#include<stdio.h>
#include<conio.h>
void main()
{
int a,b,c,
printf (“Enter two numbers”);
scanf (“%d%d”, &a,&b);
c=a+b;
printf(“/n Addition is %d”, C);
printf(“/n Address of variable C is %d”, &C);
getch ();
}
Above program is the program for addition of two numbers in ‘C’ language. In the sevent instruction of above program we used operator ‘%’ and ‘&’ ‘%d’ is the access specifier which tells compiler to take integer value as ainpute whereas. ‘&a’ tells compile to store the taken value at the address of variable ‘a’.In the ninth instruction compiler will print me value of ‘C’ so the output of 9th instruction is as follows.Addition is 5 [Note : considering a = 2 and b = 3]In the tenth instruction compiler will print me address of variable C.So the output of lenth instruction is as follows. Address of variable C is 1020.Now consider the following program.#include<stdio.h>
#include<conio.h>
void main()
{
int a,b,c;
printf(“Enter two numbers”);
scanf(“%d %d”, & a, &b);
c=a+b;
printf(“\n Addition is %d”,C);
printf(“\n Address of variable C is %d”,&C);
printf(‘\n value of variable C is %d”, *(&C));
getch();
}
Now, we all are clear about the output of instruction 9 and 10.In the 11th instruction, operator *and & is used. ‘*’ operator tells compile to pickup the value which is stored at the address of variable C. So the output of 11th instruction is as follow. Value of variable C is 5 [considering a = 24 b = 3;]Q2. Write a program to add two numbers using pointers?A2. #include <stdio.h>int main(){ int first, second, *p, *q, sum;printf("Enter two integers to add\n");scanf("%d%d", &first, &second); p = &first; q = &second; sum = *p + *q;printf("Sum of the numbers = %d\n", sum); return 0;}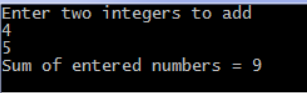
An important point to consider is that the pointer should be initialized properly before accessing, as by default it contains garbage value.Q4. Write a program to store in a data persons name age and weight using pointers?A4. #include <stdio.h>#include <string.h> struct student { float weight char name[30]; int age;}; int main() { int i; struct student record1 = {40, "Gideon", 11}; struct student *ptr;ptr = &record1;printf("Records of STUDENT1: \n");printf(" Weight is: %d \n", ptr->weight);printf(" Name is: %s \n", ptr->name);printf(" Age is: %f \n\n", ptr->age); return 0;}Q5. Explain dynamic memory allocation?A5. To allocate memory dynamically, library functions are malloc(), calloc(), realloc() and free() are used. These functions are defined in the <stdlib.h> header file.The name "malloc" stands for memory allocation.The malloc() function reserves a block of memory of the specified number of bytes. And, it returns a pointer of void which can be casted into pointers of any form.Syntax of malloc()ptr = (castType*) malloc(size);Exampleptr = (float*) malloc(100 * sizeof(float));calloc()The name "calloc" stands for contiguous allocation.The malloc() function allocates memory and leaves the memory uninitialized. Whereas, the calloc() function allocates memory and initializes all bits to zero.Syntax of calloc()ptr = (castType*)calloc(n, size);Example:ptr = (float*) calloc(25, sizeof(float));Dynamically allocated memory created with either calloc() or malloc() doesn't get freed on their own. You must explicitly use free() to release the space.Syntax of free()free(ptr);realloc()If the dynamically allocated memory is insufficient or more than required, you can change the size of previously allocated memory using the realloc() function.Syntax of realloc()ptr = realloc(ptr, x);Here, ptr is reallocated with a new size x.Q6. Elaborate on linked list.A6. Linked List is a collection of multiple nodes. Each node is connected to other node via link or pointer. Every node consists of two fields.
Data |
Address of next node |
Left link | Data | Right Link |
#include<stdio.h>
#include<conio.h>
void main()
{
int n=5;
int *np,**npp;
np=&n;
npp=&np;
printf("\n value of n is %d",n);
printf("\n value of n is %d",*np);
printf("\n value of n is %d",**npp);
getch();
}
Above program is basic program for double pointer which contains three ‘printf’ statements. The explanation of these statements are as follows. 1. In the first ‘printf’ statement compiler will directly print the value of variable ‘n’ which is ‘5’. 2. In the second ‘printf’ statement compiler will print the value of pointer variable ‘np’. ‘np’ variable contains the address of variable ‘n’ but as we put the asterisk sign before it so it will print the value which is present at the address of variable ‘n’ which is ‘5’ 3. In the third printf statement compiler will print the value of pointer to pointer variable ‘npp’. ‘npp’ variable contains the address of pointer variable ‘np’ and ‘np’ variable contains the address of variable n’ but as we put the two asterisk signs before it so it will print the value which is present at the address of variable ‘n’ which is ‘5’. If we put only one asterisk sign the it will print the address of variable ‘n’.User can allocate any name to the pointer variable by following all the rules of allocating variableQ8. Explain pointer to arrays.A8. Pointer is a special variable that can hold the address of another variable whereas arrays are the special type of linear data structure in which elements are stored in contiguous memory locations. Pointers to array are used to retrieve the values of array element from their location. Pointer to array means that a variable which is meant to hold the address of array element. Following is the syntax of assigning the address of array element to pointer.pointer-name = & array-name[element]; For example:int arr[4];
int *p;
p= &arr[1];
In the above example, pointer variable ‘p’ is pointing the address of second variable of the array ‘arr’. Following figure depict the pictorial representation of above code.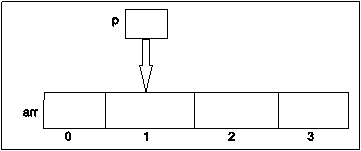
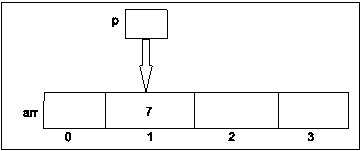
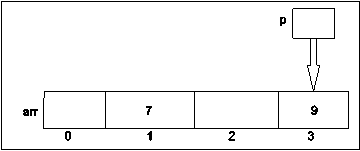
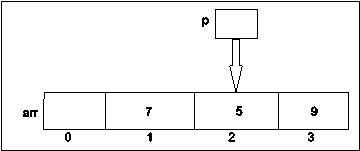
int *p,*q, r,s;
int arr[4];
arr[1]=7;
arr[2]=5;
arr[3]=9;
p=&arr[2];
q=&arr[3];
r= p-q;
s=q-p;
In the above program variable ‘r’ contains the value ‘-1’ whereas variable ‘s’ contain value ’1’.Q9. Write a program to represent pointer to array.A9. Pointer to Single Dimensional Arrays Single dimensional array are the data structure in which all the elements are stored in a single row. Indexing of single dimensional array starts with 0 whereas last element has index (array size-1). All the elements of the array are of similar data-types, C language does not allow user to store elements of two or more different data type in a single array. Suppose user wants to store string ‘Hello Raushan’ then initially user required character type of array. Minimum size of an array to store above string is 13 as it contains 12 alphabets and one space. A pointer variable can store the address of only one array variable at a single instant of time. Similar to array, pointer can also hold the address of only respective type of element. For the above string user required character type of pointer. Following is the syntax of declaring single dimensional array and a pointer to array. char arr[13]= {‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘ ’, ‘R’, ‘a’, ‘u’, ‘s’, ‘h’, ‘a’, ‘n’}; char *p; p=&arr[0];In the above syntax, First line represents the syntax of declaring and defining single dimensional array. Here we declare the character type of array of size 13. Total memory allocated to the ‘arr’ array is 13. This array can hold 13 elements having data type ‘char’ and all these elements are stored in the consecutive memory location. Here we are storing string ‘Hello Raushan’ to the array ‘arr’. The index of first element (ie ‘H’) is arr[0] where as the last element (ie ‘n’) is having index arr[12].Second line represents the syntax of declaring pointer variable. Here, we define ‘p’ as a pointer variable which is of type ‘char’. This pointer variable can perform the operation on element having data type ‘char’. Third line represents the syntax of defining pointer variable. Here, we assign the address of first element of the array ‘arr’ to pointer variable ‘p’.Memory map of above syntax is as follows#include<stdio.h>
void main()
{
int a[]={1,2,3,5,6,7,8};
int *p= a;
printf("\n This is the address of a %u, value of &a is %u ,Address of first element %d ,
Value stored at first place is %d",a,&a,&a[0],*a);
printf("\nThis is the address at p %u , value at p %u and the value pointed by p
%d",&p,p,*p);
printf("\n");
}
Output:This is the address of a 3219815716, value of &a is 3219815716 ,Address of first element 3219815716 , Value stored at first place is 1This is the address at p 3219815712 , value at p 3219815716 and the value pointed byp 1Consider the following program
#include<stdio.h>
#include<conio.h>
void main()
{
int arr[3];
int *p;
arr[0]=3;
arr[1]=6;
arr[2]=8;
for(i=0;i<3;i++)
{
p= &arr[i];
printf(“address of %d element is”,p);
printf(“\t value of %d element is \n”,*p);
}
In the above program, we define the array of size 3 in which value of first element of the array is 3, second element has value 6 and last element has value 8.Integer type of pointer variable ‘p’ initially points to the first element of the array. So, first ‘printf’ statement is responsible to print the address of array element whereas second ‘printf’ statement is used to print the value of array element using pointer. For the first iteration of ‘for’ loop, value of loop variable ‘I’ is ‘0’. So, pointer variable ‘p’ contains the address of first element of the array ‘arr’. First ‘printf’ statement will print the memory address of first element of the array and second ‘printf’ statement print the value of first element of the array ie ‘3’.In the second iteration loop variable is incremented by one. Now, ‘p’ contains the address of second element of the array ie ‘arr[1]’. So, this time ‘printf’ statements prints the address and value of second element of the array.In the third iteration loop variable is incremented by one and it becomes ‘2’. Now, ‘p’ contains the address of third element of the array ie ‘arr[2]’. So, this time ‘printf’ statements prints the address and value of third element of the array.Again the value of loop variable is incremented by one and it becomes ‘3’. So, the condition inside ‘for’ loop is not satisfied and flow of program execution comes out of loop. Q10. Write a note on pointer to multidimensional array.A10. Pointer to Multidimensional ArraysIn multidimensional array all the elements of the array are stored in multiple stacks of rows. Two dimensional array has two stacks of rows, it looks like two dimensional matrix containing rows and columns. Three dimensional array has three stacks of rows and it looks like a rubik’s cube. Like single dimensional array, all the elements of multidimensional array are of same type and C language does not allow user to store elements of two or more different data type in a single multidimensional array. Suppose user wants to store string ‘Hello Raushan’ in two dimensional array, minimum size of an array to store above strings is 13 as it contains 12 alphabets and one space. Two dimensional arrays are built with rows and columns. For the above string we need to divide total array size into rows and columns. A pointer variable can store the address of only one array variable at a single instant of time. Similar to array, pointer can also hold the address of only respective type of element. For the above string user required character type of pointer. Following is the syntax of declaring two dimensional array and a pointer to array.char arr[2][7]= {‘H’, ‘e’, ‘l’, ‘l’, ‘o’, ‘ ’, ‘R’, ‘a’, ‘u’, ‘s’, ‘h’, ‘a’, ‘n’};char *p;p=&arr[0][0];In the above syntax, First line represents the syntax of declaring and defining two dimensional array. Here we declare the character type of array of size 14, which is divided into two rows and seven columns. Total memory allocated to the ‘arr’ array is 14. This array can hold 14 elements having data type ‘char’ and all these elements are stored in the consecutive memory location. Out of 14 elements the first 7 elements are stored in the first row whereas remaining elements are stored in the second row. The index of first element (ie ‘H’) is arr[0][0] where as the last element (ie ‘n’) is having index arr[1][5]. At the last position of the array iearr[1][6] contains garbage value.Second line represents the syntax of declaring pointer variable. Here, we define ‘p’ as a pointer variable which is of type ‘char’. This pointer variable can perform the operation on element having data type ‘char’. Third line represents the syntax of defining pointer variable. Here, we assign the address of first element of the array ‘arr’ to pointer variable ‘p’. Memory map of above syntax is as follows#include<stdio.h>
#include<conio.h>
void main()
{
int arr[2][3],i,j,*p;
clrscr();
printf("enter the elements of array");
for(i=0;i<2;i++)
{
for(j=0;j<3;j++)
{
scanf("%d",&arr[i][j]);
}
}
printf("Two dimensional array is\n");
for(i=0;i<2;i++)
{
for(j=0;j<3;j++)
{
printf("\t %d",arr[i][j]);
}
printf("\n");
}
for(i=0;i<2;i++)
{
for(j=0;j<3;j++)
{
p=&arr[i][j];
printf("\n address is %d and value is %d",p,*p);
}
}
getch();
}
Output: Enter the elements of array 4 5 6 1 2 3 Two dimensional array is 4 5 6 1 2 3 address is -24 and value is 4 address is -22 and value is 5 address is -20 and value is 6 address is -18 and value is 1 address is -16 and value is 2 address is -14 and value is 3In the above program, we define the array of size 6 which is divided in to two rows and three columns. in which value of first element of the array is 4 which is stored at location of arr[0][0], second element has value 4, which is stored at location of arr[0][1]and last element has value 3, which is stored at location of arr[1][2].Q11. Explain pointer to strings.A11. In ‘C’ language we can store string in the array of character. Array of character holding string is terminated by null character (‘\0’). C language treats ‘’\0’’ as a one character. ‘\0’ represents the end og the string and also return logical false value. Each element in the array of character requires one byte of memory storage. User can define string constant by putting all the characters of string in a pain of double quotes. C compiler automatically adds ‘\0’ at the end of every string. There are two ways to initialize the string 1. Initialize the string by using arraychar array_name[size]={‘a1’, ‘a2’,…..’an’, ‘\0’};
Example: char arr[7]={‘H’, ’e’, ‘l’, ’l’, ’o’, ‘\0’}; 2. Initialize the string constant by using arraychar array_name[size]=”string”;
Example: char arr[7]=”Hello” In the above syntax, ’C’ compiler automatically introduce ‘\0’ at the end of the string. Array size is not the compulsory factor in the string initialization. User is free to define unsized character array. In unsized character array compiler will calculate the length of string and allocate the required memory space to the array of characters 3. Initialize the string constant by using pointerchar *pointer_name;
pointer_name = “string”;
char *ptr;
ptr =”Hello”;
#include<stdio.h>
#include<conio.h>
void main()
{
char str1[] = {'F','i', 'r', 's', 't','\0'};
char str2[] = "Second";
char *ptr;
int i;
clrscr();
for (i=0; str1[i]; i++)
printf("%c", str1[i]);
printf("\n");
for (i=0; str2[i]; i++)
printf("%c", str2[i]);
printf("\n");
ptr = "Third Sring with pointer";
for (i=0; *ptr; i++)
printf("%c", *ptr++);
getch();
}
#include<stdio.h>
#include<conio.h>
void main()
{
char str1[] = {'F','i', 'r', 's', 't','\0'};
char str2[] = "Second";
char *ptr;
int i,n;
clrscr();
for (i=0; str1[i]; i++)
printf("%c", str1[i]);
printf("\n");
for (i=0; str2[i]; i++)
printf("%c", str2[i]);
printf("\n");
ptr = "Third Sring with pointer";
for (i=0; *ptr; i++)
{
if(i==0)
{
printf("address of first element ie %c is %d\n",*ptr,ptr);
printf("\n Modified string is as follows\n");
}
printf("%c\t", *ptr++);
n=ptr;
if(n%2==0)
printf("$");
}
getch();
}
T | $h | I | $r | d | $ | S | $t | r | $i | n | $g |
$w | I | $t | h | $ | p | $o | i | $n | T | $e | r |
#include<stdio.h>
#include<conio.h>
void sayhi()
{
printf("hi everyone");
}
void main()
{
clrscr();
sayhi();
getch();
}
#include<stdio.h>
#include<conio.h>
void sayhi()
{
printf("hi everyone");
}
void main()
{
void(*sayhiptr)()=sayhi;
clrscr();
(*sayhiptr)();
getch();
}
#include<stdio.h>
#include<conio.h>
void total(int m1,int m2, int m3)
{
int result=m1+m2+m3;
printf("\n Total marks is %d",result);
}
void main()
{
int m1,m2,m3;
void (*totalptr)()=total;
clrscr();
printf("Enter the marks of three subjects");
scanf("%d %d %d", &m1,&m2,&m3);
(*totalptr)(m1,m2,m3);
getch();
}
1 2 3 4 5 6 7 8 9 10 11 12 | struct dog { char name[10]; char breed[10]; int age; char color[10]; };
struct dog spike;
// declaring a pointer to a structure of type struct dog struct dog *ptr_dog |
1 | ptr_dog = &spike; |
(*ptr_dog).breed – refers to the breed of dog Parentheses around *ptr_dog are necessary because the precedence of dot(.) operator is greater than that of indirection (*) operator. Using arrow (->) operator or membership operator. To access members using arrow (->) operator write pointer variable followed by -> operator, followed by name of the member.ptr_dog->name - refers to the name of dogptr_dog->breed - refers to the breed of dog We can also modify the value of members using pointer notation.
| strcpy(ptr_dog->name, "new_name");
In the above expression precedence of arrow operator (->) is greater than that of prefix decrement operator (--), so first -> operator is applied in the expression then its value is decremented by 1. #include<stdio.h>
struct dog { char name[10]; char breed[10]; int age; char color[10]; };
int main() { struct dog my_dog = {"tyke", "Bulldog", 5, "white"}; struct dog *ptr_dog; ptr_dog = &my_dog;
printf("Dog's name: %s\n", ptr_dog->name); printf("Dog's breed: %s\n", ptr_dog->breed); printf("Dog's age: %d\n", ptr_dog->age); printf("Dog's color: %s\n", ptr_dog->color);
// changing the name of dog from tyke to jack strcpy(ptr_dog->name, "jack");
// increasing age of dog by 1 year ptr_dog->age++;
printf("Dog's new name is: %s\n", ptr_dog->name); printf("Dog's age is: %d\n", ptr_dog->age);
// signal to operating system program ran fine return 0; } Expected Output: Dog's name: tyke Dog's breed: Bulldog Dog's age: 5 Dog's color: white
After changes
Dog's new name is: jack Dog's age is: 6
|