Unit – 2
Introduction to Classes and Objects, Inheritance and String Manipulations
Q1) What is an object in Java?
A1)
An entity that has state and behavior is known as an object e.g., chair, bike, marker, pen, table, car, etc. It can be physical or logical (tangible and intangible).
The example of an intangible object is the banking system.
An object has three characteristics:
a) State: represents the data (value) of an object.
b) Behavior: represents the behavior (functionality) of an object such as deposit, withdraw, etc.
c) Identity: An object identity is typically implemented via a unique ID. The value of the ID is not visible to the external user. It is used internally by the JVM to identify each uniquely.
An object is an instance of a class. A class is a template or blueprint from which objects are created. So, an object is the instance(result) of a class.
Object Definitions:
- An object is a real-world entity.
- An object is a runtime entity.
- The object is an entity which has state and behavior.
- The object is an instance of a class.
Q2) What is a class in Java?
A2)
A class is a group of objects which have common properties. It is a template or blueprint from which objects are created. It is a logical entity. It can't be physical.
A class in Java can contain:
- Fields
- Methods
- Constructors
- Blocks
- Nested class and interface
Syntax of the class:
Class<class_name>
{ No. Of fields;
No.of methods;
}
Q3) What is the constructor?
A3)
The constructor can be defined as the special type of method that is used to initialize the state of an object.
It is invoked when the class is instantiated, and the memory is allocated for the object. Every time, an object is created using the new keyword, the default constructor of the class is called. The name of the constructor must be similar to the class name. The constructor must not have an explicit return type.
There are two types of constructors in Java:
1. Default constructor (no-arg constructor)
A constructor is called "Default Constructor" when it doesn't have any parameter.
Syntax of default constructor: <class_name>(){ }
Example of default constructor that displays the default values
//Let us see example of default constructor which displays the default values
Class Student3{
Int id;
String name;
//method to display the value of id and name
Void display()
{ System.out.println(id+" "+name); }
Public static void main(String args[])
{ //creating objects
Student3 s1=new Student3();
Student3 s2=new Student3();
//displaying values of the object
s1.display();
s2.display();
}
}
OUTPUT:
0 null
0 null
Explanation:
In the above class, you are not creating any constructor so compiler provides you a default constructor. Here 0 and null values are provided by default constructor.
2. Parameterized Constructor
A constructor which has a specific number of parameters is called a parameterized constructor.
The parameterized constructor is used to provide different values to distinct objects. However, you can provide the same values also.
Example of parameterized constructor
//Java Program to demonstrate the use of the parameterized constructor.
Class Student4{
Int id;
String name;
//creating a parameterized constructor
Student4(int i,String n){
Id = i;
Name = n;
}
//method to display the values
Void display(){System.out.println(id+" "+name);}
Public static void main(String args[])
{ //creating objects and passing values
Student4 s1 = new Student4(111,"Karan");
Student4 s2 = new Student4(222,"Aryan");
//calling method to display the values of object
s1.display();
s2.display();
}
}
OUTPUT:
111 Karan
222 Aryan
Rules for creating Java constructor
There are two rules defined for the constructor.
- Constructor name must be the same as its class name
- A Java constructor cannot be abstract, static, final, and synchronized
Q4) What is the purpose of a default constructor?
A4)
The purpose of the default constructor is to assign the default value to the objects.
The java compiler creates a default constructor implicitly if there is no constructor in the class.
Example:
Class Student3
{ int id;
String name;
Void display(){System.out.println(id+" "+name);
}
Public static void main(String args[])
{
Student3 s1=new Student3();
Student3 s2=new Student3();
s1.display();
s2.display();
}
}
OUTPUT:
0 null
0 null
Explanation: In the above class, we are not creating any constructor, so compiler provides a default constructor. Here 0 and null values are provided by default constructor.
Q5) Give difference between constructor and method in Java?
A5)
Java Constructor | Java Method |
A constructor is used to initialize the state of an object. | A method is used to expose the behavior of an object. |
A constructor must not have a return type. | A method must have a return type. |
The constructor is invoked implicitly. | The method is invoked explicitly. |
The Java compiler provides a default constructor if you don't have any constructor in a class. | The method is not provided by the compiler in any case. |
The constructor name must be same as the class name. | The method name may or may not be same as the class name. |
Q6) Explain Java Static method?
A6)
When we apply static keyword with any method, it is known as static method.
- A static method belongs to the class rather than the object of a class.
- A static method can be invoked without the need for creating an instance of a class.
- A static method can access static data member and can change the value of it.
Example:
Java Program to get the cube of a given number using the static method
Class Calculate
{ static int cube(int x){
Return x*x*x;
}
Public static void main(String args[])
{ int result=Calculate.cube(5);
System.out.println(result);
}
}
OUTPUT:
125
Q7) What do you understand by copy constructor in Java?
A7)
There is no copy constructor in java. we can copy the values from one object to another like copy constructor in C++.
There are many ways to copy the values of one object into another in java. They are:
- By constructor
- By assigning the values of one object into another
- By clone() method of Object class
Example, we are going to copy the values of one object into another using java constructor. //Java program to initialize the values from one object to another
Class Student
{ int id;
String name;
//constructor to initialize integer and string
Student6(int i,String n)
{ id = i;
Name = n;
}
//constructor to initialize another object
Student(Student s)
{ id = s.id;
Name =s.name;
}
Void display()
{ System.out.println(id+" "+name); }
Public static void main(String args[])
{ Student s1 = new Student(111,"Karan");
Student s2 = new Student(s1);
s1.display();
s2.display();
}
}
OUTPUT:
111 Karan
111 Karan
Q8) What are the restrictions that are applied to the Java static methods?
A8)
Two main restrictions are applied to the static methods.
- The static method cannot use non-static data member or call the non-static method directly.
- This and super cannot be used in static context as they are non-static
- If you apply static keyword with any method, it is known as static method.
- A static method belongs to the class rather than the object of a class.
- A static method can be invoked without the need for creating an instance of a class.
- A static method can access static data member and can change the value of it.
//Java Program to get the cube of a given number using the static method
Class Calculate{
Static int cube(int x){
Return x*x*x;
} public static void main(String args[])
{ int result=Calculate.cube(5);
System.out.println(result); }
}
Output:
125
Q9) Why is the main method static? Can we override the static methods?
A9)
It is because the object is not required to call a static method. If it were a non-static method, JVM creates an object first then call main() method that will lead the problem of extra memory allocation.
No, we can’t override the static methods
Q10) What is the difference between static (class) method and instance method?
A10)
Static or class method | Instance method |
1)A method that is declared as static is known as the static method. | A method that is not declared as static is known as the instance method. |
2)We don't need to create the objects to call the static methods. | The object is required to call the instance methods. |
3)Non-static (instance) members cannot be accessed in the static context (static method, static block, and static nested class) directly. | Static and non-static variables both can be accessed in instance methods. |
4)For example: public static int cube(int n){ return n*n*n;} | For example: public void msg(){...}. |
Q11) Can we declare the static variables and methods in an abstract class?
A11)
Yes, we can declare static variables and methods in an abstract method. As we know that there is no requirement to make the object to access the static context, therefore, we can access the static context declared inside the abstract class by using the name of the abstract class.
Example
Abstract class Test
{ static int i = 102;
Static void TestMethod()
{
System.out.println("hi !! I am good !!");
}
}
Public class TestClass extends Test
{
Public static void main (String args[])
{
Test.TestMethod();
System.out.println("i = "+Test.i);
} }
OUTPUT:
Hi!! I am good!!
i=102
Q12) What is this keyword in java?
A12)
This keyword is a reference variable that refers to the current object. There are the various uses of this keyword in Java.
It can be used to refer to current class properties such as instance methods, variable, constructors, etc.
It can also be passed as an argument into the methods or constructors. It can also be returned from the method as the current class instance.
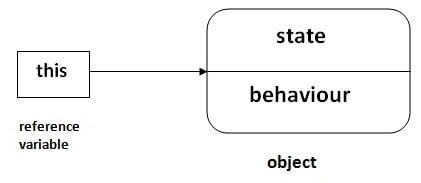
Q13) What are the main uses of this keyword?
A13)
There are the following uses of this keyword.
- This can be used to refer to the current class instance variable.
- This can be used to invoke current class method (implicitly)
- This() can be used to invoke the current class constructor.
- This can be passed as an argument in the method call.
Example:
Class S2
{ void m(S2 obj)
{ System.out.println("method is invoked");
}
Void p(){
m(this);
}//method
Public static void main(String args[]){
S2 s1 = new S2();
s1.p();
}
}
OUTPUT:
Method is invoked
- This can be passed as an argument in the constructor call.
Example
Class B{
A4 obj;
B(A4 obj) {
This.obj=obj;
}
Void display() {
System.out.println(obj.data);//using data member of A4 class
}
}
Class A4{
Int data=10;
A4(){
B b=new B(this);
b.display();
}
Public static void main(String args[]){
A4 a=new A4();
}
}
OUTPUT:
10
- This can be used to return the current class instance from the method.
We are using this keyword to distinguish local variable and instance variable.
Class Student{
Int rollno;
String name;
Float fee;
Student(int rollno,String name,float fee)
{ this.rollno=rollno;
This.name=name;
This.fee=fee;
}
Void display(){System.out.println(rollno+" "+name+" "+fee);}
}
Class TestThis2
{
Public static void main(String args[])
{
Student s1=new Student(111,"ankit",5000f);
Student s2=new Student(112,"sumit",6000f);
s1.display();
s2.display();
}//void main
} //class TestThis2
Output:
111 ankit 5000f
112 sumit 6000f
Q14) What is the Inheritance?
A14)
Inheritance is a mechanism by which one object acquires all the properties and behavior of another object of another class.
It is used for Code Reusability and Method Overriding. The idea behind inheritance in Java is that you can create new classes that are built upon existing classes.
When we inherit from an existing class, we can reuse methods and fields of the parent class. Moreover, we can add new methods and fields in our current class also.
Inheritance represents the IS-A relationship which is also known as a parent-child relationship.
There are five types of inheritance in Java.
- Single-level inheritance
- Multi-level inheritance
- Multiple Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Multiple inheritance is not supported in Java through class.
Q15) Why is Inheritance used in Java?
A15)
There are various advantages of using inheritance in Java that is given below:
- Inheritance provides code reusability. The derived class does not need to redefine the method of base class unless it needs to provide the specific implementation of the method.
- Runtime polymorphism cannot be achieved without using inheritance.
- We can simulate the inheritance of classes with the real-time objects which makes OOPs more realistic.
- Inheritance provides data hiding. The base class can hide some data from the derived class by making it private.
- Method overriding cannot be achieved without inheritance. By method overriding, we can give a specific implementation of some basic method contained by the base class.
Q16) Why is multiple inheritance not supported in java?
A16)
To reduce the complexity and simplify the language, multiple inheritance is not supported in java.
Consider a scenario where A, B, and C are three classes.
The C class inherits A and B classes. If A and B classes have the same method and you call it from child class object, there will be ambiguity to call the method of A or B class.
Since the compile-time errors are better than runtime errors, Java renders compile-time error if you inherit 2 classes.
So whether you have the same method or different, there will be a compile time error.
Class A
{
Void msg(){System.out.println("Hello");}
}
Class B{
Void msg(){System.out.println("Welcome");}
}
Class C extends A,B{//suppose if it were
Public Static void main(String args[]){
C obj=new C();
Obj.msg();//Now which msg() method would be invoked?
}
}
Compile Time Error
Q17) Why does Java not support pointers?
A17)
The pointer is a variable that refers to the memory address. They are not used in Java because they are unsafe(unsecured) and complex to understand
That is Some reasons for Java does not support Pointers
1. Memory access via pointer arithmetic: this is fundamentally unsafe. Java has a robust security model and disallows pointer arithmetic for the same reason. It would be impossible for the Virtual Machine to ensure that code containing pointer arithmetic is safe without expensive runtime checks.
2. Security: By not allowing pointers, Java effectively provides another level of abstraction to the developer. No pointer support makes Java more secure because they point to memory location or used for memory management that loses the security as we use them directly.
3. Passing argument by reference: Passing a reference which allows you to change the value of a variable in the caller's scope. Java doesn't have this, but it's a pretty rare use case and can easily be done in other ways. This is in general equivalent to changing a field in an object scope that both the caller and callee can see.
4. Manual memory management: you can use pointers to manually control and allocate blocks of memory. This is useful for some bigger applications like games, device drivers etc. but for general-purpose Object-Oriented programming it is simply not worth the effort. Java instead provides very good automatic Garbage Collection (GC) which takes care of memory management.
Q18) What is super in java?
A18)
The super keyword in Java is a reference variable that is used to refer to the immediate parent class object.
Whenever we create the instance of the subclass, an instance of the parent class is created implicitly which is referred by super reference variable.
The super() is called in the class constructor implicitly by the compiler if there is no super or this.
Class Animal
{ Animal()
{ System.out.println("animal is created");}
}
Class Dog extends Animal
{ Dog()
{
System.out.println("dog is created");
}
}
Class TestSuper4 {
Public static void main(String args[]) {
Dog d=new Dog();
}
}
OUTPUT:
Animal is created
Dog is created
Q19) What is composition?
A19)
Holding the reference of a class within some other class is known as composition.
When an object contains the other object, if the contained object cannot exist without the existence of container object, then it is called composition.
In other words, we can say that composition is the particular case of aggregation which represents a stronger relationship between two objects.
Example: A class contains students. A student cannot exist without a class. There exists composition between class and students.
// Java program to Illustrate Concept of Composition.Importing required classes
Import java.io.*;
Import java.util.*;
// Class 1 Helper class // Book class
Class Book {// Member variables of this class
Public String title;
Public String author; // Consstructor of this class
Book(String title, String author)
{ // This keyword refers top current instance
This.title = title;
This.author = author;
}
}
// Class 2 // Helper class // Library class contains list of books.
Class Library { // Reference to refer to list of books.
Private final List<Book> books;
// Constructor of this class
Library(List<Book> books)
{ // This keyword refers to current instance itself
This.books = books;
}
// Method of this class // Getting the list of books
Public List<Book> getListOfBooksInLibrary()
{
Return books;
}
}
// Class 3 // Main class
Class GFG {
// Main driver method
Public static void main(String[] args)
{
// Creating the objects of class 1 (Book class) inside main() method
Book b1 = new Book("EffectiveJ Java", "Joshua Bloch");
Book b2 = new Book("Thinking in Java", "Bruce Eckel");
Book b3 = new Book("Java: The Complete Reference” "Herbert Schildt");
// Creating the list which contains the // no. Of books.
List<Book> book = new ArrayList<Book>();
// Adding books to List object // using standard add() method
Book.add(b1);
Book.add(b2);
Book.add(b3);
// Creating an object of class 2
Library library = new Library(book);
// Calling method of class 2 and storing list of
// books in List Here List is declared of type // Books(user-defined)
List<Book> books
= library.getListOfBooksInLibrary();
// Iterating over for each loop
For (Book bk : books) {
// Print and display the title and author of
// books inside List object
System.out.println("Title : " + bk.title + " and ” + " Author : " + bk.author);
}//for
}//main
}//class GFG
Output:
Title: Effective Java and Author: Joshua Bloch
Title: Thinking in Java and Author: Bruce Eckel
Title: Java: The Complete Reference and Author: Herbert Schildt
Q20) What are the differences between this and super keyword?
A20)
There are the following differences between this and super keyword.
- The super keyword always points to the parent class contexts whereas this keyword always points to the current class context.
- The super keyword is primarily used for initializing the base class variables within the derived class constructor whereas this keyword primarily used to differentiate between local and instance variables when passed in the class constructor.
- The super and this must be the first statement inside constructor otherwise the compiler will throw an error.
Q21) Can we overload the main() method?
A21)
Yes, we can have any number of main methods in a Java program by using method overloading.
Main() Method Overloading :-The main() method is the starting point of any Java program. The JVM starts the execution of any Java program form the main() method. Without the main() method, JVM will not execute the program.
Syntax: public static void main(String args[])
It is a default signature that is predefined in the JVM. It is called by JVM to execute a program line by line and ends the execution after completion of the method.
MainMethodOverload1.java
Public class MainMethodOverload1
{ // Overloaded main() method 1//invoked when an int value is passed
Public static void main(Integer args)
{ System.out.println("Overloaded main() method invoked that parses an integer argument");
}
// Overloaded main() method 2 //invoked when a char is passed
Public static void main(char args)
{
System.out.println("Overloaded main() method invoked that parses a char argument");
}
//Original main() method
Public static void main(String[] args)
{ System.out.println("Original main() method invoked");
}
}
Output:
Original main() method invoked.
When we execute the above example, it always invokes the original main() method not the overloaded main() method. It is because the JVM executes the original main() method by default.
Q22) Difference between String and StringBuffer?
A22)
String | StringBuffer |
The String class is immutable. | The StringBuffer class is mutable. |
String is slow and consumes more memory when we concatenate too many strings because every time it creates new instance. | StringBuffer is fast and consumes less memory when we concatenate t strings. |
String class overrides the equals() method of Object class. So you can compare the contents of two strings by equals() method. | StringBuffer class doesn't override the equals() method of Object class. |
String class is slower while performing concatenation operation. | StringBuffer class is faster while performing concatenation operation. |
String class uses String constant pool. | StringBuffer uses Heap memory |
Performance Test of String and StringBuffer
ConcatTest.java
Public class ConcatTest{
Public static String concatWithString() {
String t = "Java";
For (int i=0; i<10000; i++){
t = t + "Tpoint";
}
Return t;
}
Public static String concatWithStringBuffer(){
StringBuffer sb = new StringBuffer("Java");
For (int i=0; i<10000; i++){
Sb.append("Tpoint");
}
Return sb.toString();
}
Public static void main(String[] args)
{
Long startTime = System.currentTimeMillis();
ConcatWithString();
System.out.println("Time taken by Concating with String: "+(System.urrentTimeMillis()-startTime)+"ms");
StartTime = System.currentTimeMillis();
ConcatWithStringBuffer();
System.out.println("Time taken by Concating with StringBuffer: "+(System.currentTimeMillis()-startTime)+"ms");
}
}
Output:
Time taken by Concating with String:587ms
Time taken by Concating with StringBuffer: 0ms
The above code, calculates the time required for concatenating a string using the String class and StringBuffer class.
Q23) How to create Immutable class?
A23)
There are many immutable classes like String, Boolean, Byte, Short, Integer, Long, Float, Double etc.
In short, all the wrapper classes and String class is immutable.
We can also create immutable class by creating final class that have final data members as the example given below:
Example to create Immutable class
In this example, we have created a final class named Employee. It have one final datamember, a parameterized constructor and getter method.
ImmutableDemo.java
Public final class Employee
{
Final String pancardNumber;
Public Employee(String pancardNumber)
{
This.pancardNumber=pancardNumber;
}
Public String getPancardNumber(){
Return pancardNumber;
}
}
Public class ImmutableDemo
{
Public static void main(String ar[])
{
Employee e = new Employee("ABC123");
String s1 = e.getPancardNumber();
System.out.println("Pancard Number: " + s1);
}
}
OUTPUT:
Pancard Number: ABC123
The above class is immutable because:
- The instance variable of the class is final i.e. we cannot change the value of it after creating an object.
- The class is final so we cannot create the subclass.
- There is no setter methods i.e. we have no option to change the value of the instance variable.
These points make this class as immutable.
Q24) How to optimize string creation?
A24)
By using string literals.
Ex: File: StringPerformance .java
Public class StringPerformance
{
Public static void main(String[] args)
{ long startTime = System.currentTimeMillis();
For (int i = 0; i < 100000; i++)
{
String str1 = "India";
String str2= "India";
} //for loop
Long endTime = System.currentTimeMillis();
System.out.println("Time taken to create literal String : " + (endTime - startTime) + " ms");
Long startTime1 = System.currentTimeMillis();
For (int i = 0; i < 100000; i++)
{
String str1 = new String("India");
String str2 = new String("India");
}
Long endTime1 = System.currentTimeMillis();
System.out.println("Time taken to create Object String: " + (endTim e1 - startTime1) + " ms");
}
}
Output:
Time taken to create literal String :9 ms
Time taken to create Object String :11 ms
Hence, String creation using literals take less time