Return Type Function name (Argument list)
{
Statement 1;
Statement 2;
…………...
Statement n;
}
Q2. Explain the scope of variables in functions?A2. Library FunctionsA function which is predefined in c language is called library function. Library function is also called as built in function of C language. The definition of library function is stored in respective header file. Library functions are used to perform dedicated operation like taking input from user, displaying output, string handling operation, etc. Library functions are readily available and programmer can directly use it without writing any extra code. For example, printf () and scanf () are library function and their definition is stored in stdio header file. 2. User Defined FunctionsUser define function is the block of code written by programmer to perform a particular task. As compiler doesn’t have any idea about the user define function so programmer has to define and declare these functions inside the program body. Programmer can define these function outside the main function but declaration of user define function should present in main function only. Whenever compiler executes function call (function declaration) then compiler shift the flow of program execution to the definition part of user define function. Example#include <stdio.h>
#include<conio.h>
int add (int x, int y)
{
int sum;
sum = x + y;
return (sum);
}
main ()
{
int a,b,c;
a = 15;
b = 25;
c = add(a,b);
printf ("\n Addition is %d ", c);
}
Output:Addition is 40There are two ways to pass the parameters to the function#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
void swap( int p1, int p2 );
int main()
{
int a = 10;
int b = 20;
printf("Before: Value of a = %d and value of b = %d\n", a, b );
swap( a, b );
printf("After: Value of a = %d and value of b = %d\n", a, b );
getch();
}
void swap( int p1, int p2 )
{
int t;
t = p2;
p2 = p1;
p1 = t;
printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output :Before: Value of a = 10 and value of b = 20Value of a (p1) = 20 and value of b (p2) = 10After: Value of a = 10 and value of b = 20Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.Q4. Write a program to swap two numbers using call by value and reference.A4.#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
void swap( int p1, int p2 );
int main()
{
int a = 10;
int b = 20;
printf("Before: Value of a = %d and value of b = %d\n", a, b );
swap( a, b );
printf("After: Value of a = %d and value of b = %d\n", a, b );
getch();
}
void swap( int p1, int p2 )
{
int t;
t = p2;
p2 = p1;
p1 = t;
printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output :Before: Value of a = 10 and value of b = 20Value of a (p1) = 20 and value of b (p2) = 10After: Value of a = 10 and value of b = 20Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.Q5. Explain how you pass arrays to functions?A5. Array is a data structure which stores the collection of similar types of element in consecutive memory locations. Indexing of array always start with ‘0’ where as non-graphical variable ‘\0’ indicates the end of array. Syntax for declaring array is data type array name[Maximum size];Sr. No. | Instructions | Description |
#include<stdio.h> | Header file included | |
2. | #include<conio.h> | Header file included |
3. | void main() | Execution of program begins |
4. | { | Memory is allocated for variable i,n and array a |
5. | int i,n,a[10]; | |
6. | clrscr(); | Clear the output of previous screen |
7. | printf("enter a number"); | Print “enter a number” |
8. | scanf("%d",&n); | Input value is stored at the addres of variable n |
9. | for(i=0;i<=10;i++) | For loop started from value of i=0 to i=10 |
10. | { | Compound statement(scope of for loop starts) |
11. | a[i]=n*i; | Result of multiplication of n and I is stored at the ith location of array ieasi=0 so it is stores at first location. |
12. | printf("\n %d",a[i]); | Value of ith location of the array is printed |
13. | } | Compound statement(scope of for loop ends) |
14. | printf("\n first element in array is %d",a[0]); | Value of first element of the array is printed |
15. | printf("\n fifth element in array is %d",a[4]); | Value of fifth element of the array is printed |
16. | printf("\n tenth element in array is %d",a[9]); | Value of tenth element of the array is printed |
17. | getch(); | Used to hold the output screen |
18. | } | Indicates end of scope of main function |
#include <stdio.h>
#include<conio.h>
/* function declaration goes here.*/
void swap( int p1, int p2 );
int main()
{
int a = 10;
int b = 20;
printf("Before: Value of a = %d and value of b = %d\n", a, b );
swap( a, b );
printf("After: Value of a = %d and value of b = %d\n", a, b );
getch();
}
void swap( int p1, int p2 )
{
int t;
t = p2;
p2 = p1;
p1 = t;
printf("Value of a (p1) = %d and value of b(p2) = %d\n", p1, p2 );
}
Output :Before: Value of a = 10 and value of b = 20Value of a (p1) = 20 and value of b (p2) = 10After: Value of a = 10 and value of b = 20Note: In the above example the values of “a” and “b” remain unchanged before calling swap function and after calling swap function.Q6. Write a program to find the sum of N natural numbers using functions?A6. /* C Program to find Sum of N Numbers using Functions */ #include<stdio.h>int Sum_Of_Natural_Numbers(int Number); int main(){ int Number, i, Sum = 0; printf("\nPlease Enter any Integer Value\n");scanf("%d", &Number); Sum = Sum_Of_Natural_Numbers(Number); printf("Sum of Natural Numbers = %d", Sum); return 0;} int Sum_Of_Natural_Numbers(int Number){ int i, Sum = 0; if (Number == 0) { return Number; } else { return (Number * (Number + 1) / 2); } }Output :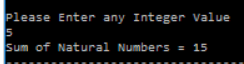
Enter two positive integers: 81
153
GCD = 9
Q8. Write a program to find the factorial of a number using functions?A8. #include<stdio.h>#include<math.h>void main(){ //clrscr();printf("Enter a Number to Find Factorial: ");fact();getch();}fact(){ int i,fact=1,n;scanf("%d",&n);for(i=1; i<=n; i++) { fact=fact*i; }printf("\nFactorial of a Given Number is: %d ",fact); return fact;} OUTPUT:Enter a Number to Find Factorial: 5Factorial of a Given Number is: 120Q9. Write a program to check if the given number is prime or not?A9. #include <conio.h>void main(){ int num,res=0; clrscr(); printf("\nENTER A NUMBER: "); scanf("%d",&num); res=prime(num); if(res==0) printf("\n%d IS A PRIME NUMBER",num); else printf("\n%d IS NOT A PRIME NUMBER",num); getch();}int prime(int n){ int i; for(i=2;i<=n/2;i++) { if(n%i!=0) continue; else return 1; } return 0;} Output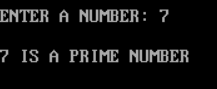