Unit - 3
Data Abstraction and Multithreading
Q1) Difference between abstract class and interface.
A1)
Abstract class | Interface |
1) Abstract class can have abstract and non-abstract methods. | Interface can have only abstract methods. Since Java 8, it can have default and static methods also. |
2) Abstract class doesn't support multiple inheritance. | Interface supports multiple inheritance. |
3) Abstract class can have final, non-final, static and non-static variables. | Interface has only static and final variables. |
4) Abstract class can provide the implementation of interface. | Interface can't provide the implementation of abstract class. |
5) The abstract keyword is used to declare abstract class. | The interface keyword is used to declare interface. |
6) An abstract class can extend another Java class and implement multiple Java interfaces. | An interface can extend another Java interface only. |
7) An abstract class can be extended using keyword "extends". | An interface can be implemented using keyword "implements". |
8) A Java abstract class can have class members like private, protected, etc. | Members of a Java interface are public by default. |
9)Example: | Example: |
Q2) When to use abstract class and interface in java?
A2)
Java provides four types of access specifiers that we can use with classes and other entities. These are:
1) Default: Whenever a specific access level is not specified, then it is assumed to be ‘default’. The scope of the default level is within the package.
2) Public: This is the most common access level and whenever the public access specifier is used with an entity, that particular entity is accessible throughout from within or outside the class, within or outside the package, etc.
3) Protected: The protected access level has a scope that is within the package. A protected entity is also accessible outside the package through inherited class or child class.
4) Private: When an entity is private, then this entity cannot be accessed outside the class. A private entity can only be accessible from within the class.
Example of: The abstract class can also be used to provide some implementation of the interface,
Interface A{
Void a();
Void b();
Void c();
Void d();
}
Abstract class B implements A
{
Public void c(){ System.out.println("I am c"); }
}
Class M extends B{
Public void a() { System.out.println("I am a"); }
Public void b() { System.out.println("I am b"); }
Public void d() { System.out.println("I am d"); }
}//class M extends B
Class Test5{
Public static void main(String args[]){
A a=new M();
a.a();
a.b();
a.c();
a.d();
}}
OUTPUT:
I am a
I am b
I am c
I am d
Q3) How to create singleton class in java?
A3)
Singleton class means we can create only one object for the given class.
We can create a singleton class by making its constructor as private so that you can restrict the creation of the object.
Provide a static method to get an instance of the object, where in you can handle the object creation inside the class only.
In this example, we are creating an object by using a static block.
Public class MySingleton {
Private static MySingleton myObj;
Static{ myObj = new MySingleton();
} private MySingleton(){ }
Public static MySingleton getInstance()
{ return myObj; }
Public void testMe()
{ System.out.println(""Hey.... It is working!!!"");
}
Public static void main(String a[]){
MySingleton ms = getInstance();
Ms.testMe();
}
}
Q4) What is a thread in java?
A4)
A thread is a lightweight program that allows multiple processes to run concurrently.
Every java program has at least one thread called the main thread, the main thread is created by JVM.
The user can define their own threads by extending the Thread class (or) by implementing the Runnable interface. Threads are executed concurrently.
Public static void main(String[] args)
{ //main thread starts here }
Example of creating thread
// Java code for thread creation by extending // the Thread class
Class MultithreadingDemo extends Thread {
Public void run()
{
Try { // Displaying the thread that is running
System.out.println(
"Thread " + Thread.currentThread().getId()+ " is running");
}
Catch (Exception e) { // Throwing an exception
System.out.println("Exception is caught");
}
}
}
// Main Class
Public class Multithread {
Public static void main(String[] args)
{
Int n = 8; // Number of threads
For (int i = 0; i < n; i++) {
MultithreadingDemo object
= new MultithreadingDemo();
Object.start();
}
}
}
Output:
Thread 15 is running
Thread 14 is running
Thread 16 is running
Thread 12 is running
Thread 11 is running
Thread 13 is running
Thread 18 is running
Thread 17 is running
Q5) What do you understand by inter-thread communication?
A5)
The process of communication between synchronized threads is termed as inter-thread communication.
- Inter-thread communication is used to avoid thread polling in Java.
- The thread is paused running in its critical section, and another thread is allowed to enter (or lock) in the same critical section to be executed.
- It can be obtained by wait(), notify(), and notifyAll() methods.
Q6) What does join() method?
A6)
The join() method waits for a thread to die.
In other words, it causes the currently running threads to stop executing until the thread it joins with completes its task.
Join method is overloaded in Thread class in the following ways.
- Public void join()throws InterruptedException
- Public void join(long milliseconds)throws InterruptedException
Example of join() method
Class TestJoinMethod1 extends Thread
{ public void run()
{ for(int i=1;i<=5;i++)
{ try
{
Thread.sleep(500);
}//try
Catch(Exception e)
{ System.out.println(e); }//Catch
System.out.println(i);
}//for loop
} //run
Public static void main(String args[])
{
TestJoinMethod1 t1=new TestJoinMethod1();
TestJoinMethod1 t2=new TestJoinMethod1();
TestJoinMethod1 t3=new TestJoinMethod1();
t1.start();
try {
t1.join();
}
Catch(Exception e){System.out.println(e);}
t2.start();
t3.start();
}//main method
} //class
OUTPUT:
1
2
3
4
5
1
1
2
2
3
3
4
4
5
5
5
Q7) What is the difference between wait() and sleep() method?
A7)
Wait() | Sleep() |
1) The wait() method is defined in Object class. | The sleep() method is defined in Thread class. |
2) The wait() method releases the lock. | The sleep() method doesn't release the lock. |
Q8) Differentiate between process and thread?
A8)
There are the following differences between the process and thread.
A Program in the execution is called the process whereas; A thread is a subset of the process
Processes are independent whereas threads are the subset of process.
Process has different address space in memory, while threads contain a shared address space.
Context switching is faster between the threads as compared to processes.
Inter-process communication is slower and expensive than inter-thread communication.
Any change in Parent process doesn't affect the child process whereas changes in parent thread can affect the child thread.
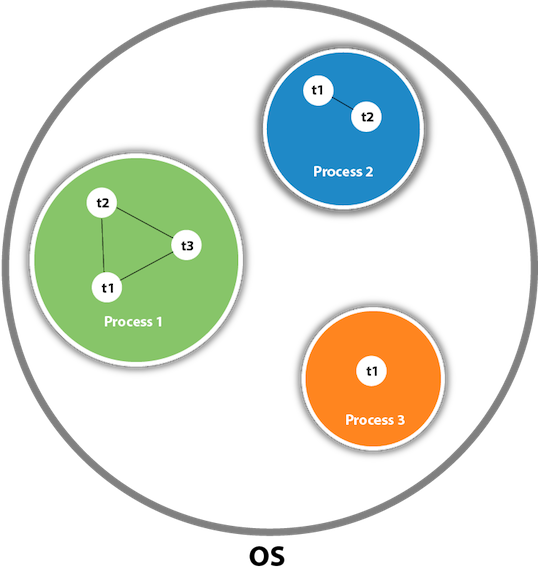
Q9) What are the states in the lifecycle of a Thread?
A9)
A thread can have one of the following states during its lifetime:
- New: In this state, a Thread class object is created using a new operator, but the thread is not alive. Thread doesn't start until we call the start() method.
- Runnable: In this state, the thread is ready to run after calling the start() method. However, the thread is not yet selected by the thread scheduler.
- Running: In this state, the thread scheduler picks the thread from the ready state, and the thread is running.
- Waiting/Blocked: In this state, a thread is not running but still alive, or it is waiting for the other thread to finish.
- Dead/Terminated: A thread is in terminated or dead state when the run() method exits.
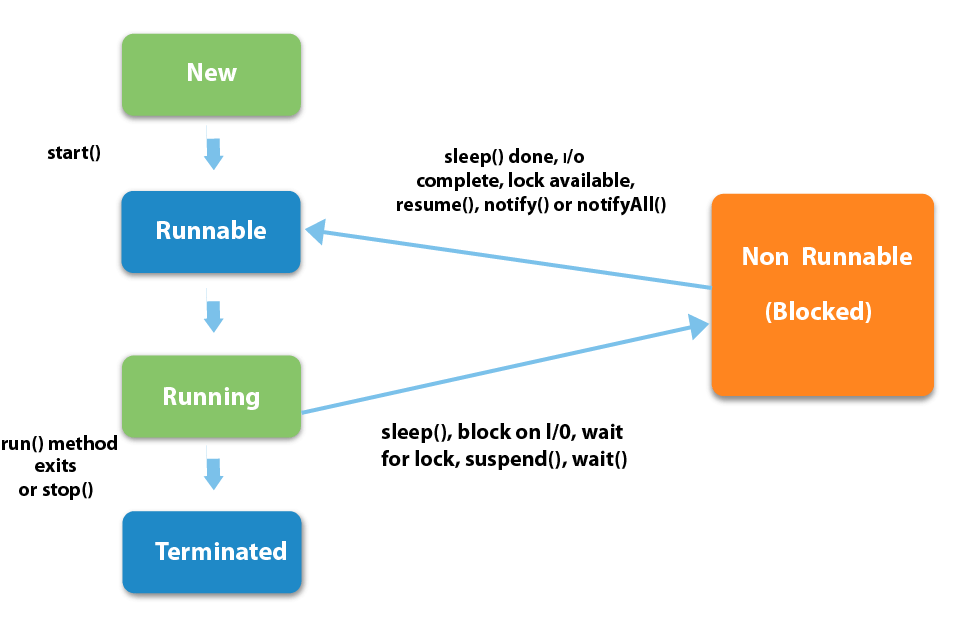
Q10) What about the daemon threads?
A10)
The daemon threads are the low priority threads that provide the background support and services to the user threads.
Daemon thread gets automatically terminated by the JVM if the program remains with the daemon thread only, and all other user threads are ended/died.
There are two methods for daemon thread available in the Thread class:
- Public void setDaemon(boolean status): It used to mark the thread daemon thread or a user thread.
- Public boolean isDaemon(): It checks the thread is daemon or not.
Methods for Java Daemon thread by Thread class
The java.lang.Thread class provides two methods for java daemon thread.
No. | Method | Description |
1) | Public void setDaemon(boolean status) | Is used to mark the current thread as daemon thread or user thread. |
2) | Public boolean isDaemon() | Is used to check that current is daemon. |
Simple example of Daemon thread in java
File: MyThread.java
Public class TestDaemonThread1 extends Thread{
Public void run()
{
If(Thread.currentThread().isDaemon())
{//checking for daemon thread
System.out.println("daemon thread work");
}
Else{ System.out.println("user thread work");
}
}//method
Public static void main(String[] args)
{ //creating thread
TestDaemonThread1 t1=new TestDaemonThread1();
TestDaemonThread1 t2=new TestDaemonThread1();
TestDaemonThread1 t3=new TestDaemonThread1();
t1.setDaemon(true);//now t1 is daemon thread
t1.start();//starting threads
t2.start();
t3.start();
}//main method
}
OUTPUT:
Daemon thread work
User thread work
User thread work
Q11) How many types of exception used in java? Explain?
A11)
In Java, exception is an event that occurs during the execution of a program and disrupts the normal flow of the program's instructions.
Bugs or errors that we don't want and restrict our program's normal execution of code are referred to as exceptions.
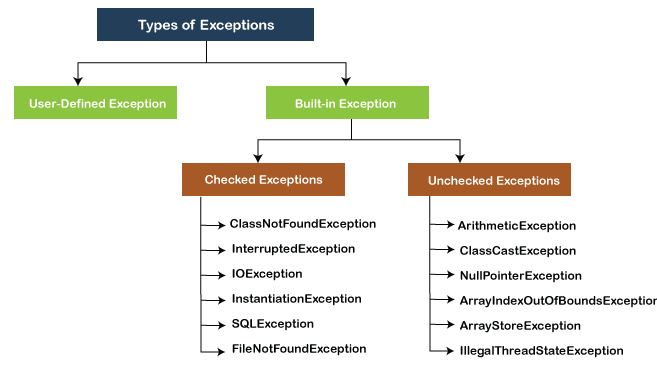
- Built-in Exception
Exceptions that are already available in Java libraries are referred to as built-in exception.
These exceptions are able to define the error situation so that we can understand the reason of getting this error.
It can be categorized into two broad categories, i.e., checked exceptions and unchecked exception
- Arithmetic Exception: It is thrown when an exceptional condition has occurred in an arithmetic operation.
- Array Index Out of Bounds Exception: It is thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
- Class Not Found Exception: This Exception is raised when we try to access a class whose definition is not found
- FileNotFoundException: This Exception is raised when a file is not accessible or does not open.
- IOException: It is thrown when an input-output operation failed or interrupted
- InterruptedException: It is thrown when a thread is waiting, sleeping, or doing some processing, and it is interrupted.
- NoSuchFieldException: It is thrown when a class does not contain the field (or variable) specified
- NoSuchMethodException: It is thrown when accessing a method which is not found.
- NullPointerException: This exception is raised when referring to the members of a null object. Null represents nothing
- NumberFormatException: This exception is raised when a method could not convert a string into a numeric format.
- RuntimeException: This represents any exception which occurs during runtime.
- StringIndexOutOfBoundsException: It is thrown by String class methods to indicate that an index is either negative or greater than the size of the string
A) Checked Exception
Checked exceptions are called compile-time exceptions because these exceptions are checked at compile-time by the compiler.
The compiler ensures whether the programmer handles the exception or not.
The programmer should have to handle the exception; otherwise, the system has shown a compilation error.
B) Unchecked Exceptions
The unchecked exceptions are just opposite to the checked exceptions.
The compiler will not check these exceptions at compile time.
In simple words, if a program throws an unchecked exception, and even if we didn't handle or declare it, the program would not give a compilation error.
Usually, it occurs when the user provides bad data during the interaction with the program.
- User-defined Exception
In Java, we already have some built-in exception classes like ArrayIndexOutOfBoundsException, NullPointerException, and ArithmeticException.
These exceptions are restricted to trigger on some predefined conditions.
In Java, we can write our own exception class by extends the Exception class.
We can throw our own exception on a particular condition using the throw keyword. For creating a user-defined exception, we should have basic knowledge of the try-catch block and throw keyword.
Q12) Differentiate between the Thread class and Runnable interface for creating a Thread?
A12)
The Thread can be created by using two ways.
- By extending the Thread class
- By implementing the Runnable interface
However, the primary differences between both the ways are given below:
- By extending the Thread class, we cannot extend any other class, as Java does not allow multiple inheritances while implementing the Runnable interface; we can also extend other base class(if required).
- By extending the Thread class, each of thread creates the unique object and associates with it while implementing the Runnable interface; multiple threads share the same object
- Thread class provides various inbuilt methods such as getPriority(), isAlive and many more while the Runnable interface provides a single method, i.e., run().
Q13) How to detect a deadlock condition? How can it be avoided?
A13)
We can detect the deadlock condition by running the code on cmd and collecting the Thread Dump, and if any deadlock is present in the code, then a message will appear on cmd.
Ways to avoid the deadlock condition in Java:
- Avoid Nested lock: Nested lock is the common reason for deadlock as deadlock occurs when we provide locks to various threads so we should give one lock to only one thread at some particular time.
- Avoid unnecessary locks: we must avoid the locks which are not required.
- Using thread join: Thread join helps to wait for a thread until another thread doesn't finish its execution so we can avoid deadlock by maximum use of join method.
Example of Deadlock in Java
TestDeadlockExample1.java
Public class TestDeadlockExample1
{
Public static void main(String[] args)
{
Final String resource1 = "ratan jaiswal";
Final String resource2 = "vimal jaiswal";
// t1 tries to lock resource1 then resource2
Thread t1 = new Thread()
{
Public void run()
{
Synchronized (resource1)
{ System.out.println("Thread 1: locked resource 1");
Try {
Thread.sleep(100);
}
Catch (Exception e) {}
Synchronized (resource2)
{ System.out.println("Thread 1: locked resource 2");
}
}
}
}; // t2 tries to lock resource2 then resource1
Thread t2 = new Thread()
{ public void run()
{ synchronized (resource2)
{ System.out.println("Thread 2: locked resource 2");
Try { Thread.sleep(100);}
Catch (Exception e) {}
Synchronized (resource1)
{ System.out.println("Thread 2: locked resource 1");
} //resource 1
} //resource 2
}
};
t1.start();
t2.start();
} //run method
} //thread
Output:
Thread 1: locked resource 1
Thread 2: locked resource 2
Q14) What is race-condition?
A14)
A Race condition is a problem which occurs in the multithreaded programming when various threads execute simultaneously accessing a shared resource at the same time. The proper use of synchronization can avoid the Race condition.
Q15) What is the difference between Java Callable interface and Runnable interface?
A15)
The Callable interface and Runnable interface both are used by the classes which wanted to execute with multiple threads. However, there are two main differences between the both:
- A Callable <V> interface can return a result, whereas the Runnable interface cannot return any result.
- A Callable <V> interface can throw a checked exception, whereas the Runnable interface cannot throw checked exception.
- A Callable <V> interface cannot be used before the Java 5 whereas the Runnable interface can be used.
Q16) What is the difference between Synchronous programming and Asynchronous programming regarding a thread?
A16)
Synchronous programming: In Synchronous programming model, a thread is assigned to complete a task and hence thread started working on it, and it is only available for other tasks once it will end the assigned task.
Asynchronous Programming: In Asynchronous programming, one job can be completed by multiple threads and hence it provides maximum usability of the various threads.
Q17) What is lock interface in Concurrency API in Java?
A17)
The java.util.concurrent.locks.Lock interface is used as the synchronization mechanism. It works similar to the synchronized block. There are a few differences between the lock and synchronized block that are given below.
- Lock interface provides the guarantee of sequence in which the waiting thread will be given the access, whereas the synchronized block doesn't guarantee it.
- Lock interface provides the option of timeout if the lock is not granted whereas the synchronized block doesn't provide that.
- The methods of Lock interface, i.e., Lock() and Unlock() can be called in different methods whereas single synchronized block must be fully contained in a single method.
Q18) What are the main components of concurrency API?
A18)
Concurrency API can be developed using the class and interfaces of java.util.Concurrent package. There are the following classes and interfaces in java.util.Concurrent package.
- Executor
- FarkJoinPool
- ExecutorService
- ScheduledExecutorService
- Future
- TimeUnit(Enum)
- CountDownLatch
- CyclicBarrier
- Semaphore
- ThreadFactory
- BlockingQueue
- DelayQueue
- Locks
- Phaser
Q19) What is the Executor interface in Concurrency API in Java?
A19)
The Executor Interface provided by the package java.util.concurrent is the simple interface used to execute the new task. The execute() method of Executor interface is used to execute some given command. The syntax of the execute() method is given below.
Void execute(Runnable command)
Example:
Import java.util.concurrent.Executor;
Import java.util.concurrent.Executors;
Import java.util.concurrent.ThreadPoolExecutor;
Import java.util.concurrent.TimeUnit;
Public class TestThread {
Public static void main(final String[] arguments) throws InterruptedException {
Executor e = Executors.newCachedThreadPool();
e.execute(new Thread());
ThreadPoolExecutor pool = (ThreadPoolExecutor)e;
Pool.shutdown();
}//main method
Static class Thread implements Runnable
{ public void run()
{
Try {
Long duration = (long) (Math.random() * 5);
System.out.println("Running Thread!");
TimeUnit.SECONDS.sleep(duration);
System.out.println("Thread Completed");
}
Catch (InterruptedException ex)
{ ex.printStackTrace();
}
} //run method
}//static class thread
}//public class thread
OUTPUT:
Running Thread
Thread Complected.
Q20) How to perform multiple tasks by multiple threads (multitasking in multithreading)?
A20)
Program of performing two tasks by two threads
Class Simple1 extends Thread{
Public void run()
{ System.out.println("task one");
}
} //simple1
Class Simple2 extends Thread
{
Public void run()
{ System.out.println("task two"); }//run
} //simple 2
Class TestMultitasking3
{
Public static void main(String args[])
{
Simple1 t1=new Simple1();
Simple2 t2=new Simple2();
t1.start();
t2.start();
}//main method
} //multitasking3
OUTPUT:
Task one
Task two