Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | void main() | Execution of program begins |
4. | { | Memory is allocated for variable i,n and array a |
5. | inti,n,a[10]; | |
6. | clrscr(); | Clear the output of previous screen |
7. | printf("enter a number"); | Print “enter a number” |
8. | scanf("%d",&n); | Input value is stored at the addres of variable n |
9. | for(i=0;i<=10;i++) | For loop started from value of i=0 to i=10 |
10. | { | Compound statement(scope of for loop starts) |
11. | a[i]=n*i; | Result of multiplication of n and I is stored at the ith location of array ieasi=0 so it is stores at first location. |
12. | printf("\n %d",a[i]); | Value of ith location of the array is printed |
13. | } | Compound statement(scope of for loop ends) |
14. | printf("\n first element in array is %d",a[0]); | Value of first element of the array is printed |
15. | printf("\n fifth element in array is %d",a[4]); | Value of fifth element of the array is printed |
16. | printf("\n tenth element in array is %d",a[9]); | Value of tenth element of the array is printed |
17. | getch(); | Used to hold the output screen |
18. | } | Indicates end of scope of main function |
#include<conio.h>
void main()
{
int a[20];
intn,i;
printf("\n Enter the size of array :");
scanf("%d",&n);
printf("size of array is %d",n);
printf("\n Enter Values to store in array one by one by pressing ENTER :");
for(i=0;i<n;i++)
{
scanf("%d",&a[i]);
}
printf("\n The Values in array are..");
for(i=0;i<n;i++)
{
printf("\n %d",a[i]);
}
getch();
}
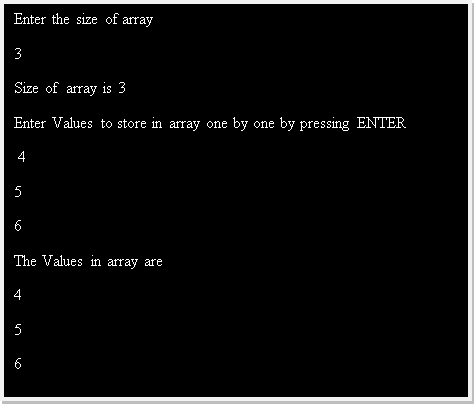
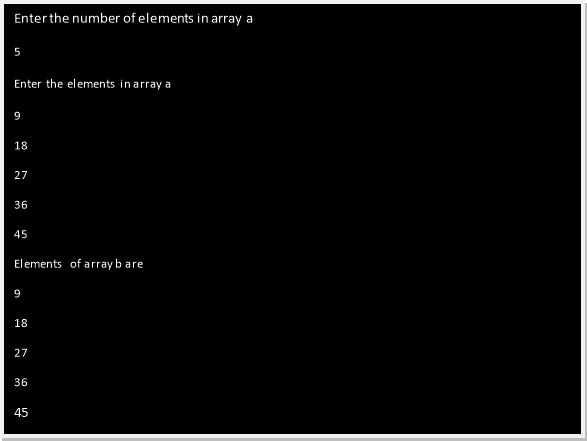
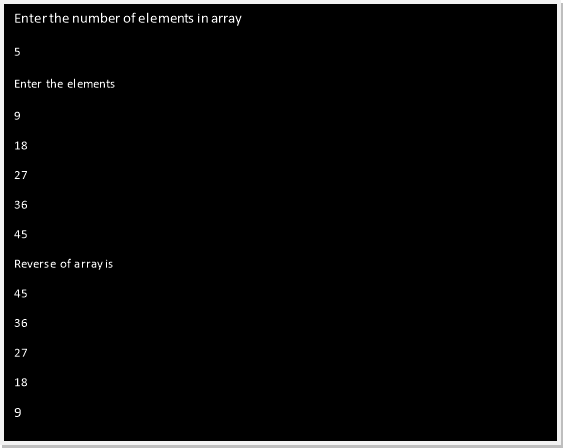
#include<conio.h>
void main()
{
int a[20];
intn,i;
printf("\n Enter the size of array :");
scanf("%d",&n);
printf("size of array is %d",n);
printf("\n Enter Values to store in array one by one by pressing ENTER :");
for(i=0;i<n;i++)
{
scanf("%d",&a[i]);
}
printf("\n The Values in array are..");for(i=0;i<n;i++)
{
printf("\n %d",a[i]);
}
getch();
}
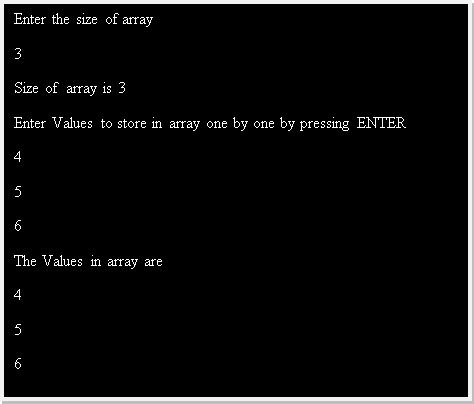
0 1 2 3 4 |
|