Unit I
Introduction to C and Decision making, Branching, Lopping
1 Explain Basic structure of C program
C program basically consists of the following parts −
- Preprocessor Commands
- Functions
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" –
#include <stdio.h>
Int main() {
/* my first program in C */
Printf("Hello, World! \n");
return 0;
}
The various parts of the above program −
- The first line of the program #include <stdio.h> is a preprocessor command, which tells a C compiler to include stdio.h file before going to actual compilation.
- The next line int main() is the main function where the program execution begins.
- The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. Such lines are called comments in the program.
- The next line printf(...) is another function available in C which causes the message "Hello, World!" to be displayed on the screen.
- The next line return 0; terminates the main() function and returns the value 0.
2 What is Constants?
A constant is a value or variable that can't be changed in the program, for example: 10, 20, 'a', 3.4, "c programming" etc.
There are different types of constants in C programming.
List of Constants in C
Constant | Example |
Decimal Constant | 10, 20, 450 etc. |
Real or Floating-point Constant | 10.3, 20.2, 450.6 etc. |
Octal Constant | 021, 033, 046 etc. |
Hexadecimal Constant | 0x2a, 0x7b, 0xaa etc. |
Character Constant | 'a', 'b', 'x' etc. |
String Constant | "c", "c program", "c in javatpoint" etc. |
2 ways to define constant in C
There are two ways to define constant
- Const keyword
- #define preprocessor
1) C const keyword
The const keyword is used to define constant in C programming.
- Const float PI=3.14;
Now, the value of PI variable can't be changed.
- #include<stdio.h>
- Int main(){
- Const float PI=3.14;
- Printf("The value of PI is: %f",PI);
- Return 0;
- }
Output:
The value of PI is: 3.140000
If you try to change the the value of PI, it will render compile time error.
- #include<stdio.h>
- Int main(){
- Const float PI=3.14;
- PI=4.5;
- Printf("The value of PI is: %f",PI);
- Return 0;
- }
Output:
Compile Time Error: Cannot modify a const object
3 Explain variables
Variables are the names you give to computer memory locations which are used to store values in a computer program.
Here are the following three simple steps −
- Create variables with appropriate names.
- Store your values in those two variables.
- Retrieve and use the stored values from the variables.
When creating a variable, we need to declare the data type it contains.
Programming languages define data types differently.
For example, almost all languages differentiate between ‘integers’ (or whole numbers, eg 12), ‘non-integers’ (numbers with decimals, eg 0.24), and ‘characters’ (letters of the alphabet or words).
- Char – a single 16-bit Unicode character, such as a letter, decimal or punctuation symbol.
- Boolean – can have only two possible values: true (1) or false (0). This data type is useful in conditional statements.
- Byte - has a minimum value of -128 and a maximum value of 127 (inclusive).
- Short– has a minimum value of -32,768 and a maximum value of 32,767
- Int: – has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive).
- Long – has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive).
- Float – a floating point number with 32-bits of precision
- Double – this is a double precision floating point number.
4 What are Data types?
Data Declarations
Declarations serve two purposes:
They tell the compiler to set aside an appropriate amount of space in memory for the program’s data (variables).
They enable the compiler to correctly operate on the variables. The compiler needs to know the data type to correctly operate on a variable. The compiler needs to know how many bytes are used by variables and the format of the bits. The meaning of the binary bits of a variable are different for different data types.
- Char is 8 bit (1 byte) ASCII, but can also store numeric data.
- Int is 4 byte 2’s complement.
- Short is 2 byte 2’s complement.
- Long is 8 byte 2’s complement.
- Unsigned (int, short, and long) are straight binary
- Float is 4 byte IEEE Floating Point Single Precision Standard.
- Double is 8 byte IEEE Floating Point Double Precision Standard.
Character - integer relation
The ASCII code is a set of integer numbers used to represent characters.
Char c = 'a'; /* 'a' has ASCII value 97 */
Inti = 65; /* 65 is ASCII for 'A' */
Printf( "%c", c + 1 ); /* b */
Printf( "%d", c + 2 ); /* 99 */
Printf( "%c", i + 3 ); /* D */
Integers
A variations of int (unsigned, long, ...) are stored binary data which is directly translated to it’s base-10 value.
Floating point data
Variables of type float and double are stored in three parts: the sign, the mantissa (normalized value), and an exponent.
Sizeof
Because some variables take different amount of memory of different systems, C provides an operator which returns the number of bytes needed to store a given type of data.
i = sizeof(char);
j = sizeof(long);
k = sizeof(double);
The sizeof operator is very useful when manually allocating memory and dealing with complex data structures.
Conversions and Casts
Two mechanisms exist to convert data from one data type to another, implicit conversion and explicit conversion, which is also called casting.
Implicit conversion
If an arithmetic operation on two variables of differing types is performed, one of the variables is converted or promoted to the same data as the other before the operation is performed. In general, smaller data types are always promoted to the larger data type.
Explicit conversion or casts
The programmer can tell the compiler what types of conversions should be performed by using a cast. A cast is formed by putting a data type keyword in parenthesis in front of a variable name or expression.
x = (float)(m * j);
i = (int)x + k;
5 Explain Arithmetic Operators
Arithmetic Operator: These are the operators which are useful in performing mathematical calculations. Following are the list of arithmetic operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
+ | Used to add two operands | Result of A+B is 15 | |
2. | - | Used to subtract two operands | Result of A-B is 5 |
3. | * | Used to multiply two operands | Result of A*B is 50 |
4. | / | Used to divide two operands | Result of A/B is 2 |
5. | % | Find reminder of the division of two operand | Result of A%B is 0 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Inta,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of addition of %d and %d is %d",a,b,a+b);
Printf("\n Result of subtraction of %d and %d is %d",a,b,a-b);
Printf("\n Result of multiplication %d and %d is %d",a,b,a*b);
Printf("\n Result of division of %d and %d is %d",a,b,a/b);
Printf("\n Result of modulus of %d and %d is %d",a,b,a%b);
Getch();
}
Output:
Enter two numbers
5
3
Result of addition of 5 and 3 is 8
Result of subtraction of 5 and 3 is 2
Result of multiplication of 5 and 3 is 15
Result of division of 5 and 3 is 1
Result of modulus of 5 and 3 is 2
6 Explain Relational Operators
Relational Operators: Relational operator used to compare two operands. Relational operator produce result in terms of binary values. It returns 1 when the result is true and 0 when result is false. Following are the list of relational operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | < | This is less than operator which is used to check whether the value of left operand is less than the value of right operand or not | Result of A<B is false
|
2. | > | This is greater than operator which is used to check whether the value of left operand is greater than the value of right operand or not | Result of A>B is true |
3. | <= | This is less than or equal to operator | Result of A<=B is false |
4. | >= | This is greater than or equal to operator | Result of A>=B is true |
5. | == | This is equal to operator which is used to check value of both operands are equal or not | Result of A==B is false |
6. | != | This is not equal to operator which is used to check value of both operands are equal or not | Result of A!=B is true |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Inta,b,c;
Clrscr();
Printf("Enter two numbers");
Scanf("%d %d",&a,&b);
Printf("\n Result of less than operator of %d and %d is %d",a,b,a<b);
Printf("\n Result of greater than operator of %d and %d is %d",a,b,a>b);
Printf("\n Result of leass than or equal to operator %d and %d is %d",a,b,a<=b);
Printf("\n Result of greater than or equal to operator of %d and %d is %d", a,b,a>=b);
Printf("\n Result of double equal to operator of %d and %d is %d",a,b,a==b);
Printf("\n Result of not equal to operator of %d and %d is %d",a,b,a!=b);
Getch();
}
Output:
Enter two numbers
5
3
Result of less than operator of 5 and 3 is 0
Result of greater than operator of 5 and 3 is 1
Result of less than or equal to operator of 5 and 3 is 0
Result of greater than or equal to operator of 5 and 3 is 1
Result of double equal to operator of 5 and 3 is 0
Result of not equal to operator of 5 and 3 is 1
7 Explain Arithmetic Expressions
An arithmetic expression is an expression that consists of operands and arithmetic operators. An arithmetic expression computes a value of type int, float or double.
When an expression contains only integral operands, then it is known as pure integer expression when it contains only real operands, it is known as pure real expression, and when it contains both integral and real operands, it is known as mixed mode expression.
Evaluation of Arithmetic Expressions
The expressions are evaluated by performing one operation at a time. The precedence and associativity of operators decide the order of the evaluation of individual operations.
When individual operations are performed, the following cases can be happened:
- When both the operands are of type integer, then arithmetic will be performed, and the result of the operation would be an integer value. For example, 3/2 will yield 1 not 1.5 as the fractional part is ignored.
- When both the operands are of type float, then arithmetic will be performed, and the result of the operation would be a real value. For example, 2.0/2.0 will yield 1.0, not 1.
- If one operand is of type integer and another operand is of type real, then the mixed arithmetic will be performed. In this case, the first operand is converted into a real operand, and then arithmetic is performed to produce the real value. For example, 6/2.0 will yield 3.0 as the first value of 6 is converted into 6.0 and then arithmetic is performed to produce 3.0.
Let's understand through an example.
6*2/ (2+1 * 2/3 + 6) + 8 * (8/4)
Evaluation of expression | Description of each operation |
6*2/( 2+1 * 2/3 +6) +8 * (8/4) | An expression is given. |
6*2/(2+2/3 + 6) + 8 * (8/4) | 2 is multiplied by 1, giving value 2. |
6*2/(2+0+6) + 8 * (8/4) | 2 is divided by 3, giving value 0. |
6*2/ 8+ 8 * (8/4) | 2 is added to 6, giving value 8. |
6*2/8 + 8 * 2 | 8 is divided by 4, giving value 2. |
12/8 +8 * 2 | 6 is multiplied by 2, giving value 12. |
1 + 8 * 2 | 12 is divided by 8, giving value 1. |
1 + 16 | 8 is multiplied by 2, giving value 16. |
17 | 1 is added to 16, giving value 17. |
8 Explain Relational Expressions
- A relational expression is an expression used to compare two operands.
- It is a condition which is used to decide whether the action should be taken or not.
- In relational expressions, a numeric value cannot be compared with the string value.
- The result of the relational expression can be either zero or non-zero value. Here, the zero value is equivalent to a false and non-zero value is equivalent to true.
Relational Expression | Description |
x%2 = = 0 | This condition is used to check whether the x is an even number or not. The relational expression results in value 1 if x is an even number otherwise results in value 0. |
a!=b | It is used to check whether a is not equal to b. This relational expression results in 1 if a is not equal to b otherwise 0. |
a+b = = x+y | It is used to check whether the expression "a+b" is equal to the expression "x+y". |
a>=9 | It is used to check whether the value of a is greater than or equal to 9. |
Let's see a simple example:
- #include <stdio.h>
- Int main()
- {
- Int x=4;
- If(x%2==0)
- {
- Printf("The number x is even");
- }
- Else
- Printf("The number x is not even");
- Return 0;
- }
Output
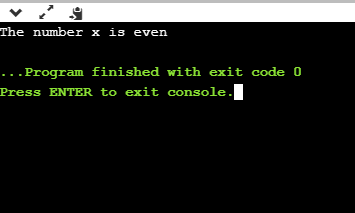
9 Explain Arithmetic precedence and associativity
Arithmetic Expressions consist of numeric literals, arithmetic operators, and numeric variables. They simplify to a single value, when evaluated.
Here is an example of an arithmetic expression with no variables:
3.14*10*10
This expression evaluates to 314, the approximate area of a circle with radius 10. Similarly, the expression
3.14*radius*radius
Would also evaluate to 314, if the variable radius stored the value 10.
Note that the parentheses in the last expression helps dictate which order to evaluate the expression.
Multiplication and division have a higher order of precedence than addition and subtraction. In an arithmetic expression, it is evaluated left to right, only performing the multiplications and divisions.
After doing this, process the expression again from left to right, doing all the additions and subtractions.
So,
3+4*5
First evaluates to
3+20 which then evaluates to 23.
Consider this expression:
3 + 4*5 - 6/3*4/8 + 2*6 - 4*3*2
First go through and do all the multiplications and divisions:
3 + 20 - 1 + 12 - 24
Integer Division: In particular, if the division has a leftover remainder or fraction, this is simply discarded.
For example:
13/4 evaluates to 3
19/3 evaluates to 6 but
Similarly, if you have an expression with integer variables part of a division, this evaluates to an integer as well.
For example, in this segment of code, y gets set to 2.
Int x = 8;
Int y = x/3;
However, if we did the following,
Double x = 8;
Double y = x/3;
y would equal 2.66666666 (approximately).
The way C decides whether it will do an integer division (as in the first example), or a real number division (as in the second example), is based on the TYPE of the operands.
If both operands are ints, an integer division is done.
If either operand is a float or a double, then a real division is done. The compiler will treat constants without the decimal point as integers, and constants with the decimal point as a float. Thus, the expressions 13/4 and 13/4.0 will evaluate to 3 and 3.25 respectively.
The mod operator (%)
The one new operator is the mod operator, which is denoted by the percent sign(%). This operator is ONLY defined for integer operands. It is defined as follows:
a%b evaluates to the remainder of a divided by b.
For example,
12%5 = 2
19%6 = 1
14%7 = 0
19%200 = 19
The precedence of the mod operator is the same as the precedence of multiplication and division.
10 What is Type Conversion?
Implicit type conversion:
Also known as ‘automatic type conversion’.
- Done by the compiler on its own, without any external trigger from the user.
- Generally, takes place when in an expression more than one data type is present. In such condition type conversion (type promotion) takes place to avoid to loose data.
- All the data types of the variables are upgraded to the data type of the variable with largest data type.
Bool -> char -> short int ->int ->
unsigned int -> long -> unsigned ->
long long -> float -> double -> long double
It is possible for implicit conversions to lose information, signs can be lost (when signed is implicitly converted to unsigned), and overflow can occur (when long is implicitly converted to float).
Example of Type Implicit Conversion:
// An example of implicit conversion #include<stdio.h> Intmain() { Intx = 10; // integer x Chary = 'a'; // character c
// y implicitly converted to int. ASCII // value of 'a' is 97 x = x + y;
// x is implicitly converted to float Floatz = x + 1.0;
Printf("x = %d, z = %f", x, z); Return0; } |
|
Output:
x=107, z = 108.000000
Explicit Type Conversion–
This process is also called type casting and it is user defined. Here the user can type cast the result to make it of a particular data type.
The syntax in C:
(type) expression
Type indicated the data type to which the result is converted.
// C program to demonstrate explicit type casting #include<stdio.h>
Intmain() { Doublex = 1.2;
// Explicit conversion from double to int Intsum = (int)x + 1;
Printf("sum = %d", sum);
Return0; }
Output:
Sum =2
Advantages of type conversion:
This is done to take advantage of certain features of type hierarchies or type representations.
It helps us to compute the expression containing variables of different data types.
|