Unit - 4
IO Streams (java.io package) and Applet
Q1) What is the Collection framework in Java?
A1)
Collection Framework is a combination of classes and interface, which is used to store and manipulate the data in the form of objects.
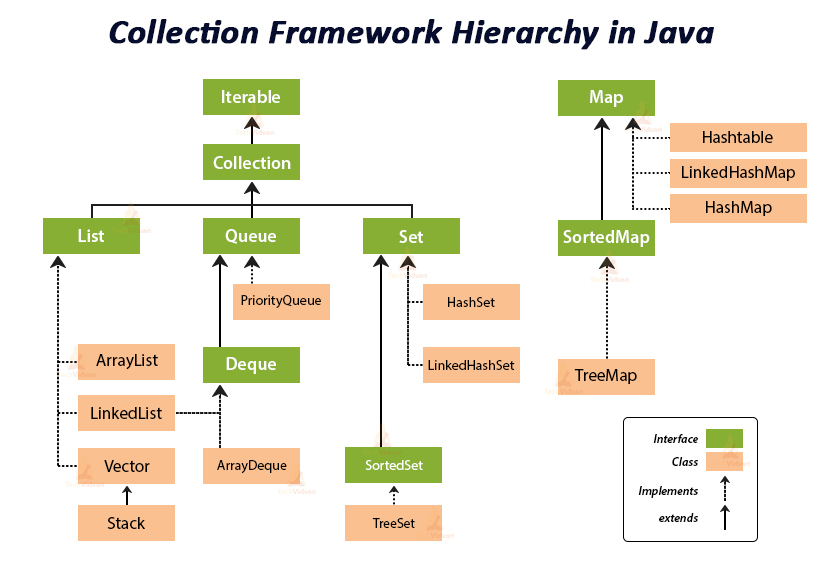
It provides various classes such as Array List, Vector, Stack, and HashSet, etc. and interfaces such as List, Queue, Set, etc. for this purpose.
Following are some advantages of using Collection Frameworks:
1. Consistent API: Java Collection Framework provides a consistent API that has an essential arrangement of interfaces like Collection, List, Set, List, Queue or Map. Each class, for example, ArrayList, LinkedList, Vector and so on, which implements these interfaces have some normal arrangement of strategies.
2. Reduces Programming Exertion: Using a Collection Framework, a software developer can focus on the best use of the program rather than focusing on an outline of Collection.
3. Increases Program Quality and Speed: Collection Framework helps to increase the execution speed and quality by giving the best use of valuable information structures and calculations.
Q2) What are the main differences between array and collection?
A2)
Array and Collection are similar regarding storing the references of objects and manipulating the data, but they differ in many ways. The main differences between the array and Collection are defined below:
- Arrays are always of fixed size, i.e., a user cannot increase or decrease the length of the array according to their requirement or at runtime, but In Collection, size can be changed dynamically as per need.
- Arrays can only store homogeneous or similar type objects, but in Collection, heterogeneous objects can be stored.
- Arrays cannot provide the ready-made methods for user requirements as sorting, searching, etc. but Collection includes readymade methods to use.
Q3) Explain various interfaces used in Collection framework?
A3)
Collection framework implements various interfaces, Collection interface and Map interface (java.util.Map) are the mainly used interfaces of Java Collection Framework. List of interfaces of Collection Framework is given below:
1. Collection interface: Collection (java.util.Collection) is the primary interface, and every collection must implement this interface.
Syntax: public interface Collection<E>extends Iterable
Where <E> represents that this interface is of Generic type
2. List interface: List interface extends the Collection interface, and it is an ordered collection of objects. It contains duplicate elements. It also allows random access of elements.
Syntax: public interface List<E> extends Collection<E>
3. Set interface: Set (java.util.Set) interface is a collection which cannot contain duplicate elements. It can only include inherited methods of Collection interface
Syntax: public interface Set<E> extends Collection<E>
4. Queue interface: Queue (java.util.Queue) interface defines queue data structure, which stores the elements in the form FIFO (first in first out).
Syntax: public interface Queue<E> extends Collection<E>
5. Dequeue interface: it is a double-ended-queue. It allows the insertion and removal of elements from both ends. It implants the properties of both Stack and queue so it can perform LIFO (Last in first out) stack and FIFO (first in first out) queue, operations.
Syntax: public interface Dequeue<E> extends Queue<E>
6. Map interface: A Map (java.util.Map) represents a key, value pair storage of elements. Map interface does not implement the Collection interface. It can only contain a unique key but can have duplicate elements. There are two interfaces which implement Map in java that are Map interface and Sorted Map.
Q4) What is the difference between Array List and Vector?
A4)
No. | Array List | Vector |
1) | Array List is not synchronized. | Vector is synchronized. |
2) | Array List is not a legacy class. | Vector is a legacy class. |
3) | Array List increases its size by 50% of the array size. | Vector increases its size by doubling the array size. |
4) | Array List is not thread-safe as it is not synchronized. | Vector list is thread-safe as it’s every method is synchronized. |
Q5) How to synchronize List, Set and Map elements?
A5)
Yes, Collections class provides methods to make List, Set or Map elements as synchronized:
- Public static List synchronizedList(List l){}
- Public static Set synchronizedSet(Set s){}
- Public static SortedSet synchronizedSortedSet(SortedSet s){}
- Public static Map synchronizedMap(Map m){}
- Public static SortedMap synchronizedSortedMap(SortedMap m){}
Q6) What is the advantage of the generic collection?
A6)
There are three main advantages of using the generic collection.
1) Type-safety: We can hold only a single type of objects in generics. It doesn’t allow to store other objects.
Without Generics, we can store any type of objects.
List list = new ArrayList();
List.add(10);
List.add("10");
With Generics, it is required to specify the type of object we need to store.
List<Integer> list = new ArrayList<Integer>();
List.add(10);
List.add("10");// compile-time error
2) Type casting is not required: There is no need to typecast the object.
Before Generics, we need to type cast.
List list = new ArrayList();
List.add("hello");
String s = (String) list.get(0);//typecasting
After Generics, we don't need to typecast the object.
List<String> list = new ArrayList<String>();
List.add("hello");
String s = list.get(0);
3) Compile-Time Checking: It is checked at compile time so problem will not occur at runtime. The good programming strategy says it is far better to handle the problem at compile time than runtime.
List<String> list = new ArrayList<String>();
List.add("hello");
List.add(32);//Compile Time Error
Syntax to use generic collection
ClassOrInterface<Type>
Example to use Generics in java
ArrayList<String>
Example of Java Generics using Map
We are going to use map elements using generics. Here, we need to pass key and value.
Import java.util.*;
Class TestGenerics2{
Public static void main(String args[]){
Map<Integer,String> map=new HashMap<Integer,String>();
Map.put(1,"vijay");
Map.put(4,"umesh");
Map.put(2,"ankit");
//Now use Map.Entry for Set and Iterator
Set<Map.Entry<Integer,String>> set=map.entrySet();
Iterator<Map.Entry<Integer,String>> itr=set.iterator();
While(itr.hasNext()){
Map.Entry e=itr.next();//no need to typecast
System.out.println(e.getKey()+" "+e.getValue());
}
}}
Output:- 1 vijay
2 Ankit
4 umesh
Q7) What is the difference between Array List and Linked List?
A7)
No | Array List | Linked List |
1) | Array List uses a dynamic array. | Linked List uses a doubly linked list. |
2) | Array List is not efficient for manipulation because too much is required. | Linked List is efficient for manipulation. |
3) | Array List is better to store and fetch data. | Linked List is better to manipulate data. |
4) | Array List provides random access. | Linked List does not provide random access. |
5) | Array List takes less memory overhead as it stores only object | Linked List takes more memory overhead, as it stores the object as well as the address of that object. |
Q8) What is an Applet? Should Applets have Constructors?
A8)
Applets are small programs transferred through Internet, automatically installed and run as part of web-browser.
- Applets implements functionality of a client.
- Applet is a dynamic and interactive program that runs inside a Web page displayed by a Java-capable browser.
- We don’t have the concept of Constructors in Applets.
- Applets can be invoked either through browser or through Applet viewer utility provided by JDK.
Q9) What is the Order of Method Invocation in an Applet? Or What are the Applets Life Cycle Methods? Explain them?
A9)
- Public void init(): Initialization method called once by browser.
- Public void start(): Method called after init() and contains code to start processing. If the user leaves the page and returns without killing the current browser session, the start () method is called without being preceded by init ().
- Public void stop(): Stops all processing started by start (). Done if user moves off page.
- Public void destroy(): Called if current browser session is being terminated. Frees all resources used by applet.
Simple example of Applet by applet viewer tool:
To execute the applet by applet viewer tool, create an applet that contains applet tag in comment and compile it.
After that run it by: appletviewer First.java. Now Html file is not required but it is for testing purpose only.
//First.java
Import java.applet.Applet;
Import java.awt.Graphics;
Public class First extends Applet
{
Public void paint(Graphics g)
{
g.drawString("welcome to applet",150,150);
}
}
/* <applet code="First.class" width="300" height="300"> </applet> */
To execute the applet by appletviewer tool, write in command prompt:
c:\>javac First.java
c:\>appletviewer First.java
Q10) How do Applets differ from Applications?
A10)
Following are the main differences:
a) Application: Stand Alone, doesn’t need web-browser.
b) Applet: Needs no explicit installation on local machine. Can be transferred through Internet on to the local machine and may run as part of web-browser.
c) Application: Execution starts with main() method. Doesn’t work if main is not there.
d) Applet: Execution starts with init() method.
e) Application: May or may not be a GUI.
f) Applet: Must run within a GUI (Using AWT). This is essential feature of applets.
Q11) Define Canvas?
A11)
It is a simple drawing surface which is used for painting images or to perform other graphical operations.
Difference between the Font and Font Metrics classes
The Font Metrics class is used to define implementation specific properties such as ascent and descent, of a Font object.
Syntax: public class Canvas extends Component implements Accessible
We are creating a Canvas in the Frame and painting a red colored oval inside it.
CanvasExample.java
// importing awt class
Import java.awt.*;
// class to construct a frame and containing main method
Public class CanvasExample
{ // class constructor
Public CanvasExample()
{ // creating a frame
Frame f = new Frame("Canvas Example");
// adding canvas to frame
f.add(new MyCanvas());
// setting layout, size and visibility of frame
f.setLayout(null);
f.setSize(400, 400);
f.setVisible(true);
}//public canvas
// main method
Public static void main(String args[])
{ new CanvasExample();
}
}// class which inherits the Canvas class to create Canvas
Class MyCanvas extends Canvas
{ // class constructor
Public MyCanvas() {
SetBackground (Color.GRAY);
SetSize(300, 200);
}
// paint() method to draw inside the canvas
Public void paint(Graphics g)
{ // adding specifications
g.setColor(Color.red);
g.fillOval(75, 75, 150, 75);
}
}
Output:
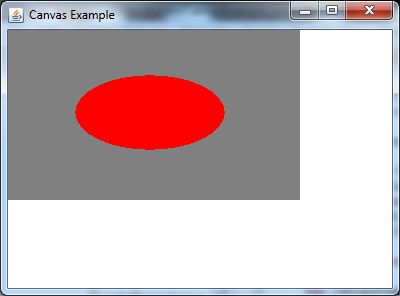
Q12) What is the difference between Exclusive and Non Exclusive?
A12)
Exclusive: Only one among a group of items can be selected at a time. If an item from the group is selected, the checkbox currently checked is deselected and the new selection is highlighted. The exclusive Checkboxes are also called as Radio buttons.
Non Exclusive: Checkboxes are not grouped together and each one can be selected of the other.
Q13) What is a container in a GUI?
A13)
A Container contains and arranges other components through the use of layout managers, which use specific layout policies to determine where components should go as a function of the size of the container.
How to Make a GUI in Java with Example:
Step 1) Copy code into an editor
In first step Copy the following code into an editor.
Import javax.swing.*;
Class gui{
Public static void main(String args[]){
JFrame frame = new JFrame("My First GUI");
Frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Frame.setSize(300,300);
JButton button = new JButton("Press");
Frame.getContentPane().add(button); // Adds Button to content pane of frame
Frame.setVisible(true);
}
}
Step 2) Run the code
Next step, Save, Compile, and Run the code
Step 3) Copy following code into an editor
Now let’s Add a Button to our frame. Copy following code into an editor from given Java UI Example
Import javax.swing.*;
Class gui{
Public static void main(String args[]){
JFrame frame = new JFrame("My First GUI");
Frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Frame.setSize(300,300);
JButton button1 = new JButton("Press");
Frame.getContentPane().add(button1);
Frame.setVisible(true);
}
}
Step 4) Execute the code
Next, Execute the code. You will get a big button.
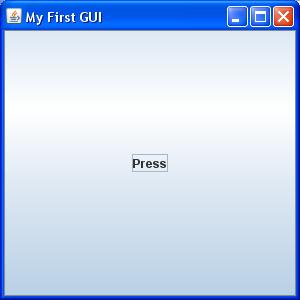
Q14) What is the difference between the paint() and repaint() method?
A14)
- Paint(): This method holds instructions to paint this component. In Java Swing, we can change the paintComponent() method instead of paint() method as paint calls paintBorder(), paintComponent() and paintChildren() methods. We cannot call this method directly instead we can call repaint().
- Repaint(): This method cannot be overridden. It controls the update() -> paint() cycle. We can call this method to get a component to repaint itself. If we have done anything to change the look of the component but not the size then we can call this method.
Example:
Import java.awt.*;
Import java.awt.event.*;
Import javax.swing.*;
Importjava.util.*;
Public class PaintRepaintTest extends JPanel implementsMouseListener { public PaintRepaintTest()
{ v = new Vector();
SetBackground(Color.white);
AddMouseListener(this);}
Private Vector v;
Public PaintRepaintTest()
{ v = new Vector();
SetBackground(Color.white);
AddMouseListener(this);}
Public void paint(Graphics g) { // paint() method
Super.paint(g);
g.setColor(Color.black);
Enumeration enumeration = v.elements();
While(enumeration.hasMoreElements()) {
Point p = (Point)(enumeration.nextElement());
g.drawRect(p.x-20, p.y-20, 40, 40);
}
}
Public void mousePressed(MouseEvent me) { v.add(me.getPoint());
Repaint(); // call repaint() method
}
Public void mouseClicked(MouseEvent me) {}
Public void mouseEntered(MouseEvent me) {}
Public void mouseExited(MouseEvent me) {}
Public void mouseReleased(MouseEvent me) {}
Public static void main(String args[]) {
JFrame frame = new JFrame();
Frame.getContentPane().add(new PaintRepaintTest());
Frame.setTitle("PaintRepaint Test");
Frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
Frame.setLocationRelativeTo(null);
Frame.setSize(375, 250);
Frame.setVisible(true);
}}
In the above program, if we click on the screen able to draw squares. In the mousePressed() method, we can call the repaint() method.
Output
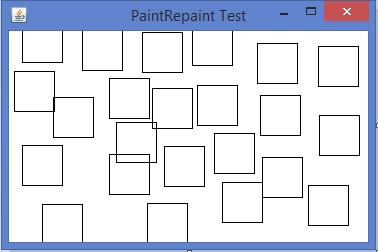
Q15) What are the benefits of Swing over AWT?
A15)
- Swing components are light weight.
- We can have a pluggable look and feel feature which shows us how they appear in other platforms.
- We can add images to Swing components. We have toolbars and tooltips in Swing.
We can add images to Swing components. We have toolbars and tooltips in Swing.
Java Swing Example
In the following example, we have created a User form by using the swing component classes provided by the javax.swing package. Consider the example.
Import javax.swing.*;
Public class SwingApp {
SwingApp(){
JFrame f = new JFrame();
JLabel firstName = new JLabel("First Name");
FirstName.setBounds(20, 50, 80, 20);
JLabel lastName = new JLabel("Last Name");
LastName.setBounds(20, 80, 80, 20);
JLabel dob = new JLabel("Date of Birth");
Dob.setBounds(20, 110, 80, 20);
JTextField firstNameTF = new JTextField();
FirstNameTF.setBounds(120, 50, 100, 20);
JTextField lastNameTF = new JTextField();
LastNameTF.setBounds(120, 80, 100, 20);
JTextField dobTF = new JTextField();
DobTF.setBounds(120, 110, 100, 20);
JButton sbmt = new JButton("Submit");
Sbmt.setBounds(20, 160, 100, 30);
JButton reset = new JButton("Reset");
Reset.setBounds(120,160,100,30);
f.add(firstName);
f.add(lastName);
f.add(dob);
f.add(firstNameTF);
f.add(lastNameTF);
f.add(dobTF);
f.add(sbmt);
f.add(reset);
f.setSize(300,300);
f.setLayout(null);
f.setVisible(true);
}
Public static void main(String[] args) {
// TODO Auto-generated method stub
SwingApp s = new SwingApp();
}
}
Output:
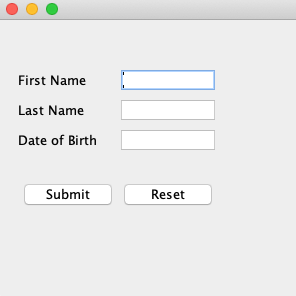
Java awt Example
To understand the differences between Awt and Swing, we have created the same example in awt as well. Consider the following example.
Import java.awt.*;
Public class AwtApp extends Frame
{
AwtApp()
{ Label firstName = new Label("First Name");
FirstName.setBounds(20, 50, 80, 20);
Label lastName = new Label("Last Name");
LastName.setBounds(20, 80, 80, 20);
Label dob = new Label("Date of Birth");
Dob.setBounds(20, 110, 80, 20);
TextField firstNameTF = new TextField();
FirstNameTF.setBounds(120, 50, 100, 20);
TextField lastNameTF = new TextField();
LastNameTF.setBounds(120, 80, 100, 20);
TextField dobTF = new TextField();
DobTF.setBounds(120, 110, 100, 20);
Button sbmt = new Button("Submit");
Sbmt.setBounds(20, 160, 100, 30);
Button reset = new Button("Reset");
Reset.setBounds(120,160,100,30);
Add(firstName);
Add(lastName);
Add(dob);
Add(firstNameTF);
Add(lastNameTF);
Add(dobTF);
Add(sbmt);
Add(reset);
SetSize(300,300);
SetLayout(null);
SetVisible(true);
}
Public static void main(String[] args)
{ // TODO Auto-generated method stub
AwtApp awt = new AwtApp();
}
Output:
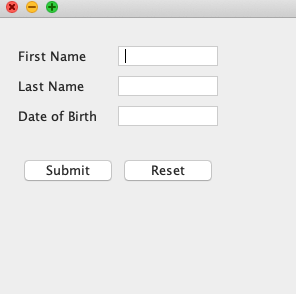
Q16) Explain how to read information from the Applet Parameters.
A16)
The getParameter() method can be used within the init() method to access the parameter data.
It takes the parameter name as an argument.
Example:
public void init()
{
String val = getParameter("foreground-color");
}
Q17) How would you communicate between Applets and Servlets?
A17)
We can use the java.net.URLConnection and java.net.URL classes to open a standard HTTP connection and "tunnel" to a Web server.
The server then passes this information to the servlet. Basically, the applet pretends to be a Web browser, and the servlet doesn't know the difference.
As far as the servlet is concerned, the applet is just another HTTP client.
Applets can communicate with servlets using GET or POST methods. The parameters can be passed between the applet and the servlet as name value pairs.
Objects can also be passed between applet and servlet using object serialization.
Objects are serialized to and from the input stream and output stream of the connection respectively.
Q18) What are the attributes of Applet Tags?
A18)
- Height: Defines height of applet
- Width: Defines width of applet
- Align: Defines the text alignment around the applet
- Alt: An alternate text to be displayed if the browser support applets but cannot run this applet
- Archive: A URL to the applet when it is stored in a Java Archive or ZIP file
- Code: A URL that points to the class of the applet
- Codebase: Indicates the base URL of the applet if the code attribute is relative
- Hspace: Defines the horizontal spacing around the applet
- Vspace: Defines the vertical spacing around the applet
- Name: Defines a name for an applet
- Object: Defines the resource name that contains a serialized representation of the applet
- Title: Display information in tool tip
Q19) Explain how to play sound in an applet.
A19)
Import java.applet.*;
import java.awt.*;
import java.awt.event.*;
Public class Audio1Applet extends Applet implements ActionListener
{ Button play,stop;
AudioClip audioClip;
public void init()
{
play = new Button(" Play ");
add(play);
play.addActionListener(this);
stop = new Button(" Stop ");
add(stop);
stop.addActionListener(this);
audioClip = getAudioClip(getCodeBase(), "abc.wav");
}
Public void actionPerformed(ActionEvent ae)
{
Button source = (Button)ae.getSource();
if (source.getLabel() == " Play ")
{ audioClip.play(); }
else if(source.getLabel() == " Stop ")
{ audioClip.stop(); }
}
}
<APPLET CODE="Audio1Applet"> </APPLET><H5>
Q20) How to display the image in an applet? Explain Them?
A20)
Applet is mostly used in games and animation. For this purpose image is required to be displayed. The java.awt.Graphics class provide a method drawImage() to display the image.
Syntax of drawImage ()Methods:
Public abstract boolean drawImage(Image img, int x, int y, ImageObserver observer):
Is used draw the specified image.
How to get the object of Image:
The java.applet.Applet class provides getImage() method that returns the object of Image.
Syntax: public Image getImage(URL u, String image){}
Example of displaying image in applet
Import java.awt.*;
Import java.applet.*;
Public class DisplayImage extends Applet {
Image picture;
Public void init() {
Picture = getImage(getDocumentBase(),"sonoo.jpg");
}
Public void paint(Graphics g) {
g.drawImage(picture, 30,30, this);
}
}
In the above example, drawImage() method of Graphics class is used to display the image. The 4th argument of drawImage() method of is ImageObserver object. The Component class implements ImageObserver interface. So current class object would also be treated as ImageObserver because Applet class indirectly extends the Component class.
Myapplet.html
<html>
<body>
<applet code="DisplayImage.class" width="300" height="300">
</applet> </body> </html>