Unit 3
Operators and Expression in ‘C’
- Explain arithmetic operator with example
Arithmetic Operator: These are the operators which are useful in performing mathematical calculations. Following are the list of arithmetic operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
+ | Used to add two operands | Result of A+B is 15 | |
2. | - | Used to subtract two operands | Result of A-B is 5 |
3. | * | Used to multiply two operands | Result of A*B is 50 |
4. | / | Used to divide two operands | Result of A/B is 2 |
5. | % | Find reminder of the division of two operand | Result of A%B is 0 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
int a,b,c;
clrscr();
printf("Enter two numbers");
scanf("%d %d",&a,&b);
printf("\n Result of addition of %d and %d is %d",a,b,a+b);
printf("\n Result of subtraction of %d and %d is %d",a,b,a-b);
printf("\n Result of multiplication %d and %d is %d",a,b,a*b);
printf("\n Result of division of %d and %d is %d",a,b,a/b);
printf("\n Result of modulus of %d and %d is %d",a,b,a%b);
getch();
}
Output:
Enter two numbers
5
3
Result of addition of 5 and 3 is 8
Result of subtraction of 5 and 3 is 2
Result of multiplication of 5 and 3 is 15
Result of division of 5 and 3 is 1
Result of modulus of 5 and 3 is 2
2. Explain relational operator with example
Relational Operators: Relational operator used to compare two operands. Relational operator produce result in terms of binary values. It returns 1 when the result is true and 0 when result is false. Following are the list of relational operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | < | This is less than operator which is used to check whether the value of left operand is less than the value of right operand or not | Result of A<B is false
|
2. | > | This is greater than operator which is used to check whether the value of left operand is greater than the value of right operand or not | Result of A>B is true |
3. | <= | This is less than or equal to operator | Result of A<=B is false |
4. | >= | This is greater than or equal to operator | Result of A>=B is true |
5. | == | This is equal to operator which is used to check value of both operands are equal or not | Result of A==B is false |
6. | != | This is not equal to operator which is used to check value of both operands are equal or not | Result of A!=B is true |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
int a,b,c;
clrscr();
printf("Enter two numbers");
scanf("%d %d",&a,&b);
printf("\n Result of less than operator of %d and %d is %d",a,b,a<b);
printf("\n Result of greater than operator of %d and %d is %d",a,b,a>b);
printf("\n Result of leass than or equal to operator %d and %d is %d",a,b,a<=b);
Printf("\n Result of greater than or equal to operator of %d and %d is %d", a,b,a>=b);
printf("\n Result of double equal to operator of %d and %d is %d",a,b,a==b);
printf("\n Result of not equal to operator of %d and %d is %d",a,b,a!=b);
getch();
}
Output:
Enter two numbers
5
3
Result of less than operator of 5 and 3 is 0
Result of greater than operator of 5 and 3 is 1
Result of less than or equal to operator of 5 and 3 is 0
Result of greater than or equal to operator of 5 and 3 is 1
Result of double equal to operator of 5 and 3 is 0
Result of not equal to operator of 5 and 3 is 1
3. Explain logical operator with example
Logical Operators: Logical operators are used to compare logical values of two operands. Following are the list of logical operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5.
Sr. No. | Operator | Description | Example |
1. | && | This is logical AND, it returns true when both the values are non zero | Result of (A&&B) is true |
2. | || | This is logical OR, it returns true when any of two value is non zero | Result of (A||B) is true |
3. | ! | This is logical NOT operator, it is used to reverse the logical state of operand | Result of !(A&&B) is false |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
clrscr();
printf("Enter two numbers");
scanf("%d %d",&a,&b);
printf("\n Result of logical and operator of %d and %d is %d",a,b,a&&b);
printf("\n Result of logical or operator of %d and %d is %d",a,b,a||b);
printf("\n Result of logical not operator of %d and and %d is %d",a,b,!(a&&b));
getch();
}
Output:
Enter two numbers
5
3
Result of logical and operator of 5 and 3 is 1
Result of logical or operator of 5 and 3 is 1
Result of logical not operator of 5 and 3 is 0
4. Explain assignment operator with example
Assignment Operators: Assignment operator is used to assign the value to variable. To understand the operation assume variable A contains value 10 and variable contains value 5.
Example: A=B;
In the above example value of variable B is assign to variable A. Result of this expression is value of A is 5 and value of B is 5.
- Increments and Decrement Operators: These operator are used to increase or decrease the value of variable by one. Following are the list of logical operator in C language. To understand the operation assume variable A contains value 10.
Sr. No. | Operator | Description | Example |
1. | ++ | This is incremental operator which is used to increase the value of variable by 1 | Result of (A++) is value of A is 11 |
2. | -- | This is decremental operator which is used to decrease the value of variable by 1 | Result of (A--) is value of A is 9 |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a=5,b=5,c=5,d=5;
clrscr();
printf("Original value of a, b, c, d is %d \t %d \t %d \t %d",a,b,c,d);
a++;
printf("\n New value of a is %d",a);
++b;
printf("\n New value of b is %d",b);
printf("\n New value of c is %d",c++);
printf("\n New value of d is %d",++d);
getch();
}
Output:
Original value of a, b, c, d is 5555
New value of a is 6
New value of b is 6
New value of c is 5
New value of d is 6
5. Explain bitwise operator with example
Bitwise Operators: These operators are used to work on the each bits of data. These operators can work on the binary data. If the data is non binary then computer first of all convert it into binary form and then perform the operation. Following are the list of logical operator in C language. To understand the operation assume variable A contains value 10 and variable contains value 5. So the binary values of
A = 0000 1010 and
B = 0000 0101
Sr. No. | Operator | Description | Example |
1. | & | This is bitwise AND | Result of (A&B) is 0000 0000 |
2. | | | This is bitwise OR | Result of (A|B) is 0000 1111 |
3. | ^ | This is bitwise Exclusive | Result of (A^B) is 0000 1111 |
4. | << | This is used to shift bit on left side | Result of (A<<B) is |
5. | >> | This is used to shift bit on right side | Result of (A>>B) is |
Example:
#include<stdio.h>
#include<conio.h>
Void main()
{
Int a,b,c;
clrscr();
printf("Enter two numbers");
scanf("%d %d",&a,&b);
printf("\n Result of bitwise and operator of %d and %d is %d",a,b,a&b);
printf("\n Result of bitwise or operator of %d and %d is %d",a,b,a|b);
printf("\n Result of bitwise exclusive operator of %d and %d is %d",a,b,a^b);
printf("\n Result of bitwise left shift operator of %d and %d is %d",a,b,a<<b);
printf("\n Result of bitwise right shift operator of %d and %d is %d",a,b,a>>b);
getch();
}
Output:
Enter two numbers
5
3
Result of bitwise and operator of 5 and 3 is 1
Result of bitwise or operator of 5 and 3 is 7
Result of bitwise exclusive operator of 5 and 3 is 6
Result of bitwise left shift operator of 5 and 3 is 40
Result of bitwise right shift operator of 5 and 3 is 0
6. Explain conditional operator in detail
Conditional Operator
The conditional operator is also known as a ternary operator. The conditional statements are the decision-making statements which depends upon the output of the expression. It is represented by two symbols, i.e., '?' and ':'.
As conditional operator works on three operands, so it is also known as the ternary operator.
The behavior of the conditional operator is similar to the 'if-else' statement as 'if-else' statement is also a decision-making statement.
Syntax of a conditional operator
- Expression1? expression2: expression3;
The pictorial representation of the above syntax is shown below:
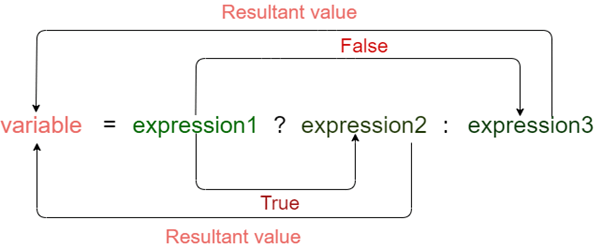
Meaning of the above syntax.
- In the above syntax, the expression1 is a Boolean condition that can be either true or false value.
- If the expression1 results into a true value, then the expression2 will execute.
- The expression2 is said to be true only when it returns a non-zero value.
- If the expression1 returns false value then the expression3 will execute.
- The expression3 is said to be false only when it returns zero value.
Let's understand the ternary or conditional operator through an example.
- #include <stdio.h>
- Int main()
- {
- Int age; // variable declaration
- Printf("Enter your age");
- Scanf("%d",&age); // taking user input for age variable
- (age>=18)? (printf("eligible for voting")) : (printf("not eligible for voting")); // conditional operator
- Return 0;
- }
In the above code, we are taking input as the 'age' of the user. After taking input, we have applied the condition by using a conditional operator. In this condition, we are checking the age of the user. If the age of the user is greater than or equal to 18, then the statement1 will execute, i.e., (printf("eligible for voting")) otherwise, statement2 will execute, i.e., (printf("not eligible for voting")).
Let's observe the output of the above program.
If we provide the age of user below 18, then the output would be:
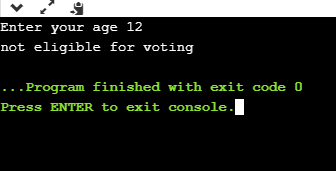
If we provide the age of user above 18, then the output would be:
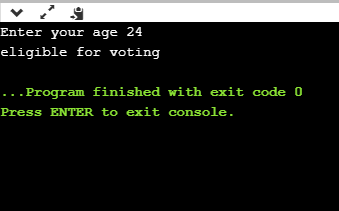
As we can observe from the above two outputs that if the condition is true, then the statement1 is executed; otherwise, statement2 will be executed.
Till now, we have observed that how conditional operator checks the condition and based on condition, it executes the statements. Now, we will see how a conditional operator is used to assign the value to a variable.
Let's understand this scenario through an example.
- #include <stdio.h>
- Int main()
- {
- Int a=5,b; // variable declaration
- b=((a==5)?(3):(2)); // conditional operator
- Printf("The value of 'b' variable is : %d",b);
- Return 0;
- }
In the above code, we have declared two variables, i.e., 'a' and 'b', and assign 5 value to the 'a' variable. After the declaration, we are assigning value to the 'b' variable by using the conditional operator. If the value of 'a' is equal to 5 then 'b' is assigned with a 3 value otherwise 2.
Output
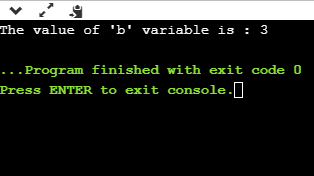
The above output shows that the value of 'b' variable is 3 because the value of 'a' variable is equal to 5.
As we know that the behavior of conditional operator and 'if-else' is similar but they have some differences. Let's look at their differences.
- A conditional operator is a single programming statement, while the 'if-else' statement is a programming block in which statements come under the parenthesis.
- A conditional operator can also be used for assigning a value to the variable, whereas the 'if-else' statement cannot be used for the assignment purpose.
- It is not useful for executing the statements when the statements are multiple, whereas the 'if-else' statement proves more suitable when executing multiple statements.
- The nested ternary operator is more complex and cannot be easily debugged, while the nested 'if-else' statement is easy to read and maintain.
7. What is special operator in C explain with example?
Special Operators in C:
Below are some of the special operators that the C programming language offers.
Operators | Description |
& | This is used to get the address of the variable. Example : &a will give address of a. |
* | This is used as pointer to a variable. Example : * a where, * is pointer to the variable a. |
Sizeof () | This gives the size of the variable. Example : size of (char) will give us 1. |
Example program for & and * operators in C:
In this program, “&” symbol is used to get the address of the variable and “*” symbol is used to get the value of the variable that the pointer is pointing to. Please refer C – pointer topic to know more about pointers.
1 2 3 4 5 6 7 8 9 10 11 | #include <stdio.h> Int main() { Int *ptr, q; q = 50; /* address of q is assigned to ptr */ Ptr = &q; /* display q's value using ptr variable */ Printf("%d", *ptr); Return 0; } |
Output:
50 |
Example program for sizeof() operator in C:
Sizeof() operator is used to find the memory space allocated for each C data types.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <stdio.h> #include <limits.h>
Int main() { Int a; Char b; Float c; Double d; Printf("Storage size for int data type:%d \n",sizeof(a)); Printf("Storage size for char data type:%d \n",sizeof(b)); Printf("Storage size for float data type:%d \n",sizeof(c)); Printf("Storage size for double data type:%d\n",sizeof(d)); Return 0; } |
Output:
Storage size for int data type:4 Storage size for char data type:1 |
Types of Operators | Description |
Arithmetic operators | These are used to perform mathematical calculations like addition, subtraction, multiplication, division and modulus |
Assignment operators | These are used to assign the values for the variables in C programs. |
Relational operators | These operators are used to compare the value of two variables. |
Logical operators | These operators are used to perform logical operations on the given two variables. |
Bit wise operators | These operators are used to perform bit operations on given two variables. |
Conditional (ternary) operators | Conditional operators return one value if condition is true and returns another value is condition is false. |
Increment/decrement operators | These operators are used to either increase or decrease the value of the variable by one. |
Special operators | &, *, sizeof( ) and ternary operators. |
8. Explain Unary, Binary and Ternary Operators
Unary operator
Only one operand is required to perform calculation.
++(increment operator)
-- (decrement operator)
Example
a = 5;
++a;
--a;
Binary Operator
Two operands are required to perform calculation.
+, -, *, % etc.
Example
a = 5;
b = 15;
a + b;
a - b;
Ternary Operator
Three operands are required to perform calculation.
Example
(expression 1)? (expression 2): (expression 3);
a > b?printf("a"):printf("b");
It is also called as a conditional operator in c.
9. What is Typecasting in C?
Typecasting is converting one data type into another one. It is also called as data conversion or type conversion. It is one of the important concepts introduced in 'C' programming.
'C' programming provides two types of type casting operations:
Implicit type casting
Implicit type casting means conversion of data types without losing its original meaning. This type of typecasting is essential when you want to change data types without changing the significance of the values stored inside the variable.
Implicit type conversion happens automatically when a value is copied to its compatible data type. During conversion, strict rules for type conversion are applied. If the operands are of two different data types, then an operand having lower data type is automatically converted into a higher data type. This type of type conversion can be seen in the following example.
#include<stdio.h>
Int main(){
Short a=10; //initializing variable of short data type
Int b; //declaring int variable
b=a; //implicit type casting
Printf("%d\n",a);
Printf("%d\n",b);
}
Output
10. Explain Precedence and Associativity with example
Operator precedence determines the grouping of terms in an expression and decides how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has a higher precedence than the addition operator.
For example, x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has a higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Category | Operator | Associativity |
Postfix | () [] -> . ++ - - | Left to right |
Unary | + - ! ~ ++ - - (type)* & sizeof | Right to left |
Multiplicative | * / % | Left to right |
Additive | + - | Left to right |
Shift | << >> | Left to right |
Relational | < <= > >= | Left to right |
Equality | == != | Left to right |
Bitwise AND | & | Left to right |
Bitwise XOR | ^ | Left to right |
Bitwise OR | | | Left to right |
Logical AND | && | Left to right |
Logical OR | || | Left to right |
Conditional | ?: | Right to left |
Assignment | = += -= *= /= %=>>= <<= &= ^= |= | Right to left |
Comma | , | Left to right |
Example Code
#include <stdio.h>
Main() {
Int a = 20;
Int b = 10;
Int c = 15;
Int d = 5;
Int e;
e = (a + b) * c / d; // ( 30 * 15 ) / 5
Printf("Value of (a + b) * c / d is : %d\n", e );
e = ((a + b) * c) / d; // (30 * 15 ) / 5
Printf("Value of ((a + b) * c) / d is : %d\n" , e );
e = (a + b) * (c / d); // (30) * (15/5)
Printf("Value of (a + b) * (c / d) is : %d\n", e );
e = a + (b * c) / d; // 20 + (150/5)
Printf("Value of a + (b * c) / d is : %d\n" , e );
Return 0;
}
Output
Value of (a + b) * c / d is : 90
Value of ((a + b) * c) / d is : 90
Value of (a + b) * (c / d) is : 90
Value of a + (b * c) / d is : 50