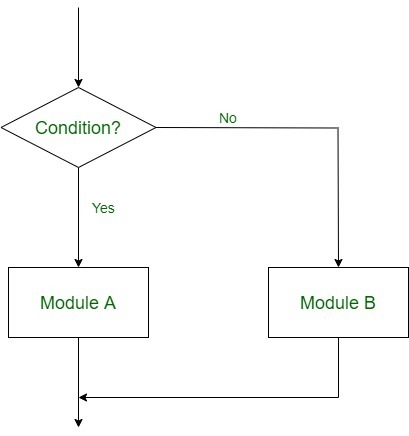
Double Alternative Control Flow
3. Iteration Logic (Repetitive Flow)
The Iteration logic employs a loop which involves a repeat statement followed by a module known as the body of a loop.
The two types of these structures are:
- Repeat-For Structure
This structure has the form: - Repeat for i = A to N by I:
- [Module]
- [End of loop]
Here, A is the initial value, N is the end value and I is the increment. The loop ends when A>B. K increases or decreases according to the positive and negative value of I respectively.
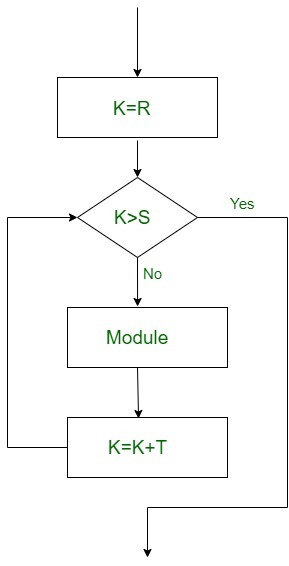
Repeat-For Flow
- Repeat-While Structure
It also uses a condition to control the loop. This structure has the form: - Repeat while condition:
- [Module]
- [End of Loop]
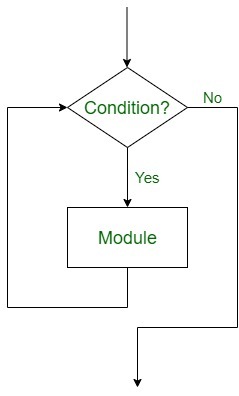
Repeat While Flow
2. Explain Single Character Output: the putchar Function
Single characters can be displayed using the C library function putchar. This function is complementary to the character input function getchar.
The putchar function like getchar is a part of the standard C input/output library. It transmits a single character to the standard output device (the computer screen). The character being transmitted will be represented as a character-type variable. It must be expressed as an argument to the function, enclosed in parentheses, following the word putchar.
In general, a function reference would be written as:
Putchar(character variable)
Where character variable refers to some previously declared character variable.
A simple example demonstrating the use of getchar and putchar is given below:
#include<stdio.h>
Int main()
{
Char c;
Printf("n Please enter a character:");
c=getchar();
Printf("n the character entered is: ");
Putchar(c);
Return 0;
}
In the above program, the statement c=getchar(); accepts a character entered by the user and stores it in the variable c. The character entered by the user can be anything from the C character set. The statement putchar(c); prints the character stored in the variable c.
The putchar function can be used to output a string constant by storing the string within a one-dimensional character-type array. Each character can then be written separately within a loop. The most convenient way to do this is to utilize the for statement, which we will discuss in future.
3. Write some Pre-processors Examples
Analyse the following examples to understand various directives.
#define MAX_ARRAY_LENGTH 20
This directive tells the CPP to replace instances of MAX_ARRAY_LENGTH with 20. Use #define for constants to increase readability.
#include <stdio.h>
#include "myheader.h"
These directives tell the CPP to get stdio.h from System Libraries and add the text to the current source file. The next line tells CPP to get myheader.h from the local directory and add the content to the current source file.
#undef FILE_SIZE
#define FILE_SIZE 42
It tells the CPP to undefine existing FILE_SIZE and define it as 42.
#ifndef MESSAGE
#define MESSAGE "You wish!"
#endif
It tells the CPP to define MESSAGE only if MESSAGE isn't already defined.
#ifdef DEBUG
/* Your debugging statements here */
#endif
It tells the CPP to process the statements enclosed if DEBUG is defined. This is useful if you pass the -DDEBUG flag to the gcc compiler at the time of compilation. This will define DEBUG, so you can turn debugging on and off on the fly during compilation.
4. What are Pre-processor Operators explain in brief?
The C pre-processor offers the following operators to help create macros −
The Macro Continuation (\) Operator
A macro is normally confined to a single line. The macro continuation operator (\) is used to continue a macro that is too long for a single line. For example −
#define message_for(a, b) \
Printf(#a " and " #b ": We love you!\n")
The Stringize (#) Operator
The stringize or number-sign operator ( '#' ), when used within a macro definition, converts a macro parameter into a string constant. This operator may be used only in a macro having a specified argument or parameter list. For example −
#include <stdio.h>
#define message_for(a, b) \
Printf(#a " and " #b ": We love you!\n")
Int main(void) {
Message_for(Carole, Debra);
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Carole and Debra: We love you!
The Token Pasting (##) Operator
The token-pasting operator (##) within a macro definition combines two arguments. It permits two separate tokens in the macro definition to be joined into a single token. For example −
#include <stdio.h>
#define tokenpaster(n) printf ("token" #n " = %d", token##n)
Int main(void) {
Int token34 = 40;
Tokenpaster(34);
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Token34 = 40
It happened so because this example results in the following actual output from the pre-processor −
Printf ("token34 = %d", token34);
This example shows the concatenation of token##n into token34 and here we have used both stringize and token-pasting.
The Defined() Operator
The pre-processor defined operator is used in constant expressions to determine if an identifier is defined using #define. If the specified identifier is defined, the value is true (non-zero). If the symbol is not defined, the value is false (zero). The defined operator is specified as follows −
#include <stdio.h>
#if !defined (MESSAGE)
#define MESSAGE "You wish!"
#endif
Int main(void) {
Printf("Here is the message: %s\n", MESSAGE);
Return 0;
}
When the above code is compiled and executed, it produces the following result −
Here is the message: You wish!
5. Write some Example for Function without argument and return value
Example 1
- #include<stdio.h>
- Void printName();
- Void main ()
- {
- Printf("Hello ");
- PrintName();
- }
- Void printName()
- {
- Printf("Javatpoint");
- }
Output
Hello Javatpoint
Example 2
- #include<stdio.h>
- Void sum();
- Void main()
- {
- Printf("\nGoing to calculate the sum of two numbers:");
- Sum();
- }
- Void sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Printf("The sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
6. Write some Example for Function without argument and with return value
Example 1
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Int result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Result = sum();
- Printf("%d",result);
- }
- Int sum()
- {
- Int a,b;
- Printf("\nEnter two numbers");
- Scanf("%d %d",&a,&b);
- Return a+b;
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the area of the square
- #include<stdio.h>
- Int sum();
- Void main()
- {
- Printf("Going to calculate the area of the square\n");
- Float area = square();
- Printf("The area of the square: %f\n",area);
- }
- Int square()
- {
- Float side;
- Printf("Enter the length of the side in meters: ");
- Scanf("%f",&side);
- Return side * side;
- }
Output
Going to calculate the area of the square
Enter the length of the side in meters: 10
The area of the square: 100.000000
7. Write some Example for Function with argument and without return value
Example 1
- #include<stdio.h>
- Void sum(int, int);
- Void main()
- {
- Int a,b,result;
- Printf("\nGoing to calculate the sum of two numbers:");
- Printf("\nEnter two numbers:");
- Scanf("%d %d",&a,&b);
- Sum(a,b);
- }
- Void sum(int a, int b)
- {
- Printf("\nThe sum is %d",a+b);
- }
Output
Going to calculate the sum of two numbers:
Enter two numbers 10
24
The sum is 34
Example 2: program to calculate the average of five numbers.
- #include<stdio.h>
- Void average(int, int, int, int, int);
- Void main()
- {
- Int a,b,c,d,e;
- Printf("\nGoing to calculate the average of five numbers:");
- Printf("\nEnter five numbers:");
- Scanf("%d %d %d %d %d",&a,&b,&c,&d,&e);
- Average(a,b,c,d,e);
- }
- Void average(int a, int b, int c, int d, int e)
- {
- Float avg;
- Avg = (a+b+c+d+e)/5;
- Printf("The average of given five numbers : %f",avg);
- }
Output
Going to calculate the average of five numbers:
Enter five numbers:10
20
30
40
50
The average of given five numbers : 30.000000
8. What are C Library Functions?
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers. All C standard library functions are defined inside the different header files saved with the extension .h. We need to include these header files in our program to make use of the library functions defined in such header files. For example, to use the library functions such as printf/scanf we need to include stdio.h in our program which is a header file that contains all the library functions regarding standard input/output.
The list of mostly used header files is given in the following table.
SN | Header file | Description |
1 | Stdio.h | This is a standard input/output header file. It contains all the library functions regarding standard input/output. |
2 | Conio.h | This is a console input/output header file. |
3 | String.h | It contains all string related library functions like gets(), puts(),etc. |
4 | Stdlib.h | This header file contains all the general library functions like malloc(), calloc(), exit(), etc. |
5 | Math.h | This header file contains all the math operations related functions like sqrt(), pow(), etc. |
6 | Time.h | This header file contains all the time-related functions. |
7 | Ctype.h | This header file contains all character handling functions. |
8 | Stdarg.h | Variable argument functions are defined in this header file. |
9 | Signal.h | All the signal handling functions are defined in this header file. |
10 | Setjmp.h | This file contains all the jump functions. |
11 | Locale.h | This file contains locale functions. |
12 | Errno.h | This file contains error handling functions. |
13 | Assert.h | This file contains diagnostics functions. |
9. Give Call by Value Example: Swapping the values of the two variables
- #include <stdio.h>
- Void swap(int , int); //prototype of the function
- Int main()
- {
- Int a = 10;
- Int b = 20;
- Printf("Before swapping the values in main a = %d, b = %d\n",a,b); // printing the value of a and b in main
- Swap(a,b);
- Printf("After swapping values in main a = %d, b = %d\n",a,b); // The value of actual parameters do not change by changing the formal parameters in call by value, a = 10, b = 20
- }
- Void swap (int a, int b)
- {
- Int temp;
- Temp = a;
- a=b;
- b=temp;
- Printf("After swapping values in function a = %d, b = %d\n",a,b); // Formal parameters, a = 20, b = 10
- }
Output
Before swapping the values in main a = 10, b = 20
After swapping values in function a = 20, b = 10
After swapping values in main a = 10, b = 20
10. Difference between call by value and call by reference in c
No. | Call by value | Call by reference |
1 | A copy of the value is passed into the function | An address of value is passed into the function |
2 | Changes made inside the function is limited to the function only. The values of the actual parameters do not change by changing the formal parameters. | Changes made inside the function validate outside of the function also. The values of the actual parameters do change by changing the formal parameters. |
3 | Actual and formal arguments are created at the different memory location | Actual and formal arguments are created at the same memory location |